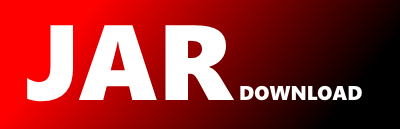
com.paypal.api.payments.ProcessorResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.liferay.commerce.payment.method.paypal
Show all versions of com.liferay.commerce.payment.method.paypal
Liferay Commerce Payment Method PayPal
The newest version!
// Generated by delombok at Thu Nov 16 13:48:05 CST 2017
package com.paypal.api.payments;
import com.paypal.base.rest.PayPalModel;
public class ProcessorResponse extends PayPalModel {
/**
* Paypal normalized response code, generated from the processor's specific response code
*/
private String responseCode;
/**
* Address Verification System response code. https://developer.paypal.com/webapps/developer/docs/classic/api/AVSResponseCodes/
*/
private String avsCode;
/**
* CVV System response code. https://developer.paypal.com/webapps/developer/docs/classic/api/AVSResponseCodes/
*/
private String cvvCode;
/**
* Provides merchant advice on how to handle declines related to recurring payments
*/
private String adviceCode;
/**
* Response back from the authorization. Provided by the processor
*/
private String eciSubmitted;
/**
* Visa Payer Authentication Service status. Will be return from processor
*/
private String vpas;
/**
* Default Constructor
*/
public ProcessorResponse() {
}
/**
* Parameterized Constructor
*/
public ProcessorResponse(String responseCode) {
this.responseCode = responseCode;
}
/**
* Paypal normalized response code, generated from the processor's specific response code
*/
@java.lang.SuppressWarnings("all")
public String getResponseCode() {
return this.responseCode;
}
/**
* Address Verification System response code. https://developer.paypal.com/webapps/developer/docs/classic/api/AVSResponseCodes/
*/
@java.lang.SuppressWarnings("all")
public String getAvsCode() {
return this.avsCode;
}
/**
* CVV System response code. https://developer.paypal.com/webapps/developer/docs/classic/api/AVSResponseCodes/
*/
@java.lang.SuppressWarnings("all")
public String getCvvCode() {
return this.cvvCode;
}
/**
* Provides merchant advice on how to handle declines related to recurring payments
*/
@java.lang.SuppressWarnings("all")
public String getAdviceCode() {
return this.adviceCode;
}
/**
* Response back from the authorization. Provided by the processor
*/
@java.lang.SuppressWarnings("all")
public String getEciSubmitted() {
return this.eciSubmitted;
}
/**
* Visa Payer Authentication Service status. Will be return from processor
*/
@java.lang.SuppressWarnings("all")
public String getVpas() {
return this.vpas;
}
/**
* Paypal normalized response code, generated from the processor's specific response code
* @return this
*/
@java.lang.SuppressWarnings("all")
public ProcessorResponse setResponseCode(final String responseCode) {
this.responseCode = responseCode;
return this;
}
/**
* Address Verification System response code. https://developer.paypal.com/webapps/developer/docs/classic/api/AVSResponseCodes/
* @return this
*/
@java.lang.SuppressWarnings("all")
public ProcessorResponse setAvsCode(final String avsCode) {
this.avsCode = avsCode;
return this;
}
/**
* CVV System response code. https://developer.paypal.com/webapps/developer/docs/classic/api/AVSResponseCodes/
* @return this
*/
@java.lang.SuppressWarnings("all")
public ProcessorResponse setCvvCode(final String cvvCode) {
this.cvvCode = cvvCode;
return this;
}
/**
* Provides merchant advice on how to handle declines related to recurring payments
* @return this
*/
@java.lang.SuppressWarnings("all")
public ProcessorResponse setAdviceCode(final String adviceCode) {
this.adviceCode = adviceCode;
return this;
}
/**
* Response back from the authorization. Provided by the processor
* @return this
*/
@java.lang.SuppressWarnings("all")
public ProcessorResponse setEciSubmitted(final String eciSubmitted) {
this.eciSubmitted = eciSubmitted;
return this;
}
/**
* Visa Payer Authentication Service status. Will be return from processor
* @return this
*/
@java.lang.SuppressWarnings("all")
public ProcessorResponse setVpas(final String vpas) {
this.vpas = vpas;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof ProcessorResponse)) return false;
final ProcessorResponse other = (ProcessorResponse) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (!super.equals(o)) return false;
final java.lang.Object this$responseCode = this.getResponseCode();
final java.lang.Object other$responseCode = other.getResponseCode();
if (this$responseCode == null ? other$responseCode != null : !this$responseCode.equals(other$responseCode)) return false;
final java.lang.Object this$avsCode = this.getAvsCode();
final java.lang.Object other$avsCode = other.getAvsCode();
if (this$avsCode == null ? other$avsCode != null : !this$avsCode.equals(other$avsCode)) return false;
final java.lang.Object this$cvvCode = this.getCvvCode();
final java.lang.Object other$cvvCode = other.getCvvCode();
if (this$cvvCode == null ? other$cvvCode != null : !this$cvvCode.equals(other$cvvCode)) return false;
final java.lang.Object this$adviceCode = this.getAdviceCode();
final java.lang.Object other$adviceCode = other.getAdviceCode();
if (this$adviceCode == null ? other$adviceCode != null : !this$adviceCode.equals(other$adviceCode)) return false;
final java.lang.Object this$eciSubmitted = this.getEciSubmitted();
final java.lang.Object other$eciSubmitted = other.getEciSubmitted();
if (this$eciSubmitted == null ? other$eciSubmitted != null : !this$eciSubmitted.equals(other$eciSubmitted)) return false;
final java.lang.Object this$vpas = this.getVpas();
final java.lang.Object other$vpas = other.getVpas();
if (this$vpas == null ? other$vpas != null : !this$vpas.equals(other$vpas)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof ProcessorResponse;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + super.hashCode();
final java.lang.Object $responseCode = this.getResponseCode();
result = result * PRIME + ($responseCode == null ? 43 : $responseCode.hashCode());
final java.lang.Object $avsCode = this.getAvsCode();
result = result * PRIME + ($avsCode == null ? 43 : $avsCode.hashCode());
final java.lang.Object $cvvCode = this.getCvvCode();
result = result * PRIME + ($cvvCode == null ? 43 : $cvvCode.hashCode());
final java.lang.Object $adviceCode = this.getAdviceCode();
result = result * PRIME + ($adviceCode == null ? 43 : $adviceCode.hashCode());
final java.lang.Object $eciSubmitted = this.getEciSubmitted();
result = result * PRIME + ($eciSubmitted == null ? 43 : $eciSubmitted.hashCode());
final java.lang.Object $vpas = this.getVpas();
result = result * PRIME + ($vpas == null ? 43 : $vpas.hashCode());
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy