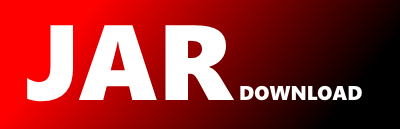
com.paypal.api.payments.PaymentExecution Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.liferay.commerce.payment.method.paypal
Show all versions of com.liferay.commerce.payment.method.paypal
Liferay Commerce Payment Method PayPal
The newest version!
// Generated by delombok at Thu Nov 16 13:48:05 CST 2017
package com.paypal.api.payments;
import com.paypal.base.rest.PayPalModel;
import java.util.List;
public class PaymentExecution extends PayPalModel {
/**
* The ID of the Payer, passed in the `return_url` by PayPal.
*/
private String payerId;
/**
* Carrier account id for a carrier billing payment. For a carrier billing payment, payer_id is not applicable.
*/
private String carrierAccountId;
/**
* Transactional details including the amount and item details.
*/
private List transactions;
/**
* Default Constructor
*/
public PaymentExecution() {
}
/**
* The ID of the Payer, passed in the `return_url` by PayPal.
*/
@java.lang.SuppressWarnings("all")
public String getPayerId() {
return this.payerId;
}
/**
* Carrier account id for a carrier billing payment. For a carrier billing payment, payer_id is not applicable.
*/
@java.lang.SuppressWarnings("all")
public String getCarrierAccountId() {
return this.carrierAccountId;
}
/**
* Transactional details including the amount and item details.
*/
@java.lang.SuppressWarnings("all")
public List getTransactions() {
return this.transactions;
}
/**
* The ID of the Payer, passed in the `return_url` by PayPal.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PaymentExecution setPayerId(final String payerId) {
this.payerId = payerId;
return this;
}
/**
* Carrier account id for a carrier billing payment. For a carrier billing payment, payer_id is not applicable.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PaymentExecution setCarrierAccountId(final String carrierAccountId) {
this.carrierAccountId = carrierAccountId;
return this;
}
/**
* Transactional details including the amount and item details.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PaymentExecution setTransactions(final List transactions) {
this.transactions = transactions;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof PaymentExecution)) return false;
final PaymentExecution other = (PaymentExecution) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (!super.equals(o)) return false;
final java.lang.Object this$payerId = this.getPayerId();
final java.lang.Object other$payerId = other.getPayerId();
if (this$payerId == null ? other$payerId != null : !this$payerId.equals(other$payerId)) return false;
final java.lang.Object this$carrierAccountId = this.getCarrierAccountId();
final java.lang.Object other$carrierAccountId = other.getCarrierAccountId();
if (this$carrierAccountId == null ? other$carrierAccountId != null : !this$carrierAccountId.equals(other$carrierAccountId)) return false;
final java.lang.Object this$transactions = this.getTransactions();
final java.lang.Object other$transactions = other.getTransactions();
if (this$transactions == null ? other$transactions != null : !this$transactions.equals(other$transactions)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof PaymentExecution;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + super.hashCode();
final java.lang.Object $payerId = this.getPayerId();
result = result * PRIME + ($payerId == null ? 43 : $payerId.hashCode());
final java.lang.Object $carrierAccountId = this.getCarrierAccountId();
result = result * PRIME + ($carrierAccountId == null ? 43 : $carrierAccountId.hashCode());
final java.lang.Object $transactions = this.getTransactions();
result = result * PRIME + ($transactions == null ? 43 : $transactions.hashCode());
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy