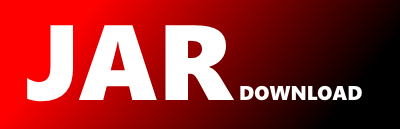
com.liferay.commerce.product.service.impl.CPDefinitionLinkServiceImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.liferay.commerce.product.service
Show all versions of com.liferay.commerce.product.service
Liferay Commerce Product Service
/**
* Copyright (c) 2000-present Liferay, Inc. All rights reserved.
*
* This library is free software; you can redistribute it and/or modify it under
* the terms of the GNU Lesser General Public License as published by the Free
* Software Foundation; either version 2.1 of the License, or (at your option)
* any later version.
*
* This library is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License for more
* details.
*/
package com.liferay.commerce.product.service.impl;
import com.liferay.commerce.product.exception.NoSuchCPDefinitionException;
import com.liferay.commerce.product.model.CPDefinition;
import com.liferay.commerce.product.model.CPDefinitionLink;
import com.liferay.commerce.product.model.CProduct;
import com.liferay.commerce.product.model.CommerceCatalog;
import com.liferay.commerce.product.service.base.CPDefinitionLinkServiceBaseImpl;
import com.liferay.portal.kernel.exception.PortalException;
import com.liferay.portal.kernel.security.auth.PrincipalException;
import com.liferay.portal.kernel.security.permission.ActionKeys;
import com.liferay.portal.kernel.security.permission.resource.ModelResourcePermission;
import com.liferay.portal.kernel.security.permission.resource.ModelResourcePermissionFactory;
import com.liferay.portal.kernel.service.ServiceContext;
import com.liferay.portal.kernel.util.OrderByComparator;
import java.util.List;
/**
* @author Alessio Antonio Rendina
* @author Marco Leo
*/
public class CPDefinitionLinkServiceImpl
extends CPDefinitionLinkServiceBaseImpl {
@Override
public CPDefinitionLink addCPDefinitionLink(
long cpDefinitionId, long cProductId, double priority, String type,
ServiceContext serviceContext)
throws PortalException {
_checkCommerceCatalogPermissionByCPDefinitionId(
cpDefinitionId, ActionKeys.UPDATE);
return cpDefinitionLinkLocalService.addCPDefinitionLinkByCProductId(
cpDefinitionId, cProductId, priority, type, serviceContext);
}
@Override
public void deleteCPDefinitionLink(long cpDefinitionLinkId)
throws PortalException {
CPDefinitionLink cpDefinitionLink =
cpDefinitionLinkLocalService.getCPDefinitionLink(
cpDefinitionLinkId);
_checkCommerceCatalogPermissionByCPDefinitionId(
cpDefinitionLink.getCPDefinitionId(), ActionKeys.UPDATE);
CProduct cProduct = cpDefinitionLink.getCProduct();
_checkCommerceCatalogPermissionByCPDefinitionId(
cProduct.getPublishedCPDefinitionId(), ActionKeys.UPDATE);
cpDefinitionLinkLocalService.deleteCPDefinitionLink(cpDefinitionLinkId);
}
@Override
public CPDefinitionLink fetchCPDefinitionLink(long cpDefinitionLinkId)
throws PortalException {
CPDefinitionLink cpDefinitionLink =
cpDefinitionLinkLocalService.fetchCPDefinitionLink(
cpDefinitionLinkId);
if (cpDefinitionLink != null) {
_checkCommerceCatalogPermissionByCPDefinitionId(
cpDefinitionLink.getCPDefinitionId(), ActionKeys.VIEW);
CProduct cProduct = cpDefinitionLink.getCProduct();
_checkCommerceCatalogPermissionByCPDefinitionId(
cProduct.getPublishedCPDefinitionId(), ActionKeys.VIEW);
}
return cpDefinitionLink;
}
@Override
public CPDefinitionLink getCPDefinitionLink(long cpDefinitionLinkId)
throws PortalException {
CPDefinitionLink cpDefinitionLink =
cpDefinitionLinkLocalService.getCPDefinitionLink(
cpDefinitionLinkId);
CProduct cProduct = cpDefinitionLink.getCProduct();
_checkCommerceCatalogPermissionByCPDefinitionId(
cProduct.getPublishedCPDefinitionId(), ActionKeys.VIEW);
_checkCommerceCatalogPermissionByCPDefinitionId(
cpDefinitionLink.getCPDefinitionId(), ActionKeys.VIEW);
return cpDefinitionLinkLocalService.getCPDefinitionLink(
cpDefinitionLinkId);
}
@Override
public List getCPDefinitionLinks(long cpDefinitionId)
throws PortalException {
_checkCommerceCatalogPermissionByCPDefinitionId(
cpDefinitionId, ActionKeys.VIEW);
return cpDefinitionLinkLocalService.getCPDefinitionLinks(
cpDefinitionId);
}
@Override
public List getCPDefinitionLinks(
long cpDefinitionId, int start, int end)
throws PortalException {
_checkCommerceCatalogPermissionByCPDefinitionId(
cpDefinitionId, ActionKeys.VIEW);
return cpDefinitionLinkLocalService.getCPDefinitionLinks(
cpDefinitionId, start, end);
}
@Override
public List getCPDefinitionLinks(
long cpDefinitionId, String type)
throws PortalException {
_checkCommerceCatalogPermissionByCPDefinitionId(
cpDefinitionId, ActionKeys.VIEW);
return cpDefinitionLinkLocalService.getCPDefinitionLinks(
cpDefinitionId, type);
}
@Override
public List getCPDefinitionLinks(
long cpDefinitionId, String type, int start, int end,
OrderByComparator orderByComparator)
throws PortalException {
_checkCommerceCatalogPermissionByCPDefinitionId(
cpDefinitionId, ActionKeys.VIEW);
return cpDefinitionLinkLocalService.getCPDefinitionLinks(
cpDefinitionId, type, start, end, orderByComparator);
}
@Override
public int getCPDefinitionLinksCount(long cpDefinitionId)
throws PortalException {
_checkCommerceCatalogPermissionByCPDefinitionId(
cpDefinitionId, ActionKeys.VIEW);
return cpDefinitionLinkLocalService.getCPDefinitionLinksCount(
cpDefinitionId);
}
@Override
public int getCPDefinitionLinksCount(long cpDefinitionId, String type)
throws PortalException {
_checkCommerceCatalogPermissionByCPDefinitionId(
cpDefinitionId, ActionKeys.VIEW);
return cpDefinitionLinkLocalService.getCPDefinitionLinksCount(
cpDefinitionId, type);
}
@Override
public CPDefinitionLink updateCPDefinitionLink(
long cpDefinitionLinkId, double priority,
ServiceContext serviceContext)
throws PortalException {
CPDefinitionLink cpDefinitionLink =
cpDefinitionLinkLocalService.getCPDefinitionLink(
cpDefinitionLinkId);
_checkCommerceCatalogPermissionByCPDefinitionId(
cpDefinitionLink.getCPDefinitionId(), ActionKeys.UPDATE);
CProduct cProduct = cpDefinitionLink.getCProduct();
_checkCommerceCatalogPermissionByCPDefinitionId(
cProduct.getPublishedCPDefinitionId(), ActionKeys.UPDATE);
return cpDefinitionLinkLocalService.updateCPDefinitionLink(
cpDefinitionLinkId, priority, serviceContext);
}
@Override
public void updateCPDefinitionLinks(
long cpDefinitionId, long[] cpDefinitionIds2, String type,
ServiceContext serviceContext)
throws PortalException {
_checkCommerceCatalogPermissionByCPDefinitionId(
cpDefinitionId, ActionKeys.UPDATE);
cpDefinitionLinkLocalService.updateCPDefinitionLinks(
cpDefinitionId, cpDefinitionIds2, type, serviceContext);
}
private void _checkCommerceCatalogPermissionByCPDefinitionId(
long cpDefinitionId, String actionId)
throws PortalException {
CPDefinition cpDefinition = cpDefinitionLocalService.fetchCPDefinition(
cpDefinitionId);
if (cpDefinition == null) {
throw new NoSuchCPDefinitionException();
}
CommerceCatalog commerceCatalog =
commerceCatalogLocalService.fetchCommerceCatalogByGroupId(
cpDefinition.getGroupId());
if (commerceCatalog == null) {
throw new PrincipalException();
}
_commerceCatalogModelResourcePermission.check(
getPermissionChecker(), commerceCatalog, actionId);
}
private static volatile ModelResourcePermission
_commerceCatalogModelResourcePermission =
ModelResourcePermissionFactory.getInstance(
CPDefinitionLinkServiceImpl.class,
"_commerceCatalogModelResourcePermission",
CommerceCatalog.class);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy