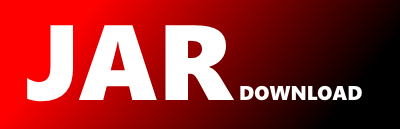
com.paypal.api.payments.Plan Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.liferay.commerce.payment.method.paypal
Show all versions of com.liferay.commerce.payment.method.paypal
Liferay Commerce Payment Method PayPal
The newest version!
// Generated by delombok at Thu Nov 16 13:48:05 CST 2017
package com.paypal.api.payments;
import com.paypal.base.rest.*;
import java.util.List;
import java.util.Map;
public class Plan extends PayPalResource {
/**
* Identifier of the billing plan. 128 characters max.
*/
private String id;
/**
* Name of the billing plan. 128 characters max.
*/
private String name;
/**
* Description of the billing plan. 128 characters max.
*/
private String description;
/**
* Type of the billing plan. Allowed values: `FIXED`, `INFINITE`.
*/
private String type;
/**
* Status of the billing plan. Allowed values: `CREATED`, `ACTIVE`, `INACTIVE`, and `DELETED`.
*/
private String state;
/**
* Time when the billing plan was created. Format YYYY-MM-DDTimeTimezone, as defined in [ISO8601](http://tools.ietf.org/html/rfc3339#section-5.6).
*/
private String createTime;
/**
* Time when this billing plan was updated. Format YYYY-MM-DDTimeTimezone, as defined in [ISO8601](http://tools.ietf.org/html/rfc3339#section-5.6).
*/
private String updateTime;
/**
* Array of payment definitions for this billing plan.
*/
private List paymentDefinitions;
/**
* Array of terms for this billing plan.
*/
private List terms;
/**
* Specific preferences such as: set up fee, max fail attempts, autobill amount, and others that are configured for this billing plan.
*/
private MerchantPreferences merchantPreferences;
/**
*/
private List links;
/**
* Default Constructor
*/
public Plan() {
}
/**
* Parameterized Constructor
*/
public Plan(String name, String description, String type) {
this.name = name;
this.description = description;
this.type = type;
}
/**
* Retrieve the details for a particular billing plan by passing the billing plan ID to the request URI.
* @deprecated Please use {@link #get(APIContext, String)} instead.
*
* @param accessToken
* Access Token used for the API call.
* @param planId
* String
* @return Plan
* @throws PayPalRESTException
*/
public static Plan get(String accessToken, String planId) throws PayPalRESTException {
APIContext apiContext = new APIContext(accessToken);
return get(apiContext, planId);
}
/**
* Retrieve the details for a particular billing plan by passing the billing plan ID to the request URI.
* @param apiContext
* {@link APIContext} used for the API call.
* @param planId
* String
* @return Plan
* @throws PayPalRESTException
*/
public static Plan get(APIContext apiContext, String planId) throws PayPalRESTException {
if (planId == null) {
throw new IllegalArgumentException("planId cannot be null");
}
Object[] parameters = new Object[] {planId};
String pattern = "v1/payments/billing-plans/{0}";
String resourcePath = RESTUtil.formatURIPath(pattern, parameters);
String payLoad = "";
return configureAndExecute(apiContext, HttpMethod.GET, resourcePath, payLoad, Plan.class);
}
/**
* Create a new billing plan by passing the details for the plan, including the plan name, description, and type, to the request URI.
* @deprecated Please use {@link #create(APIContext)} instead.
*
* @param accessToken
* Access Token used for the API call.
* @return Plan
* @throws PayPalRESTException
*/
public Plan create(String accessToken) throws PayPalRESTException {
APIContext apiContext = new APIContext(accessToken);
return create(apiContext);
}
/**
* Create a new billing plan by passing the details for the plan, including the plan name, description, and type, to the request URI.
* @param apiContext
* {@link APIContext} used for the API call.
* @return Plan
* @throws PayPalRESTException
*/
public Plan create(APIContext apiContext) throws PayPalRESTException {
String resourcePath = "v1/payments/billing-plans";
String payLoad = this.toJSON();
return configureAndExecute(apiContext, HttpMethod.POST, resourcePath, payLoad, Plan.class);
}
/**
* Replace specific fields within a billing plan by passing the ID of the billing plan to the request URI. In addition, pass a patch object in the request JSON that specifies the operation to perform, field to update, and new value for each update.
* @deprecated Please use {@link #update(APIContext, List)} instead.
*
* @param accessToken
* Access Token used for the API call.
* @param patchRequest
* PatchRequest
* @throws PayPalRESTException
*/
public void update(String accessToken, List patchRequest) throws PayPalRESTException {
APIContext apiContext = new APIContext(accessToken);
update(apiContext, patchRequest);
return;
}
/**
* Replace specific fields within a billing plan by passing the ID of the billing plan to the request URI. In addition, pass a patch object in the request JSON that specifies the operation to perform, field to update, and new value for each update.
* @param apiContext
* {@link APIContext} used for the API call.
* @param patchRequest
* PatchRequest
* @throws PayPalRESTException
*/
public void update(APIContext apiContext, List patchRequest) throws PayPalRESTException {
if (this.getId() == null) {
throw new IllegalArgumentException("Id cannot be null");
}
if (patchRequest == null) {
throw new IllegalArgumentException("patchRequest cannot be null");
}
Object[] parameters = new Object[] {this.getId()};
String pattern = "v1/payments/billing-plans/{0}";
String resourcePath = RESTUtil.formatURIPath(pattern, parameters);
String payLoad = JSONFormatter.toJSON(patchRequest);
configureAndExecute(apiContext, HttpMethod.PATCH, resourcePath, payLoad, null);
return;
}
/**
* List billing plans according to optional query string parameters specified.
* @deprecated Please use {@link #list(APIContext, Map)} instead.
*
* @param accessToken
* Access Token used for the API call.
* @param containerMap
* Map
* @return PlanList
* @throws PayPalRESTException
*/
public static PlanList list(String accessToken, Map containerMap) throws PayPalRESTException {
APIContext apiContext = new APIContext(accessToken);
return list(apiContext, containerMap);
}
/**
* List billing plans according to optional query string parameters specified.
* @param apiContext
* {@link APIContext} used for the API call.
* @param containerMap
* Map
* @return PlanList
* @throws PayPalRESTException
*/
public static PlanList list(APIContext apiContext, Map containerMap) throws PayPalRESTException {
if (containerMap == null) {
throw new IllegalArgumentException("containerMap cannot be null");
}
Object[] parameters = new Object[] {containerMap};
String pattern = "v1/payments/billing-plans?page_size={0}&status={1}&page={2}&total_required={3}";
String resourcePath = RESTUtil.formatURIPath(pattern, parameters);
String payLoad = "";
PlanList plans = configureAndExecute(apiContext, HttpMethod.GET, resourcePath, payLoad, PlanList.class);
return plans;
}
/**
* Identifier of the billing plan. 128 characters max.
*/
@java.lang.SuppressWarnings("all")
public String getId() {
return this.id;
}
/**
* Name of the billing plan. 128 characters max.
*/
@java.lang.SuppressWarnings("all")
public String getName() {
return this.name;
}
/**
* Description of the billing plan. 128 characters max.
*/
@java.lang.SuppressWarnings("all")
public String getDescription() {
return this.description;
}
/**
* Type of the billing plan. Allowed values: `FIXED`, `INFINITE`.
*/
@java.lang.SuppressWarnings("all")
public String getType() {
return this.type;
}
/**
* Status of the billing plan. Allowed values: `CREATED`, `ACTIVE`, `INACTIVE`, and `DELETED`.
*/
@java.lang.SuppressWarnings("all")
public String getState() {
return this.state;
}
/**
* Time when the billing plan was created. Format YYYY-MM-DDTimeTimezone, as defined in [ISO8601](http://tools.ietf.org/html/rfc3339#section-5.6).
*/
@java.lang.SuppressWarnings("all")
public String getCreateTime() {
return this.createTime;
}
/**
* Time when this billing plan was updated. Format YYYY-MM-DDTimeTimezone, as defined in [ISO8601](http://tools.ietf.org/html/rfc3339#section-5.6).
*/
@java.lang.SuppressWarnings("all")
public String getUpdateTime() {
return this.updateTime;
}
/**
* Array of payment definitions for this billing plan.
*/
@java.lang.SuppressWarnings("all")
public List getPaymentDefinitions() {
return this.paymentDefinitions;
}
/**
* Array of terms for this billing plan.
*/
@java.lang.SuppressWarnings("all")
public List getTerms() {
return this.terms;
}
/**
* Specific preferences such as: set up fee, max fail attempts, autobill amount, and others that are configured for this billing plan.
*/
@java.lang.SuppressWarnings("all")
public MerchantPreferences getMerchantPreferences() {
return this.merchantPreferences;
}
/**
*/
@java.lang.SuppressWarnings("all")
public List getLinks() {
return this.links;
}
/**
* Identifier of the billing plan. 128 characters max.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Plan setId(final String id) {
this.id = id;
return this;
}
/**
* Name of the billing plan. 128 characters max.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Plan setName(final String name) {
this.name = name;
return this;
}
/**
* Description of the billing plan. 128 characters max.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Plan setDescription(final String description) {
this.description = description;
return this;
}
/**
* Type of the billing plan. Allowed values: `FIXED`, `INFINITE`.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Plan setType(final String type) {
this.type = type;
return this;
}
/**
* Status of the billing plan. Allowed values: `CREATED`, `ACTIVE`, `INACTIVE`, and `DELETED`.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Plan setState(final String state) {
this.state = state;
return this;
}
/**
* Time when the billing plan was created. Format YYYY-MM-DDTimeTimezone, as defined in [ISO8601](http://tools.ietf.org/html/rfc3339#section-5.6).
* @return this
*/
@java.lang.SuppressWarnings("all")
public Plan setCreateTime(final String createTime) {
this.createTime = createTime;
return this;
}
/**
* Time when this billing plan was updated. Format YYYY-MM-DDTimeTimezone, as defined in [ISO8601](http://tools.ietf.org/html/rfc3339#section-5.6).
* @return this
*/
@java.lang.SuppressWarnings("all")
public Plan setUpdateTime(final String updateTime) {
this.updateTime = updateTime;
return this;
}
/**
* Array of payment definitions for this billing plan.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Plan setPaymentDefinitions(final List paymentDefinitions) {
this.paymentDefinitions = paymentDefinitions;
return this;
}
/**
* Array of terms for this billing plan.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Plan setTerms(final List terms) {
this.terms = terms;
return this;
}
/**
* Specific preferences such as: set up fee, max fail attempts, autobill amount, and others that are configured for this billing plan.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Plan setMerchantPreferences(final MerchantPreferences merchantPreferences) {
this.merchantPreferences = merchantPreferences;
return this;
}
/**
*
* @return this
*/
@java.lang.SuppressWarnings("all")
public Plan setLinks(final List links) {
this.links = links;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Plan)) return false;
final Plan other = (Plan) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (!super.equals(o)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$name = this.getName();
final java.lang.Object other$name = other.getName();
if (this$name == null ? other$name != null : !this$name.equals(other$name)) return false;
final java.lang.Object this$description = this.getDescription();
final java.lang.Object other$description = other.getDescription();
if (this$description == null ? other$description != null : !this$description.equals(other$description)) return false;
final java.lang.Object this$type = this.getType();
final java.lang.Object other$type = other.getType();
if (this$type == null ? other$type != null : !this$type.equals(other$type)) return false;
final java.lang.Object this$state = this.getState();
final java.lang.Object other$state = other.getState();
if (this$state == null ? other$state != null : !this$state.equals(other$state)) return false;
final java.lang.Object this$createTime = this.getCreateTime();
final java.lang.Object other$createTime = other.getCreateTime();
if (this$createTime == null ? other$createTime != null : !this$createTime.equals(other$createTime)) return false;
final java.lang.Object this$updateTime = this.getUpdateTime();
final java.lang.Object other$updateTime = other.getUpdateTime();
if (this$updateTime == null ? other$updateTime != null : !this$updateTime.equals(other$updateTime)) return false;
final java.lang.Object this$paymentDefinitions = this.getPaymentDefinitions();
final java.lang.Object other$paymentDefinitions = other.getPaymentDefinitions();
if (this$paymentDefinitions == null ? other$paymentDefinitions != null : !this$paymentDefinitions.equals(other$paymentDefinitions)) return false;
final java.lang.Object this$terms = this.getTerms();
final java.lang.Object other$terms = other.getTerms();
if (this$terms == null ? other$terms != null : !this$terms.equals(other$terms)) return false;
final java.lang.Object this$merchantPreferences = this.getMerchantPreferences();
final java.lang.Object other$merchantPreferences = other.getMerchantPreferences();
if (this$merchantPreferences == null ? other$merchantPreferences != null : !this$merchantPreferences.equals(other$merchantPreferences)) return false;
final java.lang.Object this$links = this.getLinks();
final java.lang.Object other$links = other.getLinks();
if (this$links == null ? other$links != null : !this$links.equals(other$links)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Plan;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + super.hashCode();
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $name = this.getName();
result = result * PRIME + ($name == null ? 43 : $name.hashCode());
final java.lang.Object $description = this.getDescription();
result = result * PRIME + ($description == null ? 43 : $description.hashCode());
final java.lang.Object $type = this.getType();
result = result * PRIME + ($type == null ? 43 : $type.hashCode());
final java.lang.Object $state = this.getState();
result = result * PRIME + ($state == null ? 43 : $state.hashCode());
final java.lang.Object $createTime = this.getCreateTime();
result = result * PRIME + ($createTime == null ? 43 : $createTime.hashCode());
final java.lang.Object $updateTime = this.getUpdateTime();
result = result * PRIME + ($updateTime == null ? 43 : $updateTime.hashCode());
final java.lang.Object $paymentDefinitions = this.getPaymentDefinitions();
result = result * PRIME + ($paymentDefinitions == null ? 43 : $paymentDefinitions.hashCode());
final java.lang.Object $terms = this.getTerms();
result = result * PRIME + ($terms == null ? 43 : $terms.hashCode());
final java.lang.Object $merchantPreferences = this.getMerchantPreferences();
result = result * PRIME + ($merchantPreferences == null ? 43 : $merchantPreferences.hashCode());
final java.lang.Object $links = this.getLinks();
result = result * PRIME + ($links == null ? 43 : $links.hashCode());
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy