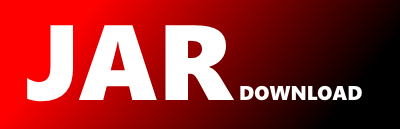
com.liferay.asset.publisher.web.portlet.DisplayPageFriendlyURLResolver Maven / Gradle / Ivy
/**
* Copyright (c) 2000-present Liferay, Inc. All rights reserved.
*
* This library is free software; you can redistribute it and/or modify it under
* the terms of the GNU Lesser General Public License as published by the Free
* Software Foundation; either version 2.1 of the License, or (at your option)
* any later version.
*
* This library is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License for more
* details.
*/
package com.liferay.asset.publisher.web.portlet;
import com.liferay.asset.kernel.AssetRendererFactoryRegistryUtil;
import com.liferay.asset.kernel.model.AssetRendererFactory;
import com.liferay.asset.kernel.model.AssetTag;
import com.liferay.asset.kernel.service.AssetTagLocalService;
import com.liferay.asset.publisher.web.constants.AssetPublisherPortletKeys;
import com.liferay.journal.model.JournalArticle;
import com.liferay.journal.model.JournalArticleConstants;
import com.liferay.journal.service.JournalArticleLocalService;
import com.liferay.portal.kernel.exception.PortalException;
import com.liferay.portal.kernel.model.Layout;
import com.liferay.portal.kernel.model.LayoutFriendlyURLComposite;
import com.liferay.portal.kernel.model.LayoutTypePortletConstants;
import com.liferay.portal.kernel.model.PortletInstance;
import com.liferay.portal.kernel.portlet.FriendlyURLResolver;
import com.liferay.portal.kernel.security.auth.AuthTokenUtil;
import com.liferay.portal.kernel.service.LayoutLocalService;
import com.liferay.portal.kernel.util.HttpUtil;
import com.liferay.portal.kernel.util.InheritableMap;
import com.liferay.portal.kernel.util.ListUtil;
import com.liferay.portal.kernel.util.PortalUtil;
import com.liferay.portal.kernel.util.StringPool;
import com.liferay.portal.kernel.util.UnicodeProperties;
import com.liferay.portal.kernel.util.Validator;
import java.util.List;
import java.util.Locale;
import java.util.Map;
import javax.portlet.WindowState;
import javax.servlet.http.HttpServletRequest;
import org.osgi.service.component.annotations.Component;
import org.osgi.service.component.annotations.Reference;
/**
* @author Eduardo Garcia
*/
@Component(service = FriendlyURLResolver.class)
public class DisplayPageFriendlyURLResolver implements FriendlyURLResolver {
@Override
public String getActualURL(
long companyId, long groupId, boolean privateLayout,
String mainPath, String friendlyURL, Map params,
Map requestContext)
throws PortalException {
String urlTitle = friendlyURL.substring(
JournalArticleConstants.CANONICAL_URL_SEPARATOR.length());
JournalArticle journalArticle =
_journalArticleLocalService.getArticleByUrlTitle(groupId, urlTitle);
Layout layout = getJournalArticleLayout(
groupId, privateLayout, friendlyURL);
String layoutActualURL = PortalUtil.getLayoutActualURL(
layout, mainPath);
InheritableMap actualParams = new InheritableMap<>();
if (params != null) {
actualParams.setParentMap(params);
}
UnicodeProperties typeSettingsProperties =
layout.getTypeSettingsProperties();
String defaultAssetPublisherPortletId = typeSettingsProperties.get(
LayoutTypePortletConstants.DEFAULT_ASSET_PUBLISHER_PORTLET_ID);
String currentDefaultAssetPublisherPortletId =
defaultAssetPublisherPortletId;
if (Validator.isNull(defaultAssetPublisherPortletId)) {
PortletInstance portletInstance = new PortletInstance(
AssetPublisherPortletKeys.ASSET_PUBLISHER);
defaultAssetPublisherPortletId =
portletInstance.getPortletInstanceKey();
}
HttpServletRequest request = (HttpServletRequest)requestContext.get(
"request");
if (Validator.isNull(currentDefaultAssetPublisherPortletId)) {
String actualPortletAuthenticationToken = AuthTokenUtil.getToken(
request, layout.getPlid(), defaultAssetPublisherPortletId);
actualParams.put(
"p_p_auth", new String[] {actualPortletAuthenticationToken});
}
actualParams.put(
"p_p_id", new String[] {defaultAssetPublisherPortletId});
actualParams.put("p_p_lifecycle", new String[] {"0"});
if (Validator.isNull(currentDefaultAssetPublisherPortletId)) {
actualParams.put(
"p_p_state", new String[] {WindowState.MAXIMIZED.toString()});
}
actualParams.put("p_p_mode", new String[] {"view"});
actualParams.put(
"p_j_a_id", new String[] {String.valueOf(journalArticle.getId())});
String namespace = PortalUtil.getPortletNamespace(
defaultAssetPublisherPortletId);
actualParams.put(
namespace + "mvcPath", new String[] {"/view_content.jsp"});
AssetRendererFactory> assetRendererFactory =
AssetRendererFactoryRegistryUtil.getAssetRendererFactoryByClassName(
JournalArticle.class.getName());
actualParams.put(
namespace + "type", new String[] {assetRendererFactory.getType()});
actualParams.put(
namespace + "urlTitle",
new String[] {journalArticle.getUrlTitle()});
String queryString = HttpUtil.parameterMapToString(actualParams, false);
if (layoutActualURL.contains(StringPool.QUESTION)) {
layoutActualURL =
layoutActualURL + StringPool.AMPERSAND + queryString;
}
else {
layoutActualURL =
layoutActualURL + StringPool.QUESTION + queryString;
}
Locale locale = PortalUtil.getLocale(request);
PortalUtil.addPageSubtitle(journalArticle.getTitle(locale), request);
PortalUtil.addPageDescription(
journalArticle.getDescription(locale), request);
List assetTags = _assetTagLocalService.getTags(
JournalArticle.class.getName(), journalArticle.getPrimaryKey());
if (!assetTags.isEmpty()) {
PortalUtil.addPageKeywords(
ListUtil.toString(assetTags, AssetTag.NAME_ACCESSOR), request);
}
return layoutActualURL;
}
@Override
public LayoutFriendlyURLComposite getLayoutFriendlyURLComposite(
long companyId, long groupId, boolean privateLayout,
String friendlyURL, Map params,
Map requestContext)
throws PortalException {
Layout layout = getJournalArticleLayout(
groupId, privateLayout, friendlyURL);
return new LayoutFriendlyURLComposite(layout, friendlyURL);
}
@Override
public String getURLSeparator() {
return JournalArticleConstants.CANONICAL_URL_SEPARATOR;
}
protected Layout getJournalArticleLayout(
long groupId, boolean privateLayout, String friendlyURL)
throws PortalException {
String urlTitle = friendlyURL.substring(
JournalArticleConstants.CANONICAL_URL_SEPARATOR.length());
JournalArticle journalArticle =
_journalArticleLocalService.getArticleByUrlTitle(groupId, urlTitle);
return _layoutLocalService.getLayoutByUuidAndGroupId(
journalArticle.getLayoutUuid(), groupId, privateLayout);
}
@Reference(unbind = "-")
protected void setAssetTagLocalService(
AssetTagLocalService assetTagLocalService) {
_assetTagLocalService = assetTagLocalService;
}
@Reference(unbind = "-")
protected void setJournalArticleLocalService(
JournalArticleLocalService journalArticleLocalService) {
_journalArticleLocalService = journalArticleLocalService;
}
@Reference(unbind = "-")
protected void setLayoutLocalService(
LayoutLocalService layoutLocalService) {
_layoutLocalService = layoutLocalService;
}
private AssetTagLocalService _assetTagLocalService;
private JournalArticleLocalService _journalArticleLocalService;
private LayoutLocalService _layoutLocalService;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy