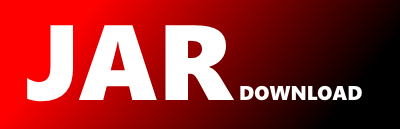
com.liferay.batch.engine.model.impl.BatchEngineExportTaskModelImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.liferay.batch.engine.service
Show all versions of com.liferay.batch.engine.service
Liferay Batch Engine Service
/**
* Copyright (c) 2000-present Liferay, Inc. All rights reserved.
*
* This library is free software; you can redistribute it and/or modify it under
* the terms of the GNU Lesser General Public License as published by the Free
* Software Foundation; either version 2.1 of the License, or (at your option)
* any later version.
*
* This library is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License for more
* details.
*/
package com.liferay.batch.engine.model.impl;
import com.liferay.batch.engine.model.BatchEngineExportTask;
import com.liferay.batch.engine.model.BatchEngineExportTaskContentBlobModel;
import com.liferay.batch.engine.model.BatchEngineExportTaskModel;
import com.liferay.batch.engine.model.BatchEngineExportTaskSoap;
import com.liferay.batch.engine.service.BatchEngineExportTaskLocalServiceUtil;
import com.liferay.expando.kernel.model.ExpandoBridge;
import com.liferay.expando.kernel.util.ExpandoBridgeFactoryUtil;
import com.liferay.exportimport.kernel.lar.StagedModelType;
import com.liferay.petra.string.StringBundler;
import com.liferay.portal.kernel.bean.AutoEscapeBeanHandler;
import com.liferay.portal.kernel.exception.PortalException;
import com.liferay.portal.kernel.json.JSON;
import com.liferay.portal.kernel.model.CacheModel;
import com.liferay.portal.kernel.model.ModelWrapper;
import com.liferay.portal.kernel.model.User;
import com.liferay.portal.kernel.model.impl.BaseModelImpl;
import com.liferay.portal.kernel.service.ServiceContext;
import com.liferay.portal.kernel.service.UserLocalServiceUtil;
import com.liferay.portal.kernel.util.GetterUtil;
import com.liferay.portal.kernel.util.PortalUtil;
import com.liferay.portal.kernel.util.ProxyUtil;
import java.io.Serializable;
import java.lang.reflect.Constructor;
import java.lang.reflect.InvocationHandler;
import java.sql.Blob;
import java.sql.Types;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Date;
import java.util.HashMap;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.function.BiConsumer;
import java.util.function.Function;
/**
* The base model implementation for the BatchEngineExportTask service. Represents a row in the "BatchEngineExportTask" database table, with each column mapped to a property of this class.
*
*
* This implementation and its corresponding interface BatchEngineExportTaskModel
exist only as a container for the default property accessors generated by ServiceBuilder. Helper methods and all application logic should be put in {@link BatchEngineExportTaskImpl}.
*
*
* @author Shuyang Zhou
* @see BatchEngineExportTaskImpl
* @generated
*/
@JSON(strict = true)
public class BatchEngineExportTaskModelImpl
extends BaseModelImpl
implements BatchEngineExportTaskModel {
/*
* NOTE FOR DEVELOPERS:
*
* Never modify or reference this class directly. All methods that expect a batch engine export task model instance should use the BatchEngineExportTask
interface instead.
*/
public static final String TABLE_NAME = "BatchEngineExportTask";
public static final Object[][] TABLE_COLUMNS = {
{"mvccVersion", Types.BIGINT}, {"uuid_", Types.VARCHAR},
{"batchEngineExportTaskId", Types.BIGINT}, {"companyId", Types.BIGINT},
{"userId", Types.BIGINT}, {"createDate", Types.TIMESTAMP},
{"modifiedDate", Types.TIMESTAMP}, {"callbackURL", Types.VARCHAR},
{"className", Types.VARCHAR}, {"content", Types.BLOB},
{"contentType", Types.VARCHAR}, {"endTime", Types.TIMESTAMP},
{"errorMessage", Types.CLOB}, {"fieldNames", Types.VARCHAR},
{"executeStatus", Types.VARCHAR}, {"parameters", Types.CLOB},
{"processedItemsCount", Types.INTEGER}, {"startTime", Types.TIMESTAMP},
{"taskItemDelegateName", Types.VARCHAR},
{"totalItemsCount", Types.INTEGER}
};
public static final Map TABLE_COLUMNS_MAP =
new HashMap();
static {
TABLE_COLUMNS_MAP.put("mvccVersion", Types.BIGINT);
TABLE_COLUMNS_MAP.put("uuid_", Types.VARCHAR);
TABLE_COLUMNS_MAP.put("batchEngineExportTaskId", Types.BIGINT);
TABLE_COLUMNS_MAP.put("companyId", Types.BIGINT);
TABLE_COLUMNS_MAP.put("userId", Types.BIGINT);
TABLE_COLUMNS_MAP.put("createDate", Types.TIMESTAMP);
TABLE_COLUMNS_MAP.put("modifiedDate", Types.TIMESTAMP);
TABLE_COLUMNS_MAP.put("callbackURL", Types.VARCHAR);
TABLE_COLUMNS_MAP.put("className", Types.VARCHAR);
TABLE_COLUMNS_MAP.put("content", Types.BLOB);
TABLE_COLUMNS_MAP.put("contentType", Types.VARCHAR);
TABLE_COLUMNS_MAP.put("endTime", Types.TIMESTAMP);
TABLE_COLUMNS_MAP.put("errorMessage", Types.CLOB);
TABLE_COLUMNS_MAP.put("fieldNames", Types.VARCHAR);
TABLE_COLUMNS_MAP.put("executeStatus", Types.VARCHAR);
TABLE_COLUMNS_MAP.put("parameters", Types.CLOB);
TABLE_COLUMNS_MAP.put("processedItemsCount", Types.INTEGER);
TABLE_COLUMNS_MAP.put("startTime", Types.TIMESTAMP);
TABLE_COLUMNS_MAP.put("taskItemDelegateName", Types.VARCHAR);
TABLE_COLUMNS_MAP.put("totalItemsCount", Types.INTEGER);
}
public static final String TABLE_SQL_CREATE =
"create table BatchEngineExportTask (mvccVersion LONG default 0 not null,uuid_ VARCHAR(75) null,batchEngineExportTaskId LONG not null primary key,companyId LONG,userId LONG,createDate DATE null,modifiedDate DATE null,callbackURL VARCHAR(75) null,className VARCHAR(255) null,content BLOB,contentType VARCHAR(75) null,endTime DATE null,errorMessage TEXT null,fieldNames VARCHAR(1000) null,executeStatus VARCHAR(75) null,parameters TEXT null,processedItemsCount INTEGER,startTime DATE null,taskItemDelegateName VARCHAR(75) null,totalItemsCount INTEGER)";
public static final String TABLE_SQL_DROP =
"drop table BatchEngineExportTask";
public static final String ORDER_BY_JPQL =
" ORDER BY batchEngineExportTask.batchEngineExportTaskId ASC";
public static final String ORDER_BY_SQL =
" ORDER BY BatchEngineExportTask.batchEngineExportTaskId ASC";
public static final String DATA_SOURCE = "liferayDataSource";
public static final String SESSION_FACTORY = "liferaySessionFactory";
public static final String TX_MANAGER = "liferayTransactionManager";
/**
* @deprecated As of Athanasius (7.3.x), replaced by {@link #getColumnBitmask(String)}
*/
@Deprecated
public static final long COMPANYID_COLUMN_BITMASK = 1L;
/**
* @deprecated As of Athanasius (7.3.x), replaced by {@link #getColumnBitmask(String)}
*/
@Deprecated
public static final long EXECUTESTATUS_COLUMN_BITMASK = 2L;
/**
* @deprecated As of Athanasius (7.3.x), replaced by {@link #getColumnBitmask(String)}
*/
@Deprecated
public static final long UUID_COLUMN_BITMASK = 4L;
/**
* @deprecated As of Athanasius (7.3.x), replaced by {@link
* #getColumnBitmask(String)}
*/
@Deprecated
public static final long BATCHENGINEEXPORTTASKID_COLUMN_BITMASK = 8L;
/**
* @deprecated As of Athanasius (7.3.x), with no direct replacement
*/
@Deprecated
public static void setEntityCacheEnabled(boolean entityCacheEnabled) {
}
/**
* @deprecated As of Athanasius (7.3.x), with no direct replacement
*/
@Deprecated
public static void setFinderCacheEnabled(boolean finderCacheEnabled) {
}
/**
* Converts the soap model instance into a normal model instance.
*
* @param soapModel the soap model instance to convert
* @return the normal model instance
* @deprecated As of Athanasius (7.3.x), with no direct replacement
*/
@Deprecated
public static BatchEngineExportTask toModel(
BatchEngineExportTaskSoap soapModel) {
if (soapModel == null) {
return null;
}
BatchEngineExportTask model = new BatchEngineExportTaskImpl();
model.setMvccVersion(soapModel.getMvccVersion());
model.setUuid(soapModel.getUuid());
model.setBatchEngineExportTaskId(
soapModel.getBatchEngineExportTaskId());
model.setCompanyId(soapModel.getCompanyId());
model.setUserId(soapModel.getUserId());
model.setCreateDate(soapModel.getCreateDate());
model.setModifiedDate(soapModel.getModifiedDate());
model.setCallbackURL(soapModel.getCallbackURL());
model.setClassName(soapModel.getClassName());
model.setContent(soapModel.getContent());
model.setContentType(soapModel.getContentType());
model.setEndTime(soapModel.getEndTime());
model.setErrorMessage(soapModel.getErrorMessage());
model.setFieldNames(soapModel.getFieldNames());
model.setExecuteStatus(soapModel.getExecuteStatus());
model.setParameters(soapModel.getParameters());
model.setProcessedItemsCount(soapModel.getProcessedItemsCount());
model.setStartTime(soapModel.getStartTime());
model.setTaskItemDelegateName(soapModel.getTaskItemDelegateName());
model.setTotalItemsCount(soapModel.getTotalItemsCount());
return model;
}
/**
* Converts the soap model instances into normal model instances.
*
* @param soapModels the soap model instances to convert
* @return the normal model instances
* @deprecated As of Athanasius (7.3.x), with no direct replacement
*/
@Deprecated
public static List toModels(
BatchEngineExportTaskSoap[] soapModels) {
if (soapModels == null) {
return null;
}
List models =
new ArrayList(soapModels.length);
for (BatchEngineExportTaskSoap soapModel : soapModels) {
models.add(toModel(soapModel));
}
return models;
}
public BatchEngineExportTaskModelImpl() {
}
@Override
public long getPrimaryKey() {
return _batchEngineExportTaskId;
}
@Override
public void setPrimaryKey(long primaryKey) {
setBatchEngineExportTaskId(primaryKey);
}
@Override
public Serializable getPrimaryKeyObj() {
return _batchEngineExportTaskId;
}
@Override
public void setPrimaryKeyObj(Serializable primaryKeyObj) {
setPrimaryKey(((Long)primaryKeyObj).longValue());
}
@Override
public Class> getModelClass() {
return BatchEngineExportTask.class;
}
@Override
public String getModelClassName() {
return BatchEngineExportTask.class.getName();
}
@Override
public Map getModelAttributes() {
Map attributes = new HashMap();
Map>
attributeGetterFunctions = getAttributeGetterFunctions();
for (Map.Entry> entry :
attributeGetterFunctions.entrySet()) {
String attributeName = entry.getKey();
Function attributeGetterFunction =
entry.getValue();
attributes.put(
attributeName,
attributeGetterFunction.apply((BatchEngineExportTask)this));
}
return attributes;
}
@Override
public void setModelAttributes(Map attributes) {
Map>
attributeSetterBiConsumers = getAttributeSetterBiConsumers();
for (Map.Entry entry : attributes.entrySet()) {
String attributeName = entry.getKey();
BiConsumer
attributeSetterBiConsumer = attributeSetterBiConsumers.get(
attributeName);
if (attributeSetterBiConsumer != null) {
attributeSetterBiConsumer.accept(
(BatchEngineExportTask)this, entry.getValue());
}
}
}
public Map>
getAttributeGetterFunctions() {
return _attributeGetterFunctions;
}
public Map>
getAttributeSetterBiConsumers() {
return _attributeSetterBiConsumers;
}
private static Function
_getProxyProviderFunction() {
Class> proxyClass = ProxyUtil.getProxyClass(
BatchEngineExportTask.class.getClassLoader(),
BatchEngineExportTask.class, ModelWrapper.class);
try {
Constructor constructor =
(Constructor)proxyClass.getConstructor(
InvocationHandler.class);
return invocationHandler -> {
try {
return constructor.newInstance(invocationHandler);
}
catch (ReflectiveOperationException
reflectiveOperationException) {
throw new InternalError(reflectiveOperationException);
}
};
}
catch (NoSuchMethodException noSuchMethodException) {
throw new InternalError(noSuchMethodException);
}
}
private static final Map>
_attributeGetterFunctions;
private static final Map>
_attributeSetterBiConsumers;
static {
Map>
attributeGetterFunctions =
new LinkedHashMap
>();
Map>
attributeSetterBiConsumers =
new LinkedHashMap
>();
attributeGetterFunctions.put(
"mvccVersion", BatchEngineExportTask::getMvccVersion);
attributeSetterBiConsumers.put(
"mvccVersion",
(BiConsumer)
BatchEngineExportTask::setMvccVersion);
attributeGetterFunctions.put("uuid", BatchEngineExportTask::getUuid);
attributeSetterBiConsumers.put(
"uuid",
(BiConsumer)
BatchEngineExportTask::setUuid);
attributeGetterFunctions.put(
"batchEngineExportTaskId",
BatchEngineExportTask::getBatchEngineExportTaskId);
attributeSetterBiConsumers.put(
"batchEngineExportTaskId",
(BiConsumer)
BatchEngineExportTask::setBatchEngineExportTaskId);
attributeGetterFunctions.put(
"companyId", BatchEngineExportTask::getCompanyId);
attributeSetterBiConsumers.put(
"companyId",
(BiConsumer)
BatchEngineExportTask::setCompanyId);
attributeGetterFunctions.put(
"userId", BatchEngineExportTask::getUserId);
attributeSetterBiConsumers.put(
"userId",
(BiConsumer)
BatchEngineExportTask::setUserId);
attributeGetterFunctions.put(
"createDate", BatchEngineExportTask::getCreateDate);
attributeSetterBiConsumers.put(
"createDate",
(BiConsumer)
BatchEngineExportTask::setCreateDate);
attributeGetterFunctions.put(
"modifiedDate", BatchEngineExportTask::getModifiedDate);
attributeSetterBiConsumers.put(
"modifiedDate",
(BiConsumer)
BatchEngineExportTask::setModifiedDate);
attributeGetterFunctions.put(
"callbackURL", BatchEngineExportTask::getCallbackURL);
attributeSetterBiConsumers.put(
"callbackURL",
(BiConsumer)
BatchEngineExportTask::setCallbackURL);
attributeGetterFunctions.put(
"className", BatchEngineExportTask::getClassName);
attributeSetterBiConsumers.put(
"className",
(BiConsumer)
BatchEngineExportTask::setClassName);
attributeGetterFunctions.put(
"content", BatchEngineExportTask::getContent);
attributeSetterBiConsumers.put(
"content",
(BiConsumer)
BatchEngineExportTask::setContent);
attributeGetterFunctions.put(
"contentType", BatchEngineExportTask::getContentType);
attributeSetterBiConsumers.put(
"contentType",
(BiConsumer)
BatchEngineExportTask::setContentType);
attributeGetterFunctions.put(
"endTime", BatchEngineExportTask::getEndTime);
attributeSetterBiConsumers.put(
"endTime",
(BiConsumer)
BatchEngineExportTask::setEndTime);
attributeGetterFunctions.put(
"errorMessage", BatchEngineExportTask::getErrorMessage);
attributeSetterBiConsumers.put(
"errorMessage",
(BiConsumer)
BatchEngineExportTask::setErrorMessage);
attributeGetterFunctions.put(
"fieldNames", BatchEngineExportTask::getFieldNames);
attributeSetterBiConsumers.put(
"fieldNames",
(BiConsumer)
BatchEngineExportTask::setFieldNames);
attributeGetterFunctions.put(
"executeStatus", BatchEngineExportTask::getExecuteStatus);
attributeSetterBiConsumers.put(
"executeStatus",
(BiConsumer)
BatchEngineExportTask::setExecuteStatus);
attributeGetterFunctions.put(
"parameters", BatchEngineExportTask::getParameters);
attributeSetterBiConsumers.put(
"parameters",
(BiConsumer>)
BatchEngineExportTask::setParameters);
attributeGetterFunctions.put(
"processedItemsCount",
BatchEngineExportTask::getProcessedItemsCount);
attributeSetterBiConsumers.put(
"processedItemsCount",
(BiConsumer)
BatchEngineExportTask::setProcessedItemsCount);
attributeGetterFunctions.put(
"startTime", BatchEngineExportTask::getStartTime);
attributeSetterBiConsumers.put(
"startTime",
(BiConsumer)
BatchEngineExportTask::setStartTime);
attributeGetterFunctions.put(
"taskItemDelegateName",
BatchEngineExportTask::getTaskItemDelegateName);
attributeSetterBiConsumers.put(
"taskItemDelegateName",
(BiConsumer)
BatchEngineExportTask::setTaskItemDelegateName);
attributeGetterFunctions.put(
"totalItemsCount", BatchEngineExportTask::getTotalItemsCount);
attributeSetterBiConsumers.put(
"totalItemsCount",
(BiConsumer)
BatchEngineExportTask::setTotalItemsCount);
_attributeGetterFunctions = Collections.unmodifiableMap(
attributeGetterFunctions);
_attributeSetterBiConsumers = Collections.unmodifiableMap(
(Map)attributeSetterBiConsumers);
}
@JSON
@Override
public long getMvccVersion() {
return _mvccVersion;
}
@Override
public void setMvccVersion(long mvccVersion) {
if (_columnOriginalValues == Collections.EMPTY_MAP) {
_setColumnOriginalValues();
}
_mvccVersion = mvccVersion;
}
@JSON
@Override
public String getUuid() {
if (_uuid == null) {
return "";
}
else {
return _uuid;
}
}
@Override
public void setUuid(String uuid) {
if (_columnOriginalValues == Collections.EMPTY_MAP) {
_setColumnOriginalValues();
}
_uuid = uuid;
}
/**
* @deprecated As of Athanasius (7.3.x), replaced by {@link
* #getColumnOriginalValue(String)}
*/
@Deprecated
public String getOriginalUuid() {
return getColumnOriginalValue("uuid_");
}
@JSON
@Override
public long getBatchEngineExportTaskId() {
return _batchEngineExportTaskId;
}
@Override
public void setBatchEngineExportTaskId(long batchEngineExportTaskId) {
if (_columnOriginalValues == Collections.EMPTY_MAP) {
_setColumnOriginalValues();
}
_batchEngineExportTaskId = batchEngineExportTaskId;
}
@JSON
@Override
public long getCompanyId() {
return _companyId;
}
@Override
public void setCompanyId(long companyId) {
if (_columnOriginalValues == Collections.EMPTY_MAP) {
_setColumnOriginalValues();
}
_companyId = companyId;
}
/**
* @deprecated As of Athanasius (7.3.x), replaced by {@link
* #getColumnOriginalValue(String)}
*/
@Deprecated
public long getOriginalCompanyId() {
return GetterUtil.getLong(
this.getColumnOriginalValue("companyId"));
}
@JSON
@Override
public long getUserId() {
return _userId;
}
@Override
public void setUserId(long userId) {
if (_columnOriginalValues == Collections.EMPTY_MAP) {
_setColumnOriginalValues();
}
_userId = userId;
}
@Override
public String getUserUuid() {
try {
User user = UserLocalServiceUtil.getUserById(getUserId());
return user.getUuid();
}
catch (PortalException portalException) {
return "";
}
}
@Override
public void setUserUuid(String userUuid) {
}
@JSON
@Override
public Date getCreateDate() {
return _createDate;
}
@Override
public void setCreateDate(Date createDate) {
if (_columnOriginalValues == Collections.EMPTY_MAP) {
_setColumnOriginalValues();
}
_createDate = createDate;
}
@JSON
@Override
public Date getModifiedDate() {
return _modifiedDate;
}
public boolean hasSetModifiedDate() {
return _setModifiedDate;
}
@Override
public void setModifiedDate(Date modifiedDate) {
_setModifiedDate = true;
if (_columnOriginalValues == Collections.EMPTY_MAP) {
_setColumnOriginalValues();
}
_modifiedDate = modifiedDate;
}
@JSON
@Override
public String getCallbackURL() {
if (_callbackURL == null) {
return "";
}
else {
return _callbackURL;
}
}
@Override
public void setCallbackURL(String callbackURL) {
if (_columnOriginalValues == Collections.EMPTY_MAP) {
_setColumnOriginalValues();
}
_callbackURL = callbackURL;
}
@JSON
@Override
public String getClassName() {
if (_className == null) {
return "";
}
else {
return _className;
}
}
@Override
public void setClassName(String className) {
if (_columnOriginalValues == Collections.EMPTY_MAP) {
_setColumnOriginalValues();
}
_className = className;
}
@JSON
@Override
public Blob getContent() {
if (_contentBlobModel == null) {
try {
_contentBlobModel =
BatchEngineExportTaskLocalServiceUtil.getContentBlobModel(
getPrimaryKey());
}
catch (Exception exception) {
}
}
Blob blob = null;
if (_contentBlobModel != null) {
blob = _contentBlobModel.getContentBlob();
}
return blob;
}
@Override
public void setContent(Blob content) {
if (_columnOriginalValues == Collections.EMPTY_MAP) {
_setColumnOriginalValues();
}
if (_contentBlobModel == null) {
_contentBlobModel = new BatchEngineExportTaskContentBlobModel(
getPrimaryKey(), content);
}
else {
_contentBlobModel.setContentBlob(content);
}
}
@JSON
@Override
public String getContentType() {
if (_contentType == null) {
return "";
}
else {
return _contentType;
}
}
@Override
public void setContentType(String contentType) {
if (_columnOriginalValues == Collections.EMPTY_MAP) {
_setColumnOriginalValues();
}
_contentType = contentType;
}
@JSON
@Override
public Date getEndTime() {
return _endTime;
}
@Override
public void setEndTime(Date endTime) {
if (_columnOriginalValues == Collections.EMPTY_MAP) {
_setColumnOriginalValues();
}
_endTime = endTime;
}
@JSON
@Override
public String getErrorMessage() {
if (_errorMessage == null) {
return "";
}
else {
return _errorMessage;
}
}
@Override
public void setErrorMessage(String errorMessage) {
if (_columnOriginalValues == Collections.EMPTY_MAP) {
_setColumnOriginalValues();
}
_errorMessage = errorMessage;
}
@JSON
@Override
public String getFieldNames() {
if (_fieldNames == null) {
return "";
}
else {
return _fieldNames;
}
}
@Override
public void setFieldNames(String fieldNames) {
if (_columnOriginalValues == Collections.EMPTY_MAP) {
_setColumnOriginalValues();
}
_fieldNames = fieldNames;
}
@JSON
@Override
public String getExecuteStatus() {
if (_executeStatus == null) {
return "";
}
else {
return _executeStatus;
}
}
@Override
public void setExecuteStatus(String executeStatus) {
if (_columnOriginalValues == Collections.EMPTY_MAP) {
_setColumnOriginalValues();
}
_executeStatus = executeStatus;
}
/**
* @deprecated As of Athanasius (7.3.x), replaced by {@link
* #getColumnOriginalValue(String)}
*/
@Deprecated
public String getOriginalExecuteStatus() {
return getColumnOriginalValue("executeStatus");
}
@JSON
@Override
public Map getParameters() {
return _parameters;
}
@Override
public void setParameters(Map parameters) {
if (_columnOriginalValues == Collections.EMPTY_MAP) {
_setColumnOriginalValues();
}
_parameters = parameters;
}
@JSON
@Override
public int getProcessedItemsCount() {
return _processedItemsCount;
}
@Override
public void setProcessedItemsCount(int processedItemsCount) {
if (_columnOriginalValues == Collections.EMPTY_MAP) {
_setColumnOriginalValues();
}
_processedItemsCount = processedItemsCount;
}
@JSON
@Override
public Date getStartTime() {
return _startTime;
}
@Override
public void setStartTime(Date startTime) {
if (_columnOriginalValues == Collections.EMPTY_MAP) {
_setColumnOriginalValues();
}
_startTime = startTime;
}
@JSON
@Override
public String getTaskItemDelegateName() {
if (_taskItemDelegateName == null) {
return "";
}
else {
return _taskItemDelegateName;
}
}
@Override
public void setTaskItemDelegateName(String taskItemDelegateName) {
if (_columnOriginalValues == Collections.EMPTY_MAP) {
_setColumnOriginalValues();
}
_taskItemDelegateName = taskItemDelegateName;
}
@JSON
@Override
public int getTotalItemsCount() {
return _totalItemsCount;
}
@Override
public void setTotalItemsCount(int totalItemsCount) {
if (_columnOriginalValues == Collections.EMPTY_MAP) {
_setColumnOriginalValues();
}
_totalItemsCount = totalItemsCount;
}
@Override
public StagedModelType getStagedModelType() {
return new StagedModelType(
PortalUtil.getClassNameId(BatchEngineExportTask.class.getName()));
}
public long getColumnBitmask() {
if (_columnBitmask > 0) {
return _columnBitmask;
}
if ((_columnOriginalValues == null) ||
(_columnOriginalValues == Collections.EMPTY_MAP)) {
return 0;
}
for (Map.Entry entry :
_columnOriginalValues.entrySet()) {
if (!Objects.equals(
entry.getValue(), getColumnValue(entry.getKey()))) {
_columnBitmask |= _columnBitmasks.get(entry.getKey());
}
}
return _columnBitmask;
}
@Override
public ExpandoBridge getExpandoBridge() {
return ExpandoBridgeFactoryUtil.getExpandoBridge(
getCompanyId(), BatchEngineExportTask.class.getName(),
getPrimaryKey());
}
@Override
public void setExpandoBridgeAttributes(ServiceContext serviceContext) {
ExpandoBridge expandoBridge = getExpandoBridge();
expandoBridge.setAttributes(serviceContext);
}
@Override
public BatchEngineExportTask toEscapedModel() {
if (_escapedModel == null) {
Function
escapedModelProxyProviderFunction =
EscapedModelProxyProviderFunctionHolder.
_escapedModelProxyProviderFunction;
_escapedModel = escapedModelProxyProviderFunction.apply(
new AutoEscapeBeanHandler(this));
}
return _escapedModel;
}
@Override
public Object clone() {
BatchEngineExportTaskImpl batchEngineExportTaskImpl =
new BatchEngineExportTaskImpl();
batchEngineExportTaskImpl.setMvccVersion(getMvccVersion());
batchEngineExportTaskImpl.setUuid(getUuid());
batchEngineExportTaskImpl.setBatchEngineExportTaskId(
getBatchEngineExportTaskId());
batchEngineExportTaskImpl.setCompanyId(getCompanyId());
batchEngineExportTaskImpl.setUserId(getUserId());
batchEngineExportTaskImpl.setCreateDate(getCreateDate());
batchEngineExportTaskImpl.setModifiedDate(getModifiedDate());
batchEngineExportTaskImpl.setCallbackURL(getCallbackURL());
batchEngineExportTaskImpl.setClassName(getClassName());
batchEngineExportTaskImpl.setContentType(getContentType());
batchEngineExportTaskImpl.setEndTime(getEndTime());
batchEngineExportTaskImpl.setErrorMessage(getErrorMessage());
batchEngineExportTaskImpl.setFieldNames(getFieldNames());
batchEngineExportTaskImpl.setExecuteStatus(getExecuteStatus());
batchEngineExportTaskImpl.setParameters(getParameters());
batchEngineExportTaskImpl.setProcessedItemsCount(
getProcessedItemsCount());
batchEngineExportTaskImpl.setStartTime(getStartTime());
batchEngineExportTaskImpl.setTaskItemDelegateName(
getTaskItemDelegateName());
batchEngineExportTaskImpl.setTotalItemsCount(getTotalItemsCount());
batchEngineExportTaskImpl.resetOriginalValues();
return batchEngineExportTaskImpl;
}
@Override
public BatchEngineExportTask cloneWithOriginalValues() {
BatchEngineExportTaskImpl batchEngineExportTaskImpl =
new BatchEngineExportTaskImpl();
batchEngineExportTaskImpl.setMvccVersion(
this.getColumnOriginalValue("mvccVersion"));
batchEngineExportTaskImpl.setUuid(
this.getColumnOriginalValue("uuid_"));
batchEngineExportTaskImpl.setBatchEngineExportTaskId(
this.getColumnOriginalValue("batchEngineExportTaskId"));
batchEngineExportTaskImpl.setCompanyId(
this.getColumnOriginalValue("companyId"));
batchEngineExportTaskImpl.setUserId(
this.getColumnOriginalValue("userId"));
batchEngineExportTaskImpl.setCreateDate(
this.getColumnOriginalValue("createDate"));
batchEngineExportTaskImpl.setModifiedDate(
this.getColumnOriginalValue("modifiedDate"));
batchEngineExportTaskImpl.setCallbackURL(
this.getColumnOriginalValue("callbackURL"));
batchEngineExportTaskImpl.setClassName(
this.getColumnOriginalValue("className"));
batchEngineExportTaskImpl.setContentType(
this.getColumnOriginalValue("contentType"));
batchEngineExportTaskImpl.setEndTime(
this.getColumnOriginalValue("endTime"));
batchEngineExportTaskImpl.setErrorMessage(
this.getColumnOriginalValue("errorMessage"));
batchEngineExportTaskImpl.setFieldNames(
this.getColumnOriginalValue("fieldNames"));
batchEngineExportTaskImpl.setExecuteStatus(
this.getColumnOriginalValue("executeStatus"));
batchEngineExportTaskImpl.setParameters(
this.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy