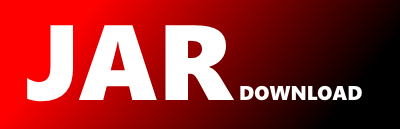
com.liferay.commerce.term.service.base.CommerceTermEntryLocalServiceBaseImpl Maven / Gradle / Ivy
Show all versions of com.liferay.commerce.term.service
/**
* SPDX-FileCopyrightText: (c) 2000 Liferay, Inc. https://liferay.com
* SPDX-License-Identifier: LGPL-2.1-or-later OR LicenseRef-Liferay-DXP-EULA-2.0.0-2023-06
*/
package com.liferay.commerce.term.service.base;
import com.liferay.commerce.term.model.CTermEntryLocalization;
import com.liferay.commerce.term.model.CommerceTermEntry;
import com.liferay.commerce.term.service.CommerceTermEntryLocalService;
import com.liferay.commerce.term.service.persistence.CTermEntryLocalizationPersistence;
import com.liferay.commerce.term.service.persistence.CommerceTermEntryPersistence;
import com.liferay.exportimport.kernel.lar.ExportImportHelperUtil;
import com.liferay.exportimport.kernel.lar.ManifestSummary;
import com.liferay.exportimport.kernel.lar.PortletDataContext;
import com.liferay.exportimport.kernel.lar.StagedModelDataHandler;
import com.liferay.exportimport.kernel.lar.StagedModelDataHandlerRegistryUtil;
import com.liferay.exportimport.kernel.lar.StagedModelDataHandlerUtil;
import com.liferay.exportimport.kernel.lar.StagedModelType;
import com.liferay.petra.sql.dsl.query.DSLQuery;
import com.liferay.portal.aop.AopService;
import com.liferay.portal.kernel.dao.db.DB;
import com.liferay.portal.kernel.dao.db.DBManagerUtil;
import com.liferay.portal.kernel.dao.jdbc.SqlUpdate;
import com.liferay.portal.kernel.dao.jdbc.SqlUpdateFactoryUtil;
import com.liferay.portal.kernel.dao.orm.ActionableDynamicQuery;
import com.liferay.portal.kernel.dao.orm.Criterion;
import com.liferay.portal.kernel.dao.orm.DefaultActionableDynamicQuery;
import com.liferay.portal.kernel.dao.orm.Disjunction;
import com.liferay.portal.kernel.dao.orm.DynamicQuery;
import com.liferay.portal.kernel.dao.orm.DynamicQueryFactoryUtil;
import com.liferay.portal.kernel.dao.orm.ExportActionableDynamicQuery;
import com.liferay.portal.kernel.dao.orm.IndexableActionableDynamicQuery;
import com.liferay.portal.kernel.dao.orm.Projection;
import com.liferay.portal.kernel.dao.orm.Property;
import com.liferay.portal.kernel.dao.orm.PropertyFactoryUtil;
import com.liferay.portal.kernel.dao.orm.RestrictionsFactoryUtil;
import com.liferay.portal.kernel.exception.PortalException;
import com.liferay.portal.kernel.exception.SystemException;
import com.liferay.portal.kernel.log.Log;
import com.liferay.portal.kernel.log.LogFactoryUtil;
import com.liferay.portal.kernel.model.PersistedModel;
import com.liferay.portal.kernel.module.framework.service.IdentifiableOSGiService;
import com.liferay.portal.kernel.search.Indexable;
import com.liferay.portal.kernel.search.IndexableType;
import com.liferay.portal.kernel.service.BaseLocalServiceImpl;
import com.liferay.portal.kernel.service.PersistedModelLocalService;
import com.liferay.portal.kernel.service.persistence.BasePersistence;
import com.liferay.portal.kernel.transaction.Transactional;
import com.liferay.portal.kernel.util.OrderByComparator;
import com.liferay.portal.kernel.util.PortalUtil;
import com.liferay.portal.kernel.workflow.WorkflowConstants;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.sql.DataSource;
import org.osgi.service.component.annotations.Deactivate;
import org.osgi.service.component.annotations.Reference;
/**
* Provides the base implementation for the commerce term entry local service.
*
*
* This implementation exists only as a container for the default service methods generated by ServiceBuilder. All custom service methods should be put in {@link com.liferay.commerce.term.service.impl.CommerceTermEntryLocalServiceImpl}.
*
*
* @author Luca Pellizzon
* @see com.liferay.commerce.term.service.impl.CommerceTermEntryLocalServiceImpl
* @generated
*/
public abstract class CommerceTermEntryLocalServiceBaseImpl
extends BaseLocalServiceImpl
implements AopService, CommerceTermEntryLocalService,
IdentifiableOSGiService {
/*
* NOTE FOR DEVELOPERS:
*
* Never modify or reference this class directly. Use CommerceTermEntryLocalService
via injection or a org.osgi.util.tracker.ServiceTracker
or use com.liferay.commerce.term.service.CommerceTermEntryLocalServiceUtil
.
*/
/**
* Adds the commerce term entry to the database. Also notifies the appropriate model listeners.
*
*
* Important: Inspect CommerceTermEntryLocalServiceImpl for overloaded versions of the method. If provided, use these entry points to the API, as the implementation logic may require the additional parameters defined there.
*
*
* @param commerceTermEntry the commerce term entry
* @return the commerce term entry that was added
*/
@Indexable(type = IndexableType.REINDEX)
@Override
public CommerceTermEntry addCommerceTermEntry(
CommerceTermEntry commerceTermEntry) {
commerceTermEntry.setNew(true);
return commerceTermEntryPersistence.update(commerceTermEntry);
}
/**
* Creates a new commerce term entry with the primary key. Does not add the commerce term entry to the database.
*
* @param commerceTermEntryId the primary key for the new commerce term entry
* @return the new commerce term entry
*/
@Override
@Transactional(enabled = false)
public CommerceTermEntry createCommerceTermEntry(long commerceTermEntryId) {
return commerceTermEntryPersistence.create(commerceTermEntryId);
}
/**
* Deletes the commerce term entry with the primary key from the database. Also notifies the appropriate model listeners.
*
*
* Important: Inspect CommerceTermEntryLocalServiceImpl for overloaded versions of the method. If provided, use these entry points to the API, as the implementation logic may require the additional parameters defined there.
*
*
* @param commerceTermEntryId the primary key of the commerce term entry
* @return the commerce term entry that was removed
* @throws PortalException if a commerce term entry with the primary key could not be found
*/
@Indexable(type = IndexableType.DELETE)
@Override
public CommerceTermEntry deleteCommerceTermEntry(long commerceTermEntryId)
throws PortalException {
return commerceTermEntryPersistence.remove(commerceTermEntryId);
}
/**
* Deletes the commerce term entry from the database. Also notifies the appropriate model listeners.
*
*
* Important: Inspect CommerceTermEntryLocalServiceImpl for overloaded versions of the method. If provided, use these entry points to the API, as the implementation logic may require the additional parameters defined there.
*
*
* @param commerceTermEntry the commerce term entry
* @return the commerce term entry that was removed
* @throws PortalException
*/
@Indexable(type = IndexableType.DELETE)
@Override
public CommerceTermEntry deleteCommerceTermEntry(
CommerceTermEntry commerceTermEntry)
throws PortalException {
return commerceTermEntryPersistence.remove(commerceTermEntry);
}
@Override
public T dslQuery(DSLQuery dslQuery) {
return commerceTermEntryPersistence.dslQuery(dslQuery);
}
@Override
public int dslQueryCount(DSLQuery dslQuery) {
Long count = dslQuery(dslQuery);
return count.intValue();
}
@Override
public DynamicQuery dynamicQuery() {
Class> clazz = getClass();
return DynamicQueryFactoryUtil.forClass(
CommerceTermEntry.class, clazz.getClassLoader());
}
/**
* Performs a dynamic query on the database and returns the matching rows.
*
* @param dynamicQuery the dynamic query
* @return the matching rows
*/
@Override
public List dynamicQuery(DynamicQuery dynamicQuery) {
return commerceTermEntryPersistence.findWithDynamicQuery(dynamicQuery);
}
/**
* Performs a dynamic query on the database and returns a range of the matching rows.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to com.liferay.portal.kernel.dao.orm.QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from com.liferay.commerce.term.model.impl.CommerceTermEntryModelImpl
.
*
*
* @param dynamicQuery the dynamic query
* @param start the lower bound of the range of model instances
* @param end the upper bound of the range of model instances (not inclusive)
* @return the range of matching rows
*/
@Override
public List dynamicQuery(
DynamicQuery dynamicQuery, int start, int end) {
return commerceTermEntryPersistence.findWithDynamicQuery(
dynamicQuery, start, end);
}
/**
* Performs a dynamic query on the database and returns an ordered range of the matching rows.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to com.liferay.portal.kernel.dao.orm.QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from com.liferay.commerce.term.model.impl.CommerceTermEntryModelImpl
.
*
*
* @param dynamicQuery the dynamic query
* @param start the lower bound of the range of model instances
* @param end the upper bound of the range of model instances (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching rows
*/
@Override
public List dynamicQuery(
DynamicQuery dynamicQuery, int start, int end,
OrderByComparator orderByComparator) {
return commerceTermEntryPersistence.findWithDynamicQuery(
dynamicQuery, start, end, orderByComparator);
}
/**
* Returns the number of rows matching the dynamic query.
*
* @param dynamicQuery the dynamic query
* @return the number of rows matching the dynamic query
*/
@Override
public long dynamicQueryCount(DynamicQuery dynamicQuery) {
return commerceTermEntryPersistence.countWithDynamicQuery(dynamicQuery);
}
/**
* Returns the number of rows matching the dynamic query.
*
* @param dynamicQuery the dynamic query
* @param projection the projection to apply to the query
* @return the number of rows matching the dynamic query
*/
@Override
public long dynamicQueryCount(
DynamicQuery dynamicQuery, Projection projection) {
return commerceTermEntryPersistence.countWithDynamicQuery(
dynamicQuery, projection);
}
@Override
public CommerceTermEntry fetchCommerceTermEntry(long commerceTermEntryId) {
return commerceTermEntryPersistence.fetchByPrimaryKey(
commerceTermEntryId);
}
/**
* Returns the commerce term entry with the matching UUID and company.
*
* @param uuid the commerce term entry's UUID
* @param companyId the primary key of the company
* @return the matching commerce term entry, or null
if a matching commerce term entry could not be found
*/
@Override
public CommerceTermEntry fetchCommerceTermEntryByUuidAndCompanyId(
String uuid, long companyId) {
return commerceTermEntryPersistence.fetchByUuid_C_First(
uuid, companyId, null);
}
@Override
public CommerceTermEntry fetchCommerceTermEntryByExternalReferenceCode(
String externalReferenceCode, long companyId) {
return commerceTermEntryPersistence.fetchByERC_C(
externalReferenceCode, companyId);
}
@Override
public CommerceTermEntry getCommerceTermEntryByExternalReferenceCode(
String externalReferenceCode, long companyId)
throws PortalException {
return commerceTermEntryPersistence.findByERC_C(
externalReferenceCode, companyId);
}
/**
* Returns the commerce term entry with the primary key.
*
* @param commerceTermEntryId the primary key of the commerce term entry
* @return the commerce term entry
* @throws PortalException if a commerce term entry with the primary key could not be found
*/
@Override
public CommerceTermEntry getCommerceTermEntry(long commerceTermEntryId)
throws PortalException {
return commerceTermEntryPersistence.findByPrimaryKey(
commerceTermEntryId);
}
@Override
public ActionableDynamicQuery getActionableDynamicQuery() {
ActionableDynamicQuery actionableDynamicQuery =
new DefaultActionableDynamicQuery();
actionableDynamicQuery.setBaseLocalService(
commerceTermEntryLocalService);
actionableDynamicQuery.setClassLoader(getClassLoader());
actionableDynamicQuery.setModelClass(CommerceTermEntry.class);
actionableDynamicQuery.setPrimaryKeyPropertyName("commerceTermEntryId");
return actionableDynamicQuery;
}
@Override
public IndexableActionableDynamicQuery
getIndexableActionableDynamicQuery() {
IndexableActionableDynamicQuery indexableActionableDynamicQuery =
new IndexableActionableDynamicQuery();
indexableActionableDynamicQuery.setBaseLocalService(
commerceTermEntryLocalService);
indexableActionableDynamicQuery.setClassLoader(getClassLoader());
indexableActionableDynamicQuery.setModelClass(CommerceTermEntry.class);
indexableActionableDynamicQuery.setPrimaryKeyPropertyName(
"commerceTermEntryId");
return indexableActionableDynamicQuery;
}
protected void initActionableDynamicQuery(
ActionableDynamicQuery actionableDynamicQuery) {
actionableDynamicQuery.setBaseLocalService(
commerceTermEntryLocalService);
actionableDynamicQuery.setClassLoader(getClassLoader());
actionableDynamicQuery.setModelClass(CommerceTermEntry.class);
actionableDynamicQuery.setPrimaryKeyPropertyName("commerceTermEntryId");
}
@Override
public ExportActionableDynamicQuery getExportActionableDynamicQuery(
final PortletDataContext portletDataContext) {
final ExportActionableDynamicQuery exportActionableDynamicQuery =
new ExportActionableDynamicQuery() {
@Override
public long performCount() throws PortalException {
ManifestSummary manifestSummary =
portletDataContext.getManifestSummary();
StagedModelType stagedModelType = getStagedModelType();
long modelAdditionCount = super.performCount();
manifestSummary.addModelAdditionCount(
stagedModelType, modelAdditionCount);
long modelDeletionCount =
ExportImportHelperUtil.getModelDeletionCount(
portletDataContext, stagedModelType);
manifestSummary.addModelDeletionCount(
stagedModelType, modelDeletionCount);
return modelAdditionCount;
}
};
initActionableDynamicQuery(exportActionableDynamicQuery);
exportActionableDynamicQuery.setAddCriteriaMethod(
new ActionableDynamicQuery.AddCriteriaMethod() {
@Override
public void addCriteria(DynamicQuery dynamicQuery) {
Criterion modifiedDateCriterion =
portletDataContext.getDateRangeCriteria("modifiedDate");
Criterion statusDateCriterion =
portletDataContext.getDateRangeCriteria("statusDate");
if ((modifiedDateCriterion != null) &&
(statusDateCriterion != null)) {
Disjunction disjunction =
RestrictionsFactoryUtil.disjunction();
disjunction.add(modifiedDateCriterion);
disjunction.add(statusDateCriterion);
dynamicQuery.add(disjunction);
}
Property workflowStatusProperty =
PropertyFactoryUtil.forName("status");
if (portletDataContext.isInitialPublication()) {
dynamicQuery.add(
workflowStatusProperty.ne(
WorkflowConstants.STATUS_IN_TRASH));
}
else {
StagedModelDataHandler> stagedModelDataHandler =
StagedModelDataHandlerRegistryUtil.
getStagedModelDataHandler(
CommerceTermEntry.class.getName());
dynamicQuery.add(
workflowStatusProperty.in(
stagedModelDataHandler.
getExportableStatuses()));
}
}
});
exportActionableDynamicQuery.setCompanyId(
portletDataContext.getCompanyId());
exportActionableDynamicQuery.setPerformActionMethod(
new ActionableDynamicQuery.PerformActionMethod
() {
@Override
public void performAction(CommerceTermEntry commerceTermEntry)
throws PortalException {
StagedModelDataHandlerUtil.exportStagedModel(
portletDataContext, commerceTermEntry);
}
});
exportActionableDynamicQuery.setStagedModelType(
new StagedModelType(
PortalUtil.getClassNameId(CommerceTermEntry.class.getName())));
return exportActionableDynamicQuery;
}
/**
* @throws PortalException
*/
@Override
public PersistedModel createPersistedModel(Serializable primaryKeyObj)
throws PortalException {
return commerceTermEntryPersistence.create(
((Long)primaryKeyObj).longValue());
}
/**
* @throws PortalException
*/
@Override
public PersistedModel deletePersistedModel(PersistedModel persistedModel)
throws PortalException {
if (_log.isWarnEnabled()) {
_log.warn(
"Implement CommerceTermEntryLocalServiceImpl#deleteCommerceTermEntry(CommerceTermEntry) to avoid orphaned data");
}
return commerceTermEntryLocalService.deleteCommerceTermEntry(
(CommerceTermEntry)persistedModel);
}
@Override
public BasePersistence getBasePersistence() {
return commerceTermEntryPersistence;
}
/**
* @throws PortalException
*/
@Override
public PersistedModel getPersistedModel(Serializable primaryKeyObj)
throws PortalException {
return commerceTermEntryPersistence.findByPrimaryKey(primaryKeyObj);
}
/**
* Returns the commerce term entry with the matching UUID and company.
*
* @param uuid the commerce term entry's UUID
* @param companyId the primary key of the company
* @return the matching commerce term entry
* @throws PortalException if a matching commerce term entry could not be found
*/
@Override
public CommerceTermEntry getCommerceTermEntryByUuidAndCompanyId(
String uuid, long companyId)
throws PortalException {
return commerceTermEntryPersistence.findByUuid_C_First(
uuid, companyId, null);
}
/**
* Returns a range of all the commerce term entries.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to com.liferay.portal.kernel.dao.orm.QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from com.liferay.commerce.term.model.impl.CommerceTermEntryModelImpl
.
*
*
* @param start the lower bound of the range of commerce term entries
* @param end the upper bound of the range of commerce term entries (not inclusive)
* @return the range of commerce term entries
*/
@Override
public List getCommerceTermEntries(int start, int end) {
return commerceTermEntryPersistence.findAll(start, end);
}
/**
* Returns the number of commerce term entries.
*
* @return the number of commerce term entries
*/
@Override
public int getCommerceTermEntriesCount() {
return commerceTermEntryPersistence.countAll();
}
/**
* Updates the commerce term entry in the database or adds it if it does not yet exist. Also notifies the appropriate model listeners.
*
*
* Important: Inspect CommerceTermEntryLocalServiceImpl for overloaded versions of the method. If provided, use these entry points to the API, as the implementation logic may require the additional parameters defined there.
*
*
* @param commerceTermEntry the commerce term entry
* @return the commerce term entry that was updated
*/
@Indexable(type = IndexableType.REINDEX)
@Override
public CommerceTermEntry updateCommerceTermEntry(
CommerceTermEntry commerceTermEntry) {
return commerceTermEntryPersistence.update(commerceTermEntry);
}
@Override
public CTermEntryLocalization fetchCTermEntryLocalization(
long commerceTermEntryId, String languageId) {
return cTermEntryLocalizationPersistence.
fetchByCommerceTermEntryId_LanguageId(
commerceTermEntryId, languageId);
}
@Override
public CTermEntryLocalization getCTermEntryLocalization(
long commerceTermEntryId, String languageId)
throws PortalException {
return cTermEntryLocalizationPersistence.
findByCommerceTermEntryId_LanguageId(
commerceTermEntryId, languageId);
}
@Override
public List getCTermEntryLocalizations(
long commerceTermEntryId) {
return cTermEntryLocalizationPersistence.findByCommerceTermEntryId(
commerceTermEntryId);
}
@Override
public CTermEntryLocalization updateCTermEntryLocalization(
CommerceTermEntry commerceTermEntry, String languageId,
String description, String label)
throws PortalException {
commerceTermEntry = commerceTermEntryPersistence.findByPrimaryKey(
commerceTermEntry.getPrimaryKey());
CTermEntryLocalization cTermEntryLocalization =
cTermEntryLocalizationPersistence.
fetchByCommerceTermEntryId_LanguageId(
commerceTermEntry.getCommerceTermEntryId(), languageId);
return _updateCTermEntryLocalization(
commerceTermEntry, cTermEntryLocalization, languageId, description,
label);
}
@Override
public List updateCTermEntryLocalizations(
CommerceTermEntry commerceTermEntry,
Map descriptionMap, Map labelMap)
throws PortalException {
commerceTermEntry = commerceTermEntryPersistence.findByPrimaryKey(
commerceTermEntry.getPrimaryKey());
Map localizedValuesMap =
new HashMap();
for (Map.Entry entry : descriptionMap.entrySet()) {
String languageId = entry.getKey();
String[] localizedValues = localizedValuesMap.get(languageId);
if (localizedValues == null) {
localizedValues = new String[2];
localizedValuesMap.put(languageId, localizedValues);
}
localizedValues[0] = entry.getValue();
}
for (Map.Entry entry : labelMap.entrySet()) {
String languageId = entry.getKey();
String[] localizedValues = localizedValuesMap.get(languageId);
if (localizedValues == null) {
localizedValues = new String[2];
localizedValuesMap.put(languageId, localizedValues);
}
localizedValues[1] = entry.getValue();
}
List cTermEntryLocalizations =
new ArrayList(localizedValuesMap.size());
for (CTermEntryLocalization cTermEntryLocalization :
cTermEntryLocalizationPersistence.findByCommerceTermEntryId(
commerceTermEntry.getCommerceTermEntryId())) {
String[] localizedValues = localizedValuesMap.remove(
cTermEntryLocalization.getLanguageId());
if (localizedValues == null) {
cTermEntryLocalizationPersistence.remove(
cTermEntryLocalization);
}
else {
cTermEntryLocalization.setCompanyId(
commerceTermEntry.getCompanyId());
cTermEntryLocalization.setDescription(localizedValues[0]);
cTermEntryLocalization.setLabel(localizedValues[1]);
cTermEntryLocalizations.add(
cTermEntryLocalizationPersistence.update(
cTermEntryLocalization));
}
}
long batchCounter =
counterLocalService.increment(
CTermEntryLocalization.class.getName(),
localizedValuesMap.size()) - localizedValuesMap.size();
for (Map.Entry entry :
localizedValuesMap.entrySet()) {
String languageId = entry.getKey();
String[] localizedValues = entry.getValue();
CTermEntryLocalization cTermEntryLocalization =
cTermEntryLocalizationPersistence.create(++batchCounter);
cTermEntryLocalization.setCommerceTermEntryId(
commerceTermEntry.getCommerceTermEntryId());
cTermEntryLocalization.setCompanyId(
commerceTermEntry.getCompanyId());
cTermEntryLocalization.setLanguageId(languageId);
cTermEntryLocalization.setDescription(localizedValues[0]);
cTermEntryLocalization.setLabel(localizedValues[1]);
cTermEntryLocalizations.add(
cTermEntryLocalizationPersistence.update(
cTermEntryLocalization));
}
return cTermEntryLocalizations;
}
private CTermEntryLocalization _updateCTermEntryLocalization(
CommerceTermEntry commerceTermEntry,
CTermEntryLocalization cTermEntryLocalization, String languageId,
String description, String label)
throws PortalException {
if (cTermEntryLocalization == null) {
long cTermEntryLocalizationId = counterLocalService.increment(
CTermEntryLocalization.class.getName());
cTermEntryLocalization = cTermEntryLocalizationPersistence.create(
cTermEntryLocalizationId);
cTermEntryLocalization.setCommerceTermEntryId(
commerceTermEntry.getCommerceTermEntryId());
cTermEntryLocalization.setLanguageId(languageId);
}
cTermEntryLocalization.setCompanyId(commerceTermEntry.getCompanyId());
cTermEntryLocalization.setDescription(description);
cTermEntryLocalization.setLabel(label);
return cTermEntryLocalizationPersistence.update(cTermEntryLocalization);
}
@Deactivate
protected void deactivate() {
}
@Override
public Class>[] getAopInterfaces() {
return new Class>[] {
CommerceTermEntryLocalService.class, IdentifiableOSGiService.class,
PersistedModelLocalService.class
};
}
@Override
public void setAopProxy(Object aopProxy) {
commerceTermEntryLocalService = (CommerceTermEntryLocalService)aopProxy;
}
/**
* Returns the OSGi service identifier.
*
* @return the OSGi service identifier
*/
@Override
public String getOSGiServiceIdentifier() {
return CommerceTermEntryLocalService.class.getName();
}
protected Class> getModelClass() {
return CommerceTermEntry.class;
}
protected String getModelClassName() {
return CommerceTermEntry.class.getName();
}
/**
* Performs a SQL query.
*
* @param sql the sql query
*/
protected void runSQL(String sql) {
try {
DataSource dataSource =
commerceTermEntryPersistence.getDataSource();
DB db = DBManagerUtil.getDB();
sql = db.buildSQL(sql);
sql = PortalUtil.transformSQL(sql);
SqlUpdate sqlUpdate = SqlUpdateFactoryUtil.getSqlUpdate(
dataSource, sql);
sqlUpdate.update();
}
catch (Exception exception) {
throw new SystemException(exception);
}
}
protected CommerceTermEntryLocalService commerceTermEntryLocalService;
@Reference
protected CommerceTermEntryPersistence commerceTermEntryPersistence;
@Reference
protected com.liferay.counter.kernel.service.CounterLocalService
counterLocalService;
@Reference
protected CTermEntryLocalizationPersistence
cTermEntryLocalizationPersistence;
private static final Log _log = LogFactoryUtil.getLog(
CommerceTermEntryLocalServiceBaseImpl.class);
}