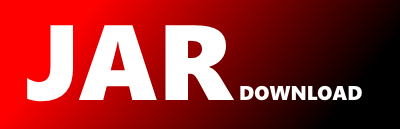
com.liferay.fragment.service.persistence.impl.FragmentEntryLinkPersistenceImpl Maven / Gradle / Ivy
/**
* SPDX-FileCopyrightText: (c) 2000 Liferay, Inc. https://liferay.com
* SPDX-License-Identifier: LGPL-2.1-or-later OR LicenseRef-Liferay-DXP-EULA-2.0.0-2023-06
*/
package com.liferay.fragment.service.persistence.impl;
import com.liferay.fragment.exception.DuplicateFragmentEntryLinkExternalReferenceCodeException;
import com.liferay.fragment.exception.NoSuchEntryLinkException;
import com.liferay.fragment.model.FragmentEntryLink;
import com.liferay.fragment.model.FragmentEntryLinkTable;
import com.liferay.fragment.model.impl.FragmentEntryLinkImpl;
import com.liferay.fragment.model.impl.FragmentEntryLinkModelImpl;
import com.liferay.fragment.service.persistence.FragmentEntryLinkPersistence;
import com.liferay.fragment.service.persistence.FragmentEntryLinkUtil;
import com.liferay.fragment.service.persistence.impl.constants.FragmentPersistenceConstants;
import com.liferay.petra.lang.SafeCloseable;
import com.liferay.petra.string.StringBundler;
import com.liferay.portal.kernel.change.tracking.CTCollectionThreadLocal;
import com.liferay.portal.kernel.change.tracking.CTColumnResolutionType;
import com.liferay.portal.kernel.configuration.Configuration;
import com.liferay.portal.kernel.dao.orm.EntityCache;
import com.liferay.portal.kernel.dao.orm.FinderCache;
import com.liferay.portal.kernel.dao.orm.FinderPath;
import com.liferay.portal.kernel.dao.orm.Query;
import com.liferay.portal.kernel.dao.orm.QueryPos;
import com.liferay.portal.kernel.dao.orm.QueryUtil;
import com.liferay.portal.kernel.dao.orm.Session;
import com.liferay.portal.kernel.dao.orm.SessionFactory;
import com.liferay.portal.kernel.exception.SystemException;
import com.liferay.portal.kernel.log.Log;
import com.liferay.portal.kernel.log.LogFactoryUtil;
import com.liferay.portal.kernel.sanitizer.Sanitizer;
import com.liferay.portal.kernel.sanitizer.SanitizerException;
import com.liferay.portal.kernel.sanitizer.SanitizerUtil;
import com.liferay.portal.kernel.security.auth.CompanyThreadLocal;
import com.liferay.portal.kernel.security.auth.PrincipalThreadLocal;
import com.liferay.portal.kernel.service.ServiceContext;
import com.liferay.portal.kernel.service.ServiceContextThreadLocal;
import com.liferay.portal.kernel.service.persistence.change.tracking.helper.CTPersistenceHelper;
import com.liferay.portal.kernel.service.persistence.impl.BasePersistenceImpl;
import com.liferay.portal.kernel.util.ArrayUtil;
import com.liferay.portal.kernel.util.ContentTypes;
import com.liferay.portal.kernel.util.GetterUtil;
import com.liferay.portal.kernel.util.OrderByComparator;
import com.liferay.portal.kernel.util.PropsKeys;
import com.liferay.portal.kernel.util.PropsUtil;
import com.liferay.portal.kernel.util.ProxyUtil;
import com.liferay.portal.kernel.util.SetUtil;
import com.liferay.portal.kernel.util.StringUtil;
import com.liferay.portal.kernel.util.Validator;
import com.liferay.portal.kernel.uuid.PortalUUIDUtil;
import java.io.Serializable;
import java.lang.reflect.InvocationHandler;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Date;
import java.util.EnumMap;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Set;
import javax.sql.DataSource;
import org.osgi.service.component.annotations.Activate;
import org.osgi.service.component.annotations.Component;
import org.osgi.service.component.annotations.Deactivate;
import org.osgi.service.component.annotations.Reference;
/**
* The persistence implementation for the fragment entry link service.
*
*
* Caching information and settings can be found in portal.properties
*
*
* @author Brian Wing Shun Chan
* @generated
*/
@Component(service = FragmentEntryLinkPersistence.class)
public class FragmentEntryLinkPersistenceImpl
extends BasePersistenceImpl
implements FragmentEntryLinkPersistence {
/*
* NOTE FOR DEVELOPERS:
*
* Never modify or reference this class directly. Always use FragmentEntryLinkUtil
to access the fragment entry link persistence. Modify service.xml
and rerun ServiceBuilder to regenerate this class.
*/
public static final String FINDER_CLASS_NAME_ENTITY =
FragmentEntryLinkImpl.class.getName();
public static final String FINDER_CLASS_NAME_LIST_WITH_PAGINATION =
FINDER_CLASS_NAME_ENTITY + ".List1";
public static final String FINDER_CLASS_NAME_LIST_WITHOUT_PAGINATION =
FINDER_CLASS_NAME_ENTITY + ".List2";
private FinderPath _finderPathWithPaginationFindAll;
private FinderPath _finderPathWithoutPaginationFindAll;
private FinderPath _finderPathCountAll;
private FinderPath _finderPathWithPaginationFindByUuid;
private FinderPath _finderPathWithoutPaginationFindByUuid;
private FinderPath _finderPathCountByUuid;
/**
* Returns all the fragment entry links where uuid = ?.
*
* @param uuid the uuid
* @return the matching fragment entry links
*/
@Override
public List findByUuid(String uuid) {
return findByUuid(uuid, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the fragment entry links where uuid = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryLinkModelImpl
.
*
*
* @param uuid the uuid
* @param start the lower bound of the range of fragment entry links
* @param end the upper bound of the range of fragment entry links (not inclusive)
* @return the range of matching fragment entry links
*/
@Override
public List findByUuid(String uuid, int start, int end) {
return findByUuid(uuid, start, end, null);
}
/**
* Returns an ordered range of all the fragment entry links where uuid = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryLinkModelImpl
.
*
*
* @param uuid the uuid
* @param start the lower bound of the range of fragment entry links
* @param end the upper bound of the range of fragment entry links (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entry links
*/
@Override
public List findByUuid(
String uuid, int start, int end,
OrderByComparator orderByComparator) {
return findByUuid(uuid, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the fragment entry links where uuid = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryLinkModelImpl
.
*
*
* @param uuid the uuid
* @param start the lower bound of the range of fragment entry links
* @param end the upper bound of the range of fragment entry links (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching fragment entry links
*/
@Override
public List findByUuid(
String uuid, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
try (SafeCloseable safeCloseable =
ctPersistenceHelper.setCTCollectionIdWithSafeCloseable(
FragmentEntryLink.class)) {
uuid = Objects.toString(uuid, "");
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache) {
finderPath = _finderPathWithoutPaginationFindByUuid;
finderArgs = new Object[] {uuid};
}
}
else if (useFinderCache) {
finderPath = _finderPathWithPaginationFindByUuid;
finderArgs = new Object[] {uuid, start, end, orderByComparator};
}
List list = null;
if (useFinderCache) {
list = (List)finderCache.getResult(
finderPath, finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (FragmentEntryLink fragmentEntryLink : list) {
if (!uuid.equals(fragmentEntryLink.getUuid())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
3 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(3);
}
sb.append(_SQL_SELECT_FRAGMENTENTRYLINK_WHERE);
boolean bindUuid = false;
if (uuid.isEmpty()) {
sb.append(_FINDER_COLUMN_UUID_UUID_3);
}
else {
bindUuid = true;
sb.append(_FINDER_COLUMN_UUID_UUID_2);
}
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(FragmentEntryLinkModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
if (bindUuid) {
queryPos.add(uuid);
}
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
}
/**
* Returns the first fragment entry link in the ordered set where uuid = ?.
*
* @param uuid the uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry link
* @throws NoSuchEntryLinkException if a matching fragment entry link could not be found
*/
@Override
public FragmentEntryLink findByUuid_First(
String uuid, OrderByComparator orderByComparator)
throws NoSuchEntryLinkException {
FragmentEntryLink fragmentEntryLink = fetchByUuid_First(
uuid, orderByComparator);
if (fragmentEntryLink != null) {
return fragmentEntryLink;
}
StringBundler sb = new StringBundler(4);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("uuid=");
sb.append(uuid);
sb.append("}");
throw new NoSuchEntryLinkException(sb.toString());
}
/**
* Returns the first fragment entry link in the ordered set where uuid = ?.
*
* @param uuid the uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry link, or null
if a matching fragment entry link could not be found
*/
@Override
public FragmentEntryLink fetchByUuid_First(
String uuid, OrderByComparator orderByComparator) {
List list = findByUuid(
uuid, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last fragment entry link in the ordered set where uuid = ?.
*
* @param uuid the uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry link
* @throws NoSuchEntryLinkException if a matching fragment entry link could not be found
*/
@Override
public FragmentEntryLink findByUuid_Last(
String uuid, OrderByComparator orderByComparator)
throws NoSuchEntryLinkException {
FragmentEntryLink fragmentEntryLink = fetchByUuid_Last(
uuid, orderByComparator);
if (fragmentEntryLink != null) {
return fragmentEntryLink;
}
StringBundler sb = new StringBundler(4);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("uuid=");
sb.append(uuid);
sb.append("}");
throw new NoSuchEntryLinkException(sb.toString());
}
/**
* Returns the last fragment entry link in the ordered set where uuid = ?.
*
* @param uuid the uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry link, or null
if a matching fragment entry link could not be found
*/
@Override
public FragmentEntryLink fetchByUuid_Last(
String uuid, OrderByComparator orderByComparator) {
int count = countByUuid(uuid);
if (count == 0) {
return null;
}
List list = findByUuid(
uuid, count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the fragment entry links before and after the current fragment entry link in the ordered set where uuid = ?.
*
* @param fragmentEntryLinkId the primary key of the current fragment entry link
* @param uuid the uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next fragment entry link
* @throws NoSuchEntryLinkException if a fragment entry link with the primary key could not be found
*/
@Override
public FragmentEntryLink[] findByUuid_PrevAndNext(
long fragmentEntryLinkId, String uuid,
OrderByComparator orderByComparator)
throws NoSuchEntryLinkException {
uuid = Objects.toString(uuid, "");
FragmentEntryLink fragmentEntryLink = findByPrimaryKey(
fragmentEntryLinkId);
Session session = null;
try {
session = openSession();
FragmentEntryLink[] array = new FragmentEntryLinkImpl[3];
array[0] = getByUuid_PrevAndNext(
session, fragmentEntryLink, uuid, orderByComparator, true);
array[1] = fragmentEntryLink;
array[2] = getByUuid_PrevAndNext(
session, fragmentEntryLink, uuid, orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected FragmentEntryLink getByUuid_PrevAndNext(
Session session, FragmentEntryLink fragmentEntryLink, String uuid,
OrderByComparator orderByComparator,
boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(3);
}
sb.append(_SQL_SELECT_FRAGMENTENTRYLINK_WHERE);
boolean bindUuid = false;
if (uuid.isEmpty()) {
sb.append(_FINDER_COLUMN_UUID_UUID_3);
}
else {
bindUuid = true;
sb.append(_FINDER_COLUMN_UUID_UUID_2);
}
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(FragmentEntryLinkModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
if (bindUuid) {
queryPos.add(uuid);
}
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
fragmentEntryLink)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the fragment entry links where uuid = ? from the database.
*
* @param uuid the uuid
*/
@Override
public void removeByUuid(String uuid) {
for (FragmentEntryLink fragmentEntryLink :
findByUuid(uuid, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null)) {
remove(fragmentEntryLink);
}
}
/**
* Returns the number of fragment entry links where uuid = ?.
*
* @param uuid the uuid
* @return the number of matching fragment entry links
*/
@Override
public int countByUuid(String uuid) {
try (SafeCloseable safeCloseable =
ctPersistenceHelper.setCTCollectionIdWithSafeCloseable(
FragmentEntryLink.class)) {
uuid = Objects.toString(uuid, "");
FinderPath finderPath = _finderPathCountByUuid;
Object[] finderArgs = new Object[] {uuid};
Long count = (Long)finderCache.getResult(
finderPath, finderArgs, this);
if (count == null) {
StringBundler sb = new StringBundler(2);
sb.append(_SQL_COUNT_FRAGMENTENTRYLINK_WHERE);
boolean bindUuid = false;
if (uuid.isEmpty()) {
sb.append(_FINDER_COLUMN_UUID_UUID_3);
}
else {
bindUuid = true;
sb.append(_FINDER_COLUMN_UUID_UUID_2);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
if (bindUuid) {
queryPos.add(uuid);
}
count = (Long)query.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
}
private static final String _FINDER_COLUMN_UUID_UUID_2 =
"fragmentEntryLink.uuid = ?";
private static final String _FINDER_COLUMN_UUID_UUID_3 =
"(fragmentEntryLink.uuid IS NULL OR fragmentEntryLink.uuid = '')";
private FinderPath _finderPathFetchByUUID_G;
private FinderPath _finderPathCountByUUID_G;
/**
* Returns the fragment entry link where uuid = ? and groupId = ? or throws a NoSuchEntryLinkException
if it could not be found.
*
* @param uuid the uuid
* @param groupId the group ID
* @return the matching fragment entry link
* @throws NoSuchEntryLinkException if a matching fragment entry link could not be found
*/
@Override
public FragmentEntryLink findByUUID_G(String uuid, long groupId)
throws NoSuchEntryLinkException {
FragmentEntryLink fragmentEntryLink = fetchByUUID_G(uuid, groupId);
if (fragmentEntryLink == null) {
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("uuid=");
sb.append(uuid);
sb.append(", groupId=");
sb.append(groupId);
sb.append("}");
if (_log.isDebugEnabled()) {
_log.debug(sb.toString());
}
throw new NoSuchEntryLinkException(sb.toString());
}
return fragmentEntryLink;
}
/**
* Returns the fragment entry link where uuid = ? and groupId = ? or returns null
if it could not be found. Uses the finder cache.
*
* @param uuid the uuid
* @param groupId the group ID
* @return the matching fragment entry link, or null
if a matching fragment entry link could not be found
*/
@Override
public FragmentEntryLink fetchByUUID_G(String uuid, long groupId) {
return fetchByUUID_G(uuid, groupId, true);
}
/**
* Returns the fragment entry link where uuid = ? and groupId = ? or returns null
if it could not be found, optionally using the finder cache.
*
* @param uuid the uuid
* @param groupId the group ID
* @param useFinderCache whether to use the finder cache
* @return the matching fragment entry link, or null
if a matching fragment entry link could not be found
*/
@Override
public FragmentEntryLink fetchByUUID_G(
String uuid, long groupId, boolean useFinderCache) {
try (SafeCloseable safeCloseable =
ctPersistenceHelper.setCTCollectionIdWithSafeCloseable(
FragmentEntryLink.class)) {
uuid = Objects.toString(uuid, "");
Object[] finderArgs = null;
if (useFinderCache) {
finderArgs = new Object[] {uuid, groupId};
}
Object result = null;
if (useFinderCache) {
result = finderCache.getResult(
_finderPathFetchByUUID_G, finderArgs, this);
}
if (result instanceof FragmentEntryLink) {
FragmentEntryLink fragmentEntryLink = (FragmentEntryLink)result;
if (!Objects.equals(uuid, fragmentEntryLink.getUuid()) ||
(groupId != fragmentEntryLink.getGroupId())) {
result = null;
}
}
if (result == null) {
StringBundler sb = new StringBundler(4);
sb.append(_SQL_SELECT_FRAGMENTENTRYLINK_WHERE);
boolean bindUuid = false;
if (uuid.isEmpty()) {
sb.append(_FINDER_COLUMN_UUID_G_UUID_3);
}
else {
bindUuid = true;
sb.append(_FINDER_COLUMN_UUID_G_UUID_2);
}
sb.append(_FINDER_COLUMN_UUID_G_GROUPID_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
if (bindUuid) {
queryPos.add(uuid);
}
queryPos.add(groupId);
List list = query.list();
if (list.isEmpty()) {
if (useFinderCache) {
finderCache.putResult(
_finderPathFetchByUUID_G, finderArgs, list);
}
}
else {
FragmentEntryLink fragmentEntryLink = list.get(0);
result = fragmentEntryLink;
cacheResult(fragmentEntryLink);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
if (result instanceof List>) {
return null;
}
else {
return (FragmentEntryLink)result;
}
}
}
/**
* Removes the fragment entry link where uuid = ? and groupId = ? from the database.
*
* @param uuid the uuid
* @param groupId the group ID
* @return the fragment entry link that was removed
*/
@Override
public FragmentEntryLink removeByUUID_G(String uuid, long groupId)
throws NoSuchEntryLinkException {
FragmentEntryLink fragmentEntryLink = findByUUID_G(uuid, groupId);
return remove(fragmentEntryLink);
}
/**
* Returns the number of fragment entry links where uuid = ? and groupId = ?.
*
* @param uuid the uuid
* @param groupId the group ID
* @return the number of matching fragment entry links
*/
@Override
public int countByUUID_G(String uuid, long groupId) {
try (SafeCloseable safeCloseable =
ctPersistenceHelper.setCTCollectionIdWithSafeCloseable(
FragmentEntryLink.class)) {
uuid = Objects.toString(uuid, "");
FinderPath finderPath = _finderPathCountByUUID_G;
Object[] finderArgs = new Object[] {uuid, groupId};
Long count = (Long)finderCache.getResult(
finderPath, finderArgs, this);
if (count == null) {
StringBundler sb = new StringBundler(3);
sb.append(_SQL_COUNT_FRAGMENTENTRYLINK_WHERE);
boolean bindUuid = false;
if (uuid.isEmpty()) {
sb.append(_FINDER_COLUMN_UUID_G_UUID_3);
}
else {
bindUuid = true;
sb.append(_FINDER_COLUMN_UUID_G_UUID_2);
}
sb.append(_FINDER_COLUMN_UUID_G_GROUPID_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
if (bindUuid) {
queryPos.add(uuid);
}
queryPos.add(groupId);
count = (Long)query.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
}
private static final String _FINDER_COLUMN_UUID_G_UUID_2 =
"fragmentEntryLink.uuid = ? AND ";
private static final String _FINDER_COLUMN_UUID_G_UUID_3 =
"(fragmentEntryLink.uuid IS NULL OR fragmentEntryLink.uuid = '') AND ";
private static final String _FINDER_COLUMN_UUID_G_GROUPID_2 =
"fragmentEntryLink.groupId = ?";
private FinderPath _finderPathWithPaginationFindByUuid_C;
private FinderPath _finderPathWithoutPaginationFindByUuid_C;
private FinderPath _finderPathCountByUuid_C;
/**
* Returns all the fragment entry links where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @return the matching fragment entry links
*/
@Override
public List findByUuid_C(String uuid, long companyId) {
return findByUuid_C(
uuid, companyId, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the fragment entry links where uuid = ? and companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryLinkModelImpl
.
*
*
* @param uuid the uuid
* @param companyId the company ID
* @param start the lower bound of the range of fragment entry links
* @param end the upper bound of the range of fragment entry links (not inclusive)
* @return the range of matching fragment entry links
*/
@Override
public List findByUuid_C(
String uuid, long companyId, int start, int end) {
return findByUuid_C(uuid, companyId, start, end, null);
}
/**
* Returns an ordered range of all the fragment entry links where uuid = ? and companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryLinkModelImpl
.
*
*
* @param uuid the uuid
* @param companyId the company ID
* @param start the lower bound of the range of fragment entry links
* @param end the upper bound of the range of fragment entry links (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entry links
*/
@Override
public List findByUuid_C(
String uuid, long companyId, int start, int end,
OrderByComparator orderByComparator) {
return findByUuid_C(
uuid, companyId, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the fragment entry links where uuid = ? and companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryLinkModelImpl
.
*
*
* @param uuid the uuid
* @param companyId the company ID
* @param start the lower bound of the range of fragment entry links
* @param end the upper bound of the range of fragment entry links (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching fragment entry links
*/
@Override
public List findByUuid_C(
String uuid, long companyId, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
try (SafeCloseable safeCloseable =
ctPersistenceHelper.setCTCollectionIdWithSafeCloseable(
FragmentEntryLink.class)) {
uuid = Objects.toString(uuid, "");
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache) {
finderPath = _finderPathWithoutPaginationFindByUuid_C;
finderArgs = new Object[] {uuid, companyId};
}
}
else if (useFinderCache) {
finderPath = _finderPathWithPaginationFindByUuid_C;
finderArgs = new Object[] {
uuid, companyId, start, end, orderByComparator
};
}
List list = null;
if (useFinderCache) {
list = (List)finderCache.getResult(
finderPath, finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (FragmentEntryLink fragmentEntryLink : list) {
if (!uuid.equals(fragmentEntryLink.getUuid()) ||
(companyId != fragmentEntryLink.getCompanyId())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_FRAGMENTENTRYLINK_WHERE);
boolean bindUuid = false;
if (uuid.isEmpty()) {
sb.append(_FINDER_COLUMN_UUID_C_UUID_3);
}
else {
bindUuid = true;
sb.append(_FINDER_COLUMN_UUID_C_UUID_2);
}
sb.append(_FINDER_COLUMN_UUID_C_COMPANYID_2);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(FragmentEntryLinkModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
if (bindUuid) {
queryPos.add(uuid);
}
queryPos.add(companyId);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
}
/**
* Returns the first fragment entry link in the ordered set where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry link
* @throws NoSuchEntryLinkException if a matching fragment entry link could not be found
*/
@Override
public FragmentEntryLink findByUuid_C_First(
String uuid, long companyId,
OrderByComparator orderByComparator)
throws NoSuchEntryLinkException {
FragmentEntryLink fragmentEntryLink = fetchByUuid_C_First(
uuid, companyId, orderByComparator);
if (fragmentEntryLink != null) {
return fragmentEntryLink;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("uuid=");
sb.append(uuid);
sb.append(", companyId=");
sb.append(companyId);
sb.append("}");
throw new NoSuchEntryLinkException(sb.toString());
}
/**
* Returns the first fragment entry link in the ordered set where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry link, or null
if a matching fragment entry link could not be found
*/
@Override
public FragmentEntryLink fetchByUuid_C_First(
String uuid, long companyId,
OrderByComparator orderByComparator) {
List list = findByUuid_C(
uuid, companyId, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last fragment entry link in the ordered set where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry link
* @throws NoSuchEntryLinkException if a matching fragment entry link could not be found
*/
@Override
public FragmentEntryLink findByUuid_C_Last(
String uuid, long companyId,
OrderByComparator orderByComparator)
throws NoSuchEntryLinkException {
FragmentEntryLink fragmentEntryLink = fetchByUuid_C_Last(
uuid, companyId, orderByComparator);
if (fragmentEntryLink != null) {
return fragmentEntryLink;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("uuid=");
sb.append(uuid);
sb.append(", companyId=");
sb.append(companyId);
sb.append("}");
throw new NoSuchEntryLinkException(sb.toString());
}
/**
* Returns the last fragment entry link in the ordered set where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry link, or null
if a matching fragment entry link could not be found
*/
@Override
public FragmentEntryLink fetchByUuid_C_Last(
String uuid, long companyId,
OrderByComparator orderByComparator) {
int count = countByUuid_C(uuid, companyId);
if (count == 0) {
return null;
}
List list = findByUuid_C(
uuid, companyId, count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the fragment entry links before and after the current fragment entry link in the ordered set where uuid = ? and companyId = ?.
*
* @param fragmentEntryLinkId the primary key of the current fragment entry link
* @param uuid the uuid
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next fragment entry link
* @throws NoSuchEntryLinkException if a fragment entry link with the primary key could not be found
*/
@Override
public FragmentEntryLink[] findByUuid_C_PrevAndNext(
long fragmentEntryLinkId, String uuid, long companyId,
OrderByComparator orderByComparator)
throws NoSuchEntryLinkException {
uuid = Objects.toString(uuid, "");
FragmentEntryLink fragmentEntryLink = findByPrimaryKey(
fragmentEntryLinkId);
Session session = null;
try {
session = openSession();
FragmentEntryLink[] array = new FragmentEntryLinkImpl[3];
array[0] = getByUuid_C_PrevAndNext(
session, fragmentEntryLink, uuid, companyId, orderByComparator,
true);
array[1] = fragmentEntryLink;
array[2] = getByUuid_C_PrevAndNext(
session, fragmentEntryLink, uuid, companyId, orderByComparator,
false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected FragmentEntryLink getByUuid_C_PrevAndNext(
Session session, FragmentEntryLink fragmentEntryLink, String uuid,
long companyId, OrderByComparator orderByComparator,
boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
5 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_FRAGMENTENTRYLINK_WHERE);
boolean bindUuid = false;
if (uuid.isEmpty()) {
sb.append(_FINDER_COLUMN_UUID_C_UUID_3);
}
else {
bindUuid = true;
sb.append(_FINDER_COLUMN_UUID_C_UUID_2);
}
sb.append(_FINDER_COLUMN_UUID_C_COMPANYID_2);
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(FragmentEntryLinkModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
if (bindUuid) {
queryPos.add(uuid);
}
queryPos.add(companyId);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
fragmentEntryLink)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the fragment entry links where uuid = ? and companyId = ? from the database.
*
* @param uuid the uuid
* @param companyId the company ID
*/
@Override
public void removeByUuid_C(String uuid, long companyId) {
for (FragmentEntryLink fragmentEntryLink :
findByUuid_C(
uuid, companyId, QueryUtil.ALL_POS, QueryUtil.ALL_POS,
null)) {
remove(fragmentEntryLink);
}
}
/**
* Returns the number of fragment entry links where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @return the number of matching fragment entry links
*/
@Override
public int countByUuid_C(String uuid, long companyId) {
try (SafeCloseable safeCloseable =
ctPersistenceHelper.setCTCollectionIdWithSafeCloseable(
FragmentEntryLink.class)) {
uuid = Objects.toString(uuid, "");
FinderPath finderPath = _finderPathCountByUuid_C;
Object[] finderArgs = new Object[] {uuid, companyId};
Long count = (Long)finderCache.getResult(
finderPath, finderArgs, this);
if (count == null) {
StringBundler sb = new StringBundler(3);
sb.append(_SQL_COUNT_FRAGMENTENTRYLINK_WHERE);
boolean bindUuid = false;
if (uuid.isEmpty()) {
sb.append(_FINDER_COLUMN_UUID_C_UUID_3);
}
else {
bindUuid = true;
sb.append(_FINDER_COLUMN_UUID_C_UUID_2);
}
sb.append(_FINDER_COLUMN_UUID_C_COMPANYID_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
if (bindUuid) {
queryPos.add(uuid);
}
queryPos.add(companyId);
count = (Long)query.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
}
private static final String _FINDER_COLUMN_UUID_C_UUID_2 =
"fragmentEntryLink.uuid = ? AND ";
private static final String _FINDER_COLUMN_UUID_C_UUID_3 =
"(fragmentEntryLink.uuid IS NULL OR fragmentEntryLink.uuid = '') AND ";
private static final String _FINDER_COLUMN_UUID_C_COMPANYID_2 =
"fragmentEntryLink.companyId = ?";
private FinderPath _finderPathWithPaginationFindByGroupId;
private FinderPath _finderPathWithoutPaginationFindByGroupId;
private FinderPath _finderPathCountByGroupId;
/**
* Returns all the fragment entry links where groupId = ?.
*
* @param groupId the group ID
* @return the matching fragment entry links
*/
@Override
public List findByGroupId(long groupId) {
return findByGroupId(
groupId, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the fragment entry links where groupId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryLinkModelImpl
.
*
*
* @param groupId the group ID
* @param start the lower bound of the range of fragment entry links
* @param end the upper bound of the range of fragment entry links (not inclusive)
* @return the range of matching fragment entry links
*/
@Override
public List findByGroupId(
long groupId, int start, int end) {
return findByGroupId(groupId, start, end, null);
}
/**
* Returns an ordered range of all the fragment entry links where groupId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryLinkModelImpl
.
*
*
* @param groupId the group ID
* @param start the lower bound of the range of fragment entry links
* @param end the upper bound of the range of fragment entry links (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entry links
*/
@Override
public List findByGroupId(
long groupId, int start, int end,
OrderByComparator orderByComparator) {
return findByGroupId(groupId, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the fragment entry links where groupId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryLinkModelImpl
.
*
*
* @param groupId the group ID
* @param start the lower bound of the range of fragment entry links
* @param end the upper bound of the range of fragment entry links (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching fragment entry links
*/
@Override
public List findByGroupId(
long groupId, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
try (SafeCloseable safeCloseable =
ctPersistenceHelper.setCTCollectionIdWithSafeCloseable(
FragmentEntryLink.class)) {
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache) {
finderPath = _finderPathWithoutPaginationFindByGroupId;
finderArgs = new Object[] {groupId};
}
}
else if (useFinderCache) {
finderPath = _finderPathWithPaginationFindByGroupId;
finderArgs = new Object[] {
groupId, start, end, orderByComparator
};
}
List list = null;
if (useFinderCache) {
list = (List)finderCache.getResult(
finderPath, finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (FragmentEntryLink fragmentEntryLink : list) {
if (groupId != fragmentEntryLink.getGroupId()) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
3 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(3);
}
sb.append(_SQL_SELECT_FRAGMENTENTRYLINK_WHERE);
sb.append(_FINDER_COLUMN_GROUPID_GROUPID_2);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(FragmentEntryLinkModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
}
/**
* Returns the first fragment entry link in the ordered set where groupId = ?.
*
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry link
* @throws NoSuchEntryLinkException if a matching fragment entry link could not be found
*/
@Override
public FragmentEntryLink findByGroupId_First(
long groupId,
OrderByComparator orderByComparator)
throws NoSuchEntryLinkException {
FragmentEntryLink fragmentEntryLink = fetchByGroupId_First(
groupId, orderByComparator);
if (fragmentEntryLink != null) {
return fragmentEntryLink;
}
StringBundler sb = new StringBundler(4);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("groupId=");
sb.append(groupId);
sb.append("}");
throw new NoSuchEntryLinkException(sb.toString());
}
/**
* Returns the first fragment entry link in the ordered set where groupId = ?.
*
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry link, or null
if a matching fragment entry link could not be found
*/
@Override
public FragmentEntryLink fetchByGroupId_First(
long groupId, OrderByComparator orderByComparator) {
List list = findByGroupId(
groupId, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last fragment entry link in the ordered set where groupId = ?.
*
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry link
* @throws NoSuchEntryLinkException if a matching fragment entry link could not be found
*/
@Override
public FragmentEntryLink findByGroupId_Last(
long groupId,
OrderByComparator orderByComparator)
throws NoSuchEntryLinkException {
FragmentEntryLink fragmentEntryLink = fetchByGroupId_Last(
groupId, orderByComparator);
if (fragmentEntryLink != null) {
return fragmentEntryLink;
}
StringBundler sb = new StringBundler(4);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("groupId=");
sb.append(groupId);
sb.append("}");
throw new NoSuchEntryLinkException(sb.toString());
}
/**
* Returns the last fragment entry link in the ordered set where groupId = ?.
*
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry link, or null
if a matching fragment entry link could not be found
*/
@Override
public FragmentEntryLink fetchByGroupId_Last(
long groupId, OrderByComparator orderByComparator) {
int count = countByGroupId(groupId);
if (count == 0) {
return null;
}
List list = findByGroupId(
groupId, count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the fragment entry links before and after the current fragment entry link in the ordered set where groupId = ?.
*
* @param fragmentEntryLinkId the primary key of the current fragment entry link
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next fragment entry link
* @throws NoSuchEntryLinkException if a fragment entry link with the primary key could not be found
*/
@Override
public FragmentEntryLink[] findByGroupId_PrevAndNext(
long fragmentEntryLinkId, long groupId,
OrderByComparator orderByComparator)
throws NoSuchEntryLinkException {
FragmentEntryLink fragmentEntryLink = findByPrimaryKey(
fragmentEntryLinkId);
Session session = null;
try {
session = openSession();
FragmentEntryLink[] array = new FragmentEntryLinkImpl[3];
array[0] = getByGroupId_PrevAndNext(
session, fragmentEntryLink, groupId, orderByComparator, true);
array[1] = fragmentEntryLink;
array[2] = getByGroupId_PrevAndNext(
session, fragmentEntryLink, groupId, orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected FragmentEntryLink getByGroupId_PrevAndNext(
Session session, FragmentEntryLink fragmentEntryLink, long groupId,
OrderByComparator orderByComparator,
boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(3);
}
sb.append(_SQL_SELECT_FRAGMENTENTRYLINK_WHERE);
sb.append(_FINDER_COLUMN_GROUPID_GROUPID_2);
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(FragmentEntryLinkModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
fragmentEntryLink)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the fragment entry links where groupId = ? from the database.
*
* @param groupId the group ID
*/
@Override
public void removeByGroupId(long groupId) {
for (FragmentEntryLink fragmentEntryLink :
findByGroupId(
groupId, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null)) {
remove(fragmentEntryLink);
}
}
/**
* Returns the number of fragment entry links where groupId = ?.
*
* @param groupId the group ID
* @return the number of matching fragment entry links
*/
@Override
public int countByGroupId(long groupId) {
try (SafeCloseable safeCloseable =
ctPersistenceHelper.setCTCollectionIdWithSafeCloseable(
FragmentEntryLink.class)) {
FinderPath finderPath = _finderPathCountByGroupId;
Object[] finderArgs = new Object[] {groupId};
Long count = (Long)finderCache.getResult(
finderPath, finderArgs, this);
if (count == null) {
StringBundler sb = new StringBundler(2);
sb.append(_SQL_COUNT_FRAGMENTENTRYLINK_WHERE);
sb.append(_FINDER_COLUMN_GROUPID_GROUPID_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
count = (Long)query.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
}
private static final String _FINDER_COLUMN_GROUPID_GROUPID_2 =
"fragmentEntryLink.groupId = ?";
private FinderPath _finderPathWithPaginationFindByFragmentEntryId;
private FinderPath _finderPathWithoutPaginationFindByFragmentEntryId;
private FinderPath _finderPathCountByFragmentEntryId;
private FinderPath _finderPathWithPaginationCountByFragmentEntryId;
/**
* Returns all the fragment entry links where fragmentEntryId = ?.
*
* @param fragmentEntryId the fragment entry ID
* @return the matching fragment entry links
*/
@Override
public List findByFragmentEntryId(long fragmentEntryId) {
return findByFragmentEntryId(
fragmentEntryId, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the fragment entry links where fragmentEntryId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryLinkModelImpl
.
*
*
* @param fragmentEntryId the fragment entry ID
* @param start the lower bound of the range of fragment entry links
* @param end the upper bound of the range of fragment entry links (not inclusive)
* @return the range of matching fragment entry links
*/
@Override
public List findByFragmentEntryId(
long fragmentEntryId, int start, int end) {
return findByFragmentEntryId(fragmentEntryId, start, end, null);
}
/**
* Returns an ordered range of all the fragment entry links where fragmentEntryId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryLinkModelImpl
.
*
*
* @param fragmentEntryId the fragment entry ID
* @param start the lower bound of the range of fragment entry links
* @param end the upper bound of the range of fragment entry links (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entry links
*/
@Override
public List findByFragmentEntryId(
long fragmentEntryId, int start, int end,
OrderByComparator orderByComparator) {
return findByFragmentEntryId(
fragmentEntryId, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the fragment entry links where fragmentEntryId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryLinkModelImpl
.
*
*
* @param fragmentEntryId the fragment entry ID
* @param start the lower bound of the range of fragment entry links
* @param end the upper bound of the range of fragment entry links (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching fragment entry links
*/
@Override
public List findByFragmentEntryId(
long fragmentEntryId, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
try (SafeCloseable safeCloseable =
ctPersistenceHelper.setCTCollectionIdWithSafeCloseable(
FragmentEntryLink.class)) {
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache) {
finderPath =
_finderPathWithoutPaginationFindByFragmentEntryId;
finderArgs = new Object[] {fragmentEntryId};
}
}
else if (useFinderCache) {
finderPath = _finderPathWithPaginationFindByFragmentEntryId;
finderArgs = new Object[] {
fragmentEntryId, start, end, orderByComparator
};
}
List list = null;
if (useFinderCache) {
list = (List)finderCache.getResult(
finderPath, finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (FragmentEntryLink fragmentEntryLink : list) {
if (fragmentEntryId !=
fragmentEntryLink.getFragmentEntryId()) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
3 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(3);
}
sb.append(_SQL_SELECT_FRAGMENTENTRYLINK_WHERE);
sb.append(_FINDER_COLUMN_FRAGMENTENTRYID_FRAGMENTENTRYID_2);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(FragmentEntryLinkModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(fragmentEntryId);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
}
/**
* Returns the first fragment entry link in the ordered set where fragmentEntryId = ?.
*
* @param fragmentEntryId the fragment entry ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry link
* @throws NoSuchEntryLinkException if a matching fragment entry link could not be found
*/
@Override
public FragmentEntryLink findByFragmentEntryId_First(
long fragmentEntryId,
OrderByComparator orderByComparator)
throws NoSuchEntryLinkException {
FragmentEntryLink fragmentEntryLink = fetchByFragmentEntryId_First(
fragmentEntryId, orderByComparator);
if (fragmentEntryLink != null) {
return fragmentEntryLink;
}
StringBundler sb = new StringBundler(4);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("fragmentEntryId=");
sb.append(fragmentEntryId);
sb.append("}");
throw new NoSuchEntryLinkException(sb.toString());
}
/**
* Returns the first fragment entry link in the ordered set where fragmentEntryId = ?.
*
* @param fragmentEntryId the fragment entry ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry link, or null
if a matching fragment entry link could not be found
*/
@Override
public FragmentEntryLink fetchByFragmentEntryId_First(
long fragmentEntryId,
OrderByComparator orderByComparator) {
List list = findByFragmentEntryId(
fragmentEntryId, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last fragment entry link in the ordered set where fragmentEntryId = ?.
*
* @param fragmentEntryId the fragment entry ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry link
* @throws NoSuchEntryLinkException if a matching fragment entry link could not be found
*/
@Override
public FragmentEntryLink findByFragmentEntryId_Last(
long fragmentEntryId,
OrderByComparator orderByComparator)
throws NoSuchEntryLinkException {
FragmentEntryLink fragmentEntryLink = fetchByFragmentEntryId_Last(
fragmentEntryId, orderByComparator);
if (fragmentEntryLink != null) {
return fragmentEntryLink;
}
StringBundler sb = new StringBundler(4);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("fragmentEntryId=");
sb.append(fragmentEntryId);
sb.append("}");
throw new NoSuchEntryLinkException(sb.toString());
}
/**
* Returns the last fragment entry link in the ordered set where fragmentEntryId = ?.
*
* @param fragmentEntryId the fragment entry ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry link, or null
if a matching fragment entry link could not be found
*/
@Override
public FragmentEntryLink fetchByFragmentEntryId_Last(
long fragmentEntryId,
OrderByComparator orderByComparator) {
int count = countByFragmentEntryId(fragmentEntryId);
if (count == 0) {
return null;
}
List list = findByFragmentEntryId(
fragmentEntryId, count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the fragment entry links before and after the current fragment entry link in the ordered set where fragmentEntryId = ?.
*
* @param fragmentEntryLinkId the primary key of the current fragment entry link
* @param fragmentEntryId the fragment entry ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next fragment entry link
* @throws NoSuchEntryLinkException if a fragment entry link with the primary key could not be found
*/
@Override
public FragmentEntryLink[] findByFragmentEntryId_PrevAndNext(
long fragmentEntryLinkId, long fragmentEntryId,
OrderByComparator orderByComparator)
throws NoSuchEntryLinkException {
FragmentEntryLink fragmentEntryLink = findByPrimaryKey(
fragmentEntryLinkId);
Session session = null;
try {
session = openSession();
FragmentEntryLink[] array = new FragmentEntryLinkImpl[3];
array[0] = getByFragmentEntryId_PrevAndNext(
session, fragmentEntryLink, fragmentEntryId, orderByComparator,
true);
array[1] = fragmentEntryLink;
array[2] = getByFragmentEntryId_PrevAndNext(
session, fragmentEntryLink, fragmentEntryId, orderByComparator,
false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected FragmentEntryLink getByFragmentEntryId_PrevAndNext(
Session session, FragmentEntryLink fragmentEntryLink,
long fragmentEntryId,
OrderByComparator orderByComparator,
boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(3);
}
sb.append(_SQL_SELECT_FRAGMENTENTRYLINK_WHERE);
sb.append(_FINDER_COLUMN_FRAGMENTENTRYID_FRAGMENTENTRYID_2);
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(FragmentEntryLinkModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(fragmentEntryId);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
fragmentEntryLink)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Returns all the fragment entry links where fragmentEntryId = any ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryLinkModelImpl
.
*
*
* @param fragmentEntryIds the fragment entry IDs
* @return the matching fragment entry links
*/
@Override
public List findByFragmentEntryId(
long[] fragmentEntryIds) {
return findByFragmentEntryId(
fragmentEntryIds, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the fragment entry links where fragmentEntryId = any ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryLinkModelImpl
.
*
*
* @param fragmentEntryIds the fragment entry IDs
* @param start the lower bound of the range of fragment entry links
* @param end the upper bound of the range of fragment entry links (not inclusive)
* @return the range of matching fragment entry links
*/
@Override
public List findByFragmentEntryId(
long[] fragmentEntryIds, int start, int end) {
return findByFragmentEntryId(fragmentEntryIds, start, end, null);
}
/**
* Returns an ordered range of all the fragment entry links where fragmentEntryId = any ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryLinkModelImpl
.
*
*
* @param fragmentEntryIds the fragment entry IDs
* @param start the lower bound of the range of fragment entry links
* @param end the upper bound of the range of fragment entry links (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entry links
*/
@Override
public List findByFragmentEntryId(
long[] fragmentEntryIds, int start, int end,
OrderByComparator orderByComparator) {
return findByFragmentEntryId(
fragmentEntryIds, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the fragment entry links where fragmentEntryId = ?, optionally using the finder cache.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryLinkModelImpl
.
*
*
* @param fragmentEntryIds the fragment entry IDs
* @param start the lower bound of the range of fragment entry links
* @param end the upper bound of the range of fragment entry links (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching fragment entry links
*/
@Override
public List findByFragmentEntryId(
long[] fragmentEntryIds, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
if (fragmentEntryIds == null) {
fragmentEntryIds = new long[0];
}
else if (fragmentEntryIds.length > 1) {
fragmentEntryIds = ArrayUtil.sortedUnique(fragmentEntryIds);
}
if (fragmentEntryIds.length == 1) {
return findByFragmentEntryId(
fragmentEntryIds[0], start, end, orderByComparator);
}
try (SafeCloseable safeCloseable =
ctPersistenceHelper.setCTCollectionIdWithSafeCloseable(
FragmentEntryLink.class)) {
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache) {
finderArgs = new Object[] {
StringUtil.merge(fragmentEntryIds)
};
}
}
else if (useFinderCache) {
finderArgs = new Object[] {
StringUtil.merge(fragmentEntryIds), start, end,
orderByComparator
};
}
List list = null;
if (useFinderCache) {
list = (List)finderCache.getResult(
_finderPathWithPaginationFindByFragmentEntryId, finderArgs,
this);
if ((list != null) && !list.isEmpty()) {
for (FragmentEntryLink fragmentEntryLink : list) {
if (!ArrayUtil.contains(
fragmentEntryIds,
fragmentEntryLink.getFragmentEntryId())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = new StringBundler();
sb.append(_SQL_SELECT_FRAGMENTENTRYLINK_WHERE);
if (fragmentEntryIds.length > 0) {
sb.append("(");
sb.append(_FINDER_COLUMN_FRAGMENTENTRYID_FRAGMENTENTRYID_7);
sb.append(StringUtil.merge(fragmentEntryIds));
sb.append(")");
sb.append(")");
}
sb.setStringAt(
removeConjunction(sb.stringAt(sb.index() - 1)),
sb.index() - 1);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(FragmentEntryLinkModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache) {
finderCache.putResult(
_finderPathWithPaginationFindByFragmentEntryId,
finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
}
/**
* Removes all the fragment entry links where fragmentEntryId = ? from the database.
*
* @param fragmentEntryId the fragment entry ID
*/
@Override
public void removeByFragmentEntryId(long fragmentEntryId) {
for (FragmentEntryLink fragmentEntryLink :
findByFragmentEntryId(
fragmentEntryId, QueryUtil.ALL_POS, QueryUtil.ALL_POS,
null)) {
remove(fragmentEntryLink);
}
}
/**
* Returns the number of fragment entry links where fragmentEntryId = ?.
*
* @param fragmentEntryId the fragment entry ID
* @return the number of matching fragment entry links
*/
@Override
public int countByFragmentEntryId(long fragmentEntryId) {
try (SafeCloseable safeCloseable =
ctPersistenceHelper.setCTCollectionIdWithSafeCloseable(
FragmentEntryLink.class)) {
FinderPath finderPath = _finderPathCountByFragmentEntryId;
Object[] finderArgs = new Object[] {fragmentEntryId};
Long count = (Long)finderCache.getResult(
finderPath, finderArgs, this);
if (count == null) {
StringBundler sb = new StringBundler(2);
sb.append(_SQL_COUNT_FRAGMENTENTRYLINK_WHERE);
sb.append(_FINDER_COLUMN_FRAGMENTENTRYID_FRAGMENTENTRYID_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(fragmentEntryId);
count = (Long)query.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
}
/**
* Returns the number of fragment entry links where fragmentEntryId = any ?.
*
* @param fragmentEntryIds the fragment entry IDs
* @return the number of matching fragment entry links
*/
@Override
public int countByFragmentEntryId(long[] fragmentEntryIds) {
if (fragmentEntryIds == null) {
fragmentEntryIds = new long[0];
}
else if (fragmentEntryIds.length > 1) {
fragmentEntryIds = ArrayUtil.sortedUnique(fragmentEntryIds);
}
try (SafeCloseable safeCloseable =
ctPersistenceHelper.setCTCollectionIdWithSafeCloseable(
FragmentEntryLink.class)) {
Object[] finderArgs = new Object[] {
StringUtil.merge(fragmentEntryIds)
};
Long count = (Long)finderCache.getResult(
_finderPathWithPaginationCountByFragmentEntryId, finderArgs,
this);
if (count == null) {
StringBundler sb = new StringBundler();
sb.append(_SQL_COUNT_FRAGMENTENTRYLINK_WHERE);
if (fragmentEntryIds.length > 0) {
sb.append("(");
sb.append(_FINDER_COLUMN_FRAGMENTENTRYID_FRAGMENTENTRYID_7);
sb.append(StringUtil.merge(fragmentEntryIds));
sb.append(")");
sb.append(")");
}
sb.setStringAt(
removeConjunction(sb.stringAt(sb.index() - 1)),
sb.index() - 1);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
count = (Long)query.uniqueResult();
finderCache.putResult(
_finderPathWithPaginationCountByFragmentEntryId,
finderArgs, count);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
}
private static final String
_FINDER_COLUMN_FRAGMENTENTRYID_FRAGMENTENTRYID_2 =
"fragmentEntryLink.fragmentEntryId = ?";
private static final String
_FINDER_COLUMN_FRAGMENTENTRYID_FRAGMENTENTRYID_7 =
"fragmentEntryLink.fragmentEntryId IN (";
private FinderPath _finderPathWithPaginationFindByRendererKey;
private FinderPath _finderPathWithoutPaginationFindByRendererKey;
private FinderPath _finderPathCountByRendererKey;
/**
* Returns all the fragment entry links where rendererKey = ?.
*
* @param rendererKey the renderer key
* @return the matching fragment entry links
*/
@Override
public List findByRendererKey(String rendererKey) {
return findByRendererKey(
rendererKey, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the fragment entry links where rendererKey = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryLinkModelImpl
.
*
*
* @param rendererKey the renderer key
* @param start the lower bound of the range of fragment entry links
* @param end the upper bound of the range of fragment entry links (not inclusive)
* @return the range of matching fragment entry links
*/
@Override
public List findByRendererKey(
String rendererKey, int start, int end) {
return findByRendererKey(rendererKey, start, end, null);
}
/**
* Returns an ordered range of all the fragment entry links where rendererKey = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryLinkModelImpl
.
*
*
* @param rendererKey the renderer key
* @param start the lower bound of the range of fragment entry links
* @param end the upper bound of the range of fragment entry links (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entry links
*/
@Override
public List findByRendererKey(
String rendererKey, int start, int end,
OrderByComparator orderByComparator) {
return findByRendererKey(
rendererKey, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the fragment entry links where rendererKey = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryLinkModelImpl
.
*
*
* @param rendererKey the renderer key
* @param start the lower bound of the range of fragment entry links
* @param end the upper bound of the range of fragment entry links (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching fragment entry links
*/
@Override
public List findByRendererKey(
String rendererKey, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
try (SafeCloseable safeCloseable =
ctPersistenceHelper.setCTCollectionIdWithSafeCloseable(
FragmentEntryLink.class)) {
rendererKey = Objects.toString(rendererKey, "");
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache) {
finderPath = _finderPathWithoutPaginationFindByRendererKey;
finderArgs = new Object[] {rendererKey};
}
}
else if (useFinderCache) {
finderPath = _finderPathWithPaginationFindByRendererKey;
finderArgs = new Object[] {
rendererKey, start, end, orderByComparator
};
}
List list = null;
if (useFinderCache) {
list = (List)finderCache.getResult(
finderPath, finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (FragmentEntryLink fragmentEntryLink : list) {
if (!rendererKey.equals(
fragmentEntryLink.getRendererKey())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
3 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(3);
}
sb.append(_SQL_SELECT_FRAGMENTENTRYLINK_WHERE);
boolean bindRendererKey = false;
if (rendererKey.isEmpty()) {
sb.append(_FINDER_COLUMN_RENDERERKEY_RENDERERKEY_3);
}
else {
bindRendererKey = true;
sb.append(_FINDER_COLUMN_RENDERERKEY_RENDERERKEY_2);
}
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(FragmentEntryLinkModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
if (bindRendererKey) {
queryPos.add(rendererKey);
}
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
}
/**
* Returns the first fragment entry link in the ordered set where rendererKey = ?.
*
* @param rendererKey the renderer key
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry link
* @throws NoSuchEntryLinkException if a matching fragment entry link could not be found
*/
@Override
public FragmentEntryLink findByRendererKey_First(
String rendererKey,
OrderByComparator orderByComparator)
throws NoSuchEntryLinkException {
FragmentEntryLink fragmentEntryLink = fetchByRendererKey_First(
rendererKey, orderByComparator);
if (fragmentEntryLink != null) {
return fragmentEntryLink;
}
StringBundler sb = new StringBundler(4);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("rendererKey=");
sb.append(rendererKey);
sb.append("}");
throw new NoSuchEntryLinkException(sb.toString());
}
/**
* Returns the first fragment entry link in the ordered set where rendererKey = ?.
*
* @param rendererKey the renderer key
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry link, or null
if a matching fragment entry link could not be found
*/
@Override
public FragmentEntryLink fetchByRendererKey_First(
String rendererKey,
OrderByComparator orderByComparator) {
List list = findByRendererKey(
rendererKey, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last fragment entry link in the ordered set where rendererKey = ?.
*
* @param rendererKey the renderer key
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry link
* @throws NoSuchEntryLinkException if a matching fragment entry link could not be found
*/
@Override
public FragmentEntryLink findByRendererKey_Last(
String rendererKey,
OrderByComparator orderByComparator)
throws NoSuchEntryLinkException {
FragmentEntryLink fragmentEntryLink = fetchByRendererKey_Last(
rendererKey, orderByComparator);
if (fragmentEntryLink != null) {
return fragmentEntryLink;
}
StringBundler sb = new StringBundler(4);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("rendererKey=");
sb.append(rendererKey);
sb.append("}");
throw new NoSuchEntryLinkException(sb.toString());
}
/**
* Returns the last fragment entry link in the ordered set where rendererKey = ?.
*
* @param rendererKey the renderer key
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry link, or null
if a matching fragment entry link could not be found
*/
@Override
public FragmentEntryLink fetchByRendererKey_Last(
String rendererKey,
OrderByComparator orderByComparator) {
int count = countByRendererKey(rendererKey);
if (count == 0) {
return null;
}
List list = findByRendererKey(
rendererKey, count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the fragment entry links before and after the current fragment entry link in the ordered set where rendererKey = ?.
*
* @param fragmentEntryLinkId the primary key of the current fragment entry link
* @param rendererKey the renderer key
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next fragment entry link
* @throws NoSuchEntryLinkException if a fragment entry link with the primary key could not be found
*/
@Override
public FragmentEntryLink[] findByRendererKey_PrevAndNext(
long fragmentEntryLinkId, String rendererKey,
OrderByComparator orderByComparator)
throws NoSuchEntryLinkException {
rendererKey = Objects.toString(rendererKey, "");
FragmentEntryLink fragmentEntryLink = findByPrimaryKey(
fragmentEntryLinkId);
Session session = null;
try {
session = openSession();
FragmentEntryLink[] array = new FragmentEntryLinkImpl[3];
array[0] = getByRendererKey_PrevAndNext(
session, fragmentEntryLink, rendererKey, orderByComparator,
true);
array[1] = fragmentEntryLink;
array[2] = getByRendererKey_PrevAndNext(
session, fragmentEntryLink, rendererKey, orderByComparator,
false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected FragmentEntryLink getByRendererKey_PrevAndNext(
Session session, FragmentEntryLink fragmentEntryLink,
String rendererKey,
OrderByComparator orderByComparator,
boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(3);
}
sb.append(_SQL_SELECT_FRAGMENTENTRYLINK_WHERE);
boolean bindRendererKey = false;
if (rendererKey.isEmpty()) {
sb.append(_FINDER_COLUMN_RENDERERKEY_RENDERERKEY_3);
}
else {
bindRendererKey = true;
sb.append(_FINDER_COLUMN_RENDERERKEY_RENDERERKEY_2);
}
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(FragmentEntryLinkModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
if (bindRendererKey) {
queryPos.add(rendererKey);
}
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
fragmentEntryLink)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the fragment entry links where rendererKey = ? from the database.
*
* @param rendererKey the renderer key
*/
@Override
public void removeByRendererKey(String rendererKey) {
for (FragmentEntryLink fragmentEntryLink :
findByRendererKey(
rendererKey, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null)) {
remove(fragmentEntryLink);
}
}
/**
* Returns the number of fragment entry links where rendererKey = ?.
*
* @param rendererKey the renderer key
* @return the number of matching fragment entry links
*/
@Override
public int countByRendererKey(String rendererKey) {
try (SafeCloseable safeCloseable =
ctPersistenceHelper.setCTCollectionIdWithSafeCloseable(
FragmentEntryLink.class)) {
rendererKey = Objects.toString(rendererKey, "");
FinderPath finderPath = _finderPathCountByRendererKey;
Object[] finderArgs = new Object[] {rendererKey};
Long count = (Long)finderCache.getResult(
finderPath, finderArgs, this);
if (count == null) {
StringBundler sb = new StringBundler(2);
sb.append(_SQL_COUNT_FRAGMENTENTRYLINK_WHERE);
boolean bindRendererKey = false;
if (rendererKey.isEmpty()) {
sb.append(_FINDER_COLUMN_RENDERERKEY_RENDERERKEY_3);
}
else {
bindRendererKey = true;
sb.append(_FINDER_COLUMN_RENDERERKEY_RENDERERKEY_2);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
if (bindRendererKey) {
queryPos.add(rendererKey);
}
count = (Long)query.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
}
private static final String _FINDER_COLUMN_RENDERERKEY_RENDERERKEY_2 =
"fragmentEntryLink.rendererKey = ?";
private static final String _FINDER_COLUMN_RENDERERKEY_RENDERERKEY_3 =
"(fragmentEntryLink.rendererKey IS NULL OR fragmentEntryLink.rendererKey = '')";
private FinderPath _finderPathWithPaginationFindByG_F;
private FinderPath _finderPathWithoutPaginationFindByG_F;
private FinderPath _finderPathCountByG_F;
/**
* Returns all the fragment entry links where groupId = ? and fragmentEntryId = ?.
*
* @param groupId the group ID
* @param fragmentEntryId the fragment entry ID
* @return the matching fragment entry links
*/
@Override
public List findByG_F(
long groupId, long fragmentEntryId) {
return findByG_F(
groupId, fragmentEntryId, QueryUtil.ALL_POS, QueryUtil.ALL_POS,
null);
}
/**
* Returns a range of all the fragment entry links where groupId = ? and fragmentEntryId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryLinkModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentEntryId the fragment entry ID
* @param start the lower bound of the range of fragment entry links
* @param end the upper bound of the range of fragment entry links (not inclusive)
* @return the range of matching fragment entry links
*/
@Override
public List findByG_F(
long groupId, long fragmentEntryId, int start, int end) {
return findByG_F(groupId, fragmentEntryId, start, end, null);
}
/**
* Returns an ordered range of all the fragment entry links where groupId = ? and fragmentEntryId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryLinkModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentEntryId the fragment entry ID
* @param start the lower bound of the range of fragment entry links
* @param end the upper bound of the range of fragment entry links (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entry links
*/
@Override
public List findByG_F(
long groupId, long fragmentEntryId, int start, int end,
OrderByComparator orderByComparator) {
return findByG_F(
groupId, fragmentEntryId, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the fragment entry links where groupId = ? and fragmentEntryId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryLinkModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentEntryId the fragment entry ID
* @param start the lower bound of the range of fragment entry links
* @param end the upper bound of the range of fragment entry links (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching fragment entry links
*/
@Override
public List findByG_F(
long groupId, long fragmentEntryId, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
try (SafeCloseable safeCloseable =
ctPersistenceHelper.setCTCollectionIdWithSafeCloseable(
FragmentEntryLink.class)) {
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache) {
finderPath = _finderPathWithoutPaginationFindByG_F;
finderArgs = new Object[] {groupId, fragmentEntryId};
}
}
else if (useFinderCache) {
finderPath = _finderPathWithPaginationFindByG_F;
finderArgs = new Object[] {
groupId, fragmentEntryId, start, end, orderByComparator
};
}
List list = null;
if (useFinderCache) {
list = (List)finderCache.getResult(
finderPath, finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (FragmentEntryLink fragmentEntryLink : list) {
if ((groupId != fragmentEntryLink.getGroupId()) ||
(fragmentEntryId !=
fragmentEntryLink.getFragmentEntryId())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_FRAGMENTENTRYLINK_WHERE);
sb.append(_FINDER_COLUMN_G_F_GROUPID_2);
sb.append(_FINDER_COLUMN_G_F_FRAGMENTENTRYID_2);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(FragmentEntryLinkModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
queryPos.add(fragmentEntryId);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
}
/**
* Returns the first fragment entry link in the ordered set where groupId = ? and fragmentEntryId = ?.
*
* @param groupId the group ID
* @param fragmentEntryId the fragment entry ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry link
* @throws NoSuchEntryLinkException if a matching fragment entry link could not be found
*/
@Override
public FragmentEntryLink findByG_F_First(
long groupId, long fragmentEntryId,
OrderByComparator orderByComparator)
throws NoSuchEntryLinkException {
FragmentEntryLink fragmentEntryLink = fetchByG_F_First(
groupId, fragmentEntryId, orderByComparator);
if (fragmentEntryLink != null) {
return fragmentEntryLink;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("groupId=");
sb.append(groupId);
sb.append(", fragmentEntryId=");
sb.append(fragmentEntryId);
sb.append("}");
throw new NoSuchEntryLinkException(sb.toString());
}
/**
* Returns the first fragment entry link in the ordered set where groupId = ? and fragmentEntryId = ?.
*
* @param groupId the group ID
* @param fragmentEntryId the fragment entry ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry link, or null
if a matching fragment entry link could not be found
*/
@Override
public FragmentEntryLink fetchByG_F_First(
long groupId, long fragmentEntryId,
OrderByComparator orderByComparator) {
List list = findByG_F(
groupId, fragmentEntryId, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last fragment entry link in the ordered set where groupId = ? and fragmentEntryId = ?.
*
* @param groupId the group ID
* @param fragmentEntryId the fragment entry ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry link
* @throws NoSuchEntryLinkException if a matching fragment entry link could not be found
*/
@Override
public FragmentEntryLink findByG_F_Last(
long groupId, long fragmentEntryId,
OrderByComparator orderByComparator)
throws NoSuchEntryLinkException {
FragmentEntryLink fragmentEntryLink = fetchByG_F_Last(
groupId, fragmentEntryId, orderByComparator);
if (fragmentEntryLink != null) {
return fragmentEntryLink;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("groupId=");
sb.append(groupId);
sb.append(", fragmentEntryId=");
sb.append(fragmentEntryId);
sb.append("}");
throw new NoSuchEntryLinkException(sb.toString());
}
/**
* Returns the last fragment entry link in the ordered set where groupId = ? and fragmentEntryId = ?.
*
* @param groupId the group ID
* @param fragmentEntryId the fragment entry ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry link, or null
if a matching fragment entry link could not be found
*/
@Override
public FragmentEntryLink fetchByG_F_Last(
long groupId, long fragmentEntryId,
OrderByComparator orderByComparator) {
int count = countByG_F(groupId, fragmentEntryId);
if (count == 0) {
return null;
}
List list = findByG_F(
groupId, fragmentEntryId, count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the fragment entry links before and after the current fragment entry link in the ordered set where groupId = ? and fragmentEntryId = ?.
*
* @param fragmentEntryLinkId the primary key of the current fragment entry link
* @param groupId the group ID
* @param fragmentEntryId the fragment entry ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next fragment entry link
* @throws NoSuchEntryLinkException if a fragment entry link with the primary key could not be found
*/
@Override
public FragmentEntryLink[] findByG_F_PrevAndNext(
long fragmentEntryLinkId, long groupId, long fragmentEntryId,
OrderByComparator orderByComparator)
throws NoSuchEntryLinkException {
FragmentEntryLink fragmentEntryLink = findByPrimaryKey(
fragmentEntryLinkId);
Session session = null;
try {
session = openSession();
FragmentEntryLink[] array = new FragmentEntryLinkImpl[3];
array[0] = getByG_F_PrevAndNext(
session, fragmentEntryLink, groupId, fragmentEntryId,
orderByComparator, true);
array[1] = fragmentEntryLink;
array[2] = getByG_F_PrevAndNext(
session, fragmentEntryLink, groupId, fragmentEntryId,
orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected FragmentEntryLink getByG_F_PrevAndNext(
Session session, FragmentEntryLink fragmentEntryLink, long groupId,
long fragmentEntryId,
OrderByComparator orderByComparator,
boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
5 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_FRAGMENTENTRYLINK_WHERE);
sb.append(_FINDER_COLUMN_G_F_GROUPID_2);
sb.append(_FINDER_COLUMN_G_F_FRAGMENTENTRYID_2);
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(FragmentEntryLinkModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
queryPos.add(fragmentEntryId);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
fragmentEntryLink)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the fragment entry links where groupId = ? and fragmentEntryId = ? from the database.
*
* @param groupId the group ID
* @param fragmentEntryId the fragment entry ID
*/
@Override
public void removeByG_F(long groupId, long fragmentEntryId) {
for (FragmentEntryLink fragmentEntryLink :
findByG_F(
groupId, fragmentEntryId, QueryUtil.ALL_POS,
QueryUtil.ALL_POS, null)) {
remove(fragmentEntryLink);
}
}
/**
* Returns the number of fragment entry links where groupId = ? and fragmentEntryId = ?.
*
* @param groupId the group ID
* @param fragmentEntryId the fragment entry ID
* @return the number of matching fragment entry links
*/
@Override
public int countByG_F(long groupId, long fragmentEntryId) {
try (SafeCloseable safeCloseable =
ctPersistenceHelper.setCTCollectionIdWithSafeCloseable(
FragmentEntryLink.class)) {
FinderPath finderPath = _finderPathCountByG_F;
Object[] finderArgs = new Object[] {groupId, fragmentEntryId};
Long count = (Long)finderCache.getResult(
finderPath, finderArgs, this);
if (count == null) {
StringBundler sb = new StringBundler(3);
sb.append(_SQL_COUNT_FRAGMENTENTRYLINK_WHERE);
sb.append(_FINDER_COLUMN_G_F_GROUPID_2);
sb.append(_FINDER_COLUMN_G_F_FRAGMENTENTRYID_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
queryPos.add(fragmentEntryId);
count = (Long)query.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
}
private static final String _FINDER_COLUMN_G_F_GROUPID_2 =
"fragmentEntryLink.groupId = ? AND ";
private static final String _FINDER_COLUMN_G_F_FRAGMENTENTRYID_2 =
"fragmentEntryLink.fragmentEntryId = ?";
private FinderPath _finderPathWithPaginationFindByG_P;
private FinderPath _finderPathWithoutPaginationFindByG_P;
private FinderPath _finderPathCountByG_P;
/**
* Returns all the fragment entry links where groupId = ? and plid = ?.
*
* @param groupId the group ID
* @param plid the plid
* @return the matching fragment entry links
*/
@Override
public List findByG_P(long groupId, long plid) {
return findByG_P(
groupId, plid, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the fragment entry links where groupId = ? and plid = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryLinkModelImpl
.
*
*
* @param groupId the group ID
* @param plid the plid
* @param start the lower bound of the range of fragment entry links
* @param end the upper bound of the range of fragment entry links (not inclusive)
* @return the range of matching fragment entry links
*/
@Override
public List findByG_P(
long groupId, long plid, int start, int end) {
return findByG_P(groupId, plid, start, end, null);
}
/**
* Returns an ordered range of all the fragment entry links where groupId = ? and plid = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryLinkModelImpl
.
*
*
* @param groupId the group ID
* @param plid the plid
* @param start the lower bound of the range of fragment entry links
* @param end the upper bound of the range of fragment entry links (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entry links
*/
@Override
public List findByG_P(
long groupId, long plid, int start, int end,
OrderByComparator orderByComparator) {
return findByG_P(groupId, plid, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the fragment entry links where groupId = ? and plid = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryLinkModelImpl
.
*
*
* @param groupId the group ID
* @param plid the plid
* @param start the lower bound of the range of fragment entry links
* @param end the upper bound of the range of fragment entry links (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching fragment entry links
*/
@Override
public List findByG_P(
long groupId, long plid, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
try (SafeCloseable safeCloseable =
ctPersistenceHelper.setCTCollectionIdWithSafeCloseable(
FragmentEntryLink.class)) {
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache) {
finderPath = _finderPathWithoutPaginationFindByG_P;
finderArgs = new Object[] {groupId, plid};
}
}
else if (useFinderCache) {
finderPath = _finderPathWithPaginationFindByG_P;
finderArgs = new Object[] {
groupId, plid, start, end, orderByComparator
};
}
List list = null;
if (useFinderCache) {
list = (List)finderCache.getResult(
finderPath, finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (FragmentEntryLink fragmentEntryLink : list) {
if ((groupId != fragmentEntryLink.getGroupId()) ||
(plid != fragmentEntryLink.getPlid())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_FRAGMENTENTRYLINK_WHERE);
sb.append(_FINDER_COLUMN_G_P_GROUPID_2);
sb.append(_FINDER_COLUMN_G_P_PLID_2);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(FragmentEntryLinkModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
queryPos.add(plid);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
}
/**
* Returns the first fragment entry link in the ordered set where groupId = ? and plid = ?.
*
* @param groupId the group ID
* @param plid the plid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry link
* @throws NoSuchEntryLinkException if a matching fragment entry link could not be found
*/
@Override
public FragmentEntryLink findByG_P_First(
long groupId, long plid,
OrderByComparator orderByComparator)
throws NoSuchEntryLinkException {
FragmentEntryLink fragmentEntryLink = fetchByG_P_First(
groupId, plid, orderByComparator);
if (fragmentEntryLink != null) {
return fragmentEntryLink;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("groupId=");
sb.append(groupId);
sb.append(", plid=");
sb.append(plid);
sb.append("}");
throw new NoSuchEntryLinkException(sb.toString());
}
/**
* Returns the first fragment entry link in the ordered set where groupId = ? and plid = ?.
*
* @param groupId the group ID
* @param plid the plid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry link, or null
if a matching fragment entry link could not be found
*/
@Override
public FragmentEntryLink fetchByG_P_First(
long groupId, long plid,
OrderByComparator orderByComparator) {
List list = findByG_P(
groupId, plid, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last fragment entry link in the ordered set where groupId = ? and plid = ?.
*
* @param groupId the group ID
* @param plid the plid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry link
* @throws NoSuchEntryLinkException if a matching fragment entry link could not be found
*/
@Override
public FragmentEntryLink findByG_P_Last(
long groupId, long plid,
OrderByComparator orderByComparator)
throws NoSuchEntryLinkException {
FragmentEntryLink fragmentEntryLink = fetchByG_P_Last(
groupId, plid, orderByComparator);
if (fragmentEntryLink != null) {
return fragmentEntryLink;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("groupId=");
sb.append(groupId);
sb.append(", plid=");
sb.append(plid);
sb.append("}");
throw new NoSuchEntryLinkException(sb.toString());
}
/**
* Returns the last fragment entry link in the ordered set where groupId = ? and plid = ?.
*
* @param groupId the group ID
* @param plid the plid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry link, or null
if a matching fragment entry link could not be found
*/
@Override
public FragmentEntryLink fetchByG_P_Last(
long groupId, long plid,
OrderByComparator orderByComparator) {
int count = countByG_P(groupId, plid);
if (count == 0) {
return null;
}
List list = findByG_P(
groupId, plid, count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the fragment entry links before and after the current fragment entry link in the ordered set where groupId = ? and plid = ?.
*
* @param fragmentEntryLinkId the primary key of the current fragment entry link
* @param groupId the group ID
* @param plid the plid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next fragment entry link
* @throws NoSuchEntryLinkException if a fragment entry link with the primary key could not be found
*/
@Override
public FragmentEntryLink[] findByG_P_PrevAndNext(
long fragmentEntryLinkId, long groupId, long plid,
OrderByComparator orderByComparator)
throws NoSuchEntryLinkException {
FragmentEntryLink fragmentEntryLink = findByPrimaryKey(
fragmentEntryLinkId);
Session session = null;
try {
session = openSession();
FragmentEntryLink[] array = new FragmentEntryLinkImpl[3];
array[0] = getByG_P_PrevAndNext(
session, fragmentEntryLink, groupId, plid, orderByComparator,
true);
array[1] = fragmentEntryLink;
array[2] = getByG_P_PrevAndNext(
session, fragmentEntryLink, groupId, plid, orderByComparator,
false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected FragmentEntryLink getByG_P_PrevAndNext(
Session session, FragmentEntryLink fragmentEntryLink, long groupId,
long plid, OrderByComparator orderByComparator,
boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
5 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_FRAGMENTENTRYLINK_WHERE);
sb.append(_FINDER_COLUMN_G_P_GROUPID_2);
sb.append(_FINDER_COLUMN_G_P_PLID_2);
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(FragmentEntryLinkModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
queryPos.add(plid);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
fragmentEntryLink)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the fragment entry links where groupId = ? and plid = ? from the database.
*
* @param groupId the group ID
* @param plid the plid
*/
@Override
public void removeByG_P(long groupId, long plid) {
for (FragmentEntryLink fragmentEntryLink :
findByG_P(
groupId, plid, QueryUtil.ALL_POS, QueryUtil.ALL_POS,
null)) {
remove(fragmentEntryLink);
}
}
/**
* Returns the number of fragment entry links where groupId = ? and plid = ?.
*
* @param groupId the group ID
* @param plid the plid
* @return the number of matching fragment entry links
*/
@Override
public int countByG_P(long groupId, long plid) {
try (SafeCloseable safeCloseable =
ctPersistenceHelper.setCTCollectionIdWithSafeCloseable(
FragmentEntryLink.class)) {
FinderPath finderPath = _finderPathCountByG_P;
Object[] finderArgs = new Object[] {groupId, plid};
Long count = (Long)finderCache.getResult(
finderPath, finderArgs, this);
if (count == null) {
StringBundler sb = new StringBundler(3);
sb.append(_SQL_COUNT_FRAGMENTENTRYLINK_WHERE);
sb.append(_FINDER_COLUMN_G_P_GROUPID_2);
sb.append(_FINDER_COLUMN_G_P_PLID_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
queryPos.add(plid);
count = (Long)query.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
}
private static final String _FINDER_COLUMN_G_P_GROUPID_2 =
"fragmentEntryLink.groupId = ? AND ";
private static final String _FINDER_COLUMN_G_P_PLID_2 =
"fragmentEntryLink.plid = ?";
private FinderPath _finderPathWithPaginationFindByC_R;
private FinderPath _finderPathWithoutPaginationFindByC_R;
private FinderPath _finderPathCountByC_R;
private FinderPath _finderPathWithPaginationCountByC_R;
/**
* Returns all the fragment entry links where companyId = ? and rendererKey = ?.
*
* @param companyId the company ID
* @param rendererKey the renderer key
* @return the matching fragment entry links
*/
@Override
public List findByC_R(
long companyId, String rendererKey) {
return findByC_R(
companyId, rendererKey, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the fragment entry links where companyId = ? and rendererKey = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryLinkModelImpl
.
*
*
* @param companyId the company ID
* @param rendererKey the renderer key
* @param start the lower bound of the range of fragment entry links
* @param end the upper bound of the range of fragment entry links (not inclusive)
* @return the range of matching fragment entry links
*/
@Override
public List findByC_R(
long companyId, String rendererKey, int start, int end) {
return findByC_R(companyId, rendererKey, start, end, null);
}
/**
* Returns an ordered range of all the fragment entry links where companyId = ? and rendererKey = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryLinkModelImpl
.
*
*
* @param companyId the company ID
* @param rendererKey the renderer key
* @param start the lower bound of the range of fragment entry links
* @param end the upper bound of the range of fragment entry links (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entry links
*/
@Override
public List findByC_R(
long companyId, String rendererKey, int start, int end,
OrderByComparator orderByComparator) {
return findByC_R(
companyId, rendererKey, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the fragment entry links where companyId = ? and rendererKey = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryLinkModelImpl
.
*
*
* @param companyId the company ID
* @param rendererKey the renderer key
* @param start the lower bound of the range of fragment entry links
* @param end the upper bound of the range of fragment entry links (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching fragment entry links
*/
@Override
public List findByC_R(
long companyId, String rendererKey, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
try (SafeCloseable safeCloseable =
ctPersistenceHelper.setCTCollectionIdWithSafeCloseable(
FragmentEntryLink.class)) {
rendererKey = Objects.toString(rendererKey, "");
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache) {
finderPath = _finderPathWithoutPaginationFindByC_R;
finderArgs = new Object[] {companyId, rendererKey};
}
}
else if (useFinderCache) {
finderPath = _finderPathWithPaginationFindByC_R;
finderArgs = new Object[] {
companyId, rendererKey, start, end, orderByComparator
};
}
List list = null;
if (useFinderCache) {
list = (List)finderCache.getResult(
finderPath, finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (FragmentEntryLink fragmentEntryLink : list) {
if ((companyId != fragmentEntryLink.getCompanyId()) ||
!rendererKey.equals(
fragmentEntryLink.getRendererKey())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_FRAGMENTENTRYLINK_WHERE);
sb.append(_FINDER_COLUMN_C_R_COMPANYID_2);
boolean bindRendererKey = false;
if (rendererKey.isEmpty()) {
sb.append(_FINDER_COLUMN_C_R_RENDERERKEY_3);
}
else {
bindRendererKey = true;
sb.append(_FINDER_COLUMN_C_R_RENDERERKEY_2);
}
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(FragmentEntryLinkModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(companyId);
if (bindRendererKey) {
queryPos.add(rendererKey);
}
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
}
/**
* Returns the first fragment entry link in the ordered set where companyId = ? and rendererKey = ?.
*
* @param companyId the company ID
* @param rendererKey the renderer key
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry link
* @throws NoSuchEntryLinkException if a matching fragment entry link could not be found
*/
@Override
public FragmentEntryLink findByC_R_First(
long companyId, String rendererKey,
OrderByComparator orderByComparator)
throws NoSuchEntryLinkException {
FragmentEntryLink fragmentEntryLink = fetchByC_R_First(
companyId, rendererKey, orderByComparator);
if (fragmentEntryLink != null) {
return fragmentEntryLink;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("companyId=");
sb.append(companyId);
sb.append(", rendererKey=");
sb.append(rendererKey);
sb.append("}");
throw new NoSuchEntryLinkException(sb.toString());
}
/**
* Returns the first fragment entry link in the ordered set where companyId = ? and rendererKey = ?.
*
* @param companyId the company ID
* @param rendererKey the renderer key
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry link, or null
if a matching fragment entry link could not be found
*/
@Override
public FragmentEntryLink fetchByC_R_First(
long companyId, String rendererKey,
OrderByComparator orderByComparator) {
List list = findByC_R(
companyId, rendererKey, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last fragment entry link in the ordered set where companyId = ? and rendererKey = ?.
*
* @param companyId the company ID
* @param rendererKey the renderer key
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry link
* @throws NoSuchEntryLinkException if a matching fragment entry link could not be found
*/
@Override
public FragmentEntryLink findByC_R_Last(
long companyId, String rendererKey,
OrderByComparator orderByComparator)
throws NoSuchEntryLinkException {
FragmentEntryLink fragmentEntryLink = fetchByC_R_Last(
companyId, rendererKey, orderByComparator);
if (fragmentEntryLink != null) {
return fragmentEntryLink;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("companyId=");
sb.append(companyId);
sb.append(", rendererKey=");
sb.append(rendererKey);
sb.append("}");
throw new NoSuchEntryLinkException(sb.toString());
}
/**
* Returns the last fragment entry link in the ordered set where companyId = ? and rendererKey = ?.
*
* @param companyId the company ID
* @param rendererKey the renderer key
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry link, or null
if a matching fragment entry link could not be found
*/
@Override
public FragmentEntryLink fetchByC_R_Last(
long companyId, String rendererKey,
OrderByComparator orderByComparator) {
int count = countByC_R(companyId, rendererKey);
if (count == 0) {
return null;
}
List list = findByC_R(
companyId, rendererKey, count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the fragment entry links before and after the current fragment entry link in the ordered set where companyId = ? and rendererKey = ?.
*
* @param fragmentEntryLinkId the primary key of the current fragment entry link
* @param companyId the company ID
* @param rendererKey the renderer key
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next fragment entry link
* @throws NoSuchEntryLinkException if a fragment entry link with the primary key could not be found
*/
@Override
public FragmentEntryLink[] findByC_R_PrevAndNext(
long fragmentEntryLinkId, long companyId, String rendererKey,
OrderByComparator orderByComparator)
throws NoSuchEntryLinkException {
rendererKey = Objects.toString(rendererKey, "");
FragmentEntryLink fragmentEntryLink = findByPrimaryKey(
fragmentEntryLinkId);
Session session = null;
try {
session = openSession();
FragmentEntryLink[] array = new FragmentEntryLinkImpl[3];
array[0] = getByC_R_PrevAndNext(
session, fragmentEntryLink, companyId, rendererKey,
orderByComparator, true);
array[1] = fragmentEntryLink;
array[2] = getByC_R_PrevAndNext(
session, fragmentEntryLink, companyId, rendererKey,
orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected FragmentEntryLink getByC_R_PrevAndNext(
Session session, FragmentEntryLink fragmentEntryLink, long companyId,
String rendererKey,
OrderByComparator orderByComparator,
boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
5 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_FRAGMENTENTRYLINK_WHERE);
sb.append(_FINDER_COLUMN_C_R_COMPANYID_2);
boolean bindRendererKey = false;
if (rendererKey.isEmpty()) {
sb.append(_FINDER_COLUMN_C_R_RENDERERKEY_3);
}
else {
bindRendererKey = true;
sb.append(_FINDER_COLUMN_C_R_RENDERERKEY_2);
}
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(FragmentEntryLinkModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(companyId);
if (bindRendererKey) {
queryPos.add(rendererKey);
}
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
fragmentEntryLink)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Returns all the fragment entry links where companyId = ? and rendererKey = any ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryLinkModelImpl
.
*
*
* @param companyId the company ID
* @param rendererKeys the renderer keys
* @return the matching fragment entry links
*/
@Override
public List findByC_R(
long companyId, String[] rendererKeys) {
return findByC_R(
companyId, rendererKeys, QueryUtil.ALL_POS, QueryUtil.ALL_POS,
null);
}
/**
* Returns a range of all the fragment entry links where companyId = ? and rendererKey = any ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryLinkModelImpl
.
*
*
* @param companyId the company ID
* @param rendererKeys the renderer keys
* @param start the lower bound of the range of fragment entry links
* @param end the upper bound of the range of fragment entry links (not inclusive)
* @return the range of matching fragment entry links
*/
@Override
public List findByC_R(
long companyId, String[] rendererKeys, int start, int end) {
return findByC_R(companyId, rendererKeys, start, end, null);
}
/**
* Returns an ordered range of all the fragment entry links where companyId = ? and rendererKey = any ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryLinkModelImpl
.
*
*
* @param companyId the company ID
* @param rendererKeys the renderer keys
* @param start the lower bound of the range of fragment entry links
* @param end the upper bound of the range of fragment entry links (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entry links
*/
@Override
public List findByC_R(
long companyId, String[] rendererKeys, int start, int end,
OrderByComparator orderByComparator) {
return findByC_R(
companyId, rendererKeys, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the fragment entry links where companyId = ? and rendererKey = ?, optionally using the finder cache.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryLinkModelImpl
.
*
*
* @param companyId the company ID
* @param rendererKeys the renderer keys
* @param start the lower bound of the range of fragment entry links
* @param end the upper bound of the range of fragment entry links (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching fragment entry links
*/
@Override
public List findByC_R(
long companyId, String[] rendererKeys, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
if (rendererKeys == null) {
rendererKeys = new String[0];
}
else if (rendererKeys.length > 1) {
for (int i = 0; i < rendererKeys.length; i++) {
rendererKeys[i] = Objects.toString(rendererKeys[i], "");
}
rendererKeys = ArrayUtil.sortedUnique(rendererKeys);
}
if (rendererKeys.length == 1) {
return findByC_R(
companyId, rendererKeys[0], start, end, orderByComparator);
}
try (SafeCloseable safeCloseable =
ctPersistenceHelper.setCTCollectionIdWithSafeCloseable(
FragmentEntryLink.class)) {
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache) {
finderArgs = new Object[] {
companyId, StringUtil.merge(rendererKeys)
};
}
}
else if (useFinderCache) {
finderArgs = new Object[] {
companyId, StringUtil.merge(rendererKeys), start, end,
orderByComparator
};
}
List list = null;
if (useFinderCache) {
list = (List)finderCache.getResult(
_finderPathWithPaginationFindByC_R, finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (FragmentEntryLink fragmentEntryLink : list) {
if ((companyId != fragmentEntryLink.getCompanyId()) ||
!ArrayUtil.contains(
rendererKeys,
fragmentEntryLink.getRendererKey())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = new StringBundler();
sb.append(_SQL_SELECT_FRAGMENTENTRYLINK_WHERE);
sb.append(_FINDER_COLUMN_C_R_COMPANYID_2);
if (rendererKeys.length > 0) {
sb.append("(");
for (int i = 0; i < rendererKeys.length; i++) {
String rendererKey = rendererKeys[i];
if (rendererKey.isEmpty()) {
sb.append(_FINDER_COLUMN_C_R_RENDERERKEY_3);
}
else {
sb.append(_FINDER_COLUMN_C_R_RENDERERKEY_2);
}
if ((i + 1) < rendererKeys.length) {
sb.append(WHERE_OR);
}
}
sb.append(")");
}
sb.setStringAt(
removeConjunction(sb.stringAt(sb.index() - 1)),
sb.index() - 1);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(FragmentEntryLinkModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(companyId);
for (String rendererKey : rendererKeys) {
if ((rendererKey != null) && !rendererKey.isEmpty()) {
queryPos.add(rendererKey);
}
}
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache) {
finderCache.putResult(
_finderPathWithPaginationFindByC_R, finderArgs,
list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
}
/**
* Removes all the fragment entry links where companyId = ? and rendererKey = ? from the database.
*
* @param companyId the company ID
* @param rendererKey the renderer key
*/
@Override
public void removeByC_R(long companyId, String rendererKey) {
for (FragmentEntryLink fragmentEntryLink :
findByC_R(
companyId, rendererKey, QueryUtil.ALL_POS,
QueryUtil.ALL_POS, null)) {
remove(fragmentEntryLink);
}
}
/**
* Returns the number of fragment entry links where companyId = ? and rendererKey = ?.
*
* @param companyId the company ID
* @param rendererKey the renderer key
* @return the number of matching fragment entry links
*/
@Override
public int countByC_R(long companyId, String rendererKey) {
try (SafeCloseable safeCloseable =
ctPersistenceHelper.setCTCollectionIdWithSafeCloseable(
FragmentEntryLink.class)) {
rendererKey = Objects.toString(rendererKey, "");
FinderPath finderPath = _finderPathCountByC_R;
Object[] finderArgs = new Object[] {companyId, rendererKey};
Long count = (Long)finderCache.getResult(
finderPath, finderArgs, this);
if (count == null) {
StringBundler sb = new StringBundler(3);
sb.append(_SQL_COUNT_FRAGMENTENTRYLINK_WHERE);
sb.append(_FINDER_COLUMN_C_R_COMPANYID_2);
boolean bindRendererKey = false;
if (rendererKey.isEmpty()) {
sb.append(_FINDER_COLUMN_C_R_RENDERERKEY_3);
}
else {
bindRendererKey = true;
sb.append(_FINDER_COLUMN_C_R_RENDERERKEY_2);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(companyId);
if (bindRendererKey) {
queryPos.add(rendererKey);
}
count = (Long)query.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
}
/**
* Returns the number of fragment entry links where companyId = ? and rendererKey = any ?.
*
* @param companyId the company ID
* @param rendererKeys the renderer keys
* @return the number of matching fragment entry links
*/
@Override
public int countByC_R(long companyId, String[] rendererKeys) {
if (rendererKeys == null) {
rendererKeys = new String[0];
}
else if (rendererKeys.length > 1) {
for (int i = 0; i < rendererKeys.length; i++) {
rendererKeys[i] = Objects.toString(rendererKeys[i], "");
}
rendererKeys = ArrayUtil.sortedUnique(rendererKeys);
}
try (SafeCloseable safeCloseable =
ctPersistenceHelper.setCTCollectionIdWithSafeCloseable(
FragmentEntryLink.class)) {
Object[] finderArgs = new Object[] {
companyId, StringUtil.merge(rendererKeys)
};
Long count = (Long)finderCache.getResult(
_finderPathWithPaginationCountByC_R, finderArgs, this);
if (count == null) {
StringBundler sb = new StringBundler();
sb.append(_SQL_COUNT_FRAGMENTENTRYLINK_WHERE);
sb.append(_FINDER_COLUMN_C_R_COMPANYID_2);
if (rendererKeys.length > 0) {
sb.append("(");
for (int i = 0; i < rendererKeys.length; i++) {
String rendererKey = rendererKeys[i];
if (rendererKey.isEmpty()) {
sb.append(_FINDER_COLUMN_C_R_RENDERERKEY_3);
}
else {
sb.append(_FINDER_COLUMN_C_R_RENDERERKEY_2);
}
if ((i + 1) < rendererKeys.length) {
sb.append(WHERE_OR);
}
}
sb.append(")");
}
sb.setStringAt(
removeConjunction(sb.stringAt(sb.index() - 1)),
sb.index() - 1);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(companyId);
for (String rendererKey : rendererKeys) {
if ((rendererKey != null) && !rendererKey.isEmpty()) {
queryPos.add(rendererKey);
}
}
count = (Long)query.uniqueResult();
finderCache.putResult(
_finderPathWithPaginationCountByC_R, finderArgs, count);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
}
private static final String _FINDER_COLUMN_C_R_COMPANYID_2 =
"fragmentEntryLink.companyId = ? AND ";
private static final String _FINDER_COLUMN_C_R_RENDERERKEY_2 =
"fragmentEntryLink.rendererKey = ?";
private static final String _FINDER_COLUMN_C_R_RENDERERKEY_3 =
"(fragmentEntryLink.rendererKey IS NULL OR fragmentEntryLink.rendererKey = '')";
private FinderPath _finderPathWithPaginationFindByF_D;
private FinderPath _finderPathWithoutPaginationFindByF_D;
private FinderPath _finderPathCountByF_D;
private FinderPath _finderPathWithPaginationCountByF_D;
/**
* Returns all the fragment entry links where fragmentEntryId = ? and deleted = ?.
*
* @param fragmentEntryId the fragment entry ID
* @param deleted the deleted
* @return the matching fragment entry links
*/
@Override
public List findByF_D(
long fragmentEntryId, boolean deleted) {
return findByF_D(
fragmentEntryId, deleted, QueryUtil.ALL_POS, QueryUtil.ALL_POS,
null);
}
/**
* Returns a range of all the fragment entry links where fragmentEntryId = ? and deleted = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryLinkModelImpl
.
*
*
* @param fragmentEntryId the fragment entry ID
* @param deleted the deleted
* @param start the lower bound of the range of fragment entry links
* @param end the upper bound of the range of fragment entry links (not inclusive)
* @return the range of matching fragment entry links
*/
@Override
public List findByF_D(
long fragmentEntryId, boolean deleted, int start, int end) {
return findByF_D(fragmentEntryId, deleted, start, end, null);
}
/**
* Returns an ordered range of all the fragment entry links where fragmentEntryId = ? and deleted = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryLinkModelImpl
.
*
*
* @param fragmentEntryId the fragment entry ID
* @param deleted the deleted
* @param start the lower bound of the range of fragment entry links
* @param end the upper bound of the range of fragment entry links (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entry links
*/
@Override
public List findByF_D(
long fragmentEntryId, boolean deleted, int start, int end,
OrderByComparator orderByComparator) {
return findByF_D(
fragmentEntryId, deleted, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the fragment entry links where fragmentEntryId = ? and deleted = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryLinkModelImpl
.
*
*
* @param fragmentEntryId the fragment entry ID
* @param deleted the deleted
* @param start the lower bound of the range of fragment entry links
* @param end the upper bound of the range of fragment entry links (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching fragment entry links
*/
@Override
public List findByF_D(
long fragmentEntryId, boolean deleted, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
try (SafeCloseable safeCloseable =
ctPersistenceHelper.setCTCollectionIdWithSafeCloseable(
FragmentEntryLink.class)) {
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache) {
finderPath = _finderPathWithoutPaginationFindByF_D;
finderArgs = new Object[] {fragmentEntryId, deleted};
}
}
else if (useFinderCache) {
finderPath = _finderPathWithPaginationFindByF_D;
finderArgs = new Object[] {
fragmentEntryId, deleted, start, end, orderByComparator
};
}
List list = null;
if (useFinderCache) {
list = (List)finderCache.getResult(
finderPath, finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (FragmentEntryLink fragmentEntryLink : list) {
if ((fragmentEntryId !=
fragmentEntryLink.getFragmentEntryId()) ||
(deleted != fragmentEntryLink.isDeleted())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_FRAGMENTENTRYLINK_WHERE);
sb.append(_FINDER_COLUMN_F_D_FRAGMENTENTRYID_2);
sb.append(_FINDER_COLUMN_F_D_DELETED_2);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(FragmentEntryLinkModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(fragmentEntryId);
queryPos.add(deleted);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
}
/**
* Returns the first fragment entry link in the ordered set where fragmentEntryId = ? and deleted = ?.
*
* @param fragmentEntryId the fragment entry ID
* @param deleted the deleted
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry link
* @throws NoSuchEntryLinkException if a matching fragment entry link could not be found
*/
@Override
public FragmentEntryLink findByF_D_First(
long fragmentEntryId, boolean deleted,
OrderByComparator orderByComparator)
throws NoSuchEntryLinkException {
FragmentEntryLink fragmentEntryLink = fetchByF_D_First(
fragmentEntryId, deleted, orderByComparator);
if (fragmentEntryLink != null) {
return fragmentEntryLink;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("fragmentEntryId=");
sb.append(fragmentEntryId);
sb.append(", deleted=");
sb.append(deleted);
sb.append("}");
throw new NoSuchEntryLinkException(sb.toString());
}
/**
* Returns the first fragment entry link in the ordered set where fragmentEntryId = ? and deleted = ?.
*
* @param fragmentEntryId the fragment entry ID
* @param deleted the deleted
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry link, or null
if a matching fragment entry link could not be found
*/
@Override
public FragmentEntryLink fetchByF_D_First(
long fragmentEntryId, boolean deleted,
OrderByComparator orderByComparator) {
List list = findByF_D(
fragmentEntryId, deleted, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last fragment entry link in the ordered set where fragmentEntryId = ? and deleted = ?.
*
* @param fragmentEntryId the fragment entry ID
* @param deleted the deleted
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry link
* @throws NoSuchEntryLinkException if a matching fragment entry link could not be found
*/
@Override
public FragmentEntryLink findByF_D_Last(
long fragmentEntryId, boolean deleted,
OrderByComparator orderByComparator)
throws NoSuchEntryLinkException {
FragmentEntryLink fragmentEntryLink = fetchByF_D_Last(
fragmentEntryId, deleted, orderByComparator);
if (fragmentEntryLink != null) {
return fragmentEntryLink;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("fragmentEntryId=");
sb.append(fragmentEntryId);
sb.append(", deleted=");
sb.append(deleted);
sb.append("}");
throw new NoSuchEntryLinkException(sb.toString());
}
/**
* Returns the last fragment entry link in the ordered set where fragmentEntryId = ? and deleted = ?.
*
* @param fragmentEntryId the fragment entry ID
* @param deleted the deleted
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry link, or null
if a matching fragment entry link could not be found
*/
@Override
public FragmentEntryLink fetchByF_D_Last(
long fragmentEntryId, boolean deleted,
OrderByComparator orderByComparator) {
int count = countByF_D(fragmentEntryId, deleted);
if (count == 0) {
return null;
}
List list = findByF_D(
fragmentEntryId, deleted, count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the fragment entry links before and after the current fragment entry link in the ordered set where fragmentEntryId = ? and deleted = ?.
*
* @param fragmentEntryLinkId the primary key of the current fragment entry link
* @param fragmentEntryId the fragment entry ID
* @param deleted the deleted
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next fragment entry link
* @throws NoSuchEntryLinkException if a fragment entry link with the primary key could not be found
*/
@Override
public FragmentEntryLink[] findByF_D_PrevAndNext(
long fragmentEntryLinkId, long fragmentEntryId, boolean deleted,
OrderByComparator orderByComparator)
throws NoSuchEntryLinkException {
FragmentEntryLink fragmentEntryLink = findByPrimaryKey(
fragmentEntryLinkId);
Session session = null;
try {
session = openSession();
FragmentEntryLink[] array = new FragmentEntryLinkImpl[3];
array[0] = getByF_D_PrevAndNext(
session, fragmentEntryLink, fragmentEntryId, deleted,
orderByComparator, true);
array[1] = fragmentEntryLink;
array[2] = getByF_D_PrevAndNext(
session, fragmentEntryLink, fragmentEntryId, deleted,
orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected FragmentEntryLink getByF_D_PrevAndNext(
Session session, FragmentEntryLink fragmentEntryLink,
long fragmentEntryId, boolean deleted,
OrderByComparator orderByComparator,
boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
5 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_FRAGMENTENTRYLINK_WHERE);
sb.append(_FINDER_COLUMN_F_D_FRAGMENTENTRYID_2);
sb.append(_FINDER_COLUMN_F_D_DELETED_2);
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(FragmentEntryLinkModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(fragmentEntryId);
queryPos.add(deleted);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
fragmentEntryLink)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Returns all the fragment entry links where fragmentEntryId = any ? and deleted = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryLinkModelImpl
.
*
*
* @param fragmentEntryIds the fragment entry IDs
* @param deleted the deleted
* @return the matching fragment entry links
*/
@Override
public List findByF_D(
long[] fragmentEntryIds, boolean deleted) {
return findByF_D(
fragmentEntryIds, deleted, QueryUtil.ALL_POS, QueryUtil.ALL_POS,
null);
}
/**
* Returns a range of all the fragment entry links where fragmentEntryId = any ? and deleted = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryLinkModelImpl
.
*
*
* @param fragmentEntryIds the fragment entry IDs
* @param deleted the deleted
* @param start the lower bound of the range of fragment entry links
* @param end the upper bound of the range of fragment entry links (not inclusive)
* @return the range of matching fragment entry links
*/
@Override
public List findByF_D(
long[] fragmentEntryIds, boolean deleted, int start, int end) {
return findByF_D(fragmentEntryIds, deleted, start, end, null);
}
/**
* Returns an ordered range of all the fragment entry links where fragmentEntryId = any ? and deleted = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryLinkModelImpl
.
*
*
* @param fragmentEntryIds the fragment entry IDs
* @param deleted the deleted
* @param start the lower bound of the range of fragment entry links
* @param end the upper bound of the range of fragment entry links (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entry links
*/
@Override
public List findByF_D(
long[] fragmentEntryIds, boolean deleted, int start, int end,
OrderByComparator orderByComparator) {
return findByF_D(
fragmentEntryIds, deleted, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the fragment entry links where fragmentEntryId = ? and deleted = ?, optionally using the finder cache.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryLinkModelImpl
.
*
*
* @param fragmentEntryIds the fragment entry IDs
* @param deleted the deleted
* @param start the lower bound of the range of fragment entry links
* @param end the upper bound of the range of fragment entry links (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching fragment entry links
*/
@Override
public List findByF_D(
long[] fragmentEntryIds, boolean deleted, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
if (fragmentEntryIds == null) {
fragmentEntryIds = new long[0];
}
else if (fragmentEntryIds.length > 1) {
fragmentEntryIds = ArrayUtil.sortedUnique(fragmentEntryIds);
}
if (fragmentEntryIds.length == 1) {
return findByF_D(
fragmentEntryIds[0], deleted, start, end, orderByComparator);
}
try (SafeCloseable safeCloseable =
ctPersistenceHelper.setCTCollectionIdWithSafeCloseable(
FragmentEntryLink.class)) {
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache) {
finderArgs = new Object[] {
StringUtil.merge(fragmentEntryIds), deleted
};
}
}
else if (useFinderCache) {
finderArgs = new Object[] {
StringUtil.merge(fragmentEntryIds), deleted, start, end,
orderByComparator
};
}
List list = null;
if (useFinderCache) {
list = (List)finderCache.getResult(
_finderPathWithPaginationFindByF_D, finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (FragmentEntryLink fragmentEntryLink : list) {
if (!ArrayUtil.contains(
fragmentEntryIds,
fragmentEntryLink.getFragmentEntryId()) ||
(deleted != fragmentEntryLink.isDeleted())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = new StringBundler();
sb.append(_SQL_SELECT_FRAGMENTENTRYLINK_WHERE);
if (fragmentEntryIds.length > 0) {
sb.append("(");
sb.append(_FINDER_COLUMN_F_D_FRAGMENTENTRYID_7);
sb.append(StringUtil.merge(fragmentEntryIds));
sb.append(")");
sb.append(")");
sb.append(WHERE_AND);
}
sb.append(_FINDER_COLUMN_F_D_DELETED_2);
sb.setStringAt(
removeConjunction(sb.stringAt(sb.index() - 1)),
sb.index() - 1);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(FragmentEntryLinkModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(deleted);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache) {
finderCache.putResult(
_finderPathWithPaginationFindByF_D, finderArgs,
list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
}
/**
* Removes all the fragment entry links where fragmentEntryId = ? and deleted = ? from the database.
*
* @param fragmentEntryId the fragment entry ID
* @param deleted the deleted
*/
@Override
public void removeByF_D(long fragmentEntryId, boolean deleted) {
for (FragmentEntryLink fragmentEntryLink :
findByF_D(
fragmentEntryId, deleted, QueryUtil.ALL_POS,
QueryUtil.ALL_POS, null)) {
remove(fragmentEntryLink);
}
}
/**
* Returns the number of fragment entry links where fragmentEntryId = ? and deleted = ?.
*
* @param fragmentEntryId the fragment entry ID
* @param deleted the deleted
* @return the number of matching fragment entry links
*/
@Override
public int countByF_D(long fragmentEntryId, boolean deleted) {
try (SafeCloseable safeCloseable =
ctPersistenceHelper.setCTCollectionIdWithSafeCloseable(
FragmentEntryLink.class)) {
FinderPath finderPath = _finderPathCountByF_D;
Object[] finderArgs = new Object[] {fragmentEntryId, deleted};
Long count = (Long)finderCache.getResult(
finderPath, finderArgs, this);
if (count == null) {
StringBundler sb = new StringBundler(3);
sb.append(_SQL_COUNT_FRAGMENTENTRYLINK_WHERE);
sb.append(_FINDER_COLUMN_F_D_FRAGMENTENTRYID_2);
sb.append(_FINDER_COLUMN_F_D_DELETED_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(fragmentEntryId);
queryPos.add(deleted);
count = (Long)query.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
}
/**
* Returns the number of fragment entry links where fragmentEntryId = any ? and deleted = ?.
*
* @param fragmentEntryIds the fragment entry IDs
* @param deleted the deleted
* @return the number of matching fragment entry links
*/
@Override
public int countByF_D(long[] fragmentEntryIds, boolean deleted) {
if (fragmentEntryIds == null) {
fragmentEntryIds = new long[0];
}
else if (fragmentEntryIds.length > 1) {
fragmentEntryIds = ArrayUtil.sortedUnique(fragmentEntryIds);
}
try (SafeCloseable safeCloseable =
ctPersistenceHelper.setCTCollectionIdWithSafeCloseable(
FragmentEntryLink.class)) {
Object[] finderArgs = new Object[] {
StringUtil.merge(fragmentEntryIds), deleted
};
Long count = (Long)finderCache.getResult(
_finderPathWithPaginationCountByF_D, finderArgs, this);
if (count == null) {
StringBundler sb = new StringBundler();
sb.append(_SQL_COUNT_FRAGMENTENTRYLINK_WHERE);
if (fragmentEntryIds.length > 0) {
sb.append("(");
sb.append(_FINDER_COLUMN_F_D_FRAGMENTENTRYID_7);
sb.append(StringUtil.merge(fragmentEntryIds));
sb.append(")");
sb.append(")");
sb.append(WHERE_AND);
}
sb.append(_FINDER_COLUMN_F_D_DELETED_2);
sb.setStringAt(
removeConjunction(sb.stringAt(sb.index() - 1)),
sb.index() - 1);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(deleted);
count = (Long)query.uniqueResult();
finderCache.putResult(
_finderPathWithPaginationCountByF_D, finderArgs, count);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
}
private static final String _FINDER_COLUMN_F_D_FRAGMENTENTRYID_2 =
"fragmentEntryLink.fragmentEntryId = ? AND ";
private static final String _FINDER_COLUMN_F_D_FRAGMENTENTRYID_7 =
"fragmentEntryLink.fragmentEntryId IN (";
private static final String _FINDER_COLUMN_F_D_DELETED_2 =
"fragmentEntryLink.deleted = ?";
private FinderPath _finderPathWithPaginationFindByG_OFELI_P;
private FinderPath _finderPathWithoutPaginationFindByG_OFELI_P;
private FinderPath _finderPathCountByG_OFELI_P;
/**
* Returns all the fragment entry links where groupId = ? and originalFragmentEntryLinkId = ? and plid = ?.
*
* @param groupId the group ID
* @param originalFragmentEntryLinkId the original fragment entry link ID
* @param plid the plid
* @return the matching fragment entry links
*/
@Override
public List findByG_OFELI_P(
long groupId, long originalFragmentEntryLinkId, long plid) {
return findByG_OFELI_P(
groupId, originalFragmentEntryLinkId, plid, QueryUtil.ALL_POS,
QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the fragment entry links where groupId = ? and originalFragmentEntryLinkId = ? and plid = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryLinkModelImpl
.
*
*
* @param groupId the group ID
* @param originalFragmentEntryLinkId the original fragment entry link ID
* @param plid the plid
* @param start the lower bound of the range of fragment entry links
* @param end the upper bound of the range of fragment entry links (not inclusive)
* @return the range of matching fragment entry links
*/
@Override
public List findByG_OFELI_P(
long groupId, long originalFragmentEntryLinkId, long plid, int start,
int end) {
return findByG_OFELI_P(
groupId, originalFragmentEntryLinkId, plid, start, end, null);
}
/**
* Returns an ordered range of all the fragment entry links where groupId = ? and originalFragmentEntryLinkId = ? and plid = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryLinkModelImpl
.
*
*
* @param groupId the group ID
* @param originalFragmentEntryLinkId the original fragment entry link ID
* @param plid the plid
* @param start the lower bound of the range of fragment entry links
* @param end the upper bound of the range of fragment entry links (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entry links
*/
@Override
public List findByG_OFELI_P(
long groupId, long originalFragmentEntryLinkId, long plid, int start,
int end, OrderByComparator orderByComparator) {
return findByG_OFELI_P(
groupId, originalFragmentEntryLinkId, plid, start, end,
orderByComparator, true);
}
/**
* Returns an ordered range of all the fragment entry links where groupId = ? and originalFragmentEntryLinkId = ? and plid = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryLinkModelImpl
.
*
*
* @param groupId the group ID
* @param originalFragmentEntryLinkId the original fragment entry link ID
* @param plid the plid
* @param start the lower bound of the range of fragment entry links
* @param end the upper bound of the range of fragment entry links (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching fragment entry links
*/
@Override
public List findByG_OFELI_P(
long groupId, long originalFragmentEntryLinkId, long plid, int start,
int end, OrderByComparator orderByComparator,
boolean useFinderCache) {
try (SafeCloseable safeCloseable =
ctPersistenceHelper.setCTCollectionIdWithSafeCloseable(
FragmentEntryLink.class)) {
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache) {
finderPath = _finderPathWithoutPaginationFindByG_OFELI_P;
finderArgs = new Object[] {
groupId, originalFragmentEntryLinkId, plid
};
}
}
else if (useFinderCache) {
finderPath = _finderPathWithPaginationFindByG_OFELI_P;
finderArgs = new Object[] {
groupId, originalFragmentEntryLinkId, plid, start, end,
orderByComparator
};
}
List list = null;
if (useFinderCache) {
list = (List)finderCache.getResult(
finderPath, finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (FragmentEntryLink fragmentEntryLink : list) {
if ((groupId != fragmentEntryLink.getGroupId()) ||
(originalFragmentEntryLinkId !=
fragmentEntryLink.
getOriginalFragmentEntryLinkId()) ||
(plid != fragmentEntryLink.getPlid())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
5 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(5);
}
sb.append(_SQL_SELECT_FRAGMENTENTRYLINK_WHERE);
sb.append(_FINDER_COLUMN_G_OFELI_P_GROUPID_2);
sb.append(
_FINDER_COLUMN_G_OFELI_P_ORIGINALFRAGMENTENTRYLINKID_2);
sb.append(_FINDER_COLUMN_G_OFELI_P_PLID_2);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(FragmentEntryLinkModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
queryPos.add(originalFragmentEntryLinkId);
queryPos.add(plid);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
}
/**
* Returns the first fragment entry link in the ordered set where groupId = ? and originalFragmentEntryLinkId = ? and plid = ?.
*
* @param groupId the group ID
* @param originalFragmentEntryLinkId the original fragment entry link ID
* @param plid the plid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry link
* @throws NoSuchEntryLinkException if a matching fragment entry link could not be found
*/
@Override
public FragmentEntryLink findByG_OFELI_P_First(
long groupId, long originalFragmentEntryLinkId, long plid,
OrderByComparator orderByComparator)
throws NoSuchEntryLinkException {
FragmentEntryLink fragmentEntryLink = fetchByG_OFELI_P_First(
groupId, originalFragmentEntryLinkId, plid, orderByComparator);
if (fragmentEntryLink != null) {
return fragmentEntryLink;
}
StringBundler sb = new StringBundler(8);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("groupId=");
sb.append(groupId);
sb.append(", originalFragmentEntryLinkId=");
sb.append(originalFragmentEntryLinkId);
sb.append(", plid=");
sb.append(plid);
sb.append("}");
throw new NoSuchEntryLinkException(sb.toString());
}
/**
* Returns the first fragment entry link in the ordered set where groupId = ? and originalFragmentEntryLinkId = ? and plid = ?.
*
* @param groupId the group ID
* @param originalFragmentEntryLinkId the original fragment entry link ID
* @param plid the plid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry link, or null
if a matching fragment entry link could not be found
*/
@Override
public FragmentEntryLink fetchByG_OFELI_P_First(
long groupId, long originalFragmentEntryLinkId, long plid,
OrderByComparator orderByComparator) {
List list = findByG_OFELI_P(
groupId, originalFragmentEntryLinkId, plid, 0, 1,
orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last fragment entry link in the ordered set where groupId = ? and originalFragmentEntryLinkId = ? and plid = ?.
*
* @param groupId the group ID
* @param originalFragmentEntryLinkId the original fragment entry link ID
* @param plid the plid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry link
* @throws NoSuchEntryLinkException if a matching fragment entry link could not be found
*/
@Override
public FragmentEntryLink findByG_OFELI_P_Last(
long groupId, long originalFragmentEntryLinkId, long plid,
OrderByComparator orderByComparator)
throws NoSuchEntryLinkException {
FragmentEntryLink fragmentEntryLink = fetchByG_OFELI_P_Last(
groupId, originalFragmentEntryLinkId, plid, orderByComparator);
if (fragmentEntryLink != null) {
return fragmentEntryLink;
}
StringBundler sb = new StringBundler(8);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("groupId=");
sb.append(groupId);
sb.append(", originalFragmentEntryLinkId=");
sb.append(originalFragmentEntryLinkId);
sb.append(", plid=");
sb.append(plid);
sb.append("}");
throw new NoSuchEntryLinkException(sb.toString());
}
/**
* Returns the last fragment entry link in the ordered set where groupId = ? and originalFragmentEntryLinkId = ? and plid = ?.
*
* @param groupId the group ID
* @param originalFragmentEntryLinkId the original fragment entry link ID
* @param plid the plid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry link, or null
if a matching fragment entry link could not be found
*/
@Override
public FragmentEntryLink fetchByG_OFELI_P_Last(
long groupId, long originalFragmentEntryLinkId, long plid,
OrderByComparator orderByComparator) {
int count = countByG_OFELI_P(
groupId, originalFragmentEntryLinkId, plid);
if (count == 0) {
return null;
}
List list = findByG_OFELI_P(
groupId, originalFragmentEntryLinkId, plid, count - 1, count,
orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the fragment entry links before and after the current fragment entry link in the ordered set where groupId = ? and originalFragmentEntryLinkId = ? and plid = ?.
*
* @param fragmentEntryLinkId the primary key of the current fragment entry link
* @param groupId the group ID
* @param originalFragmentEntryLinkId the original fragment entry link ID
* @param plid the plid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next fragment entry link
* @throws NoSuchEntryLinkException if a fragment entry link with the primary key could not be found
*/
@Override
public FragmentEntryLink[] findByG_OFELI_P_PrevAndNext(
long fragmentEntryLinkId, long groupId,
long originalFragmentEntryLinkId, long plid,
OrderByComparator orderByComparator)
throws NoSuchEntryLinkException {
FragmentEntryLink fragmentEntryLink = findByPrimaryKey(
fragmentEntryLinkId);
Session session = null;
try {
session = openSession();
FragmentEntryLink[] array = new FragmentEntryLinkImpl[3];
array[0] = getByG_OFELI_P_PrevAndNext(
session, fragmentEntryLink, groupId,
originalFragmentEntryLinkId, plid, orderByComparator, true);
array[1] = fragmentEntryLink;
array[2] = getByG_OFELI_P_PrevAndNext(
session, fragmentEntryLink, groupId,
originalFragmentEntryLinkId, plid, orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected FragmentEntryLink getByG_OFELI_P_PrevAndNext(
Session session, FragmentEntryLink fragmentEntryLink, long groupId,
long originalFragmentEntryLinkId, long plid,
OrderByComparator orderByComparator,
boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
6 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(5);
}
sb.append(_SQL_SELECT_FRAGMENTENTRYLINK_WHERE);
sb.append(_FINDER_COLUMN_G_OFELI_P_GROUPID_2);
sb.append(_FINDER_COLUMN_G_OFELI_P_ORIGINALFRAGMENTENTRYLINKID_2);
sb.append(_FINDER_COLUMN_G_OFELI_P_PLID_2);
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(FragmentEntryLinkModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
queryPos.add(originalFragmentEntryLinkId);
queryPos.add(plid);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
fragmentEntryLink)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the fragment entry links where groupId = ? and originalFragmentEntryLinkId = ? and plid = ? from the database.
*
* @param groupId the group ID
* @param originalFragmentEntryLinkId the original fragment entry link ID
* @param plid the plid
*/
@Override
public void removeByG_OFELI_P(
long groupId, long originalFragmentEntryLinkId, long plid) {
for (FragmentEntryLink fragmentEntryLink :
findByG_OFELI_P(
groupId, originalFragmentEntryLinkId, plid,
QueryUtil.ALL_POS, QueryUtil.ALL_POS, null)) {
remove(fragmentEntryLink);
}
}
/**
* Returns the number of fragment entry links where groupId = ? and originalFragmentEntryLinkId = ? and plid = ?.
*
* @param groupId the group ID
* @param originalFragmentEntryLinkId the original fragment entry link ID
* @param plid the plid
* @return the number of matching fragment entry links
*/
@Override
public int countByG_OFELI_P(
long groupId, long originalFragmentEntryLinkId, long plid) {
try (SafeCloseable safeCloseable =
ctPersistenceHelper.setCTCollectionIdWithSafeCloseable(
FragmentEntryLink.class)) {
FinderPath finderPath = _finderPathCountByG_OFELI_P;
Object[] finderArgs = new Object[] {
groupId, originalFragmentEntryLinkId, plid
};
Long count = (Long)finderCache.getResult(
finderPath, finderArgs, this);
if (count == null) {
StringBundler sb = new StringBundler(4);
sb.append(_SQL_COUNT_FRAGMENTENTRYLINK_WHERE);
sb.append(_FINDER_COLUMN_G_OFELI_P_GROUPID_2);
sb.append(
_FINDER_COLUMN_G_OFELI_P_ORIGINALFRAGMENTENTRYLINKID_2);
sb.append(_FINDER_COLUMN_G_OFELI_P_PLID_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
queryPos.add(originalFragmentEntryLinkId);
queryPos.add(plid);
count = (Long)query.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
}
private static final String _FINDER_COLUMN_G_OFELI_P_GROUPID_2 =
"fragmentEntryLink.groupId = ? AND ";
private static final String
_FINDER_COLUMN_G_OFELI_P_ORIGINALFRAGMENTENTRYLINKID_2 =
"fragmentEntryLink.originalFragmentEntryLinkId = ? AND ";
private static final String _FINDER_COLUMN_G_OFELI_P_PLID_2 =
"fragmentEntryLink.plid = ?";
private FinderPath _finderPathWithPaginationFindByG_F_C;
private FinderPath _finderPathWithoutPaginationFindByG_F_C;
private FinderPath _finderPathCountByG_F_C;
/**
* Returns all the fragment entry links where groupId = ? and fragmentEntryId = ? and classNameId = ?.
*
* @param groupId the group ID
* @param fragmentEntryId the fragment entry ID
* @param classNameId the class name ID
* @return the matching fragment entry links
*/
@Override
public List findByG_F_C(
long groupId, long fragmentEntryId, long classNameId) {
return findByG_F_C(
groupId, fragmentEntryId, classNameId, QueryUtil.ALL_POS,
QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the fragment entry links where groupId = ? and fragmentEntryId = ? and classNameId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryLinkModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentEntryId the fragment entry ID
* @param classNameId the class name ID
* @param start the lower bound of the range of fragment entry links
* @param end the upper bound of the range of fragment entry links (not inclusive)
* @return the range of matching fragment entry links
*/
@Override
public List findByG_F_C(
long groupId, long fragmentEntryId, long classNameId, int start,
int end) {
return findByG_F_C(
groupId, fragmentEntryId, classNameId, start, end, null);
}
/**
* Returns an ordered range of all the fragment entry links where groupId = ? and fragmentEntryId = ? and classNameId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryLinkModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentEntryId the fragment entry ID
* @param classNameId the class name ID
* @param start the lower bound of the range of fragment entry links
* @param end the upper bound of the range of fragment entry links (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entry links
*/
@Override
public List findByG_F_C(
long groupId, long fragmentEntryId, long classNameId, int start,
int end, OrderByComparator orderByComparator) {
return findByG_F_C(
groupId, fragmentEntryId, classNameId, start, end,
orderByComparator, true);
}
/**
* Returns an ordered range of all the fragment entry links where groupId = ? and fragmentEntryId = ? and classNameId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryLinkModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentEntryId the fragment entry ID
* @param classNameId the class name ID
* @param start the lower bound of the range of fragment entry links
* @param end the upper bound of the range of fragment entry links (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching fragment entry links
*/
@Override
public List findByG_F_C(
long groupId, long fragmentEntryId, long classNameId, int start,
int end, OrderByComparator orderByComparator,
boolean useFinderCache) {
try (SafeCloseable safeCloseable =
ctPersistenceHelper.setCTCollectionIdWithSafeCloseable(
FragmentEntryLink.class)) {
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache) {
finderPath = _finderPathWithoutPaginationFindByG_F_C;
finderArgs = new Object[] {
groupId, fragmentEntryId, classNameId
};
}
}
else if (useFinderCache) {
finderPath = _finderPathWithPaginationFindByG_F_C;
finderArgs = new Object[] {
groupId, fragmentEntryId, classNameId, start, end,
orderByComparator
};
}
List list = null;
if (useFinderCache) {
list = (List)finderCache.getResult(
finderPath, finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (FragmentEntryLink fragmentEntryLink : list) {
if ((groupId != fragmentEntryLink.getGroupId()) ||
(fragmentEntryId !=
fragmentEntryLink.getFragmentEntryId()) ||
(classNameId !=
fragmentEntryLink.getClassNameId())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
5 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(5);
}
sb.append(_SQL_SELECT_FRAGMENTENTRYLINK_WHERE);
sb.append(_FINDER_COLUMN_G_F_C_GROUPID_2);
sb.append(_FINDER_COLUMN_G_F_C_FRAGMENTENTRYID_2);
sb.append(_FINDER_COLUMN_G_F_C_CLASSNAMEID_2);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(FragmentEntryLinkModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
queryPos.add(fragmentEntryId);
queryPos.add(classNameId);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
}
/**
* Returns the first fragment entry link in the ordered set where groupId = ? and fragmentEntryId = ? and classNameId = ?.
*
* @param groupId the group ID
* @param fragmentEntryId the fragment entry ID
* @param classNameId the class name ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry link
* @throws NoSuchEntryLinkException if a matching fragment entry link could not be found
*/
@Override
public FragmentEntryLink findByG_F_C_First(
long groupId, long fragmentEntryId, long classNameId,
OrderByComparator orderByComparator)
throws NoSuchEntryLinkException {
FragmentEntryLink fragmentEntryLink = fetchByG_F_C_First(
groupId, fragmentEntryId, classNameId, orderByComparator);
if (fragmentEntryLink != null) {
return fragmentEntryLink;
}
StringBundler sb = new StringBundler(8);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("groupId=");
sb.append(groupId);
sb.append(", fragmentEntryId=");
sb.append(fragmentEntryId);
sb.append(", classNameId=");
sb.append(classNameId);
sb.append("}");
throw new NoSuchEntryLinkException(sb.toString());
}
/**
* Returns the first fragment entry link in the ordered set where groupId = ? and fragmentEntryId = ? and classNameId = ?.
*
* @param groupId the group ID
* @param fragmentEntryId the fragment entry ID
* @param classNameId the class name ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry link, or null
if a matching fragment entry link could not be found
*/
@Override
public FragmentEntryLink fetchByG_F_C_First(
long groupId, long fragmentEntryId, long classNameId,
OrderByComparator orderByComparator) {
List list = findByG_F_C(
groupId, fragmentEntryId, classNameId, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last fragment entry link in the ordered set where groupId = ? and fragmentEntryId = ? and classNameId = ?.
*
* @param groupId the group ID
* @param fragmentEntryId the fragment entry ID
* @param classNameId the class name ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry link
* @throws NoSuchEntryLinkException if a matching fragment entry link could not be found
*/
@Override
public FragmentEntryLink findByG_F_C_Last(
long groupId, long fragmentEntryId, long classNameId,
OrderByComparator orderByComparator)
throws NoSuchEntryLinkException {
FragmentEntryLink fragmentEntryLink = fetchByG_F_C_Last(
groupId, fragmentEntryId, classNameId, orderByComparator);
if (fragmentEntryLink != null) {
return fragmentEntryLink;
}
StringBundler sb = new StringBundler(8);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("groupId=");
sb.append(groupId);
sb.append(", fragmentEntryId=");
sb.append(fragmentEntryId);
sb.append(", classNameId=");
sb.append(classNameId);
sb.append("}");
throw new NoSuchEntryLinkException(sb.toString());
}
/**
* Returns the last fragment entry link in the ordered set where groupId = ? and fragmentEntryId = ? and classNameId = ?.
*
* @param groupId the group ID
* @param fragmentEntryId the fragment entry ID
* @param classNameId the class name ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry link, or null
if a matching fragment entry link could not be found
*/
@Override
public FragmentEntryLink fetchByG_F_C_Last(
long groupId, long fragmentEntryId, long classNameId,
OrderByComparator orderByComparator) {
int count = countByG_F_C(groupId, fragmentEntryId, classNameId);
if (count == 0) {
return null;
}
List list = findByG_F_C(
groupId, fragmentEntryId, classNameId, count - 1, count,
orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the fragment entry links before and after the current fragment entry link in the ordered set where groupId = ? and fragmentEntryId = ? and classNameId = ?.
*
* @param fragmentEntryLinkId the primary key of the current fragment entry link
* @param groupId the group ID
* @param fragmentEntryId the fragment entry ID
* @param classNameId the class name ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next fragment entry link
* @throws NoSuchEntryLinkException if a fragment entry link with the primary key could not be found
*/
@Override
public FragmentEntryLink[] findByG_F_C_PrevAndNext(
long fragmentEntryLinkId, long groupId, long fragmentEntryId,
long classNameId,
OrderByComparator orderByComparator)
throws NoSuchEntryLinkException {
FragmentEntryLink fragmentEntryLink = findByPrimaryKey(
fragmentEntryLinkId);
Session session = null;
try {
session = openSession();
FragmentEntryLink[] array = new FragmentEntryLinkImpl[3];
array[0] = getByG_F_C_PrevAndNext(
session, fragmentEntryLink, groupId, fragmentEntryId,
classNameId, orderByComparator, true);
array[1] = fragmentEntryLink;
array[2] = getByG_F_C_PrevAndNext(
session, fragmentEntryLink, groupId, fragmentEntryId,
classNameId, orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected FragmentEntryLink getByG_F_C_PrevAndNext(
Session session, FragmentEntryLink fragmentEntryLink, long groupId,
long fragmentEntryId, long classNameId,
OrderByComparator orderByComparator,
boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
6 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(5);
}
sb.append(_SQL_SELECT_FRAGMENTENTRYLINK_WHERE);
sb.append(_FINDER_COLUMN_G_F_C_GROUPID_2);
sb.append(_FINDER_COLUMN_G_F_C_FRAGMENTENTRYID_2);
sb.append(_FINDER_COLUMN_G_F_C_CLASSNAMEID_2);
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(FragmentEntryLinkModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
queryPos.add(fragmentEntryId);
queryPos.add(classNameId);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
fragmentEntryLink)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the fragment entry links where groupId = ? and fragmentEntryId = ? and classNameId = ? from the database.
*
* @param groupId the group ID
* @param fragmentEntryId the fragment entry ID
* @param classNameId the class name ID
*/
@Override
public void removeByG_F_C(
long groupId, long fragmentEntryId, long classNameId) {
for (FragmentEntryLink fragmentEntryLink :
findByG_F_C(
groupId, fragmentEntryId, classNameId, QueryUtil.ALL_POS,
QueryUtil.ALL_POS, null)) {
remove(fragmentEntryLink);
}
}
/**
* Returns the number of fragment entry links where groupId = ? and fragmentEntryId = ? and classNameId = ?.
*
* @param groupId the group ID
* @param fragmentEntryId the fragment entry ID
* @param classNameId the class name ID
* @return the number of matching fragment entry links
*/
@Override
public int countByG_F_C(
long groupId, long fragmentEntryId, long classNameId) {
try (SafeCloseable safeCloseable =
ctPersistenceHelper.setCTCollectionIdWithSafeCloseable(
FragmentEntryLink.class)) {
FinderPath finderPath = _finderPathCountByG_F_C;
Object[] finderArgs = new Object[] {
groupId, fragmentEntryId, classNameId
};
Long count = (Long)finderCache.getResult(
finderPath, finderArgs, this);
if (count == null) {
StringBundler sb = new StringBundler(4);
sb.append(_SQL_COUNT_FRAGMENTENTRYLINK_WHERE);
sb.append(_FINDER_COLUMN_G_F_C_GROUPID_2);
sb.append(_FINDER_COLUMN_G_F_C_FRAGMENTENTRYID_2);
sb.append(_FINDER_COLUMN_G_F_C_CLASSNAMEID_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
queryPos.add(fragmentEntryId);
queryPos.add(classNameId);
count = (Long)query.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
}
private static final String _FINDER_COLUMN_G_F_C_GROUPID_2 =
"fragmentEntryLink.groupId = ? AND ";
private static final String _FINDER_COLUMN_G_F_C_FRAGMENTENTRYID_2 =
"fragmentEntryLink.fragmentEntryId = ? AND ";
private static final String _FINDER_COLUMN_G_F_C_CLASSNAMEID_2 =
"fragmentEntryLink.classNameId = ?";
private FinderPath _finderPathWithPaginationFindByG_F_P;
private FinderPath _finderPathWithoutPaginationFindByG_F_P;
private FinderPath _finderPathCountByG_F_P;
/**
* Returns all the fragment entry links where groupId = ? and fragmentEntryId = ? and plid = ?.
*
* @param groupId the group ID
* @param fragmentEntryId the fragment entry ID
* @param plid the plid
* @return the matching fragment entry links
*/
@Override
public List findByG_F_P(
long groupId, long fragmentEntryId, long plid) {
return findByG_F_P(
groupId, fragmentEntryId, plid, QueryUtil.ALL_POS,
QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the fragment entry links where groupId = ? and fragmentEntryId = ? and plid = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryLinkModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentEntryId the fragment entry ID
* @param plid the plid
* @param start the lower bound of the range of fragment entry links
* @param end the upper bound of the range of fragment entry links (not inclusive)
* @return the range of matching fragment entry links
*/
@Override
public List findByG_F_P(
long groupId, long fragmentEntryId, long plid, int start, int end) {
return findByG_F_P(groupId, fragmentEntryId, plid, start, end, null);
}
/**
* Returns an ordered range of all the fragment entry links where groupId = ? and fragmentEntryId = ? and plid = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryLinkModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentEntryId the fragment entry ID
* @param plid the plid
* @param start the lower bound of the range of fragment entry links
* @param end the upper bound of the range of fragment entry links (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entry links
*/
@Override
public List findByG_F_P(
long groupId, long fragmentEntryId, long plid, int start, int end,
OrderByComparator orderByComparator) {
return findByG_F_P(
groupId, fragmentEntryId, plid, start, end, orderByComparator,
true);
}
/**
* Returns an ordered range of all the fragment entry links where groupId = ? and fragmentEntryId = ? and plid = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryLinkModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentEntryId the fragment entry ID
* @param plid the plid
* @param start the lower bound of the range of fragment entry links
* @param end the upper bound of the range of fragment entry links (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching fragment entry links
*/
@Override
public List findByG_F_P(
long groupId, long fragmentEntryId, long plid, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
try (SafeCloseable safeCloseable =
ctPersistenceHelper.setCTCollectionIdWithSafeCloseable(
FragmentEntryLink.class)) {
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache) {
finderPath = _finderPathWithoutPaginationFindByG_F_P;
finderArgs = new Object[] {groupId, fragmentEntryId, plid};
}
}
else if (useFinderCache) {
finderPath = _finderPathWithPaginationFindByG_F_P;
finderArgs = new Object[] {
groupId, fragmentEntryId, plid, start, end,
orderByComparator
};
}
List list = null;
if (useFinderCache) {
list = (List)finderCache.getResult(
finderPath, finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (FragmentEntryLink fragmentEntryLink : list) {
if ((groupId != fragmentEntryLink.getGroupId()) ||
(fragmentEntryId !=
fragmentEntryLink.getFragmentEntryId()) ||
(plid != fragmentEntryLink.getPlid())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
5 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(5);
}
sb.append(_SQL_SELECT_FRAGMENTENTRYLINK_WHERE);
sb.append(_FINDER_COLUMN_G_F_P_GROUPID_2);
sb.append(_FINDER_COLUMN_G_F_P_FRAGMENTENTRYID_2);
sb.append(_FINDER_COLUMN_G_F_P_PLID_2);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(FragmentEntryLinkModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
queryPos.add(fragmentEntryId);
queryPos.add(plid);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
}
/**
* Returns the first fragment entry link in the ordered set where groupId = ? and fragmentEntryId = ? and plid = ?.
*
* @param groupId the group ID
* @param fragmentEntryId the fragment entry ID
* @param plid the plid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry link
* @throws NoSuchEntryLinkException if a matching fragment entry link could not be found
*/
@Override
public FragmentEntryLink findByG_F_P_First(
long groupId, long fragmentEntryId, long plid,
OrderByComparator orderByComparator)
throws NoSuchEntryLinkException {
FragmentEntryLink fragmentEntryLink = fetchByG_F_P_First(
groupId, fragmentEntryId, plid, orderByComparator);
if (fragmentEntryLink != null) {
return fragmentEntryLink;
}
StringBundler sb = new StringBundler(8);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("groupId=");
sb.append(groupId);
sb.append(", fragmentEntryId=");
sb.append(fragmentEntryId);
sb.append(", plid=");
sb.append(plid);
sb.append("}");
throw new NoSuchEntryLinkException(sb.toString());
}
/**
* Returns the first fragment entry link in the ordered set where groupId = ? and fragmentEntryId = ? and plid = ?.
*
* @param groupId the group ID
* @param fragmentEntryId the fragment entry ID
* @param plid the plid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry link, or null
if a matching fragment entry link could not be found
*/
@Override
public FragmentEntryLink fetchByG_F_P_First(
long groupId, long fragmentEntryId, long plid,
OrderByComparator orderByComparator) {
List list = findByG_F_P(
groupId, fragmentEntryId, plid, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last fragment entry link in the ordered set where groupId = ? and fragmentEntryId = ? and plid = ?.
*
* @param groupId the group ID
* @param fragmentEntryId the fragment entry ID
* @param plid the plid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry link
* @throws NoSuchEntryLinkException if a matching fragment entry link could not be found
*/
@Override
public FragmentEntryLink findByG_F_P_Last(
long groupId, long fragmentEntryId, long plid,
OrderByComparator orderByComparator)
throws NoSuchEntryLinkException {
FragmentEntryLink fragmentEntryLink = fetchByG_F_P_Last(
groupId, fragmentEntryId, plid, orderByComparator);
if (fragmentEntryLink != null) {
return fragmentEntryLink;
}
StringBundler sb = new StringBundler(8);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("groupId=");
sb.append(groupId);
sb.append(", fragmentEntryId=");
sb.append(fragmentEntryId);
sb.append(", plid=");
sb.append(plid);
sb.append("}");
throw new NoSuchEntryLinkException(sb.toString());
}
/**
* Returns the last fragment entry link in the ordered set where groupId = ? and fragmentEntryId = ? and plid = ?.
*
* @param groupId the group ID
* @param fragmentEntryId the fragment entry ID
* @param plid the plid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry link, or null
if a matching fragment entry link could not be found
*/
@Override
public FragmentEntryLink fetchByG_F_P_Last(
long groupId, long fragmentEntryId, long plid,
OrderByComparator orderByComparator) {
int count = countByG_F_P(groupId, fragmentEntryId, plid);
if (count == 0) {
return null;
}
List list = findByG_F_P(
groupId, fragmentEntryId, plid, count - 1, count,
orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the fragment entry links before and after the current fragment entry link in the ordered set where groupId = ? and fragmentEntryId = ? and plid = ?.
*
* @param fragmentEntryLinkId the primary key of the current fragment entry link
* @param groupId the group ID
* @param fragmentEntryId the fragment entry ID
* @param plid the plid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next fragment entry link
* @throws NoSuchEntryLinkException if a fragment entry link with the primary key could not be found
*/
@Override
public FragmentEntryLink[] findByG_F_P_PrevAndNext(
long fragmentEntryLinkId, long groupId, long fragmentEntryId,
long plid, OrderByComparator orderByComparator)
throws NoSuchEntryLinkException {
FragmentEntryLink fragmentEntryLink = findByPrimaryKey(
fragmentEntryLinkId);
Session session = null;
try {
session = openSession();
FragmentEntryLink[] array = new FragmentEntryLinkImpl[3];
array[0] = getByG_F_P_PrevAndNext(
session, fragmentEntryLink, groupId, fragmentEntryId, plid,
orderByComparator, true);
array[1] = fragmentEntryLink;
array[2] = getByG_F_P_PrevAndNext(
session, fragmentEntryLink, groupId, fragmentEntryId, plid,
orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected FragmentEntryLink getByG_F_P_PrevAndNext(
Session session, FragmentEntryLink fragmentEntryLink, long groupId,
long fragmentEntryId, long plid,
OrderByComparator orderByComparator,
boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
6 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(5);
}
sb.append(_SQL_SELECT_FRAGMENTENTRYLINK_WHERE);
sb.append(_FINDER_COLUMN_G_F_P_GROUPID_2);
sb.append(_FINDER_COLUMN_G_F_P_FRAGMENTENTRYID_2);
sb.append(_FINDER_COLUMN_G_F_P_PLID_2);
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(FragmentEntryLinkModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
queryPos.add(fragmentEntryId);
queryPos.add(plid);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
fragmentEntryLink)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the fragment entry links where groupId = ? and fragmentEntryId = ? and plid = ? from the database.
*
* @param groupId the group ID
* @param fragmentEntryId the fragment entry ID
* @param plid the plid
*/
@Override
public void removeByG_F_P(long groupId, long fragmentEntryId, long plid) {
for (FragmentEntryLink fragmentEntryLink :
findByG_F_P(
groupId, fragmentEntryId, plid, QueryUtil.ALL_POS,
QueryUtil.ALL_POS, null)) {
remove(fragmentEntryLink);
}
}
/**
* Returns the number of fragment entry links where groupId = ? and fragmentEntryId = ? and plid = ?.
*
* @param groupId the group ID
* @param fragmentEntryId the fragment entry ID
* @param plid the plid
* @return the number of matching fragment entry links
*/
@Override
public int countByG_F_P(long groupId, long fragmentEntryId, long plid) {
try (SafeCloseable safeCloseable =
ctPersistenceHelper.setCTCollectionIdWithSafeCloseable(
FragmentEntryLink.class)) {
FinderPath finderPath = _finderPathCountByG_F_P;
Object[] finderArgs = new Object[] {groupId, fragmentEntryId, plid};
Long count = (Long)finderCache.getResult(
finderPath, finderArgs, this);
if (count == null) {
StringBundler sb = new StringBundler(4);
sb.append(_SQL_COUNT_FRAGMENTENTRYLINK_WHERE);
sb.append(_FINDER_COLUMN_G_F_P_GROUPID_2);
sb.append(_FINDER_COLUMN_G_F_P_FRAGMENTENTRYID_2);
sb.append(_FINDER_COLUMN_G_F_P_PLID_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
queryPos.add(fragmentEntryId);
queryPos.add(plid);
count = (Long)query.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
}
private static final String _FINDER_COLUMN_G_F_P_GROUPID_2 =
"fragmentEntryLink.groupId = ? AND ";
private static final String _FINDER_COLUMN_G_F_P_FRAGMENTENTRYID_2 =
"fragmentEntryLink.fragmentEntryId = ? AND ";
private static final String _FINDER_COLUMN_G_F_P_PLID_2 =
"fragmentEntryLink.plid = ?";
private FinderPath _finderPathWithPaginationFindByG_S_P;
private FinderPath _finderPathWithoutPaginationFindByG_S_P;
private FinderPath _finderPathCountByG_S_P;
private FinderPath _finderPathWithPaginationCountByG_S_P;
/**
* Returns all the fragment entry links where groupId = ? and segmentsExperienceId = ? and plid = ?.
*
* @param groupId the group ID
* @param segmentsExperienceId the segments experience ID
* @param plid the plid
* @return the matching fragment entry links
*/
@Override
public List findByG_S_P(
long groupId, long segmentsExperienceId, long plid) {
return findByG_S_P(
groupId, segmentsExperienceId, plid, QueryUtil.ALL_POS,
QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the fragment entry links where groupId = ? and segmentsExperienceId = ? and plid = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryLinkModelImpl
.
*
*
* @param groupId the group ID
* @param segmentsExperienceId the segments experience ID
* @param plid the plid
* @param start the lower bound of the range of fragment entry links
* @param end the upper bound of the range of fragment entry links (not inclusive)
* @return the range of matching fragment entry links
*/
@Override
public List findByG_S_P(
long groupId, long segmentsExperienceId, long plid, int start,
int end) {
return findByG_S_P(
groupId, segmentsExperienceId, plid, start, end, null);
}
/**
* Returns an ordered range of all the fragment entry links where groupId = ? and segmentsExperienceId = ? and plid = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryLinkModelImpl
.
*
*
* @param groupId the group ID
* @param segmentsExperienceId the segments experience ID
* @param plid the plid
* @param start the lower bound of the range of fragment entry links
* @param end the upper bound of the range of fragment entry links (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entry links
*/
@Override
public List findByG_S_P(
long groupId, long segmentsExperienceId, long plid, int start, int end,
OrderByComparator orderByComparator) {
return findByG_S_P(
groupId, segmentsExperienceId, plid, start, end, orderByComparator,
true);
}
/**
* Returns an ordered range of all the fragment entry links where groupId = ? and segmentsExperienceId = ? and plid = ?.
*
*
* Useful when paginating results. Returns a maximum of