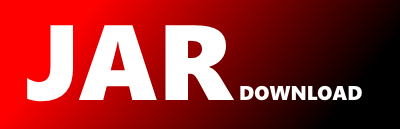
com.liferay.fragment.service.persistence.FragmentEntryVersionPersistence Maven / Gradle / Ivy
/**
* SPDX-FileCopyrightText: (c) 2000 Liferay, Inc. https://liferay.com
* SPDX-License-Identifier: LGPL-2.1-or-later OR LicenseRef-Liferay-DXP-EULA-2.0.0-2023-06
*/
package com.liferay.fragment.service.persistence;
import com.liferay.fragment.exception.NoSuchEntryVersionException;
import com.liferay.fragment.model.FragmentEntryVersion;
import com.liferay.portal.kernel.service.persistence.BasePersistence;
import com.liferay.portal.kernel.service.persistence.change.tracking.CTPersistence;
import org.osgi.annotation.versioning.ProviderType;
/**
* The persistence interface for the fragment entry version service.
*
*
* Caching information and settings can be found in portal.properties
*
*
* @author Brian Wing Shun Chan
* @see FragmentEntryVersionUtil
* @generated
*/
@ProviderType
public interface FragmentEntryVersionPersistence
extends BasePersistence,
CTPersistence {
/*
* NOTE FOR DEVELOPERS:
*
* Never modify or reference this interface directly. Always use {@link FragmentEntryVersionUtil} to access the fragment entry version persistence. Modify service.xml
and rerun ServiceBuilder to regenerate this interface.
*/
/**
* Returns all the fragment entry versions where fragmentEntryId = ?.
*
* @param fragmentEntryId the fragment entry ID
* @return the matching fragment entry versions
*/
public java.util.List findByFragmentEntryId(
long fragmentEntryId);
/**
* Returns a range of all the fragment entry versions where fragmentEntryId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param fragmentEntryId the fragment entry ID
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @return the range of matching fragment entry versions
*/
public java.util.List findByFragmentEntryId(
long fragmentEntryId, int start, int end);
/**
* Returns an ordered range of all the fragment entry versions where fragmentEntryId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param fragmentEntryId the fragment entry ID
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entry versions
*/
public java.util.List findByFragmentEntryId(
long fragmentEntryId, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns an ordered range of all the fragment entry versions where fragmentEntryId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param fragmentEntryId the fragment entry ID
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching fragment entry versions
*/
public java.util.List findByFragmentEntryId(
long fragmentEntryId, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator,
boolean useFinderCache);
/**
* Returns the first fragment entry version in the ordered set where fragmentEntryId = ?.
*
* @param fragmentEntryId the fragment entry ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry version
* @throws NoSuchEntryVersionException if a matching fragment entry version could not be found
*/
public FragmentEntryVersion findByFragmentEntryId_First(
long fragmentEntryId,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Returns the first fragment entry version in the ordered set where fragmentEntryId = ?.
*
* @param fragmentEntryId the fragment entry ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry version, or null
if a matching fragment entry version could not be found
*/
public FragmentEntryVersion fetchByFragmentEntryId_First(
long fragmentEntryId,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the last fragment entry version in the ordered set where fragmentEntryId = ?.
*
* @param fragmentEntryId the fragment entry ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry version
* @throws NoSuchEntryVersionException if a matching fragment entry version could not be found
*/
public FragmentEntryVersion findByFragmentEntryId_Last(
long fragmentEntryId,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Returns the last fragment entry version in the ordered set where fragmentEntryId = ?.
*
* @param fragmentEntryId the fragment entry ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry version, or null
if a matching fragment entry version could not be found
*/
public FragmentEntryVersion fetchByFragmentEntryId_Last(
long fragmentEntryId,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the fragment entry versions before and after the current fragment entry version in the ordered set where fragmentEntryId = ?.
*
* @param fragmentEntryVersionId the primary key of the current fragment entry version
* @param fragmentEntryId the fragment entry ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next fragment entry version
* @throws NoSuchEntryVersionException if a fragment entry version with the primary key could not be found
*/
public FragmentEntryVersion[] findByFragmentEntryId_PrevAndNext(
long fragmentEntryVersionId, long fragmentEntryId,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Removes all the fragment entry versions where fragmentEntryId = ? from the database.
*
* @param fragmentEntryId the fragment entry ID
*/
public void removeByFragmentEntryId(long fragmentEntryId);
/**
* Returns the number of fragment entry versions where fragmentEntryId = ?.
*
* @param fragmentEntryId the fragment entry ID
* @return the number of matching fragment entry versions
*/
public int countByFragmentEntryId(long fragmentEntryId);
/**
* Returns the fragment entry version where fragmentEntryId = ? and version = ? or throws a NoSuchEntryVersionException
if it could not be found.
*
* @param fragmentEntryId the fragment entry ID
* @param version the version
* @return the matching fragment entry version
* @throws NoSuchEntryVersionException if a matching fragment entry version could not be found
*/
public FragmentEntryVersion findByFragmentEntryId_Version(
long fragmentEntryId, int version)
throws NoSuchEntryVersionException;
/**
* Returns the fragment entry version where fragmentEntryId = ? and version = ? or returns null
if it could not be found. Uses the finder cache.
*
* @param fragmentEntryId the fragment entry ID
* @param version the version
* @return the matching fragment entry version, or null
if a matching fragment entry version could not be found
*/
public FragmentEntryVersion fetchByFragmentEntryId_Version(
long fragmentEntryId, int version);
/**
* Returns the fragment entry version where fragmentEntryId = ? and version = ? or returns null
if it could not be found, optionally using the finder cache.
*
* @param fragmentEntryId the fragment entry ID
* @param version the version
* @param useFinderCache whether to use the finder cache
* @return the matching fragment entry version, or null
if a matching fragment entry version could not be found
*/
public FragmentEntryVersion fetchByFragmentEntryId_Version(
long fragmentEntryId, int version, boolean useFinderCache);
/**
* Removes the fragment entry version where fragmentEntryId = ? and version = ? from the database.
*
* @param fragmentEntryId the fragment entry ID
* @param version the version
* @return the fragment entry version that was removed
*/
public FragmentEntryVersion removeByFragmentEntryId_Version(
long fragmentEntryId, int version)
throws NoSuchEntryVersionException;
/**
* Returns the number of fragment entry versions where fragmentEntryId = ? and version = ?.
*
* @param fragmentEntryId the fragment entry ID
* @param version the version
* @return the number of matching fragment entry versions
*/
public int countByFragmentEntryId_Version(
long fragmentEntryId, int version);
/**
* Returns all the fragment entry versions where uuid = ?.
*
* @param uuid the uuid
* @return the matching fragment entry versions
*/
public java.util.List findByUuid(String uuid);
/**
* Returns a range of all the fragment entry versions where uuid = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param uuid the uuid
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @return the range of matching fragment entry versions
*/
public java.util.List findByUuid(
String uuid, int start, int end);
/**
* Returns an ordered range of all the fragment entry versions where uuid = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param uuid the uuid
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entry versions
*/
public java.util.List findByUuid(
String uuid, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns an ordered range of all the fragment entry versions where uuid = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param uuid the uuid
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching fragment entry versions
*/
public java.util.List findByUuid(
String uuid, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator,
boolean useFinderCache);
/**
* Returns the first fragment entry version in the ordered set where uuid = ?.
*
* @param uuid the uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry version
* @throws NoSuchEntryVersionException if a matching fragment entry version could not be found
*/
public FragmentEntryVersion findByUuid_First(
String uuid,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Returns the first fragment entry version in the ordered set where uuid = ?.
*
* @param uuid the uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry version, or null
if a matching fragment entry version could not be found
*/
public FragmentEntryVersion fetchByUuid_First(
String uuid,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the last fragment entry version in the ordered set where uuid = ?.
*
* @param uuid the uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry version
* @throws NoSuchEntryVersionException if a matching fragment entry version could not be found
*/
public FragmentEntryVersion findByUuid_Last(
String uuid,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Returns the last fragment entry version in the ordered set where uuid = ?.
*
* @param uuid the uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry version, or null
if a matching fragment entry version could not be found
*/
public FragmentEntryVersion fetchByUuid_Last(
String uuid,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the fragment entry versions before and after the current fragment entry version in the ordered set where uuid = ?.
*
* @param fragmentEntryVersionId the primary key of the current fragment entry version
* @param uuid the uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next fragment entry version
* @throws NoSuchEntryVersionException if a fragment entry version with the primary key could not be found
*/
public FragmentEntryVersion[] findByUuid_PrevAndNext(
long fragmentEntryVersionId, String uuid,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Removes all the fragment entry versions where uuid = ? from the database.
*
* @param uuid the uuid
*/
public void removeByUuid(String uuid);
/**
* Returns the number of fragment entry versions where uuid = ?.
*
* @param uuid the uuid
* @return the number of matching fragment entry versions
*/
public int countByUuid(String uuid);
/**
* Returns all the fragment entry versions where uuid = ? and version = ?.
*
* @param uuid the uuid
* @param version the version
* @return the matching fragment entry versions
*/
public java.util.List findByUuid_Version(
String uuid, int version);
/**
* Returns a range of all the fragment entry versions where uuid = ? and version = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param uuid the uuid
* @param version the version
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @return the range of matching fragment entry versions
*/
public java.util.List findByUuid_Version(
String uuid, int version, int start, int end);
/**
* Returns an ordered range of all the fragment entry versions where uuid = ? and version = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param uuid the uuid
* @param version the version
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entry versions
*/
public java.util.List findByUuid_Version(
String uuid, int version, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns an ordered range of all the fragment entry versions where uuid = ? and version = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param uuid the uuid
* @param version the version
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching fragment entry versions
*/
public java.util.List findByUuid_Version(
String uuid, int version, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator,
boolean useFinderCache);
/**
* Returns the first fragment entry version in the ordered set where uuid = ? and version = ?.
*
* @param uuid the uuid
* @param version the version
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry version
* @throws NoSuchEntryVersionException if a matching fragment entry version could not be found
*/
public FragmentEntryVersion findByUuid_Version_First(
String uuid, int version,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Returns the first fragment entry version in the ordered set where uuid = ? and version = ?.
*
* @param uuid the uuid
* @param version the version
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry version, or null
if a matching fragment entry version could not be found
*/
public FragmentEntryVersion fetchByUuid_Version_First(
String uuid, int version,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the last fragment entry version in the ordered set where uuid = ? and version = ?.
*
* @param uuid the uuid
* @param version the version
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry version
* @throws NoSuchEntryVersionException if a matching fragment entry version could not be found
*/
public FragmentEntryVersion findByUuid_Version_Last(
String uuid, int version,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Returns the last fragment entry version in the ordered set where uuid = ? and version = ?.
*
* @param uuid the uuid
* @param version the version
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry version, or null
if a matching fragment entry version could not be found
*/
public FragmentEntryVersion fetchByUuid_Version_Last(
String uuid, int version,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the fragment entry versions before and after the current fragment entry version in the ordered set where uuid = ? and version = ?.
*
* @param fragmentEntryVersionId the primary key of the current fragment entry version
* @param uuid the uuid
* @param version the version
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next fragment entry version
* @throws NoSuchEntryVersionException if a fragment entry version with the primary key could not be found
*/
public FragmentEntryVersion[] findByUuid_Version_PrevAndNext(
long fragmentEntryVersionId, String uuid, int version,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Removes all the fragment entry versions where uuid = ? and version = ? from the database.
*
* @param uuid the uuid
* @param version the version
*/
public void removeByUuid_Version(String uuid, int version);
/**
* Returns the number of fragment entry versions where uuid = ? and version = ?.
*
* @param uuid the uuid
* @param version the version
* @return the number of matching fragment entry versions
*/
public int countByUuid_Version(String uuid, int version);
/**
* Returns all the fragment entry versions where uuid = ? and groupId = ?.
*
* @param uuid the uuid
* @param groupId the group ID
* @return the matching fragment entry versions
*/
public java.util.List findByUUID_G(
String uuid, long groupId);
/**
* Returns a range of all the fragment entry versions where uuid = ? and groupId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param uuid the uuid
* @param groupId the group ID
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @return the range of matching fragment entry versions
*/
public java.util.List findByUUID_G(
String uuid, long groupId, int start, int end);
/**
* Returns an ordered range of all the fragment entry versions where uuid = ? and groupId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param uuid the uuid
* @param groupId the group ID
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entry versions
*/
public java.util.List findByUUID_G(
String uuid, long groupId, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns an ordered range of all the fragment entry versions where uuid = ? and groupId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param uuid the uuid
* @param groupId the group ID
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching fragment entry versions
*/
public java.util.List findByUUID_G(
String uuid, long groupId, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator,
boolean useFinderCache);
/**
* Returns the first fragment entry version in the ordered set where uuid = ? and groupId = ?.
*
* @param uuid the uuid
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry version
* @throws NoSuchEntryVersionException if a matching fragment entry version could not be found
*/
public FragmentEntryVersion findByUUID_G_First(
String uuid, long groupId,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Returns the first fragment entry version in the ordered set where uuid = ? and groupId = ?.
*
* @param uuid the uuid
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry version, or null
if a matching fragment entry version could not be found
*/
public FragmentEntryVersion fetchByUUID_G_First(
String uuid, long groupId,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the last fragment entry version in the ordered set where uuid = ? and groupId = ?.
*
* @param uuid the uuid
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry version
* @throws NoSuchEntryVersionException if a matching fragment entry version could not be found
*/
public FragmentEntryVersion findByUUID_G_Last(
String uuid, long groupId,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Returns the last fragment entry version in the ordered set where uuid = ? and groupId = ?.
*
* @param uuid the uuid
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry version, or null
if a matching fragment entry version could not be found
*/
public FragmentEntryVersion fetchByUUID_G_Last(
String uuid, long groupId,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the fragment entry versions before and after the current fragment entry version in the ordered set where uuid = ? and groupId = ?.
*
* @param fragmentEntryVersionId the primary key of the current fragment entry version
* @param uuid the uuid
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next fragment entry version
* @throws NoSuchEntryVersionException if a fragment entry version with the primary key could not be found
*/
public FragmentEntryVersion[] findByUUID_G_PrevAndNext(
long fragmentEntryVersionId, String uuid, long groupId,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Removes all the fragment entry versions where uuid = ? and groupId = ? from the database.
*
* @param uuid the uuid
* @param groupId the group ID
*/
public void removeByUUID_G(String uuid, long groupId);
/**
* Returns the number of fragment entry versions where uuid = ? and groupId = ?.
*
* @param uuid the uuid
* @param groupId the group ID
* @return the number of matching fragment entry versions
*/
public int countByUUID_G(String uuid, long groupId);
/**
* Returns the fragment entry version where uuid = ? and groupId = ? and version = ? or throws a NoSuchEntryVersionException
if it could not be found.
*
* @param uuid the uuid
* @param groupId the group ID
* @param version the version
* @return the matching fragment entry version
* @throws NoSuchEntryVersionException if a matching fragment entry version could not be found
*/
public FragmentEntryVersion findByUUID_G_Version(
String uuid, long groupId, int version)
throws NoSuchEntryVersionException;
/**
* Returns the fragment entry version where uuid = ? and groupId = ? and version = ? or returns null
if it could not be found. Uses the finder cache.
*
* @param uuid the uuid
* @param groupId the group ID
* @param version the version
* @return the matching fragment entry version, or null
if a matching fragment entry version could not be found
*/
public FragmentEntryVersion fetchByUUID_G_Version(
String uuid, long groupId, int version);
/**
* Returns the fragment entry version where uuid = ? and groupId = ? and version = ? or returns null
if it could not be found, optionally using the finder cache.
*
* @param uuid the uuid
* @param groupId the group ID
* @param version the version
* @param useFinderCache whether to use the finder cache
* @return the matching fragment entry version, or null
if a matching fragment entry version could not be found
*/
public FragmentEntryVersion fetchByUUID_G_Version(
String uuid, long groupId, int version, boolean useFinderCache);
/**
* Removes the fragment entry version where uuid = ? and groupId = ? and version = ? from the database.
*
* @param uuid the uuid
* @param groupId the group ID
* @param version the version
* @return the fragment entry version that was removed
*/
public FragmentEntryVersion removeByUUID_G_Version(
String uuid, long groupId, int version)
throws NoSuchEntryVersionException;
/**
* Returns the number of fragment entry versions where uuid = ? and groupId = ? and version = ?.
*
* @param uuid the uuid
* @param groupId the group ID
* @param version the version
* @return the number of matching fragment entry versions
*/
public int countByUUID_G_Version(String uuid, long groupId, int version);
/**
* Returns all the fragment entry versions where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @return the matching fragment entry versions
*/
public java.util.List findByUuid_C(
String uuid, long companyId);
/**
* Returns a range of all the fragment entry versions where uuid = ? and companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param uuid the uuid
* @param companyId the company ID
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @return the range of matching fragment entry versions
*/
public java.util.List findByUuid_C(
String uuid, long companyId, int start, int end);
/**
* Returns an ordered range of all the fragment entry versions where uuid = ? and companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param uuid the uuid
* @param companyId the company ID
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entry versions
*/
public java.util.List findByUuid_C(
String uuid, long companyId, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns an ordered range of all the fragment entry versions where uuid = ? and companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param uuid the uuid
* @param companyId the company ID
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching fragment entry versions
*/
public java.util.List findByUuid_C(
String uuid, long companyId, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator,
boolean useFinderCache);
/**
* Returns the first fragment entry version in the ordered set where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry version
* @throws NoSuchEntryVersionException if a matching fragment entry version could not be found
*/
public FragmentEntryVersion findByUuid_C_First(
String uuid, long companyId,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Returns the first fragment entry version in the ordered set where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry version, or null
if a matching fragment entry version could not be found
*/
public FragmentEntryVersion fetchByUuid_C_First(
String uuid, long companyId,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the last fragment entry version in the ordered set where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry version
* @throws NoSuchEntryVersionException if a matching fragment entry version could not be found
*/
public FragmentEntryVersion findByUuid_C_Last(
String uuid, long companyId,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Returns the last fragment entry version in the ordered set where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry version, or null
if a matching fragment entry version could not be found
*/
public FragmentEntryVersion fetchByUuid_C_Last(
String uuid, long companyId,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the fragment entry versions before and after the current fragment entry version in the ordered set where uuid = ? and companyId = ?.
*
* @param fragmentEntryVersionId the primary key of the current fragment entry version
* @param uuid the uuid
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next fragment entry version
* @throws NoSuchEntryVersionException if a fragment entry version with the primary key could not be found
*/
public FragmentEntryVersion[] findByUuid_C_PrevAndNext(
long fragmentEntryVersionId, String uuid, long companyId,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Removes all the fragment entry versions where uuid = ? and companyId = ? from the database.
*
* @param uuid the uuid
* @param companyId the company ID
*/
public void removeByUuid_C(String uuid, long companyId);
/**
* Returns the number of fragment entry versions where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @return the number of matching fragment entry versions
*/
public int countByUuid_C(String uuid, long companyId);
/**
* Returns all the fragment entry versions where uuid = ? and companyId = ? and version = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @param version the version
* @return the matching fragment entry versions
*/
public java.util.List findByUuid_C_Version(
String uuid, long companyId, int version);
/**
* Returns a range of all the fragment entry versions where uuid = ? and companyId = ? and version = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param uuid the uuid
* @param companyId the company ID
* @param version the version
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @return the range of matching fragment entry versions
*/
public java.util.List findByUuid_C_Version(
String uuid, long companyId, int version, int start, int end);
/**
* Returns an ordered range of all the fragment entry versions where uuid = ? and companyId = ? and version = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param uuid the uuid
* @param companyId the company ID
* @param version the version
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entry versions
*/
public java.util.List findByUuid_C_Version(
String uuid, long companyId, int version, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns an ordered range of all the fragment entry versions where uuid = ? and companyId = ? and version = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param uuid the uuid
* @param companyId the company ID
* @param version the version
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching fragment entry versions
*/
public java.util.List findByUuid_C_Version(
String uuid, long companyId, int version, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator,
boolean useFinderCache);
/**
* Returns the first fragment entry version in the ordered set where uuid = ? and companyId = ? and version = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @param version the version
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry version
* @throws NoSuchEntryVersionException if a matching fragment entry version could not be found
*/
public FragmentEntryVersion findByUuid_C_Version_First(
String uuid, long companyId, int version,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Returns the first fragment entry version in the ordered set where uuid = ? and companyId = ? and version = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @param version the version
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry version, or null
if a matching fragment entry version could not be found
*/
public FragmentEntryVersion fetchByUuid_C_Version_First(
String uuid, long companyId, int version,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the last fragment entry version in the ordered set where uuid = ? and companyId = ? and version = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @param version the version
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry version
* @throws NoSuchEntryVersionException if a matching fragment entry version could not be found
*/
public FragmentEntryVersion findByUuid_C_Version_Last(
String uuid, long companyId, int version,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Returns the last fragment entry version in the ordered set where uuid = ? and companyId = ? and version = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @param version the version
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry version, or null
if a matching fragment entry version could not be found
*/
public FragmentEntryVersion fetchByUuid_C_Version_Last(
String uuid, long companyId, int version,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the fragment entry versions before and after the current fragment entry version in the ordered set where uuid = ? and companyId = ? and version = ?.
*
* @param fragmentEntryVersionId the primary key of the current fragment entry version
* @param uuid the uuid
* @param companyId the company ID
* @param version the version
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next fragment entry version
* @throws NoSuchEntryVersionException if a fragment entry version with the primary key could not be found
*/
public FragmentEntryVersion[] findByUuid_C_Version_PrevAndNext(
long fragmentEntryVersionId, String uuid, long companyId,
int version,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Removes all the fragment entry versions where uuid = ? and companyId = ? and version = ? from the database.
*
* @param uuid the uuid
* @param companyId the company ID
* @param version the version
*/
public void removeByUuid_C_Version(
String uuid, long companyId, int version);
/**
* Returns the number of fragment entry versions where uuid = ? and companyId = ? and version = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @param version the version
* @return the number of matching fragment entry versions
*/
public int countByUuid_C_Version(String uuid, long companyId, int version);
/**
* Returns all the fragment entry versions where groupId = ?.
*
* @param groupId the group ID
* @return the matching fragment entry versions
*/
public java.util.List findByGroupId(long groupId);
/**
* Returns a range of all the fragment entry versions where groupId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param groupId the group ID
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @return the range of matching fragment entry versions
*/
public java.util.List findByGroupId(
long groupId, int start, int end);
/**
* Returns an ordered range of all the fragment entry versions where groupId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param groupId the group ID
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entry versions
*/
public java.util.List findByGroupId(
long groupId, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns an ordered range of all the fragment entry versions where groupId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param groupId the group ID
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching fragment entry versions
*/
public java.util.List findByGroupId(
long groupId, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator,
boolean useFinderCache);
/**
* Returns the first fragment entry version in the ordered set where groupId = ?.
*
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry version
* @throws NoSuchEntryVersionException if a matching fragment entry version could not be found
*/
public FragmentEntryVersion findByGroupId_First(
long groupId,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Returns the first fragment entry version in the ordered set where groupId = ?.
*
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry version, or null
if a matching fragment entry version could not be found
*/
public FragmentEntryVersion fetchByGroupId_First(
long groupId,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the last fragment entry version in the ordered set where groupId = ?.
*
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry version
* @throws NoSuchEntryVersionException if a matching fragment entry version could not be found
*/
public FragmentEntryVersion findByGroupId_Last(
long groupId,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Returns the last fragment entry version in the ordered set where groupId = ?.
*
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry version, or null
if a matching fragment entry version could not be found
*/
public FragmentEntryVersion fetchByGroupId_Last(
long groupId,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the fragment entry versions before and after the current fragment entry version in the ordered set where groupId = ?.
*
* @param fragmentEntryVersionId the primary key of the current fragment entry version
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next fragment entry version
* @throws NoSuchEntryVersionException if a fragment entry version with the primary key could not be found
*/
public FragmentEntryVersion[] findByGroupId_PrevAndNext(
long fragmentEntryVersionId, long groupId,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Removes all the fragment entry versions where groupId = ? from the database.
*
* @param groupId the group ID
*/
public void removeByGroupId(long groupId);
/**
* Returns the number of fragment entry versions where groupId = ?.
*
* @param groupId the group ID
* @return the number of matching fragment entry versions
*/
public int countByGroupId(long groupId);
/**
* Returns all the fragment entry versions where groupId = ? and version = ?.
*
* @param groupId the group ID
* @param version the version
* @return the matching fragment entry versions
*/
public java.util.List findByGroupId_Version(
long groupId, int version);
/**
* Returns a range of all the fragment entry versions where groupId = ? and version = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param groupId the group ID
* @param version the version
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @return the range of matching fragment entry versions
*/
public java.util.List findByGroupId_Version(
long groupId, int version, int start, int end);
/**
* Returns an ordered range of all the fragment entry versions where groupId = ? and version = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param groupId the group ID
* @param version the version
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entry versions
*/
public java.util.List findByGroupId_Version(
long groupId, int version, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns an ordered range of all the fragment entry versions where groupId = ? and version = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param groupId the group ID
* @param version the version
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching fragment entry versions
*/
public java.util.List findByGroupId_Version(
long groupId, int version, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator,
boolean useFinderCache);
/**
* Returns the first fragment entry version in the ordered set where groupId = ? and version = ?.
*
* @param groupId the group ID
* @param version the version
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry version
* @throws NoSuchEntryVersionException if a matching fragment entry version could not be found
*/
public FragmentEntryVersion findByGroupId_Version_First(
long groupId, int version,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Returns the first fragment entry version in the ordered set where groupId = ? and version = ?.
*
* @param groupId the group ID
* @param version the version
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry version, or null
if a matching fragment entry version could not be found
*/
public FragmentEntryVersion fetchByGroupId_Version_First(
long groupId, int version,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the last fragment entry version in the ordered set where groupId = ? and version = ?.
*
* @param groupId the group ID
* @param version the version
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry version
* @throws NoSuchEntryVersionException if a matching fragment entry version could not be found
*/
public FragmentEntryVersion findByGroupId_Version_Last(
long groupId, int version,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Returns the last fragment entry version in the ordered set where groupId = ? and version = ?.
*
* @param groupId the group ID
* @param version the version
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry version, or null
if a matching fragment entry version could not be found
*/
public FragmentEntryVersion fetchByGroupId_Version_Last(
long groupId, int version,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the fragment entry versions before and after the current fragment entry version in the ordered set where groupId = ? and version = ?.
*
* @param fragmentEntryVersionId the primary key of the current fragment entry version
* @param groupId the group ID
* @param version the version
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next fragment entry version
* @throws NoSuchEntryVersionException if a fragment entry version with the primary key could not be found
*/
public FragmentEntryVersion[] findByGroupId_Version_PrevAndNext(
long fragmentEntryVersionId, long groupId, int version,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Removes all the fragment entry versions where groupId = ? and version = ? from the database.
*
* @param groupId the group ID
* @param version the version
*/
public void removeByGroupId_Version(long groupId, int version);
/**
* Returns the number of fragment entry versions where groupId = ? and version = ?.
*
* @param groupId the group ID
* @param version the version
* @return the number of matching fragment entry versions
*/
public int countByGroupId_Version(long groupId, int version);
/**
* Returns all the fragment entry versions where fragmentCollectionId = ?.
*
* @param fragmentCollectionId the fragment collection ID
* @return the matching fragment entry versions
*/
public java.util.List findByFragmentCollectionId(
long fragmentCollectionId);
/**
* Returns a range of all the fragment entry versions where fragmentCollectionId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param fragmentCollectionId the fragment collection ID
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @return the range of matching fragment entry versions
*/
public java.util.List findByFragmentCollectionId(
long fragmentCollectionId, int start, int end);
/**
* Returns an ordered range of all the fragment entry versions where fragmentCollectionId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param fragmentCollectionId the fragment collection ID
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entry versions
*/
public java.util.List findByFragmentCollectionId(
long fragmentCollectionId, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns an ordered range of all the fragment entry versions where fragmentCollectionId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param fragmentCollectionId the fragment collection ID
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching fragment entry versions
*/
public java.util.List findByFragmentCollectionId(
long fragmentCollectionId, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator,
boolean useFinderCache);
/**
* Returns the first fragment entry version in the ordered set where fragmentCollectionId = ?.
*
* @param fragmentCollectionId the fragment collection ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry version
* @throws NoSuchEntryVersionException if a matching fragment entry version could not be found
*/
public FragmentEntryVersion findByFragmentCollectionId_First(
long fragmentCollectionId,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Returns the first fragment entry version in the ordered set where fragmentCollectionId = ?.
*
* @param fragmentCollectionId the fragment collection ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry version, or null
if a matching fragment entry version could not be found
*/
public FragmentEntryVersion fetchByFragmentCollectionId_First(
long fragmentCollectionId,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the last fragment entry version in the ordered set where fragmentCollectionId = ?.
*
* @param fragmentCollectionId the fragment collection ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry version
* @throws NoSuchEntryVersionException if a matching fragment entry version could not be found
*/
public FragmentEntryVersion findByFragmentCollectionId_Last(
long fragmentCollectionId,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Returns the last fragment entry version in the ordered set where fragmentCollectionId = ?.
*
* @param fragmentCollectionId the fragment collection ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry version, or null
if a matching fragment entry version could not be found
*/
public FragmentEntryVersion fetchByFragmentCollectionId_Last(
long fragmentCollectionId,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the fragment entry versions before and after the current fragment entry version in the ordered set where fragmentCollectionId = ?.
*
* @param fragmentEntryVersionId the primary key of the current fragment entry version
* @param fragmentCollectionId the fragment collection ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next fragment entry version
* @throws NoSuchEntryVersionException if a fragment entry version with the primary key could not be found
*/
public FragmentEntryVersion[] findByFragmentCollectionId_PrevAndNext(
long fragmentEntryVersionId, long fragmentCollectionId,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Removes all the fragment entry versions where fragmentCollectionId = ? from the database.
*
* @param fragmentCollectionId the fragment collection ID
*/
public void removeByFragmentCollectionId(long fragmentCollectionId);
/**
* Returns the number of fragment entry versions where fragmentCollectionId = ?.
*
* @param fragmentCollectionId the fragment collection ID
* @return the number of matching fragment entry versions
*/
public int countByFragmentCollectionId(long fragmentCollectionId);
/**
* Returns all the fragment entry versions where fragmentCollectionId = ? and version = ?.
*
* @param fragmentCollectionId the fragment collection ID
* @param version the version
* @return the matching fragment entry versions
*/
public java.util.List
findByFragmentCollectionId_Version(
long fragmentCollectionId, int version);
/**
* Returns a range of all the fragment entry versions where fragmentCollectionId = ? and version = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param fragmentCollectionId the fragment collection ID
* @param version the version
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @return the range of matching fragment entry versions
*/
public java.util.List
findByFragmentCollectionId_Version(
long fragmentCollectionId, int version, int start, int end);
/**
* Returns an ordered range of all the fragment entry versions where fragmentCollectionId = ? and version = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param fragmentCollectionId the fragment collection ID
* @param version the version
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entry versions
*/
public java.util.List
findByFragmentCollectionId_Version(
long fragmentCollectionId, int version, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns an ordered range of all the fragment entry versions where fragmentCollectionId = ? and version = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param fragmentCollectionId the fragment collection ID
* @param version the version
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching fragment entry versions
*/
public java.util.List
findByFragmentCollectionId_Version(
long fragmentCollectionId, int version, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator,
boolean useFinderCache);
/**
* Returns the first fragment entry version in the ordered set where fragmentCollectionId = ? and version = ?.
*
* @param fragmentCollectionId the fragment collection ID
* @param version the version
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry version
* @throws NoSuchEntryVersionException if a matching fragment entry version could not be found
*/
public FragmentEntryVersion findByFragmentCollectionId_Version_First(
long fragmentCollectionId, int version,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Returns the first fragment entry version in the ordered set where fragmentCollectionId = ? and version = ?.
*
* @param fragmentCollectionId the fragment collection ID
* @param version the version
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry version, or null
if a matching fragment entry version could not be found
*/
public FragmentEntryVersion fetchByFragmentCollectionId_Version_First(
long fragmentCollectionId, int version,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the last fragment entry version in the ordered set where fragmentCollectionId = ? and version = ?.
*
* @param fragmentCollectionId the fragment collection ID
* @param version the version
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry version
* @throws NoSuchEntryVersionException if a matching fragment entry version could not be found
*/
public FragmentEntryVersion findByFragmentCollectionId_Version_Last(
long fragmentCollectionId, int version,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Returns the last fragment entry version in the ordered set where fragmentCollectionId = ? and version = ?.
*
* @param fragmentCollectionId the fragment collection ID
* @param version the version
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry version, or null
if a matching fragment entry version could not be found
*/
public FragmentEntryVersion fetchByFragmentCollectionId_Version_Last(
long fragmentCollectionId, int version,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the fragment entry versions before and after the current fragment entry version in the ordered set where fragmentCollectionId = ? and version = ?.
*
* @param fragmentEntryVersionId the primary key of the current fragment entry version
* @param fragmentCollectionId the fragment collection ID
* @param version the version
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next fragment entry version
* @throws NoSuchEntryVersionException if a fragment entry version with the primary key could not be found
*/
public FragmentEntryVersion[]
findByFragmentCollectionId_Version_PrevAndNext(
long fragmentEntryVersionId, long fragmentCollectionId,
int version,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Removes all the fragment entry versions where fragmentCollectionId = ? and version = ? from the database.
*
* @param fragmentCollectionId the fragment collection ID
* @param version the version
*/
public void removeByFragmentCollectionId_Version(
long fragmentCollectionId, int version);
/**
* Returns the number of fragment entry versions where fragmentCollectionId = ? and version = ?.
*
* @param fragmentCollectionId the fragment collection ID
* @param version the version
* @return the number of matching fragment entry versions
*/
public int countByFragmentCollectionId_Version(
long fragmentCollectionId, int version);
/**
* Returns all the fragment entry versions where type = ?.
*
* @param type the type
* @return the matching fragment entry versions
*/
public java.util.List findByType(int type);
/**
* Returns a range of all the fragment entry versions where type = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param type the type
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @return the range of matching fragment entry versions
*/
public java.util.List findByType(
int type, int start, int end);
/**
* Returns an ordered range of all the fragment entry versions where type = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param type the type
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entry versions
*/
public java.util.List findByType(
int type, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns an ordered range of all the fragment entry versions where type = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param type the type
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching fragment entry versions
*/
public java.util.List findByType(
int type, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator,
boolean useFinderCache);
/**
* Returns the first fragment entry version in the ordered set where type = ?.
*
* @param type the type
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry version
* @throws NoSuchEntryVersionException if a matching fragment entry version could not be found
*/
public FragmentEntryVersion findByType_First(
int type,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Returns the first fragment entry version in the ordered set where type = ?.
*
* @param type the type
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry version, or null
if a matching fragment entry version could not be found
*/
public FragmentEntryVersion fetchByType_First(
int type,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the last fragment entry version in the ordered set where type = ?.
*
* @param type the type
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry version
* @throws NoSuchEntryVersionException if a matching fragment entry version could not be found
*/
public FragmentEntryVersion findByType_Last(
int type,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Returns the last fragment entry version in the ordered set where type = ?.
*
* @param type the type
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry version, or null
if a matching fragment entry version could not be found
*/
public FragmentEntryVersion fetchByType_Last(
int type,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the fragment entry versions before and after the current fragment entry version in the ordered set where type = ?.
*
* @param fragmentEntryVersionId the primary key of the current fragment entry version
* @param type the type
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next fragment entry version
* @throws NoSuchEntryVersionException if a fragment entry version with the primary key could not be found
*/
public FragmentEntryVersion[] findByType_PrevAndNext(
long fragmentEntryVersionId, int type,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Removes all the fragment entry versions where type = ? from the database.
*
* @param type the type
*/
public void removeByType(int type);
/**
* Returns the number of fragment entry versions where type = ?.
*
* @param type the type
* @return the number of matching fragment entry versions
*/
public int countByType(int type);
/**
* Returns all the fragment entry versions where type = ? and version = ?.
*
* @param type the type
* @param version the version
* @return the matching fragment entry versions
*/
public java.util.List findByType_Version(
int type, int version);
/**
* Returns a range of all the fragment entry versions where type = ? and version = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param type the type
* @param version the version
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @return the range of matching fragment entry versions
*/
public java.util.List findByType_Version(
int type, int version, int start, int end);
/**
* Returns an ordered range of all the fragment entry versions where type = ? and version = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param type the type
* @param version the version
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entry versions
*/
public java.util.List findByType_Version(
int type, int version, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns an ordered range of all the fragment entry versions where type = ? and version = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param type the type
* @param version the version
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching fragment entry versions
*/
public java.util.List findByType_Version(
int type, int version, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator,
boolean useFinderCache);
/**
* Returns the first fragment entry version in the ordered set where type = ? and version = ?.
*
* @param type the type
* @param version the version
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry version
* @throws NoSuchEntryVersionException if a matching fragment entry version could not be found
*/
public FragmentEntryVersion findByType_Version_First(
int type, int version,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Returns the first fragment entry version in the ordered set where type = ? and version = ?.
*
* @param type the type
* @param version the version
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry version, or null
if a matching fragment entry version could not be found
*/
public FragmentEntryVersion fetchByType_Version_First(
int type, int version,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the last fragment entry version in the ordered set where type = ? and version = ?.
*
* @param type the type
* @param version the version
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry version
* @throws NoSuchEntryVersionException if a matching fragment entry version could not be found
*/
public FragmentEntryVersion findByType_Version_Last(
int type, int version,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Returns the last fragment entry version in the ordered set where type = ? and version = ?.
*
* @param type the type
* @param version the version
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry version, or null
if a matching fragment entry version could not be found
*/
public FragmentEntryVersion fetchByType_Version_Last(
int type, int version,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the fragment entry versions before and after the current fragment entry version in the ordered set where type = ? and version = ?.
*
* @param fragmentEntryVersionId the primary key of the current fragment entry version
* @param type the type
* @param version the version
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next fragment entry version
* @throws NoSuchEntryVersionException if a fragment entry version with the primary key could not be found
*/
public FragmentEntryVersion[] findByType_Version_PrevAndNext(
long fragmentEntryVersionId, int type, int version,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Removes all the fragment entry versions where type = ? and version = ? from the database.
*
* @param type the type
* @param version the version
*/
public void removeByType_Version(int type, int version);
/**
* Returns the number of fragment entry versions where type = ? and version = ?.
*
* @param type the type
* @param version the version
* @return the number of matching fragment entry versions
*/
public int countByType_Version(int type, int version);
/**
* Returns all the fragment entry versions where groupId = ? and fragmentCollectionId = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @return the matching fragment entry versions
*/
public java.util.List findByG_FCI(
long groupId, long fragmentCollectionId);
/**
* Returns a range of all the fragment entry versions where groupId = ? and fragmentCollectionId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @return the range of matching fragment entry versions
*/
public java.util.List findByG_FCI(
long groupId, long fragmentCollectionId, int start, int end);
/**
* Returns an ordered range of all the fragment entry versions where groupId = ? and fragmentCollectionId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entry versions
*/
public java.util.List findByG_FCI(
long groupId, long fragmentCollectionId, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns an ordered range of all the fragment entry versions where groupId = ? and fragmentCollectionId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching fragment entry versions
*/
public java.util.List findByG_FCI(
long groupId, long fragmentCollectionId, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator,
boolean useFinderCache);
/**
* Returns the first fragment entry version in the ordered set where groupId = ? and fragmentCollectionId = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry version
* @throws NoSuchEntryVersionException if a matching fragment entry version could not be found
*/
public FragmentEntryVersion findByG_FCI_First(
long groupId, long fragmentCollectionId,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Returns the first fragment entry version in the ordered set where groupId = ? and fragmentCollectionId = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry version, or null
if a matching fragment entry version could not be found
*/
public FragmentEntryVersion fetchByG_FCI_First(
long groupId, long fragmentCollectionId,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the last fragment entry version in the ordered set where groupId = ? and fragmentCollectionId = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry version
* @throws NoSuchEntryVersionException if a matching fragment entry version could not be found
*/
public FragmentEntryVersion findByG_FCI_Last(
long groupId, long fragmentCollectionId,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Returns the last fragment entry version in the ordered set where groupId = ? and fragmentCollectionId = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry version, or null
if a matching fragment entry version could not be found
*/
public FragmentEntryVersion fetchByG_FCI_Last(
long groupId, long fragmentCollectionId,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the fragment entry versions before and after the current fragment entry version in the ordered set where groupId = ? and fragmentCollectionId = ?.
*
* @param fragmentEntryVersionId the primary key of the current fragment entry version
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next fragment entry version
* @throws NoSuchEntryVersionException if a fragment entry version with the primary key could not be found
*/
public FragmentEntryVersion[] findByG_FCI_PrevAndNext(
long fragmentEntryVersionId, long groupId,
long fragmentCollectionId,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Removes all the fragment entry versions where groupId = ? and fragmentCollectionId = ? from the database.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
*/
public void removeByG_FCI(long groupId, long fragmentCollectionId);
/**
* Returns the number of fragment entry versions where groupId = ? and fragmentCollectionId = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @return the number of matching fragment entry versions
*/
public int countByG_FCI(long groupId, long fragmentCollectionId);
/**
* Returns all the fragment entry versions where groupId = ? and fragmentCollectionId = ? and version = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param version the version
* @return the matching fragment entry versions
*/
public java.util.List findByG_FCI_Version(
long groupId, long fragmentCollectionId, int version);
/**
* Returns a range of all the fragment entry versions where groupId = ? and fragmentCollectionId = ? and version = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param version the version
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @return the range of matching fragment entry versions
*/
public java.util.List findByG_FCI_Version(
long groupId, long fragmentCollectionId, int version, int start,
int end);
/**
* Returns an ordered range of all the fragment entry versions where groupId = ? and fragmentCollectionId = ? and version = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param version the version
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entry versions
*/
public java.util.List findByG_FCI_Version(
long groupId, long fragmentCollectionId, int version, int start,
int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns an ordered range of all the fragment entry versions where groupId = ? and fragmentCollectionId = ? and version = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param version the version
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching fragment entry versions
*/
public java.util.List findByG_FCI_Version(
long groupId, long fragmentCollectionId, int version, int start,
int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator,
boolean useFinderCache);
/**
* Returns the first fragment entry version in the ordered set where groupId = ? and fragmentCollectionId = ? and version = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param version the version
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry version
* @throws NoSuchEntryVersionException if a matching fragment entry version could not be found
*/
public FragmentEntryVersion findByG_FCI_Version_First(
long groupId, long fragmentCollectionId, int version,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Returns the first fragment entry version in the ordered set where groupId = ? and fragmentCollectionId = ? and version = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param version the version
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry version, or null
if a matching fragment entry version could not be found
*/
public FragmentEntryVersion fetchByG_FCI_Version_First(
long groupId, long fragmentCollectionId, int version,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the last fragment entry version in the ordered set where groupId = ? and fragmentCollectionId = ? and version = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param version the version
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry version
* @throws NoSuchEntryVersionException if a matching fragment entry version could not be found
*/
public FragmentEntryVersion findByG_FCI_Version_Last(
long groupId, long fragmentCollectionId, int version,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Returns the last fragment entry version in the ordered set where groupId = ? and fragmentCollectionId = ? and version = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param version the version
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry version, or null
if a matching fragment entry version could not be found
*/
public FragmentEntryVersion fetchByG_FCI_Version_Last(
long groupId, long fragmentCollectionId, int version,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the fragment entry versions before and after the current fragment entry version in the ordered set where groupId = ? and fragmentCollectionId = ? and version = ?.
*
* @param fragmentEntryVersionId the primary key of the current fragment entry version
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param version the version
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next fragment entry version
* @throws NoSuchEntryVersionException if a fragment entry version with the primary key could not be found
*/
public FragmentEntryVersion[] findByG_FCI_Version_PrevAndNext(
long fragmentEntryVersionId, long groupId,
long fragmentCollectionId, int version,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Removes all the fragment entry versions where groupId = ? and fragmentCollectionId = ? and version = ? from the database.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param version the version
*/
public void removeByG_FCI_Version(
long groupId, long fragmentCollectionId, int version);
/**
* Returns the number of fragment entry versions where groupId = ? and fragmentCollectionId = ? and version = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param version the version
* @return the number of matching fragment entry versions
*/
public int countByG_FCI_Version(
long groupId, long fragmentCollectionId, int version);
/**
* Returns all the fragment entry versions where groupId = ? and fragmentEntryKey = ?.
*
* @param groupId the group ID
* @param fragmentEntryKey the fragment entry key
* @return the matching fragment entry versions
*/
public java.util.List findByG_FEK(
long groupId, String fragmentEntryKey);
/**
* Returns a range of all the fragment entry versions where groupId = ? and fragmentEntryKey = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentEntryKey the fragment entry key
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @return the range of matching fragment entry versions
*/
public java.util.List findByG_FEK(
long groupId, String fragmentEntryKey, int start, int end);
/**
* Returns an ordered range of all the fragment entry versions where groupId = ? and fragmentEntryKey = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentEntryKey the fragment entry key
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entry versions
*/
public java.util.List findByG_FEK(
long groupId, String fragmentEntryKey, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns an ordered range of all the fragment entry versions where groupId = ? and fragmentEntryKey = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentEntryKey the fragment entry key
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching fragment entry versions
*/
public java.util.List findByG_FEK(
long groupId, String fragmentEntryKey, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator,
boolean useFinderCache);
/**
* Returns the first fragment entry version in the ordered set where groupId = ? and fragmentEntryKey = ?.
*
* @param groupId the group ID
* @param fragmentEntryKey the fragment entry key
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry version
* @throws NoSuchEntryVersionException if a matching fragment entry version could not be found
*/
public FragmentEntryVersion findByG_FEK_First(
long groupId, String fragmentEntryKey,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Returns the first fragment entry version in the ordered set where groupId = ? and fragmentEntryKey = ?.
*
* @param groupId the group ID
* @param fragmentEntryKey the fragment entry key
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry version, or null
if a matching fragment entry version could not be found
*/
public FragmentEntryVersion fetchByG_FEK_First(
long groupId, String fragmentEntryKey,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the last fragment entry version in the ordered set where groupId = ? and fragmentEntryKey = ?.
*
* @param groupId the group ID
* @param fragmentEntryKey the fragment entry key
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry version
* @throws NoSuchEntryVersionException if a matching fragment entry version could not be found
*/
public FragmentEntryVersion findByG_FEK_Last(
long groupId, String fragmentEntryKey,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Returns the last fragment entry version in the ordered set where groupId = ? and fragmentEntryKey = ?.
*
* @param groupId the group ID
* @param fragmentEntryKey the fragment entry key
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry version, or null
if a matching fragment entry version could not be found
*/
public FragmentEntryVersion fetchByG_FEK_Last(
long groupId, String fragmentEntryKey,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the fragment entry versions before and after the current fragment entry version in the ordered set where groupId = ? and fragmentEntryKey = ?.
*
* @param fragmentEntryVersionId the primary key of the current fragment entry version
* @param groupId the group ID
* @param fragmentEntryKey the fragment entry key
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next fragment entry version
* @throws NoSuchEntryVersionException if a fragment entry version with the primary key could not be found
*/
public FragmentEntryVersion[] findByG_FEK_PrevAndNext(
long fragmentEntryVersionId, long groupId, String fragmentEntryKey,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Removes all the fragment entry versions where groupId = ? and fragmentEntryKey = ? from the database.
*
* @param groupId the group ID
* @param fragmentEntryKey the fragment entry key
*/
public void removeByG_FEK(long groupId, String fragmentEntryKey);
/**
* Returns the number of fragment entry versions where groupId = ? and fragmentEntryKey = ?.
*
* @param groupId the group ID
* @param fragmentEntryKey the fragment entry key
* @return the number of matching fragment entry versions
*/
public int countByG_FEK(long groupId, String fragmentEntryKey);
/**
* Returns the fragment entry version where groupId = ? and fragmentEntryKey = ? and version = ? or throws a NoSuchEntryVersionException
if it could not be found.
*
* @param groupId the group ID
* @param fragmentEntryKey the fragment entry key
* @param version the version
* @return the matching fragment entry version
* @throws NoSuchEntryVersionException if a matching fragment entry version could not be found
*/
public FragmentEntryVersion findByG_FEK_Version(
long groupId, String fragmentEntryKey, int version)
throws NoSuchEntryVersionException;
/**
* Returns the fragment entry version where groupId = ? and fragmentEntryKey = ? and version = ? or returns null
if it could not be found. Uses the finder cache.
*
* @param groupId the group ID
* @param fragmentEntryKey the fragment entry key
* @param version the version
* @return the matching fragment entry version, or null
if a matching fragment entry version could not be found
*/
public FragmentEntryVersion fetchByG_FEK_Version(
long groupId, String fragmentEntryKey, int version);
/**
* Returns the fragment entry version where groupId = ? and fragmentEntryKey = ? and version = ? or returns null
if it could not be found, optionally using the finder cache.
*
* @param groupId the group ID
* @param fragmentEntryKey the fragment entry key
* @param version the version
* @param useFinderCache whether to use the finder cache
* @return the matching fragment entry version, or null
if a matching fragment entry version could not be found
*/
public FragmentEntryVersion fetchByG_FEK_Version(
long groupId, String fragmentEntryKey, int version,
boolean useFinderCache);
/**
* Removes the fragment entry version where groupId = ? and fragmentEntryKey = ? and version = ? from the database.
*
* @param groupId the group ID
* @param fragmentEntryKey the fragment entry key
* @param version the version
* @return the fragment entry version that was removed
*/
public FragmentEntryVersion removeByG_FEK_Version(
long groupId, String fragmentEntryKey, int version)
throws NoSuchEntryVersionException;
/**
* Returns the number of fragment entry versions where groupId = ? and fragmentEntryKey = ? and version = ?.
*
* @param groupId the group ID
* @param fragmentEntryKey the fragment entry key
* @param version the version
* @return the number of matching fragment entry versions
*/
public int countByG_FEK_Version(
long groupId, String fragmentEntryKey, int version);
/**
* Returns all the fragment entry versions where groupId = ? and fragmentCollectionId = ? and name = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @return the matching fragment entry versions
*/
public java.util.List findByG_FCI_LikeN(
long groupId, long fragmentCollectionId, String name);
/**
* Returns a range of all the fragment entry versions where groupId = ? and fragmentCollectionId = ? and name = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @return the range of matching fragment entry versions
*/
public java.util.List findByG_FCI_LikeN(
long groupId, long fragmentCollectionId, String name, int start,
int end);
/**
* Returns an ordered range of all the fragment entry versions where groupId = ? and fragmentCollectionId = ? and name = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entry versions
*/
public java.util.List findByG_FCI_LikeN(
long groupId, long fragmentCollectionId, String name, int start,
int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns an ordered range of all the fragment entry versions where groupId = ? and fragmentCollectionId = ? and name = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching fragment entry versions
*/
public java.util.List findByG_FCI_LikeN(
long groupId, long fragmentCollectionId, String name, int start,
int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator,
boolean useFinderCache);
/**
* Returns the first fragment entry version in the ordered set where groupId = ? and fragmentCollectionId = ? and name = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry version
* @throws NoSuchEntryVersionException if a matching fragment entry version could not be found
*/
public FragmentEntryVersion findByG_FCI_LikeN_First(
long groupId, long fragmentCollectionId, String name,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Returns the first fragment entry version in the ordered set where groupId = ? and fragmentCollectionId = ? and name = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry version, or null
if a matching fragment entry version could not be found
*/
public FragmentEntryVersion fetchByG_FCI_LikeN_First(
long groupId, long fragmentCollectionId, String name,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the last fragment entry version in the ordered set where groupId = ? and fragmentCollectionId = ? and name = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry version
* @throws NoSuchEntryVersionException if a matching fragment entry version could not be found
*/
public FragmentEntryVersion findByG_FCI_LikeN_Last(
long groupId, long fragmentCollectionId, String name,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Returns the last fragment entry version in the ordered set where groupId = ? and fragmentCollectionId = ? and name = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry version, or null
if a matching fragment entry version could not be found
*/
public FragmentEntryVersion fetchByG_FCI_LikeN_Last(
long groupId, long fragmentCollectionId, String name,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the fragment entry versions before and after the current fragment entry version in the ordered set where groupId = ? and fragmentCollectionId = ? and name = ?.
*
* @param fragmentEntryVersionId the primary key of the current fragment entry version
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next fragment entry version
* @throws NoSuchEntryVersionException if a fragment entry version with the primary key could not be found
*/
public FragmentEntryVersion[] findByG_FCI_LikeN_PrevAndNext(
long fragmentEntryVersionId, long groupId,
long fragmentCollectionId, String name,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Removes all the fragment entry versions where groupId = ? and fragmentCollectionId = ? and name = ? from the database.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
*/
public void removeByG_FCI_LikeN(
long groupId, long fragmentCollectionId, String name);
/**
* Returns the number of fragment entry versions where groupId = ? and fragmentCollectionId = ? and name = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @return the number of matching fragment entry versions
*/
public int countByG_FCI_LikeN(
long groupId, long fragmentCollectionId, String name);
/**
* Returns all the fragment entry versions where groupId = ? and fragmentCollectionId = ? and name = ? and version = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @param version the version
* @return the matching fragment entry versions
*/
public java.util.List findByG_FCI_LikeN_Version(
long groupId, long fragmentCollectionId, String name, int version);
/**
* Returns a range of all the fragment entry versions where groupId = ? and fragmentCollectionId = ? and name = ? and version = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @param version the version
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @return the range of matching fragment entry versions
*/
public java.util.List findByG_FCI_LikeN_Version(
long groupId, long fragmentCollectionId, String name, int version,
int start, int end);
/**
* Returns an ordered range of all the fragment entry versions where groupId = ? and fragmentCollectionId = ? and name = ? and version = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @param version the version
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entry versions
*/
public java.util.List findByG_FCI_LikeN_Version(
long groupId, long fragmentCollectionId, String name, int version,
int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns an ordered range of all the fragment entry versions where groupId = ? and fragmentCollectionId = ? and name = ? and version = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @param version the version
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching fragment entry versions
*/
public java.util.List findByG_FCI_LikeN_Version(
long groupId, long fragmentCollectionId, String name, int version,
int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator,
boolean useFinderCache);
/**
* Returns the first fragment entry version in the ordered set where groupId = ? and fragmentCollectionId = ? and name = ? and version = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @param version the version
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry version
* @throws NoSuchEntryVersionException if a matching fragment entry version could not be found
*/
public FragmentEntryVersion findByG_FCI_LikeN_Version_First(
long groupId, long fragmentCollectionId, String name, int version,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Returns the first fragment entry version in the ordered set where groupId = ? and fragmentCollectionId = ? and name = ? and version = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @param version the version
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry version, or null
if a matching fragment entry version could not be found
*/
public FragmentEntryVersion fetchByG_FCI_LikeN_Version_First(
long groupId, long fragmentCollectionId, String name, int version,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the last fragment entry version in the ordered set where groupId = ? and fragmentCollectionId = ? and name = ? and version = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @param version the version
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry version
* @throws NoSuchEntryVersionException if a matching fragment entry version could not be found
*/
public FragmentEntryVersion findByG_FCI_LikeN_Version_Last(
long groupId, long fragmentCollectionId, String name, int version,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Returns the last fragment entry version in the ordered set where groupId = ? and fragmentCollectionId = ? and name = ? and version = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @param version the version
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry version, or null
if a matching fragment entry version could not be found
*/
public FragmentEntryVersion fetchByG_FCI_LikeN_Version_Last(
long groupId, long fragmentCollectionId, String name, int version,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the fragment entry versions before and after the current fragment entry version in the ordered set where groupId = ? and fragmentCollectionId = ? and name = ? and version = ?.
*
* @param fragmentEntryVersionId the primary key of the current fragment entry version
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @param version the version
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next fragment entry version
* @throws NoSuchEntryVersionException if a fragment entry version with the primary key could not be found
*/
public FragmentEntryVersion[] findByG_FCI_LikeN_Version_PrevAndNext(
long fragmentEntryVersionId, long groupId,
long fragmentCollectionId, String name, int version,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Removes all the fragment entry versions where groupId = ? and fragmentCollectionId = ? and name = ? and version = ? from the database.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @param version the version
*/
public void removeByG_FCI_LikeN_Version(
long groupId, long fragmentCollectionId, String name, int version);
/**
* Returns the number of fragment entry versions where groupId = ? and fragmentCollectionId = ? and name = ? and version = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @param version the version
* @return the number of matching fragment entry versions
*/
public int countByG_FCI_LikeN_Version(
long groupId, long fragmentCollectionId, String name, int version);
/**
* Returns all the fragment entry versions where groupId = ? and fragmentCollectionId = ? and type = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param type the type
* @return the matching fragment entry versions
*/
public java.util.List findByG_FCI_T(
long groupId, long fragmentCollectionId, int type);
/**
* Returns a range of all the fragment entry versions where groupId = ? and fragmentCollectionId = ? and type = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param type the type
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @return the range of matching fragment entry versions
*/
public java.util.List findByG_FCI_T(
long groupId, long fragmentCollectionId, int type, int start, int end);
/**
* Returns an ordered range of all the fragment entry versions where groupId = ? and fragmentCollectionId = ? and type = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param type the type
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entry versions
*/
public java.util.List findByG_FCI_T(
long groupId, long fragmentCollectionId, int type, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns an ordered range of all the fragment entry versions where groupId = ? and fragmentCollectionId = ? and type = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param type the type
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching fragment entry versions
*/
public java.util.List findByG_FCI_T(
long groupId, long fragmentCollectionId, int type, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator,
boolean useFinderCache);
/**
* Returns the first fragment entry version in the ordered set where groupId = ? and fragmentCollectionId = ? and type = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param type the type
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry version
* @throws NoSuchEntryVersionException if a matching fragment entry version could not be found
*/
public FragmentEntryVersion findByG_FCI_T_First(
long groupId, long fragmentCollectionId, int type,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Returns the first fragment entry version in the ordered set where groupId = ? and fragmentCollectionId = ? and type = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param type the type
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry version, or null
if a matching fragment entry version could not be found
*/
public FragmentEntryVersion fetchByG_FCI_T_First(
long groupId, long fragmentCollectionId, int type,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the last fragment entry version in the ordered set where groupId = ? and fragmentCollectionId = ? and type = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param type the type
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry version
* @throws NoSuchEntryVersionException if a matching fragment entry version could not be found
*/
public FragmentEntryVersion findByG_FCI_T_Last(
long groupId, long fragmentCollectionId, int type,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Returns the last fragment entry version in the ordered set where groupId = ? and fragmentCollectionId = ? and type = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param type the type
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry version, or null
if a matching fragment entry version could not be found
*/
public FragmentEntryVersion fetchByG_FCI_T_Last(
long groupId, long fragmentCollectionId, int type,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the fragment entry versions before and after the current fragment entry version in the ordered set where groupId = ? and fragmentCollectionId = ? and type = ?.
*
* @param fragmentEntryVersionId the primary key of the current fragment entry version
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param type the type
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next fragment entry version
* @throws NoSuchEntryVersionException if a fragment entry version with the primary key could not be found
*/
public FragmentEntryVersion[] findByG_FCI_T_PrevAndNext(
long fragmentEntryVersionId, long groupId,
long fragmentCollectionId, int type,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Removes all the fragment entry versions where groupId = ? and fragmentCollectionId = ? and type = ? from the database.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param type the type
*/
public void removeByG_FCI_T(
long groupId, long fragmentCollectionId, int type);
/**
* Returns the number of fragment entry versions where groupId = ? and fragmentCollectionId = ? and type = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param type the type
* @return the number of matching fragment entry versions
*/
public int countByG_FCI_T(
long groupId, long fragmentCollectionId, int type);
/**
* Returns all the fragment entry versions where groupId = ? and fragmentCollectionId = ? and type = ? and version = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param type the type
* @param version the version
* @return the matching fragment entry versions
*/
public java.util.List findByG_FCI_T_Version(
long groupId, long fragmentCollectionId, int type, int version);
/**
* Returns a range of all the fragment entry versions where groupId = ? and fragmentCollectionId = ? and type = ? and version = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param type the type
* @param version the version
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @return the range of matching fragment entry versions
*/
public java.util.List findByG_FCI_T_Version(
long groupId, long fragmentCollectionId, int type, int version,
int start, int end);
/**
* Returns an ordered range of all the fragment entry versions where groupId = ? and fragmentCollectionId = ? and type = ? and version = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param type the type
* @param version the version
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entry versions
*/
public java.util.List findByG_FCI_T_Version(
long groupId, long fragmentCollectionId, int type, int version,
int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns an ordered range of all the fragment entry versions where groupId = ? and fragmentCollectionId = ? and type = ? and version = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param type the type
* @param version the version
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching fragment entry versions
*/
public java.util.List findByG_FCI_T_Version(
long groupId, long fragmentCollectionId, int type, int version,
int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator,
boolean useFinderCache);
/**
* Returns the first fragment entry version in the ordered set where groupId = ? and fragmentCollectionId = ? and type = ? and version = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param type the type
* @param version the version
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry version
* @throws NoSuchEntryVersionException if a matching fragment entry version could not be found
*/
public FragmentEntryVersion findByG_FCI_T_Version_First(
long groupId, long fragmentCollectionId, int type, int version,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Returns the first fragment entry version in the ordered set where groupId = ? and fragmentCollectionId = ? and type = ? and version = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param type the type
* @param version the version
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry version, or null
if a matching fragment entry version could not be found
*/
public FragmentEntryVersion fetchByG_FCI_T_Version_First(
long groupId, long fragmentCollectionId, int type, int version,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the last fragment entry version in the ordered set where groupId = ? and fragmentCollectionId = ? and type = ? and version = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param type the type
* @param version the version
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry version
* @throws NoSuchEntryVersionException if a matching fragment entry version could not be found
*/
public FragmentEntryVersion findByG_FCI_T_Version_Last(
long groupId, long fragmentCollectionId, int type, int version,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Returns the last fragment entry version in the ordered set where groupId = ? and fragmentCollectionId = ? and type = ? and version = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param type the type
* @param version the version
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry version, or null
if a matching fragment entry version could not be found
*/
public FragmentEntryVersion fetchByG_FCI_T_Version_Last(
long groupId, long fragmentCollectionId, int type, int version,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the fragment entry versions before and after the current fragment entry version in the ordered set where groupId = ? and fragmentCollectionId = ? and type = ? and version = ?.
*
* @param fragmentEntryVersionId the primary key of the current fragment entry version
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param type the type
* @param version the version
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next fragment entry version
* @throws NoSuchEntryVersionException if a fragment entry version with the primary key could not be found
*/
public FragmentEntryVersion[] findByG_FCI_T_Version_PrevAndNext(
long fragmentEntryVersionId, long groupId,
long fragmentCollectionId, int type, int version,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Removes all the fragment entry versions where groupId = ? and fragmentCollectionId = ? and type = ? and version = ? from the database.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param type the type
* @param version the version
*/
public void removeByG_FCI_T_Version(
long groupId, long fragmentCollectionId, int type, int version);
/**
* Returns the number of fragment entry versions where groupId = ? and fragmentCollectionId = ? and type = ? and version = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param type the type
* @param version the version
* @return the number of matching fragment entry versions
*/
public int countByG_FCI_T_Version(
long groupId, long fragmentCollectionId, int type, int version);
/**
* Returns all the fragment entry versions where groupId = ? and fragmentCollectionId = ? and status = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param status the status
* @return the matching fragment entry versions
*/
public java.util.List findByG_FCI_S(
long groupId, long fragmentCollectionId, int status);
/**
* Returns a range of all the fragment entry versions where groupId = ? and fragmentCollectionId = ? and status = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param status the status
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @return the range of matching fragment entry versions
*/
public java.util.List findByG_FCI_S(
long groupId, long fragmentCollectionId, int status, int start,
int end);
/**
* Returns an ordered range of all the fragment entry versions where groupId = ? and fragmentCollectionId = ? and status = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param status the status
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entry versions
*/
public java.util.List findByG_FCI_S(
long groupId, long fragmentCollectionId, int status, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns an ordered range of all the fragment entry versions where groupId = ? and fragmentCollectionId = ? and status = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param status the status
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching fragment entry versions
*/
public java.util.List findByG_FCI_S(
long groupId, long fragmentCollectionId, int status, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator,
boolean useFinderCache);
/**
* Returns the first fragment entry version in the ordered set where groupId = ? and fragmentCollectionId = ? and status = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param status the status
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry version
* @throws NoSuchEntryVersionException if a matching fragment entry version could not be found
*/
public FragmentEntryVersion findByG_FCI_S_First(
long groupId, long fragmentCollectionId, int status,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Returns the first fragment entry version in the ordered set where groupId = ? and fragmentCollectionId = ? and status = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param status the status
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry version, or null
if a matching fragment entry version could not be found
*/
public FragmentEntryVersion fetchByG_FCI_S_First(
long groupId, long fragmentCollectionId, int status,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the last fragment entry version in the ordered set where groupId = ? and fragmentCollectionId = ? and status = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param status the status
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry version
* @throws NoSuchEntryVersionException if a matching fragment entry version could not be found
*/
public FragmentEntryVersion findByG_FCI_S_Last(
long groupId, long fragmentCollectionId, int status,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Returns the last fragment entry version in the ordered set where groupId = ? and fragmentCollectionId = ? and status = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param status the status
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry version, or null
if a matching fragment entry version could not be found
*/
public FragmentEntryVersion fetchByG_FCI_S_Last(
long groupId, long fragmentCollectionId, int status,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the fragment entry versions before and after the current fragment entry version in the ordered set where groupId = ? and fragmentCollectionId = ? and status = ?.
*
* @param fragmentEntryVersionId the primary key of the current fragment entry version
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param status the status
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next fragment entry version
* @throws NoSuchEntryVersionException if a fragment entry version with the primary key could not be found
*/
public FragmentEntryVersion[] findByG_FCI_S_PrevAndNext(
long fragmentEntryVersionId, long groupId,
long fragmentCollectionId, int status,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Removes all the fragment entry versions where groupId = ? and fragmentCollectionId = ? and status = ? from the database.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param status the status
*/
public void removeByG_FCI_S(
long groupId, long fragmentCollectionId, int status);
/**
* Returns the number of fragment entry versions where groupId = ? and fragmentCollectionId = ? and status = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param status the status
* @return the number of matching fragment entry versions
*/
public int countByG_FCI_S(
long groupId, long fragmentCollectionId, int status);
/**
* Returns all the fragment entry versions where groupId = ? and fragmentCollectionId = ? and status = ? and version = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param status the status
* @param version the version
* @return the matching fragment entry versions
*/
public java.util.List findByG_FCI_S_Version(
long groupId, long fragmentCollectionId, int status, int version);
/**
* Returns a range of all the fragment entry versions where groupId = ? and fragmentCollectionId = ? and status = ? and version = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param status the status
* @param version the version
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @return the range of matching fragment entry versions
*/
public java.util.List findByG_FCI_S_Version(
long groupId, long fragmentCollectionId, int status, int version,
int start, int end);
/**
* Returns an ordered range of all the fragment entry versions where groupId = ? and fragmentCollectionId = ? and status = ? and version = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param status the status
* @param version the version
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entry versions
*/
public java.util.List findByG_FCI_S_Version(
long groupId, long fragmentCollectionId, int status, int version,
int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns an ordered range of all the fragment entry versions where groupId = ? and fragmentCollectionId = ? and status = ? and version = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param status the status
* @param version the version
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching fragment entry versions
*/
public java.util.List findByG_FCI_S_Version(
long groupId, long fragmentCollectionId, int status, int version,
int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator,
boolean useFinderCache);
/**
* Returns the first fragment entry version in the ordered set where groupId = ? and fragmentCollectionId = ? and status = ? and version = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param status the status
* @param version the version
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry version
* @throws NoSuchEntryVersionException if a matching fragment entry version could not be found
*/
public FragmentEntryVersion findByG_FCI_S_Version_First(
long groupId, long fragmentCollectionId, int status, int version,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Returns the first fragment entry version in the ordered set where groupId = ? and fragmentCollectionId = ? and status = ? and version = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param status the status
* @param version the version
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry version, or null
if a matching fragment entry version could not be found
*/
public FragmentEntryVersion fetchByG_FCI_S_Version_First(
long groupId, long fragmentCollectionId, int status, int version,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the last fragment entry version in the ordered set where groupId = ? and fragmentCollectionId = ? and status = ? and version = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param status the status
* @param version the version
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry version
* @throws NoSuchEntryVersionException if a matching fragment entry version could not be found
*/
public FragmentEntryVersion findByG_FCI_S_Version_Last(
long groupId, long fragmentCollectionId, int status, int version,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Returns the last fragment entry version in the ordered set where groupId = ? and fragmentCollectionId = ? and status = ? and version = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param status the status
* @param version the version
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry version, or null
if a matching fragment entry version could not be found
*/
public FragmentEntryVersion fetchByG_FCI_S_Version_Last(
long groupId, long fragmentCollectionId, int status, int version,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the fragment entry versions before and after the current fragment entry version in the ordered set where groupId = ? and fragmentCollectionId = ? and status = ? and version = ?.
*
* @param fragmentEntryVersionId the primary key of the current fragment entry version
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param status the status
* @param version the version
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next fragment entry version
* @throws NoSuchEntryVersionException if a fragment entry version with the primary key could not be found
*/
public FragmentEntryVersion[] findByG_FCI_S_Version_PrevAndNext(
long fragmentEntryVersionId, long groupId,
long fragmentCollectionId, int status, int version,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Removes all the fragment entry versions where groupId = ? and fragmentCollectionId = ? and status = ? and version = ? from the database.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param status the status
* @param version the version
*/
public void removeByG_FCI_S_Version(
long groupId, long fragmentCollectionId, int status, int version);
/**
* Returns the number of fragment entry versions where groupId = ? and fragmentCollectionId = ? and status = ? and version = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param status the status
* @param version the version
* @return the number of matching fragment entry versions
*/
public int countByG_FCI_S_Version(
long groupId, long fragmentCollectionId, int status, int version);
/**
* Returns all the fragment entry versions where groupId = ? and fragmentCollectionId = ? and name = ? and status = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @param status the status
* @return the matching fragment entry versions
*/
public java.util.List findByG_FCI_LikeN_S(
long groupId, long fragmentCollectionId, String name, int status);
/**
* Returns a range of all the fragment entry versions where groupId = ? and fragmentCollectionId = ? and name = ? and status = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @param status the status
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @return the range of matching fragment entry versions
*/
public java.util.List findByG_FCI_LikeN_S(
long groupId, long fragmentCollectionId, String name, int status,
int start, int end);
/**
* Returns an ordered range of all the fragment entry versions where groupId = ? and fragmentCollectionId = ? and name = ? and status = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @param status the status
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entry versions
*/
public java.util.List findByG_FCI_LikeN_S(
long groupId, long fragmentCollectionId, String name, int status,
int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns an ordered range of all the fragment entry versions where groupId = ? and fragmentCollectionId = ? and name = ? and status = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @param status the status
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching fragment entry versions
*/
public java.util.List findByG_FCI_LikeN_S(
long groupId, long fragmentCollectionId, String name, int status,
int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator,
boolean useFinderCache);
/**
* Returns the first fragment entry version in the ordered set where groupId = ? and fragmentCollectionId = ? and name = ? and status = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @param status the status
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry version
* @throws NoSuchEntryVersionException if a matching fragment entry version could not be found
*/
public FragmentEntryVersion findByG_FCI_LikeN_S_First(
long groupId, long fragmentCollectionId, String name, int status,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Returns the first fragment entry version in the ordered set where groupId = ? and fragmentCollectionId = ? and name = ? and status = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @param status the status
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry version, or null
if a matching fragment entry version could not be found
*/
public FragmentEntryVersion fetchByG_FCI_LikeN_S_First(
long groupId, long fragmentCollectionId, String name, int status,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the last fragment entry version in the ordered set where groupId = ? and fragmentCollectionId = ? and name = ? and status = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @param status the status
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry version
* @throws NoSuchEntryVersionException if a matching fragment entry version could not be found
*/
public FragmentEntryVersion findByG_FCI_LikeN_S_Last(
long groupId, long fragmentCollectionId, String name, int status,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Returns the last fragment entry version in the ordered set where groupId = ? and fragmentCollectionId = ? and name = ? and status = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @param status the status
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry version, or null
if a matching fragment entry version could not be found
*/
public FragmentEntryVersion fetchByG_FCI_LikeN_S_Last(
long groupId, long fragmentCollectionId, String name, int status,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the fragment entry versions before and after the current fragment entry version in the ordered set where groupId = ? and fragmentCollectionId = ? and name = ? and status = ?.
*
* @param fragmentEntryVersionId the primary key of the current fragment entry version
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @param status the status
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next fragment entry version
* @throws NoSuchEntryVersionException if a fragment entry version with the primary key could not be found
*/
public FragmentEntryVersion[] findByG_FCI_LikeN_S_PrevAndNext(
long fragmentEntryVersionId, long groupId,
long fragmentCollectionId, String name, int status,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryVersionException;
/**
* Removes all the fragment entry versions where groupId = ? and fragmentCollectionId = ? and name = ? and status = ? from the database.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @param status the status
*/
public void removeByG_FCI_LikeN_S(
long groupId, long fragmentCollectionId, String name, int status);
/**
* Returns the number of fragment entry versions where groupId = ? and fragmentCollectionId = ? and name = ? and status = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @param status the status
* @return the number of matching fragment entry versions
*/
public int countByG_FCI_LikeN_S(
long groupId, long fragmentCollectionId, String name, int status);
/**
* Returns all the fragment entry versions where groupId = ? and fragmentCollectionId = ? and name = ? and status = ? and version = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @param status the status
* @param version the version
* @return the matching fragment entry versions
*/
public java.util.List findByG_FCI_LikeN_S_Version(
long groupId, long fragmentCollectionId, String name, int status,
int version);
/**
* Returns a range of all the fragment entry versions where groupId = ? and fragmentCollectionId = ? and name = ? and status = ? and version = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @param status the status
* @param version the version
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @return the range of matching fragment entry versions
*/
public java.util.List findByG_FCI_LikeN_S_Version(
long groupId, long fragmentCollectionId, String name, int status,
int version, int start, int end);
/**
* Returns an ordered range of all the fragment entry versions where groupId = ? and fragmentCollectionId = ? and name = ? and status = ? and version = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @param status the status
* @param version the version
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entry versions
*/
public java.util.List findByG_FCI_LikeN_S_Version(
long groupId, long fragmentCollectionId, String name, int status,
int version, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns an ordered range of all the fragment entry versions where groupId = ? and fragmentCollectionId = ? and name = ? and status = ? and version = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryVersionModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @param status the status
* @param version the version
* @param start the lower bound of the range of fragment entry versions
* @param end the upper bound of the range of fragment entry versions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching fragment entry versions
*/
public java.util.List findByG_FCI_LikeN_S_Version(
long groupId, long fragmentCollectionId, String name, int status,
int version, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator,
boolean useFinderCache);
/**
* Returns the first fragment entry version in the ordered set where groupId = ? and fragmentCollectionId = ? and name = ? and status = ? and version = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @param status the status
* @param version the version
* @param orderByComparator the comparator to order the set by (optionally