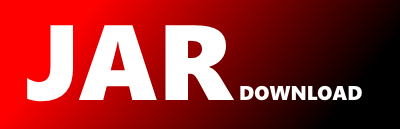
com.liferay.commerce.product.model.impl.CPDefinitionLinkModelImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.liferay.commerce.product.service
Show all versions of com.liferay.commerce.product.service
Liferay Commerce Product Service
/**
* Copyright (c) 2000-present Liferay, Inc. All rights reserved.
*
* This library is free software; you can redistribute it and/or modify it under
* the terms of the GNU Lesser General Public License as published by the Free
* Software Foundation; either version 2.1 of the License, or (at your option)
* any later version.
*
* This library is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License for more
* details.
*/
package com.liferay.commerce.product.model.impl;
import com.liferay.commerce.product.model.CPDefinitionLink;
import com.liferay.commerce.product.model.CPDefinitionLinkModel;
import com.liferay.commerce.product.model.CPDefinitionLinkSoap;
import com.liferay.expando.kernel.model.ExpandoBridge;
import com.liferay.expando.kernel.util.ExpandoBridgeFactoryUtil;
import com.liferay.exportimport.kernel.lar.StagedModelType;
import com.liferay.portal.kernel.bean.AutoEscapeBeanHandler;
import com.liferay.portal.kernel.exception.PortalException;
import com.liferay.portal.kernel.json.JSON;
import com.liferay.portal.kernel.model.CacheModel;
import com.liferay.portal.kernel.model.ModelWrapper;
import com.liferay.portal.kernel.model.User;
import com.liferay.portal.kernel.model.impl.BaseModelImpl;
import com.liferay.portal.kernel.service.ServiceContext;
import com.liferay.portal.kernel.service.UserLocalServiceUtil;
import com.liferay.portal.kernel.util.GetterUtil;
import com.liferay.portal.kernel.util.PortalUtil;
import com.liferay.portal.kernel.util.ProxyUtil;
import com.liferay.portal.kernel.util.StringBundler;
import java.io.Serializable;
import java.lang.reflect.Constructor;
import java.lang.reflect.InvocationHandler;
import java.sql.Types;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Date;
import java.util.HashMap;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.function.BiConsumer;
import java.util.function.Function;
/**
* The base model implementation for the CPDefinitionLink service. Represents a row in the "CPDefinitionLink" database table, with each column mapped to a property of this class.
*
*
* This implementation and its corresponding interface CPDefinitionLinkModel
exist only as a container for the default property accessors generated by ServiceBuilder. Helper methods and all application logic should be put in {@link CPDefinitionLinkImpl}.
*
*
* @author Marco Leo
* @see CPDefinitionLinkImpl
* @generated
*/
@JSON(strict = true)
public class CPDefinitionLinkModelImpl
extends BaseModelImpl implements CPDefinitionLinkModel {
/*
* NOTE FOR DEVELOPERS:
*
* Never modify or reference this class directly. All methods that expect a cp definition link model instance should use the CPDefinitionLink
interface instead.
*/
public static final String TABLE_NAME = "CPDefinitionLink";
public static final Object[][] TABLE_COLUMNS = {
{"uuid_", Types.VARCHAR}, {"CPDefinitionLinkId", Types.BIGINT},
{"groupId", Types.BIGINT}, {"companyId", Types.BIGINT},
{"userId", Types.BIGINT}, {"userName", Types.VARCHAR},
{"createDate", Types.TIMESTAMP}, {"modifiedDate", Types.TIMESTAMP},
{"CPDefinitionId", Types.BIGINT}, {"CProductId", Types.BIGINT},
{"priority", Types.DOUBLE}, {"type_", Types.VARCHAR}
};
public static final Map TABLE_COLUMNS_MAP =
new HashMap();
static {
TABLE_COLUMNS_MAP.put("uuid_", Types.VARCHAR);
TABLE_COLUMNS_MAP.put("CPDefinitionLinkId", Types.BIGINT);
TABLE_COLUMNS_MAP.put("groupId", Types.BIGINT);
TABLE_COLUMNS_MAP.put("companyId", Types.BIGINT);
TABLE_COLUMNS_MAP.put("userId", Types.BIGINT);
TABLE_COLUMNS_MAP.put("userName", Types.VARCHAR);
TABLE_COLUMNS_MAP.put("createDate", Types.TIMESTAMP);
TABLE_COLUMNS_MAP.put("modifiedDate", Types.TIMESTAMP);
TABLE_COLUMNS_MAP.put("CPDefinitionId", Types.BIGINT);
TABLE_COLUMNS_MAP.put("CProductId", Types.BIGINT);
TABLE_COLUMNS_MAP.put("priority", Types.DOUBLE);
TABLE_COLUMNS_MAP.put("type_", Types.VARCHAR);
}
public static final String TABLE_SQL_CREATE =
"create table CPDefinitionLink (uuid_ VARCHAR(75) null,CPDefinitionLinkId LONG not null primary key,groupId LONG,companyId LONG,userId LONG,userName VARCHAR(75) null,createDate DATE null,modifiedDate DATE null,CPDefinitionId LONG,CProductId LONG,priority DOUBLE,type_ VARCHAR(75) null)";
public static final String TABLE_SQL_DROP = "drop table CPDefinitionLink";
public static final String ORDER_BY_JPQL =
" ORDER BY cpDefinitionLink.priority ASC";
public static final String ORDER_BY_SQL =
" ORDER BY CPDefinitionLink.priority ASC";
public static final String DATA_SOURCE = "liferayDataSource";
public static final String SESSION_FACTORY = "liferaySessionFactory";
public static final String TX_MANAGER = "liferayTransactionManager";
public static final boolean ENTITY_CACHE_ENABLED = GetterUtil.getBoolean(
com.liferay.commerce.product.service.util.ServiceProps.get(
"value.object.entity.cache.enabled.com.liferay.commerce.product.model.CPDefinitionLink"),
true);
public static final boolean FINDER_CACHE_ENABLED = GetterUtil.getBoolean(
com.liferay.commerce.product.service.util.ServiceProps.get(
"value.object.finder.cache.enabled.com.liferay.commerce.product.model.CPDefinitionLink"),
true);
public static final boolean COLUMN_BITMASK_ENABLED = GetterUtil.getBoolean(
com.liferay.commerce.product.service.util.ServiceProps.get(
"value.object.column.bitmask.enabled.com.liferay.commerce.product.model.CPDefinitionLink"),
true);
public static final long CPDEFINITIONID_COLUMN_BITMASK = 1L;
public static final long CPRODUCTID_COLUMN_BITMASK = 2L;
public static final long COMPANYID_COLUMN_BITMASK = 4L;
public static final long GROUPID_COLUMN_BITMASK = 8L;
public static final long TYPE_COLUMN_BITMASK = 16L;
public static final long UUID_COLUMN_BITMASK = 32L;
public static final long PRIORITY_COLUMN_BITMASK = 64L;
/**
* Converts the soap model instance into a normal model instance.
*
* @param soapModel the soap model instance to convert
* @return the normal model instance
*/
public static CPDefinitionLink toModel(CPDefinitionLinkSoap soapModel) {
if (soapModel == null) {
return null;
}
CPDefinitionLink model = new CPDefinitionLinkImpl();
model.setUuid(soapModel.getUuid());
model.setCPDefinitionLinkId(soapModel.getCPDefinitionLinkId());
model.setGroupId(soapModel.getGroupId());
model.setCompanyId(soapModel.getCompanyId());
model.setUserId(soapModel.getUserId());
model.setUserName(soapModel.getUserName());
model.setCreateDate(soapModel.getCreateDate());
model.setModifiedDate(soapModel.getModifiedDate());
model.setCPDefinitionId(soapModel.getCPDefinitionId());
model.setCProductId(soapModel.getCProductId());
model.setPriority(soapModel.getPriority());
model.setType(soapModel.getType());
return model;
}
/**
* Converts the soap model instances into normal model instances.
*
* @param soapModels the soap model instances to convert
* @return the normal model instances
*/
public static List toModels(
CPDefinitionLinkSoap[] soapModels) {
if (soapModels == null) {
return null;
}
List models = new ArrayList(
soapModels.length);
for (CPDefinitionLinkSoap soapModel : soapModels) {
models.add(toModel(soapModel));
}
return models;
}
public static final long LOCK_EXPIRATION_TIME = GetterUtil.getLong(
com.liferay.commerce.product.service.util.ServiceProps.get(
"lock.expiration.time.com.liferay.commerce.product.model.CPDefinitionLink"));
public CPDefinitionLinkModelImpl() {
}
@Override
public long getPrimaryKey() {
return _CPDefinitionLinkId;
}
@Override
public void setPrimaryKey(long primaryKey) {
setCPDefinitionLinkId(primaryKey);
}
@Override
public Serializable getPrimaryKeyObj() {
return _CPDefinitionLinkId;
}
@Override
public void setPrimaryKeyObj(Serializable primaryKeyObj) {
setPrimaryKey(((Long)primaryKeyObj).longValue());
}
@Override
public Class> getModelClass() {
return CPDefinitionLink.class;
}
@Override
public String getModelClassName() {
return CPDefinitionLink.class.getName();
}
@Override
public Map getModelAttributes() {
Map attributes = new HashMap();
Map>
attributeGetterFunctions = getAttributeGetterFunctions();
for (Map.Entry> entry :
attributeGetterFunctions.entrySet()) {
String attributeName = entry.getKey();
Function attributeGetterFunction =
entry.getValue();
attributes.put(
attributeName,
attributeGetterFunction.apply((CPDefinitionLink)this));
}
attributes.put("entityCacheEnabled", isEntityCacheEnabled());
attributes.put("finderCacheEnabled", isFinderCacheEnabled());
return attributes;
}
@Override
public void setModelAttributes(Map attributes) {
Map>
attributeSetterBiConsumers = getAttributeSetterBiConsumers();
for (Map.Entry entry : attributes.entrySet()) {
String attributeName = entry.getKey();
BiConsumer attributeSetterBiConsumer =
attributeSetterBiConsumers.get(attributeName);
if (attributeSetterBiConsumer != null) {
attributeSetterBiConsumer.accept(
(CPDefinitionLink)this, entry.getValue());
}
}
}
public Map>
getAttributeGetterFunctions() {
return _attributeGetterFunctions;
}
public Map>
getAttributeSetterBiConsumers() {
return _attributeSetterBiConsumers;
}
private static Function
_getProxyProviderFunction() {
Class> proxyClass = ProxyUtil.getProxyClass(
CPDefinitionLink.class.getClassLoader(), CPDefinitionLink.class,
ModelWrapper.class);
try {
Constructor constructor =
(Constructor)proxyClass.getConstructor(
InvocationHandler.class);
return invocationHandler -> {
try {
return constructor.newInstance(invocationHandler);
}
catch (ReflectiveOperationException
reflectiveOperationException) {
throw new InternalError(reflectiveOperationException);
}
};
}
catch (NoSuchMethodException noSuchMethodException) {
throw new InternalError(noSuchMethodException);
}
}
private static final Map>
_attributeGetterFunctions;
private static final Map>
_attributeSetterBiConsumers;
static {
Map>
attributeGetterFunctions =
new LinkedHashMap>();
Map>
attributeSetterBiConsumers =
new LinkedHashMap>();
attributeGetterFunctions.put(
"uuid",
new Function() {
@Override
public Object apply(CPDefinitionLink cpDefinitionLink) {
return cpDefinitionLink.getUuid();
}
});
attributeSetterBiConsumers.put(
"uuid",
new BiConsumer() {
@Override
public void accept(
CPDefinitionLink cpDefinitionLink, Object uuidObject) {
cpDefinitionLink.setUuid((String)uuidObject);
}
});
attributeGetterFunctions.put(
"CPDefinitionLinkId",
new Function() {
@Override
public Object apply(CPDefinitionLink cpDefinitionLink) {
return cpDefinitionLink.getCPDefinitionLinkId();
}
});
attributeSetterBiConsumers.put(
"CPDefinitionLinkId",
new BiConsumer() {
@Override
public void accept(
CPDefinitionLink cpDefinitionLink,
Object CPDefinitionLinkIdObject) {
cpDefinitionLink.setCPDefinitionLinkId(
(Long)CPDefinitionLinkIdObject);
}
});
attributeGetterFunctions.put(
"groupId",
new Function() {
@Override
public Object apply(CPDefinitionLink cpDefinitionLink) {
return cpDefinitionLink.getGroupId();
}
});
attributeSetterBiConsumers.put(
"groupId",
new BiConsumer() {
@Override
public void accept(
CPDefinitionLink cpDefinitionLink, Object groupIdObject) {
cpDefinitionLink.setGroupId((Long)groupIdObject);
}
});
attributeGetterFunctions.put(
"companyId",
new Function() {
@Override
public Object apply(CPDefinitionLink cpDefinitionLink) {
return cpDefinitionLink.getCompanyId();
}
});
attributeSetterBiConsumers.put(
"companyId",
new BiConsumer() {
@Override
public void accept(
CPDefinitionLink cpDefinitionLink, Object companyIdObject) {
cpDefinitionLink.setCompanyId((Long)companyIdObject);
}
});
attributeGetterFunctions.put(
"userId",
new Function() {
@Override
public Object apply(CPDefinitionLink cpDefinitionLink) {
return cpDefinitionLink.getUserId();
}
});
attributeSetterBiConsumers.put(
"userId",
new BiConsumer() {
@Override
public void accept(
CPDefinitionLink cpDefinitionLink, Object userIdObject) {
cpDefinitionLink.setUserId((Long)userIdObject);
}
});
attributeGetterFunctions.put(
"userName",
new Function() {
@Override
public Object apply(CPDefinitionLink cpDefinitionLink) {
return cpDefinitionLink.getUserName();
}
});
attributeSetterBiConsumers.put(
"userName",
new BiConsumer() {
@Override
public void accept(
CPDefinitionLink cpDefinitionLink, Object userNameObject) {
cpDefinitionLink.setUserName((String)userNameObject);
}
});
attributeGetterFunctions.put(
"createDate",
new Function() {
@Override
public Object apply(CPDefinitionLink cpDefinitionLink) {
return cpDefinitionLink.getCreateDate();
}
});
attributeSetterBiConsumers.put(
"createDate",
new BiConsumer() {
@Override
public void accept(
CPDefinitionLink cpDefinitionLink,
Object createDateObject) {
cpDefinitionLink.setCreateDate((Date)createDateObject);
}
});
attributeGetterFunctions.put(
"modifiedDate",
new Function() {
@Override
public Object apply(CPDefinitionLink cpDefinitionLink) {
return cpDefinitionLink.getModifiedDate();
}
});
attributeSetterBiConsumers.put(
"modifiedDate",
new BiConsumer() {
@Override
public void accept(
CPDefinitionLink cpDefinitionLink,
Object modifiedDateObject) {
cpDefinitionLink.setModifiedDate((Date)modifiedDateObject);
}
});
attributeGetterFunctions.put(
"CPDefinitionId",
new Function() {
@Override
public Object apply(CPDefinitionLink cpDefinitionLink) {
return cpDefinitionLink.getCPDefinitionId();
}
});
attributeSetterBiConsumers.put(
"CPDefinitionId",
new BiConsumer() {
@Override
public void accept(
CPDefinitionLink cpDefinitionLink,
Object CPDefinitionIdObject) {
cpDefinitionLink.setCPDefinitionId(
(Long)CPDefinitionIdObject);
}
});
attributeGetterFunctions.put(
"CProductId",
new Function() {
@Override
public Object apply(CPDefinitionLink cpDefinitionLink) {
return cpDefinitionLink.getCProductId();
}
});
attributeSetterBiConsumers.put(
"CProductId",
new BiConsumer() {
@Override
public void accept(
CPDefinitionLink cpDefinitionLink,
Object CProductIdObject) {
cpDefinitionLink.setCProductId((Long)CProductIdObject);
}
});
attributeGetterFunctions.put(
"priority",
new Function() {
@Override
public Object apply(CPDefinitionLink cpDefinitionLink) {
return cpDefinitionLink.getPriority();
}
});
attributeSetterBiConsumers.put(
"priority",
new BiConsumer() {
@Override
public void accept(
CPDefinitionLink cpDefinitionLink, Object priorityObject) {
cpDefinitionLink.setPriority((Double)priorityObject);
}
});
attributeGetterFunctions.put(
"type",
new Function() {
@Override
public Object apply(CPDefinitionLink cpDefinitionLink) {
return cpDefinitionLink.getType();
}
});
attributeSetterBiConsumers.put(
"type",
new BiConsumer() {
@Override
public void accept(
CPDefinitionLink cpDefinitionLink, Object typeObject) {
cpDefinitionLink.setType((String)typeObject);
}
});
_attributeGetterFunctions = Collections.unmodifiableMap(
attributeGetterFunctions);
_attributeSetterBiConsumers = Collections.unmodifiableMap(
(Map)attributeSetterBiConsumers);
}
@JSON
@Override
public String getUuid() {
if (_uuid == null) {
return "";
}
else {
return _uuid;
}
}
@Override
public void setUuid(String uuid) {
_columnBitmask |= UUID_COLUMN_BITMASK;
if (_originalUuid == null) {
_originalUuid = _uuid;
}
_uuid = uuid;
}
public String getOriginalUuid() {
return GetterUtil.getString(_originalUuid);
}
@JSON
@Override
public long getCPDefinitionLinkId() {
return _CPDefinitionLinkId;
}
@Override
public void setCPDefinitionLinkId(long CPDefinitionLinkId) {
_CPDefinitionLinkId = CPDefinitionLinkId;
}
@JSON
@Override
public long getGroupId() {
return _groupId;
}
@Override
public void setGroupId(long groupId) {
_columnBitmask |= GROUPID_COLUMN_BITMASK;
if (!_setOriginalGroupId) {
_setOriginalGroupId = true;
_originalGroupId = _groupId;
}
_groupId = groupId;
}
public long getOriginalGroupId() {
return _originalGroupId;
}
@JSON
@Override
public long getCompanyId() {
return _companyId;
}
@Override
public void setCompanyId(long companyId) {
_columnBitmask |= COMPANYID_COLUMN_BITMASK;
if (!_setOriginalCompanyId) {
_setOriginalCompanyId = true;
_originalCompanyId = _companyId;
}
_companyId = companyId;
}
public long getOriginalCompanyId() {
return _originalCompanyId;
}
@JSON
@Override
public long getUserId() {
return _userId;
}
@Override
public void setUserId(long userId) {
_userId = userId;
}
@Override
public String getUserUuid() {
try {
User user = UserLocalServiceUtil.getUserById(getUserId());
return user.getUuid();
}
catch (PortalException portalException) {
return "";
}
}
@Override
public void setUserUuid(String userUuid) {
}
@JSON
@Override
public String getUserName() {
if (_userName == null) {
return "";
}
else {
return _userName;
}
}
@Override
public void setUserName(String userName) {
_userName = userName;
}
@JSON
@Override
public Date getCreateDate() {
return _createDate;
}
@Override
public void setCreateDate(Date createDate) {
_createDate = createDate;
}
@JSON
@Override
public Date getModifiedDate() {
return _modifiedDate;
}
public boolean hasSetModifiedDate() {
return _setModifiedDate;
}
@Override
public void setModifiedDate(Date modifiedDate) {
_setModifiedDate = true;
_modifiedDate = modifiedDate;
}
@JSON
@Override
public long getCPDefinitionId() {
return _CPDefinitionId;
}
@Override
public void setCPDefinitionId(long CPDefinitionId) {
_columnBitmask |= CPDEFINITIONID_COLUMN_BITMASK;
if (!_setOriginalCPDefinitionId) {
_setOriginalCPDefinitionId = true;
_originalCPDefinitionId = _CPDefinitionId;
}
_CPDefinitionId = CPDefinitionId;
}
public long getOriginalCPDefinitionId() {
return _originalCPDefinitionId;
}
@JSON
@Override
public long getCProductId() {
return _CProductId;
}
@Override
public void setCProductId(long CProductId) {
_columnBitmask |= CPRODUCTID_COLUMN_BITMASK;
if (!_setOriginalCProductId) {
_setOriginalCProductId = true;
_originalCProductId = _CProductId;
}
_CProductId = CProductId;
}
public long getOriginalCProductId() {
return _originalCProductId;
}
@JSON
@Override
public double getPriority() {
return _priority;
}
@Override
public void setPriority(double priority) {
_columnBitmask = -1L;
_priority = priority;
}
@JSON
@Override
public String getType() {
if (_type == null) {
return "";
}
else {
return _type;
}
}
@Override
public void setType(String type) {
_columnBitmask |= TYPE_COLUMN_BITMASK;
if (_originalType == null) {
_originalType = _type;
}
_type = type;
}
public String getOriginalType() {
return GetterUtil.getString(_originalType);
}
@Override
public StagedModelType getStagedModelType() {
return new StagedModelType(
PortalUtil.getClassNameId(CPDefinitionLink.class.getName()));
}
public long getColumnBitmask() {
return _columnBitmask;
}
@Override
public ExpandoBridge getExpandoBridge() {
return ExpandoBridgeFactoryUtil.getExpandoBridge(
getCompanyId(), CPDefinitionLink.class.getName(), getPrimaryKey());
}
@Override
public void setExpandoBridgeAttributes(ServiceContext serviceContext) {
ExpandoBridge expandoBridge = getExpandoBridge();
expandoBridge.setAttributes(serviceContext);
}
@Override
public CPDefinitionLink toEscapedModel() {
if (_escapedModel == null) {
Function
escapedModelProxyProviderFunction =
EscapedModelProxyProviderFunctionHolder.
_escapedModelProxyProviderFunction;
_escapedModel = escapedModelProxyProviderFunction.apply(
new AutoEscapeBeanHandler(this));
}
return _escapedModel;
}
@Override
public Object clone() {
CPDefinitionLinkImpl cpDefinitionLinkImpl = new CPDefinitionLinkImpl();
cpDefinitionLinkImpl.setUuid(getUuid());
cpDefinitionLinkImpl.setCPDefinitionLinkId(getCPDefinitionLinkId());
cpDefinitionLinkImpl.setGroupId(getGroupId());
cpDefinitionLinkImpl.setCompanyId(getCompanyId());
cpDefinitionLinkImpl.setUserId(getUserId());
cpDefinitionLinkImpl.setUserName(getUserName());
cpDefinitionLinkImpl.setCreateDate(getCreateDate());
cpDefinitionLinkImpl.setModifiedDate(getModifiedDate());
cpDefinitionLinkImpl.setCPDefinitionId(getCPDefinitionId());
cpDefinitionLinkImpl.setCProductId(getCProductId());
cpDefinitionLinkImpl.setPriority(getPriority());
cpDefinitionLinkImpl.setType(getType());
cpDefinitionLinkImpl.resetOriginalValues();
return cpDefinitionLinkImpl;
}
@Override
public int compareTo(CPDefinitionLink cpDefinitionLink) {
int value = 0;
if (getPriority() < cpDefinitionLink.getPriority()) {
value = -1;
}
else if (getPriority() > cpDefinitionLink.getPriority()) {
value = 1;
}
else {
value = 0;
}
if (value != 0) {
return value;
}
return 0;
}
@Override
public boolean equals(Object object) {
if (this == object) {
return true;
}
if (!(object instanceof CPDefinitionLink)) {
return false;
}
CPDefinitionLink cpDefinitionLink = (CPDefinitionLink)object;
long primaryKey = cpDefinitionLink.getPrimaryKey();
if (getPrimaryKey() == primaryKey) {
return true;
}
else {
return false;
}
}
@Override
public int hashCode() {
return (int)getPrimaryKey();
}
@Override
public boolean isEntityCacheEnabled() {
return ENTITY_CACHE_ENABLED;
}
@Override
public boolean isFinderCacheEnabled() {
return FINDER_CACHE_ENABLED;
}
@Override
public void resetOriginalValues() {
CPDefinitionLinkModelImpl cpDefinitionLinkModelImpl = this;
cpDefinitionLinkModelImpl._originalUuid =
cpDefinitionLinkModelImpl._uuid;
cpDefinitionLinkModelImpl._originalGroupId =
cpDefinitionLinkModelImpl._groupId;
cpDefinitionLinkModelImpl._setOriginalGroupId = false;
cpDefinitionLinkModelImpl._originalCompanyId =
cpDefinitionLinkModelImpl._companyId;
cpDefinitionLinkModelImpl._setOriginalCompanyId = false;
cpDefinitionLinkModelImpl._setModifiedDate = false;
cpDefinitionLinkModelImpl._originalCPDefinitionId =
cpDefinitionLinkModelImpl._CPDefinitionId;
cpDefinitionLinkModelImpl._setOriginalCPDefinitionId = false;
cpDefinitionLinkModelImpl._originalCProductId =
cpDefinitionLinkModelImpl._CProductId;
cpDefinitionLinkModelImpl._setOriginalCProductId = false;
cpDefinitionLinkModelImpl._originalType =
cpDefinitionLinkModelImpl._type;
cpDefinitionLinkModelImpl._columnBitmask = 0;
}
@Override
public CacheModel toCacheModel() {
CPDefinitionLinkCacheModel cpDefinitionLinkCacheModel =
new CPDefinitionLinkCacheModel();
cpDefinitionLinkCacheModel.uuid = getUuid();
String uuid = cpDefinitionLinkCacheModel.uuid;
if ((uuid != null) && (uuid.length() == 0)) {
cpDefinitionLinkCacheModel.uuid = null;
}
cpDefinitionLinkCacheModel.CPDefinitionLinkId = getCPDefinitionLinkId();
cpDefinitionLinkCacheModel.groupId = getGroupId();
cpDefinitionLinkCacheModel.companyId = getCompanyId();
cpDefinitionLinkCacheModel.userId = getUserId();
cpDefinitionLinkCacheModel.userName = getUserName();
String userName = cpDefinitionLinkCacheModel.userName;
if ((userName != null) && (userName.length() == 0)) {
cpDefinitionLinkCacheModel.userName = null;
}
Date createDate = getCreateDate();
if (createDate != null) {
cpDefinitionLinkCacheModel.createDate = createDate.getTime();
}
else {
cpDefinitionLinkCacheModel.createDate = Long.MIN_VALUE;
}
Date modifiedDate = getModifiedDate();
if (modifiedDate != null) {
cpDefinitionLinkCacheModel.modifiedDate = modifiedDate.getTime();
}
else {
cpDefinitionLinkCacheModel.modifiedDate = Long.MIN_VALUE;
}
cpDefinitionLinkCacheModel.CPDefinitionId = getCPDefinitionId();
cpDefinitionLinkCacheModel.CProductId = getCProductId();
cpDefinitionLinkCacheModel.priority = getPriority();
cpDefinitionLinkCacheModel.type = getType();
String type = cpDefinitionLinkCacheModel.type;
if ((type != null) && (type.length() == 0)) {
cpDefinitionLinkCacheModel.type = null;
}
return cpDefinitionLinkCacheModel;
}
@Override
public String toString() {
Map>
attributeGetterFunctions = getAttributeGetterFunctions();
StringBundler sb = new StringBundler(
4 * attributeGetterFunctions.size() + 2);
sb.append("{");
for (Map.Entry> entry :
attributeGetterFunctions.entrySet()) {
String attributeName = entry.getKey();
Function attributeGetterFunction =
entry.getValue();
sb.append(attributeName);
sb.append("=");
sb.append(attributeGetterFunction.apply((CPDefinitionLink)this));
sb.append(", ");
}
if (sb.index() > 1) {
sb.setIndex(sb.index() - 1);
}
sb.append("}");
return sb.toString();
}
@Override
public String toXmlString() {
Map>
attributeGetterFunctions = getAttributeGetterFunctions();
StringBundler sb = new StringBundler(
5 * attributeGetterFunctions.size() + 4);
sb.append("");
sb.append(getModelClassName());
sb.append(" ");
for (Map.Entry> entry :
attributeGetterFunctions.entrySet()) {
String attributeName = entry.getKey();
Function attributeGetterFunction =
entry.getValue();
sb.append("");
sb.append(attributeName);
sb.append(" ");
}
sb.append(" ");
return sb.toString();
}
private static class EscapedModelProxyProviderFunctionHolder {
private static final Function
_escapedModelProxyProviderFunction = _getProxyProviderFunction();
}
private String _uuid;
private String _originalUuid;
private long _CPDefinitionLinkId;
private long _groupId;
private long _originalGroupId;
private boolean _setOriginalGroupId;
private long _companyId;
private long _originalCompanyId;
private boolean _setOriginalCompanyId;
private long _userId;
private String _userName;
private Date _createDate;
private Date _modifiedDate;
private boolean _setModifiedDate;
private long _CPDefinitionId;
private long _originalCPDefinitionId;
private boolean _setOriginalCPDefinitionId;
private long _CProductId;
private long _originalCProductId;
private boolean _setOriginalCProductId;
private double _priority;
private String _type;
private String _originalType;
private long _columnBitmask;
private CPDefinitionLink _escapedModel;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy