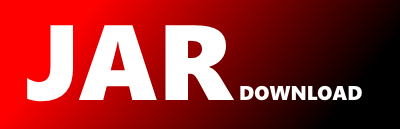
com.liferay.portal.kernel.concurrent.BatchablePipe Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.liferay.portal.kernel Show documentation
Show all versions of com.liferay.portal.kernel Show documentation
Contains interfaces for the portal services. Interfaces are only loaded by the global class loader and are shared by all plugins.
/**
* Copyright (c) 2000-present Liferay, Inc. All rights reserved.
*
* This library is free software; you can redistribute it and/or modify it under
* the terms of the GNU Lesser General Public License as published by the Free
* Software Foundation; either version 2.1 of the License, or (at your option)
* any later version.
*
* This library is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License for more
* details.
*/
package com.liferay.portal.kernel.concurrent;
import java.util.Queue;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.ConcurrentLinkedQueue;
import java.util.concurrent.ConcurrentMap;
/**
* @author Shuyang Zhou
*/
public class BatchablePipe {
public boolean put(IncreasableEntry increasableEntry) {
K key = increasableEntry.getKey();
while (true) {
IncreasableEntryWrapper previousIncreasableEntryWrapper =
concurrentMap.putIfAbsent(
key, new IncreasableEntryWrapper(increasableEntry));
if (previousIncreasableEntryWrapper == null) {
queue.offer(increasableEntry);
return true;
}
IncreasableEntry previousIncreasableEntry =
previousIncreasableEntryWrapper.increasableEntry;
IncreasableEntry newIncreasableEntry =
increasableEntry.increase(previousIncreasableEntry.getValue());
if (concurrentMap.replace(
key, previousIncreasableEntryWrapper,
new IncreasableEntryWrapper(newIncreasableEntry))) {
queue.offer(newIncreasableEntry);
return false;
}
}
}
public IncreasableEntry take() {
while (true) {
IncreasableEntry increasableEntry = queue.poll();
if (increasableEntry == null) {
return null;
}
if (concurrentMap.remove(
increasableEntry.getKey(),
new IncreasableEntryWrapper(increasableEntry))) {
return increasableEntry;
}
}
}
protected final ConcurrentMap>
concurrentMap = new ConcurrentHashMap<>();
protected final Queue> queue =
new ConcurrentLinkedQueue<>();
protected static class IncreasableEntryWrapper {
public IncreasableEntryWrapper(
IncreasableEntry increasableEntry) {
this.increasableEntry = increasableEntry;
}
@Override
public boolean equals(Object obj) {
IncreasableEntryWrapper increasableEntryWrapper =
(IncreasableEntryWrapper)obj;
if (increasableEntry == increasableEntryWrapper.increasableEntry) {
return true;
}
return false;
}
@Override
public int hashCode() {
return increasableEntry.hashCode();
}
@Override
public String toString() {
return increasableEntry.toString();
}
protected final IncreasableEntry increasableEntry;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy