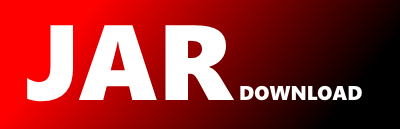
com.liferay.commerce.service.base.CommerceSubscriptionEntryServiceBaseImpl Maven / Gradle / Ivy
/**
* Copyright (c) 2000-present Liferay, Inc. All rights reserved.
*
* This library is free software; you can redistribute it and/or modify it under
* the terms of the GNU Lesser General Public License as published by the Free
* Software Foundation; either version 2.1 of the License, or (at your option)
* any later version.
*
* This library is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License for more
* details.
*/
package com.liferay.commerce.service.base;
import com.liferay.commerce.model.CommerceSubscriptionEntry;
import com.liferay.commerce.service.CommerceSubscriptionEntryService;
import com.liferay.commerce.service.persistence.CPDAvailabilityEstimatePersistence;
import com.liferay.commerce.service.persistence.CPDefinitionInventoryPersistence;
import com.liferay.commerce.service.persistence.CommerceAddressPersistence;
import com.liferay.commerce.service.persistence.CommerceAddressRestrictionPersistence;
import com.liferay.commerce.service.persistence.CommerceAvailabilityEstimatePersistence;
import com.liferay.commerce.service.persistence.CommerceCountryFinder;
import com.liferay.commerce.service.persistence.CommerceCountryPersistence;
import com.liferay.commerce.service.persistence.CommerceOrderFinder;
import com.liferay.commerce.service.persistence.CommerceOrderItemFinder;
import com.liferay.commerce.service.persistence.CommerceOrderItemPersistence;
import com.liferay.commerce.service.persistence.CommerceOrderNotePersistence;
import com.liferay.commerce.service.persistence.CommerceOrderPaymentPersistence;
import com.liferay.commerce.service.persistence.CommerceOrderPersistence;
import com.liferay.commerce.service.persistence.CommerceRegionPersistence;
import com.liferay.commerce.service.persistence.CommerceShipmentFinder;
import com.liferay.commerce.service.persistence.CommerceShipmentItemFinder;
import com.liferay.commerce.service.persistence.CommerceShipmentItemPersistence;
import com.liferay.commerce.service.persistence.CommerceShipmentPersistence;
import com.liferay.commerce.service.persistence.CommerceShippingMethodPersistence;
import com.liferay.commerce.service.persistence.CommerceSubscriptionEntryFinder;
import com.liferay.commerce.service.persistence.CommerceSubscriptionEntryPersistence;
import com.liferay.portal.kernel.bean.BeanReference;
import com.liferay.portal.kernel.dao.db.DB;
import com.liferay.portal.kernel.dao.db.DBManagerUtil;
import com.liferay.portal.kernel.dao.jdbc.SqlUpdate;
import com.liferay.portal.kernel.dao.jdbc.SqlUpdateFactoryUtil;
import com.liferay.portal.kernel.exception.SystemException;
import com.liferay.portal.kernel.module.framework.service.IdentifiableOSGiService;
import com.liferay.portal.kernel.service.BaseServiceImpl;
import com.liferay.portal.kernel.service.persistence.ClassNamePersistence;
import com.liferay.portal.kernel.service.persistence.UserPersistence;
import com.liferay.portal.kernel.util.PortalUtil;
import com.liferay.portal.spring.extender.service.ServiceReference;
import javax.sql.DataSource;
/**
* Provides the base implementation for the commerce subscription entry remote service.
*
*
* This implementation exists only as a container for the default service methods generated by ServiceBuilder. All custom service methods should be put in {@link com.liferay.commerce.service.impl.CommerceSubscriptionEntryServiceImpl}.
*
*
* @author Alessio Antonio Rendina
* @see com.liferay.commerce.service.impl.CommerceSubscriptionEntryServiceImpl
* @generated
*/
public abstract class CommerceSubscriptionEntryServiceBaseImpl
extends BaseServiceImpl
implements CommerceSubscriptionEntryService, IdentifiableOSGiService {
/*
* NOTE FOR DEVELOPERS:
*
* Never modify or reference this class directly. Use CommerceSubscriptionEntryService
via injection or a org.osgi.util.tracker.ServiceTracker
or use com.liferay.commerce.service.CommerceSubscriptionEntryServiceUtil
.
*/
/**
* Returns the commerce address local service.
*
* @return the commerce address local service
*/
public com.liferay.commerce.service.CommerceAddressLocalService
getCommerceAddressLocalService() {
return commerceAddressLocalService;
}
/**
* Sets the commerce address local service.
*
* @param commerceAddressLocalService the commerce address local service
*/
public void setCommerceAddressLocalService(
com.liferay.commerce.service.CommerceAddressLocalService
commerceAddressLocalService) {
this.commerceAddressLocalService = commerceAddressLocalService;
}
/**
* Returns the commerce address remote service.
*
* @return the commerce address remote service
*/
public com.liferay.commerce.service.CommerceAddressService
getCommerceAddressService() {
return commerceAddressService;
}
/**
* Sets the commerce address remote service.
*
* @param commerceAddressService the commerce address remote service
*/
public void setCommerceAddressService(
com.liferay.commerce.service.CommerceAddressService
commerceAddressService) {
this.commerceAddressService = commerceAddressService;
}
/**
* Returns the commerce address persistence.
*
* @return the commerce address persistence
*/
public CommerceAddressPersistence getCommerceAddressPersistence() {
return commerceAddressPersistence;
}
/**
* Sets the commerce address persistence.
*
* @param commerceAddressPersistence the commerce address persistence
*/
public void setCommerceAddressPersistence(
CommerceAddressPersistence commerceAddressPersistence) {
this.commerceAddressPersistence = commerceAddressPersistence;
}
/**
* Returns the commerce address restriction local service.
*
* @return the commerce address restriction local service
*/
public com.liferay.commerce.service.CommerceAddressRestrictionLocalService
getCommerceAddressRestrictionLocalService() {
return commerceAddressRestrictionLocalService;
}
/**
* Sets the commerce address restriction local service.
*
* @param commerceAddressRestrictionLocalService the commerce address restriction local service
*/
public void setCommerceAddressRestrictionLocalService(
com.liferay.commerce.service.CommerceAddressRestrictionLocalService
commerceAddressRestrictionLocalService) {
this.commerceAddressRestrictionLocalService =
commerceAddressRestrictionLocalService;
}
/**
* Returns the commerce address restriction remote service.
*
* @return the commerce address restriction remote service
*/
public com.liferay.commerce.service.CommerceAddressRestrictionService
getCommerceAddressRestrictionService() {
return commerceAddressRestrictionService;
}
/**
* Sets the commerce address restriction remote service.
*
* @param commerceAddressRestrictionService the commerce address restriction remote service
*/
public void setCommerceAddressRestrictionService(
com.liferay.commerce.service.CommerceAddressRestrictionService
commerceAddressRestrictionService) {
this.commerceAddressRestrictionService =
commerceAddressRestrictionService;
}
/**
* Returns the commerce address restriction persistence.
*
* @return the commerce address restriction persistence
*/
public CommerceAddressRestrictionPersistence
getCommerceAddressRestrictionPersistence() {
return commerceAddressRestrictionPersistence;
}
/**
* Sets the commerce address restriction persistence.
*
* @param commerceAddressRestrictionPersistence the commerce address restriction persistence
*/
public void setCommerceAddressRestrictionPersistence(
CommerceAddressRestrictionPersistence
commerceAddressRestrictionPersistence) {
this.commerceAddressRestrictionPersistence =
commerceAddressRestrictionPersistence;
}
/**
* Returns the commerce availability estimate local service.
*
* @return the commerce availability estimate local service
*/
public com.liferay.commerce.service.CommerceAvailabilityEstimateLocalService
getCommerceAvailabilityEstimateLocalService() {
return commerceAvailabilityEstimateLocalService;
}
/**
* Sets the commerce availability estimate local service.
*
* @param commerceAvailabilityEstimateLocalService the commerce availability estimate local service
*/
public void setCommerceAvailabilityEstimateLocalService(
com.liferay.commerce.service.CommerceAvailabilityEstimateLocalService
commerceAvailabilityEstimateLocalService) {
this.commerceAvailabilityEstimateLocalService =
commerceAvailabilityEstimateLocalService;
}
/**
* Returns the commerce availability estimate remote service.
*
* @return the commerce availability estimate remote service
*/
public com.liferay.commerce.service.CommerceAvailabilityEstimateService
getCommerceAvailabilityEstimateService() {
return commerceAvailabilityEstimateService;
}
/**
* Sets the commerce availability estimate remote service.
*
* @param commerceAvailabilityEstimateService the commerce availability estimate remote service
*/
public void setCommerceAvailabilityEstimateService(
com.liferay.commerce.service.CommerceAvailabilityEstimateService
commerceAvailabilityEstimateService) {
this.commerceAvailabilityEstimateService =
commerceAvailabilityEstimateService;
}
/**
* Returns the commerce availability estimate persistence.
*
* @return the commerce availability estimate persistence
*/
public CommerceAvailabilityEstimatePersistence
getCommerceAvailabilityEstimatePersistence() {
return commerceAvailabilityEstimatePersistence;
}
/**
* Sets the commerce availability estimate persistence.
*
* @param commerceAvailabilityEstimatePersistence the commerce availability estimate persistence
*/
public void setCommerceAvailabilityEstimatePersistence(
CommerceAvailabilityEstimatePersistence
commerceAvailabilityEstimatePersistence) {
this.commerceAvailabilityEstimatePersistence =
commerceAvailabilityEstimatePersistence;
}
/**
* Returns the commerce country local service.
*
* @return the commerce country local service
*/
public com.liferay.commerce.service.CommerceCountryLocalService
getCommerceCountryLocalService() {
return commerceCountryLocalService;
}
/**
* Sets the commerce country local service.
*
* @param commerceCountryLocalService the commerce country local service
*/
public void setCommerceCountryLocalService(
com.liferay.commerce.service.CommerceCountryLocalService
commerceCountryLocalService) {
this.commerceCountryLocalService = commerceCountryLocalService;
}
/**
* Returns the commerce country remote service.
*
* @return the commerce country remote service
*/
public com.liferay.commerce.service.CommerceCountryService
getCommerceCountryService() {
return commerceCountryService;
}
/**
* Sets the commerce country remote service.
*
* @param commerceCountryService the commerce country remote service
*/
public void setCommerceCountryService(
com.liferay.commerce.service.CommerceCountryService
commerceCountryService) {
this.commerceCountryService = commerceCountryService;
}
/**
* Returns the commerce country persistence.
*
* @return the commerce country persistence
*/
public CommerceCountryPersistence getCommerceCountryPersistence() {
return commerceCountryPersistence;
}
/**
* Sets the commerce country persistence.
*
* @param commerceCountryPersistence the commerce country persistence
*/
public void setCommerceCountryPersistence(
CommerceCountryPersistence commerceCountryPersistence) {
this.commerceCountryPersistence = commerceCountryPersistence;
}
/**
* Returns the commerce country finder.
*
* @return the commerce country finder
*/
public CommerceCountryFinder getCommerceCountryFinder() {
return commerceCountryFinder;
}
/**
* Sets the commerce country finder.
*
* @param commerceCountryFinder the commerce country finder
*/
public void setCommerceCountryFinder(
CommerceCountryFinder commerceCountryFinder) {
this.commerceCountryFinder = commerceCountryFinder;
}
/**
* Returns the commerce order local service.
*
* @return the commerce order local service
*/
public com.liferay.commerce.service.CommerceOrderLocalService
getCommerceOrderLocalService() {
return commerceOrderLocalService;
}
/**
* Sets the commerce order local service.
*
* @param commerceOrderLocalService the commerce order local service
*/
public void setCommerceOrderLocalService(
com.liferay.commerce.service.CommerceOrderLocalService
commerceOrderLocalService) {
this.commerceOrderLocalService = commerceOrderLocalService;
}
/**
* Returns the commerce order remote service.
*
* @return the commerce order remote service
*/
public com.liferay.commerce.service.CommerceOrderService
getCommerceOrderService() {
return commerceOrderService;
}
/**
* Sets the commerce order remote service.
*
* @param commerceOrderService the commerce order remote service
*/
public void setCommerceOrderService(
com.liferay.commerce.service.CommerceOrderService
commerceOrderService) {
this.commerceOrderService = commerceOrderService;
}
/**
* Returns the commerce order persistence.
*
* @return the commerce order persistence
*/
public CommerceOrderPersistence getCommerceOrderPersistence() {
return commerceOrderPersistence;
}
/**
* Sets the commerce order persistence.
*
* @param commerceOrderPersistence the commerce order persistence
*/
public void setCommerceOrderPersistence(
CommerceOrderPersistence commerceOrderPersistence) {
this.commerceOrderPersistence = commerceOrderPersistence;
}
/**
* Returns the commerce order finder.
*
* @return the commerce order finder
*/
public CommerceOrderFinder getCommerceOrderFinder() {
return commerceOrderFinder;
}
/**
* Sets the commerce order finder.
*
* @param commerceOrderFinder the commerce order finder
*/
public void setCommerceOrderFinder(
CommerceOrderFinder commerceOrderFinder) {
this.commerceOrderFinder = commerceOrderFinder;
}
/**
* Returns the commerce order item local service.
*
* @return the commerce order item local service
*/
public com.liferay.commerce.service.CommerceOrderItemLocalService
getCommerceOrderItemLocalService() {
return commerceOrderItemLocalService;
}
/**
* Sets the commerce order item local service.
*
* @param commerceOrderItemLocalService the commerce order item local service
*/
public void setCommerceOrderItemLocalService(
com.liferay.commerce.service.CommerceOrderItemLocalService
commerceOrderItemLocalService) {
this.commerceOrderItemLocalService = commerceOrderItemLocalService;
}
/**
* Returns the commerce order item remote service.
*
* @return the commerce order item remote service
*/
public com.liferay.commerce.service.CommerceOrderItemService
getCommerceOrderItemService() {
return commerceOrderItemService;
}
/**
* Sets the commerce order item remote service.
*
* @param commerceOrderItemService the commerce order item remote service
*/
public void setCommerceOrderItemService(
com.liferay.commerce.service.CommerceOrderItemService
commerceOrderItemService) {
this.commerceOrderItemService = commerceOrderItemService;
}
/**
* Returns the commerce order item persistence.
*
* @return the commerce order item persistence
*/
public CommerceOrderItemPersistence getCommerceOrderItemPersistence() {
return commerceOrderItemPersistence;
}
/**
* Sets the commerce order item persistence.
*
* @param commerceOrderItemPersistence the commerce order item persistence
*/
public void setCommerceOrderItemPersistence(
CommerceOrderItemPersistence commerceOrderItemPersistence) {
this.commerceOrderItemPersistence = commerceOrderItemPersistence;
}
/**
* Returns the commerce order item finder.
*
* @return the commerce order item finder
*/
public CommerceOrderItemFinder getCommerceOrderItemFinder() {
return commerceOrderItemFinder;
}
/**
* Sets the commerce order item finder.
*
* @param commerceOrderItemFinder the commerce order item finder
*/
public void setCommerceOrderItemFinder(
CommerceOrderItemFinder commerceOrderItemFinder) {
this.commerceOrderItemFinder = commerceOrderItemFinder;
}
/**
* Returns the commerce order note local service.
*
* @return the commerce order note local service
*/
public com.liferay.commerce.service.CommerceOrderNoteLocalService
getCommerceOrderNoteLocalService() {
return commerceOrderNoteLocalService;
}
/**
* Sets the commerce order note local service.
*
* @param commerceOrderNoteLocalService the commerce order note local service
*/
public void setCommerceOrderNoteLocalService(
com.liferay.commerce.service.CommerceOrderNoteLocalService
commerceOrderNoteLocalService) {
this.commerceOrderNoteLocalService = commerceOrderNoteLocalService;
}
/**
* Returns the commerce order note remote service.
*
* @return the commerce order note remote service
*/
public com.liferay.commerce.service.CommerceOrderNoteService
getCommerceOrderNoteService() {
return commerceOrderNoteService;
}
/**
* Sets the commerce order note remote service.
*
* @param commerceOrderNoteService the commerce order note remote service
*/
public void setCommerceOrderNoteService(
com.liferay.commerce.service.CommerceOrderNoteService
commerceOrderNoteService) {
this.commerceOrderNoteService = commerceOrderNoteService;
}
/**
* Returns the commerce order note persistence.
*
* @return the commerce order note persistence
*/
public CommerceOrderNotePersistence getCommerceOrderNotePersistence() {
return commerceOrderNotePersistence;
}
/**
* Sets the commerce order note persistence.
*
* @param commerceOrderNotePersistence the commerce order note persistence
*/
public void setCommerceOrderNotePersistence(
CommerceOrderNotePersistence commerceOrderNotePersistence) {
this.commerceOrderNotePersistence = commerceOrderNotePersistence;
}
/**
* Returns the commerce order payment local service.
*
* @return the commerce order payment local service
*/
public com.liferay.commerce.service.CommerceOrderPaymentLocalService
getCommerceOrderPaymentLocalService() {
return commerceOrderPaymentLocalService;
}
/**
* Sets the commerce order payment local service.
*
* @param commerceOrderPaymentLocalService the commerce order payment local service
*/
public void setCommerceOrderPaymentLocalService(
com.liferay.commerce.service.CommerceOrderPaymentLocalService
commerceOrderPaymentLocalService) {
this.commerceOrderPaymentLocalService =
commerceOrderPaymentLocalService;
}
/**
* Returns the commerce order payment persistence.
*
* @return the commerce order payment persistence
*/
public CommerceOrderPaymentPersistence
getCommerceOrderPaymentPersistence() {
return commerceOrderPaymentPersistence;
}
/**
* Sets the commerce order payment persistence.
*
* @param commerceOrderPaymentPersistence the commerce order payment persistence
*/
public void setCommerceOrderPaymentPersistence(
CommerceOrderPaymentPersistence commerceOrderPaymentPersistence) {
this.commerceOrderPaymentPersistence = commerceOrderPaymentPersistence;
}
/**
* Returns the commerce region local service.
*
* @return the commerce region local service
*/
public com.liferay.commerce.service.CommerceRegionLocalService
getCommerceRegionLocalService() {
return commerceRegionLocalService;
}
/**
* Sets the commerce region local service.
*
* @param commerceRegionLocalService the commerce region local service
*/
public void setCommerceRegionLocalService(
com.liferay.commerce.service.CommerceRegionLocalService
commerceRegionLocalService) {
this.commerceRegionLocalService = commerceRegionLocalService;
}
/**
* Returns the commerce region remote service.
*
* @return the commerce region remote service
*/
public com.liferay.commerce.service.CommerceRegionService
getCommerceRegionService() {
return commerceRegionService;
}
/**
* Sets the commerce region remote service.
*
* @param commerceRegionService the commerce region remote service
*/
public void setCommerceRegionService(
com.liferay.commerce.service.CommerceRegionService
commerceRegionService) {
this.commerceRegionService = commerceRegionService;
}
/**
* Returns the commerce region persistence.
*
* @return the commerce region persistence
*/
public CommerceRegionPersistence getCommerceRegionPersistence() {
return commerceRegionPersistence;
}
/**
* Sets the commerce region persistence.
*
* @param commerceRegionPersistence the commerce region persistence
*/
public void setCommerceRegionPersistence(
CommerceRegionPersistence commerceRegionPersistence) {
this.commerceRegionPersistence = commerceRegionPersistence;
}
/**
* Returns the commerce shipment local service.
*
* @return the commerce shipment local service
*/
public com.liferay.commerce.service.CommerceShipmentLocalService
getCommerceShipmentLocalService() {
return commerceShipmentLocalService;
}
/**
* Sets the commerce shipment local service.
*
* @param commerceShipmentLocalService the commerce shipment local service
*/
public void setCommerceShipmentLocalService(
com.liferay.commerce.service.CommerceShipmentLocalService
commerceShipmentLocalService) {
this.commerceShipmentLocalService = commerceShipmentLocalService;
}
/**
* Returns the commerce shipment remote service.
*
* @return the commerce shipment remote service
*/
public com.liferay.commerce.service.CommerceShipmentService
getCommerceShipmentService() {
return commerceShipmentService;
}
/**
* Sets the commerce shipment remote service.
*
* @param commerceShipmentService the commerce shipment remote service
*/
public void setCommerceShipmentService(
com.liferay.commerce.service.CommerceShipmentService
commerceShipmentService) {
this.commerceShipmentService = commerceShipmentService;
}
/**
* Returns the commerce shipment persistence.
*
* @return the commerce shipment persistence
*/
public CommerceShipmentPersistence getCommerceShipmentPersistence() {
return commerceShipmentPersistence;
}
/**
* Sets the commerce shipment persistence.
*
* @param commerceShipmentPersistence the commerce shipment persistence
*/
public void setCommerceShipmentPersistence(
CommerceShipmentPersistence commerceShipmentPersistence) {
this.commerceShipmentPersistence = commerceShipmentPersistence;
}
/**
* Returns the commerce shipment finder.
*
* @return the commerce shipment finder
*/
public CommerceShipmentFinder getCommerceShipmentFinder() {
return commerceShipmentFinder;
}
/**
* Sets the commerce shipment finder.
*
* @param commerceShipmentFinder the commerce shipment finder
*/
public void setCommerceShipmentFinder(
CommerceShipmentFinder commerceShipmentFinder) {
this.commerceShipmentFinder = commerceShipmentFinder;
}
/**
* Returns the commerce shipment item local service.
*
* @return the commerce shipment item local service
*/
public com.liferay.commerce.service.CommerceShipmentItemLocalService
getCommerceShipmentItemLocalService() {
return commerceShipmentItemLocalService;
}
/**
* Sets the commerce shipment item local service.
*
* @param commerceShipmentItemLocalService the commerce shipment item local service
*/
public void setCommerceShipmentItemLocalService(
com.liferay.commerce.service.CommerceShipmentItemLocalService
commerceShipmentItemLocalService) {
this.commerceShipmentItemLocalService =
commerceShipmentItemLocalService;
}
/**
* Returns the commerce shipment item remote service.
*
* @return the commerce shipment item remote service
*/
public com.liferay.commerce.service.CommerceShipmentItemService
getCommerceShipmentItemService() {
return commerceShipmentItemService;
}
/**
* Sets the commerce shipment item remote service.
*
* @param commerceShipmentItemService the commerce shipment item remote service
*/
public void setCommerceShipmentItemService(
com.liferay.commerce.service.CommerceShipmentItemService
commerceShipmentItemService) {
this.commerceShipmentItemService = commerceShipmentItemService;
}
/**
* Returns the commerce shipment item persistence.
*
* @return the commerce shipment item persistence
*/
public CommerceShipmentItemPersistence
getCommerceShipmentItemPersistence() {
return commerceShipmentItemPersistence;
}
/**
* Sets the commerce shipment item persistence.
*
* @param commerceShipmentItemPersistence the commerce shipment item persistence
*/
public void setCommerceShipmentItemPersistence(
CommerceShipmentItemPersistence commerceShipmentItemPersistence) {
this.commerceShipmentItemPersistence = commerceShipmentItemPersistence;
}
/**
* Returns the commerce shipment item finder.
*
* @return the commerce shipment item finder
*/
public CommerceShipmentItemFinder getCommerceShipmentItemFinder() {
return commerceShipmentItemFinder;
}
/**
* Sets the commerce shipment item finder.
*
* @param commerceShipmentItemFinder the commerce shipment item finder
*/
public void setCommerceShipmentItemFinder(
CommerceShipmentItemFinder commerceShipmentItemFinder) {
this.commerceShipmentItemFinder = commerceShipmentItemFinder;
}
/**
* Returns the commerce shipping method local service.
*
* @return the commerce shipping method local service
*/
public com.liferay.commerce.service.CommerceShippingMethodLocalService
getCommerceShippingMethodLocalService() {
return commerceShippingMethodLocalService;
}
/**
* Sets the commerce shipping method local service.
*
* @param commerceShippingMethodLocalService the commerce shipping method local service
*/
public void setCommerceShippingMethodLocalService(
com.liferay.commerce.service.CommerceShippingMethodLocalService
commerceShippingMethodLocalService) {
this.commerceShippingMethodLocalService =
commerceShippingMethodLocalService;
}
/**
* Returns the commerce shipping method remote service.
*
* @return the commerce shipping method remote service
*/
public com.liferay.commerce.service.CommerceShippingMethodService
getCommerceShippingMethodService() {
return commerceShippingMethodService;
}
/**
* Sets the commerce shipping method remote service.
*
* @param commerceShippingMethodService the commerce shipping method remote service
*/
public void setCommerceShippingMethodService(
com.liferay.commerce.service.CommerceShippingMethodService
commerceShippingMethodService) {
this.commerceShippingMethodService = commerceShippingMethodService;
}
/**
* Returns the commerce shipping method persistence.
*
* @return the commerce shipping method persistence
*/
public CommerceShippingMethodPersistence
getCommerceShippingMethodPersistence() {
return commerceShippingMethodPersistence;
}
/**
* Sets the commerce shipping method persistence.
*
* @param commerceShippingMethodPersistence the commerce shipping method persistence
*/
public void setCommerceShippingMethodPersistence(
CommerceShippingMethodPersistence commerceShippingMethodPersistence) {
this.commerceShippingMethodPersistence =
commerceShippingMethodPersistence;
}
/**
* Returns the commerce subscription entry local service.
*
* @return the commerce subscription entry local service
*/
public com.liferay.commerce.service.CommerceSubscriptionEntryLocalService
getCommerceSubscriptionEntryLocalService() {
return commerceSubscriptionEntryLocalService;
}
/**
* Sets the commerce subscription entry local service.
*
* @param commerceSubscriptionEntryLocalService the commerce subscription entry local service
*/
public void setCommerceSubscriptionEntryLocalService(
com.liferay.commerce.service.CommerceSubscriptionEntryLocalService
commerceSubscriptionEntryLocalService) {
this.commerceSubscriptionEntryLocalService =
commerceSubscriptionEntryLocalService;
}
/**
* Returns the commerce subscription entry remote service.
*
* @return the commerce subscription entry remote service
*/
public CommerceSubscriptionEntryService
getCommerceSubscriptionEntryService() {
return commerceSubscriptionEntryService;
}
/**
* Sets the commerce subscription entry remote service.
*
* @param commerceSubscriptionEntryService the commerce subscription entry remote service
*/
public void setCommerceSubscriptionEntryService(
CommerceSubscriptionEntryService commerceSubscriptionEntryService) {
this.commerceSubscriptionEntryService =
commerceSubscriptionEntryService;
}
/**
* Returns the commerce subscription entry persistence.
*
* @return the commerce subscription entry persistence
*/
public CommerceSubscriptionEntryPersistence
getCommerceSubscriptionEntryPersistence() {
return commerceSubscriptionEntryPersistence;
}
/**
* Sets the commerce subscription entry persistence.
*
* @param commerceSubscriptionEntryPersistence the commerce subscription entry persistence
*/
public void setCommerceSubscriptionEntryPersistence(
CommerceSubscriptionEntryPersistence
commerceSubscriptionEntryPersistence) {
this.commerceSubscriptionEntryPersistence =
commerceSubscriptionEntryPersistence;
}
/**
* Returns the commerce subscription entry finder.
*
* @return the commerce subscription entry finder
*/
public CommerceSubscriptionEntryFinder
getCommerceSubscriptionEntryFinder() {
return commerceSubscriptionEntryFinder;
}
/**
* Sets the commerce subscription entry finder.
*
* @param commerceSubscriptionEntryFinder the commerce subscription entry finder
*/
public void setCommerceSubscriptionEntryFinder(
CommerceSubscriptionEntryFinder commerceSubscriptionEntryFinder) {
this.commerceSubscriptionEntryFinder = commerceSubscriptionEntryFinder;
}
/**
* Returns the cpd availability estimate local service.
*
* @return the cpd availability estimate local service
*/
public com.liferay.commerce.service.CPDAvailabilityEstimateLocalService
getCPDAvailabilityEstimateLocalService() {
return cpdAvailabilityEstimateLocalService;
}
/**
* Sets the cpd availability estimate local service.
*
* @param cpdAvailabilityEstimateLocalService the cpd availability estimate local service
*/
public void setCPDAvailabilityEstimateLocalService(
com.liferay.commerce.service.CPDAvailabilityEstimateLocalService
cpdAvailabilityEstimateLocalService) {
this.cpdAvailabilityEstimateLocalService =
cpdAvailabilityEstimateLocalService;
}
/**
* Returns the cpd availability estimate remote service.
*
* @return the cpd availability estimate remote service
*/
public com.liferay.commerce.service.CPDAvailabilityEstimateService
getCPDAvailabilityEstimateService() {
return cpdAvailabilityEstimateService;
}
/**
* Sets the cpd availability estimate remote service.
*
* @param cpdAvailabilityEstimateService the cpd availability estimate remote service
*/
public void setCPDAvailabilityEstimateService(
com.liferay.commerce.service.CPDAvailabilityEstimateService
cpdAvailabilityEstimateService) {
this.cpdAvailabilityEstimateService = cpdAvailabilityEstimateService;
}
/**
* Returns the cpd availability estimate persistence.
*
* @return the cpd availability estimate persistence
*/
public CPDAvailabilityEstimatePersistence
getCPDAvailabilityEstimatePersistence() {
return cpdAvailabilityEstimatePersistence;
}
/**
* Sets the cpd availability estimate persistence.
*
* @param cpdAvailabilityEstimatePersistence the cpd availability estimate persistence
*/
public void setCPDAvailabilityEstimatePersistence(
CPDAvailabilityEstimatePersistence cpdAvailabilityEstimatePersistence) {
this.cpdAvailabilityEstimatePersistence =
cpdAvailabilityEstimatePersistence;
}
/**
* Returns the cp definition inventory local service.
*
* @return the cp definition inventory local service
*/
public com.liferay.commerce.service.CPDefinitionInventoryLocalService
getCPDefinitionInventoryLocalService() {
return cpDefinitionInventoryLocalService;
}
/**
* Sets the cp definition inventory local service.
*
* @param cpDefinitionInventoryLocalService the cp definition inventory local service
*/
public void setCPDefinitionInventoryLocalService(
com.liferay.commerce.service.CPDefinitionInventoryLocalService
cpDefinitionInventoryLocalService) {
this.cpDefinitionInventoryLocalService =
cpDefinitionInventoryLocalService;
}
/**
* Returns the cp definition inventory remote service.
*
* @return the cp definition inventory remote service
*/
public com.liferay.commerce.service.CPDefinitionInventoryService
getCPDefinitionInventoryService() {
return cpDefinitionInventoryService;
}
/**
* Sets the cp definition inventory remote service.
*
* @param cpDefinitionInventoryService the cp definition inventory remote service
*/
public void setCPDefinitionInventoryService(
com.liferay.commerce.service.CPDefinitionInventoryService
cpDefinitionInventoryService) {
this.cpDefinitionInventoryService = cpDefinitionInventoryService;
}
/**
* Returns the cp definition inventory persistence.
*
* @return the cp definition inventory persistence
*/
public CPDefinitionInventoryPersistence
getCPDefinitionInventoryPersistence() {
return cpDefinitionInventoryPersistence;
}
/**
* Sets the cp definition inventory persistence.
*
* @param cpDefinitionInventoryPersistence the cp definition inventory persistence
*/
public void setCPDefinitionInventoryPersistence(
CPDefinitionInventoryPersistence cpDefinitionInventoryPersistence) {
this.cpDefinitionInventoryPersistence =
cpDefinitionInventoryPersistence;
}
/**
* Returns the counter local service.
*
* @return the counter local service
*/
public com.liferay.counter.kernel.service.CounterLocalService
getCounterLocalService() {
return counterLocalService;
}
/**
* Sets the counter local service.
*
* @param counterLocalService the counter local service
*/
public void setCounterLocalService(
com.liferay.counter.kernel.service.CounterLocalService
counterLocalService) {
this.counterLocalService = counterLocalService;
}
/**
* Returns the class name local service.
*
* @return the class name local service
*/
public com.liferay.portal.kernel.service.ClassNameLocalService
getClassNameLocalService() {
return classNameLocalService;
}
/**
* Sets the class name local service.
*
* @param classNameLocalService the class name local service
*/
public void setClassNameLocalService(
com.liferay.portal.kernel.service.ClassNameLocalService
classNameLocalService) {
this.classNameLocalService = classNameLocalService;
}
/**
* Returns the class name remote service.
*
* @return the class name remote service
*/
public com.liferay.portal.kernel.service.ClassNameService
getClassNameService() {
return classNameService;
}
/**
* Sets the class name remote service.
*
* @param classNameService the class name remote service
*/
public void setClassNameService(
com.liferay.portal.kernel.service.ClassNameService classNameService) {
this.classNameService = classNameService;
}
/**
* Returns the class name persistence.
*
* @return the class name persistence
*/
public ClassNamePersistence getClassNamePersistence() {
return classNamePersistence;
}
/**
* Sets the class name persistence.
*
* @param classNamePersistence the class name persistence
*/
public void setClassNamePersistence(
ClassNamePersistence classNamePersistence) {
this.classNamePersistence = classNamePersistence;
}
/**
* Returns the resource local service.
*
* @return the resource local service
*/
public com.liferay.portal.kernel.service.ResourceLocalService
getResourceLocalService() {
return resourceLocalService;
}
/**
* Sets the resource local service.
*
* @param resourceLocalService the resource local service
*/
public void setResourceLocalService(
com.liferay.portal.kernel.service.ResourceLocalService
resourceLocalService) {
this.resourceLocalService = resourceLocalService;
}
/**
* Returns the user local service.
*
* @return the user local service
*/
public com.liferay.portal.kernel.service.UserLocalService
getUserLocalService() {
return userLocalService;
}
/**
* Sets the user local service.
*
* @param userLocalService the user local service
*/
public void setUserLocalService(
com.liferay.portal.kernel.service.UserLocalService userLocalService) {
this.userLocalService = userLocalService;
}
/**
* Returns the user remote service.
*
* @return the user remote service
*/
public com.liferay.portal.kernel.service.UserService getUserService() {
return userService;
}
/**
* Sets the user remote service.
*
* @param userService the user remote service
*/
public void setUserService(
com.liferay.portal.kernel.service.UserService userService) {
this.userService = userService;
}
/**
* Returns the user persistence.
*
* @return the user persistence
*/
public UserPersistence getUserPersistence() {
return userPersistence;
}
/**
* Sets the user persistence.
*
* @param userPersistence the user persistence
*/
public void setUserPersistence(UserPersistence userPersistence) {
this.userPersistence = userPersistence;
}
public void afterPropertiesSet() {
}
public void destroy() {
}
/**
* Returns the OSGi service identifier.
*
* @return the OSGi service identifier
*/
@Override
public String getOSGiServiceIdentifier() {
return CommerceSubscriptionEntryService.class.getName();
}
protected Class> getModelClass() {
return CommerceSubscriptionEntry.class;
}
protected String getModelClassName() {
return CommerceSubscriptionEntry.class.getName();
}
/**
* Performs a SQL query.
*
* @param sql the sql query
*/
protected void runSQL(String sql) {
try {
DataSource dataSource =
commerceSubscriptionEntryPersistence.getDataSource();
DB db = DBManagerUtil.getDB();
sql = db.buildSQL(sql);
sql = PortalUtil.transformSQL(sql);
SqlUpdate sqlUpdate = SqlUpdateFactoryUtil.getSqlUpdate(
dataSource, sql);
sqlUpdate.update();
}
catch (Exception exception) {
throw new SystemException(exception);
}
}
@BeanReference(
type = com.liferay.commerce.service.CommerceAddressLocalService.class
)
protected com.liferay.commerce.service.CommerceAddressLocalService
commerceAddressLocalService;
@BeanReference(
type = com.liferay.commerce.service.CommerceAddressService.class
)
protected com.liferay.commerce.service.CommerceAddressService
commerceAddressService;
@BeanReference(type = CommerceAddressPersistence.class)
protected CommerceAddressPersistence commerceAddressPersistence;
@BeanReference(
type = com.liferay.commerce.service.CommerceAddressRestrictionLocalService.class
)
protected
com.liferay.commerce.service.CommerceAddressRestrictionLocalService
commerceAddressRestrictionLocalService;
@BeanReference(
type = com.liferay.commerce.service.CommerceAddressRestrictionService.class
)
protected com.liferay.commerce.service.CommerceAddressRestrictionService
commerceAddressRestrictionService;
@BeanReference(type = CommerceAddressRestrictionPersistence.class)
protected CommerceAddressRestrictionPersistence
commerceAddressRestrictionPersistence;
@BeanReference(
type = com.liferay.commerce.service.CommerceAvailabilityEstimateLocalService.class
)
protected
com.liferay.commerce.service.CommerceAvailabilityEstimateLocalService
commerceAvailabilityEstimateLocalService;
@BeanReference(
type = com.liferay.commerce.service.CommerceAvailabilityEstimateService.class
)
protected com.liferay.commerce.service.CommerceAvailabilityEstimateService
commerceAvailabilityEstimateService;
@BeanReference(type = CommerceAvailabilityEstimatePersistence.class)
protected CommerceAvailabilityEstimatePersistence
commerceAvailabilityEstimatePersistence;
@BeanReference(
type = com.liferay.commerce.service.CommerceCountryLocalService.class
)
protected com.liferay.commerce.service.CommerceCountryLocalService
commerceCountryLocalService;
@BeanReference(
type = com.liferay.commerce.service.CommerceCountryService.class
)
protected com.liferay.commerce.service.CommerceCountryService
commerceCountryService;
@BeanReference(type = CommerceCountryPersistence.class)
protected CommerceCountryPersistence commerceCountryPersistence;
@BeanReference(type = CommerceCountryFinder.class)
protected CommerceCountryFinder commerceCountryFinder;
@BeanReference(
type = com.liferay.commerce.service.CommerceOrderLocalService.class
)
protected com.liferay.commerce.service.CommerceOrderLocalService
commerceOrderLocalService;
@BeanReference(
type = com.liferay.commerce.service.CommerceOrderService.class
)
protected com.liferay.commerce.service.CommerceOrderService
commerceOrderService;
@BeanReference(type = CommerceOrderPersistence.class)
protected CommerceOrderPersistence commerceOrderPersistence;
@BeanReference(type = CommerceOrderFinder.class)
protected CommerceOrderFinder commerceOrderFinder;
@BeanReference(
type = com.liferay.commerce.service.CommerceOrderItemLocalService.class
)
protected com.liferay.commerce.service.CommerceOrderItemLocalService
commerceOrderItemLocalService;
@BeanReference(
type = com.liferay.commerce.service.CommerceOrderItemService.class
)
protected com.liferay.commerce.service.CommerceOrderItemService
commerceOrderItemService;
@BeanReference(type = CommerceOrderItemPersistence.class)
protected CommerceOrderItemPersistence commerceOrderItemPersistence;
@BeanReference(type = CommerceOrderItemFinder.class)
protected CommerceOrderItemFinder commerceOrderItemFinder;
@BeanReference(
type = com.liferay.commerce.service.CommerceOrderNoteLocalService.class
)
protected com.liferay.commerce.service.CommerceOrderNoteLocalService
commerceOrderNoteLocalService;
@BeanReference(
type = com.liferay.commerce.service.CommerceOrderNoteService.class
)
protected com.liferay.commerce.service.CommerceOrderNoteService
commerceOrderNoteService;
@BeanReference(type = CommerceOrderNotePersistence.class)
protected CommerceOrderNotePersistence commerceOrderNotePersistence;
@BeanReference(
type = com.liferay.commerce.service.CommerceOrderPaymentLocalService.class
)
protected com.liferay.commerce.service.CommerceOrderPaymentLocalService
commerceOrderPaymentLocalService;
@BeanReference(type = CommerceOrderPaymentPersistence.class)
protected CommerceOrderPaymentPersistence commerceOrderPaymentPersistence;
@BeanReference(
type = com.liferay.commerce.service.CommerceRegionLocalService.class
)
protected com.liferay.commerce.service.CommerceRegionLocalService
commerceRegionLocalService;
@BeanReference(
type = com.liferay.commerce.service.CommerceRegionService.class
)
protected com.liferay.commerce.service.CommerceRegionService
commerceRegionService;
@BeanReference(type = CommerceRegionPersistence.class)
protected CommerceRegionPersistence commerceRegionPersistence;
@BeanReference(
type = com.liferay.commerce.service.CommerceShipmentLocalService.class
)
protected com.liferay.commerce.service.CommerceShipmentLocalService
commerceShipmentLocalService;
@BeanReference(
type = com.liferay.commerce.service.CommerceShipmentService.class
)
protected com.liferay.commerce.service.CommerceShipmentService
commerceShipmentService;
@BeanReference(type = CommerceShipmentPersistence.class)
protected CommerceShipmentPersistence commerceShipmentPersistence;
@BeanReference(type = CommerceShipmentFinder.class)
protected CommerceShipmentFinder commerceShipmentFinder;
@BeanReference(
type = com.liferay.commerce.service.CommerceShipmentItemLocalService.class
)
protected com.liferay.commerce.service.CommerceShipmentItemLocalService
commerceShipmentItemLocalService;
@BeanReference(
type = com.liferay.commerce.service.CommerceShipmentItemService.class
)
protected com.liferay.commerce.service.CommerceShipmentItemService
commerceShipmentItemService;
@BeanReference(type = CommerceShipmentItemPersistence.class)
protected CommerceShipmentItemPersistence commerceShipmentItemPersistence;
@BeanReference(type = CommerceShipmentItemFinder.class)
protected CommerceShipmentItemFinder commerceShipmentItemFinder;
@BeanReference(
type = com.liferay.commerce.service.CommerceShippingMethodLocalService.class
)
protected com.liferay.commerce.service.CommerceShippingMethodLocalService
commerceShippingMethodLocalService;
@BeanReference(
type = com.liferay.commerce.service.CommerceShippingMethodService.class
)
protected com.liferay.commerce.service.CommerceShippingMethodService
commerceShippingMethodService;
@BeanReference(type = CommerceShippingMethodPersistence.class)
protected CommerceShippingMethodPersistence
commerceShippingMethodPersistence;
@BeanReference(
type = com.liferay.commerce.service.CommerceSubscriptionEntryLocalService.class
)
protected com.liferay.commerce.service.CommerceSubscriptionEntryLocalService
commerceSubscriptionEntryLocalService;
@BeanReference(type = CommerceSubscriptionEntryService.class)
protected CommerceSubscriptionEntryService commerceSubscriptionEntryService;
@BeanReference(type = CommerceSubscriptionEntryPersistence.class)
protected CommerceSubscriptionEntryPersistence
commerceSubscriptionEntryPersistence;
@BeanReference(type = CommerceSubscriptionEntryFinder.class)
protected CommerceSubscriptionEntryFinder commerceSubscriptionEntryFinder;
@BeanReference(
type = com.liferay.commerce.service.CPDAvailabilityEstimateLocalService.class
)
protected com.liferay.commerce.service.CPDAvailabilityEstimateLocalService
cpdAvailabilityEstimateLocalService;
@BeanReference(
type = com.liferay.commerce.service.CPDAvailabilityEstimateService.class
)
protected com.liferay.commerce.service.CPDAvailabilityEstimateService
cpdAvailabilityEstimateService;
@BeanReference(type = CPDAvailabilityEstimatePersistence.class)
protected CPDAvailabilityEstimatePersistence
cpdAvailabilityEstimatePersistence;
@BeanReference(
type = com.liferay.commerce.service.CPDefinitionInventoryLocalService.class
)
protected com.liferay.commerce.service.CPDefinitionInventoryLocalService
cpDefinitionInventoryLocalService;
@BeanReference(
type = com.liferay.commerce.service.CPDefinitionInventoryService.class
)
protected com.liferay.commerce.service.CPDefinitionInventoryService
cpDefinitionInventoryService;
@BeanReference(type = CPDefinitionInventoryPersistence.class)
protected CPDefinitionInventoryPersistence cpDefinitionInventoryPersistence;
@ServiceReference(
type = com.liferay.counter.kernel.service.CounterLocalService.class
)
protected com.liferay.counter.kernel.service.CounterLocalService
counterLocalService;
@ServiceReference(
type = com.liferay.portal.kernel.service.ClassNameLocalService.class
)
protected com.liferay.portal.kernel.service.ClassNameLocalService
classNameLocalService;
@ServiceReference(
type = com.liferay.portal.kernel.service.ClassNameService.class
)
protected com.liferay.portal.kernel.service.ClassNameService
classNameService;
@ServiceReference(type = ClassNamePersistence.class)
protected ClassNamePersistence classNamePersistence;
@ServiceReference(
type = com.liferay.portal.kernel.service.ResourceLocalService.class
)
protected com.liferay.portal.kernel.service.ResourceLocalService
resourceLocalService;
@ServiceReference(
type = com.liferay.portal.kernel.service.UserLocalService.class
)
protected com.liferay.portal.kernel.service.UserLocalService
userLocalService;
@ServiceReference(
type = com.liferay.portal.kernel.service.UserService.class
)
protected com.liferay.portal.kernel.service.UserService userService;
@ServiceReference(type = UserPersistence.class)
protected UserPersistence userPersistence;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy