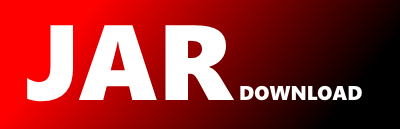
com.liferay.gradle.plugins.dependency.checker.internal.impl.MaxAgeDependencyCheckerImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.liferay.gradle.plugins.dependency.checker Show documentation
Show all versions of com.liferay.gradle.plugins.dependency.checker Show documentation
The Dependency Checker Gradle plugin lets you warn users if a specific configuration dependency is not the latest one available from the Maven central repository. The plugin eventually fails the build if the dependency age (the difference between the timestamp of the current version and the latest version) is above a predetermined threshold.
/**
* Copyright (c) 2000-present Liferay, Inc. All rights reserved.
*
* This library is free software; you can redistribute it and/or modify it under
* the terms of the GNU Lesser General Public License as published by the Free
* Software Foundation; either version 2.1 of the License, or (at your option)
* any later version.
*
* This library is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License for more
* details.
*/
package com.liferay.gradle.plugins.dependency.checker.internal.impl;
import com.liferay.gradle.plugins.dependency.checker.DependencyCheckerException;
import com.liferay.gradle.util.Validator;
import groovy.json.JsonSlurper;
import groovy.time.Duration;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.Date;
import java.util.List;
import java.util.Map;
import net.jodah.expiringmap.ExpiringMap;
import org.gradle.api.logging.Logger;
/**
* @author Andrea Di Giorgi
*/
public class MaxAgeDependencyCheckerImpl extends BaseDependencyCheckerImpl {
public MaxAgeDependencyCheckerImpl(Logger logger) {
_logger = logger;
}
@Override
public void check(String group, String name, String version)
throws Exception {
VersionInfo latestVersionInfo = _getVersionInfo(group, name, null);
if (latestVersionInfo.equals(VersionInfo.MISSING)) {
if (_logger.isWarnEnabled()) {
_logger.warn(
"Unable to get information about the latest version of " +
"'{}:{}'",
group, name);
}
return;
}
Date latestDate = new Date(latestVersionInfo.timestamp);
if (latestVersionInfo.version.equals(version)) {
if (_logger.isInfoEnabled()) {
_logger.info(
"Dependency '{}:{}:{}', published {}, is the latest one " +
"available",
group, name, version, latestDate);
}
return;
}
VersionInfo currentVersionInfo = _getVersionInfo(group, name, version);
if (currentVersionInfo.equals(VersionInfo.MISSING)) {
if (_logger.isInfoEnabled()) {
_logger.info(
"Unable to get information about '{}:{}:{}'", group, name,
version);
}
return;
}
Date currentDate = new Date(currentVersionInfo.timestamp);
if (latestVersionInfo.timestamp <=
(currentVersionInfo.timestamp +
_maxAge.toMilliseconds())) {
if (_logger.isWarnEnabled()) {
_logger.warn(
"Dependency '{}:{}:{}', published {}, is older than the " +
"latest one available ('{}:{}:{}', published {}), " +
"but not yet expired",
group, name, version, currentDate, group, name,
latestVersionInfo.version, latestDate);
}
return;
}
StringBuilder sb = new StringBuilder();
sb.append("Dependency '");
sb.append(group);
sb.append(':');
sb.append(name);
sb.append(':');
sb.append(version);
sb.append("', published ");
sb.append(currentDate);
sb.append(", is more than ");
sb.append(_maxAge);
sb.append(" older than the latest one available ('");
sb.append(group);
sb.append(':');
sb.append(name);
sb.append(':');
sb.append(latestVersionInfo.version);
sb.append("', published ");
sb.append(latestDate);
sb.append("), and it is expired");
if (isThrowError()) {
throw new DependencyCheckerException(sb.toString());
}
if (_logger.isWarnEnabled()) {
_logger.warn(sb.toString());
}
}
public Duration getMaxAge() {
return _maxAge;
}
public void setMaxAge(Duration maxAge) {
_maxAge = maxAge;
}
private VersionInfo _getVersionInfo(
String group, String name, String version)
throws MalformedURLException {
URL url = _getVersionInfoURL(group, name, version);
VersionInfo versionInfo = _versionInfos.get(url);
if (versionInfo != null) {
if (_logger.isDebugEnabled()) {
_logger.debug("Cache hit for {}: {}", url, versionInfo);
}
return versionInfo;
}
JsonSlurper jsonSlurper = new JsonSlurper();
Map map = (Map)jsonSlurper.parse(url);
Map responseMap = (Map)map.get(
"response");
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy