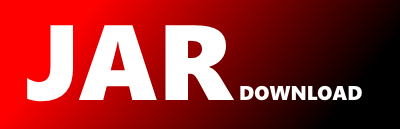
com.liferay.portal.kernel.theme.NavItem Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.liferay.portal.kernel Show documentation
Show all versions of com.liferay.portal.kernel Show documentation
Contains interfaces for the portal services. Interfaces are only loaded by the global class loader and are shared by all plugins.
/**
* Copyright (c) 2000-present Liferay, Inc. All rights reserved.
*
* This library is free software; you can redistribute it and/or modify it under
* the terms of the GNU Lesser General Public License as published by the Free
* Software Foundation; either version 2.1 of the License, or (at your option)
* any later version.
*
* This library is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License for more
* details.
*/
package com.liferay.portal.kernel.theme;
import com.liferay.portal.kernel.exception.PortalException;
import com.liferay.portal.kernel.model.Layout;
import com.liferay.portal.kernel.model.LayoutType;
import com.liferay.portal.kernel.util.HtmlUtil;
import com.liferay.portal.kernel.util.ListUtil;
import com.liferay.portal.kernel.util.PortalUtil;
import com.liferay.portal.kernel.util.PredicateFilter;
import com.liferay.portal.kernel.util.StringBundler;
import com.liferay.portal.kernel.util.StringPool;
import com.liferay.portal.kernel.util.StringUtil;
import com.liferay.portal.kernel.util.Validator;
import com.liferay.portal.kernel.util.WebKeys;
import com.liferay.portal.kernel.webserver.WebServerServletTokenUtil;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import javax.servlet.http.HttpServletRequest;
/**
* Represents a portal navigation item, providing access to layouts and metadata
* from templates, which can be found in a theme's
* portal-normal.vm
.
*
* @author Brian Wing Shun Chan
* @author Shuyang Zhou
*/
public class NavItem implements Serializable {
/**
* Creates a single level of navigation items from the layouts. Navigation
* items for nested layouts are only created when they are accessed.
*
*
* No permission checks are performed in this method. Permissions of child
* layouts are honored when accessing them via {@link #getChildren()}.
*
*
* @param request the currently served {@link HttpServletRequest}
* @param layouts the layouts from which to create the navigation items
* @return a single level of navigation items from the layouts, or
* null
if the collection of layouts was
* null
.
*/
public static List fromLayouts(
HttpServletRequest request, List layouts,
Map contextObjects) {
if (layouts == null) {
return null;
}
List navItems = new ArrayList<>(layouts.size());
for (Layout layout : layouts) {
navItems.add(new NavItem(request, layout, contextObjects));
}
return navItems;
}
public NavItem(
HttpServletRequest request, Layout layout,
Map contextObjects) {
_request = request;
_themeDisplay = (ThemeDisplay)request.getAttribute(
WebKeys.THEME_DISPLAY);
_layout = layout;
_contextObjects = contextObjects;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (!(obj instanceof NavItem)) {
return false;
}
NavItem navItem = (NavItem)obj;
if (Validator.equals(getLayoutId(), navItem.getLayoutId())) {
return true;
}
return false;
}
/**
* Returns all of the browsable child layouts that the current user has
* permission to access from this navigation item's layout.
*
* @return the list of all browsable child layouts that the current user has
* permission to access from this navigation item's layout
* @throws Exception if an exception occurred
*/
public List getBrowsableChildren() throws Exception {
if (_browsableChildren == null) {
List children = getChildren();
_browsableChildren = ListUtil.filter(
children,
new PredicateFilter() {
@Override
public boolean filter(NavItem navItem) {
return navItem.isBrowsable();
}
});
}
return _browsableChildren;
}
/**
* Returns all of child layouts that the current user has permission to
* access from this navigation item's layout.
*
* @return the list of all child layouts that the current user has
* permission to access from this navigation item's layout
* @throws Exception if an exception occurred
*/
public List getChildren() throws Exception {
if (_children == null) {
List layouts = _layout.getChildren(
_themeDisplay.getPermissionChecker());
_children = fromLayouts(_request, layouts, _contextObjects);
}
return _children;
}
/**
* Returns the navigation item's layout.
*
* @return the navigation item's layout
*/
public Layout getLayout() {
return _layout;
}
/**
* Returns the ID of the navigation item's layout.
*
* @return the ID of the navigation item's layout
*/
public long getLayoutId() {
return _layout.getLayoutId();
}
/**
* Returns the HTML-escaped name of the navigation item's layout.
*
* @return the HTML-escaped name of the navigation item's layout
*/
public String getName() {
return HtmlUtil.escape(getUnescapedName());
}
/**
* Returns the full, absolute URL (including the portal's URL) of the
* navigation item's layout.
*
* @return the full, absolute URL of the navigation item's layout
* @throws Exception if an exception occurred
*/
public String getRegularFullURL() throws Exception {
String portalURL = PortalUtil.getPortalURL(_request);
String regularURL = getRegularURL();
if (StringUtil.startsWith(regularURL, portalURL) ||
Validator.isUrl(regularURL)) {
return regularURL;
}
else {
return portalURL.concat(regularURL);
}
}
/**
* Returns the regular URL of the navigation item's layout.
*
* @return the regular URL of the navigation item's layout
* @throws Exception if an exception occurred
*/
public String getRegularURL() throws Exception {
return _layout.getRegularURL(_request);
}
public String getResetLayoutURL() throws Exception {
return _layout.getResetLayoutURL(_request);
}
public String getResetMaxStateURL() throws Exception {
return _layout.getResetMaxStateURL(_request);
}
/**
* Returns the target of the navigation item's layout.
*
* @return the target of the navigation item's layout
*/
public String getTarget() {
return _layout.getTarget();
}
/**
* Returns the title of the navigation item's layout in the current
* request's locale.
*
* @return the title of the navigation item's layout in the current
* request's locale
*/
public String getTitle() {
return _layout.getTitle(_themeDisplay.getLocale());
}
/**
* Returns the unescaped name of the navigation item's layout in the current
* request's locale.
*
* @return the unescaped name of the navigation item's layout in the current
* request's locale
*/
public String getUnescapedName() {
return _layout.getName(_themeDisplay.getLocale());
}
/**
* Returns the URL of the navigation item's layout, in a format that makes
* it safe to use the URL as an HREF attribute value
*
* @return the URL of the navigation item's layout, in a format that makes
* it safe to use the URL as an HREF attribute value
* @throws Exception if an exception occurred
*/
public String getURL() throws Exception {
return HtmlUtil.escapeHREF(getRegularFullURL());
}
/**
* Returns true
if the navigation item's layout has browsable
* child layouts.
*
* @return true
if the navigation item's layout has browsable
* child layouts; false
otherwise
* @throws Exception if an exception occurred
*/
public boolean hasBrowsableChildren() throws Exception {
List browsableChildren = getBrowsableChildren();
if (!browsableChildren.isEmpty()) {
return true;
}
else {
return false;
}
}
/**
* Returns true
if the navigation item's layout has child
* layouts.
*
* @return true
if the navigation item's layout has child
* layouts; false
otherwise
* @throws Exception if an exception occurred
*/
public boolean hasChildren() throws Exception {
List children = getChildren();
if (!children.isEmpty()) {
return true;
}
else {
return false;
}
}
@Override
public int hashCode() {
return _layout.hashCode();
}
public String iconURL() throws Exception {
if ((_layout == null) || !_layout.isIconImage()) {
return StringPool.BLANK;
}
StringBundler sb = new StringBundler(5);
sb.append(_themeDisplay.getPathImage());
sb.append("/layout_icon?img_id");
sb.append(_layout.getIconImageId());
sb.append("&t=");
sb.append(WebServerServletTokenUtil.getToken(_layout.getIconImageId()));
return sb.toString();
}
public boolean isBrowsable() {
LayoutType layoutType = _layout.getLayoutType();
return layoutType.isBrowsable();
}
public boolean isChildSelected() throws PortalException {
return _layout.isChildSelected(
_themeDisplay.isTilesSelectable(), _themeDisplay.getLayout());
}
public boolean isInNavigation(List navItems) {
if (navItems == null) {
return false;
}
return navItems.contains(this);
}
public boolean isSelected() throws Exception {
return _layout.isSelected(
_themeDisplay.isTilesSelectable(), _themeDisplay.getLayout(),
_themeDisplay.getLayout().getAncestorPlid());
}
private List _browsableChildren;
private List _children;
private final Map _contextObjects;
private final Layout _layout;
private final HttpServletRequest _request;
private final ThemeDisplay _themeDisplay;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy