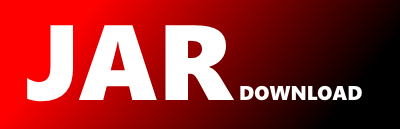
com.paypal.api.payments.PayoutItemDetails Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.liferay.commerce.payment.method.paypal
Show all versions of com.liferay.commerce.payment.method.paypal
Liferay Commerce Payment Method PayPal
The newest version!
// Generated by delombok at Thu Nov 16 13:48:05 CST 2017
package com.paypal.api.payments;
import com.paypal.base.rest.PayPalModel;
import java.util.List;
public class PayoutItemDetails extends PayPalModel {
/**
* The ID for the payout item. Viewable when you show details for a batch payout.
*/
private String payoutItemId;
/**
* The PayPal-generated ID for the transaction.
*/
private String transactionId;
/**
* The transaction status.
*/
private String transactionStatus;
/**
* The amount of money, in U.S. dollars, for fees.
*/
private Currency payoutItemFee;
/**
* The PayPal-generated ID for the batch payout.
*/
private String payoutBatchId;
/**
* A sender-specified ID number. Tracks the batch payout in an accounting system.
*/
private String senderBatchId;
/**
* The sender-provided information for the payout item.
*/
private PayoutItem payoutItem;
/**
* The date and time when this item was last processed.
*/
private String timeProcessed;
/**
*/
private Error errors;
/**
* The HATEOAS links related to the call.
*/
private List links;
/**
* Default Constructor
*/
public PayoutItemDetails() {
}
/**
* Parameterized Constructor
*/
public PayoutItemDetails(String payoutItemId, String payoutBatchId, PayoutItem payoutItem, String timeProcessed) {
this.payoutItemId = payoutItemId;
this.payoutBatchId = payoutBatchId;
this.payoutItem = payoutItem;
this.timeProcessed = timeProcessed;
}
/**
* Setter for error. Please use this over {@link #setErrors(Error)}.
* errors field in {@link PayoutItemDetails} takes one {@link Error} object.
* Not using lombok autogeneration as `setErrors` is not a feasible option.
*/
public PayoutItemDetails setError(Error error) {
this.errors = error;
return this;
}
/**
* Getter for error. Please use this over {@link #getErrors()}.
* errors field in {@link PayoutItemDetails} takes one {@link Error} object.
*/
public Error getError() {
return this.errors;
}
/**
* The ID for the payout item. Viewable when you show details for a batch payout.
*/
@java.lang.SuppressWarnings("all")
public String getPayoutItemId() {
return this.payoutItemId;
}
/**
* The PayPal-generated ID for the transaction.
*/
@java.lang.SuppressWarnings("all")
public String getTransactionId() {
return this.transactionId;
}
/**
* The transaction status.
*/
@java.lang.SuppressWarnings("all")
public String getTransactionStatus() {
return this.transactionStatus;
}
/**
* The amount of money, in U.S. dollars, for fees.
*/
@java.lang.SuppressWarnings("all")
public Currency getPayoutItemFee() {
return this.payoutItemFee;
}
/**
* The PayPal-generated ID for the batch payout.
*/
@java.lang.SuppressWarnings("all")
public String getPayoutBatchId() {
return this.payoutBatchId;
}
/**
* A sender-specified ID number. Tracks the batch payout in an accounting system.
*/
@java.lang.SuppressWarnings("all")
public String getSenderBatchId() {
return this.senderBatchId;
}
/**
* The sender-provided information for the payout item.
*/
@java.lang.SuppressWarnings("all")
public PayoutItem getPayoutItem() {
return this.payoutItem;
}
/**
* The date and time when this item was last processed.
*/
@java.lang.SuppressWarnings("all")
public String getTimeProcessed() {
return this.timeProcessed;
}
/**
*/
@java.lang.SuppressWarnings("all")
public Error getErrors() {
return this.errors;
}
/**
* The HATEOAS links related to the call.
*/
@java.lang.SuppressWarnings("all")
public List getLinks() {
return this.links;
}
/**
* The ID for the payout item. Viewable when you show details for a batch payout.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PayoutItemDetails setPayoutItemId(final String payoutItemId) {
this.payoutItemId = payoutItemId;
return this;
}
/**
* The PayPal-generated ID for the transaction.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PayoutItemDetails setTransactionId(final String transactionId) {
this.transactionId = transactionId;
return this;
}
/**
* The transaction status.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PayoutItemDetails setTransactionStatus(final String transactionStatus) {
this.transactionStatus = transactionStatus;
return this;
}
/**
* The amount of money, in U.S. dollars, for fees.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PayoutItemDetails setPayoutItemFee(final Currency payoutItemFee) {
this.payoutItemFee = payoutItemFee;
return this;
}
/**
* The PayPal-generated ID for the batch payout.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PayoutItemDetails setPayoutBatchId(final String payoutBatchId) {
this.payoutBatchId = payoutBatchId;
return this;
}
/**
* A sender-specified ID number. Tracks the batch payout in an accounting system.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PayoutItemDetails setSenderBatchId(final String senderBatchId) {
this.senderBatchId = senderBatchId;
return this;
}
/**
* The sender-provided information for the payout item.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PayoutItemDetails setPayoutItem(final PayoutItem payoutItem) {
this.payoutItem = payoutItem;
return this;
}
/**
* The date and time when this item was last processed.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PayoutItemDetails setTimeProcessed(final String timeProcessed) {
this.timeProcessed = timeProcessed;
return this;
}
/**
*
* @return this
*/
@java.lang.SuppressWarnings("all")
public PayoutItemDetails setErrors(final Error errors) {
this.errors = errors;
return this;
}
/**
* The HATEOAS links related to the call.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PayoutItemDetails setLinks(final List links) {
this.links = links;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof PayoutItemDetails)) return false;
final PayoutItemDetails other = (PayoutItemDetails) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (!super.equals(o)) return false;
final java.lang.Object this$payoutItemId = this.getPayoutItemId();
final java.lang.Object other$payoutItemId = other.getPayoutItemId();
if (this$payoutItemId == null ? other$payoutItemId != null : !this$payoutItemId.equals(other$payoutItemId)) return false;
final java.lang.Object this$transactionId = this.getTransactionId();
final java.lang.Object other$transactionId = other.getTransactionId();
if (this$transactionId == null ? other$transactionId != null : !this$transactionId.equals(other$transactionId)) return false;
final java.lang.Object this$transactionStatus = this.getTransactionStatus();
final java.lang.Object other$transactionStatus = other.getTransactionStatus();
if (this$transactionStatus == null ? other$transactionStatus != null : !this$transactionStatus.equals(other$transactionStatus)) return false;
final java.lang.Object this$payoutItemFee = this.getPayoutItemFee();
final java.lang.Object other$payoutItemFee = other.getPayoutItemFee();
if (this$payoutItemFee == null ? other$payoutItemFee != null : !this$payoutItemFee.equals(other$payoutItemFee)) return false;
final java.lang.Object this$payoutBatchId = this.getPayoutBatchId();
final java.lang.Object other$payoutBatchId = other.getPayoutBatchId();
if (this$payoutBatchId == null ? other$payoutBatchId != null : !this$payoutBatchId.equals(other$payoutBatchId)) return false;
final java.lang.Object this$senderBatchId = this.getSenderBatchId();
final java.lang.Object other$senderBatchId = other.getSenderBatchId();
if (this$senderBatchId == null ? other$senderBatchId != null : !this$senderBatchId.equals(other$senderBatchId)) return false;
final java.lang.Object this$payoutItem = this.getPayoutItem();
final java.lang.Object other$payoutItem = other.getPayoutItem();
if (this$payoutItem == null ? other$payoutItem != null : !this$payoutItem.equals(other$payoutItem)) return false;
final java.lang.Object this$timeProcessed = this.getTimeProcessed();
final java.lang.Object other$timeProcessed = other.getTimeProcessed();
if (this$timeProcessed == null ? other$timeProcessed != null : !this$timeProcessed.equals(other$timeProcessed)) return false;
final java.lang.Object this$errors = this.getErrors();
final java.lang.Object other$errors = other.getErrors();
if (this$errors == null ? other$errors != null : !this$errors.equals(other$errors)) return false;
final java.lang.Object this$links = this.getLinks();
final java.lang.Object other$links = other.getLinks();
if (this$links == null ? other$links != null : !this$links.equals(other$links)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof PayoutItemDetails;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + super.hashCode();
final java.lang.Object $payoutItemId = this.getPayoutItemId();
result = result * PRIME + ($payoutItemId == null ? 43 : $payoutItemId.hashCode());
final java.lang.Object $transactionId = this.getTransactionId();
result = result * PRIME + ($transactionId == null ? 43 : $transactionId.hashCode());
final java.lang.Object $transactionStatus = this.getTransactionStatus();
result = result * PRIME + ($transactionStatus == null ? 43 : $transactionStatus.hashCode());
final java.lang.Object $payoutItemFee = this.getPayoutItemFee();
result = result * PRIME + ($payoutItemFee == null ? 43 : $payoutItemFee.hashCode());
final java.lang.Object $payoutBatchId = this.getPayoutBatchId();
result = result * PRIME + ($payoutBatchId == null ? 43 : $payoutBatchId.hashCode());
final java.lang.Object $senderBatchId = this.getSenderBatchId();
result = result * PRIME + ($senderBatchId == null ? 43 : $senderBatchId.hashCode());
final java.lang.Object $payoutItem = this.getPayoutItem();
result = result * PRIME + ($payoutItem == null ? 43 : $payoutItem.hashCode());
final java.lang.Object $timeProcessed = this.getTimeProcessed();
result = result * PRIME + ($timeProcessed == null ? 43 : $timeProcessed.hashCode());
final java.lang.Object $errors = this.getErrors();
result = result * PRIME + ($errors == null ? 43 : $errors.hashCode());
final java.lang.Object $links = this.getLinks();
result = result * PRIME + ($links == null ? 43 : $links.hashCode());
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy