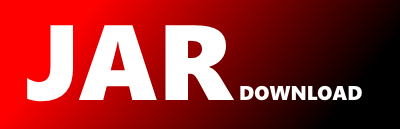
com.paypal.api.payments.PaymentDetail Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.liferay.commerce.payment.method.paypal
Show all versions of com.liferay.commerce.payment.method.paypal
Liferay Commerce Payment Method PayPal
The newest version!
// Generated by delombok at Thu Nov 16 13:48:05 CST 2017
package com.paypal.api.payments;
import com.paypal.base.rest.PayPalModel;
public class PaymentDetail extends PayPalModel {
/**
* The PayPal payment detail. Indicates whether payment was made in an invoicing flow through PayPal or externally. In the case of the mark-as-paid API, the supported payment type is `EXTERNAL`. For backward compatibility, the `PAYPAL` payment type is still supported.
*/
private String type;
/**
* The PayPal payment transaction ID. Required with the `PAYPAL` payment type.
*/
private String transactionId;
/**
* Type of the transaction.
*/
private String transactionType;
/**
* The date when the invoice was paid. The date format is *yyyy*-*MM*-*dd* *z* as defined in [Internet Date/Time Format](http://tools.ietf.org/html/rfc3339#section-5.6).
*/
private String date;
/**
* The payment mode or method. Required with the `EXTERNAL` payment type.
*/
private String method;
/**
* Optional. A note associated with the payment.
*/
private String note;
/**
* The amount to record as payment against invoice. If you omit this parameter, the total invoice amount is recorded as payment.
*/
private Currency amount;
/**
* Default Constructor
*/
public PaymentDetail() {
}
/**
* Parameterized Constructor
*/
public PaymentDetail(String method) {
this.method = method;
}
/**
* The PayPal payment detail. Indicates whether payment was made in an invoicing flow through PayPal or externally. In the case of the mark-as-paid API, the supported payment type is `EXTERNAL`. For backward compatibility, the `PAYPAL` payment type is still supported.
*/
@java.lang.SuppressWarnings("all")
public String getType() {
return this.type;
}
/**
* The PayPal payment transaction ID. Required with the `PAYPAL` payment type.
*/
@java.lang.SuppressWarnings("all")
public String getTransactionId() {
return this.transactionId;
}
/**
* Type of the transaction.
*/
@java.lang.SuppressWarnings("all")
public String getTransactionType() {
return this.transactionType;
}
/**
* The date when the invoice was paid. The date format is *yyyy*-*MM*-*dd* *z* as defined in [Internet Date/Time Format](http://tools.ietf.org/html/rfc3339#section-5.6).
*/
@java.lang.SuppressWarnings("all")
public String getDate() {
return this.date;
}
/**
* The payment mode or method. Required with the `EXTERNAL` payment type.
*/
@java.lang.SuppressWarnings("all")
public String getMethod() {
return this.method;
}
/**
* Optional. A note associated with the payment.
*/
@java.lang.SuppressWarnings("all")
public String getNote() {
return this.note;
}
/**
* The amount to record as payment against invoice. If you omit this parameter, the total invoice amount is recorded as payment.
*/
@java.lang.SuppressWarnings("all")
public Currency getAmount() {
return this.amount;
}
/**
* The PayPal payment detail. Indicates whether payment was made in an invoicing flow through PayPal or externally. In the case of the mark-as-paid API, the supported payment type is `EXTERNAL`. For backward compatibility, the `PAYPAL` payment type is still supported.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PaymentDetail setType(final String type) {
this.type = type;
return this;
}
/**
* The PayPal payment transaction ID. Required with the `PAYPAL` payment type.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PaymentDetail setTransactionId(final String transactionId) {
this.transactionId = transactionId;
return this;
}
/**
* Type of the transaction.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PaymentDetail setTransactionType(final String transactionType) {
this.transactionType = transactionType;
return this;
}
/**
* The date when the invoice was paid. The date format is *yyyy*-*MM*-*dd* *z* as defined in [Internet Date/Time Format](http://tools.ietf.org/html/rfc3339#section-5.6).
* @return this
*/
@java.lang.SuppressWarnings("all")
public PaymentDetail setDate(final String date) {
this.date = date;
return this;
}
/**
* The payment mode or method. Required with the `EXTERNAL` payment type.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PaymentDetail setMethod(final String method) {
this.method = method;
return this;
}
/**
* Optional. A note associated with the payment.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PaymentDetail setNote(final String note) {
this.note = note;
return this;
}
/**
* The amount to record as payment against invoice. If you omit this parameter, the total invoice amount is recorded as payment.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PaymentDetail setAmount(final Currency amount) {
this.amount = amount;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof PaymentDetail)) return false;
final PaymentDetail other = (PaymentDetail) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (!super.equals(o)) return false;
final java.lang.Object this$type = this.getType();
final java.lang.Object other$type = other.getType();
if (this$type == null ? other$type != null : !this$type.equals(other$type)) return false;
final java.lang.Object this$transactionId = this.getTransactionId();
final java.lang.Object other$transactionId = other.getTransactionId();
if (this$transactionId == null ? other$transactionId != null : !this$transactionId.equals(other$transactionId)) return false;
final java.lang.Object this$transactionType = this.getTransactionType();
final java.lang.Object other$transactionType = other.getTransactionType();
if (this$transactionType == null ? other$transactionType != null : !this$transactionType.equals(other$transactionType)) return false;
final java.lang.Object this$date = this.getDate();
final java.lang.Object other$date = other.getDate();
if (this$date == null ? other$date != null : !this$date.equals(other$date)) return false;
final java.lang.Object this$method = this.getMethod();
final java.lang.Object other$method = other.getMethod();
if (this$method == null ? other$method != null : !this$method.equals(other$method)) return false;
final java.lang.Object this$note = this.getNote();
final java.lang.Object other$note = other.getNote();
if (this$note == null ? other$note != null : !this$note.equals(other$note)) return false;
final java.lang.Object this$amount = this.getAmount();
final java.lang.Object other$amount = other.getAmount();
if (this$amount == null ? other$amount != null : !this$amount.equals(other$amount)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof PaymentDetail;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + super.hashCode();
final java.lang.Object $type = this.getType();
result = result * PRIME + ($type == null ? 43 : $type.hashCode());
final java.lang.Object $transactionId = this.getTransactionId();
result = result * PRIME + ($transactionId == null ? 43 : $transactionId.hashCode());
final java.lang.Object $transactionType = this.getTransactionType();
result = result * PRIME + ($transactionType == null ? 43 : $transactionType.hashCode());
final java.lang.Object $date = this.getDate();
result = result * PRIME + ($date == null ? 43 : $date.hashCode());
final java.lang.Object $method = this.getMethod();
result = result * PRIME + ($method == null ? 43 : $method.hashCode());
final java.lang.Object $note = this.getNote();
result = result * PRIME + ($note == null ? 43 : $note.hashCode());
final java.lang.Object $amount = this.getAmount();
result = result * PRIME + ($amount == null ? 43 : $amount.hashCode());
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy