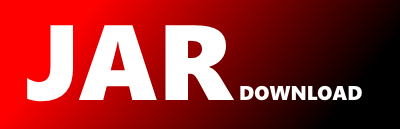
com.paypal.api.payments.TemplateData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.liferay.commerce.payment.method.paypal
Show all versions of com.liferay.commerce.payment.method.paypal
Liferay Commerce Payment Method PayPal
The newest version!
// Generated by delombok at Thu Nov 16 13:48:05 CST 2017
package com.paypal.api.payments;
import com.paypal.base.rest.PayPalModel;
import java.util.List;
public class TemplateData extends PayPalModel {
/**
* Information about the merchant who is sending the invoice.
*/
private MerchantInfo merchantInfo;
/**
* The required invoice recipient email address and any optional billing information. One recipient is supported.
*/
private List billingInfo;
/**
* For invoices sent by email, one or more email addresses to which to send a Cc: copy of the notification. Supports only email addresses under participant.
*/
private List ccInfo;
/**
* The shipping information for entities to whom items are being shipped.
*/
private ShippingInfo shippingInfo;
/**
* The list of items to include in the invoice. Maximum value is 100 items per invoice.
*/
private List items;
/**
* Optional. The payment deadline for the invoice. Value is either `term_type` or `due_date` but not both.
*/
private PaymentTerm paymentTerm;
/**
* Reference data, such as PO number, to add to the invoice. Maximum length is 60 characters.
*/
private String reference;
/**
* The invoice level discount, as a percent or an amount value.
*/
private Cost discount;
/**
* The shipping cost, as a percent or an amount value.
*/
private ShippingCost shippingCost;
/**
* The custom amount to apply on an invoice. If you include a label, the amount cannot be empty.
*/
private CustomAmount custom;
/**
* Indicates whether the invoice allows a partial payment. If set to `false`, invoice must be paid in full. If set to `true`, the invoice allows partial payments. Default is `false`.
*/
private Boolean allowPartialPayment;
/**
* If `allow_partial_payment` is set to `true`, the minimum amount allowed for a partial payment.
*/
private Currency minimumAmountDue;
/**
* Indicates whether tax is calculated before or after a discount. If set to `false`, the tax is calculated before a discount. If set to `true`, the tax is calculated after a discount. Default is `false`.
*/
private Boolean taxCalculatedAfterDiscount;
/**
* Indicates whether the unit price includes tax. Default is `false`.
*/
private Boolean taxInclusive;
/**
* General terms of the invoice. 4000 characters max.
*/
private String terms;
/**
* Note to the payer. 4000 characters max.
*/
private String note;
/**
* A private bookkeeping memo for the merchant. Maximum length is 150 characters.
*/
private String merchantMemo;
/**
* Full URL of an external image to use as the logo. Maximum length is 4000 characters.
*/
private String logoUrl;
/**
* The total amount of the invoice.
*/
private Currency totalAmount;
/**
* List of files attached to the invoice.
*/
private List attachments;
/**
* Default Constructor
*/
public TemplateData() {
}
/**
* Parameterized Constructor
*/
public TemplateData(MerchantInfo merchantInfo) {
this.merchantInfo = merchantInfo;
}
/**
* Information about the merchant who is sending the invoice.
*/
@java.lang.SuppressWarnings("all")
public MerchantInfo getMerchantInfo() {
return this.merchantInfo;
}
/**
* The required invoice recipient email address and any optional billing information. One recipient is supported.
*/
@java.lang.SuppressWarnings("all")
public List getBillingInfo() {
return this.billingInfo;
}
/**
* For invoices sent by email, one or more email addresses to which to send a Cc: copy of the notification. Supports only email addresses under participant.
*/
@java.lang.SuppressWarnings("all")
public List getCcInfo() {
return this.ccInfo;
}
/**
* The shipping information for entities to whom items are being shipped.
*/
@java.lang.SuppressWarnings("all")
public ShippingInfo getShippingInfo() {
return this.shippingInfo;
}
/**
* The list of items to include in the invoice. Maximum value is 100 items per invoice.
*/
@java.lang.SuppressWarnings("all")
public List getItems() {
return this.items;
}
/**
* Optional. The payment deadline for the invoice. Value is either `term_type` or `due_date` but not both.
*/
@java.lang.SuppressWarnings("all")
public PaymentTerm getPaymentTerm() {
return this.paymentTerm;
}
/**
* Reference data, such as PO number, to add to the invoice. Maximum length is 60 characters.
*/
@java.lang.SuppressWarnings("all")
public String getReference() {
return this.reference;
}
/**
* The invoice level discount, as a percent or an amount value.
*/
@java.lang.SuppressWarnings("all")
public Cost getDiscount() {
return this.discount;
}
/**
* The shipping cost, as a percent or an amount value.
*/
@java.lang.SuppressWarnings("all")
public ShippingCost getShippingCost() {
return this.shippingCost;
}
/**
* The custom amount to apply on an invoice. If you include a label, the amount cannot be empty.
*/
@java.lang.SuppressWarnings("all")
public CustomAmount getCustom() {
return this.custom;
}
/**
* Indicates whether the invoice allows a partial payment. If set to `false`, invoice must be paid in full. If set to `true`, the invoice allows partial payments. Default is `false`.
*/
@java.lang.SuppressWarnings("all")
public Boolean getAllowPartialPayment() {
return this.allowPartialPayment;
}
/**
* If `allow_partial_payment` is set to `true`, the minimum amount allowed for a partial payment.
*/
@java.lang.SuppressWarnings("all")
public Currency getMinimumAmountDue() {
return this.minimumAmountDue;
}
/**
* Indicates whether tax is calculated before or after a discount. If set to `false`, the tax is calculated before a discount. If set to `true`, the tax is calculated after a discount. Default is `false`.
*/
@java.lang.SuppressWarnings("all")
public Boolean getTaxCalculatedAfterDiscount() {
return this.taxCalculatedAfterDiscount;
}
/**
* Indicates whether the unit price includes tax. Default is `false`.
*/
@java.lang.SuppressWarnings("all")
public Boolean getTaxInclusive() {
return this.taxInclusive;
}
/**
* General terms of the invoice. 4000 characters max.
*/
@java.lang.SuppressWarnings("all")
public String getTerms() {
return this.terms;
}
/**
* Note to the payer. 4000 characters max.
*/
@java.lang.SuppressWarnings("all")
public String getNote() {
return this.note;
}
/**
* A private bookkeeping memo for the merchant. Maximum length is 150 characters.
*/
@java.lang.SuppressWarnings("all")
public String getMerchantMemo() {
return this.merchantMemo;
}
/**
* Full URL of an external image to use as the logo. Maximum length is 4000 characters.
*/
@java.lang.SuppressWarnings("all")
public String getLogoUrl() {
return this.logoUrl;
}
/**
* The total amount of the invoice.
*/
@java.lang.SuppressWarnings("all")
public Currency getTotalAmount() {
return this.totalAmount;
}
/**
* List of files attached to the invoice.
*/
@java.lang.SuppressWarnings("all")
public List getAttachments() {
return this.attachments;
}
/**
* Information about the merchant who is sending the invoice.
* @return this
*/
@java.lang.SuppressWarnings("all")
public TemplateData setMerchantInfo(final MerchantInfo merchantInfo) {
this.merchantInfo = merchantInfo;
return this;
}
/**
* The required invoice recipient email address and any optional billing information. One recipient is supported.
* @return this
*/
@java.lang.SuppressWarnings("all")
public TemplateData setBillingInfo(final List billingInfo) {
this.billingInfo = billingInfo;
return this;
}
/**
* For invoices sent by email, one or more email addresses to which to send a Cc: copy of the notification. Supports only email addresses under participant.
* @return this
*/
@java.lang.SuppressWarnings("all")
public TemplateData setCcInfo(final List ccInfo) {
this.ccInfo = ccInfo;
return this;
}
/**
* The shipping information for entities to whom items are being shipped.
* @return this
*/
@java.lang.SuppressWarnings("all")
public TemplateData setShippingInfo(final ShippingInfo shippingInfo) {
this.shippingInfo = shippingInfo;
return this;
}
/**
* The list of items to include in the invoice. Maximum value is 100 items per invoice.
* @return this
*/
@java.lang.SuppressWarnings("all")
public TemplateData setItems(final List items) {
this.items = items;
return this;
}
/**
* Optional. The payment deadline for the invoice. Value is either `term_type` or `due_date` but not both.
* @return this
*/
@java.lang.SuppressWarnings("all")
public TemplateData setPaymentTerm(final PaymentTerm paymentTerm) {
this.paymentTerm = paymentTerm;
return this;
}
/**
* Reference data, such as PO number, to add to the invoice. Maximum length is 60 characters.
* @return this
*/
@java.lang.SuppressWarnings("all")
public TemplateData setReference(final String reference) {
this.reference = reference;
return this;
}
/**
* The invoice level discount, as a percent or an amount value.
* @return this
*/
@java.lang.SuppressWarnings("all")
public TemplateData setDiscount(final Cost discount) {
this.discount = discount;
return this;
}
/**
* The shipping cost, as a percent or an amount value.
* @return this
*/
@java.lang.SuppressWarnings("all")
public TemplateData setShippingCost(final ShippingCost shippingCost) {
this.shippingCost = shippingCost;
return this;
}
/**
* The custom amount to apply on an invoice. If you include a label, the amount cannot be empty.
* @return this
*/
@java.lang.SuppressWarnings("all")
public TemplateData setCustom(final CustomAmount custom) {
this.custom = custom;
return this;
}
/**
* Indicates whether the invoice allows a partial payment. If set to `false`, invoice must be paid in full. If set to `true`, the invoice allows partial payments. Default is `false`.
* @return this
*/
@java.lang.SuppressWarnings("all")
public TemplateData setAllowPartialPayment(final Boolean allowPartialPayment) {
this.allowPartialPayment = allowPartialPayment;
return this;
}
/**
* If `allow_partial_payment` is set to `true`, the minimum amount allowed for a partial payment.
* @return this
*/
@java.lang.SuppressWarnings("all")
public TemplateData setMinimumAmountDue(final Currency minimumAmountDue) {
this.minimumAmountDue = minimumAmountDue;
return this;
}
/**
* Indicates whether tax is calculated before or after a discount. If set to `false`, the tax is calculated before a discount. If set to `true`, the tax is calculated after a discount. Default is `false`.
* @return this
*/
@java.lang.SuppressWarnings("all")
public TemplateData setTaxCalculatedAfterDiscount(final Boolean taxCalculatedAfterDiscount) {
this.taxCalculatedAfterDiscount = taxCalculatedAfterDiscount;
return this;
}
/**
* Indicates whether the unit price includes tax. Default is `false`.
* @return this
*/
@java.lang.SuppressWarnings("all")
public TemplateData setTaxInclusive(final Boolean taxInclusive) {
this.taxInclusive = taxInclusive;
return this;
}
/**
* General terms of the invoice. 4000 characters max.
* @return this
*/
@java.lang.SuppressWarnings("all")
public TemplateData setTerms(final String terms) {
this.terms = terms;
return this;
}
/**
* Note to the payer. 4000 characters max.
* @return this
*/
@java.lang.SuppressWarnings("all")
public TemplateData setNote(final String note) {
this.note = note;
return this;
}
/**
* A private bookkeeping memo for the merchant. Maximum length is 150 characters.
* @return this
*/
@java.lang.SuppressWarnings("all")
public TemplateData setMerchantMemo(final String merchantMemo) {
this.merchantMemo = merchantMemo;
return this;
}
/**
* Full URL of an external image to use as the logo. Maximum length is 4000 characters.
* @return this
*/
@java.lang.SuppressWarnings("all")
public TemplateData setLogoUrl(final String logoUrl) {
this.logoUrl = logoUrl;
return this;
}
/**
* The total amount of the invoice.
* @return this
*/
@java.lang.SuppressWarnings("all")
public TemplateData setTotalAmount(final Currency totalAmount) {
this.totalAmount = totalAmount;
return this;
}
/**
* List of files attached to the invoice.
* @return this
*/
@java.lang.SuppressWarnings("all")
public TemplateData setAttachments(final List attachments) {
this.attachments = attachments;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof TemplateData)) return false;
final TemplateData other = (TemplateData) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (!super.equals(o)) return false;
final java.lang.Object this$merchantInfo = this.getMerchantInfo();
final java.lang.Object other$merchantInfo = other.getMerchantInfo();
if (this$merchantInfo == null ? other$merchantInfo != null : !this$merchantInfo.equals(other$merchantInfo)) return false;
final java.lang.Object this$billingInfo = this.getBillingInfo();
final java.lang.Object other$billingInfo = other.getBillingInfo();
if (this$billingInfo == null ? other$billingInfo != null : !this$billingInfo.equals(other$billingInfo)) return false;
final java.lang.Object this$ccInfo = this.getCcInfo();
final java.lang.Object other$ccInfo = other.getCcInfo();
if (this$ccInfo == null ? other$ccInfo != null : !this$ccInfo.equals(other$ccInfo)) return false;
final java.lang.Object this$shippingInfo = this.getShippingInfo();
final java.lang.Object other$shippingInfo = other.getShippingInfo();
if (this$shippingInfo == null ? other$shippingInfo != null : !this$shippingInfo.equals(other$shippingInfo)) return false;
final java.lang.Object this$items = this.getItems();
final java.lang.Object other$items = other.getItems();
if (this$items == null ? other$items != null : !this$items.equals(other$items)) return false;
final java.lang.Object this$paymentTerm = this.getPaymentTerm();
final java.lang.Object other$paymentTerm = other.getPaymentTerm();
if (this$paymentTerm == null ? other$paymentTerm != null : !this$paymentTerm.equals(other$paymentTerm)) return false;
final java.lang.Object this$reference = this.getReference();
final java.lang.Object other$reference = other.getReference();
if (this$reference == null ? other$reference != null : !this$reference.equals(other$reference)) return false;
final java.lang.Object this$discount = this.getDiscount();
final java.lang.Object other$discount = other.getDiscount();
if (this$discount == null ? other$discount != null : !this$discount.equals(other$discount)) return false;
final java.lang.Object this$shippingCost = this.getShippingCost();
final java.lang.Object other$shippingCost = other.getShippingCost();
if (this$shippingCost == null ? other$shippingCost != null : !this$shippingCost.equals(other$shippingCost)) return false;
final java.lang.Object this$custom = this.getCustom();
final java.lang.Object other$custom = other.getCustom();
if (this$custom == null ? other$custom != null : !this$custom.equals(other$custom)) return false;
final java.lang.Object this$allowPartialPayment = this.getAllowPartialPayment();
final java.lang.Object other$allowPartialPayment = other.getAllowPartialPayment();
if (this$allowPartialPayment == null ? other$allowPartialPayment != null : !this$allowPartialPayment.equals(other$allowPartialPayment)) return false;
final java.lang.Object this$minimumAmountDue = this.getMinimumAmountDue();
final java.lang.Object other$minimumAmountDue = other.getMinimumAmountDue();
if (this$minimumAmountDue == null ? other$minimumAmountDue != null : !this$minimumAmountDue.equals(other$minimumAmountDue)) return false;
final java.lang.Object this$taxCalculatedAfterDiscount = this.getTaxCalculatedAfterDiscount();
final java.lang.Object other$taxCalculatedAfterDiscount = other.getTaxCalculatedAfterDiscount();
if (this$taxCalculatedAfterDiscount == null ? other$taxCalculatedAfterDiscount != null : !this$taxCalculatedAfterDiscount.equals(other$taxCalculatedAfterDiscount)) return false;
final java.lang.Object this$taxInclusive = this.getTaxInclusive();
final java.lang.Object other$taxInclusive = other.getTaxInclusive();
if (this$taxInclusive == null ? other$taxInclusive != null : !this$taxInclusive.equals(other$taxInclusive)) return false;
final java.lang.Object this$terms = this.getTerms();
final java.lang.Object other$terms = other.getTerms();
if (this$terms == null ? other$terms != null : !this$terms.equals(other$terms)) return false;
final java.lang.Object this$note = this.getNote();
final java.lang.Object other$note = other.getNote();
if (this$note == null ? other$note != null : !this$note.equals(other$note)) return false;
final java.lang.Object this$merchantMemo = this.getMerchantMemo();
final java.lang.Object other$merchantMemo = other.getMerchantMemo();
if (this$merchantMemo == null ? other$merchantMemo != null : !this$merchantMemo.equals(other$merchantMemo)) return false;
final java.lang.Object this$logoUrl = this.getLogoUrl();
final java.lang.Object other$logoUrl = other.getLogoUrl();
if (this$logoUrl == null ? other$logoUrl != null : !this$logoUrl.equals(other$logoUrl)) return false;
final java.lang.Object this$totalAmount = this.getTotalAmount();
final java.lang.Object other$totalAmount = other.getTotalAmount();
if (this$totalAmount == null ? other$totalAmount != null : !this$totalAmount.equals(other$totalAmount)) return false;
final java.lang.Object this$attachments = this.getAttachments();
final java.lang.Object other$attachments = other.getAttachments();
if (this$attachments == null ? other$attachments != null : !this$attachments.equals(other$attachments)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof TemplateData;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + super.hashCode();
final java.lang.Object $merchantInfo = this.getMerchantInfo();
result = result * PRIME + ($merchantInfo == null ? 43 : $merchantInfo.hashCode());
final java.lang.Object $billingInfo = this.getBillingInfo();
result = result * PRIME + ($billingInfo == null ? 43 : $billingInfo.hashCode());
final java.lang.Object $ccInfo = this.getCcInfo();
result = result * PRIME + ($ccInfo == null ? 43 : $ccInfo.hashCode());
final java.lang.Object $shippingInfo = this.getShippingInfo();
result = result * PRIME + ($shippingInfo == null ? 43 : $shippingInfo.hashCode());
final java.lang.Object $items = this.getItems();
result = result * PRIME + ($items == null ? 43 : $items.hashCode());
final java.lang.Object $paymentTerm = this.getPaymentTerm();
result = result * PRIME + ($paymentTerm == null ? 43 : $paymentTerm.hashCode());
final java.lang.Object $reference = this.getReference();
result = result * PRIME + ($reference == null ? 43 : $reference.hashCode());
final java.lang.Object $discount = this.getDiscount();
result = result * PRIME + ($discount == null ? 43 : $discount.hashCode());
final java.lang.Object $shippingCost = this.getShippingCost();
result = result * PRIME + ($shippingCost == null ? 43 : $shippingCost.hashCode());
final java.lang.Object $custom = this.getCustom();
result = result * PRIME + ($custom == null ? 43 : $custom.hashCode());
final java.lang.Object $allowPartialPayment = this.getAllowPartialPayment();
result = result * PRIME + ($allowPartialPayment == null ? 43 : $allowPartialPayment.hashCode());
final java.lang.Object $minimumAmountDue = this.getMinimumAmountDue();
result = result * PRIME + ($minimumAmountDue == null ? 43 : $minimumAmountDue.hashCode());
final java.lang.Object $taxCalculatedAfterDiscount = this.getTaxCalculatedAfterDiscount();
result = result * PRIME + ($taxCalculatedAfterDiscount == null ? 43 : $taxCalculatedAfterDiscount.hashCode());
final java.lang.Object $taxInclusive = this.getTaxInclusive();
result = result * PRIME + ($taxInclusive == null ? 43 : $taxInclusive.hashCode());
final java.lang.Object $terms = this.getTerms();
result = result * PRIME + ($terms == null ? 43 : $terms.hashCode());
final java.lang.Object $note = this.getNote();
result = result * PRIME + ($note == null ? 43 : $note.hashCode());
final java.lang.Object $merchantMemo = this.getMerchantMemo();
result = result * PRIME + ($merchantMemo == null ? 43 : $merchantMemo.hashCode());
final java.lang.Object $logoUrl = this.getLogoUrl();
result = result * PRIME + ($logoUrl == null ? 43 : $logoUrl.hashCode());
final java.lang.Object $totalAmount = this.getTotalAmount();
result = result * PRIME + ($totalAmount == null ? 43 : $totalAmount.hashCode());
final java.lang.Object $attachments = this.getAttachments();
result = result * PRIME + ($attachments == null ? 43 : $attachments.hashCode());
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy