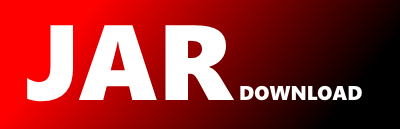
com.liferay.users.admin.kernel.util.UsersAdmin Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.liferay.portal.kernel Show documentation
Show all versions of com.liferay.portal.kernel Show documentation
Contains interfaces for the portal services. Interfaces are only loaded by the global class loader and are shared by all plugins.
/**
* Copyright (c) 2000-present Liferay, Inc. All rights reserved.
*
* This library is free software; you can redistribute it and/or modify it under
* the terms of the GNU Lesser General Public License as published by the Free
* Software Foundation; either version 2.1 of the License, or (at your option)
* any later version.
*
* This library is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License for more
* details.
*/
package com.liferay.users.admin.kernel.util;
import aQute.bnd.annotation.ProviderType;
import com.liferay.portal.kernel.exception.PortalException;
import com.liferay.portal.kernel.language.LanguageUtil;
import com.liferay.portal.kernel.model.Address;
import com.liferay.portal.kernel.model.Country;
import com.liferay.portal.kernel.model.EmailAddress;
import com.liferay.portal.kernel.model.Group;
import com.liferay.portal.kernel.model.OrgLabor;
import com.liferay.portal.kernel.model.Organization;
import com.liferay.portal.kernel.model.Phone;
import com.liferay.portal.kernel.model.Region;
import com.liferay.portal.kernel.model.Role;
import com.liferay.portal.kernel.model.User;
import com.liferay.portal.kernel.model.UserGroup;
import com.liferay.portal.kernel.model.UserGroupGroupRole;
import com.liferay.portal.kernel.model.UserGroupRole;
import com.liferay.portal.kernel.model.Website;
import com.liferay.portal.kernel.search.Hits;
import com.liferay.portal.kernel.security.permission.PermissionChecker;
import com.liferay.portal.kernel.service.CountryServiceUtil;
import com.liferay.portal.kernel.service.RegionServiceUtil;
import com.liferay.portal.kernel.service.RoleLocalServiceUtil;
import com.liferay.portal.kernel.util.Accessor;
import com.liferay.portal.kernel.util.LocaleThreadLocal;
import com.liferay.portal.kernel.util.OrderByComparator;
import com.liferay.portal.kernel.util.StringPool;
import com.liferay.portal.kernel.util.Validator;
import java.util.List;
import java.util.Locale;
import javax.portlet.ActionRequest;
import javax.portlet.PortletRequest;
import javax.portlet.RenderResponse;
import javax.servlet.http.HttpServletRequest;
/**
* @author Brian Wing Shun Chan
* @author Jorge Ferrer
* @author Julio Camarero
*/
@ProviderType
public interface UsersAdmin {
public static final String CUSTOM_QUESTION = "write-my-own-question";
public static final Accessor
ORGANIZATION_COUNTRY_NAME_ACCESSOR =
new Accessor() {
@Override
public String get(Organization organization) {
Address address = organization.getAddress();
Country country = address.getCountry();
String countryName = country.getName(
LocaleThreadLocal.getThemeDisplayLocale());
if (Validator.isNull(countryName)) {
country = CountryServiceUtil.fetchCountry(
organization.getCountryId());
if (country != null) {
countryName = country.getName(
LocaleThreadLocal.getThemeDisplayLocale());
}
}
return countryName;
}
@Override
public Class getAttributeClass() {
return String.class;
}
@Override
public Class getTypeClass() {
return Organization.class;
}
};
public static final Accessor
ORGANIZATION_REGION_NAME_ACCESSOR =
new Accessor() {
@Override
public String get(Organization organization) {
Address address = organization.getAddress();
Region region = address.getRegion();
String regionName = region.getName();
if (Validator.isNull(regionName)) {
region = RegionServiceUtil.fetchRegion(
organization.getRegionId());
if (region != null) {
regionName = LanguageUtil.get(
LocaleThreadLocal.getThemeDisplayLocale(),
region.getName());
}
}
return regionName;
}
@Override
public Class getAttributeClass() {
return String.class;
}
@Override
public Class getTypeClass() {
return Organization.class;
}
};
public static final Accessor
USER_GROUP_GROUP_ROLE_ID_ACCESSOR =
new Accessor() {
@Override
public Long get(UserGroupGroupRole userGroupGroupRole) {
Role role = RoleLocalServiceUtil.fetchRole(
userGroupGroupRole.getRoleId());
if (role == null) {
return 0L;
}
return role.getRoleId();
}
@Override
public Class getAttributeClass() {
return Long.class;
}
@Override
public Class getTypeClass() {
return UserGroupGroupRole.class;
}
};
public static final Accessor
USER_GROUP_GROUP_ROLE_TITLE_ACCESSOR =
new Accessor() {
@Override
public String get(UserGroupGroupRole userGroupGroupRole) {
Role role = RoleLocalServiceUtil.fetchRole(
userGroupGroupRole.getRoleId());
if (role == null) {
return StringPool.BLANK;
}
return role.getTitle(
LocaleThreadLocal.getThemeDisplayLocale());
}
@Override
public Class getAttributeClass() {
return String.class;
}
@Override
public Class getTypeClass() {
return UserGroupGroupRole.class;
}
};
public static final Accessor
USER_GROUP_ROLE_ID_ACCESSOR = new Accessor() {
@Override
public Long get(UserGroupRole userGroupRole) {
Role role = RoleLocalServiceUtil.fetchRole(
userGroupRole.getRoleId());
if (role == null) {
return 0L;
}
return role.getRoleId();
}
@Override
public Class getAttributeClass() {
return Long.class;
}
@Override
public Class getTypeClass() {
return UserGroupRole.class;
}
};
public static final Accessor
USER_GROUP_ROLE_TITLE_ACCESSOR = new Accessor() {
@Override
public String get(UserGroupRole userGroupRole) {
Role role = RoleLocalServiceUtil.fetchRole(
userGroupRole.getRoleId());
if (role == null) {
return StringPool.BLANK;
}
return role.getTitle(LocaleThreadLocal.getThemeDisplayLocale());
}
@Override
public Class getAttributeClass() {
return String.class;
}
@Override
public Class getTypeClass() {
return UserGroupRole.class;
}
};
public void addPortletBreadcrumbEntries(
Organization organization, HttpServletRequest request,
RenderResponse renderResponse)
throws Exception;
public long[] addRequiredRoles(long userId, long[] roleIds)
throws PortalException;
public long[] addRequiredRoles(User user, long[] roleIds)
throws PortalException;
public List filterGroupRoles(
PermissionChecker permissionChecker, long groupId, List roles)
throws PortalException;
public List filterGroups(
PermissionChecker permissionChecker, List groups)
throws PortalException;
public List filterOrganizations(
PermissionChecker permissionChecker,
List organizations)
throws PortalException;
public List filterRoles(
PermissionChecker permissionChecker, List roles);
public long[] filterUnsetGroupUserIds(
PermissionChecker permissionChecker, long groupId, long[] userIds)
throws PortalException;
public long[] filterUnsetOrganizationUserIds(
PermissionChecker permissionChecker, long organizationId,
long[] userIds)
throws PortalException;
public List filterUserGroupRoles(
PermissionChecker permissionChecker,
List userGroupRoles)
throws PortalException;
public List filterUserGroups(
PermissionChecker permissionChecker, List userGroups);
public List getAddresses(ActionRequest actionRequest);
public List getAddresses(
ActionRequest actionRequest, List defaultAddresses);
public List getEmailAddresses(ActionRequest actionRequest);
public List getEmailAddresses(
ActionRequest actionRequest, List defaultEmailAddresses);
public long[] getGroupIds(PortletRequest portletRequest)
throws PortalException;
public OrderByComparator getGroupOrderByComparator(
String orderByCol, String orderByType);
public Long[] getOrganizationIds(List organizations);
public long[] getOrganizationIds(PortletRequest portletRequest)
throws PortalException;
public OrderByComparator getOrganizationOrderByComparator(
String orderByCol, String orderByType);
public List getOrganizations(Hits hits)
throws PortalException;
public List getOrgLabors(ActionRequest actionRequest);
public List getPhones(ActionRequest actionRequest);
public List getPhones(
ActionRequest actionRequest, List defaultPhones);
public long[] getRoleIds(PortletRequest portletRequest)
throws PortalException;
public OrderByComparator getRoleOrderByComparator(
String orderByCol, String orderByType);
public String getUserColumnText(
Locale locale, List extends T> list, Accessor accessor,
int count);
public long[] getUserGroupIds(PortletRequest portletRequest)
throws PortalException;
public OrderByComparator getUserGroupOrderByComparator(
String orderByCol, String orderByType);
public List getUserGroupRoles(PortletRequest portletRequest)
throws PortalException;
public List getUserGroups(Hits hits) throws PortalException;
public OrderByComparator getUserOrderByComparator(
String orderByCol, String orderByType);
public List getUsers(Hits hits) throws PortalException;
public List getWebsites(ActionRequest actionRequest);
public List getWebsites(
ActionRequest actionRequest, List defaultWebsites);
public boolean hasUpdateFieldPermission(
PermissionChecker permissionChecker, User updatingUser,
User updatedUser, String field)
throws PortalException;
public long[] removeRequiredRoles(long userId, long[] roleIds)
throws PortalException;
public long[] removeRequiredRoles(User user, long[] roleIds)
throws PortalException;
public void updateAddresses(
String className, long classPK, List addresses)
throws PortalException;
public void updateEmailAddresses(
String className, long classPK, List emailAddresses)
throws PortalException;
public void updateOrgLabors(long classPK, List orgLabors)
throws PortalException;
public void updatePhones(String className, long classPK, List phones)
throws PortalException;
public void updateWebsites(
String className, long classPK, List websites)
throws PortalException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy