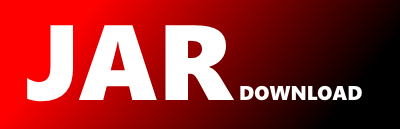
com.paypal.api.payments.RelatedResources Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.liferay.commerce.payment.method.paypal
Show all versions of com.liferay.commerce.payment.method.paypal
Liferay Commerce Payment Method PayPal
The newest version!
// Generated by delombok at Thu Nov 16 13:48:05 CST 2017
package com.paypal.api.payments;
import com.paypal.base.rest.PayPalModel;
public class RelatedResources extends PayPalModel {
/**
* Sale transaction
*/
private Sale sale;
/**
* Authorization transaction
*/
private Authorization authorization;
/**
* Order transaction
*/
private Order order;
/**
* Capture transaction
*/
private Capture capture;
/**
* Refund transaction
*/
private Refund refund;
/**
* Default Constructor
*/
public RelatedResources() {
}
/**
* Sale transaction
*/
@java.lang.SuppressWarnings("all")
public Sale getSale() {
return this.sale;
}
/**
* Authorization transaction
*/
@java.lang.SuppressWarnings("all")
public Authorization getAuthorization() {
return this.authorization;
}
/**
* Order transaction
*/
@java.lang.SuppressWarnings("all")
public Order getOrder() {
return this.order;
}
/**
* Capture transaction
*/
@java.lang.SuppressWarnings("all")
public Capture getCapture() {
return this.capture;
}
/**
* Refund transaction
*/
@java.lang.SuppressWarnings("all")
public Refund getRefund() {
return this.refund;
}
/**
* Sale transaction
* @return this
*/
@java.lang.SuppressWarnings("all")
public RelatedResources setSale(final Sale sale) {
this.sale = sale;
return this;
}
/**
* Authorization transaction
* @return this
*/
@java.lang.SuppressWarnings("all")
public RelatedResources setAuthorization(final Authorization authorization) {
this.authorization = authorization;
return this;
}
/**
* Order transaction
* @return this
*/
@java.lang.SuppressWarnings("all")
public RelatedResources setOrder(final Order order) {
this.order = order;
return this;
}
/**
* Capture transaction
* @return this
*/
@java.lang.SuppressWarnings("all")
public RelatedResources setCapture(final Capture capture) {
this.capture = capture;
return this;
}
/**
* Refund transaction
* @return this
*/
@java.lang.SuppressWarnings("all")
public RelatedResources setRefund(final Refund refund) {
this.refund = refund;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof RelatedResources)) return false;
final RelatedResources other = (RelatedResources) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (!super.equals(o)) return false;
final java.lang.Object this$sale = this.getSale();
final java.lang.Object other$sale = other.getSale();
if (this$sale == null ? other$sale != null : !this$sale.equals(other$sale)) return false;
final java.lang.Object this$authorization = this.getAuthorization();
final java.lang.Object other$authorization = other.getAuthorization();
if (this$authorization == null ? other$authorization != null : !this$authorization.equals(other$authorization)) return false;
final java.lang.Object this$order = this.getOrder();
final java.lang.Object other$order = other.getOrder();
if (this$order == null ? other$order != null : !this$order.equals(other$order)) return false;
final java.lang.Object this$capture = this.getCapture();
final java.lang.Object other$capture = other.getCapture();
if (this$capture == null ? other$capture != null : !this$capture.equals(other$capture)) return false;
final java.lang.Object this$refund = this.getRefund();
final java.lang.Object other$refund = other.getRefund();
if (this$refund == null ? other$refund != null : !this$refund.equals(other$refund)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof RelatedResources;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + super.hashCode();
final java.lang.Object $sale = this.getSale();
result = result * PRIME + ($sale == null ? 43 : $sale.hashCode());
final java.lang.Object $authorization = this.getAuthorization();
result = result * PRIME + ($authorization == null ? 43 : $authorization.hashCode());
final java.lang.Object $order = this.getOrder();
result = result * PRIME + ($order == null ? 43 : $order.hashCode());
final java.lang.Object $capture = this.getCapture();
result = result * PRIME + ($capture == null ? 43 : $capture.hashCode());
final java.lang.Object $refund = this.getRefund();
result = result * PRIME + ($refund == null ? 43 : $refund.hashCode());
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy