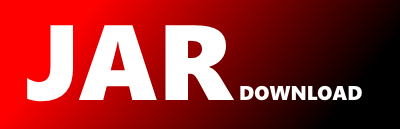
com.liferay.dispatch.service.persistence.DispatchTriggerUtil Maven / Gradle / Ivy
/**
* SPDX-FileCopyrightText: (c) 2000 Liferay, Inc. https://liferay.com
* SPDX-License-Identifier: LGPL-2.1-or-later OR LicenseRef-Liferay-DXP-EULA-2.0.0-2023-06
*/
package com.liferay.dispatch.service.persistence;
import com.liferay.dispatch.model.DispatchTrigger;
import com.liferay.portal.kernel.dao.orm.DynamicQuery;
import com.liferay.portal.kernel.service.ServiceContext;
import com.liferay.portal.kernel.util.OrderByComparator;
import java.io.Serializable;
import java.util.List;
import java.util.Map;
import java.util.Set;
/**
* The persistence utility for the dispatch trigger service. This utility wraps com.liferay.dispatch.service.persistence.impl.DispatchTriggerPersistenceImpl
and provides direct access to the database for CRUD operations. This utility should only be used by the service layer, as it must operate within a transaction. Never access this utility in a JSP, controller, model, or other front-end class.
*
*
* Caching information and settings can be found in portal.properties
*
*
* @author Matija Petanjek
* @see DispatchTriggerPersistence
* @generated
*/
public class DispatchTriggerUtil {
/*
* NOTE FOR DEVELOPERS:
*
* Never modify this class directly. Modify service.xml
and rerun ServiceBuilder to regenerate this class.
*/
/**
* @see com.liferay.portal.kernel.service.persistence.BasePersistence#clearCache()
*/
public static void clearCache() {
getPersistence().clearCache();
}
/**
* @see com.liferay.portal.kernel.service.persistence.BasePersistence#clearCache(com.liferay.portal.kernel.model.BaseModel)
*/
public static void clearCache(DispatchTrigger dispatchTrigger) {
getPersistence().clearCache(dispatchTrigger);
}
/**
* @see com.liferay.portal.kernel.service.persistence.BasePersistence#countWithDynamicQuery(DynamicQuery)
*/
public static long countWithDynamicQuery(DynamicQuery dynamicQuery) {
return getPersistence().countWithDynamicQuery(dynamicQuery);
}
/**
* @see com.liferay.portal.kernel.service.persistence.BasePersistence#fetchByPrimaryKeys(Set)
*/
public static Map fetchByPrimaryKeys(
Set primaryKeys) {
return getPersistence().fetchByPrimaryKeys(primaryKeys);
}
/**
* @see com.liferay.portal.kernel.service.persistence.BasePersistence#findWithDynamicQuery(DynamicQuery)
*/
public static List findWithDynamicQuery(
DynamicQuery dynamicQuery) {
return getPersistence().findWithDynamicQuery(dynamicQuery);
}
/**
* @see com.liferay.portal.kernel.service.persistence.BasePersistence#findWithDynamicQuery(DynamicQuery, int, int)
*/
public static List findWithDynamicQuery(
DynamicQuery dynamicQuery, int start, int end) {
return getPersistence().findWithDynamicQuery(dynamicQuery, start, end);
}
/**
* @see com.liferay.portal.kernel.service.persistence.BasePersistence#findWithDynamicQuery(DynamicQuery, int, int, OrderByComparator)
*/
public static List findWithDynamicQuery(
DynamicQuery dynamicQuery, int start, int end,
OrderByComparator orderByComparator) {
return getPersistence().findWithDynamicQuery(
dynamicQuery, start, end, orderByComparator);
}
/**
* @see com.liferay.portal.kernel.service.persistence.BasePersistence#update(com.liferay.portal.kernel.model.BaseModel)
*/
public static DispatchTrigger update(DispatchTrigger dispatchTrigger) {
return getPersistence().update(dispatchTrigger);
}
/**
* @see com.liferay.portal.kernel.service.persistence.BasePersistence#update(com.liferay.portal.kernel.model.BaseModel, ServiceContext)
*/
public static DispatchTrigger update(
DispatchTrigger dispatchTrigger, ServiceContext serviceContext) {
return getPersistence().update(dispatchTrigger, serviceContext);
}
/**
* Returns all the dispatch triggers where uuid = ?.
*
* @param uuid the uuid
* @return the matching dispatch triggers
*/
public static List findByUuid(String uuid) {
return getPersistence().findByUuid(uuid);
}
/**
* Returns a range of all the dispatch triggers where uuid = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from DispatchTriggerModelImpl
.
*
*
* @param uuid the uuid
* @param start the lower bound of the range of dispatch triggers
* @param end the upper bound of the range of dispatch triggers (not inclusive)
* @return the range of matching dispatch triggers
*/
public static List findByUuid(
String uuid, int start, int end) {
return getPersistence().findByUuid(uuid, start, end);
}
/**
* Returns an ordered range of all the dispatch triggers where uuid = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from DispatchTriggerModelImpl
.
*
*
* @param uuid the uuid
* @param start the lower bound of the range of dispatch triggers
* @param end the upper bound of the range of dispatch triggers (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching dispatch triggers
*/
public static List findByUuid(
String uuid, int start, int end,
OrderByComparator orderByComparator) {
return getPersistence().findByUuid(uuid, start, end, orderByComparator);
}
/**
* Returns an ordered range of all the dispatch triggers where uuid = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from DispatchTriggerModelImpl
.
*
*
* @param uuid the uuid
* @param start the lower bound of the range of dispatch triggers
* @param end the upper bound of the range of dispatch triggers (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching dispatch triggers
*/
public static List findByUuid(
String uuid, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
return getPersistence().findByUuid(
uuid, start, end, orderByComparator, useFinderCache);
}
/**
* Returns the first dispatch trigger in the ordered set where uuid = ?.
*
* @param uuid the uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching dispatch trigger
* @throws NoSuchTriggerException if a matching dispatch trigger could not be found
*/
public static DispatchTrigger findByUuid_First(
String uuid, OrderByComparator orderByComparator)
throws com.liferay.dispatch.exception.NoSuchTriggerException {
return getPersistence().findByUuid_First(uuid, orderByComparator);
}
/**
* Returns the first dispatch trigger in the ordered set where uuid = ?.
*
* @param uuid the uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching dispatch trigger, or null
if a matching dispatch trigger could not be found
*/
public static DispatchTrigger fetchByUuid_First(
String uuid, OrderByComparator orderByComparator) {
return getPersistence().fetchByUuid_First(uuid, orderByComparator);
}
/**
* Returns the last dispatch trigger in the ordered set where uuid = ?.
*
* @param uuid the uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching dispatch trigger
* @throws NoSuchTriggerException if a matching dispatch trigger could not be found
*/
public static DispatchTrigger findByUuid_Last(
String uuid, OrderByComparator orderByComparator)
throws com.liferay.dispatch.exception.NoSuchTriggerException {
return getPersistence().findByUuid_Last(uuid, orderByComparator);
}
/**
* Returns the last dispatch trigger in the ordered set where uuid = ?.
*
* @param uuid the uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching dispatch trigger, or null
if a matching dispatch trigger could not be found
*/
public static DispatchTrigger fetchByUuid_Last(
String uuid, OrderByComparator orderByComparator) {
return getPersistence().fetchByUuid_Last(uuid, orderByComparator);
}
/**
* Returns the dispatch triggers before and after the current dispatch trigger in the ordered set where uuid = ?.
*
* @param dispatchTriggerId the primary key of the current dispatch trigger
* @param uuid the uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next dispatch trigger
* @throws NoSuchTriggerException if a dispatch trigger with the primary key could not be found
*/
public static DispatchTrigger[] findByUuid_PrevAndNext(
long dispatchTriggerId, String uuid,
OrderByComparator orderByComparator)
throws com.liferay.dispatch.exception.NoSuchTriggerException {
return getPersistence().findByUuid_PrevAndNext(
dispatchTriggerId, uuid, orderByComparator);
}
/**
* Returns all the dispatch triggers that the user has permission to view where uuid = ?.
*
* @param uuid the uuid
* @return the matching dispatch triggers that the user has permission to view
*/
public static List filterFindByUuid(String uuid) {
return getPersistence().filterFindByUuid(uuid);
}
/**
* Returns a range of all the dispatch triggers that the user has permission to view where uuid = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from DispatchTriggerModelImpl
.
*
*
* @param uuid the uuid
* @param start the lower bound of the range of dispatch triggers
* @param end the upper bound of the range of dispatch triggers (not inclusive)
* @return the range of matching dispatch triggers that the user has permission to view
*/
public static List filterFindByUuid(
String uuid, int start, int end) {
return getPersistence().filterFindByUuid(uuid, start, end);
}
/**
* Returns an ordered range of all the dispatch triggers that the user has permissions to view where uuid = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from DispatchTriggerModelImpl
.
*
*
* @param uuid the uuid
* @param start the lower bound of the range of dispatch triggers
* @param end the upper bound of the range of dispatch triggers (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching dispatch triggers that the user has permission to view
*/
public static List filterFindByUuid(
String uuid, int start, int end,
OrderByComparator orderByComparator) {
return getPersistence().filterFindByUuid(
uuid, start, end, orderByComparator);
}
/**
* Returns the dispatch triggers before and after the current dispatch trigger in the ordered set of dispatch triggers that the user has permission to view where uuid = ?.
*
* @param dispatchTriggerId the primary key of the current dispatch trigger
* @param uuid the uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next dispatch trigger
* @throws NoSuchTriggerException if a dispatch trigger with the primary key could not be found
*/
public static DispatchTrigger[] filterFindByUuid_PrevAndNext(
long dispatchTriggerId, String uuid,
OrderByComparator orderByComparator)
throws com.liferay.dispatch.exception.NoSuchTriggerException {
return getPersistence().filterFindByUuid_PrevAndNext(
dispatchTriggerId, uuid, orderByComparator);
}
/**
* Removes all the dispatch triggers where uuid = ? from the database.
*
* @param uuid the uuid
*/
public static void removeByUuid(String uuid) {
getPersistence().removeByUuid(uuid);
}
/**
* Returns the number of dispatch triggers where uuid = ?.
*
* @param uuid the uuid
* @return the number of matching dispatch triggers
*/
public static int countByUuid(String uuid) {
return getPersistence().countByUuid(uuid);
}
/**
* Returns the number of dispatch triggers that the user has permission to view where uuid = ?.
*
* @param uuid the uuid
* @return the number of matching dispatch triggers that the user has permission to view
*/
public static int filterCountByUuid(String uuid) {
return getPersistence().filterCountByUuid(uuid);
}
/**
* Returns all the dispatch triggers where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @return the matching dispatch triggers
*/
public static List findByUuid_C(
String uuid, long companyId) {
return getPersistence().findByUuid_C(uuid, companyId);
}
/**
* Returns a range of all the dispatch triggers where uuid = ? and companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from DispatchTriggerModelImpl
.
*
*
* @param uuid the uuid
* @param companyId the company ID
* @param start the lower bound of the range of dispatch triggers
* @param end the upper bound of the range of dispatch triggers (not inclusive)
* @return the range of matching dispatch triggers
*/
public static List findByUuid_C(
String uuid, long companyId, int start, int end) {
return getPersistence().findByUuid_C(uuid, companyId, start, end);
}
/**
* Returns an ordered range of all the dispatch triggers where uuid = ? and companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from DispatchTriggerModelImpl
.
*
*
* @param uuid the uuid
* @param companyId the company ID
* @param start the lower bound of the range of dispatch triggers
* @param end the upper bound of the range of dispatch triggers (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching dispatch triggers
*/
public static List findByUuid_C(
String uuid, long companyId, int start, int end,
OrderByComparator orderByComparator) {
return getPersistence().findByUuid_C(
uuid, companyId, start, end, orderByComparator);
}
/**
* Returns an ordered range of all the dispatch triggers where uuid = ? and companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from DispatchTriggerModelImpl
.
*
*
* @param uuid the uuid
* @param companyId the company ID
* @param start the lower bound of the range of dispatch triggers
* @param end the upper bound of the range of dispatch triggers (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching dispatch triggers
*/
public static List findByUuid_C(
String uuid, long companyId, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
return getPersistence().findByUuid_C(
uuid, companyId, start, end, orderByComparator, useFinderCache);
}
/**
* Returns the first dispatch trigger in the ordered set where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching dispatch trigger
* @throws NoSuchTriggerException if a matching dispatch trigger could not be found
*/
public static DispatchTrigger findByUuid_C_First(
String uuid, long companyId,
OrderByComparator orderByComparator)
throws com.liferay.dispatch.exception.NoSuchTriggerException {
return getPersistence().findByUuid_C_First(
uuid, companyId, orderByComparator);
}
/**
* Returns the first dispatch trigger in the ordered set where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching dispatch trigger, or null
if a matching dispatch trigger could not be found
*/
public static DispatchTrigger fetchByUuid_C_First(
String uuid, long companyId,
OrderByComparator orderByComparator) {
return getPersistence().fetchByUuid_C_First(
uuid, companyId, orderByComparator);
}
/**
* Returns the last dispatch trigger in the ordered set where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching dispatch trigger
* @throws NoSuchTriggerException if a matching dispatch trigger could not be found
*/
public static DispatchTrigger findByUuid_C_Last(
String uuid, long companyId,
OrderByComparator orderByComparator)
throws com.liferay.dispatch.exception.NoSuchTriggerException {
return getPersistence().findByUuid_C_Last(
uuid, companyId, orderByComparator);
}
/**
* Returns the last dispatch trigger in the ordered set where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching dispatch trigger, or null
if a matching dispatch trigger could not be found
*/
public static DispatchTrigger fetchByUuid_C_Last(
String uuid, long companyId,
OrderByComparator orderByComparator) {
return getPersistence().fetchByUuid_C_Last(
uuid, companyId, orderByComparator);
}
/**
* Returns the dispatch triggers before and after the current dispatch trigger in the ordered set where uuid = ? and companyId = ?.
*
* @param dispatchTriggerId the primary key of the current dispatch trigger
* @param uuid the uuid
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next dispatch trigger
* @throws NoSuchTriggerException if a dispatch trigger with the primary key could not be found
*/
public static DispatchTrigger[] findByUuid_C_PrevAndNext(
long dispatchTriggerId, String uuid, long companyId,
OrderByComparator orderByComparator)
throws com.liferay.dispatch.exception.NoSuchTriggerException {
return getPersistence().findByUuid_C_PrevAndNext(
dispatchTriggerId, uuid, companyId, orderByComparator);
}
/**
* Returns all the dispatch triggers that the user has permission to view where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @return the matching dispatch triggers that the user has permission to view
*/
public static List filterFindByUuid_C(
String uuid, long companyId) {
return getPersistence().filterFindByUuid_C(uuid, companyId);
}
/**
* Returns a range of all the dispatch triggers that the user has permission to view where uuid = ? and companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from DispatchTriggerModelImpl
.
*
*
* @param uuid the uuid
* @param companyId the company ID
* @param start the lower bound of the range of dispatch triggers
* @param end the upper bound of the range of dispatch triggers (not inclusive)
* @return the range of matching dispatch triggers that the user has permission to view
*/
public static List filterFindByUuid_C(
String uuid, long companyId, int start, int end) {
return getPersistence().filterFindByUuid_C(uuid, companyId, start, end);
}
/**
* Returns an ordered range of all the dispatch triggers that the user has permissions to view where uuid = ? and companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from DispatchTriggerModelImpl
.
*
*
* @param uuid the uuid
* @param companyId the company ID
* @param start the lower bound of the range of dispatch triggers
* @param end the upper bound of the range of dispatch triggers (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching dispatch triggers that the user has permission to view
*/
public static List filterFindByUuid_C(
String uuid, long companyId, int start, int end,
OrderByComparator orderByComparator) {
return getPersistence().filterFindByUuid_C(
uuid, companyId, start, end, orderByComparator);
}
/**
* Returns the dispatch triggers before and after the current dispatch trigger in the ordered set of dispatch triggers that the user has permission to view where uuid = ? and companyId = ?.
*
* @param dispatchTriggerId the primary key of the current dispatch trigger
* @param uuid the uuid
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next dispatch trigger
* @throws NoSuchTriggerException if a dispatch trigger with the primary key could not be found
*/
public static DispatchTrigger[] filterFindByUuid_C_PrevAndNext(
long dispatchTriggerId, String uuid, long companyId,
OrderByComparator orderByComparator)
throws com.liferay.dispatch.exception.NoSuchTriggerException {
return getPersistence().filterFindByUuid_C_PrevAndNext(
dispatchTriggerId, uuid, companyId, orderByComparator);
}
/**
* Removes all the dispatch triggers where uuid = ? and companyId = ? from the database.
*
* @param uuid the uuid
* @param companyId the company ID
*/
public static void removeByUuid_C(String uuid, long companyId) {
getPersistence().removeByUuid_C(uuid, companyId);
}
/**
* Returns the number of dispatch triggers where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @return the number of matching dispatch triggers
*/
public static int countByUuid_C(String uuid, long companyId) {
return getPersistence().countByUuid_C(uuid, companyId);
}
/**
* Returns the number of dispatch triggers that the user has permission to view where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @return the number of matching dispatch triggers that the user has permission to view
*/
public static int filterCountByUuid_C(String uuid, long companyId) {
return getPersistence().filterCountByUuid_C(uuid, companyId);
}
/**
* Returns all the dispatch triggers where companyId = ?.
*
* @param companyId the company ID
* @return the matching dispatch triggers
*/
public static List findByCompanyId(long companyId) {
return getPersistence().findByCompanyId(companyId);
}
/**
* Returns a range of all the dispatch triggers where companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from DispatchTriggerModelImpl
.
*
*
* @param companyId the company ID
* @param start the lower bound of the range of dispatch triggers
* @param end the upper bound of the range of dispatch triggers (not inclusive)
* @return the range of matching dispatch triggers
*/
public static List findByCompanyId(
long companyId, int start, int end) {
return getPersistence().findByCompanyId(companyId, start, end);
}
/**
* Returns an ordered range of all the dispatch triggers where companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from DispatchTriggerModelImpl
.
*
*
* @param companyId the company ID
* @param start the lower bound of the range of dispatch triggers
* @param end the upper bound of the range of dispatch triggers (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching dispatch triggers
*/
public static List findByCompanyId(
long companyId, int start, int end,
OrderByComparator orderByComparator) {
return getPersistence().findByCompanyId(
companyId, start, end, orderByComparator);
}
/**
* Returns an ordered range of all the dispatch triggers where companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from DispatchTriggerModelImpl
.
*
*
* @param companyId the company ID
* @param start the lower bound of the range of dispatch triggers
* @param end the upper bound of the range of dispatch triggers (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching dispatch triggers
*/
public static List findByCompanyId(
long companyId, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
return getPersistence().findByCompanyId(
companyId, start, end, orderByComparator, useFinderCache);
}
/**
* Returns the first dispatch trigger in the ordered set where companyId = ?.
*
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching dispatch trigger
* @throws NoSuchTriggerException if a matching dispatch trigger could not be found
*/
public static DispatchTrigger findByCompanyId_First(
long companyId,
OrderByComparator orderByComparator)
throws com.liferay.dispatch.exception.NoSuchTriggerException {
return getPersistence().findByCompanyId_First(
companyId, orderByComparator);
}
/**
* Returns the first dispatch trigger in the ordered set where companyId = ?.
*
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching dispatch trigger, or null
if a matching dispatch trigger could not be found
*/
public static DispatchTrigger fetchByCompanyId_First(
long companyId, OrderByComparator orderByComparator) {
return getPersistence().fetchByCompanyId_First(
companyId, orderByComparator);
}
/**
* Returns the last dispatch trigger in the ordered set where companyId = ?.
*
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching dispatch trigger
* @throws NoSuchTriggerException if a matching dispatch trigger could not be found
*/
public static DispatchTrigger findByCompanyId_Last(
long companyId,
OrderByComparator orderByComparator)
throws com.liferay.dispatch.exception.NoSuchTriggerException {
return getPersistence().findByCompanyId_Last(
companyId, orderByComparator);
}
/**
* Returns the last dispatch trigger in the ordered set where companyId = ?.
*
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching dispatch trigger, or null
if a matching dispatch trigger could not be found
*/
public static DispatchTrigger fetchByCompanyId_Last(
long companyId, OrderByComparator orderByComparator) {
return getPersistence().fetchByCompanyId_Last(
companyId, orderByComparator);
}
/**
* Returns the dispatch triggers before and after the current dispatch trigger in the ordered set where companyId = ?.
*
* @param dispatchTriggerId the primary key of the current dispatch trigger
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next dispatch trigger
* @throws NoSuchTriggerException if a dispatch trigger with the primary key could not be found
*/
public static DispatchTrigger[] findByCompanyId_PrevAndNext(
long dispatchTriggerId, long companyId,
OrderByComparator orderByComparator)
throws com.liferay.dispatch.exception.NoSuchTriggerException {
return getPersistence().findByCompanyId_PrevAndNext(
dispatchTriggerId, companyId, orderByComparator);
}
/**
* Returns all the dispatch triggers that the user has permission to view where companyId = ?.
*
* @param companyId the company ID
* @return the matching dispatch triggers that the user has permission to view
*/
public static List filterFindByCompanyId(long companyId) {
return getPersistence().filterFindByCompanyId(companyId);
}
/**
* Returns a range of all the dispatch triggers that the user has permission to view where companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from DispatchTriggerModelImpl
.
*
*
* @param companyId the company ID
* @param start the lower bound of the range of dispatch triggers
* @param end the upper bound of the range of dispatch triggers (not inclusive)
* @return the range of matching dispatch triggers that the user has permission to view
*/
public static List filterFindByCompanyId(
long companyId, int start, int end) {
return getPersistence().filterFindByCompanyId(companyId, start, end);
}
/**
* Returns an ordered range of all the dispatch triggers that the user has permissions to view where companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from DispatchTriggerModelImpl
.
*
*
* @param companyId the company ID
* @param start the lower bound of the range of dispatch triggers
* @param end the upper bound of the range of dispatch triggers (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching dispatch triggers that the user has permission to view
*/
public static List filterFindByCompanyId(
long companyId, int start, int end,
OrderByComparator orderByComparator) {
return getPersistence().filterFindByCompanyId(
companyId, start, end, orderByComparator);
}
/**
* Returns the dispatch triggers before and after the current dispatch trigger in the ordered set of dispatch triggers that the user has permission to view where companyId = ?.
*
* @param dispatchTriggerId the primary key of the current dispatch trigger
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next dispatch trigger
* @throws NoSuchTriggerException if a dispatch trigger with the primary key could not be found
*/
public static DispatchTrigger[] filterFindByCompanyId_PrevAndNext(
long dispatchTriggerId, long companyId,
OrderByComparator orderByComparator)
throws com.liferay.dispatch.exception.NoSuchTriggerException {
return getPersistence().filterFindByCompanyId_PrevAndNext(
dispatchTriggerId, companyId, orderByComparator);
}
/**
* Removes all the dispatch triggers where companyId = ? from the database.
*
* @param companyId the company ID
*/
public static void removeByCompanyId(long companyId) {
getPersistence().removeByCompanyId(companyId);
}
/**
* Returns the number of dispatch triggers where companyId = ?.
*
* @param companyId the company ID
* @return the number of matching dispatch triggers
*/
public static int countByCompanyId(long companyId) {
return getPersistence().countByCompanyId(companyId);
}
/**
* Returns the number of dispatch triggers that the user has permission to view where companyId = ?.
*
* @param companyId the company ID
* @return the number of matching dispatch triggers that the user has permission to view
*/
public static int filterCountByCompanyId(long companyId) {
return getPersistence().filterCountByCompanyId(companyId);
}
/**
* Returns all the dispatch triggers where active = ?.
*
* @param active the active
* @return the matching dispatch triggers
*/
public static List findByActive(boolean active) {
return getPersistence().findByActive(active);
}
/**
* Returns a range of all the dispatch triggers where active = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from DispatchTriggerModelImpl
.
*
*
* @param active the active
* @param start the lower bound of the range of dispatch triggers
* @param end the upper bound of the range of dispatch triggers (not inclusive)
* @return the range of matching dispatch triggers
*/
public static List findByActive(
boolean active, int start, int end) {
return getPersistence().findByActive(active, start, end);
}
/**
* Returns an ordered range of all the dispatch triggers where active = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from DispatchTriggerModelImpl
.
*
*
* @param active the active
* @param start the lower bound of the range of dispatch triggers
* @param end the upper bound of the range of dispatch triggers (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching dispatch triggers
*/
public static List findByActive(
boolean active, int start, int end,
OrderByComparator orderByComparator) {
return getPersistence().findByActive(
active, start, end, orderByComparator);
}
/**
* Returns an ordered range of all the dispatch triggers where active = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from DispatchTriggerModelImpl
.
*
*
* @param active the active
* @param start the lower bound of the range of dispatch triggers
* @param end the upper bound of the range of dispatch triggers (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching dispatch triggers
*/
public static List findByActive(
boolean active, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
return getPersistence().findByActive(
active, start, end, orderByComparator, useFinderCache);
}
/**
* Returns the first dispatch trigger in the ordered set where active = ?.
*
* @param active the active
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching dispatch trigger
* @throws NoSuchTriggerException if a matching dispatch trigger could not be found
*/
public static DispatchTrigger findByActive_First(
boolean active,
OrderByComparator orderByComparator)
throws com.liferay.dispatch.exception.NoSuchTriggerException {
return getPersistence().findByActive_First(active, orderByComparator);
}
/**
* Returns the first dispatch trigger in the ordered set where active = ?.
*
* @param active the active
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching dispatch trigger, or null
if a matching dispatch trigger could not be found
*/
public static DispatchTrigger fetchByActive_First(
boolean active, OrderByComparator orderByComparator) {
return getPersistence().fetchByActive_First(active, orderByComparator);
}
/**
* Returns the last dispatch trigger in the ordered set where active = ?.
*
* @param active the active
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching dispatch trigger
* @throws NoSuchTriggerException if a matching dispatch trigger could not be found
*/
public static DispatchTrigger findByActive_Last(
boolean active,
OrderByComparator orderByComparator)
throws com.liferay.dispatch.exception.NoSuchTriggerException {
return getPersistence().findByActive_Last(active, orderByComparator);
}
/**
* Returns the last dispatch trigger in the ordered set where active = ?.
*
* @param active the active
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching dispatch trigger, or null
if a matching dispatch trigger could not be found
*/
public static DispatchTrigger fetchByActive_Last(
boolean active, OrderByComparator orderByComparator) {
return getPersistence().fetchByActive_Last(active, orderByComparator);
}
/**
* Returns the dispatch triggers before and after the current dispatch trigger in the ordered set where active = ?.
*
* @param dispatchTriggerId the primary key of the current dispatch trigger
* @param active the active
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next dispatch trigger
* @throws NoSuchTriggerException if a dispatch trigger with the primary key could not be found
*/
public static DispatchTrigger[] findByActive_PrevAndNext(
long dispatchTriggerId, boolean active,
OrderByComparator orderByComparator)
throws com.liferay.dispatch.exception.NoSuchTriggerException {
return getPersistence().findByActive_PrevAndNext(
dispatchTriggerId, active, orderByComparator);
}
/**
* Returns all the dispatch triggers that the user has permission to view where active = ?.
*
* @param active the active
* @return the matching dispatch triggers that the user has permission to view
*/
public static List filterFindByActive(boolean active) {
return getPersistence().filterFindByActive(active);
}
/**
* Returns a range of all the dispatch triggers that the user has permission to view where active = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from DispatchTriggerModelImpl
.
*
*
* @param active the active
* @param start the lower bound of the range of dispatch triggers
* @param end the upper bound of the range of dispatch triggers (not inclusive)
* @return the range of matching dispatch triggers that the user has permission to view
*/
public static List filterFindByActive(
boolean active, int start, int end) {
return getPersistence().filterFindByActive(active, start, end);
}
/**
* Returns an ordered range of all the dispatch triggers that the user has permissions to view where active = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from DispatchTriggerModelImpl
.
*
*
* @param active the active
* @param start the lower bound of the range of dispatch triggers
* @param end the upper bound of the range of dispatch triggers (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching dispatch triggers that the user has permission to view
*/
public static List filterFindByActive(
boolean active, int start, int end,
OrderByComparator orderByComparator) {
return getPersistence().filterFindByActive(
active, start, end, orderByComparator);
}
/**
* Returns the dispatch triggers before and after the current dispatch trigger in the ordered set of dispatch triggers that the user has permission to view where active = ?.
*
* @param dispatchTriggerId the primary key of the current dispatch trigger
* @param active the active
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next dispatch trigger
* @throws NoSuchTriggerException if a dispatch trigger with the primary key could not be found
*/
public static DispatchTrigger[] filterFindByActive_PrevAndNext(
long dispatchTriggerId, boolean active,
OrderByComparator orderByComparator)
throws com.liferay.dispatch.exception.NoSuchTriggerException {
return getPersistence().filterFindByActive_PrevAndNext(
dispatchTriggerId, active, orderByComparator);
}
/**
* Removes all the dispatch triggers where active = ? from the database.
*
* @param active the active
*/
public static void removeByActive(boolean active) {
getPersistence().removeByActive(active);
}
/**
* Returns the number of dispatch triggers where active = ?.
*
* @param active the active
* @return the number of matching dispatch triggers
*/
public static int countByActive(boolean active) {
return getPersistence().countByActive(active);
}
/**
* Returns the number of dispatch triggers that the user has permission to view where active = ?.
*
* @param active the active
* @return the number of matching dispatch triggers that the user has permission to view
*/
public static int filterCountByActive(boolean active) {
return getPersistence().filterCountByActive(active);
}
/**
* Returns all the dispatch triggers where companyId = ? and userId = ?.
*
* @param companyId the company ID
* @param userId the user ID
* @return the matching dispatch triggers
*/
public static List findByC_U(long companyId, long userId) {
return getPersistence().findByC_U(companyId, userId);
}
/**
* Returns a range of all the dispatch triggers where companyId = ? and userId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from DispatchTriggerModelImpl
.
*
*
* @param companyId the company ID
* @param userId the user ID
* @param start the lower bound of the range of dispatch triggers
* @param end the upper bound of the range of dispatch triggers (not inclusive)
* @return the range of matching dispatch triggers
*/
public static List findByC_U(
long companyId, long userId, int start, int end) {
return getPersistence().findByC_U(companyId, userId, start, end);
}
/**
* Returns an ordered range of all the dispatch triggers where companyId = ? and userId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from DispatchTriggerModelImpl
.
*
*
* @param companyId the company ID
* @param userId the user ID
* @param start the lower bound of the range of dispatch triggers
* @param end the upper bound of the range of dispatch triggers (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching dispatch triggers
*/
public static List findByC_U(
long companyId, long userId, int start, int end,
OrderByComparator orderByComparator) {
return getPersistence().findByC_U(
companyId, userId, start, end, orderByComparator);
}
/**
* Returns an ordered range of all the dispatch triggers where companyId = ? and userId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from DispatchTriggerModelImpl
.
*
*
* @param companyId the company ID
* @param userId the user ID
* @param start the lower bound of the range of dispatch triggers
* @param end the upper bound of the range of dispatch triggers (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching dispatch triggers
*/
public static List findByC_U(
long companyId, long userId, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
return getPersistence().findByC_U(
companyId, userId, start, end, orderByComparator, useFinderCache);
}
/**
* Returns the first dispatch trigger in the ordered set where companyId = ? and userId = ?.
*
* @param companyId the company ID
* @param userId the user ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching dispatch trigger
* @throws NoSuchTriggerException if a matching dispatch trigger could not be found
*/
public static DispatchTrigger findByC_U_First(
long companyId, long userId,
OrderByComparator orderByComparator)
throws com.liferay.dispatch.exception.NoSuchTriggerException {
return getPersistence().findByC_U_First(
companyId, userId, orderByComparator);
}
/**
* Returns the first dispatch trigger in the ordered set where companyId = ? and userId = ?.
*
* @param companyId the company ID
* @param userId the user ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching dispatch trigger, or null
if a matching dispatch trigger could not be found
*/
public static DispatchTrigger fetchByC_U_First(
long companyId, long userId,
OrderByComparator orderByComparator) {
return getPersistence().fetchByC_U_First(
companyId, userId, orderByComparator);
}
/**
* Returns the last dispatch trigger in the ordered set where companyId = ? and userId = ?.
*
* @param companyId the company ID
* @param userId the user ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching dispatch trigger
* @throws NoSuchTriggerException if a matching dispatch trigger could not be found
*/
public static DispatchTrigger findByC_U_Last(
long companyId, long userId,
OrderByComparator orderByComparator)
throws com.liferay.dispatch.exception.NoSuchTriggerException {
return getPersistence().findByC_U_Last(
companyId, userId, orderByComparator);
}
/**
* Returns the last dispatch trigger in the ordered set where companyId = ? and userId = ?.
*
* @param companyId the company ID
* @param userId the user ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching dispatch trigger, or null
if a matching dispatch trigger could not be found
*/
public static DispatchTrigger fetchByC_U_Last(
long companyId, long userId,
OrderByComparator orderByComparator) {
return getPersistence().fetchByC_U_Last(
companyId, userId, orderByComparator);
}
/**
* Returns the dispatch triggers before and after the current dispatch trigger in the ordered set where companyId = ? and userId = ?.
*
* @param dispatchTriggerId the primary key of the current dispatch trigger
* @param companyId the company ID
* @param userId the user ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next dispatch trigger
* @throws NoSuchTriggerException if a dispatch trigger with the primary key could not be found
*/
public static DispatchTrigger[] findByC_U_PrevAndNext(
long dispatchTriggerId, long companyId, long userId,
OrderByComparator orderByComparator)
throws com.liferay.dispatch.exception.NoSuchTriggerException {
return getPersistence().findByC_U_PrevAndNext(
dispatchTriggerId, companyId, userId, orderByComparator);
}
/**
* Returns all the dispatch triggers that the user has permission to view where companyId = ? and userId = ?.
*
* @param companyId the company ID
* @param userId the user ID
* @return the matching dispatch triggers that the user has permission to view
*/
public static List filterFindByC_U(
long companyId, long userId) {
return getPersistence().filterFindByC_U(companyId, userId);
}
/**
* Returns a range of all the dispatch triggers that the user has permission to view where companyId = ? and userId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from DispatchTriggerModelImpl
.
*
*
* @param companyId the company ID
* @param userId the user ID
* @param start the lower bound of the range of dispatch triggers
* @param end the upper bound of the range of dispatch triggers (not inclusive)
* @return the range of matching dispatch triggers that the user has permission to view
*/
public static List filterFindByC_U(
long companyId, long userId, int start, int end) {
return getPersistence().filterFindByC_U(companyId, userId, start, end);
}
/**
* Returns an ordered range of all the dispatch triggers that the user has permissions to view where companyId = ? and userId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from DispatchTriggerModelImpl
.
*
*
* @param companyId the company ID
* @param userId the user ID
* @param start the lower bound of the range of dispatch triggers
* @param end the upper bound of the range of dispatch triggers (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching dispatch triggers that the user has permission to view
*/
public static List filterFindByC_U(
long companyId, long userId, int start, int end,
OrderByComparator orderByComparator) {
return getPersistence().filterFindByC_U(
companyId, userId, start, end, orderByComparator);
}
/**
* Returns the dispatch triggers before and after the current dispatch trigger in the ordered set of dispatch triggers that the user has permission to view where companyId = ? and userId = ?.
*
* @param dispatchTriggerId the primary key of the current dispatch trigger
* @param companyId the company ID
* @param userId the user ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next dispatch trigger
* @throws NoSuchTriggerException if a dispatch trigger with the primary key could not be found
*/
public static DispatchTrigger[] filterFindByC_U_PrevAndNext(
long dispatchTriggerId, long companyId, long userId,
OrderByComparator orderByComparator)
throws com.liferay.dispatch.exception.NoSuchTriggerException {
return getPersistence().filterFindByC_U_PrevAndNext(
dispatchTriggerId, companyId, userId, orderByComparator);
}
/**
* Removes all the dispatch triggers where companyId = ? and userId = ? from the database.
*
* @param companyId the company ID
* @param userId the user ID
*/
public static void removeByC_U(long companyId, long userId) {
getPersistence().removeByC_U(companyId, userId);
}
/**
* Returns the number of dispatch triggers where companyId = ? and userId = ?.
*
* @param companyId the company ID
* @param userId the user ID
* @return the number of matching dispatch triggers
*/
public static int countByC_U(long companyId, long userId) {
return getPersistence().countByC_U(companyId, userId);
}
/**
* Returns the number of dispatch triggers that the user has permission to view where companyId = ? and userId = ?.
*
* @param companyId the company ID
* @param userId the user ID
* @return the number of matching dispatch triggers that the user has permission to view
*/
public static int filterCountByC_U(long companyId, long userId) {
return getPersistence().filterCountByC_U(companyId, userId);
}
/**
* Returns all the dispatch triggers where companyId = ? and dispatchTaskExecutorType = ?.
*
* @param companyId the company ID
* @param dispatchTaskExecutorType the dispatch task executor type
* @return the matching dispatch triggers
*/
public static List findByC_DTET(
long companyId, String dispatchTaskExecutorType) {
return getPersistence().findByC_DTET(
companyId, dispatchTaskExecutorType);
}
/**
* Returns a range of all the dispatch triggers where companyId = ? and dispatchTaskExecutorType = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from DispatchTriggerModelImpl
.
*
*
* @param companyId the company ID
* @param dispatchTaskExecutorType the dispatch task executor type
* @param start the lower bound of the range of dispatch triggers
* @param end the upper bound of the range of dispatch triggers (not inclusive)
* @return the range of matching dispatch triggers
*/
public static List findByC_DTET(
long companyId, String dispatchTaskExecutorType, int start, int end) {
return getPersistence().findByC_DTET(
companyId, dispatchTaskExecutorType, start, end);
}
/**
* Returns an ordered range of all the dispatch triggers where companyId = ? and dispatchTaskExecutorType = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from DispatchTriggerModelImpl
.
*
*
* @param companyId the company ID
* @param dispatchTaskExecutorType the dispatch task executor type
* @param start the lower bound of the range of dispatch triggers
* @param end the upper bound of the range of dispatch triggers (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching dispatch triggers
*/
public static List findByC_DTET(
long companyId, String dispatchTaskExecutorType, int start, int end,
OrderByComparator orderByComparator) {
return getPersistence().findByC_DTET(
companyId, dispatchTaskExecutorType, start, end, orderByComparator);
}
/**
* Returns an ordered range of all the dispatch triggers where companyId = ? and dispatchTaskExecutorType = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from DispatchTriggerModelImpl
.
*
*
* @param companyId the company ID
* @param dispatchTaskExecutorType the dispatch task executor type
* @param start the lower bound of the range of dispatch triggers
* @param end the upper bound of the range of dispatch triggers (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching dispatch triggers
*/
public static List findByC_DTET(
long companyId, String dispatchTaskExecutorType, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
return getPersistence().findByC_DTET(
companyId, dispatchTaskExecutorType, start, end, orderByComparator,
useFinderCache);
}
/**
* Returns the first dispatch trigger in the ordered set where companyId = ? and dispatchTaskExecutorType = ?.
*
* @param companyId the company ID
* @param dispatchTaskExecutorType the dispatch task executor type
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching dispatch trigger
* @throws NoSuchTriggerException if a matching dispatch trigger could not be found
*/
public static DispatchTrigger findByC_DTET_First(
long companyId, String dispatchTaskExecutorType,
OrderByComparator orderByComparator)
throws com.liferay.dispatch.exception.NoSuchTriggerException {
return getPersistence().findByC_DTET_First(
companyId, dispatchTaskExecutorType, orderByComparator);
}
/**
* Returns the first dispatch trigger in the ordered set where companyId = ? and dispatchTaskExecutorType = ?.
*
* @param companyId the company ID
* @param dispatchTaskExecutorType the dispatch task executor type
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching dispatch trigger, or null
if a matching dispatch trigger could not be found
*/
public static DispatchTrigger fetchByC_DTET_First(
long companyId, String dispatchTaskExecutorType,
OrderByComparator orderByComparator) {
return getPersistence().fetchByC_DTET_First(
companyId, dispatchTaskExecutorType, orderByComparator);
}
/**
* Returns the last dispatch trigger in the ordered set where companyId = ? and dispatchTaskExecutorType = ?.
*
* @param companyId the company ID
* @param dispatchTaskExecutorType the dispatch task executor type
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching dispatch trigger
* @throws NoSuchTriggerException if a matching dispatch trigger could not be found
*/
public static DispatchTrigger findByC_DTET_Last(
long companyId, String dispatchTaskExecutorType,
OrderByComparator orderByComparator)
throws com.liferay.dispatch.exception.NoSuchTriggerException {
return getPersistence().findByC_DTET_Last(
companyId, dispatchTaskExecutorType, orderByComparator);
}
/**
* Returns the last dispatch trigger in the ordered set where companyId = ? and dispatchTaskExecutorType = ?.
*
* @param companyId the company ID
* @param dispatchTaskExecutorType the dispatch task executor type
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching dispatch trigger, or null
if a matching dispatch trigger could not be found
*/
public static DispatchTrigger fetchByC_DTET_Last(
long companyId, String dispatchTaskExecutorType,
OrderByComparator orderByComparator) {
return getPersistence().fetchByC_DTET_Last(
companyId, dispatchTaskExecutorType, orderByComparator);
}
/**
* Returns the dispatch triggers before and after the current dispatch trigger in the ordered set where companyId = ? and dispatchTaskExecutorType = ?.
*
* @param dispatchTriggerId the primary key of the current dispatch trigger
* @param companyId the company ID
* @param dispatchTaskExecutorType the dispatch task executor type
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next dispatch trigger
* @throws NoSuchTriggerException if a dispatch trigger with the primary key could not be found
*/
public static DispatchTrigger[] findByC_DTET_PrevAndNext(
long dispatchTriggerId, long companyId,
String dispatchTaskExecutorType,
OrderByComparator orderByComparator)
throws com.liferay.dispatch.exception.NoSuchTriggerException {
return getPersistence().findByC_DTET_PrevAndNext(
dispatchTriggerId, companyId, dispatchTaskExecutorType,
orderByComparator);
}
/**
* Returns all the dispatch triggers that the user has permission to view where companyId = ? and dispatchTaskExecutorType = ?.
*
* @param companyId the company ID
* @param dispatchTaskExecutorType the dispatch task executor type
* @return the matching dispatch triggers that the user has permission to view
*/
public static List filterFindByC_DTET(
long companyId, String dispatchTaskExecutorType) {
return getPersistence().filterFindByC_DTET(
companyId, dispatchTaskExecutorType);
}
/**
* Returns a range of all the dispatch triggers that the user has permission to view where companyId = ? and dispatchTaskExecutorType = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from DispatchTriggerModelImpl
.
*
*
* @param companyId the company ID
* @param dispatchTaskExecutorType the dispatch task executor type
* @param start the lower bound of the range of dispatch triggers
* @param end the upper bound of the range of dispatch triggers (not inclusive)
* @return the range of matching dispatch triggers that the user has permission to view
*/
public static List filterFindByC_DTET(
long companyId, String dispatchTaskExecutorType, int start, int end) {
return getPersistence().filterFindByC_DTET(
companyId, dispatchTaskExecutorType, start, end);
}
/**
* Returns an ordered range of all the dispatch triggers that the user has permissions to view where companyId = ? and dispatchTaskExecutorType = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from DispatchTriggerModelImpl
.
*
*
* @param companyId the company ID
* @param dispatchTaskExecutorType the dispatch task executor type
* @param start the lower bound of the range of dispatch triggers
* @param end the upper bound of the range of dispatch triggers (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching dispatch triggers that the user has permission to view
*/
public static List filterFindByC_DTET(
long companyId, String dispatchTaskExecutorType, int start, int end,
OrderByComparator orderByComparator) {
return getPersistence().filterFindByC_DTET(
companyId, dispatchTaskExecutorType, start, end, orderByComparator);
}
/**
* Returns the dispatch triggers before and after the current dispatch trigger in the ordered set of dispatch triggers that the user has permission to view where companyId = ? and dispatchTaskExecutorType = ?.
*
* @param dispatchTriggerId the primary key of the current dispatch trigger
* @param companyId the company ID
* @param dispatchTaskExecutorType the dispatch task executor type
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next dispatch trigger
* @throws NoSuchTriggerException if a dispatch trigger with the primary key could not be found
*/
public static DispatchTrigger[] filterFindByC_DTET_PrevAndNext(
long dispatchTriggerId, long companyId,
String dispatchTaskExecutorType,
OrderByComparator orderByComparator)
throws com.liferay.dispatch.exception.NoSuchTriggerException {
return getPersistence().filterFindByC_DTET_PrevAndNext(
dispatchTriggerId, companyId, dispatchTaskExecutorType,
orderByComparator);
}
/**
* Removes all the dispatch triggers where companyId = ? and dispatchTaskExecutorType = ? from the database.
*
* @param companyId the company ID
* @param dispatchTaskExecutorType the dispatch task executor type
*/
public static void removeByC_DTET(
long companyId, String dispatchTaskExecutorType) {
getPersistence().removeByC_DTET(companyId, dispatchTaskExecutorType);
}
/**
* Returns the number of dispatch triggers where companyId = ? and dispatchTaskExecutorType = ?.
*
* @param companyId the company ID
* @param dispatchTaskExecutorType the dispatch task executor type
* @return the number of matching dispatch triggers
*/
public static int countByC_DTET(
long companyId, String dispatchTaskExecutorType) {
return getPersistence().countByC_DTET(
companyId, dispatchTaskExecutorType);
}
/**
* Returns the number of dispatch triggers that the user has permission to view where companyId = ? and dispatchTaskExecutorType = ?.
*
* @param companyId the company ID
* @param dispatchTaskExecutorType the dispatch task executor type
* @return the number of matching dispatch triggers that the user has permission to view
*/
public static int filterCountByC_DTET(
long companyId, String dispatchTaskExecutorType) {
return getPersistence().filterCountByC_DTET(
companyId, dispatchTaskExecutorType);
}
/**
* Returns the dispatch trigger where companyId = ? and name = ? or throws a NoSuchTriggerException
if it could not be found.
*
* @param companyId the company ID
* @param name the name
* @return the matching dispatch trigger
* @throws NoSuchTriggerException if a matching dispatch trigger could not be found
*/
public static DispatchTrigger findByC_N(long companyId, String name)
throws com.liferay.dispatch.exception.NoSuchTriggerException {
return getPersistence().findByC_N(companyId, name);
}
/**
* Returns the dispatch trigger where companyId = ? and name = ? or returns null
if it could not be found. Uses the finder cache.
*
* @param companyId the company ID
* @param name the name
* @return the matching dispatch trigger, or null
if a matching dispatch trigger could not be found
*/
public static DispatchTrigger fetchByC_N(long companyId, String name) {
return getPersistence().fetchByC_N(companyId, name);
}
/**
* Returns the dispatch trigger where companyId = ? and name = ? or returns null
if it could not be found, optionally using the finder cache.
*
* @param companyId the company ID
* @param name the name
* @param useFinderCache whether to use the finder cache
* @return the matching dispatch trigger, or null
if a matching dispatch trigger could not be found
*/
public static DispatchTrigger fetchByC_N(
long companyId, String name, boolean useFinderCache) {
return getPersistence().fetchByC_N(companyId, name, useFinderCache);
}
/**
* Removes the dispatch trigger where companyId = ? and name = ? from the database.
*
* @param companyId the company ID
* @param name the name
* @return the dispatch trigger that was removed
*/
public static DispatchTrigger removeByC_N(long companyId, String name)
throws com.liferay.dispatch.exception.NoSuchTriggerException {
return getPersistence().removeByC_N(companyId, name);
}
/**
* Returns the number of dispatch triggers where companyId = ? and name = ?.
*
* @param companyId the company ID
* @param name the name
* @return the number of matching dispatch triggers
*/
public static int countByC_N(long companyId, String name) {
return getPersistence().countByC_N(companyId, name);
}
/**
* Returns all the dispatch triggers where active = ? and dispatchTaskClusterMode = ?.
*
* @param active the active
* @param dispatchTaskClusterMode the dispatch task cluster mode
* @return the matching dispatch triggers
*/
public static List findByA_DTCM(
boolean active, int dispatchTaskClusterMode) {
return getPersistence().findByA_DTCM(active, dispatchTaskClusterMode);
}
/**
* Returns a range of all the dispatch triggers where active = ? and dispatchTaskClusterMode = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from DispatchTriggerModelImpl
.
*
*
* @param active the active
* @param dispatchTaskClusterMode the dispatch task cluster mode
* @param start the lower bound of the range of dispatch triggers
* @param end the upper bound of the range of dispatch triggers (not inclusive)
* @return the range of matching dispatch triggers
*/
public static List findByA_DTCM(
boolean active, int dispatchTaskClusterMode, int start, int end) {
return getPersistence().findByA_DTCM(
active, dispatchTaskClusterMode, start, end);
}
/**
* Returns an ordered range of all the dispatch triggers where active = ? and dispatchTaskClusterMode = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from DispatchTriggerModelImpl
.
*
*
* @param active the active
* @param dispatchTaskClusterMode the dispatch task cluster mode
* @param start the lower bound of the range of dispatch triggers
* @param end the upper bound of the range of dispatch triggers (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching dispatch triggers
*/
public static List findByA_DTCM(
boolean active, int dispatchTaskClusterMode, int start, int end,
OrderByComparator orderByComparator) {
return getPersistence().findByA_DTCM(
active, dispatchTaskClusterMode, start, end, orderByComparator);
}
/**
* Returns an ordered range of all the dispatch triggers where active = ? and dispatchTaskClusterMode = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from DispatchTriggerModelImpl
.
*
*
* @param active the active
* @param dispatchTaskClusterMode the dispatch task cluster mode
* @param start the lower bound of the range of dispatch triggers
* @param end the upper bound of the range of dispatch triggers (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching dispatch triggers
*/
public static List findByA_DTCM(
boolean active, int dispatchTaskClusterMode, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
return getPersistence().findByA_DTCM(
active, dispatchTaskClusterMode, start, end, orderByComparator,
useFinderCache);
}
/**
* Returns the first dispatch trigger in the ordered set where active = ? and dispatchTaskClusterMode = ?.
*
* @param active the active
* @param dispatchTaskClusterMode the dispatch task cluster mode
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching dispatch trigger
* @throws NoSuchTriggerException if a matching dispatch trigger could not be found
*/
public static DispatchTrigger findByA_DTCM_First(
boolean active, int dispatchTaskClusterMode,
OrderByComparator orderByComparator)
throws com.liferay.dispatch.exception.NoSuchTriggerException {
return getPersistence().findByA_DTCM_First(
active, dispatchTaskClusterMode, orderByComparator);
}
/**
* Returns the first dispatch trigger in the ordered set where active = ? and dispatchTaskClusterMode = ?.
*
* @param active the active
* @param dispatchTaskClusterMode the dispatch task cluster mode
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching dispatch trigger, or null
if a matching dispatch trigger could not be found
*/
public static DispatchTrigger fetchByA_DTCM_First(
boolean active, int dispatchTaskClusterMode,
OrderByComparator orderByComparator) {
return getPersistence().fetchByA_DTCM_First(
active, dispatchTaskClusterMode, orderByComparator);
}
/**
* Returns the last dispatch trigger in the ordered set where active = ? and dispatchTaskClusterMode = ?.
*
* @param active the active
* @param dispatchTaskClusterMode the dispatch task cluster mode
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching dispatch trigger
* @throws NoSuchTriggerException if a matching dispatch trigger could not be found
*/
public static DispatchTrigger findByA_DTCM_Last(
boolean active, int dispatchTaskClusterMode,
OrderByComparator orderByComparator)
throws com.liferay.dispatch.exception.NoSuchTriggerException {
return getPersistence().findByA_DTCM_Last(
active, dispatchTaskClusterMode, orderByComparator);
}
/**
* Returns the last dispatch trigger in the ordered set where active = ? and dispatchTaskClusterMode = ?.
*
* @param active the active
* @param dispatchTaskClusterMode the dispatch task cluster mode
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching dispatch trigger, or null
if a matching dispatch trigger could not be found
*/
public static DispatchTrigger fetchByA_DTCM_Last(
boolean active, int dispatchTaskClusterMode,
OrderByComparator orderByComparator) {
return getPersistence().fetchByA_DTCM_Last(
active, dispatchTaskClusterMode, orderByComparator);
}
/**
* Returns the dispatch triggers before and after the current dispatch trigger in the ordered set where active = ? and dispatchTaskClusterMode = ?.
*
* @param dispatchTriggerId the primary key of the current dispatch trigger
* @param active the active
* @param dispatchTaskClusterMode the dispatch task cluster mode
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next dispatch trigger
* @throws NoSuchTriggerException if a dispatch trigger with the primary key could not be found
*/
public static DispatchTrigger[] findByA_DTCM_PrevAndNext(
long dispatchTriggerId, boolean active, int dispatchTaskClusterMode,
OrderByComparator orderByComparator)
throws com.liferay.dispatch.exception.NoSuchTriggerException {
return getPersistence().findByA_DTCM_PrevAndNext(
dispatchTriggerId, active, dispatchTaskClusterMode,
orderByComparator);
}
/**
* Returns all the dispatch triggers that the user has permission to view where active = ? and dispatchTaskClusterMode = ?.
*
* @param active the active
* @param dispatchTaskClusterMode the dispatch task cluster mode
* @return the matching dispatch triggers that the user has permission to view
*/
public static List filterFindByA_DTCM(
boolean active, int dispatchTaskClusterMode) {
return getPersistence().filterFindByA_DTCM(
active, dispatchTaskClusterMode);
}
/**
* Returns a range of all the dispatch triggers that the user has permission to view where active = ? and dispatchTaskClusterMode = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from DispatchTriggerModelImpl
.
*
*
* @param active the active
* @param dispatchTaskClusterMode the dispatch task cluster mode
* @param start the lower bound of the range of dispatch triggers
* @param end the upper bound of the range of dispatch triggers (not inclusive)
* @return the range of matching dispatch triggers that the user has permission to view
*/
public static List filterFindByA_DTCM(
boolean active, int dispatchTaskClusterMode, int start, int end) {
return getPersistence().filterFindByA_DTCM(
active, dispatchTaskClusterMode, start, end);
}
/**
* Returns an ordered range of all the dispatch triggers that the user has permissions to view where active = ? and dispatchTaskClusterMode = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from DispatchTriggerModelImpl
.
*
*
* @param active the active
* @param dispatchTaskClusterMode the dispatch task cluster mode
* @param start the lower bound of the range of dispatch triggers
* @param end the upper bound of the range of dispatch triggers (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching dispatch triggers that the user has permission to view
*/
public static List filterFindByA_DTCM(
boolean active, int dispatchTaskClusterMode, int start, int end,
OrderByComparator orderByComparator) {
return getPersistence().filterFindByA_DTCM(
active, dispatchTaskClusterMode, start, end, orderByComparator);
}
/**
* Returns the dispatch triggers before and after the current dispatch trigger in the ordered set of dispatch triggers that the user has permission to view where active = ? and dispatchTaskClusterMode = ?.
*
* @param dispatchTriggerId the primary key of the current dispatch trigger
* @param active the active
* @param dispatchTaskClusterMode the dispatch task cluster mode
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next dispatch trigger
* @throws NoSuchTriggerException if a dispatch trigger with the primary key could not be found
*/
public static DispatchTrigger[] filterFindByA_DTCM_PrevAndNext(
long dispatchTriggerId, boolean active, int dispatchTaskClusterMode,
OrderByComparator orderByComparator)
throws com.liferay.dispatch.exception.NoSuchTriggerException {
return getPersistence().filterFindByA_DTCM_PrevAndNext(
dispatchTriggerId, active, dispatchTaskClusterMode,
orderByComparator);
}
/**
* Returns all the dispatch triggers that the user has permission to view where active = ? and dispatchTaskClusterMode = any ?.
*
* @param active the active
* @param dispatchTaskClusterModes the dispatch task cluster modes
* @return the matching dispatch triggers that the user has permission to view
*/
public static List filterFindByA_DTCM(
boolean active, int[] dispatchTaskClusterModes) {
return getPersistence().filterFindByA_DTCM(
active, dispatchTaskClusterModes);
}
/**
* Returns a range of all the dispatch triggers that the user has permission to view where active = ? and dispatchTaskClusterMode = any ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from DispatchTriggerModelImpl
.
*
*
* @param active the active
* @param dispatchTaskClusterModes the dispatch task cluster modes
* @param start the lower bound of the range of dispatch triggers
* @param end the upper bound of the range of dispatch triggers (not inclusive)
* @return the range of matching dispatch triggers that the user has permission to view
*/
public static List filterFindByA_DTCM(
boolean active, int[] dispatchTaskClusterModes, int start, int end) {
return getPersistence().filterFindByA_DTCM(
active, dispatchTaskClusterModes, start, end);
}
/**
* Returns an ordered range of all the dispatch triggers that the user has permission to view where active = ? and dispatchTaskClusterMode = any ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from DispatchTriggerModelImpl
.
*
*
* @param active the active
* @param dispatchTaskClusterModes the dispatch task cluster modes
* @param start the lower bound of the range of dispatch triggers
* @param end the upper bound of the range of dispatch triggers (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching dispatch triggers that the user has permission to view
*/
public static List filterFindByA_DTCM(
boolean active, int[] dispatchTaskClusterModes, int start, int end,
OrderByComparator orderByComparator) {
return getPersistence().filterFindByA_DTCM(
active, dispatchTaskClusterModes, start, end, orderByComparator);
}
/**
* Returns all the dispatch triggers where active = ? and dispatchTaskClusterMode = any ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from DispatchTriggerModelImpl
.
*
*
* @param active the active
* @param dispatchTaskClusterModes the dispatch task cluster modes
* @return the matching dispatch triggers
*/
public static List findByA_DTCM(
boolean active, int[] dispatchTaskClusterModes) {
return getPersistence().findByA_DTCM(active, dispatchTaskClusterModes);
}
/**
* Returns a range of all the dispatch triggers where active = ? and dispatchTaskClusterMode = any ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from DispatchTriggerModelImpl
.
*
*
* @param active the active
* @param dispatchTaskClusterModes the dispatch task cluster modes
* @param start the lower bound of the range of dispatch triggers
* @param end the upper bound of the range of dispatch triggers (not inclusive)
* @return the range of matching dispatch triggers
*/
public static List findByA_DTCM(
boolean active, int[] dispatchTaskClusterModes, int start, int end) {
return getPersistence().findByA_DTCM(
active, dispatchTaskClusterModes, start, end);
}
/**
* Returns an ordered range of all the dispatch triggers where active = ? and dispatchTaskClusterMode = any ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from DispatchTriggerModelImpl
.
*
*
* @param active the active
* @param dispatchTaskClusterModes the dispatch task cluster modes
* @param start the lower bound of the range of dispatch triggers
* @param end the upper bound of the range of dispatch triggers (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching dispatch triggers
*/
public static List findByA_DTCM(
boolean active, int[] dispatchTaskClusterModes, int start, int end,
OrderByComparator orderByComparator) {
return getPersistence().findByA_DTCM(
active, dispatchTaskClusterModes, start, end, orderByComparator);
}
/**
* Returns an ordered range of all the dispatch triggers where active = ? and dispatchTaskClusterMode = ?, optionally using the finder cache.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from DispatchTriggerModelImpl
.
*
*
* @param active the active
* @param dispatchTaskClusterModes the dispatch task cluster modes
* @param start the lower bound of the range of dispatch triggers
* @param end the upper bound of the range of dispatch triggers (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching dispatch triggers
*/
public static List findByA_DTCM(
boolean active, int[] dispatchTaskClusterModes, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
return getPersistence().findByA_DTCM(
active, dispatchTaskClusterModes, start, end, orderByComparator,
useFinderCache);
}
/**
* Removes all the dispatch triggers where active = ? and dispatchTaskClusterMode = ? from the database.
*
* @param active the active
* @param dispatchTaskClusterMode the dispatch task cluster mode
*/
public static void removeByA_DTCM(
boolean active, int dispatchTaskClusterMode) {
getPersistence().removeByA_DTCM(active, dispatchTaskClusterMode);
}
/**
* Returns the number of dispatch triggers where active = ? and dispatchTaskClusterMode = ?.
*
* @param active the active
* @param dispatchTaskClusterMode the dispatch task cluster mode
* @return the number of matching dispatch triggers
*/
public static int countByA_DTCM(
boolean active, int dispatchTaskClusterMode) {
return getPersistence().countByA_DTCM(active, dispatchTaskClusterMode);
}
/**
* Returns the number of dispatch triggers where active = ? and dispatchTaskClusterMode = any ?.
*
* @param active the active
* @param dispatchTaskClusterModes the dispatch task cluster modes
* @return the number of matching dispatch triggers
*/
public static int countByA_DTCM(
boolean active, int[] dispatchTaskClusterModes) {
return getPersistence().countByA_DTCM(active, dispatchTaskClusterModes);
}
/**
* Returns the number of dispatch triggers that the user has permission to view where active = ? and dispatchTaskClusterMode = ?.
*
* @param active the active
* @param dispatchTaskClusterMode the dispatch task cluster mode
* @return the number of matching dispatch triggers that the user has permission to view
*/
public static int filterCountByA_DTCM(
boolean active, int dispatchTaskClusterMode) {
return getPersistence().filterCountByA_DTCM(
active, dispatchTaskClusterMode);
}
/**
* Returns the number of dispatch triggers that the user has permission to view where active = ? and dispatchTaskClusterMode = any ?.
*
* @param active the active
* @param dispatchTaskClusterModes the dispatch task cluster modes
* @return the number of matching dispatch triggers that the user has permission to view
*/
public static int filterCountByA_DTCM(
boolean active, int[] dispatchTaskClusterModes) {
return getPersistence().filterCountByA_DTCM(
active, dispatchTaskClusterModes);
}
/**
* Returns the dispatch trigger where externalReferenceCode = ? and companyId = ? or throws a NoSuchTriggerException
if it could not be found.
*
* @param externalReferenceCode the external reference code
* @param companyId the company ID
* @return the matching dispatch trigger
* @throws NoSuchTriggerException if a matching dispatch trigger could not be found
*/
public static DispatchTrigger findByERC_C(
String externalReferenceCode, long companyId)
throws com.liferay.dispatch.exception.NoSuchTriggerException {
return getPersistence().findByERC_C(externalReferenceCode, companyId);
}
/**
* Returns the dispatch trigger where externalReferenceCode = ? and companyId = ? or returns null
if it could not be found. Uses the finder cache.
*
* @param externalReferenceCode the external reference code
* @param companyId the company ID
* @return the matching dispatch trigger, or null
if a matching dispatch trigger could not be found
*/
public static DispatchTrigger fetchByERC_C(
String externalReferenceCode, long companyId) {
return getPersistence().fetchByERC_C(externalReferenceCode, companyId);
}
/**
* Returns the dispatch trigger where externalReferenceCode = ? and companyId = ? or returns null
if it could not be found, optionally using the finder cache.
*
* @param externalReferenceCode the external reference code
* @param companyId the company ID
* @param useFinderCache whether to use the finder cache
* @return the matching dispatch trigger, or null
if a matching dispatch trigger could not be found
*/
public static DispatchTrigger fetchByERC_C(
String externalReferenceCode, long companyId, boolean useFinderCache) {
return getPersistence().fetchByERC_C(
externalReferenceCode, companyId, useFinderCache);
}
/**
* Removes the dispatch trigger where externalReferenceCode = ? and companyId = ? from the database.
*
* @param externalReferenceCode the external reference code
* @param companyId the company ID
* @return the dispatch trigger that was removed
*/
public static DispatchTrigger removeByERC_C(
String externalReferenceCode, long companyId)
throws com.liferay.dispatch.exception.NoSuchTriggerException {
return getPersistence().removeByERC_C(externalReferenceCode, companyId);
}
/**
* Returns the number of dispatch triggers where externalReferenceCode = ? and companyId = ?.
*
* @param externalReferenceCode the external reference code
* @param companyId the company ID
* @return the number of matching dispatch triggers
*/
public static int countByERC_C(
String externalReferenceCode, long companyId) {
return getPersistence().countByERC_C(externalReferenceCode, companyId);
}
/**
* Caches the dispatch trigger in the entity cache if it is enabled.
*
* @param dispatchTrigger the dispatch trigger
*/
public static void cacheResult(DispatchTrigger dispatchTrigger) {
getPersistence().cacheResult(dispatchTrigger);
}
/**
* Caches the dispatch triggers in the entity cache if it is enabled.
*
* @param dispatchTriggers the dispatch triggers
*/
public static void cacheResult(List dispatchTriggers) {
getPersistence().cacheResult(dispatchTriggers);
}
/**
* Creates a new dispatch trigger with the primary key. Does not add the dispatch trigger to the database.
*
* @param dispatchTriggerId the primary key for the new dispatch trigger
* @return the new dispatch trigger
*/
public static DispatchTrigger create(long dispatchTriggerId) {
return getPersistence().create(dispatchTriggerId);
}
/**
* Removes the dispatch trigger with the primary key from the database. Also notifies the appropriate model listeners.
*
* @param dispatchTriggerId the primary key of the dispatch trigger
* @return the dispatch trigger that was removed
* @throws NoSuchTriggerException if a dispatch trigger with the primary key could not be found
*/
public static DispatchTrigger remove(long dispatchTriggerId)
throws com.liferay.dispatch.exception.NoSuchTriggerException {
return getPersistence().remove(dispatchTriggerId);
}
public static DispatchTrigger updateImpl(DispatchTrigger dispatchTrigger) {
return getPersistence().updateImpl(dispatchTrigger);
}
/**
* Returns the dispatch trigger with the primary key or throws a NoSuchTriggerException
if it could not be found.
*
* @param dispatchTriggerId the primary key of the dispatch trigger
* @return the dispatch trigger
* @throws NoSuchTriggerException if a dispatch trigger with the primary key could not be found
*/
public static DispatchTrigger findByPrimaryKey(long dispatchTriggerId)
throws com.liferay.dispatch.exception.NoSuchTriggerException {
return getPersistence().findByPrimaryKey(dispatchTriggerId);
}
/**
* Returns the dispatch trigger with the primary key or returns null
if it could not be found.
*
* @param dispatchTriggerId the primary key of the dispatch trigger
* @return the dispatch trigger, or null
if a dispatch trigger with the primary key could not be found
*/
public static DispatchTrigger fetchByPrimaryKey(long dispatchTriggerId) {
return getPersistence().fetchByPrimaryKey(dispatchTriggerId);
}
/**
* Returns all the dispatch triggers.
*
* @return the dispatch triggers
*/
public static List findAll() {
return getPersistence().findAll();
}
/**
* Returns a range of all the dispatch triggers.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from DispatchTriggerModelImpl
.
*
*
* @param start the lower bound of the range of dispatch triggers
* @param end the upper bound of the range of dispatch triggers (not inclusive)
* @return the range of dispatch triggers
*/
public static List findAll(int start, int end) {
return getPersistence().findAll(start, end);
}
/**
* Returns an ordered range of all the dispatch triggers.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from DispatchTriggerModelImpl
.
*
*
* @param start the lower bound of the range of dispatch triggers
* @param end the upper bound of the range of dispatch triggers (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of dispatch triggers
*/
public static List findAll(
int start, int end,
OrderByComparator orderByComparator) {
return getPersistence().findAll(start, end, orderByComparator);
}
/**
* Returns an ordered range of all the dispatch triggers.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from DispatchTriggerModelImpl
.
*
*
* @param start the lower bound of the range of dispatch triggers
* @param end the upper bound of the range of dispatch triggers (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of dispatch triggers
*/
public static List findAll(
int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
return getPersistence().findAll(
start, end, orderByComparator, useFinderCache);
}
/**
* Removes all the dispatch triggers from the database.
*/
public static void removeAll() {
getPersistence().removeAll();
}
/**
* Returns the number of dispatch triggers.
*
* @return the number of dispatch triggers
*/
public static int countAll() {
return getPersistence().countAll();
}
public static DispatchTriggerPersistence getPersistence() {
return _persistence;
}
public static void setPersistence(DispatchTriggerPersistence persistence) {
_persistence = persistence;
}
private static volatile DispatchTriggerPersistence _persistence;
}