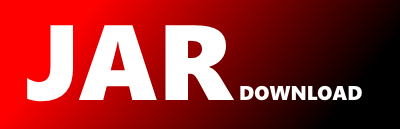
com.liferay.change.tracking.service.persistence.impl.CTSchemaVersionPersistenceImpl Maven / Gradle / Ivy
Show all versions of com.liferay.change.tracking.service
/**
* SPDX-FileCopyrightText: (c) 2000 Liferay, Inc. https://liferay.com
* SPDX-License-Identifier: LGPL-2.1-or-later OR LicenseRef-Liferay-DXP-EULA-2.0.0-2023-06
*/
package com.liferay.change.tracking.service.persistence.impl;
import com.liferay.change.tracking.exception.NoSuchSchemaVersionException;
import com.liferay.change.tracking.model.CTSchemaVersion;
import com.liferay.change.tracking.model.CTSchemaVersionTable;
import com.liferay.change.tracking.model.impl.CTSchemaVersionImpl;
import com.liferay.change.tracking.model.impl.CTSchemaVersionModelImpl;
import com.liferay.change.tracking.service.persistence.CTSchemaVersionPersistence;
import com.liferay.change.tracking.service.persistence.CTSchemaVersionUtil;
import com.liferay.change.tracking.service.persistence.impl.constants.CTPersistenceConstants;
import com.liferay.petra.string.StringBundler;
import com.liferay.portal.kernel.configuration.Configuration;
import com.liferay.portal.kernel.dao.orm.EntityCache;
import com.liferay.portal.kernel.dao.orm.FinderCache;
import com.liferay.portal.kernel.dao.orm.FinderPath;
import com.liferay.portal.kernel.dao.orm.Query;
import com.liferay.portal.kernel.dao.orm.QueryPos;
import com.liferay.portal.kernel.dao.orm.QueryUtil;
import com.liferay.portal.kernel.dao.orm.Session;
import com.liferay.portal.kernel.dao.orm.SessionFactory;
import com.liferay.portal.kernel.log.Log;
import com.liferay.portal.kernel.log.LogFactoryUtil;
import com.liferay.portal.kernel.security.auth.CompanyThreadLocal;
import com.liferay.portal.kernel.service.persistence.impl.BasePersistenceImpl;
import com.liferay.portal.kernel.util.GetterUtil;
import com.liferay.portal.kernel.util.OrderByComparator;
import com.liferay.portal.kernel.util.PropsKeys;
import com.liferay.portal.kernel.util.PropsUtil;
import com.liferay.portal.kernel.util.ProxyUtil;
import java.io.Serializable;
import java.lang.reflect.InvocationHandler;
import java.util.List;
import java.util.Map;
import java.util.Set;
import javax.sql.DataSource;
import org.osgi.service.component.annotations.Activate;
import org.osgi.service.component.annotations.Component;
import org.osgi.service.component.annotations.Deactivate;
import org.osgi.service.component.annotations.Reference;
/**
* The persistence implementation for the ct schema version service.
*
*
* Caching information and settings can be found in portal.properties
*
*
* @author Brian Wing Shun Chan
* @generated
*/
@Component(service = CTSchemaVersionPersistence.class)
public class CTSchemaVersionPersistenceImpl
extends BasePersistenceImpl
implements CTSchemaVersionPersistence {
/*
* NOTE FOR DEVELOPERS:
*
* Never modify or reference this class directly. Always use CTSchemaVersionUtil
to access the ct schema version persistence. Modify service.xml
and rerun ServiceBuilder to regenerate this class.
*/
public static final String FINDER_CLASS_NAME_ENTITY =
CTSchemaVersionImpl.class.getName();
public static final String FINDER_CLASS_NAME_LIST_WITH_PAGINATION =
FINDER_CLASS_NAME_ENTITY + ".List1";
public static final String FINDER_CLASS_NAME_LIST_WITHOUT_PAGINATION =
FINDER_CLASS_NAME_ENTITY + ".List2";
private FinderPath _finderPathWithPaginationFindAll;
private FinderPath _finderPathWithoutPaginationFindAll;
private FinderPath _finderPathCountAll;
private FinderPath _finderPathWithPaginationFindByCompanyId;
private FinderPath _finderPathWithoutPaginationFindByCompanyId;
private FinderPath _finderPathCountByCompanyId;
/**
* Returns all the ct schema versions where companyId = ?.
*
* @param companyId the company ID
* @return the matching ct schema versions
*/
@Override
public List findByCompanyId(long companyId) {
return findByCompanyId(
companyId, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the ct schema versions where companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from CTSchemaVersionModelImpl
.
*
*
* @param companyId the company ID
* @param start the lower bound of the range of ct schema versions
* @param end the upper bound of the range of ct schema versions (not inclusive)
* @return the range of matching ct schema versions
*/
@Override
public List findByCompanyId(
long companyId, int start, int end) {
return findByCompanyId(companyId, start, end, null);
}
/**
* Returns an ordered range of all the ct schema versions where companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from CTSchemaVersionModelImpl
.
*
*
* @param companyId the company ID
* @param start the lower bound of the range of ct schema versions
* @param end the upper bound of the range of ct schema versions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching ct schema versions
*/
@Override
public List findByCompanyId(
long companyId, int start, int end,
OrderByComparator orderByComparator) {
return findByCompanyId(companyId, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the ct schema versions where companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from CTSchemaVersionModelImpl
.
*
*
* @param companyId the company ID
* @param start the lower bound of the range of ct schema versions
* @param end the upper bound of the range of ct schema versions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching ct schema versions
*/
@Override
public List findByCompanyId(
long companyId, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache) {
finderPath = _finderPathWithoutPaginationFindByCompanyId;
finderArgs = new Object[] {companyId};
}
}
else if (useFinderCache) {
finderPath = _finderPathWithPaginationFindByCompanyId;
finderArgs = new Object[] {
companyId, start, end, orderByComparator
};
}
List list = null;
if (useFinderCache) {
list = (List)finderCache.getResult(
finderPath, finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (CTSchemaVersion ctSchemaVersion : list) {
if (companyId != ctSchemaVersion.getCompanyId()) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
3 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(3);
}
sb.append(_SQL_SELECT_CTSCHEMAVERSION_WHERE);
sb.append(_FINDER_COLUMN_COMPANYID_COMPANYID_2);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(CTSchemaVersionModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(companyId);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first ct schema version in the ordered set where companyId = ?.
*
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching ct schema version
* @throws NoSuchSchemaVersionException if a matching ct schema version could not be found
*/
@Override
public CTSchemaVersion findByCompanyId_First(
long companyId,
OrderByComparator orderByComparator)
throws NoSuchSchemaVersionException {
CTSchemaVersion ctSchemaVersion = fetchByCompanyId_First(
companyId, orderByComparator);
if (ctSchemaVersion != null) {
return ctSchemaVersion;
}
StringBundler sb = new StringBundler(4);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("companyId=");
sb.append(companyId);
sb.append("}");
throw new NoSuchSchemaVersionException(sb.toString());
}
/**
* Returns the first ct schema version in the ordered set where companyId = ?.
*
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching ct schema version, or null
if a matching ct schema version could not be found
*/
@Override
public CTSchemaVersion fetchByCompanyId_First(
long companyId, OrderByComparator orderByComparator) {
List list = findByCompanyId(
companyId, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last ct schema version in the ordered set where companyId = ?.
*
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching ct schema version
* @throws NoSuchSchemaVersionException if a matching ct schema version could not be found
*/
@Override
public CTSchemaVersion findByCompanyId_Last(
long companyId,
OrderByComparator orderByComparator)
throws NoSuchSchemaVersionException {
CTSchemaVersion ctSchemaVersion = fetchByCompanyId_Last(
companyId, orderByComparator);
if (ctSchemaVersion != null) {
return ctSchemaVersion;
}
StringBundler sb = new StringBundler(4);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("companyId=");
sb.append(companyId);
sb.append("}");
throw new NoSuchSchemaVersionException(sb.toString());
}
/**
* Returns the last ct schema version in the ordered set where companyId = ?.
*
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching ct schema version, or null
if a matching ct schema version could not be found
*/
@Override
public CTSchemaVersion fetchByCompanyId_Last(
long companyId, OrderByComparator orderByComparator) {
int count = countByCompanyId(companyId);
if (count == 0) {
return null;
}
List list = findByCompanyId(
companyId, count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the ct schema versions before and after the current ct schema version in the ordered set where companyId = ?.
*
* @param schemaVersionId the primary key of the current ct schema version
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next ct schema version
* @throws NoSuchSchemaVersionException if a ct schema version with the primary key could not be found
*/
@Override
public CTSchemaVersion[] findByCompanyId_PrevAndNext(
long schemaVersionId, long companyId,
OrderByComparator orderByComparator)
throws NoSuchSchemaVersionException {
CTSchemaVersion ctSchemaVersion = findByPrimaryKey(schemaVersionId);
Session session = null;
try {
session = openSession();
CTSchemaVersion[] array = new CTSchemaVersionImpl[3];
array[0] = getByCompanyId_PrevAndNext(
session, ctSchemaVersion, companyId, orderByComparator, true);
array[1] = ctSchemaVersion;
array[2] = getByCompanyId_PrevAndNext(
session, ctSchemaVersion, companyId, orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected CTSchemaVersion getByCompanyId_PrevAndNext(
Session session, CTSchemaVersion ctSchemaVersion, long companyId,
OrderByComparator orderByComparator,
boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(3);
}
sb.append(_SQL_SELECT_CTSCHEMAVERSION_WHERE);
sb.append(_FINDER_COLUMN_COMPANYID_COMPANYID_2);
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(CTSchemaVersionModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(companyId);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
ctSchemaVersion)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the ct schema versions where companyId = ? from the database.
*
* @param companyId the company ID
*/
@Override
public void removeByCompanyId(long companyId) {
for (CTSchemaVersion ctSchemaVersion :
findByCompanyId(
companyId, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null)) {
remove(ctSchemaVersion);
}
}
/**
* Returns the number of ct schema versions where companyId = ?.
*
* @param companyId the company ID
* @return the number of matching ct schema versions
*/
@Override
public int countByCompanyId(long companyId) {
FinderPath finderPath = _finderPathCountByCompanyId;
Object[] finderArgs = new Object[] {companyId};
Long count = (Long)finderCache.getResult(finderPath, finderArgs, this);
if (count == null) {
StringBundler sb = new StringBundler(2);
sb.append(_SQL_COUNT_CTSCHEMAVERSION_WHERE);
sb.append(_FINDER_COLUMN_COMPANYID_COMPANYID_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(companyId);
count = (Long)query.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String _FINDER_COLUMN_COMPANYID_COMPANYID_2 =
"ctSchemaVersion.companyId = ?";
public CTSchemaVersionPersistenceImpl() {
setModelClass(CTSchemaVersion.class);
setModelImplClass(CTSchemaVersionImpl.class);
setModelPKClass(long.class);
setTable(CTSchemaVersionTable.INSTANCE);
}
/**
* Caches the ct schema version in the entity cache if it is enabled.
*
* @param ctSchemaVersion the ct schema version
*/
@Override
public void cacheResult(CTSchemaVersion ctSchemaVersion) {
entityCache.putResult(
CTSchemaVersionImpl.class, ctSchemaVersion.getPrimaryKey(),
ctSchemaVersion);
}
private int _valueObjectFinderCacheListThreshold;
/**
* Caches the ct schema versions in the entity cache if it is enabled.
*
* @param ctSchemaVersions the ct schema versions
*/
@Override
public void cacheResult(List ctSchemaVersions) {
if ((_valueObjectFinderCacheListThreshold == 0) ||
((_valueObjectFinderCacheListThreshold > 0) &&
(ctSchemaVersions.size() >
_valueObjectFinderCacheListThreshold))) {
return;
}
for (CTSchemaVersion ctSchemaVersion : ctSchemaVersions) {
if (entityCache.getResult(
CTSchemaVersionImpl.class,
ctSchemaVersion.getPrimaryKey()) == null) {
cacheResult(ctSchemaVersion);
}
}
}
/**
* Clears the cache for all ct schema versions.
*
*
* The EntityCache
and FinderCache
are both cleared by this method.
*
*/
@Override
public void clearCache() {
entityCache.clearCache(CTSchemaVersionImpl.class);
finderCache.clearCache(CTSchemaVersionImpl.class);
}
/**
* Clears the cache for the ct schema version.
*
*
* The EntityCache
and FinderCache
are both cleared by this method.
*
*/
@Override
public void clearCache(CTSchemaVersion ctSchemaVersion) {
entityCache.removeResult(CTSchemaVersionImpl.class, ctSchemaVersion);
}
@Override
public void clearCache(List ctSchemaVersions) {
for (CTSchemaVersion ctSchemaVersion : ctSchemaVersions) {
entityCache.removeResult(
CTSchemaVersionImpl.class, ctSchemaVersion);
}
}
@Override
public void clearCache(Set primaryKeys) {
finderCache.clearCache(CTSchemaVersionImpl.class);
for (Serializable primaryKey : primaryKeys) {
entityCache.removeResult(CTSchemaVersionImpl.class, primaryKey);
}
}
/**
* Creates a new ct schema version with the primary key. Does not add the ct schema version to the database.
*
* @param schemaVersionId the primary key for the new ct schema version
* @return the new ct schema version
*/
@Override
public CTSchemaVersion create(long schemaVersionId) {
CTSchemaVersion ctSchemaVersion = new CTSchemaVersionImpl();
ctSchemaVersion.setNew(true);
ctSchemaVersion.setPrimaryKey(schemaVersionId);
ctSchemaVersion.setCompanyId(CompanyThreadLocal.getCompanyId());
return ctSchemaVersion;
}
/**
* Removes the ct schema version with the primary key from the database. Also notifies the appropriate model listeners.
*
* @param schemaVersionId the primary key of the ct schema version
* @return the ct schema version that was removed
* @throws NoSuchSchemaVersionException if a ct schema version with the primary key could not be found
*/
@Override
public CTSchemaVersion remove(long schemaVersionId)
throws NoSuchSchemaVersionException {
return remove((Serializable)schemaVersionId);
}
/**
* Removes the ct schema version with the primary key from the database. Also notifies the appropriate model listeners.
*
* @param primaryKey the primary key of the ct schema version
* @return the ct schema version that was removed
* @throws NoSuchSchemaVersionException if a ct schema version with the primary key could not be found
*/
@Override
public CTSchemaVersion remove(Serializable primaryKey)
throws NoSuchSchemaVersionException {
Session session = null;
try {
session = openSession();
CTSchemaVersion ctSchemaVersion = (CTSchemaVersion)session.get(
CTSchemaVersionImpl.class, primaryKey);
if (ctSchemaVersion == null) {
if (_log.isDebugEnabled()) {
_log.debug(_NO_SUCH_ENTITY_WITH_PRIMARY_KEY + primaryKey);
}
throw new NoSuchSchemaVersionException(
_NO_SUCH_ENTITY_WITH_PRIMARY_KEY + primaryKey);
}
return remove(ctSchemaVersion);
}
catch (NoSuchSchemaVersionException noSuchEntityException) {
throw noSuchEntityException;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
@Override
protected CTSchemaVersion removeImpl(CTSchemaVersion ctSchemaVersion) {
Session session = null;
try {
session = openSession();
if (!session.contains(ctSchemaVersion)) {
ctSchemaVersion = (CTSchemaVersion)session.get(
CTSchemaVersionImpl.class,
ctSchemaVersion.getPrimaryKeyObj());
}
if (ctSchemaVersion != null) {
session.delete(ctSchemaVersion);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
if (ctSchemaVersion != null) {
clearCache(ctSchemaVersion);
}
return ctSchemaVersion;
}
@Override
public CTSchemaVersion updateImpl(CTSchemaVersion ctSchemaVersion) {
boolean isNew = ctSchemaVersion.isNew();
if (!(ctSchemaVersion instanceof CTSchemaVersionModelImpl)) {
InvocationHandler invocationHandler = null;
if (ProxyUtil.isProxyClass(ctSchemaVersion.getClass())) {
invocationHandler = ProxyUtil.getInvocationHandler(
ctSchemaVersion);
throw new IllegalArgumentException(
"Implement ModelWrapper in ctSchemaVersion proxy " +
invocationHandler.getClass());
}
throw new IllegalArgumentException(
"Implement ModelWrapper in custom CTSchemaVersion implementation " +
ctSchemaVersion.getClass());
}
CTSchemaVersionModelImpl ctSchemaVersionModelImpl =
(CTSchemaVersionModelImpl)ctSchemaVersion;
Session session = null;
try {
session = openSession();
if (isNew) {
session.save(ctSchemaVersion);
}
else {
ctSchemaVersion = (CTSchemaVersion)session.merge(
ctSchemaVersion);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
entityCache.putResult(
CTSchemaVersionImpl.class, ctSchemaVersionModelImpl, false, true);
if (isNew) {
ctSchemaVersion.setNew(false);
}
ctSchemaVersion.resetOriginalValues();
return ctSchemaVersion;
}
/**
* Returns the ct schema version with the primary key or throws a com.liferay.portal.kernel.exception.NoSuchModelException
if it could not be found.
*
* @param primaryKey the primary key of the ct schema version
* @return the ct schema version
* @throws NoSuchSchemaVersionException if a ct schema version with the primary key could not be found
*/
@Override
public CTSchemaVersion findByPrimaryKey(Serializable primaryKey)
throws NoSuchSchemaVersionException {
CTSchemaVersion ctSchemaVersion = fetchByPrimaryKey(primaryKey);
if (ctSchemaVersion == null) {
if (_log.isDebugEnabled()) {
_log.debug(_NO_SUCH_ENTITY_WITH_PRIMARY_KEY + primaryKey);
}
throw new NoSuchSchemaVersionException(
_NO_SUCH_ENTITY_WITH_PRIMARY_KEY + primaryKey);
}
return ctSchemaVersion;
}
/**
* Returns the ct schema version with the primary key or throws a NoSuchSchemaVersionException
if it could not be found.
*
* @param schemaVersionId the primary key of the ct schema version
* @return the ct schema version
* @throws NoSuchSchemaVersionException if a ct schema version with the primary key could not be found
*/
@Override
public CTSchemaVersion findByPrimaryKey(long schemaVersionId)
throws NoSuchSchemaVersionException {
return findByPrimaryKey((Serializable)schemaVersionId);
}
/**
* Returns the ct schema version with the primary key or returns null
if it could not be found.
*
* @param schemaVersionId the primary key of the ct schema version
* @return the ct schema version, or null
if a ct schema version with the primary key could not be found
*/
@Override
public CTSchemaVersion fetchByPrimaryKey(long schemaVersionId) {
return fetchByPrimaryKey((Serializable)schemaVersionId);
}
/**
* Returns all the ct schema versions.
*
* @return the ct schema versions
*/
@Override
public List findAll() {
return findAll(QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the ct schema versions.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from CTSchemaVersionModelImpl
.
*
*
* @param start the lower bound of the range of ct schema versions
* @param end the upper bound of the range of ct schema versions (not inclusive)
* @return the range of ct schema versions
*/
@Override
public List findAll(int start, int end) {
return findAll(start, end, null);
}
/**
* Returns an ordered range of all the ct schema versions.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from CTSchemaVersionModelImpl
.
*
*
* @param start the lower bound of the range of ct schema versions
* @param end the upper bound of the range of ct schema versions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of ct schema versions
*/
@Override
public List findAll(
int start, int end,
OrderByComparator orderByComparator) {
return findAll(start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the ct schema versions.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from CTSchemaVersionModelImpl
.
*
*
* @param start the lower bound of the range of ct schema versions
* @param end the upper bound of the range of ct schema versions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of ct schema versions
*/
@Override
public List findAll(
int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache) {
finderPath = _finderPathWithoutPaginationFindAll;
finderArgs = FINDER_ARGS_EMPTY;
}
}
else if (useFinderCache) {
finderPath = _finderPathWithPaginationFindAll;
finderArgs = new Object[] {start, end, orderByComparator};
}
List list = null;
if (useFinderCache) {
list = (List)finderCache.getResult(
finderPath, finderArgs, this);
}
if (list == null) {
StringBundler sb = null;
String sql = null;
if (orderByComparator != null) {
sb = new StringBundler(
2 + (orderByComparator.getOrderByFields().length * 2));
sb.append(_SQL_SELECT_CTSCHEMAVERSION);
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
sql = sb.toString();
}
else {
sql = _SQL_SELECT_CTSCHEMAVERSION;
sql = sql.concat(CTSchemaVersionModelImpl.ORDER_BY_JPQL);
}
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Removes all the ct schema versions from the database.
*
*/
@Override
public void removeAll() {
for (CTSchemaVersion ctSchemaVersion : findAll()) {
remove(ctSchemaVersion);
}
}
/**
* Returns the number of ct schema versions.
*
* @return the number of ct schema versions
*/
@Override
public int countAll() {
Long count = (Long)finderCache.getResult(
_finderPathCountAll, FINDER_ARGS_EMPTY, this);
if (count == null) {
Session session = null;
try {
session = openSession();
Query query = session.createQuery(_SQL_COUNT_CTSCHEMAVERSION);
count = (Long)query.uniqueResult();
finderCache.putResult(
_finderPathCountAll, FINDER_ARGS_EMPTY, count);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
@Override
protected EntityCache getEntityCache() {
return entityCache;
}
@Override
protected String getPKDBName() {
return "schemaVersionId";
}
@Override
protected String getSelectSQL() {
return _SQL_SELECT_CTSCHEMAVERSION;
}
@Override
protected Map getTableColumnsMap() {
return CTSchemaVersionModelImpl.TABLE_COLUMNS_MAP;
}
/**
* Initializes the ct schema version persistence.
*/
@Activate
public void activate() {
_valueObjectFinderCacheListThreshold = GetterUtil.getInteger(
PropsUtil.get(PropsKeys.VALUE_OBJECT_FINDER_CACHE_LIST_THRESHOLD));
_finderPathWithPaginationFindAll = new FinderPath(
FINDER_CLASS_NAME_LIST_WITH_PAGINATION, "findAll", new String[0],
new String[0], true);
_finderPathWithoutPaginationFindAll = new FinderPath(
FINDER_CLASS_NAME_LIST_WITHOUT_PAGINATION, "findAll", new String[0],
new String[0], true);
_finderPathCountAll = new FinderPath(
FINDER_CLASS_NAME_LIST_WITHOUT_PAGINATION, "countAll",
new String[0], new String[0], false);
_finderPathWithPaginationFindByCompanyId = new FinderPath(
FINDER_CLASS_NAME_LIST_WITH_PAGINATION, "findByCompanyId",
new String[] {
Long.class.getName(), Integer.class.getName(),
Integer.class.getName(), OrderByComparator.class.getName()
},
new String[] {"companyId"}, true);
_finderPathWithoutPaginationFindByCompanyId = new FinderPath(
FINDER_CLASS_NAME_LIST_WITHOUT_PAGINATION, "findByCompanyId",
new String[] {Long.class.getName()}, new String[] {"companyId"},
true);
_finderPathCountByCompanyId = new FinderPath(
FINDER_CLASS_NAME_LIST_WITHOUT_PAGINATION, "countByCompanyId",
new String[] {Long.class.getName()}, new String[] {"companyId"},
false);
CTSchemaVersionUtil.setPersistence(this);
}
@Deactivate
public void deactivate() {
CTSchemaVersionUtil.setPersistence(null);
entityCache.removeCache(CTSchemaVersionImpl.class.getName());
}
@Override
@Reference(
target = CTPersistenceConstants.SERVICE_CONFIGURATION_FILTER,
unbind = "-"
)
public void setConfiguration(Configuration configuration) {
}
@Override
@Reference(
target = CTPersistenceConstants.ORIGIN_BUNDLE_SYMBOLIC_NAME_FILTER,
unbind = "-"
)
public void setDataSource(DataSource dataSource) {
super.setDataSource(dataSource);
}
@Override
@Reference(
target = CTPersistenceConstants.ORIGIN_BUNDLE_SYMBOLIC_NAME_FILTER,
unbind = "-"
)
public void setSessionFactory(SessionFactory sessionFactory) {
super.setSessionFactory(sessionFactory);
}
@Reference
protected EntityCache entityCache;
@Reference
protected FinderCache finderCache;
private static final String _SQL_SELECT_CTSCHEMAVERSION =
"SELECT ctSchemaVersion FROM CTSchemaVersion ctSchemaVersion";
private static final String _SQL_SELECT_CTSCHEMAVERSION_WHERE =
"SELECT ctSchemaVersion FROM CTSchemaVersion ctSchemaVersion WHERE ";
private static final String _SQL_COUNT_CTSCHEMAVERSION =
"SELECT COUNT(ctSchemaVersion) FROM CTSchemaVersion ctSchemaVersion";
private static final String _SQL_COUNT_CTSCHEMAVERSION_WHERE =
"SELECT COUNT(ctSchemaVersion) FROM CTSchemaVersion ctSchemaVersion WHERE ";
private static final String _ORDER_BY_ENTITY_ALIAS = "ctSchemaVersion.";
private static final String _NO_SUCH_ENTITY_WITH_PRIMARY_KEY =
"No CTSchemaVersion exists with the primary key ";
private static final String _NO_SUCH_ENTITY_WITH_KEY =
"No CTSchemaVersion exists with the key {";
private static final Log _log = LogFactoryUtil.getLog(
CTSchemaVersionPersistenceImpl.class);
@Override
protected FinderCache getFinderCache() {
return finderCache;
}
}