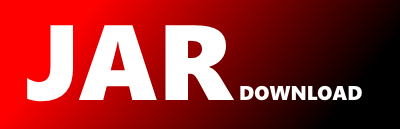
com.paypal.api.payments.ShippingInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.liferay.commerce.payment.method.paypal
Show all versions of com.liferay.commerce.payment.method.paypal
Liferay Commerce Payment Method PayPal
The newest version!
// Generated by delombok at Thu Nov 16 13:48:05 CST 2017
package com.paypal.api.payments;
import com.paypal.base.rest.PayPalModel;
public class ShippingInfo extends PayPalModel {
/**
* The invoice recipient first name. Maximum length is 30 characters.
*/
private String firstName;
/**
* The invoice recipient last name. Maximum length is 30 characters.
*/
private String lastName;
/**
* The invoice recipient company business name. Maximum length is 100 characters.
*/
private String businessName;
/**
* The invoice recipient address.
*/
private InvoiceAddress address;
/**
* The invoice recipient phone number.
*/
private Phone phone;
/**
* Default Constructor
*/
public ShippingInfo() {
}
/**
* The invoice recipient first name. Maximum length is 30 characters.
*/
@java.lang.SuppressWarnings("all")
public String getFirstName() {
return this.firstName;
}
/**
* The invoice recipient last name. Maximum length is 30 characters.
*/
@java.lang.SuppressWarnings("all")
public String getLastName() {
return this.lastName;
}
/**
* The invoice recipient company business name. Maximum length is 100 characters.
*/
@java.lang.SuppressWarnings("all")
public String getBusinessName() {
return this.businessName;
}
/**
* The invoice recipient address.
*/
@java.lang.SuppressWarnings("all")
public InvoiceAddress getAddress() {
return this.address;
}
/**
* The invoice recipient phone number.
*/
@java.lang.SuppressWarnings("all")
public Phone getPhone() {
return this.phone;
}
/**
* The invoice recipient first name. Maximum length is 30 characters.
* @return this
*/
@java.lang.SuppressWarnings("all")
public ShippingInfo setFirstName(final String firstName) {
this.firstName = firstName;
return this;
}
/**
* The invoice recipient last name. Maximum length is 30 characters.
* @return this
*/
@java.lang.SuppressWarnings("all")
public ShippingInfo setLastName(final String lastName) {
this.lastName = lastName;
return this;
}
/**
* The invoice recipient company business name. Maximum length is 100 characters.
* @return this
*/
@java.lang.SuppressWarnings("all")
public ShippingInfo setBusinessName(final String businessName) {
this.businessName = businessName;
return this;
}
/**
* The invoice recipient address.
* @return this
*/
@java.lang.SuppressWarnings("all")
public ShippingInfo setAddress(final InvoiceAddress address) {
this.address = address;
return this;
}
/**
* The invoice recipient phone number.
* @return this
*/
@java.lang.SuppressWarnings("all")
public ShippingInfo setPhone(final Phone phone) {
this.phone = phone;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof ShippingInfo)) return false;
final ShippingInfo other = (ShippingInfo) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (!super.equals(o)) return false;
final java.lang.Object this$firstName = this.getFirstName();
final java.lang.Object other$firstName = other.getFirstName();
if (this$firstName == null ? other$firstName != null : !this$firstName.equals(other$firstName)) return false;
final java.lang.Object this$lastName = this.getLastName();
final java.lang.Object other$lastName = other.getLastName();
if (this$lastName == null ? other$lastName != null : !this$lastName.equals(other$lastName)) return false;
final java.lang.Object this$businessName = this.getBusinessName();
final java.lang.Object other$businessName = other.getBusinessName();
if (this$businessName == null ? other$businessName != null : !this$businessName.equals(other$businessName)) return false;
final java.lang.Object this$address = this.getAddress();
final java.lang.Object other$address = other.getAddress();
if (this$address == null ? other$address != null : !this$address.equals(other$address)) return false;
final java.lang.Object this$phone = this.getPhone();
final java.lang.Object other$phone = other.getPhone();
if (this$phone == null ? other$phone != null : !this$phone.equals(other$phone)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof ShippingInfo;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + super.hashCode();
final java.lang.Object $firstName = this.getFirstName();
result = result * PRIME + ($firstName == null ? 43 : $firstName.hashCode());
final java.lang.Object $lastName = this.getLastName();
result = result * PRIME + ($lastName == null ? 43 : $lastName.hashCode());
final java.lang.Object $businessName = this.getBusinessName();
result = result * PRIME + ($businessName == null ? 43 : $businessName.hashCode());
final java.lang.Object $address = this.getAddress();
result = result * PRIME + ($address == null ? 43 : $address.hashCode());
final java.lang.Object $phone = this.getPhone();
result = result * PRIME + ($phone == null ? 43 : $phone.hashCode());
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy