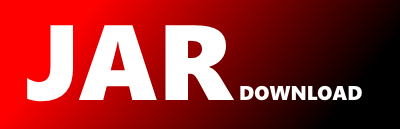
com.liferay.portal.kernel.util.TreePathUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.liferay.portal.kernel Show documentation
Show all versions of com.liferay.portal.kernel Show documentation
Contains interfaces for the portal services. Interfaces are only loaded by the global class loader and are shared by all plugins.
/**
* Copyright (c) 2000-present Liferay, Inc. All rights reserved.
*
* This library is free software; you can redistribute it and/or modify it under
* the terms of the GNU Lesser General Public License as published by the Free
* Software Foundation; either version 2.1 of the License, or (at your option)
* any later version.
*
* This library is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License for more
* details.
*/
package com.liferay.portal.kernel.util;
import com.liferay.portal.kernel.exception.PortalException;
import com.liferay.portal.kernel.model.TreeModel;
import java.util.Deque;
import java.util.LinkedList;
import java.util.List;
import java.util.concurrent.ForkJoinPool;
import java.util.concurrent.RecursiveAction;
/**
* @deprecated As of 7.0.0, moved to {@link
* com.liferay.portal.kernel.tree.TreePathUtil}
* @author Shinn Lok
*/
@Deprecated
public class TreePathUtil {
public static void rebuildTree(
long companyId, long parentPrimaryKey, String parentTreePath,
TreeModelTasks> treeModelTasks)
throws PortalException {
if (VerifyThreadLocal.isVerifyInProgress() &&
_VERIFY_DATABASE_TRANSACTIONS_DISABLED) {
ForkJoinPool forkJoinPool = new ForkJoinPool();
try {
forkJoinPool.invoke(
new RecursiveRebuildTreeTask(
treeModelTasks, companyId, parentPrimaryKey,
parentTreePath, 0L));
}
finally {
forkJoinPool.shutdown();
}
return;
}
Deque
© 2015 - 2025 Weber Informatics LLC | Privacy Policy