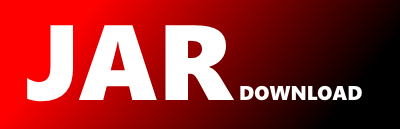
com.paypal.api.payments.PaymentCardToken Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.liferay.commerce.payment.method.paypal
Show all versions of com.liferay.commerce.payment.method.paypal
Liferay Commerce Payment Method PayPal
The newest version!
// Generated by delombok at Thu Nov 16 13:48:05 CST 2017
package com.paypal.api.payments;
import com.paypal.base.rest.PayPalModel;
public class PaymentCardToken extends PayPalModel {
/**
* ID of a previously saved Payment Card resource.
*/
private String paymentCardId;
/**
* The unique identifier of the payer used when saving this payment card.
*/
private String externalCustomerId;
/**
* Last 4 digits of the card number from the saved card.
*/
private String last4;
/**
* Type of the Card.
*/
private String type;
/**
* card expiry month from the saved card with value 1 - 12
*/
private int expireMonth;
/**
* 4 digit card expiry year from the saved card
*/
private int expireYear;
/**
* Default Constructor
*/
public PaymentCardToken() {
}
/**
* Parameterized Constructor
*/
public PaymentCardToken(String paymentCardId, String externalCustomerId, String type) {
this.paymentCardId = paymentCardId;
this.externalCustomerId = externalCustomerId;
this.type = type;
}
/**
* ID of a previously saved Payment Card resource.
*/
@java.lang.SuppressWarnings("all")
public String getPaymentCardId() {
return this.paymentCardId;
}
/**
* The unique identifier of the payer used when saving this payment card.
*/
@java.lang.SuppressWarnings("all")
public String getExternalCustomerId() {
return this.externalCustomerId;
}
/**
* Last 4 digits of the card number from the saved card.
*/
@java.lang.SuppressWarnings("all")
public String getLast4() {
return this.last4;
}
/**
* Type of the Card.
*/
@java.lang.SuppressWarnings("all")
public String getType() {
return this.type;
}
/**
* card expiry month from the saved card with value 1 - 12
*/
@java.lang.SuppressWarnings("all")
public int getExpireMonth() {
return this.expireMonth;
}
/**
* 4 digit card expiry year from the saved card
*/
@java.lang.SuppressWarnings("all")
public int getExpireYear() {
return this.expireYear;
}
/**
* ID of a previously saved Payment Card resource.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PaymentCardToken setPaymentCardId(final String paymentCardId) {
this.paymentCardId = paymentCardId;
return this;
}
/**
* The unique identifier of the payer used when saving this payment card.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PaymentCardToken setExternalCustomerId(final String externalCustomerId) {
this.externalCustomerId = externalCustomerId;
return this;
}
/**
* Last 4 digits of the card number from the saved card.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PaymentCardToken setLast4(final String last4) {
this.last4 = last4;
return this;
}
/**
* Type of the Card.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PaymentCardToken setType(final String type) {
this.type = type;
return this;
}
/**
* card expiry month from the saved card with value 1 - 12
* @return this
*/
@java.lang.SuppressWarnings("all")
public PaymentCardToken setExpireMonth(final int expireMonth) {
this.expireMonth = expireMonth;
return this;
}
/**
* 4 digit card expiry year from the saved card
* @return this
*/
@java.lang.SuppressWarnings("all")
public PaymentCardToken setExpireYear(final int expireYear) {
this.expireYear = expireYear;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof PaymentCardToken)) return false;
final PaymentCardToken other = (PaymentCardToken) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (!super.equals(o)) return false;
final java.lang.Object this$paymentCardId = this.getPaymentCardId();
final java.lang.Object other$paymentCardId = other.getPaymentCardId();
if (this$paymentCardId == null ? other$paymentCardId != null : !this$paymentCardId.equals(other$paymentCardId)) return false;
final java.lang.Object this$externalCustomerId = this.getExternalCustomerId();
final java.lang.Object other$externalCustomerId = other.getExternalCustomerId();
if (this$externalCustomerId == null ? other$externalCustomerId != null : !this$externalCustomerId.equals(other$externalCustomerId)) return false;
final java.lang.Object this$last4 = this.getLast4();
final java.lang.Object other$last4 = other.getLast4();
if (this$last4 == null ? other$last4 != null : !this$last4.equals(other$last4)) return false;
final java.lang.Object this$type = this.getType();
final java.lang.Object other$type = other.getType();
if (this$type == null ? other$type != null : !this$type.equals(other$type)) return false;
if (this.getExpireMonth() != other.getExpireMonth()) return false;
if (this.getExpireYear() != other.getExpireYear()) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof PaymentCardToken;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + super.hashCode();
final java.lang.Object $paymentCardId = this.getPaymentCardId();
result = result * PRIME + ($paymentCardId == null ? 43 : $paymentCardId.hashCode());
final java.lang.Object $externalCustomerId = this.getExternalCustomerId();
result = result * PRIME + ($externalCustomerId == null ? 43 : $externalCustomerId.hashCode());
final java.lang.Object $last4 = this.getLast4();
result = result * PRIME + ($last4 == null ? 43 : $last4.hashCode());
final java.lang.Object $type = this.getType();
result = result * PRIME + ($type == null ? 43 : $type.hashCode());
result = result * PRIME + this.getExpireMonth();
result = result * PRIME + this.getExpireYear();
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy