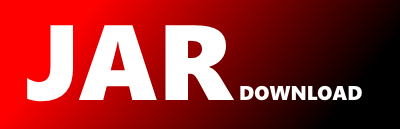
com.paypal.api.payments.Tax Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.liferay.commerce.payment.method.paypal
Show all versions of com.liferay.commerce.payment.method.paypal
Liferay Commerce Payment Method PayPal
The newest version!
// Generated by delombok at Thu Nov 16 13:48:05 CST 2017
package com.paypal.api.payments;
import com.paypal.base.rest.PayPalModel;
public class Tax extends PayPalModel {
/**
* The resource ID.
*/
private String id;
/**
* The tax name. Maximum length is 20 characters.
*/
private String name;
/**
* The rate of the specified tax. Valid range is from 0.001 to 99.999.
*/
private float percent;
/**
* The tax as a monetary amount. Cannot be specified in a request.
*/
private Currency amount;
/**
* Default Constructor
*/
public Tax() {
}
/**
* Parameterized Constructor
*/
public Tax(String name, float percent) {
this.name = name;
this.percent = percent;
}
/**
* The resource ID.
*/
@java.lang.SuppressWarnings("all")
public String getId() {
return this.id;
}
/**
* The tax name. Maximum length is 20 characters.
*/
@java.lang.SuppressWarnings("all")
public String getName() {
return this.name;
}
/**
* The rate of the specified tax. Valid range is from 0.001 to 99.999.
*/
@java.lang.SuppressWarnings("all")
public float getPercent() {
return this.percent;
}
/**
* The tax as a monetary amount. Cannot be specified in a request.
*/
@java.lang.SuppressWarnings("all")
public Currency getAmount() {
return this.amount;
}
/**
* The resource ID.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Tax setId(final String id) {
this.id = id;
return this;
}
/**
* The tax name. Maximum length is 20 characters.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Tax setName(final String name) {
this.name = name;
return this;
}
/**
* The rate of the specified tax. Valid range is from 0.001 to 99.999.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Tax setPercent(final float percent) {
this.percent = percent;
return this;
}
/**
* The tax as a monetary amount. Cannot be specified in a request.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Tax setAmount(final Currency amount) {
this.amount = amount;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Tax)) return false;
final Tax other = (Tax) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (!super.equals(o)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$name = this.getName();
final java.lang.Object other$name = other.getName();
if (this$name == null ? other$name != null : !this$name.equals(other$name)) return false;
if (java.lang.Float.compare(this.getPercent(), other.getPercent()) != 0) return false;
final java.lang.Object this$amount = this.getAmount();
final java.lang.Object other$amount = other.getAmount();
if (this$amount == null ? other$amount != null : !this$amount.equals(other$amount)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Tax;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + super.hashCode();
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $name = this.getName();
result = result * PRIME + ($name == null ? 43 : $name.hashCode());
result = result * PRIME + java.lang.Float.floatToIntBits(this.getPercent());
final java.lang.Object $amount = this.getAmount();
result = result * PRIME + ($amount == null ? 43 : $amount.hashCode());
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy