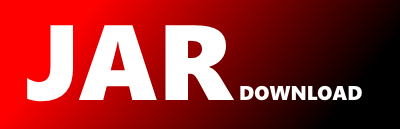
com.liferay.commerce.payment.service.persistence.impl.CommercePaymentMethodGroupRelPersistenceImpl Maven / Gradle / Ivy
Show all versions of com.liferay.commerce.payment.service
/**
* Copyright (c) 2000-present Liferay, Inc. All rights reserved.
*
* This library is free software; you can redistribute it and/or modify it under
* the terms of the GNU Lesser General Public License as published by the Free
* Software Foundation; either version 2.1 of the License, or (at your option)
* any later version.
*
* This library is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License for more
* details.
*/
package com.liferay.commerce.payment.service.persistence.impl;
import com.liferay.commerce.payment.exception.NoSuchPaymentMethodGroupRelException;
import com.liferay.commerce.payment.model.CommercePaymentMethodGroupRel;
import com.liferay.commerce.payment.model.CommercePaymentMethodGroupRelTable;
import com.liferay.commerce.payment.model.impl.CommercePaymentMethodGroupRelImpl;
import com.liferay.commerce.payment.model.impl.CommercePaymentMethodGroupRelModelImpl;
import com.liferay.commerce.payment.service.persistence.CommercePaymentMethodGroupRelPersistence;
import com.liferay.petra.string.StringBundler;
import com.liferay.portal.kernel.dao.orm.ArgumentsResolver;
import com.liferay.portal.kernel.dao.orm.EntityCache;
import com.liferay.portal.kernel.dao.orm.FinderCache;
import com.liferay.portal.kernel.dao.orm.FinderPath;
import com.liferay.portal.kernel.dao.orm.Query;
import com.liferay.portal.kernel.dao.orm.QueryPos;
import com.liferay.portal.kernel.dao.orm.QueryUtil;
import com.liferay.portal.kernel.dao.orm.Session;
import com.liferay.portal.kernel.log.Log;
import com.liferay.portal.kernel.log.LogFactoryUtil;
import com.liferay.portal.kernel.model.BaseModel;
import com.liferay.portal.kernel.security.auth.CompanyThreadLocal;
import com.liferay.portal.kernel.service.ServiceContext;
import com.liferay.portal.kernel.service.ServiceContextThreadLocal;
import com.liferay.portal.kernel.service.persistence.impl.BasePersistenceImpl;
import com.liferay.portal.kernel.util.HashMapDictionary;
import com.liferay.portal.kernel.util.OrderByComparator;
import com.liferay.portal.kernel.util.ProxyUtil;
import com.liferay.portal.kernel.util.SetUtil;
import com.liferay.portal.spring.extender.service.ServiceReference;
import java.io.Serializable;
import java.lang.reflect.InvocationHandler;
import java.util.Date;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Set;
import java.util.concurrent.ConcurrentHashMap;
import org.osgi.framework.Bundle;
import org.osgi.framework.BundleContext;
import org.osgi.framework.FrameworkUtil;
import org.osgi.framework.ServiceRegistration;
/**
* The persistence implementation for the commerce payment method group rel service.
*
*
* Caching information and settings can be found in portal.properties
*
*
* @author Luca Pellizzon
* @generated
*/
public class CommercePaymentMethodGroupRelPersistenceImpl
extends BasePersistenceImpl
implements CommercePaymentMethodGroupRelPersistence {
/*
* NOTE FOR DEVELOPERS:
*
* Never modify or reference this class directly. Always use CommercePaymentMethodGroupRelUtil
to access the commerce payment method group rel persistence. Modify service.xml
and rerun ServiceBuilder to regenerate this class.
*/
public static final String FINDER_CLASS_NAME_ENTITY =
CommercePaymentMethodGroupRelImpl.class.getName();
public static final String FINDER_CLASS_NAME_LIST_WITH_PAGINATION =
FINDER_CLASS_NAME_ENTITY + ".List1";
public static final String FINDER_CLASS_NAME_LIST_WITHOUT_PAGINATION =
FINDER_CLASS_NAME_ENTITY + ".List2";
private FinderPath _finderPathWithPaginationFindAll;
private FinderPath _finderPathWithoutPaginationFindAll;
private FinderPath _finderPathCountAll;
private FinderPath _finderPathWithPaginationFindByGroupId;
private FinderPath _finderPathWithoutPaginationFindByGroupId;
private FinderPath _finderPathCountByGroupId;
/**
* Returns all the commerce payment method group rels where groupId = ?.
*
* @param groupId the group ID
* @return the matching commerce payment method group rels
*/
@Override
public List findByGroupId(long groupId) {
return findByGroupId(
groupId, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the commerce payment method group rels where groupId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from CommercePaymentMethodGroupRelModelImpl
.
*
*
* @param groupId the group ID
* @param start the lower bound of the range of commerce payment method group rels
* @param end the upper bound of the range of commerce payment method group rels (not inclusive)
* @return the range of matching commerce payment method group rels
*/
@Override
public List findByGroupId(
long groupId, int start, int end) {
return findByGroupId(groupId, start, end, null);
}
/**
* Returns an ordered range of all the commerce payment method group rels where groupId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from CommercePaymentMethodGroupRelModelImpl
.
*
*
* @param groupId the group ID
* @param start the lower bound of the range of commerce payment method group rels
* @param end the upper bound of the range of commerce payment method group rels (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching commerce payment method group rels
*/
@Override
public List findByGroupId(
long groupId, int start, int end,
OrderByComparator orderByComparator) {
return findByGroupId(groupId, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the commerce payment method group rels where groupId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from CommercePaymentMethodGroupRelModelImpl
.
*
*
* @param groupId the group ID
* @param start the lower bound of the range of commerce payment method group rels
* @param end the upper bound of the range of commerce payment method group rels (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching commerce payment method group rels
*/
@Override
public List findByGroupId(
long groupId, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache) {
finderPath = _finderPathWithoutPaginationFindByGroupId;
finderArgs = new Object[] {groupId};
}
}
else if (useFinderCache) {
finderPath = _finderPathWithPaginationFindByGroupId;
finderArgs = new Object[] {groupId, start, end, orderByComparator};
}
List list = null;
if (useFinderCache) {
list = (List)finderCache.getResult(
finderPath, finderArgs);
if ((list != null) && !list.isEmpty()) {
for (CommercePaymentMethodGroupRel
commercePaymentMethodGroupRel : list) {
if (groupId != commercePaymentMethodGroupRel.getGroupId()) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
3 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(3);
}
sb.append(_SQL_SELECT_COMMERCEPAYMENTMETHODGROUPREL_WHERE);
sb.append(_FINDER_COLUMN_GROUPID_GROUPID_2);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(CommercePaymentMethodGroupRelModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first commerce payment method group rel in the ordered set where groupId = ?.
*
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching commerce payment method group rel
* @throws NoSuchPaymentMethodGroupRelException if a matching commerce payment method group rel could not be found
*/
@Override
public CommercePaymentMethodGroupRel findByGroupId_First(
long groupId,
OrderByComparator orderByComparator)
throws NoSuchPaymentMethodGroupRelException {
CommercePaymentMethodGroupRel commercePaymentMethodGroupRel =
fetchByGroupId_First(groupId, orderByComparator);
if (commercePaymentMethodGroupRel != null) {
return commercePaymentMethodGroupRel;
}
StringBundler sb = new StringBundler(4);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("groupId=");
sb.append(groupId);
sb.append("}");
throw new NoSuchPaymentMethodGroupRelException(sb.toString());
}
/**
* Returns the first commerce payment method group rel in the ordered set where groupId = ?.
*
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching commerce payment method group rel, or null
if a matching commerce payment method group rel could not be found
*/
@Override
public CommercePaymentMethodGroupRel fetchByGroupId_First(
long groupId,
OrderByComparator orderByComparator) {
List list = findByGroupId(
groupId, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last commerce payment method group rel in the ordered set where groupId = ?.
*
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching commerce payment method group rel
* @throws NoSuchPaymentMethodGroupRelException if a matching commerce payment method group rel could not be found
*/
@Override
public CommercePaymentMethodGroupRel findByGroupId_Last(
long groupId,
OrderByComparator orderByComparator)
throws NoSuchPaymentMethodGroupRelException {
CommercePaymentMethodGroupRel commercePaymentMethodGroupRel =
fetchByGroupId_Last(groupId, orderByComparator);
if (commercePaymentMethodGroupRel != null) {
return commercePaymentMethodGroupRel;
}
StringBundler sb = new StringBundler(4);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("groupId=");
sb.append(groupId);
sb.append("}");
throw new NoSuchPaymentMethodGroupRelException(sb.toString());
}
/**
* Returns the last commerce payment method group rel in the ordered set where groupId = ?.
*
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching commerce payment method group rel, or null
if a matching commerce payment method group rel could not be found
*/
@Override
public CommercePaymentMethodGroupRel fetchByGroupId_Last(
long groupId,
OrderByComparator orderByComparator) {
int count = countByGroupId(groupId);
if (count == 0) {
return null;
}
List list = findByGroupId(
groupId, count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the commerce payment method group rels before and after the current commerce payment method group rel in the ordered set where groupId = ?.
*
* @param commercePaymentMethodGroupRelId the primary key of the current commerce payment method group rel
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next commerce payment method group rel
* @throws NoSuchPaymentMethodGroupRelException if a commerce payment method group rel with the primary key could not be found
*/
@Override
public CommercePaymentMethodGroupRel[] findByGroupId_PrevAndNext(
long commercePaymentMethodGroupRelId, long groupId,
OrderByComparator orderByComparator)
throws NoSuchPaymentMethodGroupRelException {
CommercePaymentMethodGroupRel commercePaymentMethodGroupRel =
findByPrimaryKey(commercePaymentMethodGroupRelId);
Session session = null;
try {
session = openSession();
CommercePaymentMethodGroupRel[] array =
new CommercePaymentMethodGroupRelImpl[3];
array[0] = getByGroupId_PrevAndNext(
session, commercePaymentMethodGroupRel, groupId,
orderByComparator, true);
array[1] = commercePaymentMethodGroupRel;
array[2] = getByGroupId_PrevAndNext(
session, commercePaymentMethodGroupRel, groupId,
orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected CommercePaymentMethodGroupRel getByGroupId_PrevAndNext(
Session session,
CommercePaymentMethodGroupRel commercePaymentMethodGroupRel,
long groupId,
OrderByComparator orderByComparator,
boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(3);
}
sb.append(_SQL_SELECT_COMMERCEPAYMENTMETHODGROUPREL_WHERE);
sb.append(_FINDER_COLUMN_GROUPID_GROUPID_2);
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(CommercePaymentMethodGroupRelModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
commercePaymentMethodGroupRel)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the commerce payment method group rels where groupId = ? from the database.
*
* @param groupId the group ID
*/
@Override
public void removeByGroupId(long groupId) {
for (CommercePaymentMethodGroupRel commercePaymentMethodGroupRel :
findByGroupId(
groupId, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null)) {
remove(commercePaymentMethodGroupRel);
}
}
/**
* Returns the number of commerce payment method group rels where groupId = ?.
*
* @param groupId the group ID
* @return the number of matching commerce payment method group rels
*/
@Override
public int countByGroupId(long groupId) {
FinderPath finderPath = _finderPathCountByGroupId;
Object[] finderArgs = new Object[] {groupId};
Long count = (Long)finderCache.getResult(finderPath, finderArgs);
if (count == null) {
StringBundler sb = new StringBundler(2);
sb.append(_SQL_COUNT_COMMERCEPAYMENTMETHODGROUPREL_WHERE);
sb.append(_FINDER_COLUMN_GROUPID_GROUPID_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
count = (Long)query.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String _FINDER_COLUMN_GROUPID_GROUPID_2 =
"commercePaymentMethodGroupRel.groupId = ?";
private FinderPath _finderPathFetchByG_E;
private FinderPath _finderPathCountByG_E;
/**
* Returns the commerce payment method group rel where groupId = ? and engineKey = ? or throws a NoSuchPaymentMethodGroupRelException
if it could not be found.
*
* @param groupId the group ID
* @param engineKey the engine key
* @return the matching commerce payment method group rel
* @throws NoSuchPaymentMethodGroupRelException if a matching commerce payment method group rel could not be found
*/
@Override
public CommercePaymentMethodGroupRel findByG_E(
long groupId, String engineKey)
throws NoSuchPaymentMethodGroupRelException {
CommercePaymentMethodGroupRel commercePaymentMethodGroupRel =
fetchByG_E(groupId, engineKey);
if (commercePaymentMethodGroupRel == null) {
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("groupId=");
sb.append(groupId);
sb.append(", engineKey=");
sb.append(engineKey);
sb.append("}");
if (_log.isDebugEnabled()) {
_log.debug(sb.toString());
}
throw new NoSuchPaymentMethodGroupRelException(sb.toString());
}
return commercePaymentMethodGroupRel;
}
/**
* Returns the commerce payment method group rel where groupId = ? and engineKey = ? or returns null
if it could not be found. Uses the finder cache.
*
* @param groupId the group ID
* @param engineKey the engine key
* @return the matching commerce payment method group rel, or null
if a matching commerce payment method group rel could not be found
*/
@Override
public CommercePaymentMethodGroupRel fetchByG_E(
long groupId, String engineKey) {
return fetchByG_E(groupId, engineKey, true);
}
/**
* Returns the commerce payment method group rel where groupId = ? and engineKey = ? or returns null
if it could not be found, optionally using the finder cache.
*
* @param groupId the group ID
* @param engineKey the engine key
* @param useFinderCache whether to use the finder cache
* @return the matching commerce payment method group rel, or null
if a matching commerce payment method group rel could not be found
*/
@Override
public CommercePaymentMethodGroupRel fetchByG_E(
long groupId, String engineKey, boolean useFinderCache) {
engineKey = Objects.toString(engineKey, "");
Object[] finderArgs = null;
if (useFinderCache) {
finderArgs = new Object[] {groupId, engineKey};
}
Object result = null;
if (useFinderCache) {
result = finderCache.getResult(_finderPathFetchByG_E, finderArgs);
}
if (result instanceof CommercePaymentMethodGroupRel) {
CommercePaymentMethodGroupRel commercePaymentMethodGroupRel =
(CommercePaymentMethodGroupRel)result;
if ((groupId != commercePaymentMethodGroupRel.getGroupId()) ||
!Objects.equals(
engineKey, commercePaymentMethodGroupRel.getEngineKey())) {
result = null;
}
}
if (result == null) {
StringBundler sb = new StringBundler(4);
sb.append(_SQL_SELECT_COMMERCEPAYMENTMETHODGROUPREL_WHERE);
sb.append(_FINDER_COLUMN_G_E_GROUPID_2);
boolean bindEngineKey = false;
if (engineKey.isEmpty()) {
sb.append(_FINDER_COLUMN_G_E_ENGINEKEY_3);
}
else {
bindEngineKey = true;
sb.append(_FINDER_COLUMN_G_E_ENGINEKEY_2);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
if (bindEngineKey) {
queryPos.add(engineKey);
}
List list = query.list();
if (list.isEmpty()) {
if (useFinderCache) {
finderCache.putResult(
_finderPathFetchByG_E, finderArgs, list);
}
}
else {
CommercePaymentMethodGroupRel
commercePaymentMethodGroupRel = list.get(0);
result = commercePaymentMethodGroupRel;
cacheResult(commercePaymentMethodGroupRel);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
if (result instanceof List>) {
return null;
}
else {
return (CommercePaymentMethodGroupRel)result;
}
}
/**
* Removes the commerce payment method group rel where groupId = ? and engineKey = ? from the database.
*
* @param groupId the group ID
* @param engineKey the engine key
* @return the commerce payment method group rel that was removed
*/
@Override
public CommercePaymentMethodGroupRel removeByG_E(
long groupId, String engineKey)
throws NoSuchPaymentMethodGroupRelException {
CommercePaymentMethodGroupRel commercePaymentMethodGroupRel = findByG_E(
groupId, engineKey);
return remove(commercePaymentMethodGroupRel);
}
/**
* Returns the number of commerce payment method group rels where groupId = ? and engineKey = ?.
*
* @param groupId the group ID
* @param engineKey the engine key
* @return the number of matching commerce payment method group rels
*/
@Override
public int countByG_E(long groupId, String engineKey) {
engineKey = Objects.toString(engineKey, "");
FinderPath finderPath = _finderPathCountByG_E;
Object[] finderArgs = new Object[] {groupId, engineKey};
Long count = (Long)finderCache.getResult(finderPath, finderArgs);
if (count == null) {
StringBundler sb = new StringBundler(3);
sb.append(_SQL_COUNT_COMMERCEPAYMENTMETHODGROUPREL_WHERE);
sb.append(_FINDER_COLUMN_G_E_GROUPID_2);
boolean bindEngineKey = false;
if (engineKey.isEmpty()) {
sb.append(_FINDER_COLUMN_G_E_ENGINEKEY_3);
}
else {
bindEngineKey = true;
sb.append(_FINDER_COLUMN_G_E_ENGINEKEY_2);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
if (bindEngineKey) {
queryPos.add(engineKey);
}
count = (Long)query.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String _FINDER_COLUMN_G_E_GROUPID_2 =
"commercePaymentMethodGroupRel.groupId = ? AND ";
private static final String _FINDER_COLUMN_G_E_ENGINEKEY_2 =
"commercePaymentMethodGroupRel.engineKey = ?";
private static final String _FINDER_COLUMN_G_E_ENGINEKEY_3 =
"(commercePaymentMethodGroupRel.engineKey IS NULL OR commercePaymentMethodGroupRel.engineKey = '')";
private FinderPath _finderPathWithPaginationFindByG_A;
private FinderPath _finderPathWithoutPaginationFindByG_A;
private FinderPath _finderPathCountByG_A;
/**
* Returns all the commerce payment method group rels where groupId = ? and active = ?.
*
* @param groupId the group ID
* @param active the active
* @return the matching commerce payment method group rels
*/
@Override
public List findByG_A(
long groupId, boolean active) {
return findByG_A(
groupId, active, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the commerce payment method group rels where groupId = ? and active = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from CommercePaymentMethodGroupRelModelImpl
.
*
*
* @param groupId the group ID
* @param active the active
* @param start the lower bound of the range of commerce payment method group rels
* @param end the upper bound of the range of commerce payment method group rels (not inclusive)
* @return the range of matching commerce payment method group rels
*/
@Override
public List findByG_A(
long groupId, boolean active, int start, int end) {
return findByG_A(groupId, active, start, end, null);
}
/**
* Returns an ordered range of all the commerce payment method group rels where groupId = ? and active = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from CommercePaymentMethodGroupRelModelImpl
.
*
*
* @param groupId the group ID
* @param active the active
* @param start the lower bound of the range of commerce payment method group rels
* @param end the upper bound of the range of commerce payment method group rels (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching commerce payment method group rels
*/
@Override
public List findByG_A(
long groupId, boolean active, int start, int end,
OrderByComparator orderByComparator) {
return findByG_A(groupId, active, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the commerce payment method group rels where groupId = ? and active = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from CommercePaymentMethodGroupRelModelImpl
.
*
*
* @param groupId the group ID
* @param active the active
* @param start the lower bound of the range of commerce payment method group rels
* @param end the upper bound of the range of commerce payment method group rels (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching commerce payment method group rels
*/
@Override
public List findByG_A(
long groupId, boolean active, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache) {
finderPath = _finderPathWithoutPaginationFindByG_A;
finderArgs = new Object[] {groupId, active};
}
}
else if (useFinderCache) {
finderPath = _finderPathWithPaginationFindByG_A;
finderArgs = new Object[] {
groupId, active, start, end, orderByComparator
};
}
List list = null;
if (useFinderCache) {
list = (List)finderCache.getResult(
finderPath, finderArgs);
if ((list != null) && !list.isEmpty()) {
for (CommercePaymentMethodGroupRel
commercePaymentMethodGroupRel : list) {
if ((groupId !=
commercePaymentMethodGroupRel.getGroupId()) ||
(active != commercePaymentMethodGroupRel.isActive())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_COMMERCEPAYMENTMETHODGROUPREL_WHERE);
sb.append(_FINDER_COLUMN_G_A_GROUPID_2);
sb.append(_FINDER_COLUMN_G_A_ACTIVE_2);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(CommercePaymentMethodGroupRelModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
queryPos.add(active);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first commerce payment method group rel in the ordered set where groupId = ? and active = ?.
*
* @param groupId the group ID
* @param active the active
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching commerce payment method group rel
* @throws NoSuchPaymentMethodGroupRelException if a matching commerce payment method group rel could not be found
*/
@Override
public CommercePaymentMethodGroupRel findByG_A_First(
long groupId, boolean active,
OrderByComparator orderByComparator)
throws NoSuchPaymentMethodGroupRelException {
CommercePaymentMethodGroupRel commercePaymentMethodGroupRel =
fetchByG_A_First(groupId, active, orderByComparator);
if (commercePaymentMethodGroupRel != null) {
return commercePaymentMethodGroupRel;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("groupId=");
sb.append(groupId);
sb.append(", active=");
sb.append(active);
sb.append("}");
throw new NoSuchPaymentMethodGroupRelException(sb.toString());
}
/**
* Returns the first commerce payment method group rel in the ordered set where groupId = ? and active = ?.
*
* @param groupId the group ID
* @param active the active
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching commerce payment method group rel, or null
if a matching commerce payment method group rel could not be found
*/
@Override
public CommercePaymentMethodGroupRel fetchByG_A_First(
long groupId, boolean active,
OrderByComparator orderByComparator) {
List list = findByG_A(
groupId, active, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last commerce payment method group rel in the ordered set where groupId = ? and active = ?.
*
* @param groupId the group ID
* @param active the active
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching commerce payment method group rel
* @throws NoSuchPaymentMethodGroupRelException if a matching commerce payment method group rel could not be found
*/
@Override
public CommercePaymentMethodGroupRel findByG_A_Last(
long groupId, boolean active,
OrderByComparator orderByComparator)
throws NoSuchPaymentMethodGroupRelException {
CommercePaymentMethodGroupRel commercePaymentMethodGroupRel =
fetchByG_A_Last(groupId, active, orderByComparator);
if (commercePaymentMethodGroupRel != null) {
return commercePaymentMethodGroupRel;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("groupId=");
sb.append(groupId);
sb.append(", active=");
sb.append(active);
sb.append("}");
throw new NoSuchPaymentMethodGroupRelException(sb.toString());
}
/**
* Returns the last commerce payment method group rel in the ordered set where groupId = ? and active = ?.
*
* @param groupId the group ID
* @param active the active
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching commerce payment method group rel, or null
if a matching commerce payment method group rel could not be found
*/
@Override
public CommercePaymentMethodGroupRel fetchByG_A_Last(
long groupId, boolean active,
OrderByComparator orderByComparator) {
int count = countByG_A(groupId, active);
if (count == 0) {
return null;
}
List list = findByG_A(
groupId, active, count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the commerce payment method group rels before and after the current commerce payment method group rel in the ordered set where groupId = ? and active = ?.
*
* @param commercePaymentMethodGroupRelId the primary key of the current commerce payment method group rel
* @param groupId the group ID
* @param active the active
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next commerce payment method group rel
* @throws NoSuchPaymentMethodGroupRelException if a commerce payment method group rel with the primary key could not be found
*/
@Override
public CommercePaymentMethodGroupRel[] findByG_A_PrevAndNext(
long commercePaymentMethodGroupRelId, long groupId, boolean active,
OrderByComparator orderByComparator)
throws NoSuchPaymentMethodGroupRelException {
CommercePaymentMethodGroupRel commercePaymentMethodGroupRel =
findByPrimaryKey(commercePaymentMethodGroupRelId);
Session session = null;
try {
session = openSession();
CommercePaymentMethodGroupRel[] array =
new CommercePaymentMethodGroupRelImpl[3];
array[0] = getByG_A_PrevAndNext(
session, commercePaymentMethodGroupRel, groupId, active,
orderByComparator, true);
array[1] = commercePaymentMethodGroupRel;
array[2] = getByG_A_PrevAndNext(
session, commercePaymentMethodGroupRel, groupId, active,
orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected CommercePaymentMethodGroupRel getByG_A_PrevAndNext(
Session session,
CommercePaymentMethodGroupRel commercePaymentMethodGroupRel,
long groupId, boolean active,
OrderByComparator orderByComparator,
boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
5 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_COMMERCEPAYMENTMETHODGROUPREL_WHERE);
sb.append(_FINDER_COLUMN_G_A_GROUPID_2);
sb.append(_FINDER_COLUMN_G_A_ACTIVE_2);
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(CommercePaymentMethodGroupRelModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
queryPos.add(active);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
commercePaymentMethodGroupRel)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the commerce payment method group rels where groupId = ? and active = ? from the database.
*
* @param groupId the group ID
* @param active the active
*/
@Override
public void removeByG_A(long groupId, boolean active) {
for (CommercePaymentMethodGroupRel commercePaymentMethodGroupRel :
findByG_A(
groupId, active, QueryUtil.ALL_POS, QueryUtil.ALL_POS,
null)) {
remove(commercePaymentMethodGroupRel);
}
}
/**
* Returns the number of commerce payment method group rels where groupId = ? and active = ?.
*
* @param groupId the group ID
* @param active the active
* @return the number of matching commerce payment method group rels
*/
@Override
public int countByG_A(long groupId, boolean active) {
FinderPath finderPath = _finderPathCountByG_A;
Object[] finderArgs = new Object[] {groupId, active};
Long count = (Long)finderCache.getResult(finderPath, finderArgs);
if (count == null) {
StringBundler sb = new StringBundler(3);
sb.append(_SQL_COUNT_COMMERCEPAYMENTMETHODGROUPREL_WHERE);
sb.append(_FINDER_COLUMN_G_A_GROUPID_2);
sb.append(_FINDER_COLUMN_G_A_ACTIVE_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
queryPos.add(active);
count = (Long)query.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String _FINDER_COLUMN_G_A_GROUPID_2 =
"commercePaymentMethodGroupRel.groupId = ? AND ";
private static final String _FINDER_COLUMN_G_A_ACTIVE_2 =
"commercePaymentMethodGroupRel.active = ?";
public CommercePaymentMethodGroupRelPersistenceImpl() {
Map dbColumnNames = new HashMap();
dbColumnNames.put(
"commercePaymentMethodGroupRelId", "CPaymentMethodGroupRelId");
dbColumnNames.put("active", "active_");
setDBColumnNames(dbColumnNames);
setModelClass(CommercePaymentMethodGroupRel.class);
setModelImplClass(CommercePaymentMethodGroupRelImpl.class);
setModelPKClass(long.class);
setTable(CommercePaymentMethodGroupRelTable.INSTANCE);
}
/**
* Caches the commerce payment method group rel in the entity cache if it is enabled.
*
* @param commercePaymentMethodGroupRel the commerce payment method group rel
*/
@Override
public void cacheResult(
CommercePaymentMethodGroupRel commercePaymentMethodGroupRel) {
entityCache.putResult(
CommercePaymentMethodGroupRelImpl.class,
commercePaymentMethodGroupRel.getPrimaryKey(),
commercePaymentMethodGroupRel);
finderCache.putResult(
_finderPathFetchByG_E,
new Object[] {
commercePaymentMethodGroupRel.getGroupId(),
commercePaymentMethodGroupRel.getEngineKey()
},
commercePaymentMethodGroupRel);
}
/**
* Caches the commerce payment method group rels in the entity cache if it is enabled.
*
* @param commercePaymentMethodGroupRels the commerce payment method group rels
*/
@Override
public void cacheResult(
List commercePaymentMethodGroupRels) {
for (CommercePaymentMethodGroupRel commercePaymentMethodGroupRel :
commercePaymentMethodGroupRels) {
if (entityCache.getResult(
CommercePaymentMethodGroupRelImpl.class,
commercePaymentMethodGroupRel.getPrimaryKey()) == null) {
cacheResult(commercePaymentMethodGroupRel);
}
}
}
/**
* Clears the cache for all commerce payment method group rels.
*
*
* The EntityCache
and FinderCache
are both cleared by this method.
*
*/
@Override
public void clearCache() {
entityCache.clearCache(CommercePaymentMethodGroupRelImpl.class);
finderCache.clearCache(CommercePaymentMethodGroupRelImpl.class);
}
/**
* Clears the cache for the commerce payment method group rel.
*
*
* The EntityCache
and FinderCache
are both cleared by this method.
*
*/
@Override
public void clearCache(
CommercePaymentMethodGroupRel commercePaymentMethodGroupRel) {
entityCache.removeResult(
CommercePaymentMethodGroupRelImpl.class,
commercePaymentMethodGroupRel);
}
@Override
public void clearCache(
List commercePaymentMethodGroupRels) {
for (CommercePaymentMethodGroupRel commercePaymentMethodGroupRel :
commercePaymentMethodGroupRels) {
entityCache.removeResult(
CommercePaymentMethodGroupRelImpl.class,
commercePaymentMethodGroupRel);
}
}
@Override
public void clearCache(Set primaryKeys) {
finderCache.clearCache(CommercePaymentMethodGroupRelImpl.class);
for (Serializable primaryKey : primaryKeys) {
entityCache.removeResult(
CommercePaymentMethodGroupRelImpl.class, primaryKey);
}
}
protected void cacheUniqueFindersCache(
CommercePaymentMethodGroupRelModelImpl
commercePaymentMethodGroupRelModelImpl) {
Object[] args = new Object[] {
commercePaymentMethodGroupRelModelImpl.getGroupId(),
commercePaymentMethodGroupRelModelImpl.getEngineKey()
};
finderCache.putResult(_finderPathCountByG_E, args, Long.valueOf(1));
finderCache.putResult(
_finderPathFetchByG_E, args,
commercePaymentMethodGroupRelModelImpl);
}
/**
* Creates a new commerce payment method group rel with the primary key. Does not add the commerce payment method group rel to the database.
*
* @param commercePaymentMethodGroupRelId the primary key for the new commerce payment method group rel
* @return the new commerce payment method group rel
*/
@Override
public CommercePaymentMethodGroupRel create(
long commercePaymentMethodGroupRelId) {
CommercePaymentMethodGroupRel commercePaymentMethodGroupRel =
new CommercePaymentMethodGroupRelImpl();
commercePaymentMethodGroupRel.setNew(true);
commercePaymentMethodGroupRel.setPrimaryKey(
commercePaymentMethodGroupRelId);
commercePaymentMethodGroupRel.setCompanyId(
CompanyThreadLocal.getCompanyId());
return commercePaymentMethodGroupRel;
}
/**
* Removes the commerce payment method group rel with the primary key from the database. Also notifies the appropriate model listeners.
*
* @param commercePaymentMethodGroupRelId the primary key of the commerce payment method group rel
* @return the commerce payment method group rel that was removed
* @throws NoSuchPaymentMethodGroupRelException if a commerce payment method group rel with the primary key could not be found
*/
@Override
public CommercePaymentMethodGroupRel remove(
long commercePaymentMethodGroupRelId)
throws NoSuchPaymentMethodGroupRelException {
return remove((Serializable)commercePaymentMethodGroupRelId);
}
/**
* Removes the commerce payment method group rel with the primary key from the database. Also notifies the appropriate model listeners.
*
* @param primaryKey the primary key of the commerce payment method group rel
* @return the commerce payment method group rel that was removed
* @throws NoSuchPaymentMethodGroupRelException if a commerce payment method group rel with the primary key could not be found
*/
@Override
public CommercePaymentMethodGroupRel remove(Serializable primaryKey)
throws NoSuchPaymentMethodGroupRelException {
Session session = null;
try {
session = openSession();
CommercePaymentMethodGroupRel commercePaymentMethodGroupRel =
(CommercePaymentMethodGroupRel)session.get(
CommercePaymentMethodGroupRelImpl.class, primaryKey);
if (commercePaymentMethodGroupRel == null) {
if (_log.isDebugEnabled()) {
_log.debug(_NO_SUCH_ENTITY_WITH_PRIMARY_KEY + primaryKey);
}
throw new NoSuchPaymentMethodGroupRelException(
_NO_SUCH_ENTITY_WITH_PRIMARY_KEY + primaryKey);
}
return remove(commercePaymentMethodGroupRel);
}
catch (NoSuchPaymentMethodGroupRelException noSuchEntityException) {
throw noSuchEntityException;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
@Override
protected CommercePaymentMethodGroupRel removeImpl(
CommercePaymentMethodGroupRel commercePaymentMethodGroupRel) {
Session session = null;
try {
session = openSession();
if (!session.contains(commercePaymentMethodGroupRel)) {
commercePaymentMethodGroupRel =
(CommercePaymentMethodGroupRel)session.get(
CommercePaymentMethodGroupRelImpl.class,
commercePaymentMethodGroupRel.getPrimaryKeyObj());
}
if (commercePaymentMethodGroupRel != null) {
session.delete(commercePaymentMethodGroupRel);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
if (commercePaymentMethodGroupRel != null) {
clearCache(commercePaymentMethodGroupRel);
}
return commercePaymentMethodGroupRel;
}
@Override
public CommercePaymentMethodGroupRel updateImpl(
CommercePaymentMethodGroupRel commercePaymentMethodGroupRel) {
boolean isNew = commercePaymentMethodGroupRel.isNew();
if (!(commercePaymentMethodGroupRel instanceof
CommercePaymentMethodGroupRelModelImpl)) {
InvocationHandler invocationHandler = null;
if (ProxyUtil.isProxyClass(
commercePaymentMethodGroupRel.getClass())) {
invocationHandler = ProxyUtil.getInvocationHandler(
commercePaymentMethodGroupRel);
throw new IllegalArgumentException(
"Implement ModelWrapper in commercePaymentMethodGroupRel proxy " +
invocationHandler.getClass());
}
throw new IllegalArgumentException(
"Implement ModelWrapper in custom CommercePaymentMethodGroupRel implementation " +
commercePaymentMethodGroupRel.getClass());
}
CommercePaymentMethodGroupRelModelImpl
commercePaymentMethodGroupRelModelImpl =
(CommercePaymentMethodGroupRelModelImpl)
commercePaymentMethodGroupRel;
ServiceContext serviceContext =
ServiceContextThreadLocal.getServiceContext();
Date now = new Date();
if (isNew && (commercePaymentMethodGroupRel.getCreateDate() == null)) {
if (serviceContext == null) {
commercePaymentMethodGroupRel.setCreateDate(now);
}
else {
commercePaymentMethodGroupRel.setCreateDate(
serviceContext.getCreateDate(now));
}
}
if (!commercePaymentMethodGroupRelModelImpl.hasSetModifiedDate()) {
if (serviceContext == null) {
commercePaymentMethodGroupRel.setModifiedDate(now);
}
else {
commercePaymentMethodGroupRel.setModifiedDate(
serviceContext.getModifiedDate(now));
}
}
Session session = null;
try {
session = openSession();
if (isNew) {
session.save(commercePaymentMethodGroupRel);
}
else {
commercePaymentMethodGroupRel =
(CommercePaymentMethodGroupRel)session.merge(
commercePaymentMethodGroupRel);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
entityCache.putResult(
CommercePaymentMethodGroupRelImpl.class,
commercePaymentMethodGroupRelModelImpl, false, true);
cacheUniqueFindersCache(commercePaymentMethodGroupRelModelImpl);
if (isNew) {
commercePaymentMethodGroupRel.setNew(false);
}
commercePaymentMethodGroupRel.resetOriginalValues();
return commercePaymentMethodGroupRel;
}
/**
* Returns the commerce payment method group rel with the primary key or throws a com.liferay.portal.kernel.exception.NoSuchModelException
if it could not be found.
*
* @param primaryKey the primary key of the commerce payment method group rel
* @return the commerce payment method group rel
* @throws NoSuchPaymentMethodGroupRelException if a commerce payment method group rel with the primary key could not be found
*/
@Override
public CommercePaymentMethodGroupRel findByPrimaryKey(
Serializable primaryKey)
throws NoSuchPaymentMethodGroupRelException {
CommercePaymentMethodGroupRel commercePaymentMethodGroupRel =
fetchByPrimaryKey(primaryKey);
if (commercePaymentMethodGroupRel == null) {
if (_log.isDebugEnabled()) {
_log.debug(_NO_SUCH_ENTITY_WITH_PRIMARY_KEY + primaryKey);
}
throw new NoSuchPaymentMethodGroupRelException(
_NO_SUCH_ENTITY_WITH_PRIMARY_KEY + primaryKey);
}
return commercePaymentMethodGroupRel;
}
/**
* Returns the commerce payment method group rel with the primary key or throws a NoSuchPaymentMethodGroupRelException
if it could not be found.
*
* @param commercePaymentMethodGroupRelId the primary key of the commerce payment method group rel
* @return the commerce payment method group rel
* @throws NoSuchPaymentMethodGroupRelException if a commerce payment method group rel with the primary key could not be found
*/
@Override
public CommercePaymentMethodGroupRel findByPrimaryKey(
long commercePaymentMethodGroupRelId)
throws NoSuchPaymentMethodGroupRelException {
return findByPrimaryKey((Serializable)commercePaymentMethodGroupRelId);
}
/**
* Returns the commerce payment method group rel with the primary key or returns null
if it could not be found.
*
* @param commercePaymentMethodGroupRelId the primary key of the commerce payment method group rel
* @return the commerce payment method group rel, or null
if a commerce payment method group rel with the primary key could not be found
*/
@Override
public CommercePaymentMethodGroupRel fetchByPrimaryKey(
long commercePaymentMethodGroupRelId) {
return fetchByPrimaryKey((Serializable)commercePaymentMethodGroupRelId);
}
/**
* Returns all the commerce payment method group rels.
*
* @return the commerce payment method group rels
*/
@Override
public List findAll() {
return findAll(QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the commerce payment method group rels.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from CommercePaymentMethodGroupRelModelImpl
.
*
*
* @param start the lower bound of the range of commerce payment method group rels
* @param end the upper bound of the range of commerce payment method group rels (not inclusive)
* @return the range of commerce payment method group rels
*/
@Override
public List findAll(int start, int end) {
return findAll(start, end, null);
}
/**
* Returns an ordered range of all the commerce payment method group rels.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from CommercePaymentMethodGroupRelModelImpl
.
*
*
* @param start the lower bound of the range of commerce payment method group rels
* @param end the upper bound of the range of commerce payment method group rels (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of commerce payment method group rels
*/
@Override
public List findAll(
int start, int end,
OrderByComparator orderByComparator) {
return findAll(start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the commerce payment method group rels.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from CommercePaymentMethodGroupRelModelImpl
.
*
*
* @param start the lower bound of the range of commerce payment method group rels
* @param end the upper bound of the range of commerce payment method group rels (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of commerce payment method group rels
*/
@Override
public List findAll(
int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache) {
finderPath = _finderPathWithoutPaginationFindAll;
finderArgs = FINDER_ARGS_EMPTY;
}
}
else if (useFinderCache) {
finderPath = _finderPathWithPaginationFindAll;
finderArgs = new Object[] {start, end, orderByComparator};
}
List list = null;
if (useFinderCache) {
list = (List)finderCache.getResult(
finderPath, finderArgs);
}
if (list == null) {
StringBundler sb = null;
String sql = null;
if (orderByComparator != null) {
sb = new StringBundler(
2 + (orderByComparator.getOrderByFields().length * 2));
sb.append(_SQL_SELECT_COMMERCEPAYMENTMETHODGROUPREL);
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
sql = sb.toString();
}
else {
sql = _SQL_SELECT_COMMERCEPAYMENTMETHODGROUPREL;
sql = sql.concat(
CommercePaymentMethodGroupRelModelImpl.ORDER_BY_JPQL);
}
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Removes all the commerce payment method group rels from the database.
*
*/
@Override
public void removeAll() {
for (CommercePaymentMethodGroupRel commercePaymentMethodGroupRel :
findAll()) {
remove(commercePaymentMethodGroupRel);
}
}
/**
* Returns the number of commerce payment method group rels.
*
* @return the number of commerce payment method group rels
*/
@Override
public int countAll() {
Long count = (Long)finderCache.getResult(
_finderPathCountAll, FINDER_ARGS_EMPTY);
if (count == null) {
Session session = null;
try {
session = openSession();
Query query = session.createQuery(
_SQL_COUNT_COMMERCEPAYMENTMETHODGROUPREL);
count = (Long)query.uniqueResult();
finderCache.putResult(
_finderPathCountAll, FINDER_ARGS_EMPTY, count);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
@Override
public Set getBadColumnNames() {
return _badColumnNames;
}
@Override
protected EntityCache getEntityCache() {
return entityCache;
}
@Override
protected String getPKDBName() {
return "CPaymentMethodGroupRelId";
}
@Override
protected String getSelectSQL() {
return _SQL_SELECT_COMMERCEPAYMENTMETHODGROUPREL;
}
@Override
protected Map getTableColumnsMap() {
return CommercePaymentMethodGroupRelModelImpl.TABLE_COLUMNS_MAP;
}
/**
* Initializes the commerce payment method group rel persistence.
*/
public void afterPropertiesSet() {
Bundle bundle = FrameworkUtil.getBundle(
CommercePaymentMethodGroupRelPersistenceImpl.class);
_bundleContext = bundle.getBundleContext();
_argumentsResolverServiceRegistration = _bundleContext.registerService(
ArgumentsResolver.class,
new CommercePaymentMethodGroupRelModelArgumentsResolver(),
new HashMapDictionary<>());
_finderPathWithPaginationFindAll = new FinderPath(
FINDER_CLASS_NAME_LIST_WITH_PAGINATION, "findAll", new String[0],
new String[0], true);
_finderPathWithoutPaginationFindAll = new FinderPath(
FINDER_CLASS_NAME_LIST_WITHOUT_PAGINATION, "findAll", new String[0],
new String[0], true);
_finderPathCountAll = new FinderPath(
FINDER_CLASS_NAME_LIST_WITHOUT_PAGINATION, "countAll",
new String[0], new String[0], false);
_finderPathWithPaginationFindByGroupId = new FinderPath(
FINDER_CLASS_NAME_LIST_WITH_PAGINATION, "findByGroupId",
new String[] {
Long.class.getName(), Integer.class.getName(),
Integer.class.getName(), OrderByComparator.class.getName()
},
new String[] {"groupId"}, true);
_finderPathWithoutPaginationFindByGroupId = new FinderPath(
FINDER_CLASS_NAME_LIST_WITHOUT_PAGINATION, "findByGroupId",
new String[] {Long.class.getName()}, new String[] {"groupId"},
true);
_finderPathCountByGroupId = new FinderPath(
FINDER_CLASS_NAME_LIST_WITHOUT_PAGINATION, "countByGroupId",
new String[] {Long.class.getName()}, new String[] {"groupId"},
false);
_finderPathFetchByG_E = new FinderPath(
FINDER_CLASS_NAME_ENTITY, "fetchByG_E",
new String[] {Long.class.getName(), String.class.getName()},
new String[] {"groupId", "engineKey"}, true);
_finderPathCountByG_E = new FinderPath(
FINDER_CLASS_NAME_LIST_WITHOUT_PAGINATION, "countByG_E",
new String[] {Long.class.getName(), String.class.getName()},
new String[] {"groupId", "engineKey"}, false);
_finderPathWithPaginationFindByG_A = new FinderPath(
FINDER_CLASS_NAME_LIST_WITH_PAGINATION, "findByG_A",
new String[] {
Long.class.getName(), Boolean.class.getName(),
Integer.class.getName(), Integer.class.getName(),
OrderByComparator.class.getName()
},
new String[] {"groupId", "active_"}, true);
_finderPathWithoutPaginationFindByG_A = new FinderPath(
FINDER_CLASS_NAME_LIST_WITHOUT_PAGINATION, "findByG_A",
new String[] {Long.class.getName(), Boolean.class.getName()},
new String[] {"groupId", "active_"}, true);
_finderPathCountByG_A = new FinderPath(
FINDER_CLASS_NAME_LIST_WITHOUT_PAGINATION, "countByG_A",
new String[] {Long.class.getName(), Boolean.class.getName()},
new String[] {"groupId", "active_"}, false);
}
public void destroy() {
entityCache.removeCache(
CommercePaymentMethodGroupRelImpl.class.getName());
_argumentsResolverServiceRegistration.unregister();
}
private BundleContext _bundleContext;
@ServiceReference(type = EntityCache.class)
protected EntityCache entityCache;
@ServiceReference(type = FinderCache.class)
protected FinderCache finderCache;
private static final String _SQL_SELECT_COMMERCEPAYMENTMETHODGROUPREL =
"SELECT commercePaymentMethodGroupRel FROM CommercePaymentMethodGroupRel commercePaymentMethodGroupRel";
private static final String
_SQL_SELECT_COMMERCEPAYMENTMETHODGROUPREL_WHERE =
"SELECT commercePaymentMethodGroupRel FROM CommercePaymentMethodGroupRel commercePaymentMethodGroupRel WHERE ";
private static final String _SQL_COUNT_COMMERCEPAYMENTMETHODGROUPREL =
"SELECT COUNT(commercePaymentMethodGroupRel) FROM CommercePaymentMethodGroupRel commercePaymentMethodGroupRel";
private static final String _SQL_COUNT_COMMERCEPAYMENTMETHODGROUPREL_WHERE =
"SELECT COUNT(commercePaymentMethodGroupRel) FROM CommercePaymentMethodGroupRel commercePaymentMethodGroupRel WHERE ";
private static final String _ORDER_BY_ENTITY_ALIAS =
"commercePaymentMethodGroupRel.";
private static final String _NO_SUCH_ENTITY_WITH_PRIMARY_KEY =
"No CommercePaymentMethodGroupRel exists with the primary key ";
private static final String _NO_SUCH_ENTITY_WITH_KEY =
"No CommercePaymentMethodGroupRel exists with the key {";
private static final Log _log = LogFactoryUtil.getLog(
CommercePaymentMethodGroupRelPersistenceImpl.class);
private static final Set _badColumnNames = SetUtil.fromArray(
new String[] {"commercePaymentMethodGroupRelId", "active"});
@Override
protected FinderCache getFinderCache() {
return finderCache;
}
private ServiceRegistration
_argumentsResolverServiceRegistration;
private static class CommercePaymentMethodGroupRelModelArgumentsResolver
implements ArgumentsResolver {
@Override
public Object[] getArguments(
FinderPath finderPath, BaseModel> baseModel, boolean checkColumn,
boolean original) {
String[] columnNames = finderPath.getColumnNames();
if ((columnNames == null) || (columnNames.length == 0)) {
if (baseModel.isNew()) {
return FINDER_ARGS_EMPTY;
}
return null;
}
CommercePaymentMethodGroupRelModelImpl
commercePaymentMethodGroupRelModelImpl =
(CommercePaymentMethodGroupRelModelImpl)baseModel;
long columnBitmask =
commercePaymentMethodGroupRelModelImpl.getColumnBitmask();
if (!checkColumn || (columnBitmask == 0)) {
return _getValue(
commercePaymentMethodGroupRelModelImpl, columnNames,
original);
}
Long finderPathColumnBitmask = _finderPathColumnBitmasksCache.get(
finderPath);
if (finderPathColumnBitmask == null) {
finderPathColumnBitmask = 0L;
for (String columnName : columnNames) {
finderPathColumnBitmask |=
commercePaymentMethodGroupRelModelImpl.getColumnBitmask(
columnName);
}
_finderPathColumnBitmasksCache.put(
finderPath, finderPathColumnBitmask);
}
if ((columnBitmask & finderPathColumnBitmask) != 0) {
return _getValue(
commercePaymentMethodGroupRelModelImpl, columnNames,
original);
}
return null;
}
@Override
public String getClassName() {
return CommercePaymentMethodGroupRelImpl.class.getName();
}
@Override
public String getTableName() {
return CommercePaymentMethodGroupRelTable.INSTANCE.getTableName();
}
private Object[] _getValue(
CommercePaymentMethodGroupRelModelImpl
commercePaymentMethodGroupRelModelImpl,
String[] columnNames, boolean original) {
Object[] arguments = new Object[columnNames.length];
for (int i = 0; i < arguments.length; i++) {
String columnName = columnNames[i];
if (original) {
arguments[i] =
commercePaymentMethodGroupRelModelImpl.
getColumnOriginalValue(columnName);
}
else {
arguments[i] =
commercePaymentMethodGroupRelModelImpl.getColumnValue(
columnName);
}
}
return arguments;
}
private static Map _finderPathColumnBitmasksCache =
new ConcurrentHashMap<>();
}
}