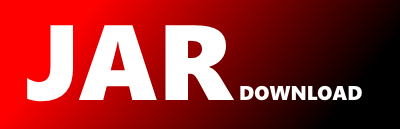
com.liferay.depot.service.impl.DepotEntryLocalServiceImpl Maven / Gradle / Ivy
/**
* Copyright (c) 2000-present Liferay, Inc. All rights reserved.
*
* This library is free software; you can redistribute it and/or modify it under
* the terms of the GNU Lesser General Public License as published by the Free
* Software Foundation; either version 2.1 of the License, or (at your option)
* any later version.
*
* This library is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License for more
* details.
*/
package com.liferay.depot.service.impl;
import com.liferay.depot.constants.DepotRolesConstants;
import com.liferay.depot.exception.DepotEntryNameException;
import com.liferay.depot.model.DepotEntry;
import com.liferay.depot.model.DepotEntryGroupRel;
import com.liferay.depot.service.DepotAppCustomizationLocalService;
import com.liferay.depot.service.base.DepotEntryLocalServiceBaseImpl;
import com.liferay.depot.service.persistence.DepotEntryGroupRelPersistence;
import com.liferay.petra.string.StringPool;
import com.liferay.portal.aop.AopService;
import com.liferay.portal.kernel.exception.GroupKeyException;
import com.liferay.portal.kernel.exception.LocaleException;
import com.liferay.portal.kernel.exception.PortalException;
import com.liferay.portal.kernel.language.Language;
import com.liferay.portal.kernel.language.LanguageUtil;
import com.liferay.portal.kernel.model.Group;
import com.liferay.portal.kernel.model.GroupConstants;
import com.liferay.portal.kernel.model.ModelHintsUtil;
import com.liferay.portal.kernel.model.Role;
import com.liferay.portal.kernel.model.User;
import com.liferay.portal.kernel.service.GroupLocalService;
import com.liferay.portal.kernel.service.RoleLocalService;
import com.liferay.portal.kernel.service.ServiceContext;
import com.liferay.portal.kernel.service.UserGroupRoleLocalService;
import com.liferay.portal.kernel.service.UserLocalService;
import com.liferay.portal.kernel.util.GetterUtil;
import com.liferay.portal.kernel.util.LocaleUtil;
import com.liferay.portal.kernel.util.MapUtil;
import com.liferay.portal.kernel.util.PropsKeys;
import com.liferay.portal.kernel.util.ResourceBundleUtil;
import com.liferay.portal.kernel.util.StringUtil;
import com.liferay.portal.kernel.util.UnicodeProperties;
import com.liferay.portal.kernel.util.Validator;
import java.util.ArrayList;
import java.util.List;
import java.util.Locale;
import java.util.Map;
import java.util.Optional;
import java.util.ResourceBundle;
import org.osgi.service.component.annotations.Component;
import org.osgi.service.component.annotations.Reference;
/**
* @author Brian Wing Shun Chan
*/
@Component(
property = "model.class.name=com.liferay.depot.model.DepotEntry",
service = AopService.class
)
public class DepotEntryLocalServiceImpl extends DepotEntryLocalServiceBaseImpl {
@Override
public DepotEntry addDepotEntry(
Map nameMap, Map descriptionMap,
ServiceContext serviceContext)
throws PortalException {
_validateNameMap(nameMap, LocaleUtil.getDefault());
DepotEntry depotEntry = depotEntryPersistence.create(
counterLocalService.increment());
depotEntry.setUuid(serviceContext.getUuid());
Group group = _groupLocalService.addGroup(
serviceContext.getUserId(), GroupConstants.DEFAULT_PARENT_GROUP_ID,
DepotEntry.class.getName(), depotEntry.getDepotEntryId(),
GroupConstants.DEFAULT_LIVE_GROUP_ID, nameMap, descriptionMap,
GroupConstants.TYPE_DEPOT, true,
GroupConstants.DEFAULT_MEMBERSHIP_RESTRICTION, null, false, false,
true, serviceContext);
_userLocalService.addGroupUsers(
group.getGroupId(), new long[] {serviceContext.getUserId()});
User user = _userLocalService.getUser(serviceContext.getUserId());
if (!user.isDefaultUser()) {
Role role = _roleLocalService.getRole(
group.getCompanyId(), DepotRolesConstants.ASSET_LIBRARY_OWNER);
_userGroupRoleLocalService.addUserGroupRoles(
user.getUserId(), group.getGroupId(),
new long[] {role.getRoleId()});
_userLocalService.addGroupUsers(
group.getGroupId(), new long[] {user.getUserId()});
}
depotEntry.setGroupId(group.getGroupId());
depotEntry.setCompanyId(serviceContext.getCompanyId());
depotEntry.setUserId(serviceContext.getUserId());
depotEntry = depotEntryPersistence.update(depotEntry);
resourceLocalService.addResources(
serviceContext.getCompanyId(), 0, serviceContext.getUserId(),
DepotEntry.class.getName(), depotEntry.getDepotEntryId(), false,
false, false);
return depotEntry;
}
@Override
public DepotEntry fetchGroupDepotEntry(long groupId) {
return depotEntryPersistence.fetchByGroupId(groupId);
}
@Override
public List getGroupConnectedDepotEntries(
long groupId, int start, int end)
throws PortalException {
List depotEntries = new ArrayList<>();
List depotEntryGroupRels =
_depotEntryGroupRelPersistence.findByToGroupId(groupId, start, end);
for (DepotEntryGroupRel depotEntryGroupRel : depotEntryGroupRels) {
DepotEntry depotEntry = depotEntryLocalService.getDepotEntry(
depotEntryGroupRel.getDepotEntryId());
depotEntries.add(depotEntry);
}
return depotEntries;
}
@Override
public int getGroupConnectedDepotEntriesCount(long groupId) {
return _depotEntryGroupRelPersistence.countByToGroupId(groupId);
}
@Override
public DepotEntry getGroupDepotEntry(long groupId) throws PortalException {
return depotEntryPersistence.findByGroupId(groupId);
}
@Override
public DepotEntry updateDepotEntry(
long depotEntryId, Map nameMap,
Map descriptionMap,
Map depotAppCustomizationMap,
UnicodeProperties typeSettingsUnicodeProperties,
ServiceContext serviceContext)
throws PortalException {
DepotEntry depotEntry = getDepotEntry(depotEntryId);
depotEntry.setModifiedDate(serviceContext.getModifiedDate());
depotEntry = depotEntryPersistence.update(depotEntry);
for (Map.Entry entry :
depotAppCustomizationMap.entrySet()) {
_depotAppCustomizationLocalService.updateDepotAppCustomization(
depotEntryId, entry.getValue(), entry.getKey());
}
_validateTypeSettingsProperties(
depotEntry, typeSettingsUnicodeProperties);
for (String name : nameMap.values()) {
_validateName(name);
}
Group group = _groupLocalService.getGroup(depotEntry.getGroupId());
UnicodeProperties currentTypeSettingsUnicodeProperties =
group.getTypeSettingsProperties();
boolean inheritLocales = GetterUtil.getBoolean(
currentTypeSettingsUnicodeProperties.getProperty("inheritLocales"),
true);
inheritLocales = GetterUtil.getBoolean(
typeSettingsUnicodeProperties.getProperty("inheritLocales"),
inheritLocales);
if (inheritLocales) {
typeSettingsUnicodeProperties.setProperty(
PropsKeys.LOCALES,
StringUtil.merge(
LocaleUtil.toLanguageIds(
LanguageUtil.getAvailableLocales())));
}
currentTypeSettingsUnicodeProperties.putAll(
typeSettingsUnicodeProperties);
Locale locale = LocaleUtil.fromLanguageId(
currentTypeSettingsUnicodeProperties.getProperty("languageId"));
Optional defaultNameOptional = _getDefaultNameOptional(
nameMap, locale);
defaultNameOptional.ifPresent(
defaultName -> nameMap.put(locale, defaultName));
group = _groupLocalService.updateGroup(
depotEntry.getGroupId(), group.getParentGroupId(), nameMap,
descriptionMap, group.getType(), group.isManualMembership(),
group.getMembershipRestriction(), group.getFriendlyURL(),
group.isInheritContent(), group.isActive(), serviceContext);
_groupLocalService.updateGroup(
group.getGroupId(),
currentTypeSettingsUnicodeProperties.toString());
return depotEntry;
}
private Optional _getDefaultNameOptional(
Map nameMap, Locale defaultLocale) {
if (Validator.isNotNull(nameMap.get(defaultLocale))) {
return Optional.empty();
}
ResourceBundle resourceBundle = ResourceBundleUtil.getBundle(
defaultLocale, DepotEntryLocalServiceImpl.class);
return Optional.of(
_language.get(resourceBundle, "unnamed-asset-library"));
}
private void _validateName(String name) throws PortalException {
int groupKeyMaxLength = ModelHintsUtil.getMaxLength(
Group.class.getName(), "name");
if (Validator.isNotNull(name) &&
(Validator.isNumber(name) || name.contains(StringPool.STAR) ||
name.contains(_ORGANIZATION_NAME_SUFFIX) ||
(name.length() > groupKeyMaxLength))) {
throw new GroupKeyException();
}
}
private void _validateNameMap(
Map nameMap, Locale defaultLocale)
throws DepotEntryNameException {
if (MapUtil.isEmpty(nameMap) ||
Validator.isNull(nameMap.get(defaultLocale))) {
throw new DepotEntryNameException();
}
}
private void _validateTypeSettingsProperties(
DepotEntry depotEntry,
UnicodeProperties typeSettingsUnicodeProperties)
throws LocaleException {
if (!typeSettingsUnicodeProperties.containsKey("inheritLocales")) {
return;
}
if (typeSettingsUnicodeProperties.containsKey(PropsKeys.LOCALES) &&
Validator.isNull(
typeSettingsUnicodeProperties.getProperty(PropsKeys.LOCALES))) {
throw new LocaleException(
LocaleException.TYPE_DEFAULT,
"Must have at least one valid locale for asset library " +
depotEntry.getGroupId());
}
boolean inheritLocales = GetterUtil.getBoolean(
typeSettingsUnicodeProperties.getProperty("inheritLocales"));
if (!inheritLocales &&
!typeSettingsUnicodeProperties.containsKey(PropsKeys.LOCALES)) {
throw new LocaleException(
LocaleException.TYPE_DEFAULT,
"Must have at least one valid locale for asset library " +
depotEntry.getGroupId());
}
}
private static final String _ORGANIZATION_NAME_SUFFIX = " LFR_ORGANIZATION";
@Reference
private DepotAppCustomizationLocalService
_depotAppCustomizationLocalService;
@Reference
private DepotEntryGroupRelPersistence _depotEntryGroupRelPersistence;
@Reference
private GroupLocalService _groupLocalService;
@Reference
private Language _language;
@Reference
private RoleLocalService _roleLocalService;
@Reference
private UserGroupRoleLocalService _userGroupRoleLocalService;
@Reference
private UserLocalService _userLocalService;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy