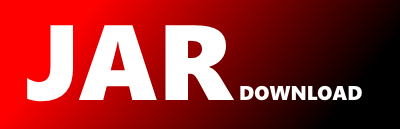
com.liferay.portal.kernel.template.TemplateManagerUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.liferay.portal.kernel Show documentation
Show all versions of com.liferay.portal.kernel Show documentation
Contains interfaces for the portal services. Interfaces are only loaded by the global class loader and are shared by all plugins.
/**
* Copyright (c) 2000-present Liferay, Inc. All rights reserved.
*
* This library is free software; you can redistribute it and/or modify it under
* the terms of the GNU Lesser General Public License as published by the Free
* Software Foundation; either version 2.1 of the License, or (at your option)
* any later version.
*
* This library is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License for more
* details.
*/
package com.liferay.portal.kernel.template;
import com.liferay.portal.kernel.configuration.Filter;
import com.liferay.portal.kernel.log.Log;
import com.liferay.portal.kernel.log.LogFactoryUtil;
import com.liferay.portal.kernel.util.PropsUtil;
import com.liferay.portal.kernel.util.Validator;
import com.liferay.registry.Registry;
import com.liferay.registry.RegistryUtil;
import com.liferay.registry.ServiceReference;
import com.liferay.registry.ServiceTracker;
import com.liferay.registry.ServiceTrackerCustomizer;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.concurrent.ConcurrentHashMap;
/**
* @author Tina Tian
* @author Raymond Augé
*/
public class TemplateManagerUtil {
public static void destroy() {
_instance._destroy();
}
public static void destroy(ClassLoader classLoader) {
_instance._destroy(classLoader);
}
public static Set getSupportedLanguageTypes(String propertyKey) {
return _instance._getSupportedLanguageTypes(propertyKey);
}
public static Template getTemplate(
String templateManagerName,
List templateResources, boolean restricted)
throws TemplateException {
return _instance._getTemplate(
templateManagerName, templateResources, restricted);
}
public static Template getTemplate(
String templateManagerName,
List templateResources,
TemplateResource errorTemplateResource, boolean restricted)
throws TemplateException {
return _instance._getTemplate(
templateManagerName, templateResources, errorTemplateResource,
restricted);
}
public static Template getTemplate(
String templateManagerName, TemplateResource templateResource,
boolean restricted)
throws TemplateException {
return _instance._getTemplate(
templateManagerName, templateResource, restricted);
}
public static Template getTemplate(
String templateManagerName, TemplateResource templateResource,
TemplateResource errorTemplateResource, boolean restricted)
throws TemplateException {
return _instance._getTemplate(
templateManagerName, templateResource, errorTemplateResource,
restricted);
}
public static TemplateManager getTemplateManager(
String templateManagerName) {
return _instance._getTemplateManager(templateManagerName);
}
public static Set getTemplateManagerNames() {
return _instance._getTemplateManagerNames();
}
public static Map getTemplateManagers() {
return _instance._getTemplateManagers();
}
public static boolean hasTemplateManager(String templateManagerName) {
return _instance._hasTemplateManager(templateManagerName);
}
private TemplateManagerUtil() {
Registry registry = RegistryUtil.getRegistry();
com.liferay.registry.Filter filter = registry.getFilter(
"(&(language.type=*)(objectClass=" +
TemplateManager.class.getName() + "))");
_serviceTracker = registry.trackServices(
filter, new TemplateManagerServiceTrackerCustomizer());
_serviceTracker.open();
}
private void _destroy() {
Map templateManagers = _getTemplateManagers();
for (TemplateManager templateManager : templateManagers.values()) {
templateManager.destroy();
}
templateManagers.clear();
}
private void _destroy(ClassLoader classLoader) {
Map templateManagers = _getTemplateManagers();
for (TemplateManager templateManager : templateManagers.values()) {
templateManager.destroy(classLoader);
}
}
private Set _getSupportedLanguageTypes(String propertyKey) {
Set supportedLanguageTypes = _supportedLanguageTypes.get(
propertyKey);
if (supportedLanguageTypes != null) {
return supportedLanguageTypes;
}
Map templateManagers = _getTemplateManagers();
supportedLanguageTypes = new HashSet<>();
for (String templateManagerName : templateManagers.keySet()) {
String content = PropsUtil.get(
propertyKey, new Filter(templateManagerName));
if (Validator.isNotNull(content)) {
supportedLanguageTypes.add(templateManagerName);
}
}
supportedLanguageTypes = Collections.unmodifiableSet(
supportedLanguageTypes);
_supportedLanguageTypes.put(propertyKey, supportedLanguageTypes);
return supportedLanguageTypes;
}
private Template _getTemplate(
String templateManagerName,
List templateResources, boolean restricted)
throws TemplateException {
TemplateManager templateManager = _getTemplateManagerChecked(
templateManagerName);
return templateManager.getTemplate(templateResources, restricted);
}
private Template _getTemplate(
String templateManagerName,
List templateResources,
TemplateResource errorTemplateResource, boolean restricted)
throws TemplateException {
TemplateManager templateManager = _getTemplateManagerChecked(
templateManagerName);
return templateManager.getTemplate(
templateResources, errorTemplateResource, restricted);
}
private Template _getTemplate(
String templateManagerName, TemplateResource templateResource,
boolean restricted)
throws TemplateException {
TemplateManager templateManager = _getTemplateManagerChecked(
templateManagerName);
return templateManager.getTemplate(templateResource, restricted);
}
private Template _getTemplate(
String templateManagerName, TemplateResource templateResource,
TemplateResource errorTemplateResource, boolean restricted)
throws TemplateException {
TemplateManager templateManager = _getTemplateManagerChecked(
templateManagerName);
return templateManager.getTemplate(
templateResource, errorTemplateResource, restricted);
}
private TemplateManager _getTemplateManager(String templateManagerName) {
Collection templateManagers =
_templateManagers.values();
for (TemplateManager templateManager : templateManagers) {
if (templateManagerName.equals(templateManager.getName())) {
return templateManager;
}
}
return null;
}
private TemplateManager _getTemplateManagerChecked(
String templateManagerName)
throws TemplateException {
Collection templateManagers =
_templateManagers.values();
for (TemplateManager templateManager : templateManagers) {
if (templateManagerName.equals(templateManager.getName())) {
return templateManager;
}
}
throw new TemplateException(
"Unsupported template manager " + templateManagerName);
}
private Set _getTemplateManagerNames() {
Map templateManagers = _getTemplateManagers();
return templateManagers.keySet();
}
private Map _getTemplateManagers() {
Map map = new HashMap<>();
Collection templateManagers =
_templateManagers.values();
for (TemplateManager templateManager : templateManagers) {
map.put(templateManager.getName(), templateManager);
}
return Collections.unmodifiableMap(map);
}
private boolean _hasTemplateManager(String templateManagerName) {
Collection templateManagers =
_templateManagers.values();
for (TemplateManager templateManager : templateManagers) {
if (templateManagerName.equals(templateManager.getName())) {
return true;
}
}
return false;
}
private static final Log _log = LogFactoryUtil.getLog(
TemplateManagerUtil.class);
private static final TemplateManagerUtil _instance =
new TemplateManagerUtil();
private final ServiceTracker
_serviceTracker;
private final Map> _supportedLanguageTypes =
new ConcurrentHashMap<>();
private final Map, TemplateManager>
_templateManagers = new ConcurrentHashMap<>();
private class TemplateManagerServiceTrackerCustomizer
implements ServiceTrackerCustomizer {
@Override
public TemplateManager addingService(
ServiceReference serviceReference) {
Registry registry = RegistryUtil.getRegistry();
TemplateManager templateManager = registry.getService(
serviceReference);
String name = templateManager.getName();
try {
templateManager.init();
_templateManagers.put(serviceReference, templateManager);
}
catch (TemplateException te) {
if (_log.isWarnEnabled()) {
_log.warn(
"unable to init " + name + " Template Manager ", te);
}
}
return templateManager;
}
@Override
public void modifiedService(
ServiceReference serviceReference,
TemplateManager templateManager) {
}
@Override
public void removedService(
ServiceReference serviceReference,
TemplateManager templateManager) {
Registry registry = RegistryUtil.getRegistry();
registry.ungetService(serviceReference);
_templateManagers.remove(serviceReference);
templateManager.destroy();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy