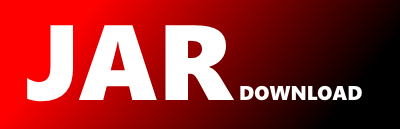
com.liferay.fragment.service.persistence.impl.FragmentEntryPersistenceImpl Maven / Gradle / Ivy
/**
* Copyright (c) 2000-present Liferay, Inc. All rights reserved.
*
* This library is free software; you can redistribute it and/or modify it under
* the terms of the GNU Lesser General Public License as published by the Free
* Software Foundation; either version 2.1 of the License, or (at your option)
* any later version.
*
* This library is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License for more
* details.
*/
package com.liferay.fragment.service.persistence.impl;
import com.liferay.fragment.exception.NoSuchEntryException;
import com.liferay.fragment.model.FragmentEntry;
import com.liferay.fragment.model.FragmentEntryTable;
import com.liferay.fragment.model.impl.FragmentEntryImpl;
import com.liferay.fragment.model.impl.FragmentEntryModelImpl;
import com.liferay.fragment.service.persistence.FragmentEntryPersistence;
import com.liferay.fragment.service.persistence.FragmentEntryUtil;
import com.liferay.fragment.service.persistence.impl.constants.FragmentPersistenceConstants;
import com.liferay.petra.string.StringBundler;
import com.liferay.portal.kernel.change.tracking.CTColumnResolutionType;
import com.liferay.portal.kernel.configuration.Configuration;
import com.liferay.portal.kernel.dao.orm.EntityCache;
import com.liferay.portal.kernel.dao.orm.FinderCache;
import com.liferay.portal.kernel.dao.orm.FinderPath;
import com.liferay.portal.kernel.dao.orm.Query;
import com.liferay.portal.kernel.dao.orm.QueryPos;
import com.liferay.portal.kernel.dao.orm.QueryUtil;
import com.liferay.portal.kernel.dao.orm.Session;
import com.liferay.portal.kernel.dao.orm.SessionFactory;
import com.liferay.portal.kernel.log.Log;
import com.liferay.portal.kernel.log.LogFactoryUtil;
import com.liferay.portal.kernel.security.auth.CompanyThreadLocal;
import com.liferay.portal.kernel.service.ServiceContext;
import com.liferay.portal.kernel.service.ServiceContextThreadLocal;
import com.liferay.portal.kernel.service.persistence.BasePersistence;
import com.liferay.portal.kernel.service.persistence.change.tracking.helper.CTPersistenceHelper;
import com.liferay.portal.kernel.service.persistence.impl.BasePersistenceImpl;
import com.liferay.portal.kernel.util.GetterUtil;
import com.liferay.portal.kernel.util.OrderByComparator;
import com.liferay.portal.kernel.util.PropsKeys;
import com.liferay.portal.kernel.util.PropsUtil;
import com.liferay.portal.kernel.util.ProxyUtil;
import com.liferay.portal.kernel.util.SetUtil;
import com.liferay.portal.kernel.util.StringUtil;
import com.liferay.portal.kernel.util.Validator;
import com.liferay.portal.kernel.uuid.PortalUUIDUtil;
import java.io.Serializable;
import java.lang.reflect.Field;
import java.lang.reflect.InvocationHandler;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Date;
import java.util.EnumMap;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Set;
import javax.sql.DataSource;
import org.osgi.service.component.annotations.Activate;
import org.osgi.service.component.annotations.Component;
import org.osgi.service.component.annotations.Deactivate;
import org.osgi.service.component.annotations.Reference;
/**
* The persistence implementation for the fragment entry service.
*
*
* Caching information and settings can be found in portal.properties
*
*
* @author Brian Wing Shun Chan
* @generated
*/
@Component(service = {FragmentEntryPersistence.class, BasePersistence.class})
public class FragmentEntryPersistenceImpl
extends BasePersistenceImpl
implements FragmentEntryPersistence {
/*
* NOTE FOR DEVELOPERS:
*
* Never modify or reference this class directly. Always use FragmentEntryUtil
to access the fragment entry persistence. Modify service.xml
and rerun ServiceBuilder to regenerate this class.
*/
public static final String FINDER_CLASS_NAME_ENTITY =
FragmentEntryImpl.class.getName();
public static final String FINDER_CLASS_NAME_LIST_WITH_PAGINATION =
FINDER_CLASS_NAME_ENTITY + ".List1";
public static final String FINDER_CLASS_NAME_LIST_WITHOUT_PAGINATION =
FINDER_CLASS_NAME_ENTITY + ".List2";
private FinderPath _finderPathWithPaginationFindAll;
private FinderPath _finderPathWithoutPaginationFindAll;
private FinderPath _finderPathCountAll;
private FinderPath _finderPathWithPaginationFindByUuid;
private FinderPath _finderPathWithoutPaginationFindByUuid;
private FinderPath _finderPathCountByUuid;
/**
* Returns all the fragment entries where uuid = ?.
*
* @param uuid the uuid
* @return the matching fragment entries
*/
@Override
public List findByUuid(String uuid) {
return findByUuid(uuid, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the fragment entries where uuid = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryModelImpl
.
*
*
* @param uuid the uuid
* @param start the lower bound of the range of fragment entries
* @param end the upper bound of the range of fragment entries (not inclusive)
* @return the range of matching fragment entries
*/
@Override
public List findByUuid(String uuid, int start, int end) {
return findByUuid(uuid, start, end, null);
}
/**
* Returns an ordered range of all the fragment entries where uuid = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryModelImpl
.
*
*
* @param uuid the uuid
* @param start the lower bound of the range of fragment entries
* @param end the upper bound of the range of fragment entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entries
*/
@Override
public List findByUuid(
String uuid, int start, int end,
OrderByComparator orderByComparator) {
return findByUuid(uuid, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the fragment entries where uuid = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryModelImpl
.
*
*
* @param uuid the uuid
* @param start the lower bound of the range of fragment entries
* @param end the upper bound of the range of fragment entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching fragment entries
*/
@Override
public List findByUuid(
String uuid, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
uuid = Objects.toString(uuid, "");
boolean productionMode = ctPersistenceHelper.isProductionMode(
FragmentEntry.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache && productionMode) {
finderPath = _finderPathWithoutPaginationFindByUuid;
finderArgs = new Object[] {uuid};
}
}
else if (useFinderCache && productionMode) {
finderPath = _finderPathWithPaginationFindByUuid;
finderArgs = new Object[] {uuid, start, end, orderByComparator};
}
List list = null;
if (useFinderCache && productionMode) {
list = (List)finderCache.getResult(
finderPath, finderArgs);
if ((list != null) && !list.isEmpty()) {
for (FragmentEntry fragmentEntry : list) {
if (!uuid.equals(fragmentEntry.getUuid())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
3 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(3);
}
sb.append(_SQL_SELECT_FRAGMENTENTRY_WHERE);
boolean bindUuid = false;
if (uuid.isEmpty()) {
sb.append(_FINDER_COLUMN_UUID_UUID_3);
}
else {
bindUuid = true;
sb.append(_FINDER_COLUMN_UUID_UUID_2);
}
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(FragmentEntryModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
if (bindUuid) {
queryPos.add(uuid);
}
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache && productionMode) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first fragment entry in the ordered set where uuid = ?.
*
* @param uuid the uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry
* @throws NoSuchEntryException if a matching fragment entry could not be found
*/
@Override
public FragmentEntry findByUuid_First(
String uuid, OrderByComparator orderByComparator)
throws NoSuchEntryException {
FragmentEntry fragmentEntry = fetchByUuid_First(
uuid, orderByComparator);
if (fragmentEntry != null) {
return fragmentEntry;
}
StringBundler sb = new StringBundler(4);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("uuid=");
sb.append(uuid);
sb.append("}");
throw new NoSuchEntryException(sb.toString());
}
/**
* Returns the first fragment entry in the ordered set where uuid = ?.
*
* @param uuid the uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry, or null
if a matching fragment entry could not be found
*/
@Override
public FragmentEntry fetchByUuid_First(
String uuid, OrderByComparator orderByComparator) {
List list = findByUuid(uuid, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last fragment entry in the ordered set where uuid = ?.
*
* @param uuid the uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry
* @throws NoSuchEntryException if a matching fragment entry could not be found
*/
@Override
public FragmentEntry findByUuid_Last(
String uuid, OrderByComparator orderByComparator)
throws NoSuchEntryException {
FragmentEntry fragmentEntry = fetchByUuid_Last(uuid, orderByComparator);
if (fragmentEntry != null) {
return fragmentEntry;
}
StringBundler sb = new StringBundler(4);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("uuid=");
sb.append(uuid);
sb.append("}");
throw new NoSuchEntryException(sb.toString());
}
/**
* Returns the last fragment entry in the ordered set where uuid = ?.
*
* @param uuid the uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry, or null
if a matching fragment entry could not be found
*/
@Override
public FragmentEntry fetchByUuid_Last(
String uuid, OrderByComparator orderByComparator) {
int count = countByUuid(uuid);
if (count == 0) {
return null;
}
List list = findByUuid(
uuid, count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the fragment entries before and after the current fragment entry in the ordered set where uuid = ?.
*
* @param fragmentEntryId the primary key of the current fragment entry
* @param uuid the uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next fragment entry
* @throws NoSuchEntryException if a fragment entry with the primary key could not be found
*/
@Override
public FragmentEntry[] findByUuid_PrevAndNext(
long fragmentEntryId, String uuid,
OrderByComparator orderByComparator)
throws NoSuchEntryException {
uuid = Objects.toString(uuid, "");
FragmentEntry fragmentEntry = findByPrimaryKey(fragmentEntryId);
Session session = null;
try {
session = openSession();
FragmentEntry[] array = new FragmentEntryImpl[3];
array[0] = getByUuid_PrevAndNext(
session, fragmentEntry, uuid, orderByComparator, true);
array[1] = fragmentEntry;
array[2] = getByUuid_PrevAndNext(
session, fragmentEntry, uuid, orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected FragmentEntry getByUuid_PrevAndNext(
Session session, FragmentEntry fragmentEntry, String uuid,
OrderByComparator orderByComparator, boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(3);
}
sb.append(_SQL_SELECT_FRAGMENTENTRY_WHERE);
boolean bindUuid = false;
if (uuid.isEmpty()) {
sb.append(_FINDER_COLUMN_UUID_UUID_3);
}
else {
bindUuid = true;
sb.append(_FINDER_COLUMN_UUID_UUID_2);
}
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(FragmentEntryModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
if (bindUuid) {
queryPos.add(uuid);
}
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
fragmentEntry)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the fragment entries where uuid = ? from the database.
*
* @param uuid the uuid
*/
@Override
public void removeByUuid(String uuid) {
for (FragmentEntry fragmentEntry :
findByUuid(uuid, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null)) {
remove(fragmentEntry);
}
}
/**
* Returns the number of fragment entries where uuid = ?.
*
* @param uuid the uuid
* @return the number of matching fragment entries
*/
@Override
public int countByUuid(String uuid) {
uuid = Objects.toString(uuid, "");
boolean productionMode = ctPersistenceHelper.isProductionMode(
FragmentEntry.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
Long count = null;
if (productionMode) {
finderPath = _finderPathCountByUuid;
finderArgs = new Object[] {uuid};
count = (Long)finderCache.getResult(finderPath, finderArgs);
}
if (count == null) {
StringBundler sb = new StringBundler(2);
sb.append(_SQL_COUNT_FRAGMENTENTRY_WHERE);
boolean bindUuid = false;
if (uuid.isEmpty()) {
sb.append(_FINDER_COLUMN_UUID_UUID_3);
}
else {
bindUuid = true;
sb.append(_FINDER_COLUMN_UUID_UUID_2);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
if (bindUuid) {
queryPos.add(uuid);
}
count = (Long)query.uniqueResult();
if (productionMode) {
finderCache.putResult(finderPath, finderArgs, count);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String _FINDER_COLUMN_UUID_UUID_2 =
"fragmentEntry.uuid = ?";
private static final String _FINDER_COLUMN_UUID_UUID_3 =
"(fragmentEntry.uuid IS NULL OR fragmentEntry.uuid = '')";
private FinderPath _finderPathWithPaginationFindByUuid_Head;
private FinderPath _finderPathWithoutPaginationFindByUuid_Head;
private FinderPath _finderPathCountByUuid_Head;
/**
* Returns all the fragment entries where uuid = ? and head = ?.
*
* @param uuid the uuid
* @param head the head
* @return the matching fragment entries
*/
@Override
public List findByUuid_Head(String uuid, boolean head) {
return findByUuid_Head(
uuid, head, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the fragment entries where uuid = ? and head = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryModelImpl
.
*
*
* @param uuid the uuid
* @param head the head
* @param start the lower bound of the range of fragment entries
* @param end the upper bound of the range of fragment entries (not inclusive)
* @return the range of matching fragment entries
*/
@Override
public List findByUuid_Head(
String uuid, boolean head, int start, int end) {
return findByUuid_Head(uuid, head, start, end, null);
}
/**
* Returns an ordered range of all the fragment entries where uuid = ? and head = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryModelImpl
.
*
*
* @param uuid the uuid
* @param head the head
* @param start the lower bound of the range of fragment entries
* @param end the upper bound of the range of fragment entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entries
*/
@Override
public List findByUuid_Head(
String uuid, boolean head, int start, int end,
OrderByComparator orderByComparator) {
return findByUuid_Head(uuid, head, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the fragment entries where uuid = ? and head = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryModelImpl
.
*
*
* @param uuid the uuid
* @param head the head
* @param start the lower bound of the range of fragment entries
* @param end the upper bound of the range of fragment entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching fragment entries
*/
@Override
public List findByUuid_Head(
String uuid, boolean head, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
uuid = Objects.toString(uuid, "");
boolean productionMode = ctPersistenceHelper.isProductionMode(
FragmentEntry.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache && productionMode) {
finderPath = _finderPathWithoutPaginationFindByUuid_Head;
finderArgs = new Object[] {uuid, head};
}
}
else if (useFinderCache && productionMode) {
finderPath = _finderPathWithPaginationFindByUuid_Head;
finderArgs = new Object[] {
uuid, head, start, end, orderByComparator
};
}
List list = null;
if (useFinderCache && productionMode) {
list = (List)finderCache.getResult(
finderPath, finderArgs);
if ((list != null) && !list.isEmpty()) {
for (FragmentEntry fragmentEntry : list) {
if (!uuid.equals(fragmentEntry.getUuid()) ||
(head != fragmentEntry.isHead())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_FRAGMENTENTRY_WHERE);
boolean bindUuid = false;
if (uuid.isEmpty()) {
sb.append(_FINDER_COLUMN_UUID_HEAD_UUID_3);
}
else {
bindUuid = true;
sb.append(_FINDER_COLUMN_UUID_HEAD_UUID_2);
}
sb.append(_FINDER_COLUMN_UUID_HEAD_HEAD_2);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(FragmentEntryModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
if (bindUuid) {
queryPos.add(uuid);
}
queryPos.add(head);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache && productionMode) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first fragment entry in the ordered set where uuid = ? and head = ?.
*
* @param uuid the uuid
* @param head the head
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry
* @throws NoSuchEntryException if a matching fragment entry could not be found
*/
@Override
public FragmentEntry findByUuid_Head_First(
String uuid, boolean head,
OrderByComparator orderByComparator)
throws NoSuchEntryException {
FragmentEntry fragmentEntry = fetchByUuid_Head_First(
uuid, head, orderByComparator);
if (fragmentEntry != null) {
return fragmentEntry;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("uuid=");
sb.append(uuid);
sb.append(", head=");
sb.append(head);
sb.append("}");
throw new NoSuchEntryException(sb.toString());
}
/**
* Returns the first fragment entry in the ordered set where uuid = ? and head = ?.
*
* @param uuid the uuid
* @param head the head
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry, or null
if a matching fragment entry could not be found
*/
@Override
public FragmentEntry fetchByUuid_Head_First(
String uuid, boolean head,
OrderByComparator orderByComparator) {
List list = findByUuid_Head(
uuid, head, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last fragment entry in the ordered set where uuid = ? and head = ?.
*
* @param uuid the uuid
* @param head the head
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry
* @throws NoSuchEntryException if a matching fragment entry could not be found
*/
@Override
public FragmentEntry findByUuid_Head_Last(
String uuid, boolean head,
OrderByComparator orderByComparator)
throws NoSuchEntryException {
FragmentEntry fragmentEntry = fetchByUuid_Head_Last(
uuid, head, orderByComparator);
if (fragmentEntry != null) {
return fragmentEntry;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("uuid=");
sb.append(uuid);
sb.append(", head=");
sb.append(head);
sb.append("}");
throw new NoSuchEntryException(sb.toString());
}
/**
* Returns the last fragment entry in the ordered set where uuid = ? and head = ?.
*
* @param uuid the uuid
* @param head the head
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry, or null
if a matching fragment entry could not be found
*/
@Override
public FragmentEntry fetchByUuid_Head_Last(
String uuid, boolean head,
OrderByComparator orderByComparator) {
int count = countByUuid_Head(uuid, head);
if (count == 0) {
return null;
}
List list = findByUuid_Head(
uuid, head, count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the fragment entries before and after the current fragment entry in the ordered set where uuid = ? and head = ?.
*
* @param fragmentEntryId the primary key of the current fragment entry
* @param uuid the uuid
* @param head the head
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next fragment entry
* @throws NoSuchEntryException if a fragment entry with the primary key could not be found
*/
@Override
public FragmentEntry[] findByUuid_Head_PrevAndNext(
long fragmentEntryId, String uuid, boolean head,
OrderByComparator orderByComparator)
throws NoSuchEntryException {
uuid = Objects.toString(uuid, "");
FragmentEntry fragmentEntry = findByPrimaryKey(fragmentEntryId);
Session session = null;
try {
session = openSession();
FragmentEntry[] array = new FragmentEntryImpl[3];
array[0] = getByUuid_Head_PrevAndNext(
session, fragmentEntry, uuid, head, orderByComparator, true);
array[1] = fragmentEntry;
array[2] = getByUuid_Head_PrevAndNext(
session, fragmentEntry, uuid, head, orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected FragmentEntry getByUuid_Head_PrevAndNext(
Session session, FragmentEntry fragmentEntry, String uuid, boolean head,
OrderByComparator orderByComparator, boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
5 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_FRAGMENTENTRY_WHERE);
boolean bindUuid = false;
if (uuid.isEmpty()) {
sb.append(_FINDER_COLUMN_UUID_HEAD_UUID_3);
}
else {
bindUuid = true;
sb.append(_FINDER_COLUMN_UUID_HEAD_UUID_2);
}
sb.append(_FINDER_COLUMN_UUID_HEAD_HEAD_2);
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(FragmentEntryModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
if (bindUuid) {
queryPos.add(uuid);
}
queryPos.add(head);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
fragmentEntry)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the fragment entries where uuid = ? and head = ? from the database.
*
* @param uuid the uuid
* @param head the head
*/
@Override
public void removeByUuid_Head(String uuid, boolean head) {
for (FragmentEntry fragmentEntry :
findByUuid_Head(
uuid, head, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null)) {
remove(fragmentEntry);
}
}
/**
* Returns the number of fragment entries where uuid = ? and head = ?.
*
* @param uuid the uuid
* @param head the head
* @return the number of matching fragment entries
*/
@Override
public int countByUuid_Head(String uuid, boolean head) {
uuid = Objects.toString(uuid, "");
boolean productionMode = ctPersistenceHelper.isProductionMode(
FragmentEntry.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
Long count = null;
if (productionMode) {
finderPath = _finderPathCountByUuid_Head;
finderArgs = new Object[] {uuid, head};
count = (Long)finderCache.getResult(finderPath, finderArgs);
}
if (count == null) {
StringBundler sb = new StringBundler(3);
sb.append(_SQL_COUNT_FRAGMENTENTRY_WHERE);
boolean bindUuid = false;
if (uuid.isEmpty()) {
sb.append(_FINDER_COLUMN_UUID_HEAD_UUID_3);
}
else {
bindUuid = true;
sb.append(_FINDER_COLUMN_UUID_HEAD_UUID_2);
}
sb.append(_FINDER_COLUMN_UUID_HEAD_HEAD_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
if (bindUuid) {
queryPos.add(uuid);
}
queryPos.add(head);
count = (Long)query.uniqueResult();
if (productionMode) {
finderCache.putResult(finderPath, finderArgs, count);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String _FINDER_COLUMN_UUID_HEAD_UUID_2 =
"fragmentEntry.uuid = ? AND ";
private static final String _FINDER_COLUMN_UUID_HEAD_UUID_3 =
"(fragmentEntry.uuid IS NULL OR fragmentEntry.uuid = '') AND ";
private static final String _FINDER_COLUMN_UUID_HEAD_HEAD_2 =
"fragmentEntry.head = ?";
private FinderPath _finderPathWithPaginationFindByUUID_G;
private FinderPath _finderPathWithoutPaginationFindByUUID_G;
private FinderPath _finderPathCountByUUID_G;
/**
* Returns all the fragment entries where uuid = ? and groupId = ?.
*
* @param uuid the uuid
* @param groupId the group ID
* @return the matching fragment entries
*/
@Override
public List findByUUID_G(String uuid, long groupId) {
return findByUUID_G(
uuid, groupId, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the fragment entries where uuid = ? and groupId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryModelImpl
.
*
*
* @param uuid the uuid
* @param groupId the group ID
* @param start the lower bound of the range of fragment entries
* @param end the upper bound of the range of fragment entries (not inclusive)
* @return the range of matching fragment entries
*/
@Override
public List findByUUID_G(
String uuid, long groupId, int start, int end) {
return findByUUID_G(uuid, groupId, start, end, null);
}
/**
* Returns an ordered range of all the fragment entries where uuid = ? and groupId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryModelImpl
.
*
*
* @param uuid the uuid
* @param groupId the group ID
* @param start the lower bound of the range of fragment entries
* @param end the upper bound of the range of fragment entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entries
*/
@Override
public List findByUUID_G(
String uuid, long groupId, int start, int end,
OrderByComparator orderByComparator) {
return findByUUID_G(uuid, groupId, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the fragment entries where uuid = ? and groupId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryModelImpl
.
*
*
* @param uuid the uuid
* @param groupId the group ID
* @param start the lower bound of the range of fragment entries
* @param end the upper bound of the range of fragment entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching fragment entries
*/
@Override
public List findByUUID_G(
String uuid, long groupId, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
uuid = Objects.toString(uuid, "");
boolean productionMode = ctPersistenceHelper.isProductionMode(
FragmentEntry.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache && productionMode) {
finderPath = _finderPathWithoutPaginationFindByUUID_G;
finderArgs = new Object[] {uuid, groupId};
}
}
else if (useFinderCache && productionMode) {
finderPath = _finderPathWithPaginationFindByUUID_G;
finderArgs = new Object[] {
uuid, groupId, start, end, orderByComparator
};
}
List list = null;
if (useFinderCache && productionMode) {
list = (List)finderCache.getResult(
finderPath, finderArgs);
if ((list != null) && !list.isEmpty()) {
for (FragmentEntry fragmentEntry : list) {
if (!uuid.equals(fragmentEntry.getUuid()) ||
(groupId != fragmentEntry.getGroupId())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_FRAGMENTENTRY_WHERE);
boolean bindUuid = false;
if (uuid.isEmpty()) {
sb.append(_FINDER_COLUMN_UUID_G_UUID_3);
}
else {
bindUuid = true;
sb.append(_FINDER_COLUMN_UUID_G_UUID_2);
}
sb.append(_FINDER_COLUMN_UUID_G_GROUPID_2);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(FragmentEntryModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
if (bindUuid) {
queryPos.add(uuid);
}
queryPos.add(groupId);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache && productionMode) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first fragment entry in the ordered set where uuid = ? and groupId = ?.
*
* @param uuid the uuid
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry
* @throws NoSuchEntryException if a matching fragment entry could not be found
*/
@Override
public FragmentEntry findByUUID_G_First(
String uuid, long groupId,
OrderByComparator orderByComparator)
throws NoSuchEntryException {
FragmentEntry fragmentEntry = fetchByUUID_G_First(
uuid, groupId, orderByComparator);
if (fragmentEntry != null) {
return fragmentEntry;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("uuid=");
sb.append(uuid);
sb.append(", groupId=");
sb.append(groupId);
sb.append("}");
throw new NoSuchEntryException(sb.toString());
}
/**
* Returns the first fragment entry in the ordered set where uuid = ? and groupId = ?.
*
* @param uuid the uuid
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry, or null
if a matching fragment entry could not be found
*/
@Override
public FragmentEntry fetchByUUID_G_First(
String uuid, long groupId,
OrderByComparator orderByComparator) {
List list = findByUUID_G(
uuid, groupId, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last fragment entry in the ordered set where uuid = ? and groupId = ?.
*
* @param uuid the uuid
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry
* @throws NoSuchEntryException if a matching fragment entry could not be found
*/
@Override
public FragmentEntry findByUUID_G_Last(
String uuid, long groupId,
OrderByComparator orderByComparator)
throws NoSuchEntryException {
FragmentEntry fragmentEntry = fetchByUUID_G_Last(
uuid, groupId, orderByComparator);
if (fragmentEntry != null) {
return fragmentEntry;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("uuid=");
sb.append(uuid);
sb.append(", groupId=");
sb.append(groupId);
sb.append("}");
throw new NoSuchEntryException(sb.toString());
}
/**
* Returns the last fragment entry in the ordered set where uuid = ? and groupId = ?.
*
* @param uuid the uuid
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry, or null
if a matching fragment entry could not be found
*/
@Override
public FragmentEntry fetchByUUID_G_Last(
String uuid, long groupId,
OrderByComparator orderByComparator) {
int count = countByUUID_G(uuid, groupId);
if (count == 0) {
return null;
}
List list = findByUUID_G(
uuid, groupId, count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the fragment entries before and after the current fragment entry in the ordered set where uuid = ? and groupId = ?.
*
* @param fragmentEntryId the primary key of the current fragment entry
* @param uuid the uuid
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next fragment entry
* @throws NoSuchEntryException if a fragment entry with the primary key could not be found
*/
@Override
public FragmentEntry[] findByUUID_G_PrevAndNext(
long fragmentEntryId, String uuid, long groupId,
OrderByComparator orderByComparator)
throws NoSuchEntryException {
uuid = Objects.toString(uuid, "");
FragmentEntry fragmentEntry = findByPrimaryKey(fragmentEntryId);
Session session = null;
try {
session = openSession();
FragmentEntry[] array = new FragmentEntryImpl[3];
array[0] = getByUUID_G_PrevAndNext(
session, fragmentEntry, uuid, groupId, orderByComparator, true);
array[1] = fragmentEntry;
array[2] = getByUUID_G_PrevAndNext(
session, fragmentEntry, uuid, groupId, orderByComparator,
false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected FragmentEntry getByUUID_G_PrevAndNext(
Session session, FragmentEntry fragmentEntry, String uuid, long groupId,
OrderByComparator orderByComparator, boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
5 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_FRAGMENTENTRY_WHERE);
boolean bindUuid = false;
if (uuid.isEmpty()) {
sb.append(_FINDER_COLUMN_UUID_G_UUID_3);
}
else {
bindUuid = true;
sb.append(_FINDER_COLUMN_UUID_G_UUID_2);
}
sb.append(_FINDER_COLUMN_UUID_G_GROUPID_2);
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(FragmentEntryModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
if (bindUuid) {
queryPos.add(uuid);
}
queryPos.add(groupId);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
fragmentEntry)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the fragment entries where uuid = ? and groupId = ? from the database.
*
* @param uuid the uuid
* @param groupId the group ID
*/
@Override
public void removeByUUID_G(String uuid, long groupId) {
for (FragmentEntry fragmentEntry :
findByUUID_G(
uuid, groupId, QueryUtil.ALL_POS, QueryUtil.ALL_POS,
null)) {
remove(fragmentEntry);
}
}
/**
* Returns the number of fragment entries where uuid = ? and groupId = ?.
*
* @param uuid the uuid
* @param groupId the group ID
* @return the number of matching fragment entries
*/
@Override
public int countByUUID_G(String uuid, long groupId) {
uuid = Objects.toString(uuid, "");
boolean productionMode = ctPersistenceHelper.isProductionMode(
FragmentEntry.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
Long count = null;
if (productionMode) {
finderPath = _finderPathCountByUUID_G;
finderArgs = new Object[] {uuid, groupId};
count = (Long)finderCache.getResult(finderPath, finderArgs);
}
if (count == null) {
StringBundler sb = new StringBundler(3);
sb.append(_SQL_COUNT_FRAGMENTENTRY_WHERE);
boolean bindUuid = false;
if (uuid.isEmpty()) {
sb.append(_FINDER_COLUMN_UUID_G_UUID_3);
}
else {
bindUuid = true;
sb.append(_FINDER_COLUMN_UUID_G_UUID_2);
}
sb.append(_FINDER_COLUMN_UUID_G_GROUPID_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
if (bindUuid) {
queryPos.add(uuid);
}
queryPos.add(groupId);
count = (Long)query.uniqueResult();
if (productionMode) {
finderCache.putResult(finderPath, finderArgs, count);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String _FINDER_COLUMN_UUID_G_UUID_2 =
"fragmentEntry.uuid = ? AND ";
private static final String _FINDER_COLUMN_UUID_G_UUID_3 =
"(fragmentEntry.uuid IS NULL OR fragmentEntry.uuid = '') AND ";
private static final String _FINDER_COLUMN_UUID_G_GROUPID_2 =
"fragmentEntry.groupId = ?";
private FinderPath _finderPathFetchByUUID_G_Head;
private FinderPath _finderPathCountByUUID_G_Head;
/**
* Returns the fragment entry where uuid = ? and groupId = ? and head = ? or throws a NoSuchEntryException
if it could not be found.
*
* @param uuid the uuid
* @param groupId the group ID
* @param head the head
* @return the matching fragment entry
* @throws NoSuchEntryException if a matching fragment entry could not be found
*/
@Override
public FragmentEntry findByUUID_G_Head(
String uuid, long groupId, boolean head)
throws NoSuchEntryException {
FragmentEntry fragmentEntry = fetchByUUID_G_Head(uuid, groupId, head);
if (fragmentEntry == null) {
StringBundler sb = new StringBundler(8);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("uuid=");
sb.append(uuid);
sb.append(", groupId=");
sb.append(groupId);
sb.append(", head=");
sb.append(head);
sb.append("}");
if (_log.isDebugEnabled()) {
_log.debug(sb.toString());
}
throw new NoSuchEntryException(sb.toString());
}
return fragmentEntry;
}
/**
* Returns the fragment entry where uuid = ? and groupId = ? and head = ? or returns null
if it could not be found. Uses the finder cache.
*
* @param uuid the uuid
* @param groupId the group ID
* @param head the head
* @return the matching fragment entry, or null
if a matching fragment entry could not be found
*/
@Override
public FragmentEntry fetchByUUID_G_Head(
String uuid, long groupId, boolean head) {
return fetchByUUID_G_Head(uuid, groupId, head, true);
}
/**
* Returns the fragment entry where uuid = ? and groupId = ? and head = ? or returns null
if it could not be found, optionally using the finder cache.
*
* @param uuid the uuid
* @param groupId the group ID
* @param head the head
* @param useFinderCache whether to use the finder cache
* @return the matching fragment entry, or null
if a matching fragment entry could not be found
*/
@Override
public FragmentEntry fetchByUUID_G_Head(
String uuid, long groupId, boolean head, boolean useFinderCache) {
uuid = Objects.toString(uuid, "");
boolean productionMode = ctPersistenceHelper.isProductionMode(
FragmentEntry.class);
Object[] finderArgs = null;
if (useFinderCache && productionMode) {
finderArgs = new Object[] {uuid, groupId, head};
}
Object result = null;
if (useFinderCache && productionMode) {
result = finderCache.getResult(
_finderPathFetchByUUID_G_Head, finderArgs);
}
if (result instanceof FragmentEntry) {
FragmentEntry fragmentEntry = (FragmentEntry)result;
if (!Objects.equals(uuid, fragmentEntry.getUuid()) ||
(groupId != fragmentEntry.getGroupId()) ||
(head != fragmentEntry.isHead())) {
result = null;
}
}
if (result == null) {
StringBundler sb = new StringBundler(5);
sb.append(_SQL_SELECT_FRAGMENTENTRY_WHERE);
boolean bindUuid = false;
if (uuid.isEmpty()) {
sb.append(_FINDER_COLUMN_UUID_G_HEAD_UUID_3);
}
else {
bindUuid = true;
sb.append(_FINDER_COLUMN_UUID_G_HEAD_UUID_2);
}
sb.append(_FINDER_COLUMN_UUID_G_HEAD_GROUPID_2);
sb.append(_FINDER_COLUMN_UUID_G_HEAD_HEAD_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
if (bindUuid) {
queryPos.add(uuid);
}
queryPos.add(groupId);
queryPos.add(head);
List list = query.list();
if (list.isEmpty()) {
if (useFinderCache && productionMode) {
finderCache.putResult(
_finderPathFetchByUUID_G_Head, finderArgs, list);
}
}
else {
FragmentEntry fragmentEntry = list.get(0);
result = fragmentEntry;
cacheResult(fragmentEntry);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
if (result instanceof List>) {
return null;
}
else {
return (FragmentEntry)result;
}
}
/**
* Removes the fragment entry where uuid = ? and groupId = ? and head = ? from the database.
*
* @param uuid the uuid
* @param groupId the group ID
* @param head the head
* @return the fragment entry that was removed
*/
@Override
public FragmentEntry removeByUUID_G_Head(
String uuid, long groupId, boolean head)
throws NoSuchEntryException {
FragmentEntry fragmentEntry = findByUUID_G_Head(uuid, groupId, head);
return remove(fragmentEntry);
}
/**
* Returns the number of fragment entries where uuid = ? and groupId = ? and head = ?.
*
* @param uuid the uuid
* @param groupId the group ID
* @param head the head
* @return the number of matching fragment entries
*/
@Override
public int countByUUID_G_Head(String uuid, long groupId, boolean head) {
uuid = Objects.toString(uuid, "");
boolean productionMode = ctPersistenceHelper.isProductionMode(
FragmentEntry.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
Long count = null;
if (productionMode) {
finderPath = _finderPathCountByUUID_G_Head;
finderArgs = new Object[] {uuid, groupId, head};
count = (Long)finderCache.getResult(finderPath, finderArgs);
}
if (count == null) {
StringBundler sb = new StringBundler(4);
sb.append(_SQL_COUNT_FRAGMENTENTRY_WHERE);
boolean bindUuid = false;
if (uuid.isEmpty()) {
sb.append(_FINDER_COLUMN_UUID_G_HEAD_UUID_3);
}
else {
bindUuid = true;
sb.append(_FINDER_COLUMN_UUID_G_HEAD_UUID_2);
}
sb.append(_FINDER_COLUMN_UUID_G_HEAD_GROUPID_2);
sb.append(_FINDER_COLUMN_UUID_G_HEAD_HEAD_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
if (bindUuid) {
queryPos.add(uuid);
}
queryPos.add(groupId);
queryPos.add(head);
count = (Long)query.uniqueResult();
if (productionMode) {
finderCache.putResult(finderPath, finderArgs, count);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String _FINDER_COLUMN_UUID_G_HEAD_UUID_2 =
"fragmentEntry.uuid = ? AND ";
private static final String _FINDER_COLUMN_UUID_G_HEAD_UUID_3 =
"(fragmentEntry.uuid IS NULL OR fragmentEntry.uuid = '') AND ";
private static final String _FINDER_COLUMN_UUID_G_HEAD_GROUPID_2 =
"fragmentEntry.groupId = ? AND ";
private static final String _FINDER_COLUMN_UUID_G_HEAD_HEAD_2 =
"fragmentEntry.head = ?";
private FinderPath _finderPathWithPaginationFindByUuid_C;
private FinderPath _finderPathWithoutPaginationFindByUuid_C;
private FinderPath _finderPathCountByUuid_C;
/**
* Returns all the fragment entries where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @return the matching fragment entries
*/
@Override
public List findByUuid_C(String uuid, long companyId) {
return findByUuid_C(
uuid, companyId, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the fragment entries where uuid = ? and companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryModelImpl
.
*
*
* @param uuid the uuid
* @param companyId the company ID
* @param start the lower bound of the range of fragment entries
* @param end the upper bound of the range of fragment entries (not inclusive)
* @return the range of matching fragment entries
*/
@Override
public List findByUuid_C(
String uuid, long companyId, int start, int end) {
return findByUuid_C(uuid, companyId, start, end, null);
}
/**
* Returns an ordered range of all the fragment entries where uuid = ? and companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryModelImpl
.
*
*
* @param uuid the uuid
* @param companyId the company ID
* @param start the lower bound of the range of fragment entries
* @param end the upper bound of the range of fragment entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entries
*/
@Override
public List findByUuid_C(
String uuid, long companyId, int start, int end,
OrderByComparator orderByComparator) {
return findByUuid_C(
uuid, companyId, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the fragment entries where uuid = ? and companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryModelImpl
.
*
*
* @param uuid the uuid
* @param companyId the company ID
* @param start the lower bound of the range of fragment entries
* @param end the upper bound of the range of fragment entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching fragment entries
*/
@Override
public List findByUuid_C(
String uuid, long companyId, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
uuid = Objects.toString(uuid, "");
boolean productionMode = ctPersistenceHelper.isProductionMode(
FragmentEntry.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache && productionMode) {
finderPath = _finderPathWithoutPaginationFindByUuid_C;
finderArgs = new Object[] {uuid, companyId};
}
}
else if (useFinderCache && productionMode) {
finderPath = _finderPathWithPaginationFindByUuid_C;
finderArgs = new Object[] {
uuid, companyId, start, end, orderByComparator
};
}
List list = null;
if (useFinderCache && productionMode) {
list = (List)finderCache.getResult(
finderPath, finderArgs);
if ((list != null) && !list.isEmpty()) {
for (FragmentEntry fragmentEntry : list) {
if (!uuid.equals(fragmentEntry.getUuid()) ||
(companyId != fragmentEntry.getCompanyId())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_FRAGMENTENTRY_WHERE);
boolean bindUuid = false;
if (uuid.isEmpty()) {
sb.append(_FINDER_COLUMN_UUID_C_UUID_3);
}
else {
bindUuid = true;
sb.append(_FINDER_COLUMN_UUID_C_UUID_2);
}
sb.append(_FINDER_COLUMN_UUID_C_COMPANYID_2);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(FragmentEntryModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
if (bindUuid) {
queryPos.add(uuid);
}
queryPos.add(companyId);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache && productionMode) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first fragment entry in the ordered set where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry
* @throws NoSuchEntryException if a matching fragment entry could not be found
*/
@Override
public FragmentEntry findByUuid_C_First(
String uuid, long companyId,
OrderByComparator orderByComparator)
throws NoSuchEntryException {
FragmentEntry fragmentEntry = fetchByUuid_C_First(
uuid, companyId, orderByComparator);
if (fragmentEntry != null) {
return fragmentEntry;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("uuid=");
sb.append(uuid);
sb.append(", companyId=");
sb.append(companyId);
sb.append("}");
throw new NoSuchEntryException(sb.toString());
}
/**
* Returns the first fragment entry in the ordered set where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry, or null
if a matching fragment entry could not be found
*/
@Override
public FragmentEntry fetchByUuid_C_First(
String uuid, long companyId,
OrderByComparator orderByComparator) {
List list = findByUuid_C(
uuid, companyId, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last fragment entry in the ordered set where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry
* @throws NoSuchEntryException if a matching fragment entry could not be found
*/
@Override
public FragmentEntry findByUuid_C_Last(
String uuid, long companyId,
OrderByComparator orderByComparator)
throws NoSuchEntryException {
FragmentEntry fragmentEntry = fetchByUuid_C_Last(
uuid, companyId, orderByComparator);
if (fragmentEntry != null) {
return fragmentEntry;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("uuid=");
sb.append(uuid);
sb.append(", companyId=");
sb.append(companyId);
sb.append("}");
throw new NoSuchEntryException(sb.toString());
}
/**
* Returns the last fragment entry in the ordered set where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry, or null
if a matching fragment entry could not be found
*/
@Override
public FragmentEntry fetchByUuid_C_Last(
String uuid, long companyId,
OrderByComparator orderByComparator) {
int count = countByUuid_C(uuid, companyId);
if (count == 0) {
return null;
}
List list = findByUuid_C(
uuid, companyId, count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the fragment entries before and after the current fragment entry in the ordered set where uuid = ? and companyId = ?.
*
* @param fragmentEntryId the primary key of the current fragment entry
* @param uuid the uuid
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next fragment entry
* @throws NoSuchEntryException if a fragment entry with the primary key could not be found
*/
@Override
public FragmentEntry[] findByUuid_C_PrevAndNext(
long fragmentEntryId, String uuid, long companyId,
OrderByComparator orderByComparator)
throws NoSuchEntryException {
uuid = Objects.toString(uuid, "");
FragmentEntry fragmentEntry = findByPrimaryKey(fragmentEntryId);
Session session = null;
try {
session = openSession();
FragmentEntry[] array = new FragmentEntryImpl[3];
array[0] = getByUuid_C_PrevAndNext(
session, fragmentEntry, uuid, companyId, orderByComparator,
true);
array[1] = fragmentEntry;
array[2] = getByUuid_C_PrevAndNext(
session, fragmentEntry, uuid, companyId, orderByComparator,
false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected FragmentEntry getByUuid_C_PrevAndNext(
Session session, FragmentEntry fragmentEntry, String uuid,
long companyId, OrderByComparator orderByComparator,
boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
5 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_FRAGMENTENTRY_WHERE);
boolean bindUuid = false;
if (uuid.isEmpty()) {
sb.append(_FINDER_COLUMN_UUID_C_UUID_3);
}
else {
bindUuid = true;
sb.append(_FINDER_COLUMN_UUID_C_UUID_2);
}
sb.append(_FINDER_COLUMN_UUID_C_COMPANYID_2);
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(FragmentEntryModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
if (bindUuid) {
queryPos.add(uuid);
}
queryPos.add(companyId);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
fragmentEntry)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the fragment entries where uuid = ? and companyId = ? from the database.
*
* @param uuid the uuid
* @param companyId the company ID
*/
@Override
public void removeByUuid_C(String uuid, long companyId) {
for (FragmentEntry fragmentEntry :
findByUuid_C(
uuid, companyId, QueryUtil.ALL_POS, QueryUtil.ALL_POS,
null)) {
remove(fragmentEntry);
}
}
/**
* Returns the number of fragment entries where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @return the number of matching fragment entries
*/
@Override
public int countByUuid_C(String uuid, long companyId) {
uuid = Objects.toString(uuid, "");
boolean productionMode = ctPersistenceHelper.isProductionMode(
FragmentEntry.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
Long count = null;
if (productionMode) {
finderPath = _finderPathCountByUuid_C;
finderArgs = new Object[] {uuid, companyId};
count = (Long)finderCache.getResult(finderPath, finderArgs);
}
if (count == null) {
StringBundler sb = new StringBundler(3);
sb.append(_SQL_COUNT_FRAGMENTENTRY_WHERE);
boolean bindUuid = false;
if (uuid.isEmpty()) {
sb.append(_FINDER_COLUMN_UUID_C_UUID_3);
}
else {
bindUuid = true;
sb.append(_FINDER_COLUMN_UUID_C_UUID_2);
}
sb.append(_FINDER_COLUMN_UUID_C_COMPANYID_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
if (bindUuid) {
queryPos.add(uuid);
}
queryPos.add(companyId);
count = (Long)query.uniqueResult();
if (productionMode) {
finderCache.putResult(finderPath, finderArgs, count);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String _FINDER_COLUMN_UUID_C_UUID_2 =
"fragmentEntry.uuid = ? AND ";
private static final String _FINDER_COLUMN_UUID_C_UUID_3 =
"(fragmentEntry.uuid IS NULL OR fragmentEntry.uuid = '') AND ";
private static final String _FINDER_COLUMN_UUID_C_COMPANYID_2 =
"fragmentEntry.companyId = ?";
private FinderPath _finderPathWithPaginationFindByUuid_C_Head;
private FinderPath _finderPathWithoutPaginationFindByUuid_C_Head;
private FinderPath _finderPathCountByUuid_C_Head;
/**
* Returns all the fragment entries where uuid = ? and companyId = ? and head = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @param head the head
* @return the matching fragment entries
*/
@Override
public List findByUuid_C_Head(
String uuid, long companyId, boolean head) {
return findByUuid_C_Head(
uuid, companyId, head, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the fragment entries where uuid = ? and companyId = ? and head = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryModelImpl
.
*
*
* @param uuid the uuid
* @param companyId the company ID
* @param head the head
* @param start the lower bound of the range of fragment entries
* @param end the upper bound of the range of fragment entries (not inclusive)
* @return the range of matching fragment entries
*/
@Override
public List findByUuid_C_Head(
String uuid, long companyId, boolean head, int start, int end) {
return findByUuid_C_Head(uuid, companyId, head, start, end, null);
}
/**
* Returns an ordered range of all the fragment entries where uuid = ? and companyId = ? and head = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryModelImpl
.
*
*
* @param uuid the uuid
* @param companyId the company ID
* @param head the head
* @param start the lower bound of the range of fragment entries
* @param end the upper bound of the range of fragment entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entries
*/
@Override
public List findByUuid_C_Head(
String uuid, long companyId, boolean head, int start, int end,
OrderByComparator orderByComparator) {
return findByUuid_C_Head(
uuid, companyId, head, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the fragment entries where uuid = ? and companyId = ? and head = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryModelImpl
.
*
*
* @param uuid the uuid
* @param companyId the company ID
* @param head the head
* @param start the lower bound of the range of fragment entries
* @param end the upper bound of the range of fragment entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching fragment entries
*/
@Override
public List findByUuid_C_Head(
String uuid, long companyId, boolean head, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
uuid = Objects.toString(uuid, "");
boolean productionMode = ctPersistenceHelper.isProductionMode(
FragmentEntry.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache && productionMode) {
finderPath = _finderPathWithoutPaginationFindByUuid_C_Head;
finderArgs = new Object[] {uuid, companyId, head};
}
}
else if (useFinderCache && productionMode) {
finderPath = _finderPathWithPaginationFindByUuid_C_Head;
finderArgs = new Object[] {
uuid, companyId, head, start, end, orderByComparator
};
}
List list = null;
if (useFinderCache && productionMode) {
list = (List)finderCache.getResult(
finderPath, finderArgs);
if ((list != null) && !list.isEmpty()) {
for (FragmentEntry fragmentEntry : list) {
if (!uuid.equals(fragmentEntry.getUuid()) ||
(companyId != fragmentEntry.getCompanyId()) ||
(head != fragmentEntry.isHead())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
5 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(5);
}
sb.append(_SQL_SELECT_FRAGMENTENTRY_WHERE);
boolean bindUuid = false;
if (uuid.isEmpty()) {
sb.append(_FINDER_COLUMN_UUID_C_HEAD_UUID_3);
}
else {
bindUuid = true;
sb.append(_FINDER_COLUMN_UUID_C_HEAD_UUID_2);
}
sb.append(_FINDER_COLUMN_UUID_C_HEAD_COMPANYID_2);
sb.append(_FINDER_COLUMN_UUID_C_HEAD_HEAD_2);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(FragmentEntryModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
if (bindUuid) {
queryPos.add(uuid);
}
queryPos.add(companyId);
queryPos.add(head);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache && productionMode) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first fragment entry in the ordered set where uuid = ? and companyId = ? and head = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @param head the head
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry
* @throws NoSuchEntryException if a matching fragment entry could not be found
*/
@Override
public FragmentEntry findByUuid_C_Head_First(
String uuid, long companyId, boolean head,
OrderByComparator orderByComparator)
throws NoSuchEntryException {
FragmentEntry fragmentEntry = fetchByUuid_C_Head_First(
uuid, companyId, head, orderByComparator);
if (fragmentEntry != null) {
return fragmentEntry;
}
StringBundler sb = new StringBundler(8);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("uuid=");
sb.append(uuid);
sb.append(", companyId=");
sb.append(companyId);
sb.append(", head=");
sb.append(head);
sb.append("}");
throw new NoSuchEntryException(sb.toString());
}
/**
* Returns the first fragment entry in the ordered set where uuid = ? and companyId = ? and head = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @param head the head
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry, or null
if a matching fragment entry could not be found
*/
@Override
public FragmentEntry fetchByUuid_C_Head_First(
String uuid, long companyId, boolean head,
OrderByComparator orderByComparator) {
List list = findByUuid_C_Head(
uuid, companyId, head, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last fragment entry in the ordered set where uuid = ? and companyId = ? and head = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @param head the head
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry
* @throws NoSuchEntryException if a matching fragment entry could not be found
*/
@Override
public FragmentEntry findByUuid_C_Head_Last(
String uuid, long companyId, boolean head,
OrderByComparator orderByComparator)
throws NoSuchEntryException {
FragmentEntry fragmentEntry = fetchByUuid_C_Head_Last(
uuid, companyId, head, orderByComparator);
if (fragmentEntry != null) {
return fragmentEntry;
}
StringBundler sb = new StringBundler(8);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("uuid=");
sb.append(uuid);
sb.append(", companyId=");
sb.append(companyId);
sb.append(", head=");
sb.append(head);
sb.append("}");
throw new NoSuchEntryException(sb.toString());
}
/**
* Returns the last fragment entry in the ordered set where uuid = ? and companyId = ? and head = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @param head the head
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry, or null
if a matching fragment entry could not be found
*/
@Override
public FragmentEntry fetchByUuid_C_Head_Last(
String uuid, long companyId, boolean head,
OrderByComparator orderByComparator) {
int count = countByUuid_C_Head(uuid, companyId, head);
if (count == 0) {
return null;
}
List list = findByUuid_C_Head(
uuid, companyId, head, count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the fragment entries before and after the current fragment entry in the ordered set where uuid = ? and companyId = ? and head = ?.
*
* @param fragmentEntryId the primary key of the current fragment entry
* @param uuid the uuid
* @param companyId the company ID
* @param head the head
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next fragment entry
* @throws NoSuchEntryException if a fragment entry with the primary key could not be found
*/
@Override
public FragmentEntry[] findByUuid_C_Head_PrevAndNext(
long fragmentEntryId, String uuid, long companyId, boolean head,
OrderByComparator orderByComparator)
throws NoSuchEntryException {
uuid = Objects.toString(uuid, "");
FragmentEntry fragmentEntry = findByPrimaryKey(fragmentEntryId);
Session session = null;
try {
session = openSession();
FragmentEntry[] array = new FragmentEntryImpl[3];
array[0] = getByUuid_C_Head_PrevAndNext(
session, fragmentEntry, uuid, companyId, head,
orderByComparator, true);
array[1] = fragmentEntry;
array[2] = getByUuid_C_Head_PrevAndNext(
session, fragmentEntry, uuid, companyId, head,
orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected FragmentEntry getByUuid_C_Head_PrevAndNext(
Session session, FragmentEntry fragmentEntry, String uuid,
long companyId, boolean head,
OrderByComparator orderByComparator, boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
6 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(5);
}
sb.append(_SQL_SELECT_FRAGMENTENTRY_WHERE);
boolean bindUuid = false;
if (uuid.isEmpty()) {
sb.append(_FINDER_COLUMN_UUID_C_HEAD_UUID_3);
}
else {
bindUuid = true;
sb.append(_FINDER_COLUMN_UUID_C_HEAD_UUID_2);
}
sb.append(_FINDER_COLUMN_UUID_C_HEAD_COMPANYID_2);
sb.append(_FINDER_COLUMN_UUID_C_HEAD_HEAD_2);
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(FragmentEntryModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
if (bindUuid) {
queryPos.add(uuid);
}
queryPos.add(companyId);
queryPos.add(head);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
fragmentEntry)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the fragment entries where uuid = ? and companyId = ? and head = ? from the database.
*
* @param uuid the uuid
* @param companyId the company ID
* @param head the head
*/
@Override
public void removeByUuid_C_Head(String uuid, long companyId, boolean head) {
for (FragmentEntry fragmentEntry :
findByUuid_C_Head(
uuid, companyId, head, QueryUtil.ALL_POS, QueryUtil.ALL_POS,
null)) {
remove(fragmentEntry);
}
}
/**
* Returns the number of fragment entries where uuid = ? and companyId = ? and head = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @param head the head
* @return the number of matching fragment entries
*/
@Override
public int countByUuid_C_Head(String uuid, long companyId, boolean head) {
uuid = Objects.toString(uuid, "");
boolean productionMode = ctPersistenceHelper.isProductionMode(
FragmentEntry.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
Long count = null;
if (productionMode) {
finderPath = _finderPathCountByUuid_C_Head;
finderArgs = new Object[] {uuid, companyId, head};
count = (Long)finderCache.getResult(finderPath, finderArgs);
}
if (count == null) {
StringBundler sb = new StringBundler(4);
sb.append(_SQL_COUNT_FRAGMENTENTRY_WHERE);
boolean bindUuid = false;
if (uuid.isEmpty()) {
sb.append(_FINDER_COLUMN_UUID_C_HEAD_UUID_3);
}
else {
bindUuid = true;
sb.append(_FINDER_COLUMN_UUID_C_HEAD_UUID_2);
}
sb.append(_FINDER_COLUMN_UUID_C_HEAD_COMPANYID_2);
sb.append(_FINDER_COLUMN_UUID_C_HEAD_HEAD_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
if (bindUuid) {
queryPos.add(uuid);
}
queryPos.add(companyId);
queryPos.add(head);
count = (Long)query.uniqueResult();
if (productionMode) {
finderCache.putResult(finderPath, finderArgs, count);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String _FINDER_COLUMN_UUID_C_HEAD_UUID_2 =
"fragmentEntry.uuid = ? AND ";
private static final String _FINDER_COLUMN_UUID_C_HEAD_UUID_3 =
"(fragmentEntry.uuid IS NULL OR fragmentEntry.uuid = '') AND ";
private static final String _FINDER_COLUMN_UUID_C_HEAD_COMPANYID_2 =
"fragmentEntry.companyId = ? AND ";
private static final String _FINDER_COLUMN_UUID_C_HEAD_HEAD_2 =
"fragmentEntry.head = ?";
private FinderPath _finderPathWithPaginationFindByGroupId;
private FinderPath _finderPathWithoutPaginationFindByGroupId;
private FinderPath _finderPathCountByGroupId;
/**
* Returns all the fragment entries where groupId = ?.
*
* @param groupId the group ID
* @return the matching fragment entries
*/
@Override
public List findByGroupId(long groupId) {
return findByGroupId(
groupId, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the fragment entries where groupId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryModelImpl
.
*
*
* @param groupId the group ID
* @param start the lower bound of the range of fragment entries
* @param end the upper bound of the range of fragment entries (not inclusive)
* @return the range of matching fragment entries
*/
@Override
public List findByGroupId(long groupId, int start, int end) {
return findByGroupId(groupId, start, end, null);
}
/**
* Returns an ordered range of all the fragment entries where groupId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryModelImpl
.
*
*
* @param groupId the group ID
* @param start the lower bound of the range of fragment entries
* @param end the upper bound of the range of fragment entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entries
*/
@Override
public List findByGroupId(
long groupId, int start, int end,
OrderByComparator orderByComparator) {
return findByGroupId(groupId, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the fragment entries where groupId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryModelImpl
.
*
*
* @param groupId the group ID
* @param start the lower bound of the range of fragment entries
* @param end the upper bound of the range of fragment entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching fragment entries
*/
@Override
public List findByGroupId(
long groupId, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
boolean productionMode = ctPersistenceHelper.isProductionMode(
FragmentEntry.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache && productionMode) {
finderPath = _finderPathWithoutPaginationFindByGroupId;
finderArgs = new Object[] {groupId};
}
}
else if (useFinderCache && productionMode) {
finderPath = _finderPathWithPaginationFindByGroupId;
finderArgs = new Object[] {groupId, start, end, orderByComparator};
}
List list = null;
if (useFinderCache && productionMode) {
list = (List)finderCache.getResult(
finderPath, finderArgs);
if ((list != null) && !list.isEmpty()) {
for (FragmentEntry fragmentEntry : list) {
if (groupId != fragmentEntry.getGroupId()) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
3 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(3);
}
sb.append(_SQL_SELECT_FRAGMENTENTRY_WHERE);
sb.append(_FINDER_COLUMN_GROUPID_GROUPID_2);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(FragmentEntryModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache && productionMode) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first fragment entry in the ordered set where groupId = ?.
*
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry
* @throws NoSuchEntryException if a matching fragment entry could not be found
*/
@Override
public FragmentEntry findByGroupId_First(
long groupId, OrderByComparator orderByComparator)
throws NoSuchEntryException {
FragmentEntry fragmentEntry = fetchByGroupId_First(
groupId, orderByComparator);
if (fragmentEntry != null) {
return fragmentEntry;
}
StringBundler sb = new StringBundler(4);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("groupId=");
sb.append(groupId);
sb.append("}");
throw new NoSuchEntryException(sb.toString());
}
/**
* Returns the first fragment entry in the ordered set where groupId = ?.
*
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry, or null
if a matching fragment entry could not be found
*/
@Override
public FragmentEntry fetchByGroupId_First(
long groupId, OrderByComparator orderByComparator) {
List list = findByGroupId(
groupId, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last fragment entry in the ordered set where groupId = ?.
*
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry
* @throws NoSuchEntryException if a matching fragment entry could not be found
*/
@Override
public FragmentEntry findByGroupId_Last(
long groupId, OrderByComparator orderByComparator)
throws NoSuchEntryException {
FragmentEntry fragmentEntry = fetchByGroupId_Last(
groupId, orderByComparator);
if (fragmentEntry != null) {
return fragmentEntry;
}
StringBundler sb = new StringBundler(4);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("groupId=");
sb.append(groupId);
sb.append("}");
throw new NoSuchEntryException(sb.toString());
}
/**
* Returns the last fragment entry in the ordered set where groupId = ?.
*
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry, or null
if a matching fragment entry could not be found
*/
@Override
public FragmentEntry fetchByGroupId_Last(
long groupId, OrderByComparator orderByComparator) {
int count = countByGroupId(groupId);
if (count == 0) {
return null;
}
List list = findByGroupId(
groupId, count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the fragment entries before and after the current fragment entry in the ordered set where groupId = ?.
*
* @param fragmentEntryId the primary key of the current fragment entry
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next fragment entry
* @throws NoSuchEntryException if a fragment entry with the primary key could not be found
*/
@Override
public FragmentEntry[] findByGroupId_PrevAndNext(
long fragmentEntryId, long groupId,
OrderByComparator orderByComparator)
throws NoSuchEntryException {
FragmentEntry fragmentEntry = findByPrimaryKey(fragmentEntryId);
Session session = null;
try {
session = openSession();
FragmentEntry[] array = new FragmentEntryImpl[3];
array[0] = getByGroupId_PrevAndNext(
session, fragmentEntry, groupId, orderByComparator, true);
array[1] = fragmentEntry;
array[2] = getByGroupId_PrevAndNext(
session, fragmentEntry, groupId, orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected FragmentEntry getByGroupId_PrevAndNext(
Session session, FragmentEntry fragmentEntry, long groupId,
OrderByComparator orderByComparator, boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(3);
}
sb.append(_SQL_SELECT_FRAGMENTENTRY_WHERE);
sb.append(_FINDER_COLUMN_GROUPID_GROUPID_2);
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(FragmentEntryModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
fragmentEntry)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the fragment entries where groupId = ? from the database.
*
* @param groupId the group ID
*/
@Override
public void removeByGroupId(long groupId) {
for (FragmentEntry fragmentEntry :
findByGroupId(
groupId, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null)) {
remove(fragmentEntry);
}
}
/**
* Returns the number of fragment entries where groupId = ?.
*
* @param groupId the group ID
* @return the number of matching fragment entries
*/
@Override
public int countByGroupId(long groupId) {
boolean productionMode = ctPersistenceHelper.isProductionMode(
FragmentEntry.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
Long count = null;
if (productionMode) {
finderPath = _finderPathCountByGroupId;
finderArgs = new Object[] {groupId};
count = (Long)finderCache.getResult(finderPath, finderArgs);
}
if (count == null) {
StringBundler sb = new StringBundler(2);
sb.append(_SQL_COUNT_FRAGMENTENTRY_WHERE);
sb.append(_FINDER_COLUMN_GROUPID_GROUPID_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
count = (Long)query.uniqueResult();
if (productionMode) {
finderCache.putResult(finderPath, finderArgs, count);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String _FINDER_COLUMN_GROUPID_GROUPID_2 =
"fragmentEntry.groupId = ?";
private FinderPath _finderPathWithPaginationFindByGroupId_Head;
private FinderPath _finderPathWithoutPaginationFindByGroupId_Head;
private FinderPath _finderPathCountByGroupId_Head;
/**
* Returns all the fragment entries where groupId = ? and head = ?.
*
* @param groupId the group ID
* @param head the head
* @return the matching fragment entries
*/
@Override
public List findByGroupId_Head(long groupId, boolean head) {
return findByGroupId_Head(
groupId, head, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the fragment entries where groupId = ? and head = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryModelImpl
.
*
*
* @param groupId the group ID
* @param head the head
* @param start the lower bound of the range of fragment entries
* @param end the upper bound of the range of fragment entries (not inclusive)
* @return the range of matching fragment entries
*/
@Override
public List findByGroupId_Head(
long groupId, boolean head, int start, int end) {
return findByGroupId_Head(groupId, head, start, end, null);
}
/**
* Returns an ordered range of all the fragment entries where groupId = ? and head = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryModelImpl
.
*
*
* @param groupId the group ID
* @param head the head
* @param start the lower bound of the range of fragment entries
* @param end the upper bound of the range of fragment entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entries
*/
@Override
public List findByGroupId_Head(
long groupId, boolean head, int start, int end,
OrderByComparator orderByComparator) {
return findByGroupId_Head(
groupId, head, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the fragment entries where groupId = ? and head = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryModelImpl
.
*
*
* @param groupId the group ID
* @param head the head
* @param start the lower bound of the range of fragment entries
* @param end the upper bound of the range of fragment entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching fragment entries
*/
@Override
public List findByGroupId_Head(
long groupId, boolean head, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
boolean productionMode = ctPersistenceHelper.isProductionMode(
FragmentEntry.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache && productionMode) {
finderPath = _finderPathWithoutPaginationFindByGroupId_Head;
finderArgs = new Object[] {groupId, head};
}
}
else if (useFinderCache && productionMode) {
finderPath = _finderPathWithPaginationFindByGroupId_Head;
finderArgs = new Object[] {
groupId, head, start, end, orderByComparator
};
}
List list = null;
if (useFinderCache && productionMode) {
list = (List)finderCache.getResult(
finderPath, finderArgs);
if ((list != null) && !list.isEmpty()) {
for (FragmentEntry fragmentEntry : list) {
if ((groupId != fragmentEntry.getGroupId()) ||
(head != fragmentEntry.isHead())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_FRAGMENTENTRY_WHERE);
sb.append(_FINDER_COLUMN_GROUPID_HEAD_GROUPID_2);
sb.append(_FINDER_COLUMN_GROUPID_HEAD_HEAD_2);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(FragmentEntryModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
queryPos.add(head);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache && productionMode) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first fragment entry in the ordered set where groupId = ? and head = ?.
*
* @param groupId the group ID
* @param head the head
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry
* @throws NoSuchEntryException if a matching fragment entry could not be found
*/
@Override
public FragmentEntry findByGroupId_Head_First(
long groupId, boolean head,
OrderByComparator orderByComparator)
throws NoSuchEntryException {
FragmentEntry fragmentEntry = fetchByGroupId_Head_First(
groupId, head, orderByComparator);
if (fragmentEntry != null) {
return fragmentEntry;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("groupId=");
sb.append(groupId);
sb.append(", head=");
sb.append(head);
sb.append("}");
throw new NoSuchEntryException(sb.toString());
}
/**
* Returns the first fragment entry in the ordered set where groupId = ? and head = ?.
*
* @param groupId the group ID
* @param head the head
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry, or null
if a matching fragment entry could not be found
*/
@Override
public FragmentEntry fetchByGroupId_Head_First(
long groupId, boolean head,
OrderByComparator orderByComparator) {
List list = findByGroupId_Head(
groupId, head, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last fragment entry in the ordered set where groupId = ? and head = ?.
*
* @param groupId the group ID
* @param head the head
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry
* @throws NoSuchEntryException if a matching fragment entry could not be found
*/
@Override
public FragmentEntry findByGroupId_Head_Last(
long groupId, boolean head,
OrderByComparator orderByComparator)
throws NoSuchEntryException {
FragmentEntry fragmentEntry = fetchByGroupId_Head_Last(
groupId, head, orderByComparator);
if (fragmentEntry != null) {
return fragmentEntry;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("groupId=");
sb.append(groupId);
sb.append(", head=");
sb.append(head);
sb.append("}");
throw new NoSuchEntryException(sb.toString());
}
/**
* Returns the last fragment entry in the ordered set where groupId = ? and head = ?.
*
* @param groupId the group ID
* @param head the head
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry, or null
if a matching fragment entry could not be found
*/
@Override
public FragmentEntry fetchByGroupId_Head_Last(
long groupId, boolean head,
OrderByComparator orderByComparator) {
int count = countByGroupId_Head(groupId, head);
if (count == 0) {
return null;
}
List list = findByGroupId_Head(
groupId, head, count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the fragment entries before and after the current fragment entry in the ordered set where groupId = ? and head = ?.
*
* @param fragmentEntryId the primary key of the current fragment entry
* @param groupId the group ID
* @param head the head
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next fragment entry
* @throws NoSuchEntryException if a fragment entry with the primary key could not be found
*/
@Override
public FragmentEntry[] findByGroupId_Head_PrevAndNext(
long fragmentEntryId, long groupId, boolean head,
OrderByComparator orderByComparator)
throws NoSuchEntryException {
FragmentEntry fragmentEntry = findByPrimaryKey(fragmentEntryId);
Session session = null;
try {
session = openSession();
FragmentEntry[] array = new FragmentEntryImpl[3];
array[0] = getByGroupId_Head_PrevAndNext(
session, fragmentEntry, groupId, head, orderByComparator, true);
array[1] = fragmentEntry;
array[2] = getByGroupId_Head_PrevAndNext(
session, fragmentEntry, groupId, head, orderByComparator,
false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected FragmentEntry getByGroupId_Head_PrevAndNext(
Session session, FragmentEntry fragmentEntry, long groupId,
boolean head, OrderByComparator orderByComparator,
boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
5 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_FRAGMENTENTRY_WHERE);
sb.append(_FINDER_COLUMN_GROUPID_HEAD_GROUPID_2);
sb.append(_FINDER_COLUMN_GROUPID_HEAD_HEAD_2);
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(FragmentEntryModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
queryPos.add(head);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
fragmentEntry)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the fragment entries where groupId = ? and head = ? from the database.
*
* @param groupId the group ID
* @param head the head
*/
@Override
public void removeByGroupId_Head(long groupId, boolean head) {
for (FragmentEntry fragmentEntry :
findByGroupId_Head(
groupId, head, QueryUtil.ALL_POS, QueryUtil.ALL_POS,
null)) {
remove(fragmentEntry);
}
}
/**
* Returns the number of fragment entries where groupId = ? and head = ?.
*
* @param groupId the group ID
* @param head the head
* @return the number of matching fragment entries
*/
@Override
public int countByGroupId_Head(long groupId, boolean head) {
boolean productionMode = ctPersistenceHelper.isProductionMode(
FragmentEntry.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
Long count = null;
if (productionMode) {
finderPath = _finderPathCountByGroupId_Head;
finderArgs = new Object[] {groupId, head};
count = (Long)finderCache.getResult(finderPath, finderArgs);
}
if (count == null) {
StringBundler sb = new StringBundler(3);
sb.append(_SQL_COUNT_FRAGMENTENTRY_WHERE);
sb.append(_FINDER_COLUMN_GROUPID_HEAD_GROUPID_2);
sb.append(_FINDER_COLUMN_GROUPID_HEAD_HEAD_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
queryPos.add(head);
count = (Long)query.uniqueResult();
if (productionMode) {
finderCache.putResult(finderPath, finderArgs, count);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String _FINDER_COLUMN_GROUPID_HEAD_GROUPID_2 =
"fragmentEntry.groupId = ? AND ";
private static final String _FINDER_COLUMN_GROUPID_HEAD_HEAD_2 =
"fragmentEntry.head = ?";
private FinderPath _finderPathWithPaginationFindByFragmentCollectionId;
private FinderPath _finderPathWithoutPaginationFindByFragmentCollectionId;
private FinderPath _finderPathCountByFragmentCollectionId;
/**
* Returns all the fragment entries where fragmentCollectionId = ?.
*
* @param fragmentCollectionId the fragment collection ID
* @return the matching fragment entries
*/
@Override
public List findByFragmentCollectionId(
long fragmentCollectionId) {
return findByFragmentCollectionId(
fragmentCollectionId, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the fragment entries where fragmentCollectionId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryModelImpl
.
*
*
* @param fragmentCollectionId the fragment collection ID
* @param start the lower bound of the range of fragment entries
* @param end the upper bound of the range of fragment entries (not inclusive)
* @return the range of matching fragment entries
*/
@Override
public List findByFragmentCollectionId(
long fragmentCollectionId, int start, int end) {
return findByFragmentCollectionId(
fragmentCollectionId, start, end, null);
}
/**
* Returns an ordered range of all the fragment entries where fragmentCollectionId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryModelImpl
.
*
*
* @param fragmentCollectionId the fragment collection ID
* @param start the lower bound of the range of fragment entries
* @param end the upper bound of the range of fragment entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entries
*/
@Override
public List findByFragmentCollectionId(
long fragmentCollectionId, int start, int end,
OrderByComparator orderByComparator) {
return findByFragmentCollectionId(
fragmentCollectionId, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the fragment entries where fragmentCollectionId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryModelImpl
.
*
*
* @param fragmentCollectionId the fragment collection ID
* @param start the lower bound of the range of fragment entries
* @param end the upper bound of the range of fragment entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching fragment entries
*/
@Override
public List findByFragmentCollectionId(
long fragmentCollectionId, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
boolean productionMode = ctPersistenceHelper.isProductionMode(
FragmentEntry.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache && productionMode) {
finderPath =
_finderPathWithoutPaginationFindByFragmentCollectionId;
finderArgs = new Object[] {fragmentCollectionId};
}
}
else if (useFinderCache && productionMode) {
finderPath = _finderPathWithPaginationFindByFragmentCollectionId;
finderArgs = new Object[] {
fragmentCollectionId, start, end, orderByComparator
};
}
List list = null;
if (useFinderCache && productionMode) {
list = (List)finderCache.getResult(
finderPath, finderArgs);
if ((list != null) && !list.isEmpty()) {
for (FragmentEntry fragmentEntry : list) {
if (fragmentCollectionId !=
fragmentEntry.getFragmentCollectionId()) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
3 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(3);
}
sb.append(_SQL_SELECT_FRAGMENTENTRY_WHERE);
sb.append(
_FINDER_COLUMN_FRAGMENTCOLLECTIONID_FRAGMENTCOLLECTIONID_2);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(FragmentEntryModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(fragmentCollectionId);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache && productionMode) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first fragment entry in the ordered set where fragmentCollectionId = ?.
*
* @param fragmentCollectionId the fragment collection ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry
* @throws NoSuchEntryException if a matching fragment entry could not be found
*/
@Override
public FragmentEntry findByFragmentCollectionId_First(
long fragmentCollectionId,
OrderByComparator orderByComparator)
throws NoSuchEntryException {
FragmentEntry fragmentEntry = fetchByFragmentCollectionId_First(
fragmentCollectionId, orderByComparator);
if (fragmentEntry != null) {
return fragmentEntry;
}
StringBundler sb = new StringBundler(4);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("fragmentCollectionId=");
sb.append(fragmentCollectionId);
sb.append("}");
throw new NoSuchEntryException(sb.toString());
}
/**
* Returns the first fragment entry in the ordered set where fragmentCollectionId = ?.
*
* @param fragmentCollectionId the fragment collection ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry, or null
if a matching fragment entry could not be found
*/
@Override
public FragmentEntry fetchByFragmentCollectionId_First(
long fragmentCollectionId,
OrderByComparator orderByComparator) {
List list = findByFragmentCollectionId(
fragmentCollectionId, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last fragment entry in the ordered set where fragmentCollectionId = ?.
*
* @param fragmentCollectionId the fragment collection ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry
* @throws NoSuchEntryException if a matching fragment entry could not be found
*/
@Override
public FragmentEntry findByFragmentCollectionId_Last(
long fragmentCollectionId,
OrderByComparator orderByComparator)
throws NoSuchEntryException {
FragmentEntry fragmentEntry = fetchByFragmentCollectionId_Last(
fragmentCollectionId, orderByComparator);
if (fragmentEntry != null) {
return fragmentEntry;
}
StringBundler sb = new StringBundler(4);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("fragmentCollectionId=");
sb.append(fragmentCollectionId);
sb.append("}");
throw new NoSuchEntryException(sb.toString());
}
/**
* Returns the last fragment entry in the ordered set where fragmentCollectionId = ?.
*
* @param fragmentCollectionId the fragment collection ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry, or null
if a matching fragment entry could not be found
*/
@Override
public FragmentEntry fetchByFragmentCollectionId_Last(
long fragmentCollectionId,
OrderByComparator orderByComparator) {
int count = countByFragmentCollectionId(fragmentCollectionId);
if (count == 0) {
return null;
}
List list = findByFragmentCollectionId(
fragmentCollectionId, count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the fragment entries before and after the current fragment entry in the ordered set where fragmentCollectionId = ?.
*
* @param fragmentEntryId the primary key of the current fragment entry
* @param fragmentCollectionId the fragment collection ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next fragment entry
* @throws NoSuchEntryException if a fragment entry with the primary key could not be found
*/
@Override
public FragmentEntry[] findByFragmentCollectionId_PrevAndNext(
long fragmentEntryId, long fragmentCollectionId,
OrderByComparator orderByComparator)
throws NoSuchEntryException {
FragmentEntry fragmentEntry = findByPrimaryKey(fragmentEntryId);
Session session = null;
try {
session = openSession();
FragmentEntry[] array = new FragmentEntryImpl[3];
array[0] = getByFragmentCollectionId_PrevAndNext(
session, fragmentEntry, fragmentCollectionId, orderByComparator,
true);
array[1] = fragmentEntry;
array[2] = getByFragmentCollectionId_PrevAndNext(
session, fragmentEntry, fragmentCollectionId, orderByComparator,
false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected FragmentEntry getByFragmentCollectionId_PrevAndNext(
Session session, FragmentEntry fragmentEntry, long fragmentCollectionId,
OrderByComparator orderByComparator, boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(3);
}
sb.append(_SQL_SELECT_FRAGMENTENTRY_WHERE);
sb.append(_FINDER_COLUMN_FRAGMENTCOLLECTIONID_FRAGMENTCOLLECTIONID_2);
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(FragmentEntryModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(fragmentCollectionId);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
fragmentEntry)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the fragment entries where fragmentCollectionId = ? from the database.
*
* @param fragmentCollectionId the fragment collection ID
*/
@Override
public void removeByFragmentCollectionId(long fragmentCollectionId) {
for (FragmentEntry fragmentEntry :
findByFragmentCollectionId(
fragmentCollectionId, QueryUtil.ALL_POS, QueryUtil.ALL_POS,
null)) {
remove(fragmentEntry);
}
}
/**
* Returns the number of fragment entries where fragmentCollectionId = ?.
*
* @param fragmentCollectionId the fragment collection ID
* @return the number of matching fragment entries
*/
@Override
public int countByFragmentCollectionId(long fragmentCollectionId) {
boolean productionMode = ctPersistenceHelper.isProductionMode(
FragmentEntry.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
Long count = null;
if (productionMode) {
finderPath = _finderPathCountByFragmentCollectionId;
finderArgs = new Object[] {fragmentCollectionId};
count = (Long)finderCache.getResult(finderPath, finderArgs);
}
if (count == null) {
StringBundler sb = new StringBundler(2);
sb.append(_SQL_COUNT_FRAGMENTENTRY_WHERE);
sb.append(
_FINDER_COLUMN_FRAGMENTCOLLECTIONID_FRAGMENTCOLLECTIONID_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(fragmentCollectionId);
count = (Long)query.uniqueResult();
if (productionMode) {
finderCache.putResult(finderPath, finderArgs, count);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String
_FINDER_COLUMN_FRAGMENTCOLLECTIONID_FRAGMENTCOLLECTIONID_2 =
"fragmentEntry.fragmentCollectionId = ?";
private FinderPath _finderPathWithPaginationFindByFragmentCollectionId_Head;
private FinderPath
_finderPathWithoutPaginationFindByFragmentCollectionId_Head;
private FinderPath _finderPathCountByFragmentCollectionId_Head;
/**
* Returns all the fragment entries where fragmentCollectionId = ? and head = ?.
*
* @param fragmentCollectionId the fragment collection ID
* @param head the head
* @return the matching fragment entries
*/
@Override
public List findByFragmentCollectionId_Head(
long fragmentCollectionId, boolean head) {
return findByFragmentCollectionId_Head(
fragmentCollectionId, head, QueryUtil.ALL_POS, QueryUtil.ALL_POS,
null);
}
/**
* Returns a range of all the fragment entries where fragmentCollectionId = ? and head = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryModelImpl
.
*
*
* @param fragmentCollectionId the fragment collection ID
* @param head the head
* @param start the lower bound of the range of fragment entries
* @param end the upper bound of the range of fragment entries (not inclusive)
* @return the range of matching fragment entries
*/
@Override
public List findByFragmentCollectionId_Head(
long fragmentCollectionId, boolean head, int start, int end) {
return findByFragmentCollectionId_Head(
fragmentCollectionId, head, start, end, null);
}
/**
* Returns an ordered range of all the fragment entries where fragmentCollectionId = ? and head = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryModelImpl
.
*
*
* @param fragmentCollectionId the fragment collection ID
* @param head the head
* @param start the lower bound of the range of fragment entries
* @param end the upper bound of the range of fragment entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entries
*/
@Override
public List findByFragmentCollectionId_Head(
long fragmentCollectionId, boolean head, int start, int end,
OrderByComparator orderByComparator) {
return findByFragmentCollectionId_Head(
fragmentCollectionId, head, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the fragment entries where fragmentCollectionId = ? and head = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryModelImpl
.
*
*
* @param fragmentCollectionId the fragment collection ID
* @param head the head
* @param start the lower bound of the range of fragment entries
* @param end the upper bound of the range of fragment entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching fragment entries
*/
@Override
public List findByFragmentCollectionId_Head(
long fragmentCollectionId, boolean head, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
boolean productionMode = ctPersistenceHelper.isProductionMode(
FragmentEntry.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache && productionMode) {
finderPath =
_finderPathWithoutPaginationFindByFragmentCollectionId_Head;
finderArgs = new Object[] {fragmentCollectionId, head};
}
}
else if (useFinderCache && productionMode) {
finderPath =
_finderPathWithPaginationFindByFragmentCollectionId_Head;
finderArgs = new Object[] {
fragmentCollectionId, head, start, end, orderByComparator
};
}
List list = null;
if (useFinderCache && productionMode) {
list = (List)finderCache.getResult(
finderPath, finderArgs);
if ((list != null) && !list.isEmpty()) {
for (FragmentEntry fragmentEntry : list) {
if ((fragmentCollectionId !=
fragmentEntry.getFragmentCollectionId()) ||
(head != fragmentEntry.isHead())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_FRAGMENTENTRY_WHERE);
sb.append(
_FINDER_COLUMN_FRAGMENTCOLLECTIONID_HEAD_FRAGMENTCOLLECTIONID_2);
sb.append(_FINDER_COLUMN_FRAGMENTCOLLECTIONID_HEAD_HEAD_2);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(FragmentEntryModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(fragmentCollectionId);
queryPos.add(head);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache && productionMode) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first fragment entry in the ordered set where fragmentCollectionId = ? and head = ?.
*
* @param fragmentCollectionId the fragment collection ID
* @param head the head
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry
* @throws NoSuchEntryException if a matching fragment entry could not be found
*/
@Override
public FragmentEntry findByFragmentCollectionId_Head_First(
long fragmentCollectionId, boolean head,
OrderByComparator orderByComparator)
throws NoSuchEntryException {
FragmentEntry fragmentEntry = fetchByFragmentCollectionId_Head_First(
fragmentCollectionId, head, orderByComparator);
if (fragmentEntry != null) {
return fragmentEntry;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("fragmentCollectionId=");
sb.append(fragmentCollectionId);
sb.append(", head=");
sb.append(head);
sb.append("}");
throw new NoSuchEntryException(sb.toString());
}
/**
* Returns the first fragment entry in the ordered set where fragmentCollectionId = ? and head = ?.
*
* @param fragmentCollectionId the fragment collection ID
* @param head the head
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry, or null
if a matching fragment entry could not be found
*/
@Override
public FragmentEntry fetchByFragmentCollectionId_Head_First(
long fragmentCollectionId, boolean head,
OrderByComparator orderByComparator) {
List list = findByFragmentCollectionId_Head(
fragmentCollectionId, head, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last fragment entry in the ordered set where fragmentCollectionId = ? and head = ?.
*
* @param fragmentCollectionId the fragment collection ID
* @param head the head
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry
* @throws NoSuchEntryException if a matching fragment entry could not be found
*/
@Override
public FragmentEntry findByFragmentCollectionId_Head_Last(
long fragmentCollectionId, boolean head,
OrderByComparator orderByComparator)
throws NoSuchEntryException {
FragmentEntry fragmentEntry = fetchByFragmentCollectionId_Head_Last(
fragmentCollectionId, head, orderByComparator);
if (fragmentEntry != null) {
return fragmentEntry;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("fragmentCollectionId=");
sb.append(fragmentCollectionId);
sb.append(", head=");
sb.append(head);
sb.append("}");
throw new NoSuchEntryException(sb.toString());
}
/**
* Returns the last fragment entry in the ordered set where fragmentCollectionId = ? and head = ?.
*
* @param fragmentCollectionId the fragment collection ID
* @param head the head
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry, or null
if a matching fragment entry could not be found
*/
@Override
public FragmentEntry fetchByFragmentCollectionId_Head_Last(
long fragmentCollectionId, boolean head,
OrderByComparator orderByComparator) {
int count = countByFragmentCollectionId_Head(
fragmentCollectionId, head);
if (count == 0) {
return null;
}
List list = findByFragmentCollectionId_Head(
fragmentCollectionId, head, count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the fragment entries before and after the current fragment entry in the ordered set where fragmentCollectionId = ? and head = ?.
*
* @param fragmentEntryId the primary key of the current fragment entry
* @param fragmentCollectionId the fragment collection ID
* @param head the head
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next fragment entry
* @throws NoSuchEntryException if a fragment entry with the primary key could not be found
*/
@Override
public FragmentEntry[] findByFragmentCollectionId_Head_PrevAndNext(
long fragmentEntryId, long fragmentCollectionId, boolean head,
OrderByComparator orderByComparator)
throws NoSuchEntryException {
FragmentEntry fragmentEntry = findByPrimaryKey(fragmentEntryId);
Session session = null;
try {
session = openSession();
FragmentEntry[] array = new FragmentEntryImpl[3];
array[0] = getByFragmentCollectionId_Head_PrevAndNext(
session, fragmentEntry, fragmentCollectionId, head,
orderByComparator, true);
array[1] = fragmentEntry;
array[2] = getByFragmentCollectionId_Head_PrevAndNext(
session, fragmentEntry, fragmentCollectionId, head,
orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected FragmentEntry getByFragmentCollectionId_Head_PrevAndNext(
Session session, FragmentEntry fragmentEntry, long fragmentCollectionId,
boolean head, OrderByComparator orderByComparator,
boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
5 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_FRAGMENTENTRY_WHERE);
sb.append(
_FINDER_COLUMN_FRAGMENTCOLLECTIONID_HEAD_FRAGMENTCOLLECTIONID_2);
sb.append(_FINDER_COLUMN_FRAGMENTCOLLECTIONID_HEAD_HEAD_2);
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(FragmentEntryModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(fragmentCollectionId);
queryPos.add(head);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
fragmentEntry)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the fragment entries where fragmentCollectionId = ? and head = ? from the database.
*
* @param fragmentCollectionId the fragment collection ID
* @param head the head
*/
@Override
public void removeByFragmentCollectionId_Head(
long fragmentCollectionId, boolean head) {
for (FragmentEntry fragmentEntry :
findByFragmentCollectionId_Head(
fragmentCollectionId, head, QueryUtil.ALL_POS,
QueryUtil.ALL_POS, null)) {
remove(fragmentEntry);
}
}
/**
* Returns the number of fragment entries where fragmentCollectionId = ? and head = ?.
*
* @param fragmentCollectionId the fragment collection ID
* @param head the head
* @return the number of matching fragment entries
*/
@Override
public int countByFragmentCollectionId_Head(
long fragmentCollectionId, boolean head) {
boolean productionMode = ctPersistenceHelper.isProductionMode(
FragmentEntry.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
Long count = null;
if (productionMode) {
finderPath = _finderPathCountByFragmentCollectionId_Head;
finderArgs = new Object[] {fragmentCollectionId, head};
count = (Long)finderCache.getResult(finderPath, finderArgs);
}
if (count == null) {
StringBundler sb = new StringBundler(3);
sb.append(_SQL_COUNT_FRAGMENTENTRY_WHERE);
sb.append(
_FINDER_COLUMN_FRAGMENTCOLLECTIONID_HEAD_FRAGMENTCOLLECTIONID_2);
sb.append(_FINDER_COLUMN_FRAGMENTCOLLECTIONID_HEAD_HEAD_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(fragmentCollectionId);
queryPos.add(head);
count = (Long)query.uniqueResult();
if (productionMode) {
finderCache.putResult(finderPath, finderArgs, count);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String
_FINDER_COLUMN_FRAGMENTCOLLECTIONID_HEAD_FRAGMENTCOLLECTIONID_2 =
"fragmentEntry.fragmentCollectionId = ? AND ";
private static final String
_FINDER_COLUMN_FRAGMENTCOLLECTIONID_HEAD_HEAD_2 =
"fragmentEntry.head = ?";
private FinderPath _finderPathWithPaginationFindByG_FCI;
private FinderPath _finderPathWithoutPaginationFindByG_FCI;
private FinderPath _finderPathCountByG_FCI;
/**
* Returns all the fragment entries where groupId = ? and fragmentCollectionId = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @return the matching fragment entries
*/
@Override
public List findByG_FCI(
long groupId, long fragmentCollectionId) {
return findByG_FCI(
groupId, fragmentCollectionId, QueryUtil.ALL_POS, QueryUtil.ALL_POS,
null);
}
/**
* Returns a range of all the fragment entries where groupId = ? and fragmentCollectionId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param start the lower bound of the range of fragment entries
* @param end the upper bound of the range of fragment entries (not inclusive)
* @return the range of matching fragment entries
*/
@Override
public List findByG_FCI(
long groupId, long fragmentCollectionId, int start, int end) {
return findByG_FCI(groupId, fragmentCollectionId, start, end, null);
}
/**
* Returns an ordered range of all the fragment entries where groupId = ? and fragmentCollectionId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param start the lower bound of the range of fragment entries
* @param end the upper bound of the range of fragment entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entries
*/
@Override
public List findByG_FCI(
long groupId, long fragmentCollectionId, int start, int end,
OrderByComparator orderByComparator) {
return findByG_FCI(
groupId, fragmentCollectionId, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the fragment entries where groupId = ? and fragmentCollectionId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param start the lower bound of the range of fragment entries
* @param end the upper bound of the range of fragment entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching fragment entries
*/
@Override
public List findByG_FCI(
long groupId, long fragmentCollectionId, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
boolean productionMode = ctPersistenceHelper.isProductionMode(
FragmentEntry.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache && productionMode) {
finderPath = _finderPathWithoutPaginationFindByG_FCI;
finderArgs = new Object[] {groupId, fragmentCollectionId};
}
}
else if (useFinderCache && productionMode) {
finderPath = _finderPathWithPaginationFindByG_FCI;
finderArgs = new Object[] {
groupId, fragmentCollectionId, start, end, orderByComparator
};
}
List list = null;
if (useFinderCache && productionMode) {
list = (List)finderCache.getResult(
finderPath, finderArgs);
if ((list != null) && !list.isEmpty()) {
for (FragmentEntry fragmentEntry : list) {
if ((groupId != fragmentEntry.getGroupId()) ||
(fragmentCollectionId !=
fragmentEntry.getFragmentCollectionId())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_FRAGMENTENTRY_WHERE);
sb.append(_FINDER_COLUMN_G_FCI_GROUPID_2);
sb.append(_FINDER_COLUMN_G_FCI_FRAGMENTCOLLECTIONID_2);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(FragmentEntryModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
queryPos.add(fragmentCollectionId);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache && productionMode) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first fragment entry in the ordered set where groupId = ? and fragmentCollectionId = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry
* @throws NoSuchEntryException if a matching fragment entry could not be found
*/
@Override
public FragmentEntry findByG_FCI_First(
long groupId, long fragmentCollectionId,
OrderByComparator orderByComparator)
throws NoSuchEntryException {
FragmentEntry fragmentEntry = fetchByG_FCI_First(
groupId, fragmentCollectionId, orderByComparator);
if (fragmentEntry != null) {
return fragmentEntry;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("groupId=");
sb.append(groupId);
sb.append(", fragmentCollectionId=");
sb.append(fragmentCollectionId);
sb.append("}");
throw new NoSuchEntryException(sb.toString());
}
/**
* Returns the first fragment entry in the ordered set where groupId = ? and fragmentCollectionId = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry, or null
if a matching fragment entry could not be found
*/
@Override
public FragmentEntry fetchByG_FCI_First(
long groupId, long fragmentCollectionId,
OrderByComparator orderByComparator) {
List list = findByG_FCI(
groupId, fragmentCollectionId, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last fragment entry in the ordered set where groupId = ? and fragmentCollectionId = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry
* @throws NoSuchEntryException if a matching fragment entry could not be found
*/
@Override
public FragmentEntry findByG_FCI_Last(
long groupId, long fragmentCollectionId,
OrderByComparator orderByComparator)
throws NoSuchEntryException {
FragmentEntry fragmentEntry = fetchByG_FCI_Last(
groupId, fragmentCollectionId, orderByComparator);
if (fragmentEntry != null) {
return fragmentEntry;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("groupId=");
sb.append(groupId);
sb.append(", fragmentCollectionId=");
sb.append(fragmentCollectionId);
sb.append("}");
throw new NoSuchEntryException(sb.toString());
}
/**
* Returns the last fragment entry in the ordered set where groupId = ? and fragmentCollectionId = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry, or null
if a matching fragment entry could not be found
*/
@Override
public FragmentEntry fetchByG_FCI_Last(
long groupId, long fragmentCollectionId,
OrderByComparator orderByComparator) {
int count = countByG_FCI(groupId, fragmentCollectionId);
if (count == 0) {
return null;
}
List list = findByG_FCI(
groupId, fragmentCollectionId, count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the fragment entries before and after the current fragment entry in the ordered set where groupId = ? and fragmentCollectionId = ?.
*
* @param fragmentEntryId the primary key of the current fragment entry
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next fragment entry
* @throws NoSuchEntryException if a fragment entry with the primary key could not be found
*/
@Override
public FragmentEntry[] findByG_FCI_PrevAndNext(
long fragmentEntryId, long groupId, long fragmentCollectionId,
OrderByComparator orderByComparator)
throws NoSuchEntryException {
FragmentEntry fragmentEntry = findByPrimaryKey(fragmentEntryId);
Session session = null;
try {
session = openSession();
FragmentEntry[] array = new FragmentEntryImpl[3];
array[0] = getByG_FCI_PrevAndNext(
session, fragmentEntry, groupId, fragmentCollectionId,
orderByComparator, true);
array[1] = fragmentEntry;
array[2] = getByG_FCI_PrevAndNext(
session, fragmentEntry, groupId, fragmentCollectionId,
orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected FragmentEntry getByG_FCI_PrevAndNext(
Session session, FragmentEntry fragmentEntry, long groupId,
long fragmentCollectionId,
OrderByComparator orderByComparator, boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
5 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_FRAGMENTENTRY_WHERE);
sb.append(_FINDER_COLUMN_G_FCI_GROUPID_2);
sb.append(_FINDER_COLUMN_G_FCI_FRAGMENTCOLLECTIONID_2);
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(FragmentEntryModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
queryPos.add(fragmentCollectionId);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
fragmentEntry)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the fragment entries where groupId = ? and fragmentCollectionId = ? from the database.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
*/
@Override
public void removeByG_FCI(long groupId, long fragmentCollectionId) {
for (FragmentEntry fragmentEntry :
findByG_FCI(
groupId, fragmentCollectionId, QueryUtil.ALL_POS,
QueryUtil.ALL_POS, null)) {
remove(fragmentEntry);
}
}
/**
* Returns the number of fragment entries where groupId = ? and fragmentCollectionId = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @return the number of matching fragment entries
*/
@Override
public int countByG_FCI(long groupId, long fragmentCollectionId) {
boolean productionMode = ctPersistenceHelper.isProductionMode(
FragmentEntry.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
Long count = null;
if (productionMode) {
finderPath = _finderPathCountByG_FCI;
finderArgs = new Object[] {groupId, fragmentCollectionId};
count = (Long)finderCache.getResult(finderPath, finderArgs);
}
if (count == null) {
StringBundler sb = new StringBundler(3);
sb.append(_SQL_COUNT_FRAGMENTENTRY_WHERE);
sb.append(_FINDER_COLUMN_G_FCI_GROUPID_2);
sb.append(_FINDER_COLUMN_G_FCI_FRAGMENTCOLLECTIONID_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
queryPos.add(fragmentCollectionId);
count = (Long)query.uniqueResult();
if (productionMode) {
finderCache.putResult(finderPath, finderArgs, count);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String _FINDER_COLUMN_G_FCI_GROUPID_2 =
"fragmentEntry.groupId = ? AND ";
private static final String _FINDER_COLUMN_G_FCI_FRAGMENTCOLLECTIONID_2 =
"fragmentEntry.fragmentCollectionId = ?";
private FinderPath _finderPathWithPaginationFindByG_FCI_Head;
private FinderPath _finderPathWithoutPaginationFindByG_FCI_Head;
private FinderPath _finderPathCountByG_FCI_Head;
/**
* Returns all the fragment entries where groupId = ? and fragmentCollectionId = ? and head = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param head the head
* @return the matching fragment entries
*/
@Override
public List findByG_FCI_Head(
long groupId, long fragmentCollectionId, boolean head) {
return findByG_FCI_Head(
groupId, fragmentCollectionId, head, QueryUtil.ALL_POS,
QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the fragment entries where groupId = ? and fragmentCollectionId = ? and head = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param head the head
* @param start the lower bound of the range of fragment entries
* @param end the upper bound of the range of fragment entries (not inclusive)
* @return the range of matching fragment entries
*/
@Override
public List findByG_FCI_Head(
long groupId, long fragmentCollectionId, boolean head, int start,
int end) {
return findByG_FCI_Head(
groupId, fragmentCollectionId, head, start, end, null);
}
/**
* Returns an ordered range of all the fragment entries where groupId = ? and fragmentCollectionId = ? and head = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param head the head
* @param start the lower bound of the range of fragment entries
* @param end the upper bound of the range of fragment entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entries
*/
@Override
public List findByG_FCI_Head(
long groupId, long fragmentCollectionId, boolean head, int start,
int end, OrderByComparator orderByComparator) {
return findByG_FCI_Head(
groupId, fragmentCollectionId, head, start, end, orderByComparator,
true);
}
/**
* Returns an ordered range of all the fragment entries where groupId = ? and fragmentCollectionId = ? and head = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param head the head
* @param start the lower bound of the range of fragment entries
* @param end the upper bound of the range of fragment entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching fragment entries
*/
@Override
public List findByG_FCI_Head(
long groupId, long fragmentCollectionId, boolean head, int start,
int end, OrderByComparator orderByComparator,
boolean useFinderCache) {
boolean productionMode = ctPersistenceHelper.isProductionMode(
FragmentEntry.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache && productionMode) {
finderPath = _finderPathWithoutPaginationFindByG_FCI_Head;
finderArgs = new Object[] {groupId, fragmentCollectionId, head};
}
}
else if (useFinderCache && productionMode) {
finderPath = _finderPathWithPaginationFindByG_FCI_Head;
finderArgs = new Object[] {
groupId, fragmentCollectionId, head, start, end,
orderByComparator
};
}
List list = null;
if (useFinderCache && productionMode) {
list = (List)finderCache.getResult(
finderPath, finderArgs);
if ((list != null) && !list.isEmpty()) {
for (FragmentEntry fragmentEntry : list) {
if ((groupId != fragmentEntry.getGroupId()) ||
(fragmentCollectionId !=
fragmentEntry.getFragmentCollectionId()) ||
(head != fragmentEntry.isHead())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
5 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(5);
}
sb.append(_SQL_SELECT_FRAGMENTENTRY_WHERE);
sb.append(_FINDER_COLUMN_G_FCI_HEAD_GROUPID_2);
sb.append(_FINDER_COLUMN_G_FCI_HEAD_FRAGMENTCOLLECTIONID_2);
sb.append(_FINDER_COLUMN_G_FCI_HEAD_HEAD_2);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(FragmentEntryModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
queryPos.add(fragmentCollectionId);
queryPos.add(head);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache && productionMode) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first fragment entry in the ordered set where groupId = ? and fragmentCollectionId = ? and head = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param head the head
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry
* @throws NoSuchEntryException if a matching fragment entry could not be found
*/
@Override
public FragmentEntry findByG_FCI_Head_First(
long groupId, long fragmentCollectionId, boolean head,
OrderByComparator orderByComparator)
throws NoSuchEntryException {
FragmentEntry fragmentEntry = fetchByG_FCI_Head_First(
groupId, fragmentCollectionId, head, orderByComparator);
if (fragmentEntry != null) {
return fragmentEntry;
}
StringBundler sb = new StringBundler(8);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("groupId=");
sb.append(groupId);
sb.append(", fragmentCollectionId=");
sb.append(fragmentCollectionId);
sb.append(", head=");
sb.append(head);
sb.append("}");
throw new NoSuchEntryException(sb.toString());
}
/**
* Returns the first fragment entry in the ordered set where groupId = ? and fragmentCollectionId = ? and head = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param head the head
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry, or null
if a matching fragment entry could not be found
*/
@Override
public FragmentEntry fetchByG_FCI_Head_First(
long groupId, long fragmentCollectionId, boolean head,
OrderByComparator orderByComparator) {
List list = findByG_FCI_Head(
groupId, fragmentCollectionId, head, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last fragment entry in the ordered set where groupId = ? and fragmentCollectionId = ? and head = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param head the head
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry
* @throws NoSuchEntryException if a matching fragment entry could not be found
*/
@Override
public FragmentEntry findByG_FCI_Head_Last(
long groupId, long fragmentCollectionId, boolean head,
OrderByComparator orderByComparator)
throws NoSuchEntryException {
FragmentEntry fragmentEntry = fetchByG_FCI_Head_Last(
groupId, fragmentCollectionId, head, orderByComparator);
if (fragmentEntry != null) {
return fragmentEntry;
}
StringBundler sb = new StringBundler(8);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("groupId=");
sb.append(groupId);
sb.append(", fragmentCollectionId=");
sb.append(fragmentCollectionId);
sb.append(", head=");
sb.append(head);
sb.append("}");
throw new NoSuchEntryException(sb.toString());
}
/**
* Returns the last fragment entry in the ordered set where groupId = ? and fragmentCollectionId = ? and head = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param head the head
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry, or null
if a matching fragment entry could not be found
*/
@Override
public FragmentEntry fetchByG_FCI_Head_Last(
long groupId, long fragmentCollectionId, boolean head,
OrderByComparator orderByComparator) {
int count = countByG_FCI_Head(groupId, fragmentCollectionId, head);
if (count == 0) {
return null;
}
List list = findByG_FCI_Head(
groupId, fragmentCollectionId, head, count - 1, count,
orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the fragment entries before and after the current fragment entry in the ordered set where groupId = ? and fragmentCollectionId = ? and head = ?.
*
* @param fragmentEntryId the primary key of the current fragment entry
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param head the head
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next fragment entry
* @throws NoSuchEntryException if a fragment entry with the primary key could not be found
*/
@Override
public FragmentEntry[] findByG_FCI_Head_PrevAndNext(
long fragmentEntryId, long groupId, long fragmentCollectionId,
boolean head, OrderByComparator orderByComparator)
throws NoSuchEntryException {
FragmentEntry fragmentEntry = findByPrimaryKey(fragmentEntryId);
Session session = null;
try {
session = openSession();
FragmentEntry[] array = new FragmentEntryImpl[3];
array[0] = getByG_FCI_Head_PrevAndNext(
session, fragmentEntry, groupId, fragmentCollectionId, head,
orderByComparator, true);
array[1] = fragmentEntry;
array[2] = getByG_FCI_Head_PrevAndNext(
session, fragmentEntry, groupId, fragmentCollectionId, head,
orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected FragmentEntry getByG_FCI_Head_PrevAndNext(
Session session, FragmentEntry fragmentEntry, long groupId,
long fragmentCollectionId, boolean head,
OrderByComparator orderByComparator, boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
6 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(5);
}
sb.append(_SQL_SELECT_FRAGMENTENTRY_WHERE);
sb.append(_FINDER_COLUMN_G_FCI_HEAD_GROUPID_2);
sb.append(_FINDER_COLUMN_G_FCI_HEAD_FRAGMENTCOLLECTIONID_2);
sb.append(_FINDER_COLUMN_G_FCI_HEAD_HEAD_2);
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(FragmentEntryModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
queryPos.add(fragmentCollectionId);
queryPos.add(head);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
fragmentEntry)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the fragment entries where groupId = ? and fragmentCollectionId = ? and head = ? from the database.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param head the head
*/
@Override
public void removeByG_FCI_Head(
long groupId, long fragmentCollectionId, boolean head) {
for (FragmentEntry fragmentEntry :
findByG_FCI_Head(
groupId, fragmentCollectionId, head, QueryUtil.ALL_POS,
QueryUtil.ALL_POS, null)) {
remove(fragmentEntry);
}
}
/**
* Returns the number of fragment entries where groupId = ? and fragmentCollectionId = ? and head = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param head the head
* @return the number of matching fragment entries
*/
@Override
public int countByG_FCI_Head(
long groupId, long fragmentCollectionId, boolean head) {
boolean productionMode = ctPersistenceHelper.isProductionMode(
FragmentEntry.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
Long count = null;
if (productionMode) {
finderPath = _finderPathCountByG_FCI_Head;
finderArgs = new Object[] {groupId, fragmentCollectionId, head};
count = (Long)finderCache.getResult(finderPath, finderArgs);
}
if (count == null) {
StringBundler sb = new StringBundler(4);
sb.append(_SQL_COUNT_FRAGMENTENTRY_WHERE);
sb.append(_FINDER_COLUMN_G_FCI_HEAD_GROUPID_2);
sb.append(_FINDER_COLUMN_G_FCI_HEAD_FRAGMENTCOLLECTIONID_2);
sb.append(_FINDER_COLUMN_G_FCI_HEAD_HEAD_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
queryPos.add(fragmentCollectionId);
queryPos.add(head);
count = (Long)query.uniqueResult();
if (productionMode) {
finderCache.putResult(finderPath, finderArgs, count);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String _FINDER_COLUMN_G_FCI_HEAD_GROUPID_2 =
"fragmentEntry.groupId = ? AND ";
private static final String
_FINDER_COLUMN_G_FCI_HEAD_FRAGMENTCOLLECTIONID_2 =
"fragmentEntry.fragmentCollectionId = ? AND ";
private static final String _FINDER_COLUMN_G_FCI_HEAD_HEAD_2 =
"fragmentEntry.head = ?";
private FinderPath _finderPathWithPaginationFindByG_FEK;
private FinderPath _finderPathWithoutPaginationFindByG_FEK;
private FinderPath _finderPathCountByG_FEK;
/**
* Returns all the fragment entries where groupId = ? and fragmentEntryKey = ?.
*
* @param groupId the group ID
* @param fragmentEntryKey the fragment entry key
* @return the matching fragment entries
*/
@Override
public List findByG_FEK(
long groupId, String fragmentEntryKey) {
return findByG_FEK(
groupId, fragmentEntryKey, QueryUtil.ALL_POS, QueryUtil.ALL_POS,
null);
}
/**
* Returns a range of all the fragment entries where groupId = ? and fragmentEntryKey = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentEntryKey the fragment entry key
* @param start the lower bound of the range of fragment entries
* @param end the upper bound of the range of fragment entries (not inclusive)
* @return the range of matching fragment entries
*/
@Override
public List findByG_FEK(
long groupId, String fragmentEntryKey, int start, int end) {
return findByG_FEK(groupId, fragmentEntryKey, start, end, null);
}
/**
* Returns an ordered range of all the fragment entries where groupId = ? and fragmentEntryKey = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentEntryKey the fragment entry key
* @param start the lower bound of the range of fragment entries
* @param end the upper bound of the range of fragment entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entries
*/
@Override
public List findByG_FEK(
long groupId, String fragmentEntryKey, int start, int end,
OrderByComparator orderByComparator) {
return findByG_FEK(
groupId, fragmentEntryKey, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the fragment entries where groupId = ? and fragmentEntryKey = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentEntryKey the fragment entry key
* @param start the lower bound of the range of fragment entries
* @param end the upper bound of the range of fragment entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching fragment entries
*/
@Override
public List findByG_FEK(
long groupId, String fragmentEntryKey, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
fragmentEntryKey = Objects.toString(fragmentEntryKey, "");
boolean productionMode = ctPersistenceHelper.isProductionMode(
FragmentEntry.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache && productionMode) {
finderPath = _finderPathWithoutPaginationFindByG_FEK;
finderArgs = new Object[] {groupId, fragmentEntryKey};
}
}
else if (useFinderCache && productionMode) {
finderPath = _finderPathWithPaginationFindByG_FEK;
finderArgs = new Object[] {
groupId, fragmentEntryKey, start, end, orderByComparator
};
}
List list = null;
if (useFinderCache && productionMode) {
list = (List)finderCache.getResult(
finderPath, finderArgs);
if ((list != null) && !list.isEmpty()) {
for (FragmentEntry fragmentEntry : list) {
if ((groupId != fragmentEntry.getGroupId()) ||
!fragmentEntryKey.equals(
fragmentEntry.getFragmentEntryKey())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_FRAGMENTENTRY_WHERE);
sb.append(_FINDER_COLUMN_G_FEK_GROUPID_2);
boolean bindFragmentEntryKey = false;
if (fragmentEntryKey.isEmpty()) {
sb.append(_FINDER_COLUMN_G_FEK_FRAGMENTENTRYKEY_3);
}
else {
bindFragmentEntryKey = true;
sb.append(_FINDER_COLUMN_G_FEK_FRAGMENTENTRYKEY_2);
}
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(FragmentEntryModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
if (bindFragmentEntryKey) {
queryPos.add(fragmentEntryKey);
}
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache && productionMode) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first fragment entry in the ordered set where groupId = ? and fragmentEntryKey = ?.
*
* @param groupId the group ID
* @param fragmentEntryKey the fragment entry key
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry
* @throws NoSuchEntryException if a matching fragment entry could not be found
*/
@Override
public FragmentEntry findByG_FEK_First(
long groupId, String fragmentEntryKey,
OrderByComparator orderByComparator)
throws NoSuchEntryException {
FragmentEntry fragmentEntry = fetchByG_FEK_First(
groupId, fragmentEntryKey, orderByComparator);
if (fragmentEntry != null) {
return fragmentEntry;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("groupId=");
sb.append(groupId);
sb.append(", fragmentEntryKey=");
sb.append(fragmentEntryKey);
sb.append("}");
throw new NoSuchEntryException(sb.toString());
}
/**
* Returns the first fragment entry in the ordered set where groupId = ? and fragmentEntryKey = ?.
*
* @param groupId the group ID
* @param fragmentEntryKey the fragment entry key
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry, or null
if a matching fragment entry could not be found
*/
@Override
public FragmentEntry fetchByG_FEK_First(
long groupId, String fragmentEntryKey,
OrderByComparator orderByComparator) {
List list = findByG_FEK(
groupId, fragmentEntryKey, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last fragment entry in the ordered set where groupId = ? and fragmentEntryKey = ?.
*
* @param groupId the group ID
* @param fragmentEntryKey the fragment entry key
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry
* @throws NoSuchEntryException if a matching fragment entry could not be found
*/
@Override
public FragmentEntry findByG_FEK_Last(
long groupId, String fragmentEntryKey,
OrderByComparator orderByComparator)
throws NoSuchEntryException {
FragmentEntry fragmentEntry = fetchByG_FEK_Last(
groupId, fragmentEntryKey, orderByComparator);
if (fragmentEntry != null) {
return fragmentEntry;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("groupId=");
sb.append(groupId);
sb.append(", fragmentEntryKey=");
sb.append(fragmentEntryKey);
sb.append("}");
throw new NoSuchEntryException(sb.toString());
}
/**
* Returns the last fragment entry in the ordered set where groupId = ? and fragmentEntryKey = ?.
*
* @param groupId the group ID
* @param fragmentEntryKey the fragment entry key
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry, or null
if a matching fragment entry could not be found
*/
@Override
public FragmentEntry fetchByG_FEK_Last(
long groupId, String fragmentEntryKey,
OrderByComparator orderByComparator) {
int count = countByG_FEK(groupId, fragmentEntryKey);
if (count == 0) {
return null;
}
List list = findByG_FEK(
groupId, fragmentEntryKey, count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the fragment entries before and after the current fragment entry in the ordered set where groupId = ? and fragmentEntryKey = ?.
*
* @param fragmentEntryId the primary key of the current fragment entry
* @param groupId the group ID
* @param fragmentEntryKey the fragment entry key
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next fragment entry
* @throws NoSuchEntryException if a fragment entry with the primary key could not be found
*/
@Override
public FragmentEntry[] findByG_FEK_PrevAndNext(
long fragmentEntryId, long groupId, String fragmentEntryKey,
OrderByComparator orderByComparator)
throws NoSuchEntryException {
fragmentEntryKey = Objects.toString(fragmentEntryKey, "");
FragmentEntry fragmentEntry = findByPrimaryKey(fragmentEntryId);
Session session = null;
try {
session = openSession();
FragmentEntry[] array = new FragmentEntryImpl[3];
array[0] = getByG_FEK_PrevAndNext(
session, fragmentEntry, groupId, fragmentEntryKey,
orderByComparator, true);
array[1] = fragmentEntry;
array[2] = getByG_FEK_PrevAndNext(
session, fragmentEntry, groupId, fragmentEntryKey,
orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected FragmentEntry getByG_FEK_PrevAndNext(
Session session, FragmentEntry fragmentEntry, long groupId,
String fragmentEntryKey,
OrderByComparator orderByComparator, boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
5 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_FRAGMENTENTRY_WHERE);
sb.append(_FINDER_COLUMN_G_FEK_GROUPID_2);
boolean bindFragmentEntryKey = false;
if (fragmentEntryKey.isEmpty()) {
sb.append(_FINDER_COLUMN_G_FEK_FRAGMENTENTRYKEY_3);
}
else {
bindFragmentEntryKey = true;
sb.append(_FINDER_COLUMN_G_FEK_FRAGMENTENTRYKEY_2);
}
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(FragmentEntryModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
if (bindFragmentEntryKey) {
queryPos.add(fragmentEntryKey);
}
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
fragmentEntry)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the fragment entries where groupId = ? and fragmentEntryKey = ? from the database.
*
* @param groupId the group ID
* @param fragmentEntryKey the fragment entry key
*/
@Override
public void removeByG_FEK(long groupId, String fragmentEntryKey) {
for (FragmentEntry fragmentEntry :
findByG_FEK(
groupId, fragmentEntryKey, QueryUtil.ALL_POS,
QueryUtil.ALL_POS, null)) {
remove(fragmentEntry);
}
}
/**
* Returns the number of fragment entries where groupId = ? and fragmentEntryKey = ?.
*
* @param groupId the group ID
* @param fragmentEntryKey the fragment entry key
* @return the number of matching fragment entries
*/
@Override
public int countByG_FEK(long groupId, String fragmentEntryKey) {
fragmentEntryKey = Objects.toString(fragmentEntryKey, "");
boolean productionMode = ctPersistenceHelper.isProductionMode(
FragmentEntry.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
Long count = null;
if (productionMode) {
finderPath = _finderPathCountByG_FEK;
finderArgs = new Object[] {groupId, fragmentEntryKey};
count = (Long)finderCache.getResult(finderPath, finderArgs);
}
if (count == null) {
StringBundler sb = new StringBundler(3);
sb.append(_SQL_COUNT_FRAGMENTENTRY_WHERE);
sb.append(_FINDER_COLUMN_G_FEK_GROUPID_2);
boolean bindFragmentEntryKey = false;
if (fragmentEntryKey.isEmpty()) {
sb.append(_FINDER_COLUMN_G_FEK_FRAGMENTENTRYKEY_3);
}
else {
bindFragmentEntryKey = true;
sb.append(_FINDER_COLUMN_G_FEK_FRAGMENTENTRYKEY_2);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
if (bindFragmentEntryKey) {
queryPos.add(fragmentEntryKey);
}
count = (Long)query.uniqueResult();
if (productionMode) {
finderCache.putResult(finderPath, finderArgs, count);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String _FINDER_COLUMN_G_FEK_GROUPID_2 =
"fragmentEntry.groupId = ? AND ";
private static final String _FINDER_COLUMN_G_FEK_FRAGMENTENTRYKEY_2 =
"fragmentEntry.fragmentEntryKey = ?";
private static final String _FINDER_COLUMN_G_FEK_FRAGMENTENTRYKEY_3 =
"(fragmentEntry.fragmentEntryKey IS NULL OR fragmentEntry.fragmentEntryKey = '')";
private FinderPath _finderPathFetchByG_FEK_Head;
private FinderPath _finderPathCountByG_FEK_Head;
/**
* Returns the fragment entry where groupId = ? and fragmentEntryKey = ? and head = ? or throws a NoSuchEntryException
if it could not be found.
*
* @param groupId the group ID
* @param fragmentEntryKey the fragment entry key
* @param head the head
* @return the matching fragment entry
* @throws NoSuchEntryException if a matching fragment entry could not be found
*/
@Override
public FragmentEntry findByG_FEK_Head(
long groupId, String fragmentEntryKey, boolean head)
throws NoSuchEntryException {
FragmentEntry fragmentEntry = fetchByG_FEK_Head(
groupId, fragmentEntryKey, head);
if (fragmentEntry == null) {
StringBundler sb = new StringBundler(8);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("groupId=");
sb.append(groupId);
sb.append(", fragmentEntryKey=");
sb.append(fragmentEntryKey);
sb.append(", head=");
sb.append(head);
sb.append("}");
if (_log.isDebugEnabled()) {
_log.debug(sb.toString());
}
throw new NoSuchEntryException(sb.toString());
}
return fragmentEntry;
}
/**
* Returns the fragment entry where groupId = ? and fragmentEntryKey = ? and head = ? or returns null
if it could not be found. Uses the finder cache.
*
* @param groupId the group ID
* @param fragmentEntryKey the fragment entry key
* @param head the head
* @return the matching fragment entry, or null
if a matching fragment entry could not be found
*/
@Override
public FragmentEntry fetchByG_FEK_Head(
long groupId, String fragmentEntryKey, boolean head) {
return fetchByG_FEK_Head(groupId, fragmentEntryKey, head, true);
}
/**
* Returns the fragment entry where groupId = ? and fragmentEntryKey = ? and head = ? or returns null
if it could not be found, optionally using the finder cache.
*
* @param groupId the group ID
* @param fragmentEntryKey the fragment entry key
* @param head the head
* @param useFinderCache whether to use the finder cache
* @return the matching fragment entry, or null
if a matching fragment entry could not be found
*/
@Override
public FragmentEntry fetchByG_FEK_Head(
long groupId, String fragmentEntryKey, boolean head,
boolean useFinderCache) {
fragmentEntryKey = Objects.toString(fragmentEntryKey, "");
boolean productionMode = ctPersistenceHelper.isProductionMode(
FragmentEntry.class);
Object[] finderArgs = null;
if (useFinderCache && productionMode) {
finderArgs = new Object[] {groupId, fragmentEntryKey, head};
}
Object result = null;
if (useFinderCache && productionMode) {
result = finderCache.getResult(
_finderPathFetchByG_FEK_Head, finderArgs);
}
if (result instanceof FragmentEntry) {
FragmentEntry fragmentEntry = (FragmentEntry)result;
if ((groupId != fragmentEntry.getGroupId()) ||
!Objects.equals(
fragmentEntryKey, fragmentEntry.getFragmentEntryKey()) ||
(head != fragmentEntry.isHead())) {
result = null;
}
}
if (result == null) {
StringBundler sb = new StringBundler(5);
sb.append(_SQL_SELECT_FRAGMENTENTRY_WHERE);
sb.append(_FINDER_COLUMN_G_FEK_HEAD_GROUPID_2);
boolean bindFragmentEntryKey = false;
if (fragmentEntryKey.isEmpty()) {
sb.append(_FINDER_COLUMN_G_FEK_HEAD_FRAGMENTENTRYKEY_3);
}
else {
bindFragmentEntryKey = true;
sb.append(_FINDER_COLUMN_G_FEK_HEAD_FRAGMENTENTRYKEY_2);
}
sb.append(_FINDER_COLUMN_G_FEK_HEAD_HEAD_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
if (bindFragmentEntryKey) {
queryPos.add(fragmentEntryKey);
}
queryPos.add(head);
List list = query.list();
if (list.isEmpty()) {
if (useFinderCache && productionMode) {
finderCache.putResult(
_finderPathFetchByG_FEK_Head, finderArgs, list);
}
}
else {
FragmentEntry fragmentEntry = list.get(0);
result = fragmentEntry;
cacheResult(fragmentEntry);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
if (result instanceof List>) {
return null;
}
else {
return (FragmentEntry)result;
}
}
/**
* Removes the fragment entry where groupId = ? and fragmentEntryKey = ? and head = ? from the database.
*
* @param groupId the group ID
* @param fragmentEntryKey the fragment entry key
* @param head the head
* @return the fragment entry that was removed
*/
@Override
public FragmentEntry removeByG_FEK_Head(
long groupId, String fragmentEntryKey, boolean head)
throws NoSuchEntryException {
FragmentEntry fragmentEntry = findByG_FEK_Head(
groupId, fragmentEntryKey, head);
return remove(fragmentEntry);
}
/**
* Returns the number of fragment entries where groupId = ? and fragmentEntryKey = ? and head = ?.
*
* @param groupId the group ID
* @param fragmentEntryKey the fragment entry key
* @param head the head
* @return the number of matching fragment entries
*/
@Override
public int countByG_FEK_Head(
long groupId, String fragmentEntryKey, boolean head) {
fragmentEntryKey = Objects.toString(fragmentEntryKey, "");
boolean productionMode = ctPersistenceHelper.isProductionMode(
FragmentEntry.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
Long count = null;
if (productionMode) {
finderPath = _finderPathCountByG_FEK_Head;
finderArgs = new Object[] {groupId, fragmentEntryKey, head};
count = (Long)finderCache.getResult(finderPath, finderArgs);
}
if (count == null) {
StringBundler sb = new StringBundler(4);
sb.append(_SQL_COUNT_FRAGMENTENTRY_WHERE);
sb.append(_FINDER_COLUMN_G_FEK_HEAD_GROUPID_2);
boolean bindFragmentEntryKey = false;
if (fragmentEntryKey.isEmpty()) {
sb.append(_FINDER_COLUMN_G_FEK_HEAD_FRAGMENTENTRYKEY_3);
}
else {
bindFragmentEntryKey = true;
sb.append(_FINDER_COLUMN_G_FEK_HEAD_FRAGMENTENTRYKEY_2);
}
sb.append(_FINDER_COLUMN_G_FEK_HEAD_HEAD_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
if (bindFragmentEntryKey) {
queryPos.add(fragmentEntryKey);
}
queryPos.add(head);
count = (Long)query.uniqueResult();
if (productionMode) {
finderCache.putResult(finderPath, finderArgs, count);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String _FINDER_COLUMN_G_FEK_HEAD_GROUPID_2 =
"fragmentEntry.groupId = ? AND ";
private static final String _FINDER_COLUMN_G_FEK_HEAD_FRAGMENTENTRYKEY_2 =
"fragmentEntry.fragmentEntryKey = ? AND ";
private static final String _FINDER_COLUMN_G_FEK_HEAD_FRAGMENTENTRYKEY_3 =
"(fragmentEntry.fragmentEntryKey IS NULL OR fragmentEntry.fragmentEntryKey = '') AND ";
private static final String _FINDER_COLUMN_G_FEK_HEAD_HEAD_2 =
"fragmentEntry.head = ?";
private FinderPath _finderPathWithPaginationFindByG_FCI_LikeN;
private FinderPath _finderPathWithPaginationCountByG_FCI_LikeN;
/**
* Returns all the fragment entries where groupId = ? and fragmentCollectionId = ? and name LIKE ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @return the matching fragment entries
*/
@Override
public List findByG_FCI_LikeN(
long groupId, long fragmentCollectionId, String name) {
return findByG_FCI_LikeN(
groupId, fragmentCollectionId, name, QueryUtil.ALL_POS,
QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the fragment entries where groupId = ? and fragmentCollectionId = ? and name LIKE ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @param start the lower bound of the range of fragment entries
* @param end the upper bound of the range of fragment entries (not inclusive)
* @return the range of matching fragment entries
*/
@Override
public List findByG_FCI_LikeN(
long groupId, long fragmentCollectionId, String name, int start,
int end) {
return findByG_FCI_LikeN(
groupId, fragmentCollectionId, name, start, end, null);
}
/**
* Returns an ordered range of all the fragment entries where groupId = ? and fragmentCollectionId = ? and name LIKE ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @param start the lower bound of the range of fragment entries
* @param end the upper bound of the range of fragment entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entries
*/
@Override
public List findByG_FCI_LikeN(
long groupId, long fragmentCollectionId, String name, int start,
int end, OrderByComparator orderByComparator) {
return findByG_FCI_LikeN(
groupId, fragmentCollectionId, name, start, end, orderByComparator,
true);
}
/**
* Returns an ordered range of all the fragment entries where groupId = ? and fragmentCollectionId = ? and name LIKE ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @param start the lower bound of the range of fragment entries
* @param end the upper bound of the range of fragment entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching fragment entries
*/
@Override
public List findByG_FCI_LikeN(
long groupId, long fragmentCollectionId, String name, int start,
int end, OrderByComparator orderByComparator,
boolean useFinderCache) {
name = Objects.toString(name, "");
boolean productionMode = ctPersistenceHelper.isProductionMode(
FragmentEntry.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
finderPath = _finderPathWithPaginationFindByG_FCI_LikeN;
finderArgs = new Object[] {
groupId, fragmentCollectionId, name, start, end, orderByComparator
};
List list = null;
if (useFinderCache && productionMode) {
list = (List)finderCache.getResult(
finderPath, finderArgs);
if ((list != null) && !list.isEmpty()) {
for (FragmentEntry fragmentEntry : list) {
if ((groupId != fragmentEntry.getGroupId()) ||
(fragmentCollectionId !=
fragmentEntry.getFragmentCollectionId()) ||
!StringUtil.wildcardMatches(
fragmentEntry.getName(), name, '_', '%', '\\',
true)) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
5 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(5);
}
sb.append(_SQL_SELECT_FRAGMENTENTRY_WHERE);
sb.append(_FINDER_COLUMN_G_FCI_LIKEN_GROUPID_2);
sb.append(_FINDER_COLUMN_G_FCI_LIKEN_FRAGMENTCOLLECTIONID_2);
boolean bindName = false;
if (name.isEmpty()) {
sb.append(_FINDER_COLUMN_G_FCI_LIKEN_NAME_3);
}
else {
bindName = true;
sb.append(_FINDER_COLUMN_G_FCI_LIKEN_NAME_2);
}
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(FragmentEntryModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
queryPos.add(fragmentCollectionId);
if (bindName) {
queryPos.add(name);
}
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache && productionMode) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first fragment entry in the ordered set where groupId = ? and fragmentCollectionId = ? and name LIKE ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry
* @throws NoSuchEntryException if a matching fragment entry could not be found
*/
@Override
public FragmentEntry findByG_FCI_LikeN_First(
long groupId, long fragmentCollectionId, String name,
OrderByComparator orderByComparator)
throws NoSuchEntryException {
FragmentEntry fragmentEntry = fetchByG_FCI_LikeN_First(
groupId, fragmentCollectionId, name, orderByComparator);
if (fragmentEntry != null) {
return fragmentEntry;
}
StringBundler sb = new StringBundler(8);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("groupId=");
sb.append(groupId);
sb.append(", fragmentCollectionId=");
sb.append(fragmentCollectionId);
sb.append(", nameLIKE");
sb.append(name);
sb.append("}");
throw new NoSuchEntryException(sb.toString());
}
/**
* Returns the first fragment entry in the ordered set where groupId = ? and fragmentCollectionId = ? and name LIKE ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry, or null
if a matching fragment entry could not be found
*/
@Override
public FragmentEntry fetchByG_FCI_LikeN_First(
long groupId, long fragmentCollectionId, String name,
OrderByComparator orderByComparator) {
List list = findByG_FCI_LikeN(
groupId, fragmentCollectionId, name, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last fragment entry in the ordered set where groupId = ? and fragmentCollectionId = ? and name LIKE ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry
* @throws NoSuchEntryException if a matching fragment entry could not be found
*/
@Override
public FragmentEntry findByG_FCI_LikeN_Last(
long groupId, long fragmentCollectionId, String name,
OrderByComparator orderByComparator)
throws NoSuchEntryException {
FragmentEntry fragmentEntry = fetchByG_FCI_LikeN_Last(
groupId, fragmentCollectionId, name, orderByComparator);
if (fragmentEntry != null) {
return fragmentEntry;
}
StringBundler sb = new StringBundler(8);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("groupId=");
sb.append(groupId);
sb.append(", fragmentCollectionId=");
sb.append(fragmentCollectionId);
sb.append(", nameLIKE");
sb.append(name);
sb.append("}");
throw new NoSuchEntryException(sb.toString());
}
/**
* Returns the last fragment entry in the ordered set where groupId = ? and fragmentCollectionId = ? and name LIKE ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry, or null
if a matching fragment entry could not be found
*/
@Override
public FragmentEntry fetchByG_FCI_LikeN_Last(
long groupId, long fragmentCollectionId, String name,
OrderByComparator orderByComparator) {
int count = countByG_FCI_LikeN(groupId, fragmentCollectionId, name);
if (count == 0) {
return null;
}
List list = findByG_FCI_LikeN(
groupId, fragmentCollectionId, name, count - 1, count,
orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the fragment entries before and after the current fragment entry in the ordered set where groupId = ? and fragmentCollectionId = ? and name LIKE ?.
*
* @param fragmentEntryId the primary key of the current fragment entry
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next fragment entry
* @throws NoSuchEntryException if a fragment entry with the primary key could not be found
*/
@Override
public FragmentEntry[] findByG_FCI_LikeN_PrevAndNext(
long fragmentEntryId, long groupId, long fragmentCollectionId,
String name, OrderByComparator orderByComparator)
throws NoSuchEntryException {
name = Objects.toString(name, "");
FragmentEntry fragmentEntry = findByPrimaryKey(fragmentEntryId);
Session session = null;
try {
session = openSession();
FragmentEntry[] array = new FragmentEntryImpl[3];
array[0] = getByG_FCI_LikeN_PrevAndNext(
session, fragmentEntry, groupId, fragmentCollectionId, name,
orderByComparator, true);
array[1] = fragmentEntry;
array[2] = getByG_FCI_LikeN_PrevAndNext(
session, fragmentEntry, groupId, fragmentCollectionId, name,
orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected FragmentEntry getByG_FCI_LikeN_PrevAndNext(
Session session, FragmentEntry fragmentEntry, long groupId,
long fragmentCollectionId, String name,
OrderByComparator orderByComparator, boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
6 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(5);
}
sb.append(_SQL_SELECT_FRAGMENTENTRY_WHERE);
sb.append(_FINDER_COLUMN_G_FCI_LIKEN_GROUPID_2);
sb.append(_FINDER_COLUMN_G_FCI_LIKEN_FRAGMENTCOLLECTIONID_2);
boolean bindName = false;
if (name.isEmpty()) {
sb.append(_FINDER_COLUMN_G_FCI_LIKEN_NAME_3);
}
else {
bindName = true;
sb.append(_FINDER_COLUMN_G_FCI_LIKEN_NAME_2);
}
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(FragmentEntryModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
queryPos.add(fragmentCollectionId);
if (bindName) {
queryPos.add(name);
}
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
fragmentEntry)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the fragment entries where groupId = ? and fragmentCollectionId = ? and name LIKE ? from the database.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
*/
@Override
public void removeByG_FCI_LikeN(
long groupId, long fragmentCollectionId, String name) {
for (FragmentEntry fragmentEntry :
findByG_FCI_LikeN(
groupId, fragmentCollectionId, name, QueryUtil.ALL_POS,
QueryUtil.ALL_POS, null)) {
remove(fragmentEntry);
}
}
/**
* Returns the number of fragment entries where groupId = ? and fragmentCollectionId = ? and name LIKE ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @return the number of matching fragment entries
*/
@Override
public int countByG_FCI_LikeN(
long groupId, long fragmentCollectionId, String name) {
name = Objects.toString(name, "");
boolean productionMode = ctPersistenceHelper.isProductionMode(
FragmentEntry.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
Long count = null;
if (productionMode) {
finderPath = _finderPathWithPaginationCountByG_FCI_LikeN;
finderArgs = new Object[] {groupId, fragmentCollectionId, name};
count = (Long)finderCache.getResult(finderPath, finderArgs);
}
if (count == null) {
StringBundler sb = new StringBundler(4);
sb.append(_SQL_COUNT_FRAGMENTENTRY_WHERE);
sb.append(_FINDER_COLUMN_G_FCI_LIKEN_GROUPID_2);
sb.append(_FINDER_COLUMN_G_FCI_LIKEN_FRAGMENTCOLLECTIONID_2);
boolean bindName = false;
if (name.isEmpty()) {
sb.append(_FINDER_COLUMN_G_FCI_LIKEN_NAME_3);
}
else {
bindName = true;
sb.append(_FINDER_COLUMN_G_FCI_LIKEN_NAME_2);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
queryPos.add(fragmentCollectionId);
if (bindName) {
queryPos.add(name);
}
count = (Long)query.uniqueResult();
if (productionMode) {
finderCache.putResult(finderPath, finderArgs, count);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String _FINDER_COLUMN_G_FCI_LIKEN_GROUPID_2 =
"fragmentEntry.groupId = ? AND ";
private static final String
_FINDER_COLUMN_G_FCI_LIKEN_FRAGMENTCOLLECTIONID_2 =
"fragmentEntry.fragmentCollectionId = ? AND ";
private static final String _FINDER_COLUMN_G_FCI_LIKEN_NAME_2 =
"fragmentEntry.name LIKE ?";
private static final String _FINDER_COLUMN_G_FCI_LIKEN_NAME_3 =
"(fragmentEntry.name IS NULL OR fragmentEntry.name LIKE '')";
private FinderPath _finderPathWithPaginationFindByG_FCI_LikeN_Head;
private FinderPath _finderPathWithPaginationCountByG_FCI_LikeN_Head;
/**
* Returns all the fragment entries where groupId = ? and fragmentCollectionId = ? and name LIKE ? and head = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @param head the head
* @return the matching fragment entries
*/
@Override
public List findByG_FCI_LikeN_Head(
long groupId, long fragmentCollectionId, String name, boolean head) {
return findByG_FCI_LikeN_Head(
groupId, fragmentCollectionId, name, head, QueryUtil.ALL_POS,
QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the fragment entries where groupId = ? and fragmentCollectionId = ? and name LIKE ? and head = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @param head the head
* @param start the lower bound of the range of fragment entries
* @param end the upper bound of the range of fragment entries (not inclusive)
* @return the range of matching fragment entries
*/
@Override
public List findByG_FCI_LikeN_Head(
long groupId, long fragmentCollectionId, String name, boolean head,
int start, int end) {
return findByG_FCI_LikeN_Head(
groupId, fragmentCollectionId, name, head, start, end, null);
}
/**
* Returns an ordered range of all the fragment entries where groupId = ? and fragmentCollectionId = ? and name LIKE ? and head = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @param head the head
* @param start the lower bound of the range of fragment entries
* @param end the upper bound of the range of fragment entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching fragment entries
*/
@Override
public List findByG_FCI_LikeN_Head(
long groupId, long fragmentCollectionId, String name, boolean head,
int start, int end,
OrderByComparator orderByComparator) {
return findByG_FCI_LikeN_Head(
groupId, fragmentCollectionId, name, head, start, end,
orderByComparator, true);
}
/**
* Returns an ordered range of all the fragment entries where groupId = ? and fragmentCollectionId = ? and name LIKE ? and head = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from FragmentEntryModelImpl
.
*
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @param head the head
* @param start the lower bound of the range of fragment entries
* @param end the upper bound of the range of fragment entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching fragment entries
*/
@Override
public List findByG_FCI_LikeN_Head(
long groupId, long fragmentCollectionId, String name, boolean head,
int start, int end, OrderByComparator orderByComparator,
boolean useFinderCache) {
name = Objects.toString(name, "");
boolean productionMode = ctPersistenceHelper.isProductionMode(
FragmentEntry.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
finderPath = _finderPathWithPaginationFindByG_FCI_LikeN_Head;
finderArgs = new Object[] {
groupId, fragmentCollectionId, name, head, start, end,
orderByComparator
};
List list = null;
if (useFinderCache && productionMode) {
list = (List)finderCache.getResult(
finderPath, finderArgs);
if ((list != null) && !list.isEmpty()) {
for (FragmentEntry fragmentEntry : list) {
if ((groupId != fragmentEntry.getGroupId()) ||
(fragmentCollectionId !=
fragmentEntry.getFragmentCollectionId()) ||
!StringUtil.wildcardMatches(
fragmentEntry.getName(), name, '_', '%', '\\',
true) ||
(head != fragmentEntry.isHead())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
6 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(6);
}
sb.append(_SQL_SELECT_FRAGMENTENTRY_WHERE);
sb.append(_FINDER_COLUMN_G_FCI_LIKEN_HEAD_GROUPID_2);
sb.append(_FINDER_COLUMN_G_FCI_LIKEN_HEAD_FRAGMENTCOLLECTIONID_2);
boolean bindName = false;
if (name.isEmpty()) {
sb.append(_FINDER_COLUMN_G_FCI_LIKEN_HEAD_NAME_3);
}
else {
bindName = true;
sb.append(_FINDER_COLUMN_G_FCI_LIKEN_HEAD_NAME_2);
}
sb.append(_FINDER_COLUMN_G_FCI_LIKEN_HEAD_HEAD_2);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(FragmentEntryModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
queryPos.add(fragmentCollectionId);
if (bindName) {
queryPos.add(name);
}
queryPos.add(head);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache && productionMode) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first fragment entry in the ordered set where groupId = ? and fragmentCollectionId = ? and name LIKE ? and head = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @param head the head
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry
* @throws NoSuchEntryException if a matching fragment entry could not be found
*/
@Override
public FragmentEntry findByG_FCI_LikeN_Head_First(
long groupId, long fragmentCollectionId, String name, boolean head,
OrderByComparator orderByComparator)
throws NoSuchEntryException {
FragmentEntry fragmentEntry = fetchByG_FCI_LikeN_Head_First(
groupId, fragmentCollectionId, name, head, orderByComparator);
if (fragmentEntry != null) {
return fragmentEntry;
}
StringBundler sb = new StringBundler(10);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("groupId=");
sb.append(groupId);
sb.append(", fragmentCollectionId=");
sb.append(fragmentCollectionId);
sb.append(", nameLIKE");
sb.append(name);
sb.append(", head=");
sb.append(head);
sb.append("}");
throw new NoSuchEntryException(sb.toString());
}
/**
* Returns the first fragment entry in the ordered set where groupId = ? and fragmentCollectionId = ? and name LIKE ? and head = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @param head the head
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching fragment entry, or null
if a matching fragment entry could not be found
*/
@Override
public FragmentEntry fetchByG_FCI_LikeN_Head_First(
long groupId, long fragmentCollectionId, String name, boolean head,
OrderByComparator orderByComparator) {
List list = findByG_FCI_LikeN_Head(
groupId, fragmentCollectionId, name, head, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last fragment entry in the ordered set where groupId = ? and fragmentCollectionId = ? and name LIKE ? and head = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @param head the head
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching fragment entry
* @throws NoSuchEntryException if a matching fragment entry could not be found
*/
@Override
public FragmentEntry findByG_FCI_LikeN_Head_Last(
long groupId, long fragmentCollectionId, String name, boolean head,
OrderByComparator orderByComparator)
throws NoSuchEntryException {
FragmentEntry fragmentEntry = fetchByG_FCI_LikeN_Head_Last(
groupId, fragmentCollectionId, name, head, orderByComparator);
if (fragmentEntry != null) {
return fragmentEntry;
}
StringBundler sb = new StringBundler(10);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("groupId=");
sb.append(groupId);
sb.append(", fragmentCollectionId=");
sb.append(fragmentCollectionId);
sb.append(", nameLIKE");
sb.append(name);
sb.append(", head=");
sb.append(head);
sb.append("}");
throw new NoSuchEntryException(sb.toString());
}
/**
* Returns the last fragment entry in the ordered set where groupId = ? and fragmentCollectionId = ? and name LIKE ? and head = ?.
*
* @param groupId the group ID
* @param fragmentCollectionId the fragment collection ID
* @param name the name
* @param head the head
* @param orderByComparator the comparator to order the set by (optionally