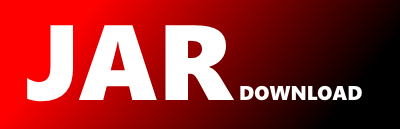
com.liferay.dynamic.data.mapping.model.impl.DDMTemplateModelImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.liferay.dynamic.data.mapping.service Show documentation
Show all versions of com.liferay.dynamic.data.mapping.service Show documentation
Liferay Dynamic Data Mapping Service
/**
* Copyright (c) 2000-present Liferay, Inc. All rights reserved.
*
* This library is free software; you can redistribute it and/or modify it under
* the terms of the GNU Lesser General Public License as published by the Free
* Software Foundation; either version 2.1 of the License, or (at your option)
* any later version.
*
* This library is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License for more
* details.
*/
package com.liferay.dynamic.data.mapping.model.impl;
import com.liferay.dynamic.data.mapping.model.DDMTemplate;
import com.liferay.dynamic.data.mapping.model.DDMTemplateModel;
import com.liferay.dynamic.data.mapping.model.DDMTemplateSoap;
import com.liferay.expando.kernel.model.ExpandoBridge;
import com.liferay.expando.kernel.util.ExpandoBridgeFactoryUtil;
import com.liferay.exportimport.kernel.lar.StagedModelType;
import com.liferay.petra.string.StringBundler;
import com.liferay.portal.kernel.bean.AutoEscapeBeanHandler;
import com.liferay.portal.kernel.exception.LocaleException;
import com.liferay.portal.kernel.exception.PortalException;
import com.liferay.portal.kernel.json.JSON;
import com.liferay.portal.kernel.model.CacheModel;
import com.liferay.portal.kernel.model.ModelWrapper;
import com.liferay.portal.kernel.model.User;
import com.liferay.portal.kernel.model.impl.BaseModelImpl;
import com.liferay.portal.kernel.service.ServiceContext;
import com.liferay.portal.kernel.service.UserLocalServiceUtil;
import com.liferay.portal.kernel.util.GetterUtil;
import com.liferay.portal.kernel.util.LocaleUtil;
import com.liferay.portal.kernel.util.LocalizationUtil;
import com.liferay.portal.kernel.util.PortalUtil;
import com.liferay.portal.kernel.util.ProxyUtil;
import com.liferay.portal.kernel.util.Validator;
import java.io.Serializable;
import java.lang.reflect.Constructor;
import java.lang.reflect.InvocationHandler;
import java.sql.Types;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Date;
import java.util.HashMap;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Locale;
import java.util.Map;
import java.util.Set;
import java.util.TreeSet;
import java.util.function.BiConsumer;
import java.util.function.Function;
/**
* The base model implementation for the DDMTemplate service. Represents a row in the "DDMTemplate" database table, with each column mapped to a property of this class.
*
*
* This implementation and its corresponding interface DDMTemplateModel
exist only as a container for the default property accessors generated by ServiceBuilder. Helper methods and all application logic should be put in {@link DDMTemplateImpl}.
*
*
* @author Brian Wing Shun Chan
* @see DDMTemplateImpl
* @generated
*/
@JSON(strict = true)
public class DDMTemplateModelImpl
extends BaseModelImpl implements DDMTemplateModel {
/*
* NOTE FOR DEVELOPERS:
*
* Never modify or reference this class directly. All methods that expect a ddm template model instance should use the DDMTemplate
interface instead.
*/
public static final String TABLE_NAME = "DDMTemplate";
public static final Object[][] TABLE_COLUMNS = {
{"mvccVersion", Types.BIGINT}, {"ctCollectionId", Types.BIGINT},
{"uuid_", Types.VARCHAR}, {"templateId", Types.BIGINT},
{"groupId", Types.BIGINT}, {"companyId", Types.BIGINT},
{"userId", Types.BIGINT}, {"userName", Types.VARCHAR},
{"versionUserId", Types.BIGINT}, {"versionUserName", Types.VARCHAR},
{"createDate", Types.TIMESTAMP}, {"modifiedDate", Types.TIMESTAMP},
{"classNameId", Types.BIGINT}, {"classPK", Types.BIGINT},
{"resourceClassNameId", Types.BIGINT}, {"templateKey", Types.VARCHAR},
{"version", Types.VARCHAR}, {"name", Types.CLOB},
{"description", Types.CLOB}, {"type_", Types.VARCHAR},
{"mode_", Types.VARCHAR}, {"language", Types.VARCHAR},
{"script", Types.CLOB}, {"cacheable", Types.BOOLEAN},
{"smallImage", Types.BOOLEAN}, {"smallImageId", Types.BIGINT},
{"smallImageURL", Types.VARCHAR}, {"lastPublishDate", Types.TIMESTAMP}
};
public static final Map TABLE_COLUMNS_MAP =
new HashMap();
static {
TABLE_COLUMNS_MAP.put("mvccVersion", Types.BIGINT);
TABLE_COLUMNS_MAP.put("ctCollectionId", Types.BIGINT);
TABLE_COLUMNS_MAP.put("uuid_", Types.VARCHAR);
TABLE_COLUMNS_MAP.put("templateId", Types.BIGINT);
TABLE_COLUMNS_MAP.put("groupId", Types.BIGINT);
TABLE_COLUMNS_MAP.put("companyId", Types.BIGINT);
TABLE_COLUMNS_MAP.put("userId", Types.BIGINT);
TABLE_COLUMNS_MAP.put("userName", Types.VARCHAR);
TABLE_COLUMNS_MAP.put("versionUserId", Types.BIGINT);
TABLE_COLUMNS_MAP.put("versionUserName", Types.VARCHAR);
TABLE_COLUMNS_MAP.put("createDate", Types.TIMESTAMP);
TABLE_COLUMNS_MAP.put("modifiedDate", Types.TIMESTAMP);
TABLE_COLUMNS_MAP.put("classNameId", Types.BIGINT);
TABLE_COLUMNS_MAP.put("classPK", Types.BIGINT);
TABLE_COLUMNS_MAP.put("resourceClassNameId", Types.BIGINT);
TABLE_COLUMNS_MAP.put("templateKey", Types.VARCHAR);
TABLE_COLUMNS_MAP.put("version", Types.VARCHAR);
TABLE_COLUMNS_MAP.put("name", Types.CLOB);
TABLE_COLUMNS_MAP.put("description", Types.CLOB);
TABLE_COLUMNS_MAP.put("type_", Types.VARCHAR);
TABLE_COLUMNS_MAP.put("mode_", Types.VARCHAR);
TABLE_COLUMNS_MAP.put("language", Types.VARCHAR);
TABLE_COLUMNS_MAP.put("script", Types.CLOB);
TABLE_COLUMNS_MAP.put("cacheable", Types.BOOLEAN);
TABLE_COLUMNS_MAP.put("smallImage", Types.BOOLEAN);
TABLE_COLUMNS_MAP.put("smallImageId", Types.BIGINT);
TABLE_COLUMNS_MAP.put("smallImageURL", Types.VARCHAR);
TABLE_COLUMNS_MAP.put("lastPublishDate", Types.TIMESTAMP);
}
public static final String TABLE_SQL_CREATE =
"create table DDMTemplate (mvccVersion LONG default 0 not null,ctCollectionId LONG default 0 not null,uuid_ VARCHAR(75) null,templateId LONG not null,groupId LONG,companyId LONG,userId LONG,userName VARCHAR(75) null,versionUserId LONG,versionUserName VARCHAR(75) null,createDate DATE null,modifiedDate DATE null,classNameId LONG,classPK LONG,resourceClassNameId LONG,templateKey VARCHAR(75) null,version VARCHAR(75) null,name TEXT null,description TEXT null,type_ VARCHAR(75) null,mode_ VARCHAR(75) null,language VARCHAR(75) null,script TEXT null,cacheable BOOLEAN,smallImage BOOLEAN,smallImageId LONG,smallImageURL STRING null,lastPublishDate DATE null,primary key (templateId, ctCollectionId))";
public static final String TABLE_SQL_DROP = "drop table DDMTemplate";
public static final String ORDER_BY_JPQL =
" ORDER BY ddmTemplate.templateId ASC";
public static final String ORDER_BY_SQL =
" ORDER BY DDMTemplate.templateId ASC";
public static final String DATA_SOURCE = "liferayDataSource";
public static final String SESSION_FACTORY = "liferaySessionFactory";
public static final String TX_MANAGER = "liferayTransactionManager";
public static final long CLASSNAMEID_COLUMN_BITMASK = 1L;
public static final long CLASSPK_COLUMN_BITMASK = 2L;
public static final long COMPANYID_COLUMN_BITMASK = 4L;
public static final long GROUPID_COLUMN_BITMASK = 8L;
public static final long LANGUAGE_COLUMN_BITMASK = 16L;
public static final long MODE_COLUMN_BITMASK = 32L;
public static final long SMALLIMAGEID_COLUMN_BITMASK = 64L;
public static final long TEMPLATEKEY_COLUMN_BITMASK = 128L;
public static final long TYPE_COLUMN_BITMASK = 256L;
public static final long UUID_COLUMN_BITMASK = 512L;
public static final long TEMPLATEID_COLUMN_BITMASK = 1024L;
public static void setEntityCacheEnabled(boolean entityCacheEnabled) {
_entityCacheEnabled = entityCacheEnabled;
}
public static void setFinderCacheEnabled(boolean finderCacheEnabled) {
_finderCacheEnabled = finderCacheEnabled;
}
/**
* Converts the soap model instance into a normal model instance.
*
* @param soapModel the soap model instance to convert
* @return the normal model instance
*/
public static DDMTemplate toModel(DDMTemplateSoap soapModel) {
if (soapModel == null) {
return null;
}
DDMTemplate model = new DDMTemplateImpl();
model.setMvccVersion(soapModel.getMvccVersion());
model.setCtCollectionId(soapModel.getCtCollectionId());
model.setUuid(soapModel.getUuid());
model.setTemplateId(soapModel.getTemplateId());
model.setGroupId(soapModel.getGroupId());
model.setCompanyId(soapModel.getCompanyId());
model.setUserId(soapModel.getUserId());
model.setUserName(soapModel.getUserName());
model.setVersionUserId(soapModel.getVersionUserId());
model.setVersionUserName(soapModel.getVersionUserName());
model.setCreateDate(soapModel.getCreateDate());
model.setModifiedDate(soapModel.getModifiedDate());
model.setClassNameId(soapModel.getClassNameId());
model.setClassPK(soapModel.getClassPK());
model.setResourceClassNameId(soapModel.getResourceClassNameId());
model.setTemplateKey(soapModel.getTemplateKey());
model.setVersion(soapModel.getVersion());
model.setName(soapModel.getName());
model.setDescription(soapModel.getDescription());
model.setType(soapModel.getType());
model.setMode(soapModel.getMode());
model.setLanguage(soapModel.getLanguage());
model.setScript(soapModel.getScript());
model.setCacheable(soapModel.isCacheable());
model.setSmallImage(soapModel.isSmallImage());
model.setSmallImageId(soapModel.getSmallImageId());
model.setSmallImageURL(soapModel.getSmallImageURL());
model.setLastPublishDate(soapModel.getLastPublishDate());
return model;
}
/**
* Converts the soap model instances into normal model instances.
*
* @param soapModels the soap model instances to convert
* @return the normal model instances
*/
public static List toModels(DDMTemplateSoap[] soapModels) {
if (soapModels == null) {
return null;
}
List models = new ArrayList(
soapModels.length);
for (DDMTemplateSoap soapModel : soapModels) {
models.add(toModel(soapModel));
}
return models;
}
public DDMTemplateModelImpl() {
}
@Override
public long getPrimaryKey() {
return _templateId;
}
@Override
public void setPrimaryKey(long primaryKey) {
setTemplateId(primaryKey);
}
@Override
public Serializable getPrimaryKeyObj() {
return _templateId;
}
@Override
public void setPrimaryKeyObj(Serializable primaryKeyObj) {
setPrimaryKey(((Long)primaryKeyObj).longValue());
}
@Override
public Class> getModelClass() {
return DDMTemplate.class;
}
@Override
public String getModelClassName() {
return DDMTemplate.class.getName();
}
@Override
public Map getModelAttributes() {
Map attributes = new HashMap();
Map> attributeGetterFunctions =
getAttributeGetterFunctions();
for (Map.Entry> entry :
attributeGetterFunctions.entrySet()) {
String attributeName = entry.getKey();
Function attributeGetterFunction =
entry.getValue();
attributes.put(
attributeName,
attributeGetterFunction.apply((DDMTemplate)this));
}
attributes.put("entityCacheEnabled", isEntityCacheEnabled());
attributes.put("finderCacheEnabled", isFinderCacheEnabled());
return attributes;
}
@Override
public void setModelAttributes(Map attributes) {
Map>
attributeSetterBiConsumers = getAttributeSetterBiConsumers();
for (Map.Entry entry : attributes.entrySet()) {
String attributeName = entry.getKey();
BiConsumer attributeSetterBiConsumer =
attributeSetterBiConsumers.get(attributeName);
if (attributeSetterBiConsumer != null) {
attributeSetterBiConsumer.accept(
(DDMTemplate)this, entry.getValue());
}
}
}
public Map>
getAttributeGetterFunctions() {
return _attributeGetterFunctions;
}
public Map>
getAttributeSetterBiConsumers() {
return _attributeSetterBiConsumers;
}
private static Function
_getProxyProviderFunction() {
Class> proxyClass = ProxyUtil.getProxyClass(
DDMTemplate.class.getClassLoader(), DDMTemplate.class,
ModelWrapper.class);
try {
Constructor constructor =
(Constructor)proxyClass.getConstructor(
InvocationHandler.class);
return invocationHandler -> {
try {
return constructor.newInstance(invocationHandler);
}
catch (ReflectiveOperationException
reflectiveOperationException) {
throw new InternalError(reflectiveOperationException);
}
};
}
catch (NoSuchMethodException noSuchMethodException) {
throw new InternalError(noSuchMethodException);
}
}
private static final Map>
_attributeGetterFunctions;
private static final Map>
_attributeSetterBiConsumers;
static {
Map> attributeGetterFunctions =
new LinkedHashMap>();
Map> attributeSetterBiConsumers =
new LinkedHashMap>();
attributeGetterFunctions.put(
"mvccVersion", DDMTemplate::getMvccVersion);
attributeSetterBiConsumers.put(
"mvccVersion",
(BiConsumer)DDMTemplate::setMvccVersion);
attributeGetterFunctions.put(
"ctCollectionId", DDMTemplate::getCtCollectionId);
attributeSetterBiConsumers.put(
"ctCollectionId",
(BiConsumer)DDMTemplate::setCtCollectionId);
attributeGetterFunctions.put("uuid", DDMTemplate::getUuid);
attributeSetterBiConsumers.put(
"uuid", (BiConsumer)DDMTemplate::setUuid);
attributeGetterFunctions.put("templateId", DDMTemplate::getTemplateId);
attributeSetterBiConsumers.put(
"templateId",
(BiConsumer)DDMTemplate::setTemplateId);
attributeGetterFunctions.put("groupId", DDMTemplate::getGroupId);
attributeSetterBiConsumers.put(
"groupId", (BiConsumer)DDMTemplate::setGroupId);
attributeGetterFunctions.put("companyId", DDMTemplate::getCompanyId);
attributeSetterBiConsumers.put(
"companyId",
(BiConsumer)DDMTemplate::setCompanyId);
attributeGetterFunctions.put("userId", DDMTemplate::getUserId);
attributeSetterBiConsumers.put(
"userId", (BiConsumer)DDMTemplate::setUserId);
attributeGetterFunctions.put("userName", DDMTemplate::getUserName);
attributeSetterBiConsumers.put(
"userName",
(BiConsumer)DDMTemplate::setUserName);
attributeGetterFunctions.put(
"versionUserId", DDMTemplate::getVersionUserId);
attributeSetterBiConsumers.put(
"versionUserId",
(BiConsumer)DDMTemplate::setVersionUserId);
attributeGetterFunctions.put(
"versionUserName", DDMTemplate::getVersionUserName);
attributeSetterBiConsumers.put(
"versionUserName",
(BiConsumer)DDMTemplate::setVersionUserName);
attributeGetterFunctions.put("createDate", DDMTemplate::getCreateDate);
attributeSetterBiConsumers.put(
"createDate",
(BiConsumer)DDMTemplate::setCreateDate);
attributeGetterFunctions.put(
"modifiedDate", DDMTemplate::getModifiedDate);
attributeSetterBiConsumers.put(
"modifiedDate",
(BiConsumer)DDMTemplate::setModifiedDate);
attributeGetterFunctions.put(
"classNameId", DDMTemplate::getClassNameId);
attributeSetterBiConsumers.put(
"classNameId",
(BiConsumer)DDMTemplate::setClassNameId);
attributeGetterFunctions.put("classPK", DDMTemplate::getClassPK);
attributeSetterBiConsumers.put(
"classPK", (BiConsumer)DDMTemplate::setClassPK);
attributeGetterFunctions.put(
"resourceClassNameId", DDMTemplate::getResourceClassNameId);
attributeSetterBiConsumers.put(
"resourceClassNameId",
(BiConsumer)DDMTemplate::setResourceClassNameId);
attributeGetterFunctions.put(
"templateKey", DDMTemplate::getTemplateKey);
attributeSetterBiConsumers.put(
"templateKey",
(BiConsumer)DDMTemplate::setTemplateKey);
attributeGetterFunctions.put("version", DDMTemplate::getVersion);
attributeSetterBiConsumers.put(
"version",
(BiConsumer)DDMTemplate::setVersion);
attributeGetterFunctions.put("name", DDMTemplate::getName);
attributeSetterBiConsumers.put(
"name", (BiConsumer)DDMTemplate::setName);
attributeGetterFunctions.put(
"description", DDMTemplate::getDescription);
attributeSetterBiConsumers.put(
"description",
(BiConsumer)DDMTemplate::setDescription);
attributeGetterFunctions.put("type", DDMTemplate::getType);
attributeSetterBiConsumers.put(
"type", (BiConsumer)DDMTemplate::setType);
attributeGetterFunctions.put("mode", DDMTemplate::getMode);
attributeSetterBiConsumers.put(
"mode", (BiConsumer)DDMTemplate::setMode);
attributeGetterFunctions.put("language", DDMTemplate::getLanguage);
attributeSetterBiConsumers.put(
"language",
(BiConsumer)DDMTemplate::setLanguage);
attributeGetterFunctions.put("script", DDMTemplate::getScript);
attributeSetterBiConsumers.put(
"script", (BiConsumer)DDMTemplate::setScript);
attributeGetterFunctions.put("cacheable", DDMTemplate::getCacheable);
attributeSetterBiConsumers.put(
"cacheable",
(BiConsumer)DDMTemplate::setCacheable);
attributeGetterFunctions.put("smallImage", DDMTemplate::getSmallImage);
attributeSetterBiConsumers.put(
"smallImage",
(BiConsumer)DDMTemplate::setSmallImage);
attributeGetterFunctions.put(
"smallImageId", DDMTemplate::getSmallImageId);
attributeSetterBiConsumers.put(
"smallImageId",
(BiConsumer)DDMTemplate::setSmallImageId);
attributeGetterFunctions.put(
"smallImageURL", DDMTemplate::getSmallImageURL);
attributeSetterBiConsumers.put(
"smallImageURL",
(BiConsumer)DDMTemplate::setSmallImageURL);
attributeGetterFunctions.put(
"lastPublishDate", DDMTemplate::getLastPublishDate);
attributeSetterBiConsumers.put(
"lastPublishDate",
(BiConsumer)DDMTemplate::setLastPublishDate);
_attributeGetterFunctions = Collections.unmodifiableMap(
attributeGetterFunctions);
_attributeSetterBiConsumers = Collections.unmodifiableMap(
(Map)attributeSetterBiConsumers);
}
@JSON
@Override
public long getMvccVersion() {
return _mvccVersion;
}
@Override
public void setMvccVersion(long mvccVersion) {
_mvccVersion = mvccVersion;
}
@JSON
@Override
public long getCtCollectionId() {
return _ctCollectionId;
}
@Override
public void setCtCollectionId(long ctCollectionId) {
_ctCollectionId = ctCollectionId;
}
@JSON
@Override
public String getUuid() {
if (_uuid == null) {
return "";
}
else {
return _uuid;
}
}
@Override
public void setUuid(String uuid) {
_columnBitmask |= UUID_COLUMN_BITMASK;
if (_originalUuid == null) {
_originalUuid = _uuid;
}
_uuid = uuid;
}
public String getOriginalUuid() {
return GetterUtil.getString(_originalUuid);
}
@JSON
@Override
public long getTemplateId() {
return _templateId;
}
@Override
public void setTemplateId(long templateId) {
_templateId = templateId;
}
@JSON
@Override
public long getGroupId() {
return _groupId;
}
@Override
public void setGroupId(long groupId) {
_columnBitmask |= GROUPID_COLUMN_BITMASK;
if (!_setOriginalGroupId) {
_setOriginalGroupId = true;
_originalGroupId = _groupId;
}
_groupId = groupId;
}
public long getOriginalGroupId() {
return _originalGroupId;
}
@JSON
@Override
public long getCompanyId() {
return _companyId;
}
@Override
public void setCompanyId(long companyId) {
_columnBitmask |= COMPANYID_COLUMN_BITMASK;
if (!_setOriginalCompanyId) {
_setOriginalCompanyId = true;
_originalCompanyId = _companyId;
}
_companyId = companyId;
}
public long getOriginalCompanyId() {
return _originalCompanyId;
}
@JSON
@Override
public long getUserId() {
return _userId;
}
@Override
public void setUserId(long userId) {
_userId = userId;
}
@Override
public String getUserUuid() {
try {
User user = UserLocalServiceUtil.getUserById(getUserId());
return user.getUuid();
}
catch (PortalException portalException) {
return "";
}
}
@Override
public void setUserUuid(String userUuid) {
}
@JSON
@Override
public String getUserName() {
if (_userName == null) {
return "";
}
else {
return _userName;
}
}
@Override
public void setUserName(String userName) {
_userName = userName;
}
@JSON
@Override
public long getVersionUserId() {
return _versionUserId;
}
@Override
public void setVersionUserId(long versionUserId) {
_versionUserId = versionUserId;
}
@Override
public String getVersionUserUuid() {
try {
User user = UserLocalServiceUtil.getUserById(getVersionUserId());
return user.getUuid();
}
catch (PortalException portalException) {
return "";
}
}
@Override
public void setVersionUserUuid(String versionUserUuid) {
}
@JSON
@Override
public String getVersionUserName() {
if (_versionUserName == null) {
return "";
}
else {
return _versionUserName;
}
}
@Override
public void setVersionUserName(String versionUserName) {
_versionUserName = versionUserName;
}
@JSON
@Override
public Date getCreateDate() {
return _createDate;
}
@Override
public void setCreateDate(Date createDate) {
_createDate = createDate;
}
@JSON
@Override
public Date getModifiedDate() {
return _modifiedDate;
}
public boolean hasSetModifiedDate() {
return _setModifiedDate;
}
@Override
public void setModifiedDate(Date modifiedDate) {
_setModifiedDate = true;
_modifiedDate = modifiedDate;
}
@Override
public String getClassName() {
if (getClassNameId() <= 0) {
return "";
}
return PortalUtil.getClassName(getClassNameId());
}
@Override
public void setClassName(String className) {
long classNameId = 0;
if (Validator.isNotNull(className)) {
classNameId = PortalUtil.getClassNameId(className);
}
setClassNameId(classNameId);
}
@JSON
@Override
public long getClassNameId() {
return _classNameId;
}
@Override
public void setClassNameId(long classNameId) {
_columnBitmask |= CLASSNAMEID_COLUMN_BITMASK;
if (!_setOriginalClassNameId) {
_setOriginalClassNameId = true;
_originalClassNameId = _classNameId;
}
_classNameId = classNameId;
}
public long getOriginalClassNameId() {
return _originalClassNameId;
}
@JSON
@Override
public long getClassPK() {
return _classPK;
}
@Override
public void setClassPK(long classPK) {
_columnBitmask |= CLASSPK_COLUMN_BITMASK;
if (!_setOriginalClassPK) {
_setOriginalClassPK = true;
_originalClassPK = _classPK;
}
_classPK = classPK;
}
public long getOriginalClassPK() {
return _originalClassPK;
}
@JSON
@Override
public long getResourceClassNameId() {
return _resourceClassNameId;
}
@Override
public void setResourceClassNameId(long resourceClassNameId) {
_resourceClassNameId = resourceClassNameId;
}
@JSON
@Override
public String getTemplateKey() {
if (_templateKey == null) {
return "";
}
else {
return _templateKey;
}
}
@Override
public void setTemplateKey(String templateKey) {
_columnBitmask |= TEMPLATEKEY_COLUMN_BITMASK;
if (_originalTemplateKey == null) {
_originalTemplateKey = _templateKey;
}
_templateKey = templateKey;
}
public String getOriginalTemplateKey() {
return GetterUtil.getString(_originalTemplateKey);
}
@JSON
@Override
public String getVersion() {
if (_version == null) {
return "";
}
else {
return _version;
}
}
@Override
public void setVersion(String version) {
_version = version;
}
@JSON
@Override
public String getName() {
if (_name == null) {
return "";
}
else {
return _name;
}
}
@Override
public String getName(Locale locale) {
String languageId = LocaleUtil.toLanguageId(locale);
return getName(languageId);
}
@Override
public String getName(Locale locale, boolean useDefault) {
String languageId = LocaleUtil.toLanguageId(locale);
return getName(languageId, useDefault);
}
@Override
public String getName(String languageId) {
return LocalizationUtil.getLocalization(getName(), languageId);
}
@Override
public String getName(String languageId, boolean useDefault) {
return LocalizationUtil.getLocalization(
getName(), languageId, useDefault);
}
@Override
public String getNameCurrentLanguageId() {
return _nameCurrentLanguageId;
}
@JSON
@Override
public String getNameCurrentValue() {
Locale locale = getLocale(_nameCurrentLanguageId);
return getName(locale);
}
@Override
public Map getNameMap() {
return LocalizationUtil.getLocalizationMap(getName());
}
@Override
public void setName(String name) {
_name = name;
}
@Override
public void setName(String name, Locale locale) {
setName(name, locale, LocaleUtil.getSiteDefault());
}
@Override
public void setName(String name, Locale locale, Locale defaultLocale) {
String languageId = LocaleUtil.toLanguageId(locale);
String defaultLanguageId = LocaleUtil.toLanguageId(defaultLocale);
if (Validator.isNotNull(name)) {
setName(
LocalizationUtil.updateLocalization(
getName(), "Name", name, languageId, defaultLanguageId));
}
else {
setName(
LocalizationUtil.removeLocalization(
getName(), "Name", languageId));
}
}
@Override
public void setNameCurrentLanguageId(String languageId) {
_nameCurrentLanguageId = languageId;
}
@Override
public void setNameMap(Map nameMap) {
setNameMap(nameMap, LocaleUtil.getSiteDefault());
}
@Override
public void setNameMap(Map nameMap, Locale defaultLocale) {
if (nameMap == null) {
return;
}
setName(
LocalizationUtil.updateLocalization(
nameMap, getName(), "Name",
LocaleUtil.toLanguageId(defaultLocale)));
}
@JSON
@Override
public String getDescription() {
if (_description == null) {
return "";
}
else {
return _description;
}
}
@Override
public String getDescription(Locale locale) {
String languageId = LocaleUtil.toLanguageId(locale);
return getDescription(languageId);
}
@Override
public String getDescription(Locale locale, boolean useDefault) {
String languageId = LocaleUtil.toLanguageId(locale);
return getDescription(languageId, useDefault);
}
@Override
public String getDescription(String languageId) {
return LocalizationUtil.getLocalization(getDescription(), languageId);
}
@Override
public String getDescription(String languageId, boolean useDefault) {
return LocalizationUtil.getLocalization(
getDescription(), languageId, useDefault);
}
@Override
public String getDescriptionCurrentLanguageId() {
return _descriptionCurrentLanguageId;
}
@JSON
@Override
public String getDescriptionCurrentValue() {
Locale locale = getLocale(_descriptionCurrentLanguageId);
return getDescription(locale);
}
@Override
public Map getDescriptionMap() {
return LocalizationUtil.getLocalizationMap(getDescription());
}
@Override
public void setDescription(String description) {
_description = description;
}
@Override
public void setDescription(String description, Locale locale) {
setDescription(description, locale, LocaleUtil.getSiteDefault());
}
@Override
public void setDescription(
String description, Locale locale, Locale defaultLocale) {
String languageId = LocaleUtil.toLanguageId(locale);
String defaultLanguageId = LocaleUtil.toLanguageId(defaultLocale);
if (Validator.isNotNull(description)) {
setDescription(
LocalizationUtil.updateLocalization(
getDescription(), "Description", description, languageId,
defaultLanguageId));
}
else {
setDescription(
LocalizationUtil.removeLocalization(
getDescription(), "Description", languageId));
}
}
@Override
public void setDescriptionCurrentLanguageId(String languageId) {
_descriptionCurrentLanguageId = languageId;
}
@Override
public void setDescriptionMap(Map descriptionMap) {
setDescriptionMap(descriptionMap, LocaleUtil.getSiteDefault());
}
@Override
public void setDescriptionMap(
Map descriptionMap, Locale defaultLocale) {
if (descriptionMap == null) {
return;
}
setDescription(
LocalizationUtil.updateLocalization(
descriptionMap, getDescription(), "Description",
LocaleUtil.toLanguageId(defaultLocale)));
}
@JSON
@Override
public String getType() {
if (_type == null) {
return "";
}
else {
return _type;
}
}
@Override
public void setType(String type) {
_columnBitmask |= TYPE_COLUMN_BITMASK;
if (_originalType == null) {
_originalType = _type;
}
_type = type;
}
public String getOriginalType() {
return GetterUtil.getString(_originalType);
}
@JSON
@Override
public String getMode() {
if (_mode == null) {
return "";
}
else {
return _mode;
}
}
@Override
public void setMode(String mode) {
_columnBitmask |= MODE_COLUMN_BITMASK;
if (_originalMode == null) {
_originalMode = _mode;
}
_mode = mode;
}
public String getOriginalMode() {
return GetterUtil.getString(_originalMode);
}
@JSON
@Override
public String getLanguage() {
if (_language == null) {
return "";
}
else {
return _language;
}
}
@Override
public void setLanguage(String language) {
_columnBitmask |= LANGUAGE_COLUMN_BITMASK;
if (_originalLanguage == null) {
_originalLanguage = _language;
}
_language = language;
}
public String getOriginalLanguage() {
return GetterUtil.getString(_originalLanguage);
}
@JSON
@Override
public String getScript() {
if (_script == null) {
return "";
}
else {
return _script;
}
}
@Override
public void setScript(String script) {
_script = script;
}
@JSON
@Override
public boolean getCacheable() {
return _cacheable;
}
@JSON
@Override
public boolean isCacheable() {
return _cacheable;
}
@Override
public void setCacheable(boolean cacheable) {
_cacheable = cacheable;
}
@JSON
@Override
public boolean getSmallImage() {
return _smallImage;
}
@JSON
@Override
public boolean isSmallImage() {
return _smallImage;
}
@Override
public void setSmallImage(boolean smallImage) {
_smallImage = smallImage;
}
@JSON
@Override
public long getSmallImageId() {
return _smallImageId;
}
@Override
public void setSmallImageId(long smallImageId) {
_columnBitmask |= SMALLIMAGEID_COLUMN_BITMASK;
if (!_setOriginalSmallImageId) {
_setOriginalSmallImageId = true;
_originalSmallImageId = _smallImageId;
}
_smallImageId = smallImageId;
}
public long getOriginalSmallImageId() {
return _originalSmallImageId;
}
@JSON
@Override
public String getSmallImageURL() {
if (_smallImageURL == null) {
return "";
}
else {
return _smallImageURL;
}
}
@Override
public void setSmallImageURL(String smallImageURL) {
_smallImageURL = smallImageURL;
}
@JSON
@Override
public Date getLastPublishDate() {
return _lastPublishDate;
}
@Override
public void setLastPublishDate(Date lastPublishDate) {
_lastPublishDate = lastPublishDate;
}
public String getResourceClassName() {
return null;
}
public void setResourceClassName(String resourceClassName) {
}
@Override
public StagedModelType getStagedModelType() {
return new StagedModelType(
PortalUtil.getClassNameId(DDMTemplate.class.getName()),
getClassNameId());
}
public long getColumnBitmask() {
return _columnBitmask;
}
@Override
public ExpandoBridge getExpandoBridge() {
return ExpandoBridgeFactoryUtil.getExpandoBridge(
getCompanyId(), DDMTemplate.class.getName(), getPrimaryKey());
}
@Override
public void setExpandoBridgeAttributes(ServiceContext serviceContext) {
ExpandoBridge expandoBridge = getExpandoBridge();
expandoBridge.setAttributes(serviceContext);
}
@Override
public String[] getAvailableLanguageIds() {
Set availableLanguageIds = new TreeSet();
Map nameMap = getNameMap();
for (Map.Entry entry : nameMap.entrySet()) {
Locale locale = entry.getKey();
String value = entry.getValue();
if (Validator.isNotNull(value)) {
availableLanguageIds.add(LocaleUtil.toLanguageId(locale));
}
}
Map descriptionMap = getDescriptionMap();
for (Map.Entry entry : descriptionMap.entrySet()) {
Locale locale = entry.getKey();
String value = entry.getValue();
if (Validator.isNotNull(value)) {
availableLanguageIds.add(LocaleUtil.toLanguageId(locale));
}
}
return availableLanguageIds.toArray(
new String[availableLanguageIds.size()]);
}
@Override
public String getDefaultLanguageId() {
String xml = getName();
if (xml == null) {
return "";
}
Locale defaultLocale = LocaleUtil.getSiteDefault();
return LocalizationUtil.getDefaultLanguageId(xml, defaultLocale);
}
@Override
public void prepareLocalizedFieldsForImport() throws LocaleException {
Locale defaultLocale = LocaleUtil.fromLanguageId(
getDefaultLanguageId());
Locale[] availableLocales = LocaleUtil.fromLanguageIds(
getAvailableLanguageIds());
Locale defaultImportLocale = LocalizationUtil.getDefaultImportLocale(
DDMTemplate.class.getName(), getPrimaryKey(), defaultLocale,
availableLocales);
prepareLocalizedFieldsForImport(defaultImportLocale);
}
@Override
@SuppressWarnings("unused")
public void prepareLocalizedFieldsForImport(Locale defaultImportLocale)
throws LocaleException {
Locale defaultLocale = LocaleUtil.getSiteDefault();
String modelDefaultLanguageId = getDefaultLanguageId();
String name = getName(defaultLocale);
if (Validator.isNull(name)) {
setName(getName(modelDefaultLanguageId), defaultLocale);
}
else {
setName(getName(defaultLocale), defaultLocale, defaultLocale);
}
String description = getDescription(defaultLocale);
if (Validator.isNull(description)) {
setDescription(
getDescription(modelDefaultLanguageId), defaultLocale);
}
else {
setDescription(
getDescription(defaultLocale), defaultLocale, defaultLocale);
}
}
@Override
public DDMTemplate toEscapedModel() {
if (_escapedModel == null) {
Function
escapedModelProxyProviderFunction =
EscapedModelProxyProviderFunctionHolder.
_escapedModelProxyProviderFunction;
_escapedModel = escapedModelProxyProviderFunction.apply(
new AutoEscapeBeanHandler(this));
}
return _escapedModel;
}
@Override
public Object clone() {
DDMTemplateImpl ddmTemplateImpl = new DDMTemplateImpl();
ddmTemplateImpl.setMvccVersion(getMvccVersion());
ddmTemplateImpl.setCtCollectionId(getCtCollectionId());
ddmTemplateImpl.setUuid(getUuid());
ddmTemplateImpl.setTemplateId(getTemplateId());
ddmTemplateImpl.setGroupId(getGroupId());
ddmTemplateImpl.setCompanyId(getCompanyId());
ddmTemplateImpl.setUserId(getUserId());
ddmTemplateImpl.setUserName(getUserName());
ddmTemplateImpl.setVersionUserId(getVersionUserId());
ddmTemplateImpl.setVersionUserName(getVersionUserName());
ddmTemplateImpl.setCreateDate(getCreateDate());
ddmTemplateImpl.setModifiedDate(getModifiedDate());
ddmTemplateImpl.setClassNameId(getClassNameId());
ddmTemplateImpl.setClassPK(getClassPK());
ddmTemplateImpl.setResourceClassNameId(getResourceClassNameId());
ddmTemplateImpl.setTemplateKey(getTemplateKey());
ddmTemplateImpl.setVersion(getVersion());
ddmTemplateImpl.setName(getName());
ddmTemplateImpl.setDescription(getDescription());
ddmTemplateImpl.setType(getType());
ddmTemplateImpl.setMode(getMode());
ddmTemplateImpl.setLanguage(getLanguage());
ddmTemplateImpl.setScript(getScript());
ddmTemplateImpl.setCacheable(isCacheable());
ddmTemplateImpl.setSmallImage(isSmallImage());
ddmTemplateImpl.setSmallImageId(getSmallImageId());
ddmTemplateImpl.setSmallImageURL(getSmallImageURL());
ddmTemplateImpl.setLastPublishDate(getLastPublishDate());
ddmTemplateImpl.resetOriginalValues();
return ddmTemplateImpl;
}
@Override
public int compareTo(DDMTemplate ddmTemplate) {
long primaryKey = ddmTemplate.getPrimaryKey();
if (getPrimaryKey() < primaryKey) {
return -1;
}
else if (getPrimaryKey() > primaryKey) {
return 1;
}
else {
return 0;
}
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (!(obj instanceof DDMTemplate)) {
return false;
}
DDMTemplate ddmTemplate = (DDMTemplate)obj;
long primaryKey = ddmTemplate.getPrimaryKey();
if (getPrimaryKey() == primaryKey) {
return true;
}
else {
return false;
}
}
@Override
public int hashCode() {
return (int)getPrimaryKey();
}
@Override
public boolean isEntityCacheEnabled() {
return _entityCacheEnabled;
}
@Override
public boolean isFinderCacheEnabled() {
return _finderCacheEnabled;
}
@Override
public void resetOriginalValues() {
DDMTemplateModelImpl ddmTemplateModelImpl = this;
ddmTemplateModelImpl._originalUuid = ddmTemplateModelImpl._uuid;
ddmTemplateModelImpl._originalGroupId = ddmTemplateModelImpl._groupId;
ddmTemplateModelImpl._setOriginalGroupId = false;
ddmTemplateModelImpl._originalCompanyId =
ddmTemplateModelImpl._companyId;
ddmTemplateModelImpl._setOriginalCompanyId = false;
ddmTemplateModelImpl._setModifiedDate = false;
ddmTemplateModelImpl._originalClassNameId =
ddmTemplateModelImpl._classNameId;
ddmTemplateModelImpl._setOriginalClassNameId = false;
ddmTemplateModelImpl._originalClassPK = ddmTemplateModelImpl._classPK;
ddmTemplateModelImpl._setOriginalClassPK = false;
ddmTemplateModelImpl._originalTemplateKey =
ddmTemplateModelImpl._templateKey;
ddmTemplateModelImpl._originalType = ddmTemplateModelImpl._type;
ddmTemplateModelImpl._originalMode = ddmTemplateModelImpl._mode;
ddmTemplateModelImpl._originalLanguage = ddmTemplateModelImpl._language;
ddmTemplateModelImpl._originalSmallImageId =
ddmTemplateModelImpl._smallImageId;
ddmTemplateModelImpl._setOriginalSmallImageId = false;
setResourceClassName(null);
ddmTemplateModelImpl._columnBitmask = 0;
}
@Override
public CacheModel toCacheModel() {
DDMTemplateCacheModel ddmTemplateCacheModel =
new DDMTemplateCacheModel();
ddmTemplateCacheModel.mvccVersion = getMvccVersion();
ddmTemplateCacheModel.ctCollectionId = getCtCollectionId();
ddmTemplateCacheModel.uuid = getUuid();
String uuid = ddmTemplateCacheModel.uuid;
if ((uuid != null) && (uuid.length() == 0)) {
ddmTemplateCacheModel.uuid = null;
}
ddmTemplateCacheModel.templateId = getTemplateId();
ddmTemplateCacheModel.groupId = getGroupId();
ddmTemplateCacheModel.companyId = getCompanyId();
ddmTemplateCacheModel.userId = getUserId();
ddmTemplateCacheModel.userName = getUserName();
String userName = ddmTemplateCacheModel.userName;
if ((userName != null) && (userName.length() == 0)) {
ddmTemplateCacheModel.userName = null;
}
ddmTemplateCacheModel.versionUserId = getVersionUserId();
ddmTemplateCacheModel.versionUserName = getVersionUserName();
String versionUserName = ddmTemplateCacheModel.versionUserName;
if ((versionUserName != null) && (versionUserName.length() == 0)) {
ddmTemplateCacheModel.versionUserName = null;
}
Date createDate = getCreateDate();
if (createDate != null) {
ddmTemplateCacheModel.createDate = createDate.getTime();
}
else {
ddmTemplateCacheModel.createDate = Long.MIN_VALUE;
}
Date modifiedDate = getModifiedDate();
if (modifiedDate != null) {
ddmTemplateCacheModel.modifiedDate = modifiedDate.getTime();
}
else {
ddmTemplateCacheModel.modifiedDate = Long.MIN_VALUE;
}
ddmTemplateCacheModel.classNameId = getClassNameId();
ddmTemplateCacheModel.classPK = getClassPK();
ddmTemplateCacheModel.resourceClassNameId = getResourceClassNameId();
ddmTemplateCacheModel.templateKey = getTemplateKey();
String templateKey = ddmTemplateCacheModel.templateKey;
if ((templateKey != null) && (templateKey.length() == 0)) {
ddmTemplateCacheModel.templateKey = null;
}
ddmTemplateCacheModel.version = getVersion();
String version = ddmTemplateCacheModel.version;
if ((version != null) && (version.length() == 0)) {
ddmTemplateCacheModel.version = null;
}
ddmTemplateCacheModel.name = getName();
String name = ddmTemplateCacheModel.name;
if ((name != null) && (name.length() == 0)) {
ddmTemplateCacheModel.name = null;
}
ddmTemplateCacheModel.description = getDescription();
String description = ddmTemplateCacheModel.description;
if ((description != null) && (description.length() == 0)) {
ddmTemplateCacheModel.description = null;
}
ddmTemplateCacheModel.type = getType();
String type = ddmTemplateCacheModel.type;
if ((type != null) && (type.length() == 0)) {
ddmTemplateCacheModel.type = null;
}
ddmTemplateCacheModel.mode = getMode();
String mode = ddmTemplateCacheModel.mode;
if ((mode != null) && (mode.length() == 0)) {
ddmTemplateCacheModel.mode = null;
}
ddmTemplateCacheModel.language = getLanguage();
String language = ddmTemplateCacheModel.language;
if ((language != null) && (language.length() == 0)) {
ddmTemplateCacheModel.language = null;
}
ddmTemplateCacheModel.script = getScript();
String script = ddmTemplateCacheModel.script;
if ((script != null) && (script.length() == 0)) {
ddmTemplateCacheModel.script = null;
}
ddmTemplateCacheModel.cacheable = isCacheable();
ddmTemplateCacheModel.smallImage = isSmallImage();
ddmTemplateCacheModel.smallImageId = getSmallImageId();
ddmTemplateCacheModel.smallImageURL = getSmallImageURL();
String smallImageURL = ddmTemplateCacheModel.smallImageURL;
if ((smallImageURL != null) && (smallImageURL.length() == 0)) {
ddmTemplateCacheModel.smallImageURL = null;
}
Date lastPublishDate = getLastPublishDate();
if (lastPublishDate != null) {
ddmTemplateCacheModel.lastPublishDate = lastPublishDate.getTime();
}
else {
ddmTemplateCacheModel.lastPublishDate = Long.MIN_VALUE;
}
ddmTemplateCacheModel._resourceClassName = getResourceClassName();
return ddmTemplateCacheModel;
}
@Override
public String toString() {
Map> attributeGetterFunctions =
getAttributeGetterFunctions();
StringBundler sb = new StringBundler(
4 * attributeGetterFunctions.size() + 2);
sb.append("{");
for (Map.Entry> entry :
attributeGetterFunctions.entrySet()) {
String attributeName = entry.getKey();
Function attributeGetterFunction =
entry.getValue();
sb.append(attributeName);
sb.append("=");
sb.append(attributeGetterFunction.apply((DDMTemplate)this));
sb.append(", ");
}
if (sb.index() > 1) {
sb.setIndex(sb.index() - 1);
}
sb.append("}");
return sb.toString();
}
@Override
public String toXmlString() {
Map> attributeGetterFunctions =
getAttributeGetterFunctions();
StringBundler sb = new StringBundler(
5 * attributeGetterFunctions.size() + 4);
sb.append("");
sb.append(getModelClassName());
sb.append(" ");
for (Map.Entry> entry :
attributeGetterFunctions.entrySet()) {
String attributeName = entry.getKey();
Function attributeGetterFunction =
entry.getValue();
sb.append("");
sb.append(attributeName);
sb.append(" ");
}
sb.append(" ");
return sb.toString();
}
private static class EscapedModelProxyProviderFunctionHolder {
private static final Function
_escapedModelProxyProviderFunction = _getProxyProviderFunction();
}
private static boolean _entityCacheEnabled;
private static boolean _finderCacheEnabled;
private long _mvccVersion;
private long _ctCollectionId;
private String _uuid;
private String _originalUuid;
private long _templateId;
private long _groupId;
private long _originalGroupId;
private boolean _setOriginalGroupId;
private long _companyId;
private long _originalCompanyId;
private boolean _setOriginalCompanyId;
private long _userId;
private String _userName;
private long _versionUserId;
private String _versionUserName;
private Date _createDate;
private Date _modifiedDate;
private boolean _setModifiedDate;
private long _classNameId;
private long _originalClassNameId;
private boolean _setOriginalClassNameId;
private long _classPK;
private long _originalClassPK;
private boolean _setOriginalClassPK;
private long _resourceClassNameId;
private String _templateKey;
private String _originalTemplateKey;
private String _version;
private String _name;
private String _nameCurrentLanguageId;
private String _description;
private String _descriptionCurrentLanguageId;
private String _type;
private String _originalType;
private String _mode;
private String _originalMode;
private String _language;
private String _originalLanguage;
private String _script;
private boolean _cacheable;
private boolean _smallImage;
private long _smallImageId;
private long _originalSmallImageId;
private boolean _setOriginalSmallImageId;
private String _smallImageURL;
private Date _lastPublishDate;
private long _columnBitmask;
private DDMTemplate _escapedModel;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy