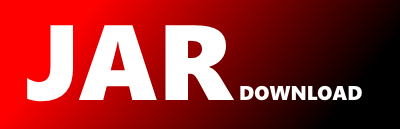
com.liferay.journal.service.persistence.impl.JournalArticlePersistenceImpl Maven / Gradle / Ivy
/**
* Copyright (c) 2000-present Liferay, Inc. All rights reserved.
*
* This library is free software; you can redistribute it and/or modify it under
* the terms of the GNU Lesser General Public License as published by the Free
* Software Foundation; either version 2.1 of the License, or (at your option)
* any later version.
*
* This library is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License for more
* details.
*/
package com.liferay.journal.service.persistence.impl;
import aQute.bnd.annotation.ProviderType;
import com.liferay.journal.exception.NoSuchArticleException;
import com.liferay.journal.model.JournalArticle;
import com.liferay.journal.model.impl.JournalArticleImpl;
import com.liferay.journal.model.impl.JournalArticleModelImpl;
import com.liferay.journal.service.persistence.JournalArticlePersistence;
import com.liferay.portal.kernel.dao.orm.EntityCache;
import com.liferay.portal.kernel.dao.orm.FinderCache;
import com.liferay.portal.kernel.dao.orm.FinderPath;
import com.liferay.portal.kernel.dao.orm.Query;
import com.liferay.portal.kernel.dao.orm.QueryPos;
import com.liferay.portal.kernel.dao.orm.QueryUtil;
import com.liferay.portal.kernel.dao.orm.SQLQuery;
import com.liferay.portal.kernel.dao.orm.Session;
import com.liferay.portal.kernel.log.Log;
import com.liferay.portal.kernel.log.LogFactoryUtil;
import com.liferay.portal.kernel.security.permission.InlineSQLHelperUtil;
import com.liferay.portal.kernel.service.ServiceContext;
import com.liferay.portal.kernel.service.ServiceContextThreadLocal;
import com.liferay.portal.kernel.service.persistence.CompanyProvider;
import com.liferay.portal.kernel.service.persistence.CompanyProviderWrapper;
import com.liferay.portal.kernel.service.persistence.impl.BasePersistenceImpl;
import com.liferay.portal.kernel.util.ArrayUtil;
import com.liferay.portal.kernel.util.OrderByComparator;
import com.liferay.portal.kernel.util.SetUtil;
import com.liferay.portal.kernel.util.StringBundler;
import com.liferay.portal.kernel.util.StringPool;
import com.liferay.portal.kernel.util.StringUtil;
import com.liferay.portal.kernel.util.Validator;
import com.liferay.portal.kernel.uuid.PortalUUIDUtil;
import com.liferay.portal.spring.extender.service.ServiceReference;
import java.io.Serializable;
import java.sql.Timestamp;
import java.util.Arrays;
import java.util.Collections;
import java.util.Date;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Set;
/**
* The persistence implementation for the journal article service.
*
*
* Caching information and settings can be found in portal.properties
*
*
* @author Brian Wing Shun Chan
* @see JournalArticlePersistence
* @see com.liferay.journal.service.persistence.JournalArticleUtil
* @generated
*/
@ProviderType
public class JournalArticlePersistenceImpl extends BasePersistenceImpl
implements JournalArticlePersistence {
/*
* NOTE FOR DEVELOPERS:
*
* Never modify or reference this class directly. Always use {@link JournalArticleUtil} to access the journal article persistence. Modify service.xml
and rerun ServiceBuilder to regenerate this class.
*/
public static final String FINDER_CLASS_NAME_ENTITY = JournalArticleImpl.class.getName();
public static final String FINDER_CLASS_NAME_LIST_WITH_PAGINATION = FINDER_CLASS_NAME_ENTITY +
".List1";
public static final String FINDER_CLASS_NAME_LIST_WITHOUT_PAGINATION = FINDER_CLASS_NAME_ENTITY +
".List2";
public static final FinderPath FINDER_PATH_WITH_PAGINATION_FIND_ALL = new FinderPath(JournalArticleModelImpl.ENTITY_CACHE_ENABLED,
JournalArticleModelImpl.FINDER_CACHE_ENABLED,
JournalArticleImpl.class, FINDER_CLASS_NAME_LIST_WITH_PAGINATION,
"findAll", new String[0]);
public static final FinderPath FINDER_PATH_WITHOUT_PAGINATION_FIND_ALL = new FinderPath(JournalArticleModelImpl.ENTITY_CACHE_ENABLED,
JournalArticleModelImpl.FINDER_CACHE_ENABLED,
JournalArticleImpl.class,
FINDER_CLASS_NAME_LIST_WITHOUT_PAGINATION, "findAll", new String[0]);
public static final FinderPath FINDER_PATH_COUNT_ALL = new FinderPath(JournalArticleModelImpl.ENTITY_CACHE_ENABLED,
JournalArticleModelImpl.FINDER_CACHE_ENABLED, Long.class,
FINDER_CLASS_NAME_LIST_WITHOUT_PAGINATION, "countAll", new String[0]);
public static final FinderPath FINDER_PATH_WITH_PAGINATION_FIND_BY_UUID = new FinderPath(JournalArticleModelImpl.ENTITY_CACHE_ENABLED,
JournalArticleModelImpl.FINDER_CACHE_ENABLED,
JournalArticleImpl.class, FINDER_CLASS_NAME_LIST_WITH_PAGINATION,
"findByUuid",
new String[] {
String.class.getName(),
Integer.class.getName(), Integer.class.getName(),
OrderByComparator.class.getName()
});
public static final FinderPath FINDER_PATH_WITHOUT_PAGINATION_FIND_BY_UUID = new FinderPath(JournalArticleModelImpl.ENTITY_CACHE_ENABLED,
JournalArticleModelImpl.FINDER_CACHE_ENABLED,
JournalArticleImpl.class,
FINDER_CLASS_NAME_LIST_WITHOUT_PAGINATION, "findByUuid",
new String[] { String.class.getName() },
JournalArticleModelImpl.UUID_COLUMN_BITMASK |
JournalArticleModelImpl.ARTICLEID_COLUMN_BITMASK |
JournalArticleModelImpl.VERSION_COLUMN_BITMASK);
public static final FinderPath FINDER_PATH_COUNT_BY_UUID = new FinderPath(JournalArticleModelImpl.ENTITY_CACHE_ENABLED,
JournalArticleModelImpl.FINDER_CACHE_ENABLED, Long.class,
FINDER_CLASS_NAME_LIST_WITHOUT_PAGINATION, "countByUuid",
new String[] { String.class.getName() });
/**
* Returns all the journal articles where uuid = ?.
*
* @param uuid the uuid
* @return the matching journal articles
*/
@Override
public List findByUuid(String uuid) {
return findByUuid(uuid, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the journal articles where uuid = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to {@link QueryUtil#ALL_POS} will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent and pagination is required (start
and end
are not {@link QueryUtil#ALL_POS}), then the query will include the default ORDER BY logic from {@link JournalArticleModelImpl}. If both orderByComparator
and pagination are absent, for performance reasons, the query will not have an ORDER BY clause and the returned result set will be sorted on by the primary key in an ascending order.
*
*
* @param uuid the uuid
* @param start the lower bound of the range of journal articles
* @param end the upper bound of the range of journal articles (not inclusive)
* @return the range of matching journal articles
*/
@Override
public List findByUuid(String uuid, int start, int end) {
return findByUuid(uuid, start, end, null);
}
/**
* Returns an ordered range of all the journal articles where uuid = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to {@link QueryUtil#ALL_POS} will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent and pagination is required (start
and end
are not {@link QueryUtil#ALL_POS}), then the query will include the default ORDER BY logic from {@link JournalArticleModelImpl}. If both orderByComparator
and pagination are absent, for performance reasons, the query will not have an ORDER BY clause and the returned result set will be sorted on by the primary key in an ascending order.
*
*
* @param uuid the uuid
* @param start the lower bound of the range of journal articles
* @param end the upper bound of the range of journal articles (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching journal articles
*/
@Override
public List findByUuid(String uuid, int start, int end,
OrderByComparator orderByComparator) {
return findByUuid(uuid, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the journal articles where uuid = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to {@link QueryUtil#ALL_POS} will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent and pagination is required (start
and end
are not {@link QueryUtil#ALL_POS}), then the query will include the default ORDER BY logic from {@link JournalArticleModelImpl}. If both orderByComparator
and pagination are absent, for performance reasons, the query will not have an ORDER BY clause and the returned result set will be sorted on by the primary key in an ascending order.
*
*
* @param uuid the uuid
* @param start the lower bound of the range of journal articles
* @param end the upper bound of the range of journal articles (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param retrieveFromCache whether to retrieve from the finder cache
* @return the ordered range of matching journal articles
*/
@Override
public List findByUuid(String uuid, int start, int end,
OrderByComparator orderByComparator,
boolean retrieveFromCache) {
boolean pagination = true;
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
pagination = false;
finderPath = FINDER_PATH_WITHOUT_PAGINATION_FIND_BY_UUID;
finderArgs = new Object[] { uuid };
}
else {
finderPath = FINDER_PATH_WITH_PAGINATION_FIND_BY_UUID;
finderArgs = new Object[] { uuid, start, end, orderByComparator };
}
List list = null;
if (retrieveFromCache) {
list = (List)finderCache.getResult(finderPath,
finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (JournalArticle journalArticle : list) {
if (!Objects.equals(uuid, journalArticle.getUuid())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler query = null;
if (orderByComparator != null) {
query = new StringBundler(3 +
(orderByComparator.getOrderByFields().length * 2));
}
else {
query = new StringBundler(3);
}
query.append(_SQL_SELECT_JOURNALARTICLE_WHERE);
boolean bindUuid = false;
if (uuid == null) {
query.append(_FINDER_COLUMN_UUID_UUID_1);
}
else if (uuid.equals(StringPool.BLANK)) {
query.append(_FINDER_COLUMN_UUID_UUID_3);
}
else {
bindUuid = true;
query.append(_FINDER_COLUMN_UUID_UUID_2);
}
if (orderByComparator != null) {
appendOrderByComparator(query, _ORDER_BY_ENTITY_ALIAS,
orderByComparator);
}
else
if (pagination) {
query.append(JournalArticleModelImpl.ORDER_BY_JPQL);
}
String sql = query.toString();
Session session = null;
try {
session = openSession();
Query q = session.createQuery(sql);
QueryPos qPos = QueryPos.getInstance(q);
if (bindUuid) {
qPos.add(uuid);
}
if (!pagination) {
list = (List)QueryUtil.list(q,
getDialect(), start, end, false);
Collections.sort(list);
list = Collections.unmodifiableList(list);
}
else {
list = (List)QueryUtil.list(q,
getDialect(), start, end);
}
cacheResult(list);
finderCache.putResult(finderPath, finderArgs, list);
}
catch (Exception e) {
finderCache.removeResult(finderPath, finderArgs);
throw processException(e);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first journal article in the ordered set where uuid = ?.
*
* @param uuid the uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching journal article
* @throws NoSuchArticleException if a matching journal article could not be found
*/
@Override
public JournalArticle findByUuid_First(String uuid,
OrderByComparator orderByComparator)
throws NoSuchArticleException {
JournalArticle journalArticle = fetchByUuid_First(uuid,
orderByComparator);
if (journalArticle != null) {
return journalArticle;
}
StringBundler msg = new StringBundler(4);
msg.append(_NO_SUCH_ENTITY_WITH_KEY);
msg.append("uuid=");
msg.append(uuid);
msg.append(StringPool.CLOSE_CURLY_BRACE);
throw new NoSuchArticleException(msg.toString());
}
/**
* Returns the first journal article in the ordered set where uuid = ?.
*
* @param uuid the uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching journal article, or null
if a matching journal article could not be found
*/
@Override
public JournalArticle fetchByUuid_First(String uuid,
OrderByComparator orderByComparator) {
List list = findByUuid(uuid, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last journal article in the ordered set where uuid = ?.
*
* @param uuid the uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching journal article
* @throws NoSuchArticleException if a matching journal article could not be found
*/
@Override
public JournalArticle findByUuid_Last(String uuid,
OrderByComparator orderByComparator)
throws NoSuchArticleException {
JournalArticle journalArticle = fetchByUuid_Last(uuid, orderByComparator);
if (journalArticle != null) {
return journalArticle;
}
StringBundler msg = new StringBundler(4);
msg.append(_NO_SUCH_ENTITY_WITH_KEY);
msg.append("uuid=");
msg.append(uuid);
msg.append(StringPool.CLOSE_CURLY_BRACE);
throw new NoSuchArticleException(msg.toString());
}
/**
* Returns the last journal article in the ordered set where uuid = ?.
*
* @param uuid the uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching journal article, or null
if a matching journal article could not be found
*/
@Override
public JournalArticle fetchByUuid_Last(String uuid,
OrderByComparator orderByComparator) {
int count = countByUuid(uuid);
if (count == 0) {
return null;
}
List list = findByUuid(uuid, count - 1, count,
orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the journal articles before and after the current journal article in the ordered set where uuid = ?.
*
* @param id the primary key of the current journal article
* @param uuid the uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next journal article
* @throws NoSuchArticleException if a journal article with the primary key could not be found
*/
@Override
public JournalArticle[] findByUuid_PrevAndNext(long id, String uuid,
OrderByComparator orderByComparator)
throws NoSuchArticleException {
JournalArticle journalArticle = findByPrimaryKey(id);
Session session = null;
try {
session = openSession();
JournalArticle[] array = new JournalArticleImpl[3];
array[0] = getByUuid_PrevAndNext(session, journalArticle, uuid,
orderByComparator, true);
array[1] = journalArticle;
array[2] = getByUuid_PrevAndNext(session, journalArticle, uuid,
orderByComparator, false);
return array;
}
catch (Exception e) {
throw processException(e);
}
finally {
closeSession(session);
}
}
protected JournalArticle getByUuid_PrevAndNext(Session session,
JournalArticle journalArticle, String uuid,
OrderByComparator orderByComparator, boolean previous) {
StringBundler query = null;
if (orderByComparator != null) {
query = new StringBundler(4 +
(orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
query = new StringBundler(3);
}
query.append(_SQL_SELECT_JOURNALARTICLE_WHERE);
boolean bindUuid = false;
if (uuid == null) {
query.append(_FINDER_COLUMN_UUID_UUID_1);
}
else if (uuid.equals(StringPool.BLANK)) {
query.append(_FINDER_COLUMN_UUID_UUID_3);
}
else {
bindUuid = true;
query.append(_FINDER_COLUMN_UUID_UUID_2);
}
if (orderByComparator != null) {
String[] orderByConditionFields = orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
query.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
query.append(_ORDER_BY_ENTITY_ALIAS);
query.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
query.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
query.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
query.append(WHERE_GREATER_THAN);
}
else {
query.append(WHERE_LESSER_THAN);
}
}
}
query.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
query.append(_ORDER_BY_ENTITY_ALIAS);
query.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
query.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
query.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
query.append(ORDER_BY_ASC);
}
else {
query.append(ORDER_BY_DESC);
}
}
}
}
else {
query.append(JournalArticleModelImpl.ORDER_BY_JPQL);
}
String sql = query.toString();
Query q = session.createQuery(sql);
q.setFirstResult(0);
q.setMaxResults(2);
QueryPos qPos = QueryPos.getInstance(q);
if (bindUuid) {
qPos.add(uuid);
}
if (orderByComparator != null) {
Object[] values = orderByComparator.getOrderByConditionValues(journalArticle);
for (Object value : values) {
qPos.add(value);
}
}
List list = q.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the journal articles where uuid = ? from the database.
*
* @param uuid the uuid
*/
@Override
public void removeByUuid(String uuid) {
for (JournalArticle journalArticle : findByUuid(uuid,
QueryUtil.ALL_POS, QueryUtil.ALL_POS, null)) {
remove(journalArticle);
}
}
/**
* Returns the number of journal articles where uuid = ?.
*
* @param uuid the uuid
* @return the number of matching journal articles
*/
@Override
public int countByUuid(String uuid) {
FinderPath finderPath = FINDER_PATH_COUNT_BY_UUID;
Object[] finderArgs = new Object[] { uuid };
Long count = (Long)finderCache.getResult(finderPath, finderArgs, this);
if (count == null) {
StringBundler query = new StringBundler(2);
query.append(_SQL_COUNT_JOURNALARTICLE_WHERE);
boolean bindUuid = false;
if (uuid == null) {
query.append(_FINDER_COLUMN_UUID_UUID_1);
}
else if (uuid.equals(StringPool.BLANK)) {
query.append(_FINDER_COLUMN_UUID_UUID_3);
}
else {
bindUuid = true;
query.append(_FINDER_COLUMN_UUID_UUID_2);
}
String sql = query.toString();
Session session = null;
try {
session = openSession();
Query q = session.createQuery(sql);
QueryPos qPos = QueryPos.getInstance(q);
if (bindUuid) {
qPos.add(uuid);
}
count = (Long)q.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception e) {
finderCache.removeResult(finderPath, finderArgs);
throw processException(e);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String _FINDER_COLUMN_UUID_UUID_1 = "journalArticle.uuid IS NULL";
private static final String _FINDER_COLUMN_UUID_UUID_2 = "journalArticle.uuid = ?";
private static final String _FINDER_COLUMN_UUID_UUID_3 = "(journalArticle.uuid IS NULL OR journalArticle.uuid = '')";
public static final FinderPath FINDER_PATH_FETCH_BY_UUID_G = new FinderPath(JournalArticleModelImpl.ENTITY_CACHE_ENABLED,
JournalArticleModelImpl.FINDER_CACHE_ENABLED,
JournalArticleImpl.class, FINDER_CLASS_NAME_ENTITY,
"fetchByUUID_G",
new String[] { String.class.getName(), Long.class.getName() },
JournalArticleModelImpl.UUID_COLUMN_BITMASK |
JournalArticleModelImpl.GROUPID_COLUMN_BITMASK);
public static final FinderPath FINDER_PATH_COUNT_BY_UUID_G = new FinderPath(JournalArticleModelImpl.ENTITY_CACHE_ENABLED,
JournalArticleModelImpl.FINDER_CACHE_ENABLED, Long.class,
FINDER_CLASS_NAME_LIST_WITHOUT_PAGINATION, "countByUUID_G",
new String[] { String.class.getName(), Long.class.getName() });
/**
* Returns the journal article where uuid = ? and groupId = ? or throws a {@link NoSuchArticleException} if it could not be found.
*
* @param uuid the uuid
* @param groupId the group ID
* @return the matching journal article
* @throws NoSuchArticleException if a matching journal article could not be found
*/
@Override
public JournalArticle findByUUID_G(String uuid, long groupId)
throws NoSuchArticleException {
JournalArticle journalArticle = fetchByUUID_G(uuid, groupId);
if (journalArticle == null) {
StringBundler msg = new StringBundler(6);
msg.append(_NO_SUCH_ENTITY_WITH_KEY);
msg.append("uuid=");
msg.append(uuid);
msg.append(", groupId=");
msg.append(groupId);
msg.append(StringPool.CLOSE_CURLY_BRACE);
if (_log.isDebugEnabled()) {
_log.debug(msg.toString());
}
throw new NoSuchArticleException(msg.toString());
}
return journalArticle;
}
/**
* Returns the journal article where uuid = ? and groupId = ? or returns null
if it could not be found. Uses the finder cache.
*
* @param uuid the uuid
* @param groupId the group ID
* @return the matching journal article, or null
if a matching journal article could not be found
*/
@Override
public JournalArticle fetchByUUID_G(String uuid, long groupId) {
return fetchByUUID_G(uuid, groupId, true);
}
/**
* Returns the journal article where uuid = ? and groupId = ? or returns null
if it could not be found, optionally using the finder cache.
*
* @param uuid the uuid
* @param groupId the group ID
* @param retrieveFromCache whether to retrieve from the finder cache
* @return the matching journal article, or null
if a matching journal article could not be found
*/
@Override
public JournalArticle fetchByUUID_G(String uuid, long groupId,
boolean retrieveFromCache) {
Object[] finderArgs = new Object[] { uuid, groupId };
Object result = null;
if (retrieveFromCache) {
result = finderCache.getResult(FINDER_PATH_FETCH_BY_UUID_G,
finderArgs, this);
}
if (result instanceof JournalArticle) {
JournalArticle journalArticle = (JournalArticle)result;
if (!Objects.equals(uuid, journalArticle.getUuid()) ||
(groupId != journalArticle.getGroupId())) {
result = null;
}
}
if (result == null) {
StringBundler query = new StringBundler(4);
query.append(_SQL_SELECT_JOURNALARTICLE_WHERE);
boolean bindUuid = false;
if (uuid == null) {
query.append(_FINDER_COLUMN_UUID_G_UUID_1);
}
else if (uuid.equals(StringPool.BLANK)) {
query.append(_FINDER_COLUMN_UUID_G_UUID_3);
}
else {
bindUuid = true;
query.append(_FINDER_COLUMN_UUID_G_UUID_2);
}
query.append(_FINDER_COLUMN_UUID_G_GROUPID_2);
String sql = query.toString();
Session session = null;
try {
session = openSession();
Query q = session.createQuery(sql);
QueryPos qPos = QueryPos.getInstance(q);
if (bindUuid) {
qPos.add(uuid);
}
qPos.add(groupId);
List list = q.list();
if (list.isEmpty()) {
finderCache.putResult(FINDER_PATH_FETCH_BY_UUID_G,
finderArgs, list);
}
else {
JournalArticle journalArticle = list.get(0);
result = journalArticle;
cacheResult(journalArticle);
if ((journalArticle.getUuid() == null) ||
!journalArticle.getUuid().equals(uuid) ||
(journalArticle.getGroupId() != groupId)) {
finderCache.putResult(FINDER_PATH_FETCH_BY_UUID_G,
finderArgs, journalArticle);
}
}
}
catch (Exception e) {
finderCache.removeResult(FINDER_PATH_FETCH_BY_UUID_G, finderArgs);
throw processException(e);
}
finally {
closeSession(session);
}
}
if (result instanceof List>) {
return null;
}
else {
return (JournalArticle)result;
}
}
/**
* Removes the journal article where uuid = ? and groupId = ? from the database.
*
* @param uuid the uuid
* @param groupId the group ID
* @return the journal article that was removed
*/
@Override
public JournalArticle removeByUUID_G(String uuid, long groupId)
throws NoSuchArticleException {
JournalArticle journalArticle = findByUUID_G(uuid, groupId);
return remove(journalArticle);
}
/**
* Returns the number of journal articles where uuid = ? and groupId = ?.
*
* @param uuid the uuid
* @param groupId the group ID
* @return the number of matching journal articles
*/
@Override
public int countByUUID_G(String uuid, long groupId) {
FinderPath finderPath = FINDER_PATH_COUNT_BY_UUID_G;
Object[] finderArgs = new Object[] { uuid, groupId };
Long count = (Long)finderCache.getResult(finderPath, finderArgs, this);
if (count == null) {
StringBundler query = new StringBundler(3);
query.append(_SQL_COUNT_JOURNALARTICLE_WHERE);
boolean bindUuid = false;
if (uuid == null) {
query.append(_FINDER_COLUMN_UUID_G_UUID_1);
}
else if (uuid.equals(StringPool.BLANK)) {
query.append(_FINDER_COLUMN_UUID_G_UUID_3);
}
else {
bindUuid = true;
query.append(_FINDER_COLUMN_UUID_G_UUID_2);
}
query.append(_FINDER_COLUMN_UUID_G_GROUPID_2);
String sql = query.toString();
Session session = null;
try {
session = openSession();
Query q = session.createQuery(sql);
QueryPos qPos = QueryPos.getInstance(q);
if (bindUuid) {
qPos.add(uuid);
}
qPos.add(groupId);
count = (Long)q.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception e) {
finderCache.removeResult(finderPath, finderArgs);
throw processException(e);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String _FINDER_COLUMN_UUID_G_UUID_1 = "journalArticle.uuid IS NULL AND ";
private static final String _FINDER_COLUMN_UUID_G_UUID_2 = "journalArticle.uuid = ? AND ";
private static final String _FINDER_COLUMN_UUID_G_UUID_3 = "(journalArticle.uuid IS NULL OR journalArticle.uuid = '') AND ";
private static final String _FINDER_COLUMN_UUID_G_GROUPID_2 = "journalArticle.groupId = ?";
public static final FinderPath FINDER_PATH_WITH_PAGINATION_FIND_BY_UUID_C = new FinderPath(JournalArticleModelImpl.ENTITY_CACHE_ENABLED,
JournalArticleModelImpl.FINDER_CACHE_ENABLED,
JournalArticleImpl.class, FINDER_CLASS_NAME_LIST_WITH_PAGINATION,
"findByUuid_C",
new String[] {
String.class.getName(), Long.class.getName(),
Integer.class.getName(), Integer.class.getName(),
OrderByComparator.class.getName()
});
public static final FinderPath FINDER_PATH_WITHOUT_PAGINATION_FIND_BY_UUID_C =
new FinderPath(JournalArticleModelImpl.ENTITY_CACHE_ENABLED,
JournalArticleModelImpl.FINDER_CACHE_ENABLED,
JournalArticleImpl.class,
FINDER_CLASS_NAME_LIST_WITHOUT_PAGINATION, "findByUuid_C",
new String[] { String.class.getName(), Long.class.getName() },
JournalArticleModelImpl.UUID_COLUMN_BITMASK |
JournalArticleModelImpl.COMPANYID_COLUMN_BITMASK |
JournalArticleModelImpl.ARTICLEID_COLUMN_BITMASK |
JournalArticleModelImpl.VERSION_COLUMN_BITMASK);
public static final FinderPath FINDER_PATH_COUNT_BY_UUID_C = new FinderPath(JournalArticleModelImpl.ENTITY_CACHE_ENABLED,
JournalArticleModelImpl.FINDER_CACHE_ENABLED, Long.class,
FINDER_CLASS_NAME_LIST_WITHOUT_PAGINATION, "countByUuid_C",
new String[] { String.class.getName(), Long.class.getName() });
/**
* Returns all the journal articles where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @return the matching journal articles
*/
@Override
public List findByUuid_C(String uuid, long companyId) {
return findByUuid_C(uuid, companyId, QueryUtil.ALL_POS,
QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the journal articles where uuid = ? and companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to {@link QueryUtil#ALL_POS} will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent and pagination is required (start
and end
are not {@link QueryUtil#ALL_POS}), then the query will include the default ORDER BY logic from {@link JournalArticleModelImpl}. If both orderByComparator
and pagination are absent, for performance reasons, the query will not have an ORDER BY clause and the returned result set will be sorted on by the primary key in an ascending order.
*
*
* @param uuid the uuid
* @param companyId the company ID
* @param start the lower bound of the range of journal articles
* @param end the upper bound of the range of journal articles (not inclusive)
* @return the range of matching journal articles
*/
@Override
public List findByUuid_C(String uuid, long companyId,
int start, int end) {
return findByUuid_C(uuid, companyId, start, end, null);
}
/**
* Returns an ordered range of all the journal articles where uuid = ? and companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to {@link QueryUtil#ALL_POS} will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent and pagination is required (start
and end
are not {@link QueryUtil#ALL_POS}), then the query will include the default ORDER BY logic from {@link JournalArticleModelImpl}. If both orderByComparator
and pagination are absent, for performance reasons, the query will not have an ORDER BY clause and the returned result set will be sorted on by the primary key in an ascending order.
*
*
* @param uuid the uuid
* @param companyId the company ID
* @param start the lower bound of the range of journal articles
* @param end the upper bound of the range of journal articles (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching journal articles
*/
@Override
public List findByUuid_C(String uuid, long companyId,
int start, int end, OrderByComparator orderByComparator) {
return findByUuid_C(uuid, companyId, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the journal articles where uuid = ? and companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to {@link QueryUtil#ALL_POS} will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent and pagination is required (start
and end
are not {@link QueryUtil#ALL_POS}), then the query will include the default ORDER BY logic from {@link JournalArticleModelImpl}. If both orderByComparator
and pagination are absent, for performance reasons, the query will not have an ORDER BY clause and the returned result set will be sorted on by the primary key in an ascending order.
*
*
* @param uuid the uuid
* @param companyId the company ID
* @param start the lower bound of the range of journal articles
* @param end the upper bound of the range of journal articles (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param retrieveFromCache whether to retrieve from the finder cache
* @return the ordered range of matching journal articles
*/
@Override
public List findByUuid_C(String uuid, long companyId,
int start, int end,
OrderByComparator orderByComparator,
boolean retrieveFromCache) {
boolean pagination = true;
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
pagination = false;
finderPath = FINDER_PATH_WITHOUT_PAGINATION_FIND_BY_UUID_C;
finderArgs = new Object[] { uuid, companyId };
}
else {
finderPath = FINDER_PATH_WITH_PAGINATION_FIND_BY_UUID_C;
finderArgs = new Object[] {
uuid, companyId,
start, end, orderByComparator
};
}
List list = null;
if (retrieveFromCache) {
list = (List)finderCache.getResult(finderPath,
finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (JournalArticle journalArticle : list) {
if (!Objects.equals(uuid, journalArticle.getUuid()) ||
(companyId != journalArticle.getCompanyId())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler query = null;
if (orderByComparator != null) {
query = new StringBundler(4 +
(orderByComparator.getOrderByFields().length * 2));
}
else {
query = new StringBundler(4);
}
query.append(_SQL_SELECT_JOURNALARTICLE_WHERE);
boolean bindUuid = false;
if (uuid == null) {
query.append(_FINDER_COLUMN_UUID_C_UUID_1);
}
else if (uuid.equals(StringPool.BLANK)) {
query.append(_FINDER_COLUMN_UUID_C_UUID_3);
}
else {
bindUuid = true;
query.append(_FINDER_COLUMN_UUID_C_UUID_2);
}
query.append(_FINDER_COLUMN_UUID_C_COMPANYID_2);
if (orderByComparator != null) {
appendOrderByComparator(query, _ORDER_BY_ENTITY_ALIAS,
orderByComparator);
}
else
if (pagination) {
query.append(JournalArticleModelImpl.ORDER_BY_JPQL);
}
String sql = query.toString();
Session session = null;
try {
session = openSession();
Query q = session.createQuery(sql);
QueryPos qPos = QueryPos.getInstance(q);
if (bindUuid) {
qPos.add(uuid);
}
qPos.add(companyId);
if (!pagination) {
list = (List)QueryUtil.list(q,
getDialect(), start, end, false);
Collections.sort(list);
list = Collections.unmodifiableList(list);
}
else {
list = (List)QueryUtil.list(q,
getDialect(), start, end);
}
cacheResult(list);
finderCache.putResult(finderPath, finderArgs, list);
}
catch (Exception e) {
finderCache.removeResult(finderPath, finderArgs);
throw processException(e);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first journal article in the ordered set where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching journal article
* @throws NoSuchArticleException if a matching journal article could not be found
*/
@Override
public JournalArticle findByUuid_C_First(String uuid, long companyId,
OrderByComparator orderByComparator)
throws NoSuchArticleException {
JournalArticle journalArticle = fetchByUuid_C_First(uuid, companyId,
orderByComparator);
if (journalArticle != null) {
return journalArticle;
}
StringBundler msg = new StringBundler(6);
msg.append(_NO_SUCH_ENTITY_WITH_KEY);
msg.append("uuid=");
msg.append(uuid);
msg.append(", companyId=");
msg.append(companyId);
msg.append(StringPool.CLOSE_CURLY_BRACE);
throw new NoSuchArticleException(msg.toString());
}
/**
* Returns the first journal article in the ordered set where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching journal article, or null
if a matching journal article could not be found
*/
@Override
public JournalArticle fetchByUuid_C_First(String uuid, long companyId,
OrderByComparator orderByComparator) {
List list = findByUuid_C(uuid, companyId, 0, 1,
orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last journal article in the ordered set where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching journal article
* @throws NoSuchArticleException if a matching journal article could not be found
*/
@Override
public JournalArticle findByUuid_C_Last(String uuid, long companyId,
OrderByComparator orderByComparator)
throws NoSuchArticleException {
JournalArticle journalArticle = fetchByUuid_C_Last(uuid, companyId,
orderByComparator);
if (journalArticle != null) {
return journalArticle;
}
StringBundler msg = new StringBundler(6);
msg.append(_NO_SUCH_ENTITY_WITH_KEY);
msg.append("uuid=");
msg.append(uuid);
msg.append(", companyId=");
msg.append(companyId);
msg.append(StringPool.CLOSE_CURLY_BRACE);
throw new NoSuchArticleException(msg.toString());
}
/**
* Returns the last journal article in the ordered set where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching journal article, or null
if a matching journal article could not be found
*/
@Override
public JournalArticle fetchByUuid_C_Last(String uuid, long companyId,
OrderByComparator orderByComparator) {
int count = countByUuid_C(uuid, companyId);
if (count == 0) {
return null;
}
List list = findByUuid_C(uuid, companyId, count - 1,
count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the journal articles before and after the current journal article in the ordered set where uuid = ? and companyId = ?.
*
* @param id the primary key of the current journal article
* @param uuid the uuid
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next journal article
* @throws NoSuchArticleException if a journal article with the primary key could not be found
*/
@Override
public JournalArticle[] findByUuid_C_PrevAndNext(long id, String uuid,
long companyId, OrderByComparator orderByComparator)
throws NoSuchArticleException {
JournalArticle journalArticle = findByPrimaryKey(id);
Session session = null;
try {
session = openSession();
JournalArticle[] array = new JournalArticleImpl[3];
array[0] = getByUuid_C_PrevAndNext(session, journalArticle, uuid,
companyId, orderByComparator, true);
array[1] = journalArticle;
array[2] = getByUuid_C_PrevAndNext(session, journalArticle, uuid,
companyId, orderByComparator, false);
return array;
}
catch (Exception e) {
throw processException(e);
}
finally {
closeSession(session);
}
}
protected JournalArticle getByUuid_C_PrevAndNext(Session session,
JournalArticle journalArticle, String uuid, long companyId,
OrderByComparator orderByComparator, boolean previous) {
StringBundler query = null;
if (orderByComparator != null) {
query = new StringBundler(5 +
(orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
query = new StringBundler(4);
}
query.append(_SQL_SELECT_JOURNALARTICLE_WHERE);
boolean bindUuid = false;
if (uuid == null) {
query.append(_FINDER_COLUMN_UUID_C_UUID_1);
}
else if (uuid.equals(StringPool.BLANK)) {
query.append(_FINDER_COLUMN_UUID_C_UUID_3);
}
else {
bindUuid = true;
query.append(_FINDER_COLUMN_UUID_C_UUID_2);
}
query.append(_FINDER_COLUMN_UUID_C_COMPANYID_2);
if (orderByComparator != null) {
String[] orderByConditionFields = orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
query.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
query.append(_ORDER_BY_ENTITY_ALIAS);
query.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
query.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
query.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
query.append(WHERE_GREATER_THAN);
}
else {
query.append(WHERE_LESSER_THAN);
}
}
}
query.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
query.append(_ORDER_BY_ENTITY_ALIAS);
query.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
query.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
query.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
query.append(ORDER_BY_ASC);
}
else {
query.append(ORDER_BY_DESC);
}
}
}
}
else {
query.append(JournalArticleModelImpl.ORDER_BY_JPQL);
}
String sql = query.toString();
Query q = session.createQuery(sql);
q.setFirstResult(0);
q.setMaxResults(2);
QueryPos qPos = QueryPos.getInstance(q);
if (bindUuid) {
qPos.add(uuid);
}
qPos.add(companyId);
if (orderByComparator != null) {
Object[] values = orderByComparator.getOrderByConditionValues(journalArticle);
for (Object value : values) {
qPos.add(value);
}
}
List list = q.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the journal articles where uuid = ? and companyId = ? from the database.
*
* @param uuid the uuid
* @param companyId the company ID
*/
@Override
public void removeByUuid_C(String uuid, long companyId) {
for (JournalArticle journalArticle : findByUuid_C(uuid, companyId,
QueryUtil.ALL_POS, QueryUtil.ALL_POS, null)) {
remove(journalArticle);
}
}
/**
* Returns the number of journal articles where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @return the number of matching journal articles
*/
@Override
public int countByUuid_C(String uuid, long companyId) {
FinderPath finderPath = FINDER_PATH_COUNT_BY_UUID_C;
Object[] finderArgs = new Object[] { uuid, companyId };
Long count = (Long)finderCache.getResult(finderPath, finderArgs, this);
if (count == null) {
StringBundler query = new StringBundler(3);
query.append(_SQL_COUNT_JOURNALARTICLE_WHERE);
boolean bindUuid = false;
if (uuid == null) {
query.append(_FINDER_COLUMN_UUID_C_UUID_1);
}
else if (uuid.equals(StringPool.BLANK)) {
query.append(_FINDER_COLUMN_UUID_C_UUID_3);
}
else {
bindUuid = true;
query.append(_FINDER_COLUMN_UUID_C_UUID_2);
}
query.append(_FINDER_COLUMN_UUID_C_COMPANYID_2);
String sql = query.toString();
Session session = null;
try {
session = openSession();
Query q = session.createQuery(sql);
QueryPos qPos = QueryPos.getInstance(q);
if (bindUuid) {
qPos.add(uuid);
}
qPos.add(companyId);
count = (Long)q.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception e) {
finderCache.removeResult(finderPath, finderArgs);
throw processException(e);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String _FINDER_COLUMN_UUID_C_UUID_1 = "journalArticle.uuid IS NULL AND ";
private static final String _FINDER_COLUMN_UUID_C_UUID_2 = "journalArticle.uuid = ? AND ";
private static final String _FINDER_COLUMN_UUID_C_UUID_3 = "(journalArticle.uuid IS NULL OR journalArticle.uuid = '') AND ";
private static final String _FINDER_COLUMN_UUID_C_COMPANYID_2 = "journalArticle.companyId = ?";
public static final FinderPath FINDER_PATH_WITH_PAGINATION_FIND_BY_RESOURCEPRIMKEY =
new FinderPath(JournalArticleModelImpl.ENTITY_CACHE_ENABLED,
JournalArticleModelImpl.FINDER_CACHE_ENABLED,
JournalArticleImpl.class, FINDER_CLASS_NAME_LIST_WITH_PAGINATION,
"findByResourcePrimKey",
new String[] {
Long.class.getName(),
Integer.class.getName(), Integer.class.getName(),
OrderByComparator.class.getName()
});
public static final FinderPath FINDER_PATH_WITHOUT_PAGINATION_FIND_BY_RESOURCEPRIMKEY =
new FinderPath(JournalArticleModelImpl.ENTITY_CACHE_ENABLED,
JournalArticleModelImpl.FINDER_CACHE_ENABLED,
JournalArticleImpl.class,
FINDER_CLASS_NAME_LIST_WITHOUT_PAGINATION, "findByResourcePrimKey",
new String[] { Long.class.getName() },
JournalArticleModelImpl.RESOURCEPRIMKEY_COLUMN_BITMASK |
JournalArticleModelImpl.ARTICLEID_COLUMN_BITMASK |
JournalArticleModelImpl.VERSION_COLUMN_BITMASK);
public static final FinderPath FINDER_PATH_COUNT_BY_RESOURCEPRIMKEY = new FinderPath(JournalArticleModelImpl.ENTITY_CACHE_ENABLED,
JournalArticleModelImpl.FINDER_CACHE_ENABLED, Long.class,
FINDER_CLASS_NAME_LIST_WITHOUT_PAGINATION,
"countByResourcePrimKey", new String[] { Long.class.getName() });
/**
* Returns all the journal articles where resourcePrimKey = ?.
*
* @param resourcePrimKey the resource prim key
* @return the matching journal articles
*/
@Override
public List findByResourcePrimKey(long resourcePrimKey) {
return findByResourcePrimKey(resourcePrimKey, QueryUtil.ALL_POS,
QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the journal articles where resourcePrimKey = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to {@link QueryUtil#ALL_POS} will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent and pagination is required (start
and end
are not {@link QueryUtil#ALL_POS}), then the query will include the default ORDER BY logic from {@link JournalArticleModelImpl}. If both orderByComparator
and pagination are absent, for performance reasons, the query will not have an ORDER BY clause and the returned result set will be sorted on by the primary key in an ascending order.
*
*
* @param resourcePrimKey the resource prim key
* @param start the lower bound of the range of journal articles
* @param end the upper bound of the range of journal articles (not inclusive)
* @return the range of matching journal articles
*/
@Override
public List findByResourcePrimKey(long resourcePrimKey,
int start, int end) {
return findByResourcePrimKey(resourcePrimKey, start, end, null);
}
/**
* Returns an ordered range of all the journal articles where resourcePrimKey = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to {@link QueryUtil#ALL_POS} will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent and pagination is required (start
and end
are not {@link QueryUtil#ALL_POS}), then the query will include the default ORDER BY logic from {@link JournalArticleModelImpl}. If both orderByComparator
and pagination are absent, for performance reasons, the query will not have an ORDER BY clause and the returned result set will be sorted on by the primary key in an ascending order.
*
*
* @param resourcePrimKey the resource prim key
* @param start the lower bound of the range of journal articles
* @param end the upper bound of the range of journal articles (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching journal articles
*/
@Override
public List findByResourcePrimKey(long resourcePrimKey,
int start, int end, OrderByComparator orderByComparator) {
return findByResourcePrimKey(resourcePrimKey, start, end,
orderByComparator, true);
}
/**
* Returns an ordered range of all the journal articles where resourcePrimKey = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to {@link QueryUtil#ALL_POS} will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent and pagination is required (start
and end
are not {@link QueryUtil#ALL_POS}), then the query will include the default ORDER BY logic from {@link JournalArticleModelImpl}. If both orderByComparator
and pagination are absent, for performance reasons, the query will not have an ORDER BY clause and the returned result set will be sorted on by the primary key in an ascending order.
*
*
* @param resourcePrimKey the resource prim key
* @param start the lower bound of the range of journal articles
* @param end the upper bound of the range of journal articles (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param retrieveFromCache whether to retrieve from the finder cache
* @return the ordered range of matching journal articles
*/
@Override
public List findByResourcePrimKey(long resourcePrimKey,
int start, int end,
OrderByComparator orderByComparator,
boolean retrieveFromCache) {
boolean pagination = true;
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
pagination = false;
finderPath = FINDER_PATH_WITHOUT_PAGINATION_FIND_BY_RESOURCEPRIMKEY;
finderArgs = new Object[] { resourcePrimKey };
}
else {
finderPath = FINDER_PATH_WITH_PAGINATION_FIND_BY_RESOURCEPRIMKEY;
finderArgs = new Object[] {
resourcePrimKey,
start, end, orderByComparator
};
}
List list = null;
if (retrieveFromCache) {
list = (List)finderCache.getResult(finderPath,
finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (JournalArticle journalArticle : list) {
if ((resourcePrimKey != journalArticle.getResourcePrimKey())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler query = null;
if (orderByComparator != null) {
query = new StringBundler(3 +
(orderByComparator.getOrderByFields().length * 2));
}
else {
query = new StringBundler(3);
}
query.append(_SQL_SELECT_JOURNALARTICLE_WHERE);
query.append(_FINDER_COLUMN_RESOURCEPRIMKEY_RESOURCEPRIMKEY_2);
if (orderByComparator != null) {
appendOrderByComparator(query, _ORDER_BY_ENTITY_ALIAS,
orderByComparator);
}
else
if (pagination) {
query.append(JournalArticleModelImpl.ORDER_BY_JPQL);
}
String sql = query.toString();
Session session = null;
try {
session = openSession();
Query q = session.createQuery(sql);
QueryPos qPos = QueryPos.getInstance(q);
qPos.add(resourcePrimKey);
if (!pagination) {
list = (List)QueryUtil.list(q,
getDialect(), start, end, false);
Collections.sort(list);
list = Collections.unmodifiableList(list);
}
else {
list = (List)QueryUtil.list(q,
getDialect(), start, end);
}
cacheResult(list);
finderCache.putResult(finderPath, finderArgs, list);
}
catch (Exception e) {
finderCache.removeResult(finderPath, finderArgs);
throw processException(e);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first journal article in the ordered set where resourcePrimKey = ?.
*
* @param resourcePrimKey the resource prim key
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching journal article
* @throws NoSuchArticleException if a matching journal article could not be found
*/
@Override
public JournalArticle findByResourcePrimKey_First(long resourcePrimKey,
OrderByComparator orderByComparator)
throws NoSuchArticleException {
JournalArticle journalArticle = fetchByResourcePrimKey_First(resourcePrimKey,
orderByComparator);
if (journalArticle != null) {
return journalArticle;
}
StringBundler msg = new StringBundler(4);
msg.append(_NO_SUCH_ENTITY_WITH_KEY);
msg.append("resourcePrimKey=");
msg.append(resourcePrimKey);
msg.append(StringPool.CLOSE_CURLY_BRACE);
throw new NoSuchArticleException(msg.toString());
}
/**
* Returns the first journal article in the ordered set where resourcePrimKey = ?.
*
* @param resourcePrimKey the resource prim key
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching journal article, or null
if a matching journal article could not be found
*/
@Override
public JournalArticle fetchByResourcePrimKey_First(long resourcePrimKey,
OrderByComparator orderByComparator) {
List list = findByResourcePrimKey(resourcePrimKey, 0,
1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last journal article in the ordered set where resourcePrimKey = ?.
*
* @param resourcePrimKey the resource prim key
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching journal article
* @throws NoSuchArticleException if a matching journal article could not be found
*/
@Override
public JournalArticle findByResourcePrimKey_Last(long resourcePrimKey,
OrderByComparator orderByComparator)
throws NoSuchArticleException {
JournalArticle journalArticle = fetchByResourcePrimKey_Last(resourcePrimKey,
orderByComparator);
if (journalArticle != null) {
return journalArticle;
}
StringBundler msg = new StringBundler(4);
msg.append(_NO_SUCH_ENTITY_WITH_KEY);
msg.append("resourcePrimKey=");
msg.append(resourcePrimKey);
msg.append(StringPool.CLOSE_CURLY_BRACE);
throw new NoSuchArticleException(msg.toString());
}
/**
* Returns the last journal article in the ordered set where resourcePrimKey = ?.
*
* @param resourcePrimKey the resource prim key
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching journal article, or null
if a matching journal article could not be found
*/
@Override
public JournalArticle fetchByResourcePrimKey_Last(long resourcePrimKey,
OrderByComparator orderByComparator) {
int count = countByResourcePrimKey(resourcePrimKey);
if (count == 0) {
return null;
}
List list = findByResourcePrimKey(resourcePrimKey,
count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the journal articles before and after the current journal article in the ordered set where resourcePrimKey = ?.
*
* @param id the primary key of the current journal article
* @param resourcePrimKey the resource prim key
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next journal article
* @throws NoSuchArticleException if a journal article with the primary key could not be found
*/
@Override
public JournalArticle[] findByResourcePrimKey_PrevAndNext(long id,
long resourcePrimKey,
OrderByComparator orderByComparator)
throws NoSuchArticleException {
JournalArticle journalArticle = findByPrimaryKey(id);
Session session = null;
try {
session = openSession();
JournalArticle[] array = new JournalArticleImpl[3];
array[0] = getByResourcePrimKey_PrevAndNext(session,
journalArticle, resourcePrimKey, orderByComparator, true);
array[1] = journalArticle;
array[2] = getByResourcePrimKey_PrevAndNext(session,
journalArticle, resourcePrimKey, orderByComparator, false);
return array;
}
catch (Exception e) {
throw processException(e);
}
finally {
closeSession(session);
}
}
protected JournalArticle getByResourcePrimKey_PrevAndNext(Session session,
JournalArticle journalArticle, long resourcePrimKey,
OrderByComparator orderByComparator, boolean previous) {
StringBundler query = null;
if (orderByComparator != null) {
query = new StringBundler(4 +
(orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
query = new StringBundler(3);
}
query.append(_SQL_SELECT_JOURNALARTICLE_WHERE);
query.append(_FINDER_COLUMN_RESOURCEPRIMKEY_RESOURCEPRIMKEY_2);
if (orderByComparator != null) {
String[] orderByConditionFields = orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
query.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
query.append(_ORDER_BY_ENTITY_ALIAS);
query.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
query.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
query.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
query.append(WHERE_GREATER_THAN);
}
else {
query.append(WHERE_LESSER_THAN);
}
}
}
query.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
query.append(_ORDER_BY_ENTITY_ALIAS);
query.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
query.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
query.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
query.append(ORDER_BY_ASC);
}
else {
query.append(ORDER_BY_DESC);
}
}
}
}
else {
query.append(JournalArticleModelImpl.ORDER_BY_JPQL);
}
String sql = query.toString();
Query q = session.createQuery(sql);
q.setFirstResult(0);
q.setMaxResults(2);
QueryPos qPos = QueryPos.getInstance(q);
qPos.add(resourcePrimKey);
if (orderByComparator != null) {
Object[] values = orderByComparator.getOrderByConditionValues(journalArticle);
for (Object value : values) {
qPos.add(value);
}
}
List list = q.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the journal articles where resourcePrimKey = ? from the database.
*
* @param resourcePrimKey the resource prim key
*/
@Override
public void removeByResourcePrimKey(long resourcePrimKey) {
for (JournalArticle journalArticle : findByResourcePrimKey(
resourcePrimKey, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null)) {
remove(journalArticle);
}
}
/**
* Returns the number of journal articles where resourcePrimKey = ?.
*
* @param resourcePrimKey the resource prim key
* @return the number of matching journal articles
*/
@Override
public int countByResourcePrimKey(long resourcePrimKey) {
FinderPath finderPath = FINDER_PATH_COUNT_BY_RESOURCEPRIMKEY;
Object[] finderArgs = new Object[] { resourcePrimKey };
Long count = (Long)finderCache.getResult(finderPath, finderArgs, this);
if (count == null) {
StringBundler query = new StringBundler(2);
query.append(_SQL_COUNT_JOURNALARTICLE_WHERE);
query.append(_FINDER_COLUMN_RESOURCEPRIMKEY_RESOURCEPRIMKEY_2);
String sql = query.toString();
Session session = null;
try {
session = openSession();
Query q = session.createQuery(sql);
QueryPos qPos = QueryPos.getInstance(q);
qPos.add(resourcePrimKey);
count = (Long)q.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception e) {
finderCache.removeResult(finderPath, finderArgs);
throw processException(e);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String _FINDER_COLUMN_RESOURCEPRIMKEY_RESOURCEPRIMKEY_2 =
"journalArticle.resourcePrimKey = ?";
public static final FinderPath FINDER_PATH_WITH_PAGINATION_FIND_BY_GROUPID = new FinderPath(JournalArticleModelImpl.ENTITY_CACHE_ENABLED,
JournalArticleModelImpl.FINDER_CACHE_ENABLED,
JournalArticleImpl.class, FINDER_CLASS_NAME_LIST_WITH_PAGINATION,
"findByGroupId",
new String[] {
Long.class.getName(),
Integer.class.getName(), Integer.class.getName(),
OrderByComparator.class.getName()
});
public static final FinderPath FINDER_PATH_WITHOUT_PAGINATION_FIND_BY_GROUPID =
new FinderPath(JournalArticleModelImpl.ENTITY_CACHE_ENABLED,
JournalArticleModelImpl.FINDER_CACHE_ENABLED,
JournalArticleImpl.class,
FINDER_CLASS_NAME_LIST_WITHOUT_PAGINATION, "findByGroupId",
new String[] { Long.class.getName() },
JournalArticleModelImpl.GROUPID_COLUMN_BITMASK |
JournalArticleModelImpl.ARTICLEID_COLUMN_BITMASK |
JournalArticleModelImpl.VERSION_COLUMN_BITMASK);
public static final FinderPath FINDER_PATH_COUNT_BY_GROUPID = new FinderPath(JournalArticleModelImpl.ENTITY_CACHE_ENABLED,
JournalArticleModelImpl.FINDER_CACHE_ENABLED, Long.class,
FINDER_CLASS_NAME_LIST_WITHOUT_PAGINATION, "countByGroupId",
new String[] { Long.class.getName() });
/**
* Returns all the journal articles where groupId = ?.
*
* @param groupId the group ID
* @return the matching journal articles
*/
@Override
public List findByGroupId(long groupId) {
return findByGroupId(groupId, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the journal articles where groupId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to {@link QueryUtil#ALL_POS} will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent and pagination is required (start
and end
are not {@link QueryUtil#ALL_POS}), then the query will include the default ORDER BY logic from {@link JournalArticleModelImpl}. If both orderByComparator
and pagination are absent, for performance reasons, the query will not have an ORDER BY clause and the returned result set will be sorted on by the primary key in an ascending order.
*
*
* @param groupId the group ID
* @param start the lower bound of the range of journal articles
* @param end the upper bound of the range of journal articles (not inclusive)
* @return the range of matching journal articles
*/
@Override
public List findByGroupId(long groupId, int start, int end) {
return findByGroupId(groupId, start, end, null);
}
/**
* Returns an ordered range of all the journal articles where groupId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to {@link QueryUtil#ALL_POS} will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent and pagination is required (start
and end
are not {@link QueryUtil#ALL_POS}), then the query will include the default ORDER BY logic from {@link JournalArticleModelImpl}. If both orderByComparator
and pagination are absent, for performance reasons, the query will not have an ORDER BY clause and the returned result set will be sorted on by the primary key in an ascending order.
*
*
* @param groupId the group ID
* @param start the lower bound of the range of journal articles
* @param end the upper bound of the range of journal articles (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching journal articles
*/
@Override
public List findByGroupId(long groupId, int start, int end,
OrderByComparator orderByComparator) {
return findByGroupId(groupId, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the journal articles where groupId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to {@link QueryUtil#ALL_POS} will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent and pagination is required (start
and end
are not {@link QueryUtil#ALL_POS}), then the query will include the default ORDER BY logic from {@link JournalArticleModelImpl}. If both orderByComparator
and pagination are absent, for performance reasons, the query will not have an ORDER BY clause and the returned result set will be sorted on by the primary key in an ascending order.
*
*
* @param groupId the group ID
* @param start the lower bound of the range of journal articles
* @param end the upper bound of the range of journal articles (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param retrieveFromCache whether to retrieve from the finder cache
* @return the ordered range of matching journal articles
*/
@Override
public List findByGroupId(long groupId, int start, int end,
OrderByComparator orderByComparator,
boolean retrieveFromCache) {
boolean pagination = true;
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
pagination = false;
finderPath = FINDER_PATH_WITHOUT_PAGINATION_FIND_BY_GROUPID;
finderArgs = new Object[] { groupId };
}
else {
finderPath = FINDER_PATH_WITH_PAGINATION_FIND_BY_GROUPID;
finderArgs = new Object[] { groupId, start, end, orderByComparator };
}
List list = null;
if (retrieveFromCache) {
list = (List)finderCache.getResult(finderPath,
finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (JournalArticle journalArticle : list) {
if ((groupId != journalArticle.getGroupId())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler query = null;
if (orderByComparator != null) {
query = new StringBundler(3 +
(orderByComparator.getOrderByFields().length * 2));
}
else {
query = new StringBundler(3);
}
query.append(_SQL_SELECT_JOURNALARTICLE_WHERE);
query.append(_FINDER_COLUMN_GROUPID_GROUPID_2);
if (orderByComparator != null) {
appendOrderByComparator(query, _ORDER_BY_ENTITY_ALIAS,
orderByComparator);
}
else
if (pagination) {
query.append(JournalArticleModelImpl.ORDER_BY_JPQL);
}
String sql = query.toString();
Session session = null;
try {
session = openSession();
Query q = session.createQuery(sql);
QueryPos qPos = QueryPos.getInstance(q);
qPos.add(groupId);
if (!pagination) {
list = (List)QueryUtil.list(q,
getDialect(), start, end, false);
Collections.sort(list);
list = Collections.unmodifiableList(list);
}
else {
list = (List)QueryUtil.list(q,
getDialect(), start, end);
}
cacheResult(list);
finderCache.putResult(finderPath, finderArgs, list);
}
catch (Exception e) {
finderCache.removeResult(finderPath, finderArgs);
throw processException(e);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first journal article in the ordered set where groupId = ?.
*
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching journal article
* @throws NoSuchArticleException if a matching journal article could not be found
*/
@Override
public JournalArticle findByGroupId_First(long groupId,
OrderByComparator orderByComparator)
throws NoSuchArticleException {
JournalArticle journalArticle = fetchByGroupId_First(groupId,
orderByComparator);
if (journalArticle != null) {
return journalArticle;
}
StringBundler msg = new StringBundler(4);
msg.append(_NO_SUCH_ENTITY_WITH_KEY);
msg.append("groupId=");
msg.append(groupId);
msg.append(StringPool.CLOSE_CURLY_BRACE);
throw new NoSuchArticleException(msg.toString());
}
/**
* Returns the first journal article in the ordered set where groupId = ?.
*
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching journal article, or null
if a matching journal article could not be found
*/
@Override
public JournalArticle fetchByGroupId_First(long groupId,
OrderByComparator orderByComparator) {
List list = findByGroupId(groupId, 0, 1,
orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last journal article in the ordered set where groupId = ?.
*
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching journal article
* @throws NoSuchArticleException if a matching journal article could not be found
*/
@Override
public JournalArticle findByGroupId_Last(long groupId,
OrderByComparator orderByComparator)
throws NoSuchArticleException {
JournalArticle journalArticle = fetchByGroupId_Last(groupId,
orderByComparator);
if (journalArticle != null) {
return journalArticle;
}
StringBundler msg = new StringBundler(4);
msg.append(_NO_SUCH_ENTITY_WITH_KEY);
msg.append("groupId=");
msg.append(groupId);
msg.append(StringPool.CLOSE_CURLY_BRACE);
throw new NoSuchArticleException(msg.toString());
}
/**
* Returns the last journal article in the ordered set where groupId = ?.
*
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching journal article, or null
if a matching journal article could not be found
*/
@Override
public JournalArticle fetchByGroupId_Last(long groupId,
OrderByComparator orderByComparator) {
int count = countByGroupId(groupId);
if (count == 0) {
return null;
}
List list = findByGroupId(groupId, count - 1, count,
orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the journal articles before and after the current journal article in the ordered set where groupId = ?.
*
* @param id the primary key of the current journal article
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next journal article
* @throws NoSuchArticleException if a journal article with the primary key could not be found
*/
@Override
public JournalArticle[] findByGroupId_PrevAndNext(long id, long groupId,
OrderByComparator orderByComparator)
throws NoSuchArticleException {
JournalArticle journalArticle = findByPrimaryKey(id);
Session session = null;
try {
session = openSession();
JournalArticle[] array = new JournalArticleImpl[3];
array[0] = getByGroupId_PrevAndNext(session, journalArticle,
groupId, orderByComparator, true);
array[1] = journalArticle;
array[2] = getByGroupId_PrevAndNext(session, journalArticle,
groupId, orderByComparator, false);
return array;
}
catch (Exception e) {
throw processException(e);
}
finally {
closeSession(session);
}
}
protected JournalArticle getByGroupId_PrevAndNext(Session session,
JournalArticle journalArticle, long groupId,
OrderByComparator orderByComparator, boolean previous) {
StringBundler query = null;
if (orderByComparator != null) {
query = new StringBundler(4 +
(orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
query = new StringBundler(3);
}
query.append(_SQL_SELECT_JOURNALARTICLE_WHERE);
query.append(_FINDER_COLUMN_GROUPID_GROUPID_2);
if (orderByComparator != null) {
String[] orderByConditionFields = orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
query.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
query.append(_ORDER_BY_ENTITY_ALIAS);
query.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
query.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
query.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
query.append(WHERE_GREATER_THAN);
}
else {
query.append(WHERE_LESSER_THAN);
}
}
}
query.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
query.append(_ORDER_BY_ENTITY_ALIAS);
query.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
query.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
query.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
query.append(ORDER_BY_ASC);
}
else {
query.append(ORDER_BY_DESC);
}
}
}
}
else {
query.append(JournalArticleModelImpl.ORDER_BY_JPQL);
}
String sql = query.toString();
Query q = session.createQuery(sql);
q.setFirstResult(0);
q.setMaxResults(2);
QueryPos qPos = QueryPos.getInstance(q);
qPos.add(groupId);
if (orderByComparator != null) {
Object[] values = orderByComparator.getOrderByConditionValues(journalArticle);
for (Object value : values) {
qPos.add(value);
}
}
List list = q.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Returns all the journal articles that the user has permission to view where groupId = ?.
*
* @param groupId the group ID
* @return the matching journal articles that the user has permission to view
*/
@Override
public List filterFindByGroupId(long groupId) {
return filterFindByGroupId(groupId, QueryUtil.ALL_POS,
QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the journal articles that the user has permission to view where groupId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to {@link QueryUtil#ALL_POS} will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent and pagination is required (start
and end
are not {@link QueryUtil#ALL_POS}), then the query will include the default ORDER BY logic from {@link JournalArticleModelImpl}. If both orderByComparator
and pagination are absent, for performance reasons, the query will not have an ORDER BY clause and the returned result set will be sorted on by the primary key in an ascending order.
*
*
* @param groupId the group ID
* @param start the lower bound of the range of journal articles
* @param end the upper bound of the range of journal articles (not inclusive)
* @return the range of matching journal articles that the user has permission to view
*/
@Override
public List filterFindByGroupId(long groupId, int start,
int end) {
return filterFindByGroupId(groupId, start, end, null);
}
/**
* Returns an ordered range of all the journal articles that the user has permissions to view where groupId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to {@link QueryUtil#ALL_POS} will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent and pagination is required (start
and end
are not {@link QueryUtil#ALL_POS}), then the query will include the default ORDER BY logic from {@link JournalArticleModelImpl}. If both orderByComparator
and pagination are absent, for performance reasons, the query will not have an ORDER BY clause and the returned result set will be sorted on by the primary key in an ascending order.
*
*
* @param groupId the group ID
* @param start the lower bound of the range of journal articles
* @param end the upper bound of the range of journal articles (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching journal articles that the user has permission to view
*/
@Override
public List filterFindByGroupId(long groupId, int start,
int end, OrderByComparator orderByComparator) {
if (!InlineSQLHelperUtil.isEnabled(groupId)) {
return findByGroupId(groupId, start, end, orderByComparator);
}
StringBundler query = null;
if (orderByComparator != null) {
query = new StringBundler(3 +
(orderByComparator.getOrderByFields().length * 2));
}
else {
query = new StringBundler(4);
}
if (getDB().isSupportsInlineDistinct()) {
query.append(_FILTER_SQL_SELECT_JOURNALARTICLE_WHERE);
}
else {
query.append(_FILTER_SQL_SELECT_JOURNALARTICLE_NO_INLINE_DISTINCT_WHERE_1);
}
query.append(_FINDER_COLUMN_GROUPID_GROUPID_2);
if (!getDB().isSupportsInlineDistinct()) {
query.append(_FILTER_SQL_SELECT_JOURNALARTICLE_NO_INLINE_DISTINCT_WHERE_2);
}
if (orderByComparator != null) {
if (getDB().isSupportsInlineDistinct()) {
appendOrderByComparator(query, _ORDER_BY_ENTITY_ALIAS,
orderByComparator, true);
}
else {
appendOrderByComparator(query, _ORDER_BY_ENTITY_TABLE,
orderByComparator, true);
}
}
else {
if (getDB().isSupportsInlineDistinct()) {
query.append(JournalArticleModelImpl.ORDER_BY_JPQL);
}
else {
query.append(JournalArticleModelImpl.ORDER_BY_SQL);
}
}
String sql = InlineSQLHelperUtil.replacePermissionCheck(query.toString(),
JournalArticle.class.getName(),
_FILTER_ENTITY_TABLE_FILTER_PK_COLUMN, groupId);
Session session = null;
try {
session = openSession();
SQLQuery q = session.createSynchronizedSQLQuery(sql);
if (getDB().isSupportsInlineDistinct()) {
q.addEntity(_FILTER_ENTITY_ALIAS, JournalArticleImpl.class);
}
else {
q.addEntity(_FILTER_ENTITY_TABLE, JournalArticleImpl.class);
}
QueryPos qPos = QueryPos.getInstance(q);
qPos.add(groupId);
return (List)QueryUtil.list(q, getDialect(), start,
end);
}
catch (Exception e) {
throw processException(e);
}
finally {
closeSession(session);
}
}
/**
* Returns the journal articles before and after the current journal article in the ordered set of journal articles that the user has permission to view where groupId = ?.
*
* @param id the primary key of the current journal article
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next journal article
* @throws NoSuchArticleException if a journal article with the primary key could not be found
*/
@Override
public JournalArticle[] filterFindByGroupId_PrevAndNext(long id,
long groupId, OrderByComparator orderByComparator)
throws NoSuchArticleException {
if (!InlineSQLHelperUtil.isEnabled(groupId)) {
return findByGroupId_PrevAndNext(id, groupId, orderByComparator);
}
JournalArticle journalArticle = findByPrimaryKey(id);
Session session = null;
try {
session = openSession();
JournalArticle[] array = new JournalArticleImpl[3];
array[0] = filterGetByGroupId_PrevAndNext(session, journalArticle,
groupId, orderByComparator, true);
array[1] = journalArticle;
array[2] = filterGetByGroupId_PrevAndNext(session, journalArticle,
groupId, orderByComparator, false);
return array;
}
catch (Exception e) {
throw processException(e);
}
finally {
closeSession(session);
}
}
protected JournalArticle filterGetByGroupId_PrevAndNext(Session session,
JournalArticle journalArticle, long groupId,
OrderByComparator orderByComparator, boolean previous) {
StringBundler query = null;
if (orderByComparator != null) {
query = new StringBundler(5 +
(orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
query = new StringBundler(4);
}
if (getDB().isSupportsInlineDistinct()) {
query.append(_FILTER_SQL_SELECT_JOURNALARTICLE_WHERE);
}
else {
query.append(_FILTER_SQL_SELECT_JOURNALARTICLE_NO_INLINE_DISTINCT_WHERE_1);
}
query.append(_FINDER_COLUMN_GROUPID_GROUPID_2);
if (!getDB().isSupportsInlineDistinct()) {
query.append(_FILTER_SQL_SELECT_JOURNALARTICLE_NO_INLINE_DISTINCT_WHERE_2);
}
if (orderByComparator != null) {
String[] orderByConditionFields = orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
query.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
if (getDB().isSupportsInlineDistinct()) {
query.append(_ORDER_BY_ENTITY_ALIAS);
}
else {
query.append(_ORDER_BY_ENTITY_TABLE);
}
query.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
query.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
query.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
query.append(WHERE_GREATER_THAN);
}
else {
query.append(WHERE_LESSER_THAN);
}
}
}
query.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
if (getDB().isSupportsInlineDistinct()) {
query.append(_ORDER_BY_ENTITY_ALIAS);
}
else {
query.append(_ORDER_BY_ENTITY_TABLE);
}
query.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
query.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
query.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
query.append(ORDER_BY_ASC);
}
else {
query.append(ORDER_BY_DESC);
}
}
}
}
else {
if (getDB().isSupportsInlineDistinct()) {
query.append(JournalArticleModelImpl.ORDER_BY_JPQL);
}
else {
query.append(JournalArticleModelImpl.ORDER_BY_SQL);
}
}
String sql = InlineSQLHelperUtil.replacePermissionCheck(query.toString(),
JournalArticle.class.getName(),
_FILTER_ENTITY_TABLE_FILTER_PK_COLUMN, groupId);
SQLQuery q = session.createSynchronizedSQLQuery(sql);
q.setFirstResult(0);
q.setMaxResults(2);
if (getDB().isSupportsInlineDistinct()) {
q.addEntity(_FILTER_ENTITY_ALIAS, JournalArticleImpl.class);
}
else {
q.addEntity(_FILTER_ENTITY_TABLE, JournalArticleImpl.class);
}
QueryPos qPos = QueryPos.getInstance(q);
qPos.add(groupId);
if (orderByComparator != null) {
Object[] values = orderByComparator.getOrderByConditionValues(journalArticle);
for (Object value : values) {
qPos.add(value);
}
}
List list = q.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the journal articles where groupId = ? from the database.
*
* @param groupId the group ID
*/
@Override
public void removeByGroupId(long groupId) {
for (JournalArticle journalArticle : findByGroupId(groupId,
QueryUtil.ALL_POS, QueryUtil.ALL_POS, null)) {
remove(journalArticle);
}
}
/**
* Returns the number of journal articles where groupId = ?.
*
* @param groupId the group ID
* @return the number of matching journal articles
*/
@Override
public int countByGroupId(long groupId) {
FinderPath finderPath = FINDER_PATH_COUNT_BY_GROUPID;
Object[] finderArgs = new Object[] { groupId };
Long count = (Long)finderCache.getResult(finderPath, finderArgs, this);
if (count == null) {
StringBundler query = new StringBundler(2);
query.append(_SQL_COUNT_JOURNALARTICLE_WHERE);
query.append(_FINDER_COLUMN_GROUPID_GROUPID_2);
String sql = query.toString();
Session session = null;
try {
session = openSession();
Query q = session.createQuery(sql);
QueryPos qPos = QueryPos.getInstance(q);
qPos.add(groupId);
count = (Long)q.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception e) {
finderCache.removeResult(finderPath, finderArgs);
throw processException(e);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
/**
* Returns the number of journal articles that the user has permission to view where groupId = ?.
*
* @param groupId the group ID
* @return the number of matching journal articles that the user has permission to view
*/
@Override
public int filterCountByGroupId(long groupId) {
if (!InlineSQLHelperUtil.isEnabled(groupId)) {
return countByGroupId(groupId);
}
StringBundler query = new StringBundler(2);
query.append(_FILTER_SQL_COUNT_JOURNALARTICLE_WHERE);
query.append(_FINDER_COLUMN_GROUPID_GROUPID_2);
String sql = InlineSQLHelperUtil.replacePermissionCheck(query.toString(),
JournalArticle.class.getName(),
_FILTER_ENTITY_TABLE_FILTER_PK_COLUMN, groupId);
Session session = null;
try {
session = openSession();
SQLQuery q = session.createSynchronizedSQLQuery(sql);
q.addScalar(COUNT_COLUMN_NAME,
com.liferay.portal.kernel.dao.orm.Type.LONG);
QueryPos qPos = QueryPos.getInstance(q);
qPos.add(groupId);
Long count = (Long)q.uniqueResult();
return count.intValue();
}
catch (Exception e) {
throw processException(e);
}
finally {
closeSession(session);
}
}
private static final String _FINDER_COLUMN_GROUPID_GROUPID_2 = "journalArticle.groupId = ?";
public static final FinderPath FINDER_PATH_WITH_PAGINATION_FIND_BY_COMPANYID =
new FinderPath(JournalArticleModelImpl.ENTITY_CACHE_ENABLED,
JournalArticleModelImpl.FINDER_CACHE_ENABLED,
JournalArticleImpl.class, FINDER_CLASS_NAME_LIST_WITH_PAGINATION,
"findByCompanyId",
new String[] {
Long.class.getName(),
Integer.class.getName(), Integer.class.getName(),
OrderByComparator.class.getName()
});
public static final FinderPath FINDER_PATH_WITHOUT_PAGINATION_FIND_BY_COMPANYID =
new FinderPath(JournalArticleModelImpl.ENTITY_CACHE_ENABLED,
JournalArticleModelImpl.FINDER_CACHE_ENABLED,
JournalArticleImpl.class,
FINDER_CLASS_NAME_LIST_WITHOUT_PAGINATION, "findByCompanyId",
new String[] { Long.class.getName() },
JournalArticleModelImpl.COMPANYID_COLUMN_BITMASK |
JournalArticleModelImpl.ARTICLEID_COLUMN_BITMASK |
JournalArticleModelImpl.VERSION_COLUMN_BITMASK);
public static final FinderPath FINDER_PATH_COUNT_BY_COMPANYID = new FinderPath(JournalArticleModelImpl.ENTITY_CACHE_ENABLED,
JournalArticleModelImpl.FINDER_CACHE_ENABLED, Long.class,
FINDER_CLASS_NAME_LIST_WITHOUT_PAGINATION, "countByCompanyId",
new String[] { Long.class.getName() });
/**
* Returns all the journal articles where companyId = ?.
*
* @param companyId the company ID
* @return the matching journal articles
*/
@Override
public List findByCompanyId(long companyId) {
return findByCompanyId(companyId, QueryUtil.ALL_POS, QueryUtil.ALL_POS,
null);
}
/**
* Returns a range of all the journal articles where companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to {@link QueryUtil#ALL_POS} will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent and pagination is required (start
and end
are not {@link QueryUtil#ALL_POS}), then the query will include the default ORDER BY logic from {@link JournalArticleModelImpl}. If both orderByComparator
and pagination are absent, for performance reasons, the query will not have an ORDER BY clause and the returned result set will be sorted on by the primary key in an ascending order.
*
*
* @param companyId the company ID
* @param start the lower bound of the range of journal articles
* @param end the upper bound of the range of journal articles (not inclusive)
* @return the range of matching journal articles
*/
@Override
public List findByCompanyId(long companyId, int start,
int end) {
return findByCompanyId(companyId, start, end, null);
}
/**
* Returns an ordered range of all the journal articles where companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to {@link QueryUtil#ALL_POS} will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent and pagination is required (start
and end
are not {@link QueryUtil#ALL_POS}), then the query will include the default ORDER BY logic from {@link JournalArticleModelImpl}. If both orderByComparator
and pagination are absent, for performance reasons, the query will not have an ORDER BY clause and the returned result set will be sorted on by the primary key in an ascending order.
*
*
* @param companyId the company ID
* @param start the lower bound of the range of journal articles
* @param end the upper bound of the range of journal articles (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching journal articles
*/
@Override
public List findByCompanyId(long companyId, int start,
int end, OrderByComparator orderByComparator) {
return findByCompanyId(companyId, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the journal articles where companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to {@link QueryUtil#ALL_POS} will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent and pagination is required (start
and end
are not {@link QueryUtil#ALL_POS}), then the query will include the default ORDER BY logic from {@link JournalArticleModelImpl}. If both orderByComparator
and pagination are absent, for performance reasons, the query will not have an ORDER BY clause and the returned result set will be sorted on by the primary key in an ascending order.
*
*
* @param companyId the company ID
* @param start the lower bound of the range of journal articles
* @param end the upper bound of the range of journal articles (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param retrieveFromCache whether to retrieve from the finder cache
* @return the ordered range of matching journal articles
*/
@Override
public List findByCompanyId(long companyId, int start,
int end, OrderByComparator orderByComparator,
boolean retrieveFromCache) {
boolean pagination = true;
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
pagination = false;
finderPath = FINDER_PATH_WITHOUT_PAGINATION_FIND_BY_COMPANYID;
finderArgs = new Object[] { companyId };
}
else {
finderPath = FINDER_PATH_WITH_PAGINATION_FIND_BY_COMPANYID;
finderArgs = new Object[] { companyId, start, end, orderByComparator };
}
List list = null;
if (retrieveFromCache) {
list = (List)finderCache.getResult(finderPath,
finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (JournalArticle journalArticle : list) {
if ((companyId != journalArticle.getCompanyId())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler query = null;
if (orderByComparator != null) {
query = new StringBundler(3 +
(orderByComparator.getOrderByFields().length * 2));
}
else {
query = new StringBundler(3);
}
query.append(_SQL_SELECT_JOURNALARTICLE_WHERE);
query.append(_FINDER_COLUMN_COMPANYID_COMPANYID_2);
if (orderByComparator != null) {
appendOrderByComparator(query, _ORDER_BY_ENTITY_ALIAS,
orderByComparator);
}
else
if (pagination) {
query.append(JournalArticleModelImpl.ORDER_BY_JPQL);
}
String sql = query.toString();
Session session = null;
try {
session = openSession();
Query q = session.createQuery(sql);
QueryPos qPos = QueryPos.getInstance(q);
qPos.add(companyId);
if (!pagination) {
list = (List)QueryUtil.list(q,
getDialect(), start, end, false);
Collections.sort(list);
list = Collections.unmodifiableList(list);
}
else {
list = (List)QueryUtil.list(q,
getDialect(), start, end);
}
cacheResult(list);
finderCache.putResult(finderPath, finderArgs, list);
}
catch (Exception e) {
finderCache.removeResult(finderPath, finderArgs);
throw processException(e);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first journal article in the ordered set where companyId = ?.
*
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching journal article
* @throws NoSuchArticleException if a matching journal article could not be found
*/
@Override
public JournalArticle findByCompanyId_First(long companyId,
OrderByComparator orderByComparator)
throws NoSuchArticleException {
JournalArticle journalArticle = fetchByCompanyId_First(companyId,
orderByComparator);
if (journalArticle != null) {
return journalArticle;
}
StringBundler msg = new StringBundler(4);
msg.append(_NO_SUCH_ENTITY_WITH_KEY);
msg.append("companyId=");
msg.append(companyId);
msg.append(StringPool.CLOSE_CURLY_BRACE);
throw new NoSuchArticleException(msg.toString());
}
/**
* Returns the first journal article in the ordered set where companyId = ?.
*
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching journal article, or null
if a matching journal article could not be found
*/
@Override
public JournalArticle fetchByCompanyId_First(long companyId,
OrderByComparator orderByComparator) {
List list = findByCompanyId(companyId, 0, 1,
orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last journal article in the ordered set where companyId = ?.
*
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching journal article
* @throws NoSuchArticleException if a matching journal article could not be found
*/
@Override
public JournalArticle findByCompanyId_Last(long companyId,
OrderByComparator orderByComparator)
throws NoSuchArticleException {
JournalArticle journalArticle = fetchByCompanyId_Last(companyId,
orderByComparator);
if (journalArticle != null) {
return journalArticle;
}
StringBundler msg = new StringBundler(4);
msg.append(_NO_SUCH_ENTITY_WITH_KEY);
msg.append("companyId=");
msg.append(companyId);
msg.append(StringPool.CLOSE_CURLY_BRACE);
throw new NoSuchArticleException(msg.toString());
}
/**
* Returns the last journal article in the ordered set where companyId = ?.
*
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching journal article, or null
if a matching journal article could not be found
*/
@Override
public JournalArticle fetchByCompanyId_Last(long companyId,
OrderByComparator orderByComparator) {
int count = countByCompanyId(companyId);
if (count == 0) {
return null;
}
List list = findByCompanyId(companyId, count - 1,
count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the journal articles before and after the current journal article in the ordered set where companyId = ?.
*
* @param id the primary key of the current journal article
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next journal article
* @throws NoSuchArticleException if a journal article with the primary key could not be found
*/
@Override
public JournalArticle[] findByCompanyId_PrevAndNext(long id,
long companyId, OrderByComparator orderByComparator)
throws NoSuchArticleException {
JournalArticle journalArticle = findByPrimaryKey(id);
Session session = null;
try {
session = openSession();
JournalArticle[] array = new JournalArticleImpl[3];
array[0] = getByCompanyId_PrevAndNext(session, journalArticle,
companyId, orderByComparator, true);
array[1] = journalArticle;
array[2] = getByCompanyId_PrevAndNext(session, journalArticle,
companyId, orderByComparator, false);
return array;
}
catch (Exception e) {
throw processException(e);
}
finally {
closeSession(session);
}
}
protected JournalArticle getByCompanyId_PrevAndNext(Session session,
JournalArticle journalArticle, long companyId,
OrderByComparator orderByComparator, boolean previous) {
StringBundler query = null;
if (orderByComparator != null) {
query = new StringBundler(4 +
(orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
query = new StringBundler(3);
}
query.append(_SQL_SELECT_JOURNALARTICLE_WHERE);
query.append(_FINDER_COLUMN_COMPANYID_COMPANYID_2);
if (orderByComparator != null) {
String[] orderByConditionFields = orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
query.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
query.append(_ORDER_BY_ENTITY_ALIAS);
query.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
query.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
query.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
query.append(WHERE_GREATER_THAN);
}
else {
query.append(WHERE_LESSER_THAN);
}
}
}
query.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
query.append(_ORDER_BY_ENTITY_ALIAS);
query.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
query.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
query.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
query.append(ORDER_BY_ASC);
}
else {
query.append(ORDER_BY_DESC);
}
}
}
}
else {
query.append(JournalArticleModelImpl.ORDER_BY_JPQL);
}
String sql = query.toString();
Query q = session.createQuery(sql);
q.setFirstResult(0);
q.setMaxResults(2);
QueryPos qPos = QueryPos.getInstance(q);
qPos.add(companyId);
if (orderByComparator != null) {
Object[] values = orderByComparator.getOrderByConditionValues(journalArticle);
for (Object value : values) {
qPos.add(value);
}
}
List list = q.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the journal articles where companyId = ? from the database.
*
* @param companyId the company ID
*/
@Override
public void removeByCompanyId(long companyId) {
for (JournalArticle journalArticle : findByCompanyId(companyId,
QueryUtil.ALL_POS, QueryUtil.ALL_POS, null)) {
remove(journalArticle);
}
}
/**
* Returns the number of journal articles where companyId = ?.
*
* @param companyId the company ID
* @return the number of matching journal articles
*/
@Override
public int countByCompanyId(long companyId) {
FinderPath finderPath = FINDER_PATH_COUNT_BY_COMPANYID;
Object[] finderArgs = new Object[] { companyId };
Long count = (Long)finderCache.getResult(finderPath, finderArgs, this);
if (count == null) {
StringBundler query = new StringBundler(2);
query.append(_SQL_COUNT_JOURNALARTICLE_WHERE);
query.append(_FINDER_COLUMN_COMPANYID_COMPANYID_2);
String sql = query.toString();
Session session = null;
try {
session = openSession();
Query q = session.createQuery(sql);
QueryPos qPos = QueryPos.getInstance(q);
qPos.add(companyId);
count = (Long)q.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception e) {
finderCache.removeResult(finderPath, finderArgs);
throw processException(e);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String _FINDER_COLUMN_COMPANYID_COMPANYID_2 = "journalArticle.companyId = ?";
public static final FinderPath FINDER_PATH_WITH_PAGINATION_FIND_BY_DDMSTRUCTUREKEY =
new FinderPath(JournalArticleModelImpl.ENTITY_CACHE_ENABLED,
JournalArticleModelImpl.FINDER_CACHE_ENABLED,
JournalArticleImpl.class, FINDER_CLASS_NAME_LIST_WITH_PAGINATION,
"findByDDMStructureKey",
new String[] {
String.class.getName(),
Integer.class.getName(), Integer.class.getName(),
OrderByComparator.class.getName()
});
public static final FinderPath FINDER_PATH_WITHOUT_PAGINATION_FIND_BY_DDMSTRUCTUREKEY =
new FinderPath(JournalArticleModelImpl.ENTITY_CACHE_ENABLED,
JournalArticleModelImpl.FINDER_CACHE_ENABLED,
JournalArticleImpl.class,
FINDER_CLASS_NAME_LIST_WITHOUT_PAGINATION, "findByDDMStructureKey",
new String[] { String.class.getName() },
JournalArticleModelImpl.DDMSTRUCTUREKEY_COLUMN_BITMASK |
JournalArticleModelImpl.ARTICLEID_COLUMN_BITMASK |
JournalArticleModelImpl.VERSION_COLUMN_BITMASK);
public static final FinderPath FINDER_PATH_COUNT_BY_DDMSTRUCTUREKEY = new FinderPath(JournalArticleModelImpl.ENTITY_CACHE_ENABLED,
JournalArticleModelImpl.FINDER_CACHE_ENABLED, Long.class,
FINDER_CLASS_NAME_LIST_WITHOUT_PAGINATION,
"countByDDMStructureKey", new String[] { String.class.getName() });
public static final FinderPath FINDER_PATH_WITH_PAGINATION_COUNT_BY_DDMSTRUCTUREKEY =
new FinderPath(JournalArticleModelImpl.ENTITY_CACHE_ENABLED,
JournalArticleModelImpl.FINDER_CACHE_ENABLED, Long.class,
FINDER_CLASS_NAME_LIST_WITH_PAGINATION, "countByDDMStructureKey",
new String[] { String.class.getName() });
/**
* Returns all the journal articles where DDMStructureKey = ?.
*
* @param DDMStructureKey the d d m structure key
* @return the matching journal articles
*/
@Override
public List findByDDMStructureKey(String DDMStructureKey) {
return findByDDMStructureKey(DDMStructureKey, QueryUtil.ALL_POS,
QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the journal articles where DDMStructureKey = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to {@link QueryUtil#ALL_POS} will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent and pagination is required (start
and end
are not {@link QueryUtil#ALL_POS}), then the query will include the default ORDER BY logic from {@link JournalArticleModelImpl}. If both orderByComparator
and pagination are absent, for performance reasons, the query will not have an ORDER BY clause and the returned result set will be sorted on by the primary key in an ascending order.
*
*
* @param DDMStructureKey the d d m structure key
* @param start the lower bound of the range of journal articles
* @param end the upper bound of the range of journal articles (not inclusive)
* @return the range of matching journal articles
*/
@Override
public List findByDDMStructureKey(String DDMStructureKey,
int start, int end) {
return findByDDMStructureKey(DDMStructureKey, start, end, null);
}
/**
* Returns an ordered range of all the journal articles where DDMStructureKey = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to {@link QueryUtil#ALL_POS} will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent and pagination is required (start
and end
are not {@link QueryUtil#ALL_POS}), then the query will include the default ORDER BY logic from {@link JournalArticleModelImpl}. If both orderByComparator
and pagination are absent, for performance reasons, the query will not have an ORDER BY clause and the returned result set will be sorted on by the primary key in an ascending order.
*
*
* @param DDMStructureKey the d d m structure key
* @param start the lower bound of the range of journal articles
* @param end the upper bound of the range of journal articles (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching journal articles
*/
@Override
public List findByDDMStructureKey(String DDMStructureKey,
int start, int end, OrderByComparator orderByComparator) {
return findByDDMStructureKey(DDMStructureKey, start, end,
orderByComparator, true);
}
/**
* Returns an ordered range of all the journal articles where DDMStructureKey = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to {@link QueryUtil#ALL_POS} will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent and pagination is required (start
and end
are not {@link QueryUtil#ALL_POS}), then the query will include the default ORDER BY logic from {@link JournalArticleModelImpl}. If both orderByComparator
and pagination are absent, for performance reasons, the query will not have an ORDER BY clause and the returned result set will be sorted on by the primary key in an ascending order.
*
*
* @param DDMStructureKey the d d m structure key
* @param start the lower bound of the range of journal articles
* @param end the upper bound of the range of journal articles (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param retrieveFromCache whether to retrieve from the finder cache
* @return the ordered range of matching journal articles
*/
@Override
public List findByDDMStructureKey(String DDMStructureKey,
int start, int end,
OrderByComparator orderByComparator,
boolean retrieveFromCache) {
boolean pagination = true;
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
pagination = false;
finderPath = FINDER_PATH_WITHOUT_PAGINATION_FIND_BY_DDMSTRUCTUREKEY;
finderArgs = new Object[] { DDMStructureKey };
}
else {
finderPath = FINDER_PATH_WITH_PAGINATION_FIND_BY_DDMSTRUCTUREKEY;
finderArgs = new Object[] {
DDMStructureKey,
start, end, orderByComparator
};
}
List list = null;
if (retrieveFromCache) {
list = (List)finderCache.getResult(finderPath,
finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (JournalArticle journalArticle : list) {
if (!Objects.equals(DDMStructureKey,
journalArticle.getDDMStructureKey())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler query = null;
if (orderByComparator != null) {
query = new StringBundler(3 +
(orderByComparator.getOrderByFields().length * 2));
}
else {
query = new StringBundler(3);
}
query.append(_SQL_SELECT_JOURNALARTICLE_WHERE);
boolean bindDDMStructureKey = false;
if (DDMStructureKey == null) {
query.append(_FINDER_COLUMN_DDMSTRUCTUREKEY_DDMSTRUCTUREKEY_1);
}
else if (DDMStructureKey.equals(StringPool.BLANK)) {
query.append(_FINDER_COLUMN_DDMSTRUCTUREKEY_DDMSTRUCTUREKEY_3);
}
else {
bindDDMStructureKey = true;
query.append(_FINDER_COLUMN_DDMSTRUCTUREKEY_DDMSTRUCTUREKEY_2);
}
if (orderByComparator != null) {
appendOrderByComparator(query, _ORDER_BY_ENTITY_ALIAS,
orderByComparator);
}
else
if (pagination) {
query.append(JournalArticleModelImpl.ORDER_BY_JPQL);
}
String sql = query.toString();
Session session = null;
try {
session = openSession();
Query q = session.createQuery(sql);
QueryPos qPos = QueryPos.getInstance(q);
if (bindDDMStructureKey) {
qPos.add(DDMStructureKey);
}
if (!pagination) {
list = (List)QueryUtil.list(q,
getDialect(), start, end, false);
Collections.sort(list);
list = Collections.unmodifiableList(list);
}
else {
list = (List)QueryUtil.list(q,
getDialect(), start, end);
}
cacheResult(list);
finderCache.putResult(finderPath, finderArgs, list);
}
catch (Exception e) {
finderCache.removeResult(finderPath, finderArgs);
throw processException(e);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first journal article in the ordered set where DDMStructureKey = ?.
*
* @param DDMStructureKey the d d m structure key
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching journal article
* @throws NoSuchArticleException if a matching journal article could not be found
*/
@Override
public JournalArticle findByDDMStructureKey_First(String DDMStructureKey,
OrderByComparator orderByComparator)
throws NoSuchArticleException {
JournalArticle journalArticle = fetchByDDMStructureKey_First(DDMStructureKey,
orderByComparator);
if (journalArticle != null) {
return journalArticle;
}
StringBundler msg = new StringBundler(4);
msg.append(_NO_SUCH_ENTITY_WITH_KEY);
msg.append("DDMStructureKey=");
msg.append(DDMStructureKey);
msg.append(StringPool.CLOSE_CURLY_BRACE);
throw new NoSuchArticleException(msg.toString());
}
/**
* Returns the first journal article in the ordered set where DDMStructureKey = ?.
*
* @param DDMStructureKey the d d m structure key
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching journal article, or null
if a matching journal article could not be found
*/
@Override
public JournalArticle fetchByDDMStructureKey_First(String DDMStructureKey,
OrderByComparator orderByComparator) {
List list = findByDDMStructureKey(DDMStructureKey, 0,
1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last journal article in the ordered set where DDMStructureKey = ?.
*
* @param DDMStructureKey the d d m structure key
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching journal article
* @throws NoSuchArticleException if a matching journal article could not be found
*/
@Override
public JournalArticle findByDDMStructureKey_Last(String DDMStructureKey,
OrderByComparator orderByComparator)
throws NoSuchArticleException {
JournalArticle journalArticle = fetchByDDMStructureKey_Last(DDMStructureKey,
orderByComparator);
if (journalArticle != null) {
return journalArticle;
}
StringBundler msg = new StringBundler(4);
msg.append(_NO_SUCH_ENTITY_WITH_KEY);
msg.append("DDMStructureKey=");
msg.append(DDMStructureKey);
msg.append(StringPool.CLOSE_CURLY_BRACE);
throw new NoSuchArticleException(msg.toString());
}
/**
* Returns the last journal article in the ordered set where DDMStructureKey = ?.
*
* @param DDMStructureKey the d d m structure key
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching journal article, or null
if a matching journal article could not be found
*/
@Override
public JournalArticle fetchByDDMStructureKey_Last(String DDMStructureKey,
OrderByComparator orderByComparator) {
int count = countByDDMStructureKey(DDMStructureKey);
if (count == 0) {
return null;
}
List list = findByDDMStructureKey(DDMStructureKey,
count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the journal articles before and after the current journal article in the ordered set where DDMStructureKey = ?.
*
* @param id the primary key of the current journal article
* @param DDMStructureKey the d d m structure key
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next journal article
* @throws NoSuchArticleException if a journal article with the primary key could not be found
*/
@Override
public JournalArticle[] findByDDMStructureKey_PrevAndNext(long id,
String DDMStructureKey,
OrderByComparator orderByComparator)
throws NoSuchArticleException {
JournalArticle journalArticle = findByPrimaryKey(id);
Session session = null;
try {
session = openSession();
JournalArticle[] array = new JournalArticleImpl[3];
array[0] = getByDDMStructureKey_PrevAndNext(session,
journalArticle, DDMStructureKey, orderByComparator, true);
array[1] = journalArticle;
array[2] = getByDDMStructureKey_PrevAndNext(session,
journalArticle, DDMStructureKey, orderByComparator, false);
return array;
}
catch (Exception e) {
throw processException(e);
}
finally {
closeSession(session);
}
}
protected JournalArticle getByDDMStructureKey_PrevAndNext(Session session,
JournalArticle journalArticle, String DDMStructureKey,
OrderByComparator orderByComparator, boolean previous) {
StringBundler query = null;
if (orderByComparator != null) {
query = new StringBundler(4 +
(orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
query = new StringBundler(3);
}
query.append(_SQL_SELECT_JOURNALARTICLE_WHERE);
boolean bindDDMStructureKey = false;
if (DDMStructureKey == null) {
query.append(_FINDER_COLUMN_DDMSTRUCTUREKEY_DDMSTRUCTUREKEY_1);
}
else if (DDMStructureKey.equals(StringPool.BLANK)) {
query.append(_FINDER_COLUMN_DDMSTRUCTUREKEY_DDMSTRUCTUREKEY_3);
}
else {
bindDDMStructureKey = true;
query.append(_FINDER_COLUMN_DDMSTRUCTUREKEY_DDMSTRUCTUREKEY_2);
}
if (orderByComparator != null) {
String[] orderByConditionFields = orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
query.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
query.append(_ORDER_BY_ENTITY_ALIAS);
query.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
query.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
query.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
query.append(WHERE_GREATER_THAN);
}
else {
query.append(WHERE_LESSER_THAN);
}
}
}
query.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
query.append(_ORDER_BY_ENTITY_ALIAS);
query.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
query.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
query.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
query.append(ORDER_BY_ASC);
}
else {
query.append(ORDER_BY_DESC);
}
}
}
}
else {
query.append(JournalArticleModelImpl.ORDER_BY_JPQL);
}
String sql = query.toString();
Query q = session.createQuery(sql);
q.setFirstResult(0);
q.setMaxResults(2);
QueryPos qPos = QueryPos.getInstance(q);
if (bindDDMStructureKey) {
qPos.add(DDMStructureKey);
}
if (orderByComparator != null) {
Object[] values = orderByComparator.getOrderByConditionValues(journalArticle);
for (Object value : values) {
qPos.add(value);
}
}
List list = q.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Returns all the journal articles where DDMStructureKey = any ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to {@link QueryUtil#ALL_POS} will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent and pagination is required (start
and end
are not {@link QueryUtil#ALL_POS}), then the query will include the default ORDER BY logic from {@link JournalArticleModelImpl}. If both orderByComparator
and pagination are absent, for performance reasons, the query will not have an ORDER BY clause and the returned result set will be sorted on by the primary key in an ascending order.
*
*
* @param DDMStructureKeies the d d m structure keies
* @return the matching journal articles
*/
@Override
public List findByDDMStructureKey(
String[] DDMStructureKeies) {
return findByDDMStructureKey(DDMStructureKeies, QueryUtil.ALL_POS,
QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the journal articles where DDMStructureKey = any ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to {@link QueryUtil#ALL_POS} will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent and pagination is required (start
and end
are not {@link QueryUtil#ALL_POS}), then the query will include the default ORDER BY logic from {@link JournalArticleModelImpl}. If both orderByComparator
and pagination are absent, for performance reasons, the query will not have an ORDER BY clause and the returned result set will be sorted on by the primary key in an ascending order.
*
*
* @param DDMStructureKeies the d d m structure keies
* @param start the lower bound of the range of journal articles
* @param end the upper bound of the range of journal articles (not inclusive)
* @return the range of matching journal articles
*/
@Override
public List findByDDMStructureKey(
String[] DDMStructureKeies, int start, int end) {
return findByDDMStructureKey(DDMStructureKeies, start, end, null);
}
/**
* Returns an ordered range of all the journal articles where DDMStructureKey = any ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to {@link QueryUtil#ALL_POS} will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent and pagination is required (start
and end
are not {@link QueryUtil#ALL_POS}), then the query will include the default ORDER BY logic from {@link JournalArticleModelImpl}. If both orderByComparator
and pagination are absent, for performance reasons, the query will not have an ORDER BY clause and the returned result set will be sorted on by the primary key in an ascending order.
*
*
* @param DDMStructureKeies the d d m structure keies
* @param start the lower bound of the range of journal articles
* @param end the upper bound of the range of journal articles (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching journal articles
*/
@Override
public List findByDDMStructureKey(
String[] DDMStructureKeies, int start, int end,
OrderByComparator orderByComparator) {
return findByDDMStructureKey(DDMStructureKeies, start, end,
orderByComparator, true);
}
/**
* Returns an ordered range of all the journal articles where DDMStructureKey = ?, optionally using the finder cache.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to {@link QueryUtil#ALL_POS} will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent and pagination is required (start
and end
are not {@link QueryUtil#ALL_POS}), then the query will include the default ORDER BY logic from {@link JournalArticleModelImpl}. If both orderByComparator
and pagination are absent, for performance reasons, the query will not have an ORDER BY clause and the returned result set will be sorted on by the primary key in an ascending order.
*
*
* @param DDMStructureKey the d d m structure key
* @param start the lower bound of the range of journal articles
* @param end the upper bound of the range of journal articles (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param retrieveFromCache whether to retrieve from the finder cache
* @return the ordered range of matching journal articles
*/
@Override
public List findByDDMStructureKey(
String[] DDMStructureKeies, int start, int end,
OrderByComparator orderByComparator,
boolean retrieveFromCache) {
if (DDMStructureKeies == null) {
DDMStructureKeies = new String[0];
}
else if (DDMStructureKeies.length > 1) {
DDMStructureKeies = ArrayUtil.distinct(DDMStructureKeies,
NULL_SAFE_STRING_COMPARATOR);
Arrays.sort(DDMStructureKeies, NULL_SAFE_STRING_COMPARATOR);
}
if (DDMStructureKeies.length == 1) {
return findByDDMStructureKey(DDMStructureKeies[0], start, end,
orderByComparator);
}
boolean pagination = true;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
pagination = false;
finderArgs = new Object[] { StringUtil.merge(DDMStructureKeies) };
}
else {
finderArgs = new Object[] {
StringUtil.merge(DDMStructureKeies),
start, end, orderByComparator
};
}
List list = null;
if (retrieveFromCache) {
list = (List)finderCache.getResult(FINDER_PATH_WITH_PAGINATION_FIND_BY_DDMSTRUCTUREKEY,
finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (JournalArticle journalArticle : list) {
if (!ArrayUtil.contains(DDMStructureKeies,
journalArticle.getDDMStructureKey())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler query = new StringBundler();
query.append(_SQL_SELECT_JOURNALARTICLE_WHERE);
if (DDMStructureKeies.length > 0) {
query.append(StringPool.OPEN_PARENTHESIS);
for (int i = 0; i < DDMStructureKeies.length; i++) {
String DDMStructureKey = DDMStructureKeies[i];
if (DDMStructureKey == null) {
query.append(_FINDER_COLUMN_DDMSTRUCTUREKEY_DDMSTRUCTUREKEY_1);
}
else if (DDMStructureKey.equals(StringPool.BLANK)) {
query.append(_FINDER_COLUMN_DDMSTRUCTUREKEY_DDMSTRUCTUREKEY_3);
}
else {
query.append(_FINDER_COLUMN_DDMSTRUCTUREKEY_DDMSTRUCTUREKEY_2);
}
if ((i + 1) < DDMStructureKeies.length) {
query.append(WHERE_OR);
}
}
query.append(StringPool.CLOSE_PARENTHESIS);
}
query.setStringAt(removeConjunction(query.stringAt(query.index() -
1)), query.index() - 1);
if (orderByComparator != null) {
appendOrderByComparator(query, _ORDER_BY_ENTITY_ALIAS,
orderByComparator);
}
else
if (pagination) {
query.append(JournalArticleModelImpl.ORDER_BY_JPQL);
}
String sql = query.toString();
Session session = null;
try {
session = openSession();
Query q = session.createQuery(sql);
QueryPos qPos = QueryPos.getInstance(q);
for (String DDMStructureKey : DDMStructureKeies) {
if ((DDMStructureKey != null) &&
!DDMStructureKey.isEmpty()) {
qPos.add(DDMStructureKey);
}
}
if (!pagination) {
list = (List)QueryUtil.list(q,
getDialect(), start, end, false);
Collections.sort(list);
list = Collections.unmodifiableList(list);
}
else {
list = (List)QueryUtil.list(q,
getDialect(), start, end);
}
cacheResult(list);
finderCache.putResult(FINDER_PATH_WITH_PAGINATION_FIND_BY_DDMSTRUCTUREKEY,
finderArgs, list);
}
catch (Exception e) {
finderCache.removeResult(FINDER_PATH_WITH_PAGINATION_FIND_BY_DDMSTRUCTUREKEY,
finderArgs);
throw processException(e);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Removes all the journal articles where DDMStructureKey = ? from the database.
*
* @param DDMStructureKey the d d m structure key
*/
@Override
public void removeByDDMStructureKey(String DDMStructureKey) {
for (JournalArticle journalArticle : findByDDMStructureKey(
DDMStructureKey, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null)) {
remove(journalArticle);
}
}
/**
* Returns the number of journal articles where DDMStructureKey = ?.
*
* @param DDMStructureKey the d d m structure key
* @return the number of matching journal articles
*/
@Override
public int countByDDMStructureKey(String DDMStructureKey) {
FinderPath finderPath = FINDER_PATH_COUNT_BY_DDMSTRUCTUREKEY;
Object[] finderArgs = new Object[] { DDMStructureKey };
Long count = (Long)finderCache.getResult(finderPath, finderArgs, this);
if (count == null) {
StringBundler query = new StringBundler(2);
query.append(_SQL_COUNT_JOURNALARTICLE_WHERE);
boolean bindDDMStructureKey = false;
if (DDMStructureKey == null) {
query.append(_FINDER_COLUMN_DDMSTRUCTUREKEY_DDMSTRUCTUREKEY_1);
}
else if (DDMStructureKey.equals(StringPool.BLANK)) {
query.append(_FINDER_COLUMN_DDMSTRUCTUREKEY_DDMSTRUCTUREKEY_3);
}
else {
bindDDMStructureKey = true;
query.append(_FINDER_COLUMN_DDMSTRUCTUREKEY_DDMSTRUCTUREKEY_2);
}
String sql = query.toString();
Session session = null;
try {
session = openSession();
Query q = session.createQuery(sql);
QueryPos qPos = QueryPos.getInstance(q);
if (bindDDMStructureKey) {
qPos.add(DDMStructureKey);
}
count = (Long)q.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception e) {
finderCache.removeResult(finderPath, finderArgs);
throw processException(e);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
/**
* Returns the number of journal articles where DDMStructureKey = any ?.
*
* @param DDMStructureKeies the d d m structure keies
* @return the number of matching journal articles
*/
@Override
public int countByDDMStructureKey(String[] DDMStructureKeies) {
if (DDMStructureKeies == null) {
DDMStructureKeies = new String[0];
}
else if (DDMStructureKeies.length > 1) {
DDMStructureKeies = ArrayUtil.distinct(DDMStructureKeies,
NULL_SAFE_STRING_COMPARATOR);
Arrays.sort(DDMStructureKeies, NULL_SAFE_STRING_COMPARATOR);
}
Object[] finderArgs = new Object[] { StringUtil.merge(DDMStructureKeies) };
Long count = (Long)finderCache.getResult(FINDER_PATH_WITH_PAGINATION_COUNT_BY_DDMSTRUCTUREKEY,
finderArgs, this);
if (count == null) {
StringBundler query = new StringBundler();
query.append(_SQL_COUNT_JOURNALARTICLE_WHERE);
if (DDMStructureKeies.length > 0) {
query.append(StringPool.OPEN_PARENTHESIS);
for (int i = 0; i < DDMStructureKeies.length; i++) {
String DDMStructureKey = DDMStructureKeies[i];
if (DDMStructureKey == null) {
query.append(_FINDER_COLUMN_DDMSTRUCTUREKEY_DDMSTRUCTUREKEY_1);
}
else if (DDMStructureKey.equals(StringPool.BLANK)) {
query.append(_FINDER_COLUMN_DDMSTRUCTUREKEY_DDMSTRUCTUREKEY_3);
}
else {
query.append(_FINDER_COLUMN_DDMSTRUCTUREKEY_DDMSTRUCTUREKEY_2);
}
if ((i + 1) < DDMStructureKeies.length) {
query.append(WHERE_OR);
}
}
query.append(StringPool.CLOSE_PARENTHESIS);
}
query.setStringAt(removeConjunction(query.stringAt(query.index() -
1)), query.index() - 1);
String sql = query.toString();
Session session = null;
try {
session = openSession();
Query q = session.createQuery(sql);
QueryPos qPos = QueryPos.getInstance(q);
for (String DDMStructureKey : DDMStructureKeies) {
if ((DDMStructureKey != null) &&
!DDMStructureKey.isEmpty()) {
qPos.add(DDMStructureKey);
}
}
count = (Long)q.uniqueResult();
finderCache.putResult(FINDER_PATH_WITH_PAGINATION_COUNT_BY_DDMSTRUCTUREKEY,
finderArgs, count);
}
catch (Exception e) {
finderCache.removeResult(FINDER_PATH_WITH_PAGINATION_COUNT_BY_DDMSTRUCTUREKEY,
finderArgs);
throw processException(e);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String _FINDER_COLUMN_DDMSTRUCTUREKEY_DDMSTRUCTUREKEY_1 =
"journalArticle.DDMStructureKey IS NULL";
private static final String _FINDER_COLUMN_DDMSTRUCTUREKEY_DDMSTRUCTUREKEY_2 =
"journalArticle.DDMStructureKey = ?";
private static final String _FINDER_COLUMN_DDMSTRUCTUREKEY_DDMSTRUCTUREKEY_3 =
"(journalArticle.DDMStructureKey IS NULL OR journalArticle.DDMStructureKey = '')";
public static final FinderPath FINDER_PATH_WITH_PAGINATION_FIND_BY_DDMTEMPLATEKEY =
new FinderPath(JournalArticleModelImpl.ENTITY_CACHE_ENABLED,
JournalArticleModelImpl.FINDER_CACHE_ENABLED,
JournalArticleImpl.class, FINDER_CLASS_NAME_LIST_WITH_PAGINATION,
"findByDDMTemplateKey",
new String[] {
String.class.getName(),
Integer.class.getName(), Integer.class.getName(),
OrderByComparator.class.getName()
});
public static final FinderPath FINDER_PATH_WITHOUT_PAGINATION_FIND_BY_DDMTEMPLATEKEY =
new FinderPath(JournalArticleModelImpl.ENTITY_CACHE_ENABLED,
JournalArticleModelImpl.FINDER_CACHE_ENABLED,
JournalArticleImpl.class,
FINDER_CLASS_NAME_LIST_WITHOUT_PAGINATION, "findByDDMTemplateKey",
new String[] { String.class.getName() },
JournalArticleModelImpl.DDMTEMPLATEKEY_COLUMN_BITMASK |
JournalArticleModelImpl.ARTICLEID_COLUMN_BITMASK |
JournalArticleModelImpl.VERSION_COLUMN_BITMASK);
public static final FinderPath FINDER_PATH_COUNT_BY_DDMTEMPLATEKEY = new FinderPath(JournalArticleModelImpl.ENTITY_CACHE_ENABLED,
JournalArticleModelImpl.FINDER_CACHE_ENABLED, Long.class,
FINDER_CLASS_NAME_LIST_WITHOUT_PAGINATION, "countByDDMTemplateKey",
new String[] { String.class.getName() });
/**
* Returns all the journal articles where DDMTemplateKey = ?.
*
* @param DDMTemplateKey the d d m template key
* @return the matching journal articles
*/
@Override
public List findByDDMTemplateKey(String DDMTemplateKey) {
return findByDDMTemplateKey(DDMTemplateKey, QueryUtil.ALL_POS,
QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the journal articles where DDMTemplateKey = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to {@link QueryUtil#ALL_POS} will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent and pagination is required (start
and end
are not {@link QueryUtil#ALL_POS}), then the query will include the default ORDER BY logic from {@link JournalArticleModelImpl}. If both orderByComparator
and pagination are absent, for performance reasons, the query will not have an ORDER BY clause and the returned result set will be sorted on by the primary key in an ascending order.
*
*
* @param DDMTemplateKey the d d m template key
* @param start the lower bound of the range of journal articles
* @param end the upper bound of the range of journal articles (not inclusive)
* @return the range of matching journal articles
*/
@Override
public List findByDDMTemplateKey(String DDMTemplateKey,
int start, int end) {
return findByDDMTemplateKey(DDMTemplateKey, start, end, null);
}
/**
* Returns an ordered range of all the journal articles where DDMTemplateKey = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to {@link QueryUtil#ALL_POS} will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent and pagination is required (start
and end
are not {@link QueryUtil#ALL_POS}), then the query will include the default ORDER BY logic from {@link JournalArticleModelImpl}. If both orderByComparator
and pagination are absent, for performance reasons, the query will not have an ORDER BY clause and the returned result set will be sorted on by the primary key in an ascending order.
*
*
* @param DDMTemplateKey the d d m template key
* @param start the lower bound of the range of journal articles
* @param end the upper bound of the range of journal articles (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching journal articles
*/
@Override
public List findByDDMTemplateKey(String DDMTemplateKey,
int start, int end, OrderByComparator orderByComparator) {
return findByDDMTemplateKey(DDMTemplateKey, start, end,
orderByComparator, true);
}
/**
* Returns an ordered range of all the journal articles where DDMTemplateKey = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to {@link QueryUtil#ALL_POS} will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent and pagination is required (start
and end
are not {@link QueryUtil#ALL_POS}), then the query will include the default ORDER BY logic from {@link JournalArticleModelImpl}. If both orderByComparator
and pagination are absent, for performance reasons, the query will not have an ORDER BY clause and the returned result set will be sorted on by the primary key in an ascending order.
*
*
* @param DDMTemplateKey the d d m template key
* @param start the lower bound of the range of journal articles
* @param end the upper bound of the range of journal articles (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param retrieveFromCache whether to retrieve from the finder cache
* @return the ordered range of matching journal articles
*/
@Override
public List findByDDMTemplateKey(String DDMTemplateKey,
int start, int end,
OrderByComparator orderByComparator,
boolean retrieveFromCache) {
boolean pagination = true;
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
pagination = false;
finderPath = FINDER_PATH_WITHOUT_PAGINATION_FIND_BY_DDMTEMPLATEKEY;
finderArgs = new Object[] { DDMTemplateKey };
}
else {
finderPath = FINDER_PATH_WITH_PAGINATION_FIND_BY_DDMTEMPLATEKEY;
finderArgs = new Object[] {
DDMTemplateKey,
start, end, orderByComparator
};
}
List list = null;
if (retrieveFromCache) {
list = (List)finderCache.getResult(finderPath,
finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (JournalArticle journalArticle : list) {
if (!Objects.equals(DDMTemplateKey,
journalArticle.getDDMTemplateKey())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler query = null;
if (orderByComparator != null) {
query = new StringBundler(3 +
(orderByComparator.getOrderByFields().length * 2));
}
else {
query = new StringBundler(3);
}
query.append(_SQL_SELECT_JOURNALARTICLE_WHERE);
boolean bindDDMTemplateKey = false;
if (DDMTemplateKey == null) {
query.append(_FINDER_COLUMN_DDMTEMPLATEKEY_DDMTEMPLATEKEY_1);
}
else if (DDMTemplateKey.equals(StringPool.BLANK)) {
query.append(_FINDER_COLUMN_DDMTEMPLATEKEY_DDMTEMPLATEKEY_3);
}
else {
bindDDMTemplateKey = true;
query.append(_FINDER_COLUMN_DDMTEMPLATEKEY_DDMTEMPLATEKEY_2);
}
if (orderByComparator != null) {
appendOrderByComparator(query, _ORDER_BY_ENTITY_ALIAS,
orderByComparator);
}
else
if (pagination) {
query.append(JournalArticleModelImpl.ORDER_BY_JPQL);
}
String sql = query.toString();
Session session = null;
try {
session = openSession();
Query q = session.createQuery(sql);
QueryPos qPos = QueryPos.getInstance(q);
if (bindDDMTemplateKey) {
qPos.add(DDMTemplateKey);
}
if (!pagination) {
list = (List)QueryUtil.list(q,
getDialect(), start, end, false);
Collections.sort(list);
list = Collections.unmodifiableList(list);
}
else {
list = (List)QueryUtil.list(q,
getDialect(), start, end);
}
cacheResult(list);
finderCache.putResult(finderPath, finderArgs, list);
}
catch (Exception e) {
finderCache.removeResult(finderPath, finderArgs);
throw processException(e);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first journal article in the ordered set where DDMTemplateKey = ?.
*
* @param DDMTemplateKey the d d m template key
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching journal article
* @throws NoSuchArticleException if a matching journal article could not be found
*/
@Override
public JournalArticle findByDDMTemplateKey_First(String DDMTemplateKey,
OrderByComparator orderByComparator)
throws NoSuchArticleException {
JournalArticle journalArticle = fetchByDDMTemplateKey_First(DDMTemplateKey,
orderByComparator);
if (journalArticle != null) {
return journalArticle;
}
StringBundler msg = new StringBundler(4);
msg.append(_NO_SUCH_ENTITY_WITH_KEY);
msg.append("DDMTemplateKey=");
msg.append(DDMTemplateKey);
msg.append(StringPool.CLOSE_CURLY_BRACE);
throw new NoSuchArticleException(msg.toString());
}
/**
* Returns the first journal article in the ordered set where DDMTemplateKey = ?.
*
* @param DDMTemplateKey the d d m template key
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching journal article, or null
if a matching journal article could not be found
*/
@Override
public JournalArticle fetchByDDMTemplateKey_First(String DDMTemplateKey,
OrderByComparator orderByComparator) {
List list = findByDDMTemplateKey(DDMTemplateKey, 0, 1,
orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last journal article in the ordered set where DDMTemplateKey = ?.
*
* @param DDMTemplateKey the d d m template key
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching journal article
* @throws NoSuchArticleException if a matching journal article could not be found
*/
@Override
public JournalArticle findByDDMTemplateKey_Last(String DDMTemplateKey,
OrderByComparator orderByComparator)
throws NoSuchArticleException {
JournalArticle journalArticle = fetchByDDMTemplateKey_Last(DDMTemplateKey,
orderByComparator);
if (journalArticle != null) {
return journalArticle;
}
StringBundler msg = new StringBundler(4);
msg.append(_NO_SUCH_ENTITY_WITH_KEY);
msg.append("DDMTemplateKey=");
msg.append(DDMTemplateKey);
msg.append(StringPool.CLOSE_CURLY_BRACE);
throw new NoSuchArticleException(msg.toString());
}
/**
* Returns the last journal article in the ordered set where DDMTemplateKey = ?.
*
* @param DDMTemplateKey the d d m template key
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching journal article, or null
if a matching journal article could not be found
*/
@Override
public JournalArticle fetchByDDMTemplateKey_Last(String DDMTemplateKey,
OrderByComparator orderByComparator) {
int count = countByDDMTemplateKey(DDMTemplateKey);
if (count == 0) {
return null;
}
List list = findByDDMTemplateKey(DDMTemplateKey,
count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the journal articles before and after the current journal article in the ordered set where DDMTemplateKey = ?.
*
* @param id the primary key of the current journal article
* @param DDMTemplateKey the d d m template key
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next journal article
* @throws NoSuchArticleException if a journal article with the primary key could not be found
*/
@Override
public JournalArticle[] findByDDMTemplateKey_PrevAndNext(long id,
String DDMTemplateKey,
OrderByComparator orderByComparator)
throws NoSuchArticleException {
JournalArticle journalArticle = findByPrimaryKey(id);
Session session = null;
try {
session = openSession();
JournalArticle[] array = new JournalArticleImpl[3];
array[0] = getByDDMTemplateKey_PrevAndNext(session, journalArticle,
DDMTemplateKey, orderByComparator, true);
array[1] = journalArticle;
array[2] = getByDDMTemplateKey_PrevAndNext(session, journalArticle,
DDMTemplateKey, orderByComparator, false);
return array;
}
catch (Exception e) {
throw processException(e);
}
finally {
closeSession(session);
}
}
protected JournalArticle getByDDMTemplateKey_PrevAndNext(Session session,
JournalArticle journalArticle, String DDMTemplateKey,
OrderByComparator orderByComparator, boolean previous) {
StringBundler query = null;
if (orderByComparator != null) {
query = new StringBundler(4 +
(orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
query = new StringBundler(3);
}
query.append(_SQL_SELECT_JOURNALARTICLE_WHERE);
boolean bindDDMTemplateKey = false;
if (DDMTemplateKey == null) {
query.append(_FINDER_COLUMN_DDMTEMPLATEKEY_DDMTEMPLATEKEY_1);
}
else if (DDMTemplateKey.equals(StringPool.BLANK)) {
query.append(_FINDER_COLUMN_DDMTEMPLATEKEY_DDMTEMPLATEKEY_3);
}
else {
bindDDMTemplateKey = true;
query.append(_FINDER_COLUMN_DDMTEMPLATEKEY_DDMTEMPLATEKEY_2);
}
if (orderByComparator != null) {
String[] orderByConditionFields = orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
query.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
query.append(_ORDER_BY_ENTITY_ALIAS);
query.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
query.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
query.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
query.append(WHERE_GREATER_THAN);
}
else {
query.append(WHERE_LESSER_THAN);
}
}
}
query.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
query.append(_ORDER_BY_ENTITY_ALIAS);
query.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
query.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
query.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
query.append(ORDER_BY_ASC);
}
else {
query.append(ORDER_BY_DESC);
}
}
}
}
else {
query.append(JournalArticleModelImpl.ORDER_BY_JPQL);
}
String sql = query.toString();
Query q = session.createQuery(sql);
q.setFirstResult(0);
q.setMaxResults(2);
QueryPos qPos = QueryPos.getInstance(q);
if (bindDDMTemplateKey) {
qPos.add(DDMTemplateKey);
}
if (orderByComparator != null) {
Object[] values = orderByComparator.getOrderByConditionValues(journalArticle);
for (Object value : values) {
qPos.add(value);
}
}
List list = q.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the journal articles where DDMTemplateKey = ? from the database.
*
* @param DDMTemplateKey the d d m template key
*/
@Override
public void removeByDDMTemplateKey(String DDMTemplateKey) {
for (JournalArticle journalArticle : findByDDMTemplateKey(
DDMTemplateKey, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null)) {
remove(journalArticle);
}
}
/**
* Returns the number of journal articles where DDMTemplateKey = ?.
*
* @param DDMTemplateKey the d d m template key
* @return the number of matching journal articles
*/
@Override
public int countByDDMTemplateKey(String DDMTemplateKey) {
FinderPath finderPath = FINDER_PATH_COUNT_BY_DDMTEMPLATEKEY;
Object[] finderArgs = new Object[] { DDMTemplateKey };
Long count = (Long)finderCache.getResult(finderPath, finderArgs, this);
if (count == null) {
StringBundler query = new StringBundler(2);
query.append(_SQL_COUNT_JOURNALARTICLE_WHERE);
boolean bindDDMTemplateKey = false;
if (DDMTemplateKey == null) {
query.append(_FINDER_COLUMN_DDMTEMPLATEKEY_DDMTEMPLATEKEY_1);
}
else if (DDMTemplateKey.equals(StringPool.BLANK)) {
query.append(_FINDER_COLUMN_DDMTEMPLATEKEY_DDMTEMPLATEKEY_3);
}
else {
bindDDMTemplateKey = true;
query.append(_FINDER_COLUMN_DDMTEMPLATEKEY_DDMTEMPLATEKEY_2);
}
String sql = query.toString();
Session session = null;
try {
session = openSession();
Query q = session.createQuery(sql);
QueryPos qPos = QueryPos.getInstance(q);
if (bindDDMTemplateKey) {
qPos.add(DDMTemplateKey);
}
count = (Long)q.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception e) {
finderCache.removeResult(finderPath, finderArgs);
throw processException(e);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String _FINDER_COLUMN_DDMTEMPLATEKEY_DDMTEMPLATEKEY_1 = "journalArticle.DDMTemplateKey IS NULL";
private static final String _FINDER_COLUMN_DDMTEMPLATEKEY_DDMTEMPLATEKEY_2 = "journalArticle.DDMTemplateKey = ?";
private static final String _FINDER_COLUMN_DDMTEMPLATEKEY_DDMTEMPLATEKEY_3 = "(journalArticle.DDMTemplateKey IS NULL OR journalArticle.DDMTemplateKey = '')";
public static final FinderPath FINDER_PATH_WITH_PAGINATION_FIND_BY_LAYOUTUUID =
new FinderPath(JournalArticleModelImpl.ENTITY_CACHE_ENABLED,
JournalArticleModelImpl.FINDER_CACHE_ENABLED,
JournalArticleImpl.class, FINDER_CLASS_NAME_LIST_WITH_PAGINATION,
"findByLayoutUuid",
new String[] {
String.class.getName(),
Integer.class.getName(), Integer.class.getName(),
OrderByComparator.class.getName()
});
public static final FinderPath FINDER_PATH_WITHOUT_PAGINATION_FIND_BY_LAYOUTUUID =
new FinderPath(JournalArticleModelImpl.ENTITY_CACHE_ENABLED,
JournalArticleModelImpl.FINDER_CACHE_ENABLED,
JournalArticleImpl.class,
FINDER_CLASS_NAME_LIST_WITHOUT_PAGINATION, "findByLayoutUuid",
new String[] { String.class.getName() },
JournalArticleModelImpl.LAYOUTUUID_COLUMN_BITMASK |
JournalArticleModelImpl.ARTICLEID_COLUMN_BITMASK |
JournalArticleModelImpl.VERSION_COLUMN_BITMASK);
public static final FinderPath FINDER_PATH_COUNT_BY_LAYOUTUUID = new FinderPath(JournalArticleModelImpl.ENTITY_CACHE_ENABLED,
JournalArticleModelImpl.FINDER_CACHE_ENABLED, Long.class,
FINDER_CLASS_NAME_LIST_WITHOUT_PAGINATION, "countByLayoutUuid",
new String[] { String.class.getName() });
/**
* Returns all the journal articles where layoutUuid = ?.
*
* @param layoutUuid the layout uuid
* @return the matching journal articles
*/
@Override
public List findByLayoutUuid(String layoutUuid) {
return findByLayoutUuid(layoutUuid, QueryUtil.ALL_POS,
QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the journal articles where layoutUuid = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to {@link QueryUtil#ALL_POS} will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent and pagination is required (start
and end
are not {@link QueryUtil#ALL_POS}), then the query will include the default ORDER BY logic from {@link JournalArticleModelImpl}. If both orderByComparator
and pagination are absent, for performance reasons, the query will not have an ORDER BY clause and the returned result set will be sorted on by the primary key in an ascending order.
*
*
* @param layoutUuid the layout uuid
* @param start the lower bound of the range of journal articles
* @param end the upper bound of the range of journal articles (not inclusive)
* @return the range of matching journal articles
*/
@Override
public List findByLayoutUuid(String layoutUuid, int start,
int end) {
return findByLayoutUuid(layoutUuid, start, end, null);
}
/**
* Returns an ordered range of all the journal articles where layoutUuid = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to {@link QueryUtil#ALL_POS} will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent and pagination is required (start
and end
are not {@link QueryUtil#ALL_POS}), then the query will include the default ORDER BY logic from {@link JournalArticleModelImpl}. If both orderByComparator
and pagination are absent, for performance reasons, the query will not have an ORDER BY clause and the returned result set will be sorted on by the primary key in an ascending order.
*
*
* @param layoutUuid the layout uuid
* @param start the lower bound of the range of journal articles
* @param end the upper bound of the range of journal articles (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching journal articles
*/
@Override
public List findByLayoutUuid(String layoutUuid, int start,
int end, OrderByComparator orderByComparator) {
return findByLayoutUuid(layoutUuid, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the journal articles where layoutUuid = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to {@link QueryUtil#ALL_POS} will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent and pagination is required (start
and end
are not {@link QueryUtil#ALL_POS}), then the query will include the default ORDER BY logic from {@link JournalArticleModelImpl}. If both orderByComparator
and pagination are absent, for performance reasons, the query will not have an ORDER BY clause and the returned result set will be sorted on by the primary key in an ascending order.
*
*
* @param layoutUuid the layout uuid
* @param start the lower bound of the range of journal articles
* @param end the upper bound of the range of journal articles (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param retrieveFromCache whether to retrieve from the finder cache
* @return the ordered range of matching journal articles
*/
@Override
public List findByLayoutUuid(String layoutUuid, int start,
int end, OrderByComparator orderByComparator,
boolean retrieveFromCache) {
boolean pagination = true;
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
pagination = false;
finderPath = FINDER_PATH_WITHOUT_PAGINATION_FIND_BY_LAYOUTUUID;
finderArgs = new Object[] { layoutUuid };
}
else {
finderPath = FINDER_PATH_WITH_PAGINATION_FIND_BY_LAYOUTUUID;
finderArgs = new Object[] { layoutUuid, start, end, orderByComparator };
}
List list = null;
if (retrieveFromCache) {
list = (List)finderCache.getResult(finderPath,
finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (JournalArticle journalArticle : list) {
if (!Objects.equals(layoutUuid,
journalArticle.getLayoutUuid())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler query = null;
if (orderByComparator != null) {
query = new StringBundler(3 +
(orderByComparator.getOrderByFields().length * 2));
}
else {
query = new StringBundler(3);
}
query.append(_SQL_SELECT_JOURNALARTICLE_WHERE);
boolean bindLayoutUuid = false;
if (layoutUuid == null) {
query.append(_FINDER_COLUMN_LAYOUTUUID_LAYOUTUUID_1);
}
else if (layoutUuid.equals(StringPool.BLANK)) {
query.append(_FINDER_COLUMN_LAYOUTUUID_LAYOUTUUID_3);
}
else {
bindLayoutUuid = true;
query.append(_FINDER_COLUMN_LAYOUTUUID_LAYOUTUUID_2);
}
if (orderByComparator != null) {
appendOrderByComparator(query, _ORDER_BY_ENTITY_ALIAS,
orderByComparator);
}
else
if (pagination) {
query.append(JournalArticleModelImpl.ORDER_BY_JPQL);
}
String sql = query.toString();
Session session = null;
try {
session = openSession();
Query q = session.createQuery(sql);
QueryPos qPos = QueryPos.getInstance(q);
if (bindLayoutUuid) {
qPos.add(layoutUuid);
}
if (!pagination) {
list = (List)QueryUtil.list(q,
getDialect(), start, end, false);
Collections.sort(list);
list = Collections.unmodifiableList(list);
}
else {
list = (List)QueryUtil.list(q,
getDialect(), start, end);
}
cacheResult(list);
finderCache.putResult(finderPath, finderArgs, list);
}
catch (Exception e) {
finderCache.removeResult(finderPath, finderArgs);
throw processException(e);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first journal article in the ordered set where layoutUuid = ?.
*
* @param layoutUuid the layout uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching journal article
* @throws NoSuchArticleException if a matching journal article could not be found
*/
@Override
public JournalArticle findByLayoutUuid_First(String layoutUuid,
OrderByComparator orderByComparator)
throws NoSuchArticleException {
JournalArticle journalArticle = fetchByLayoutUuid_First(layoutUuid,
orderByComparator);
if (journalArticle != null) {
return journalArticle;
}
StringBundler msg = new StringBundler(4);
msg.append(_NO_SUCH_ENTITY_WITH_KEY);
msg.append("layoutUuid=");
msg.append(layoutUuid);
msg.append(StringPool.CLOSE_CURLY_BRACE);
throw new NoSuchArticleException(msg.toString());
}
/**
* Returns the first journal article in the ordered set where layoutUuid = ?.
*
* @param layoutUuid the layout uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching journal article, or null
if a matching journal article could not be found
*/
@Override
public JournalArticle fetchByLayoutUuid_First(String layoutUuid,
OrderByComparator orderByComparator) {
List list = findByLayoutUuid(layoutUuid, 0, 1,
orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last journal article in the ordered set where layoutUuid = ?.
*
* @param layoutUuid the layout uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching journal article
* @throws NoSuchArticleException if a matching journal article could not be found
*/
@Override
public JournalArticle findByLayoutUuid_Last(String layoutUuid,
OrderByComparator orderByComparator)
throws NoSuchArticleException {
JournalArticle journalArticle = fetchByLayoutUuid_Last(layoutUuid,
orderByComparator);
if (journalArticle != null) {
return journalArticle;
}
StringBundler msg = new StringBundler(4);
msg.append(_NO_SUCH_ENTITY_WITH_KEY);
msg.append("layoutUuid=");
msg.append(layoutUuid);
msg.append(StringPool.CLOSE_CURLY_BRACE);
throw new NoSuchArticleException(msg.toString());
}
/**
* Returns the last journal article in the ordered set where layoutUuid = ?.
*
* @param layoutUuid the layout uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching journal article, or null
if a matching journal article could not be found
*/
@Override
public JournalArticle fetchByLayoutUuid_Last(String layoutUuid,
OrderByComparator orderByComparator) {
int count = countByLayoutUuid(layoutUuid);
if (count == 0) {
return null;
}
List list = findByLayoutUuid(layoutUuid, count - 1,
count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the journal articles before and after the current journal article in the ordered set where layoutUuid = ?.
*
* @param id the primary key of the current journal article
* @param layoutUuid the layout uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next journal article
* @throws NoSuchArticleException if a journal article with the primary key could not be found
*/
@Override
public JournalArticle[] findByLayoutUuid_PrevAndNext(long id,
String layoutUuid, OrderByComparator orderByComparator)
throws NoSuchArticleException {
JournalArticle journalArticle = findByPrimaryKey(id);
Session session = null;
try {
session = openSession();
JournalArticle[] array = new JournalArticleImpl[3];
array[0] = getByLayoutUuid_PrevAndNext(session, journalArticle,
layoutUuid, orderByComparator, true);
array[1] = journalArticle;
array[2] = getByLayoutUuid_PrevAndNext(session, journalArticle,
layoutUuid, orderByComparator, false);
return array;
}
catch (Exception e) {
throw processException(e);
}
finally {
closeSession(session);
}
}
protected JournalArticle getByLayoutUuid_PrevAndNext(Session session,
JournalArticle journalArticle, String layoutUuid,
OrderByComparator orderByComparator, boolean previous) {
StringBundler query = null;
if (orderByComparator != null) {
query = new StringBundler(4 +
(orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
query = new StringBundler(3);
}
query.append(_SQL_SELECT_JOURNALARTICLE_WHERE);
boolean bindLayoutUuid = false;
if (layoutUuid == null) {
query.append(_FINDER_COLUMN_LAYOUTUUID_LAYOUTUUID_1);
}
else if (layoutUuid.equals(StringPool.BLANK)) {
query.append(_FINDER_COLUMN_LAYOUTUUID_LAYOUTUUID_3);
}
else {
bindLayoutUuid = true;
query.append(_FINDER_COLUMN_LAYOUTUUID_LAYOUTUUID_2);
}
if (orderByComparator != null) {
String[] orderByConditionFields = orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
query.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
query.append(_ORDER_BY_ENTITY_ALIAS);
query.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
query.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
query.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
query.append(WHERE_GREATER_THAN);
}
else {
query.append(WHERE_LESSER_THAN);
}
}
}
query.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
query.append(_ORDER_BY_ENTITY_ALIAS);
query.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
query.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
query.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
query.append(ORDER_BY_ASC);
}
else {
query.append(ORDER_BY_DESC);
}
}
}
}
else {
query.append(JournalArticleModelImpl.ORDER_BY_JPQL);
}
String sql = query.toString();
Query q = session.createQuery(sql);
q.setFirstResult(0);
q.setMaxResults(2);
QueryPos qPos = QueryPos.getInstance(q);
if (bindLayoutUuid) {
qPos.add(layoutUuid);
}
if (orderByComparator != null) {
Object[] values = orderByComparator.getOrderByConditionValues(journalArticle);
for (Object value : values) {
qPos.add(value);
}
}
List list = q.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the journal articles where layoutUuid = ? from the database.
*
* @param layoutUuid the layout uuid
*/
@Override
public void removeByLayoutUuid(String layoutUuid) {
for (JournalArticle journalArticle : findByLayoutUuid(layoutUuid,
QueryUtil.ALL_POS, QueryUtil.ALL_POS, null)) {
remove(journalArticle);
}
}
/**
* Returns the number of journal articles where layoutUuid = ?.
*
* @param layoutUuid the layout uuid
* @return the number of matching journal articles
*/
@Override
public int countByLayoutUuid(String layoutUuid) {
FinderPath finderPath = FINDER_PATH_COUNT_BY_LAYOUTUUID;
Object[] finderArgs = new Object[] { layoutUuid };
Long count = (Long)finderCache.getResult(finderPath, finderArgs, this);
if (count == null) {
StringBundler query = new StringBundler(2);
query.append(_SQL_COUNT_JOURNALARTICLE_WHERE);
boolean bindLayoutUuid = false;
if (layoutUuid == null) {
query.append(_FINDER_COLUMN_LAYOUTUUID_LAYOUTUUID_1);
}
else if (layoutUuid.equals(StringPool.BLANK)) {
query.append(_FINDER_COLUMN_LAYOUTUUID_LAYOUTUUID_3);
}
else {
bindLayoutUuid = true;
query.append(_FINDER_COLUMN_LAYOUTUUID_LAYOUTUUID_2);
}
String sql = query.toString();
Session session = null;
try {
session = openSession();
Query q = session.createQuery(sql);
QueryPos qPos = QueryPos.getInstance(q);
if (bindLayoutUuid) {
qPos.add(layoutUuid);
}
count = (Long)q.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception e) {
finderCache.removeResult(finderPath, finderArgs);
throw processException(e);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String _FINDER_COLUMN_LAYOUTUUID_LAYOUTUUID_1 = "journalArticle.layoutUuid IS NULL";
private static final String _FINDER_COLUMN_LAYOUTUUID_LAYOUTUUID_2 = "journalArticle.layoutUuid = ?";
private static final String _FINDER_COLUMN_LAYOUTUUID_LAYOUTUUID_3 = "(journalArticle.layoutUuid IS NULL OR journalArticle.layoutUuid = '')";
public static final FinderPath FINDER_PATH_WITH_PAGINATION_FIND_BY_SMALLIMAGEID =
new FinderPath(JournalArticleModelImpl.ENTITY_CACHE_ENABLED,
JournalArticleModelImpl.FINDER_CACHE_ENABLED,
JournalArticleImpl.class, FINDER_CLASS_NAME_LIST_WITH_PAGINATION,
"findBySmallImageId",
new String[] {
Long.class.getName(),
Integer.class.getName(), Integer.class.getName(),
OrderByComparator.class.getName()
});
public static final FinderPath FINDER_PATH_WITHOUT_PAGINATION_FIND_BY_SMALLIMAGEID =
new FinderPath(JournalArticleModelImpl.ENTITY_CACHE_ENABLED,
JournalArticleModelImpl.FINDER_CACHE_ENABLED,
JournalArticleImpl.class,
FINDER_CLASS_NAME_LIST_WITHOUT_PAGINATION, "findBySmallImageId",
new String[] { Long.class.getName() },
JournalArticleModelImpl.SMALLIMAGEID_COLUMN_BITMASK |
JournalArticleModelImpl.ARTICLEID_COLUMN_BITMASK |
JournalArticleModelImpl.VERSION_COLUMN_BITMASK);
public static final FinderPath FINDER_PATH_COUNT_BY_SMALLIMAGEID = new FinderPath(JournalArticleModelImpl.ENTITY_CACHE_ENABLED,
JournalArticleModelImpl.FINDER_CACHE_ENABLED, Long.class,
FINDER_CLASS_NAME_LIST_WITHOUT_PAGINATION, "countBySmallImageId",
new String[] { Long.class.getName() });
/**
* Returns all the journal articles where smallImageId = ?.
*
* @param smallImageId the small image ID
* @return the matching journal articles
*/
@Override
public List findBySmallImageId(long smallImageId) {
return findBySmallImageId(smallImageId, QueryUtil.ALL_POS,
QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the journal articles where smallImageId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to {@link QueryUtil#ALL_POS} will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent and pagination is required (start
and end
are not {@link QueryUtil#ALL_POS}), then the query will include the default ORDER BY logic from {@link JournalArticleModelImpl}. If both orderByComparator
and pagination are absent, for performance reasons, the query will not have an ORDER BY clause and the returned result set will be sorted on by the primary key in an ascending order.
*
*
* @param smallImageId the small image ID
* @param start the lower bound of the range of journal articles
* @param end the upper bound of the range of journal articles (not inclusive)
* @return the range of matching journal articles
*/
@Override
public List findBySmallImageId(long smallImageId,
int start, int end) {
return findBySmallImageId(smallImageId, start, end, null);
}
/**
* Returns an ordered range of all the journal articles where smallImageId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to {@link QueryUtil#ALL_POS} will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent and pagination is required (start
and end
are not {@link QueryUtil#ALL_POS}), then the query will include the default ORDER BY logic from {@link JournalArticleModelImpl}. If both orderByComparator
and pagination are absent, for performance reasons, the query will not have an ORDER BY clause and the returned result set will be sorted on by the primary key in an ascending order.
*
*
* @param smallImageId the small image ID
* @param start the lower bound of the range of journal articles
* @param end the upper bound of the range of journal articles (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching journal articles
*/
@Override
public List findBySmallImageId(long smallImageId,
int start, int end, OrderByComparator orderByComparator) {
return findBySmallImageId(smallImageId, start, end, orderByComparator,
true);
}
/**
* Returns an ordered range of all the journal articles where smallImageId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to {@link QueryUtil#ALL_POS} will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent and pagination is required (start
and end
are not {@link QueryUtil#ALL_POS}), then the query will include the default ORDER BY logic from {@link JournalArticleModelImpl}. If both orderByComparator
and pagination are absent, for performance reasons, the query will not have an ORDER BY clause and the returned result set will be sorted on by the primary key in an ascending order.
*
*
* @param smallImageId the small image ID
* @param start the lower bound of the range of journal articles
* @param end the upper bound of the range of journal articles (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param retrieveFromCache whether to retrieve from the finder cache
* @return the ordered range of matching journal articles
*/
@Override
public List findBySmallImageId(long smallImageId,
int start, int end,
OrderByComparator orderByComparator,
boolean retrieveFromCache) {
boolean pagination = true;
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
pagination = false;
finderPath = FINDER_PATH_WITHOUT_PAGINATION_FIND_BY_SMALLIMAGEID;
finderArgs = new Object[] { smallImageId };
}
else {
finderPath = FINDER_PATH_WITH_PAGINATION_FIND_BY_SMALLIMAGEID;
finderArgs = new Object[] {
smallImageId,
start, end, orderByComparator
};
}
List list = null;
if (retrieveFromCache) {
list = (List)finderCache.getResult(finderPath,
finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (JournalArticle journalArticle : list) {
if ((smallImageId != journalArticle.getSmallImageId())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler query = null;
if (orderByComparator != null) {
query = new StringBundler(3 +
(orderByComparator.getOrderByFields().length * 2));
}
else {
query = new StringBundler(3);
}
query.append(_SQL_SELECT_JOURNALARTICLE_WHERE);
query.append(_FINDER_COLUMN_SMALLIMAGEID_SMALLIMAGEID_2);
if (orderByComparator != null) {
appendOrderByComparator(query, _ORDER_BY_ENTITY_ALIAS,
orderByComparator);
}
else
if (pagination) {
query.append(JournalArticleModelImpl.ORDER_BY_JPQL);
}
String sql = query.toString();
Session session = null;
try {
session = openSession();
Query q = session.createQuery(sql);
QueryPos qPos = QueryPos.getInstance(q);
qPos.add(smallImageId);
if (!pagination) {
list = (List)QueryUtil.list(q,
getDialect(), start, end, false);
Collections.sort(list);
list = Collections.unmodifiableList(list);
}
else {
list = (List)QueryUtil.list(q,
getDialect(), start, end);
}
cacheResult(list);
finderCache.putResult(finderPath, finderArgs, list);
}
catch (Exception e) {
finderCache.removeResult(finderPath, finderArgs);
throw processException(e);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first journal article in the ordered set where smallImageId = ?.
*
* @param smallImageId the small image ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching journal article
* @throws NoSuchArticleException if a matching journal article could not be found
*/
@Override
public JournalArticle findBySmallImageId_First(long smallImageId,
OrderByComparator orderByComparator)
throws NoSuchArticleException {
JournalArticle journalArticle = fetchBySmallImageId_First(smallImageId,
orderByComparator);
if (journalArticle != null) {
return journalArticle;
}
StringBundler msg = new StringBundler(4);
msg.append(_NO_SUCH_ENTITY_WITH_KEY);
msg.append("smallImageId=");
msg.append(smallImageId);
msg.append(StringPool.CLOSE_CURLY_BRACE);
throw new NoSuchArticleException(msg.toString());
}
/**
* Returns the first journal article in the ordered set where smallImageId = ?.
*
* @param smallImageId the small image ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching journal article, or null
if a matching journal article could not be found
*/
@Override
public JournalArticle fetchBySmallImageId_First(long smallImageId,
OrderByComparator orderByComparator) {
List list = findBySmallImageId(smallImageId, 0, 1,
orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last journal article in the ordered set where smallImageId = ?.
*
* @param smallImageId the small image ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching journal article
* @throws NoSuchArticleException if a matching journal article could not be found
*/
@Override
public JournalArticle findBySmallImageId_Last(long smallImageId,
OrderByComparator orderByComparator)
throws NoSuchArticleException {
JournalArticle journalArticle = fetchBySmallImageId_Last(smallImageId,
orderByComparator);
if (journalArticle != null) {
return journalArticle;
}
StringBundler msg = new StringBundler(4);
msg.append(_NO_SUCH_ENTITY_WITH_KEY);
msg.append("smallImageId=");
msg.append(smallImageId);
msg.append(StringPool.CLOSE_CURLY_BRACE);
throw new NoSuchArticleException(msg.toString());
}
/**
* Returns the last journal article in the ordered set where smallImageId = ?.
*
* @param smallImageId the small image ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching journal article, or null
if a matching journal article could not be found
*/
@Override
public JournalArticle fetchBySmallImageId_Last(long smallImageId,
OrderByComparator orderByComparator) {
int count = countBySmallImageId(smallImageId);
if (count == 0) {
return null;
}
List list = findBySmallImageId(smallImageId, count - 1,
count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the journal articles before and after the current journal article in the ordered set where smallImageId = ?.
*
* @param id the primary key of the current journal article
* @param smallImageId the small image ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next journal article
* @throws NoSuchArticleException if a journal article with the primary key could not be found
*/
@Override
public JournalArticle[] findBySmallImageId_PrevAndNext(long id,
long smallImageId, OrderByComparator orderByComparator)
throws NoSuchArticleException {
JournalArticle journalArticle = findByPrimaryKey(id);
Session session = null;
try {
session = openSession();
JournalArticle[] array = new JournalArticleImpl[3];
array[0] = getBySmallImageId_PrevAndNext(session, journalArticle,
smallImageId, orderByComparator, true);
array[1] = journalArticle;
array[2] = getBySmallImageId_PrevAndNext(session, journalArticle,
smallImageId, orderByComparator, false);
return array;
}
catch (Exception e) {
throw processException(e);
}
finally {
closeSession(session);
}
}
protected JournalArticle getBySmallImageId_PrevAndNext(Session session,
JournalArticle journalArticle, long smallImageId,
OrderByComparator orderByComparator, boolean previous) {
StringBundler query = null;
if (orderByComparator != null) {
query = new StringBundler(4 +
(orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
query = new StringBundler(3);
}
query.append(_SQL_SELECT_JOURNALARTICLE_WHERE);
query.append(_FINDER_COLUMN_SMALLIMAGEID_SMALLIMAGEID_2);
if (orderByComparator != null) {
String[] orderByConditionFields = orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
query.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
query.append(_ORDER_BY_ENTITY_ALIAS);
query.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
query.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
query.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
query.append(WHERE_GREATER_THAN);
}
else {
query.append(WHERE_LESSER_THAN);
}
}
}
query.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
query.append(_ORDER_BY_ENTITY_ALIAS);
query.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
query.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
query.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
query.append(ORDER_BY_ASC);
}
else {
query.append(ORDER_BY_DESC);
}
}
}
}
else {
query.append(JournalArticleModelImpl.ORDER_BY_JPQL);
}
String sql = query.toString();
Query q = session.createQuery(sql);
q.setFirstResult(0);
q.setMaxResults(2);
QueryPos qPos = QueryPos.getInstance(q);
qPos.add(smallImageId);
if (orderByComparator != null) {
Object[] values = orderByComparator.getOrderByConditionValues(journalArticle);
for (Object value : values) {
qPos.add(value);
}
}
List list = q.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the journal articles where smallImageId = ? from the database.
*
* @param smallImageId the small image ID
*/
@Override
public void removeBySmallImageId(long smallImageId) {
for (JournalArticle journalArticle : findBySmallImageId(smallImageId,
QueryUtil.ALL_POS, QueryUtil.ALL_POS, null)) {
remove(journalArticle);
}
}
/**
* Returns the number of journal articles where smallImageId = ?.
*
* @param smallImageId the small image ID
* @return the number of matching journal articles
*/
@Override
public int countBySmallImageId(long smallImageId) {
FinderPath finderPath = FINDER_PATH_COUNT_BY_SMALLIMAGEID;
Object[] finderArgs = new Object[] { smallImageId };
Long count = (Long)finderCache.getResult(finderPath, finderArgs, this);
if (count == null) {
StringBundler query = new StringBundler(2);
query.append(_SQL_COUNT_JOURNALARTICLE_WHERE);
query.append(_FINDER_COLUMN_SMALLIMAGEID_SMALLIMAGEID_2);
String sql = query.toString();
Session session = null;
try {
session = openSession();
Query q = session.createQuery(sql);
QueryPos qPos = QueryPos.getInstance(q);
qPos.add(smallImageId);
count = (Long)q.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception e) {
finderCache.removeResult(finderPath, finderArgs);
throw processException(e);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String _FINDER_COLUMN_SMALLIMAGEID_SMALLIMAGEID_2 = "journalArticle.smallImageId = ?";
public static final FinderPath FINDER_PATH_WITH_PAGINATION_FIND_BY_R_I = new FinderPath(JournalArticleModelImpl.ENTITY_CACHE_ENABLED,
JournalArticleModelImpl.FINDER_CACHE_ENABLED,
JournalArticleImpl.class, FINDER_CLASS_NAME_LIST_WITH_PAGINATION,
"findByR_I",
new String[] {
Long.class.getName(), Boolean.class.getName(),
Integer.class.getName(), Integer.class.getName(),
OrderByComparator.class.getName()
});
public static final FinderPath FINDER_PATH_WITHOUT_PAGINATION_FIND_BY_R_I = new FinderPath(JournalArticleModelImpl.ENTITY_CACHE_ENABLED,
JournalArticleModelImpl.FINDER_CACHE_ENABLED,
JournalArticleImpl.class,
FINDER_CLASS_NAME_LIST_WITHOUT_PAGINATION, "findByR_I",
new String[] { Long.class.getName(), Boolean.class.getName() },
JournalArticleModelImpl.RESOURCEPRIMKEY_COLUMN_BITMASK |
JournalArticleModelImpl.INDEXABLE_COLUMN_BITMASK |
JournalArticleModelImpl.ARTICLEID_COLUMN_BITMASK |
JournalArticleModelImpl.VERSION_COLUMN_BITMASK);
public static final FinderPath FINDER_PATH_COUNT_BY_R_I = new FinderPath(JournalArticleModelImpl.ENTITY_CACHE_ENABLED,
JournalArticleModelImpl.FINDER_CACHE_ENABLED, Long.class,
FINDER_CLASS_NAME_LIST_WITHOUT_PAGINATION, "countByR_I",
new String[] { Long.class.getName(), Boolean.class.getName() });
/**
* Returns all the journal articles where resourcePrimKey = ? and indexable = ?.
*
* @param resourcePrimKey the resource prim key
* @param indexable the indexable
* @return the matching journal articles
*/
@Override
public List findByR_I(long resourcePrimKey,
boolean indexable) {
return findByR_I(resourcePrimKey, indexable, QueryUtil.ALL_POS,
QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the journal articles where resourcePrimKey = ? and indexable = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to {@link QueryUtil#ALL_POS} will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent and pagination is required (start
and end
are not {@link QueryUtil#ALL_POS}), then the query will include the default ORDER BY logic from {@link JournalArticleModelImpl}. If both orderByComparator
and pagination are absent, for performance reasons, the query will not have an ORDER BY clause and the returned result set will be sorted on by the primary key in an ascending order.
*
*
* @param resourcePrimKey the resource prim key
* @param indexable the indexable
* @param start the lower bound of the range of journal articles
* @param end the upper bound of the range of journal articles (not inclusive)
* @return the range of matching journal articles
*/
@Override
public List findByR_I(long resourcePrimKey,
boolean indexable, int start, int end) {
return findByR_I(resourcePrimKey, indexable, start, end, null);
}
/**
* Returns an ordered range of all the journal articles where resourcePrimKey = ? and indexable = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to {@link QueryUtil#ALL_POS} will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent and pagination is required (start
and end
are not {@link QueryUtil#ALL_POS}), then the query will include the default ORDER BY logic from {@link JournalArticleModelImpl}. If both orderByComparator
and pagination are absent, for performance reasons, the query will not have an ORDER BY clause and the returned result set will be sorted on by the primary key in an ascending order.
*
*
* @param resourcePrimKey the resource prim key
* @param indexable the indexable
* @param start the lower bound of the range of journal articles
* @param end the upper bound of the range of journal articles (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching journal articles
*/
@Override
public List findByR_I(long resourcePrimKey,
boolean indexable, int start, int end,
OrderByComparator orderByComparator) {
return findByR_I(resourcePrimKey, indexable, start, end,
orderByComparator, true);
}
/**
* Returns an ordered range of all the journal articles where resourcePrimKey = ? and indexable = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to {@link QueryUtil#ALL_POS} will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent and pagination is required (start
and end
are not {@link QueryUtil#ALL_POS}), then the query will include the default ORDER BY logic from {@link JournalArticleModelImpl}. If both orderByComparator
and pagination are absent, for performance reasons, the query will not have an ORDER BY clause and the returned result set will be sorted on by the primary key in an ascending order.
*
*
* @param resourcePrimKey the resource prim key
* @param indexable the indexable
* @param start the lower bound of the range of journal articles
* @param end the upper bound of the range of journal articles (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param retrieveFromCache whether to retrieve from the finder cache
* @return the ordered range of matching journal articles
*/
@Override
public List findByR_I(long resourcePrimKey,
boolean indexable, int start, int end,
OrderByComparator orderByComparator,
boolean retrieveFromCache) {
boolean pagination = true;
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
pagination = false;
finderPath = FINDER_PATH_WITHOUT_PAGINATION_FIND_BY_R_I;
finderArgs = new Object[] { resourcePrimKey, indexable };
}
else {
finderPath = FINDER_PATH_WITH_PAGINATION_FIND_BY_R_I;
finderArgs = new Object[] {
resourcePrimKey, indexable,
start, end, orderByComparator
};
}
List list = null;
if (retrieveFromCache) {
list = (List)finderCache.getResult(finderPath,
finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (JournalArticle journalArticle : list) {
if ((resourcePrimKey != journalArticle.getResourcePrimKey()) ||
(indexable != journalArticle.getIndexable())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler query = null;
if (orderByComparator != null) {
query = new StringBundler(4 +
(orderByComparator.getOrderByFields().length * 2));
}
else {
query = new StringBundler(4);
}
query.append(_SQL_SELECT_JOURNALARTICLE_WHERE);
query.append(_FINDER_COLUMN_R_I_RESOURCEPRIMKEY_2);
query.append(_FINDER_COLUMN_R_I_INDEXABLE_2);
if (orderByComparator != null) {
appendOrderByComparator(query, _ORDER_BY_ENTITY_ALIAS,
orderByComparator);
}
else
if (pagination) {
query.append(JournalArticleModelImpl.ORDER_BY_JPQL);
}
String sql = query.toString();
Session session = null;
try {
session = openSession();
Query q = session.createQuery(sql);
QueryPos qPos = QueryPos.getInstance(q);
qPos.add(resourcePrimKey);
qPos.add(indexable);
if (!pagination) {
list = (List)QueryUtil.list(q,
getDialect(), start, end, false);
Collections.sort(list);
list = Collections.unmodifiableList(list);
}
else {
list = (List)QueryUtil.list(q,
getDialect(), start, end);
}
cacheResult(list);
finderCache.putResult(finderPath, finderArgs, list);
}
catch (Exception e) {
finderCache.removeResult(finderPath, finderArgs);
throw processException(e);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first journal article in the ordered set where resourcePrimKey = ? and indexable = ?.
*
* @param resourcePrimKey the resource prim key
* @param indexable the indexable
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching journal article
* @throws NoSuchArticleException if a matching journal article could not be found
*/
@Override
public JournalArticle findByR_I_First(long resourcePrimKey,
boolean indexable, OrderByComparator orderByComparator)
throws NoSuchArticleException {
JournalArticle journalArticle = fetchByR_I_First(resourcePrimKey,
indexable, orderByComparator);
if (journalArticle != null) {
return journalArticle;
}
StringBundler msg = new StringBundler(6);
msg.append(_NO_SUCH_ENTITY_WITH_KEY);
msg.append("resourcePrimKey=");
msg.append(resourcePrimKey);
msg.append(", indexable=");
msg.append(indexable);
msg.append(StringPool.CLOSE_CURLY_BRACE);
throw new NoSuchArticleException(msg.toString());
}
/**
* Returns the first journal article in the ordered set where resourcePrimKey = ? and indexable = ?.
*
* @param resourcePrimKey the resource prim key
* @param indexable the indexable
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching journal article, or null
if a matching journal article could not be found
*/
@Override
public JournalArticle fetchByR_I_First(long resourcePrimKey,
boolean indexable, OrderByComparator orderByComparator) {
List list = findByR_I(resourcePrimKey, indexable, 0, 1,
orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last journal article in the ordered set where resourcePrimKey = ? and indexable = ?.
*
* @param resourcePrimKey the resource prim key
* @param indexable the indexable
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching journal article
* @throws NoSuchArticleException if a matching journal article could not be found
*/
@Override
public JournalArticle findByR_I_Last(long resourcePrimKey,
boolean indexable, OrderByComparator orderByComparator)
throws NoSuchArticleException {
JournalArticle journalArticle = fetchByR_I_Last(resourcePrimKey,
indexable, orderByComparator);
if (journalArticle != null) {
return journalArticle;
}
StringBundler msg = new StringBundler(6);
msg.append(_NO_SUCH_ENTITY_WITH_KEY);
msg.append("resourcePrimKey=");
msg.append(resourcePrimKey);
msg.append(", indexable=");
msg.append(indexable);
msg.append(StringPool.CLOSE_CURLY_BRACE);
throw new NoSuchArticleException(msg.toString());
}
/**
* Returns the last journal article in the ordered set where resourcePrimKey = ? and indexable = ?.
*
* @param resourcePrimKey the resource prim key
* @param indexable the indexable
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching journal article, or null
if a matching journal article could not be found
*/
@Override
public JournalArticle fetchByR_I_Last(long resourcePrimKey,
boolean indexable, OrderByComparator orderByComparator) {
int count = countByR_I(resourcePrimKey, indexable);
if (count == 0) {
return null;
}
List list = findByR_I(resourcePrimKey, indexable,
count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the journal articles before and after the current journal article in the ordered set where resourcePrimKey = ? and indexable = ?.
*
* @param id the primary key of the current journal article
* @param resourcePrimKey the resource prim key
* @param indexable the indexable
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next journal article
* @throws NoSuchArticleException if a journal article with the primary key could not be found
*/
@Override
public JournalArticle[] findByR_I_PrevAndNext(long id,
long resourcePrimKey, boolean indexable,
OrderByComparator orderByComparator)
throws NoSuchArticleException {
JournalArticle journalArticle = findByPrimaryKey(id);
Session session = null;
try {
session = openSession();
JournalArticle[] array = new JournalArticleImpl[3];
array[0] = getByR_I_PrevAndNext(session, journalArticle,
resourcePrimKey, indexable, orderByComparator, true);
array[1] = journalArticle;
array[2] = getByR_I_PrevAndNext(session, journalArticle,
resourcePrimKey, indexable, orderByComparator, false);
return array;
}
catch (Exception e) {
throw processException(e);
}
finally {
closeSession(session);
}
}
protected JournalArticle getByR_I_PrevAndNext(Session session,
JournalArticle journalArticle, long resourcePrimKey, boolean indexable,
OrderByComparator orderByComparator, boolean previous) {
StringBundler query = null;
if (orderByComparator != null) {
query = new StringBundler(5 +
(orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
query = new StringBundler(4);
}
query.append(_SQL_SELECT_JOURNALARTICLE_WHERE);
query.append(_FINDER_COLUMN_R_I_RESOURCEPRIMKEY_2);
query.append(_FINDER_COLUMN_R_I_INDEXABLE_2);
if (orderByComparator != null) {
String[] orderByConditionFields = orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
query.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
query.append(_ORDER_BY_ENTITY_ALIAS);
query.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
query.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
query.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
query.append(WHERE_GREATER_THAN);
}
else {
query.append(WHERE_LESSER_THAN);
}
}
}
query.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
query.append(_ORDER_BY_ENTITY_ALIAS);
query.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
query.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
query.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
query.append(ORDER_BY_ASC);
}
else {
query.append(ORDER_BY_DESC);
}
}
}
}
else {
query.append(JournalArticleModelImpl.ORDER_BY_JPQL);
}
String sql = query.toString();
Query q = session.createQuery(sql);
q.setFirstResult(0);
q.setMaxResults(2);
QueryPos qPos = QueryPos.getInstance(q);
qPos.add(resourcePrimKey);
qPos.add(indexable);
if (orderByComparator != null) {
Object[] values = orderByComparator.getOrderByConditionValues(journalArticle);
for (Object value : values) {
qPos.add(value);
}
}
List list = q.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the journal articles where resourcePrimKey = ? and indexable = ? from the database.
*
* @param resourcePrimKey the resource prim key
* @param indexable the indexable
*/
@Override
public void removeByR_I(long resourcePrimKey, boolean indexable) {
for (JournalArticle journalArticle : findByR_I(resourcePrimKey,
indexable, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null)) {
remove(journalArticle);
}
}
/**
* Returns the number of journal articles where resourcePrimKey = ? and indexable = ?.
*
* @param resourcePrimKey the resource prim key
* @param indexable the indexable
* @return the number of matching journal articles
*/
@Override
public int countByR_I(long resourcePrimKey, boolean indexable) {
FinderPath finderPath = FINDER_PATH_COUNT_BY_R_I;
Object[] finderArgs = new Object[] { resourcePrimKey, indexable };
Long count = (Long)finderCache.getResult(finderPath, finderArgs, this);
if (count == null) {
StringBundler query = new StringBundler(3);
query.append(_SQL_COUNT_JOURNALARTICLE_WHERE);
query.append(_FINDER_COLUMN_R_I_RESOURCEPRIMKEY_2);
query.append(_FINDER_COLUMN_R_I_INDEXABLE_2);
String sql = query.toString();
Session session = null;
try {
session = openSession();
Query q = session.createQuery(sql);
QueryPos qPos = QueryPos.getInstance(q);
qPos.add(resourcePrimKey);
qPos.add(indexable);
count = (Long)q.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception e) {
finderCache.removeResult(finderPath, finderArgs);
throw processException(e);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String _FINDER_COLUMN_R_I_RESOURCEPRIMKEY_2 = "journalArticle.resourcePrimKey = ? AND ";
private static final String _FINDER_COLUMN_R_I_INDEXABLE_2 = "journalArticle.indexable = ?";
public static final FinderPath FINDER_PATH_WITH_PAGINATION_FIND_BY_R_ST = new FinderPath(JournalArticleModelImpl.ENTITY_CACHE_ENABLED,
JournalArticleModelImpl.FINDER_CACHE_ENABLED,
JournalArticleImpl.class, FINDER_CLASS_NAME_LIST_WITH_PAGINATION,
"findByR_ST",
new String[] {
Long.class.getName(), Integer.class.getName(),
Integer.class.getName(), Integer.class.getName(),
OrderByComparator.class.getName()
});
public static final FinderPath FINDER_PATH_WITHOUT_PAGINATION_FIND_BY_R_ST = new FinderPath(JournalArticleModelImpl.ENTITY_CACHE_ENABLED,
JournalArticleModelImpl.FINDER_CACHE_ENABLED,
JournalArticleImpl.class,
FINDER_CLASS_NAME_LIST_WITHOUT_PAGINATION, "findByR_ST",
new String[] { Long.class.getName(), Integer.class.getName() },
JournalArticleModelImpl.RESOURCEPRIMKEY_COLUMN_BITMASK |
JournalArticleModelImpl.STATUS_COLUMN_BITMASK |
JournalArticleModelImpl.ARTICLEID_COLUMN_BITMASK |
JournalArticleModelImpl.VERSION_COLUMN_BITMASK);
public static final FinderPath FINDER_PATH_COUNT_BY_R_ST = new FinderPath(JournalArticleModelImpl.ENTITY_CACHE_ENABLED,
JournalArticleModelImpl.FINDER_CACHE_ENABLED, Long.class,
FINDER_CLASS_NAME_LIST_WITHOUT_PAGINATION, "countByR_ST",
new String[] { Long.class.getName(), Integer.class.getName() });
public static final FinderPath FINDER_PATH_WITH_PAGINATION_COUNT_BY_R_ST = new FinderPath(JournalArticleModelImpl.ENTITY_CACHE_ENABLED,
JournalArticleModelImpl.FINDER_CACHE_ENABLED, Long.class,
FINDER_CLASS_NAME_LIST_WITH_PAGINATION, "countByR_ST",
new String[] { Long.class.getName(), Integer.class.getName() });
/**
* Returns all the journal articles where resourcePrimKey = ? and status = ?.
*
* @param resourcePrimKey the resource prim key
* @param status the status
* @return the matching journal articles
*/
@Override
public List findByR_ST(long resourcePrimKey, int status) {
return findByR_ST(resourcePrimKey, status, QueryUtil.ALL_POS,
QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the journal articles where resourcePrimKey = ? and status = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to {@link QueryUtil#ALL_POS} will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent and pagination is required (start
and end
are not {@link QueryUtil#ALL_POS}), then the query will include the default ORDER BY logic from {@link JournalArticleModelImpl}. If both orderByComparator
and pagination are absent, for performance reasons, the query will not have an ORDER BY clause and the returned result set will be sorted on by the primary key in an ascending order.
*
*
* @param resourcePrimKey the resource prim key
* @param status the status
* @param start the lower bound of the range of journal articles
* @param end the upper bound of the range of journal articles (not inclusive)
* @return the range of matching journal articles
*/
@Override
public List findByR_ST(long resourcePrimKey, int status,
int start, int end) {
return findByR_ST(resourcePrimKey, status, start, end, null);
}
/**
* Returns an ordered range of all the journal articles where resourcePrimKey = ? and status = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to {@link QueryUtil#ALL_POS} will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent and pagination is required (start
and end
are not {@link QueryUtil#ALL_POS}), then the query will include the default ORDER BY logic from {@link JournalArticleModelImpl}. If both orderByComparator
and pagination are absent, for performance reasons, the query will not have an ORDER BY clause and the returned result set will be sorted on by the primary key in an ascending order.
*
*
* @param resourcePrimKey the resource prim key
* @param status the status
* @param start the lower bound of the range of journal articles
* @param end the upper bound of the range of journal articles (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching journal articles
*/
@Override
public List findByR_ST(long resourcePrimKey, int status,
int start, int end, OrderByComparator orderByComparator) {
return findByR_ST(resourcePrimKey, status, start, end,
orderByComparator, true);
}
/**
* Returns an ordered range of all the journal articles where resourcePrimKey = ? and status = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to {@link QueryUtil#ALL_POS} will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent and pagination is required (start
and end
are not {@link QueryUtil#ALL_POS}), then the query will include the default ORDER BY logic from {@link JournalArticleModelImpl}. If both orderByComparator
and pagination are absent, for performance reasons, the query will not have an ORDER BY clause and the returned result set will be sorted on by the primary key in an ascending order.
*
*
* @param resourcePrimKey the resource prim key
* @param status the status
* @param start the lower bound of the range of journal articles
* @param end the upper bound of the range of journal articles (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param retrieveFromCache whether to retrieve from the finder cache
* @return the ordered range of matching journal articles
*/
@Override
public List findByR_ST(long resourcePrimKey, int status,
int start, int end,
OrderByComparator orderByComparator,
boolean retrieveFromCache) {
boolean pagination = true;
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
pagination = false;
finderPath = FINDER_PATH_WITHOUT_PAGINATION_FIND_BY_R_ST;
finderArgs = new Object[] { resourcePrimKey, status };
}
else {
finderPath = FINDER_PATH_WITH_PAGINATION_FIND_BY_R_ST;
finderArgs = new Object[] {
resourcePrimKey, status,
start, end, orderByComparator
};
}
List list = null;
if (retrieveFromCache) {
list = (List)finderCache.getResult(finderPath,
finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (JournalArticle journalArticle : list) {
if ((resourcePrimKey != journalArticle.getResourcePrimKey()) ||
(status != journalArticle.getStatus())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler query = null;
if (orderByComparator != null) {
query = new StringBundler(4 +
(orderByComparator.getOrderByFields().length * 2));
}
else {
query = new StringBundler(4);
}
query.append(_SQL_SELECT_JOURNALARTICLE_WHERE);
query.append(_FINDER_COLUMN_R_ST_RESOURCEPRIMKEY_2);
query.append(_FINDER_COLUMN_R_ST_STATUS_2);
if (orderByComparator != null) {
appendOrderByComparator(query, _ORDER_BY_ENTITY_ALIAS,
orderByComparator);
}
else
if (pagination) {
query.append(JournalArticleModelImpl.ORDER_BY_JPQL);
}
String sql = query.toString();
Session session = null;
try {
session = openSession();
Query q = session.createQuery(sql);
QueryPos qPos = QueryPos.getInstance(q);
qPos.add(resourcePrimKey);
qPos.add(status);
if (!pagination) {
list = (List)QueryUtil.list(q,
getDialect(), start, end, false);
Collections.sort(list);
list = Collections.unmodifiableList(list);
}
else {
list = (List)QueryUtil.list(q,
getDialect(), start, end);
}
cacheResult(list);
finderCache.putResult(finderPath, finderArgs, list);
}
catch (Exception e) {
finderCache.removeResult(finderPath, finderArgs);
throw processException(e);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first journal article in the ordered set where resourcePrimKey = ? and status = ?.
*
* @param resourcePrimKey the resource prim key
* @param status the status
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching journal article
* @throws NoSuchArticleException if a matching journal article could not be found
*/
@Override
public JournalArticle findByR_ST_First(long resourcePrimKey, int status,
OrderByComparator orderByComparator)
throws NoSuchArticleException {
JournalArticle journalArticle = fetchByR_ST_First(resourcePrimKey,
status, orderByComparator);
if (journalArticle != null) {
return journalArticle;
}
StringBundler msg = new StringBundler(6);
msg.append(_NO_SUCH_ENTITY_WITH_KEY);
msg.append("resourcePrimKey=");
msg.append(resourcePrimKey);
msg.append(", status=");
msg.append(status);
msg.append(StringPool.CLOSE_CURLY_BRACE);
throw new NoSuchArticleException(msg.toString());
}
/**
* Returns the first journal article in the ordered set where resourcePrimKey = ? and status = ?.
*
* @param resourcePrimKey the resource prim key
* @param status the status
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching journal article, or null
if a matching journal article could not be found
*/
@Override
public JournalArticle fetchByR_ST_First(long resourcePrimKey, int status,
OrderByComparator orderByComparator) {
List list = findByR_ST(resourcePrimKey, status, 0, 1,
orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last journal article in the ordered set where resourcePrimKey = ? and status = ?.
*
* @param resourcePrimKey the resource prim key
* @param status the status
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching journal article
* @throws NoSuchArticleException if a matching journal article could not be found
*/
@Override
public JournalArticle findByR_ST_Last(long resourcePrimKey, int status,
OrderByComparator orderByComparator)
throws NoSuchArticleException {
JournalArticle journalArticle = fetchByR_ST_Last(resourcePrimKey,
status, orderByComparator);
if (journalArticle != null) {
return journalArticle;
}
StringBundler msg = new StringBundler(6);
msg.append(_NO_SUCH_ENTITY_WITH_KEY);
msg.append("resourcePrimKey=");
msg.append(resourcePrimKey);
msg.append(", status=");
msg.append(status);
msg.append(StringPool.CLOSE_CURLY_BRACE);
throw new NoSuchArticleException(msg.toString());
}
/**
* Returns the last journal article in the ordered set where resourcePrimKey = ? and status = ?.
*
* @param resourcePrimKey the resource prim key
* @param status the status
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching journal article, or null
if a matching journal article could not be found
*/
@Override
public JournalArticle fetchByR_ST_Last(long resourcePrimKey, int status,
OrderByComparator orderByComparator) {
int count = countByR_ST(resourcePrimKey, status);
if (count == 0) {
return null;
}
List list = findByR_ST(resourcePrimKey, status,
count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the journal articles before and after the current journal article in the ordered set where resourcePrimKey = ? and status = ?.
*
* @param id the primary key of the current journal article
* @param resourcePrimKey the resource prim key
* @param status the status
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next journal article
* @throws NoSuchArticleException if a journal article with the primary key could not be found
*/
@Override
public JournalArticle[] findByR_ST_PrevAndNext(long id,
long resourcePrimKey, int status,
OrderByComparator orderByComparator)
throws NoSuchArticleException {
JournalArticle journalArticle = findByPrimaryKey(id);
Session session = null;
try {
session = openSession();
JournalArticle[] array = new JournalArticleImpl[3];
array[0] = getByR_ST_PrevAndNext(session, journalArticle,
resourcePrimKey, status, orderByComparator, true);
array[1] = journalArticle;
array[2] = getByR_ST_PrevAndNext(session, journalArticle,
resourcePrimKey, status, orderByComparator, false);
return array;
}
catch (Exception e) {
throw processException(e);
}
finally {
closeSession(session);
}
}
protected JournalArticle getByR_ST_PrevAndNext(Session session,
JournalArticle journalArticle, long resourcePrimKey, int status,
OrderByComparator orderByComparator, boolean previous) {
StringBundler query = null;
if (orderByComparator != null) {
query = new StringBundler(5 +
(orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
query = new StringBundler(4);
}
query.append(_SQL_SELECT_JOURNALARTICLE_WHERE);
query.append(_FINDER_COLUMN_R_ST_RESOURCEPRIMKEY_2);
query.append(_FINDER_COLUMN_R_ST_STATUS_2);
if (orderByComparator != null) {
String[] orderByConditionFields = orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
query.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
query.append(_ORDER_BY_ENTITY_ALIAS);
query.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
query.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
query.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
query.append(WHERE_GREATER_THAN);
}
else {
query.append(WHERE_LESSER_THAN);
}
}
}
query.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
query.append(_ORDER_BY_ENTITY_ALIAS);
query.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
query.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
query.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
query.append(ORDER_BY_ASC);
}
else {
query.append(ORDER_BY_DESC);
}
}
}
}
else {
query.append(JournalArticleModelImpl.ORDER_BY_JPQL);
}
String sql = query.toString();
Query q = session.createQuery(sql);
q.setFirstResult(0);
q.setMaxResults(2);
QueryPos qPos = QueryPos.getInstance(q);
qPos.add(resourcePrimKey);
qPos.add(status);
if (orderByComparator != null) {
Object[] values = orderByComparator.getOrderByConditionValues(journalArticle);
for (Object value : values) {
qPos.add(value);
}
}
List list = q.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Returns all the journal articles where resourcePrimKey = ? and status = any ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to {@link QueryUtil#ALL_POS} will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent and pagination is required (start
and end
are not {@link QueryUtil#ALL_POS}), then the query will include the default ORDER BY logic from {@link JournalArticleModelImpl}. If both orderByComparator
and pagination are absent, for performance reasons, the query will not have an ORDER BY clause and the returned result set will be sorted on by the primary key in an ascending order.
*
*
* @param resourcePrimKey the resource prim key
* @param statuses the statuses
* @return the matching journal articles
*/
@Override
public List findByR_ST(long resourcePrimKey, int[] statuses) {
return findByR_ST(resourcePrimKey, statuses, QueryUtil.ALL_POS,
QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the journal articles where resourcePrimKey = ? and status = any ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to {@link QueryUtil#ALL_POS} will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent and pagination is required (start
and end
are not {@link QueryUtil#ALL_POS}), then the query will include the default ORDER BY logic from {@link JournalArticleModelImpl}. If both orderByComparator
and pagination are absent, for performance reasons, the query will not have an ORDER BY clause and the returned result set will be sorted on by the primary key in an ascending order.
*
*
* @param resourcePrimKey the resource prim key
* @param statuses the statuses
* @param start the lower bound of the range of journal articles
* @param end the upper bound of the range of journal articles (not inclusive)
* @return the range of matching journal articles
*/
@Override
public List findByR_ST(long resourcePrimKey,
int[] statuses, int start, int end) {
return findByR_ST(resourcePrimKey, statuses, start, end, null);
}
/**
* Returns an ordered range of all the journal articles where resourcePrimKey = ? and status = any ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to {@link QueryUtil#ALL_POS} will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent and pagination is required (start
and end
are not {@link QueryUtil#ALL_POS}), then the query will include the default ORDER BY logic from {@link JournalArticleModelImpl}. If both orderByComparator
and pagination are absent, for performance reasons, the query will not have an ORDER BY clause and the returned result set will be sorted on by the primary key in an ascending order.
*
*
* @param resourcePrimKey the resource prim key
* @param statuses the statuses
* @param start the lower bound of the range of journal articles
* @param end the upper bound of the range of journal articles (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching journal articles
*/
@Override
public List findByR_ST(long resourcePrimKey,
int[] statuses, int start, int end,
OrderByComparator orderByComparator) {
return findByR_ST(resourcePrimKey, statuses, start, end,
orderByComparator, true);
}
/**
* Returns an ordered range of all the journal articles where resourcePrimKey = ? and status = ?, optionally using the finder cache.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to {@link QueryUtil#ALL_POS} will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent and pagination is required (start
and end
are not {@link QueryUtil#ALL_POS}), then the query will include the default ORDER BY logic from {@link JournalArticleModelImpl}. If both orderByComparator
and pagination are absent, for performance reasons, the query will not have an ORDER BY clause and the returned result set will be sorted on by the primary key in an ascending order.
*
*
* @param resourcePrimKey the resource prim key
* @param status the status
* @param start the lower bound of the range of journal articles
* @param end the upper bound of the range of journal articles (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param retrieveFromCache whether to retrieve from the finder cache
* @return the ordered range of matching journal articles
*/
@Override
public List findByR_ST(long resourcePrimKey,
int[] statuses, int start, int end,
OrderByComparator orderByComparator,
boolean retrieveFromCache) {
if (statuses == null) {
statuses = new int[0];
}
else if (statuses.length > 1) {
statuses = ArrayUtil.unique(statuses);
Arrays.sort(statuses);
}
if (statuses.length == 1) {
return findByR_ST(resourcePrimKey, statuses[0], start, end,
orderByComparator);
}
boolean pagination = true;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
pagination = false;
finderArgs = new Object[] {
resourcePrimKey, StringUtil.merge(statuses)
};
}
else {
finderArgs = new Object[] {
resourcePrimKey, StringUtil.merge(statuses),
start, end, orderByComparator
};
}
List list = null;
if (retrieveFromCache) {
list = (List)finderCache.getResult(FINDER_PATH_WITH_PAGINATION_FIND_BY_R_ST,
finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (JournalArticle journalArticle : list) {
if ((resourcePrimKey != journalArticle.getResourcePrimKey()) ||
!ArrayUtil.contains(statuses,
journalArticle.getStatus())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler query = new StringBundler();
query.append(_SQL_SELECT_JOURNALARTICLE_WHERE);
query.append(_FINDER_COLUMN_R_ST_RESOURCEPRIMKEY_2);
if (statuses.length > 0) {
query.append(StringPool.OPEN_PARENTHESIS);
query.append(_FINDER_COLUMN_R_ST_STATUS_7);
query.append(StringUtil.merge(statuses));
query.append(StringPool.CLOSE_PARENTHESIS);
query.append(StringPool.CLOSE_PARENTHESIS);
}
query.setStringAt(removeConjunction(query.stringAt(query.index() -
1)), query.index() - 1);
if (orderByComparator != null) {
appendOrderByComparator(query, _ORDER_BY_ENTITY_ALIAS,
orderByComparator);
}
else
if (pagination) {
query.append(JournalArticleModelImpl.ORDER_BY_JPQL);
}
String sql = query.toString();
Session session = null;
try {
session = openSession();
Query q = session.createQuery(sql);
QueryPos qPos = QueryPos.getInstance(q);
qPos.add(resourcePrimKey);
if (!pagination) {
list = (List)QueryUtil.list(q,
getDialect(), start, end, false);
Collections.sort(list);
list = Collections.unmodifiableList(list);
}
else {
list = (List)QueryUtil.list(q,
getDialect(), start, end);
}
cacheResult(list);
finderCache.putResult(FINDER_PATH_WITH_PAGINATION_FIND_BY_R_ST,
finderArgs, list);
}
catch (Exception e) {
finderCache.removeResult(FINDER_PATH_WITH_PAGINATION_FIND_BY_R_ST,
finderArgs);
throw processException(e);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Removes all the journal articles where resourcePrimKey = ? and status = ? from the database.
*
* @param resourcePrimKey the resource prim key
* @param status the status
*/
@Override
public void removeByR_ST(long resourcePrimKey, int status) {
for (JournalArticle journalArticle : findByR_ST(resourcePrimKey,
status, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null)) {
remove(journalArticle);
}
}
/**
* Returns the number of journal articles where resourcePrimKey = ? and status = ?.
*
* @param resourcePrimKey the resource prim key
* @param status the status
* @return the number of matching journal articles
*/
@Override
public int countByR_ST(long resourcePrimKey, int status) {
FinderPath finderPath = FINDER_PATH_COUNT_BY_R_ST;
Object[] finderArgs = new Object[] { resourcePrimKey, status };
Long count = (Long)finderCache.getResult(finderPath, finderArgs, this);
if (count == null) {
StringBundler query = new StringBundler(3);
query.append(_SQL_COUNT_JOURNALARTICLE_WHERE);
query.append(_FINDER_COLUMN_R_ST_RESOURCEPRIMKEY_2);
query.append(_FINDER_COLUMN_R_ST_STATUS_2);
String sql = query.toString();
Session session = null;
try {
session = openSession();
Query q = session.createQuery(sql);
QueryPos qPos = QueryPos.getInstance(q);
qPos.add(resourcePrimKey);
qPos.add(status);
count = (Long)q.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception e) {
finderCache.removeResult(finderPath, finderArgs);
throw processException(e);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
/**
* Returns the number of journal articles where resourcePrimKey = ? and status = any ?.
*
* @param resourcePrimKey the resource prim key
* @param statuses the statuses
* @return the number of matching journal articles
*/
@Override
public int countByR_ST(long resourcePrimKey, int[] statuses) {
if (statuses == null) {
statuses = new int[0];
}
else if (statuses.length > 1) {
statuses = ArrayUtil.unique(statuses);
Arrays.sort(statuses);
}
Object[] finderArgs = new Object[] {
resourcePrimKey, StringUtil.merge(statuses)
};
Long count = (Long)finderCache.getResult(FINDER_PATH_WITH_PAGINATION_COUNT_BY_R_ST,
finderArgs, this);
if (count == null) {
StringBundler query = new StringBundler();
query.append(_SQL_COUNT_JOURNALARTICLE_WHERE);
query.append(_FINDER_COLUMN_R_ST_RESOURCEPRIMKEY_2);
if (statuses.length > 0) {
query.append(StringPool.OPEN_PARENTHESIS);
query.append(_FINDER_COLUMN_R_ST_STATUS_7);
query.append(StringUtil.merge(statuses));
query.append(StringPool.CLOSE_PARENTHESIS);
query.append(StringPool.CLOSE_PARENTHESIS);
}
query.setStringAt(removeConjunction(query.stringAt(query.index() -
1)), query.index() - 1);
String sql = query.toString();
Session session = null;
try {
session = openSession();
Query q = session.createQuery(sql);
QueryPos qPos = QueryPos.getInstance(q);
qPos.add(resourcePrimKey);
count = (Long)q.uniqueResult();
finderCache.putResult(FINDER_PATH_WITH_PAGINATION_COUNT_BY_R_ST,
finderArgs, count);
}
catch (Exception e) {
finderCache.removeResult(FINDER_PATH_WITH_PAGINATION_COUNT_BY_R_ST,
finderArgs);
throw processException(e);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String _FINDER_COLUMN_R_ST_RESOURCEPRIMKEY_2 = "journalArticle.resourcePrimKey = ? AND ";
private static final String _FINDER_COLUMN_R_ST_STATUS_2 = "journalArticle.status = ?";
private static final String _FINDER_COLUMN_R_ST_STATUS_7 = "journalArticle.status IN (";
public static final FinderPath FINDER_PATH_WITH_PAGINATION_FIND_BY_G_U = new FinderPath(JournalArticleModelImpl.ENTITY_CACHE_ENABLED,
JournalArticleModelImpl.FINDER_CACHE_ENABLED,
JournalArticleImpl.class, FINDER_CLASS_NAME_LIST_WITH_PAGINATION,
"findByG_U",
new String[] {
Long.class.getName(), Long.class.getName(),
Integer.class.getName(), Integer.class.getName(),
OrderByComparator.class.getName()
});
public static final FinderPath FINDER_PATH_WITHOUT_PAGINATION_FIND_BY_G_U = new FinderPath(JournalArticleModelImpl.ENTITY_CACHE_ENABLED,
JournalArticleModelImpl.FINDER_CACHE_ENABLED,
JournalArticleImpl.class,
FINDER_CLASS_NAME_LIST_WITHOUT_PAGINATION, "findByG_U",
new String[] { Long.class.getName(), Long.class.getName() },
JournalArticleModelImpl.GROUPID_COLUMN_BITMASK |
JournalArticleModelImpl.USERID_COLUMN_BITMASK |
JournalArticleModelImpl.ARTICLEID_COLUMN_BITMASK |
JournalArticleModelImpl.VERSION_COLUMN_BITMASK);
public static final FinderPath FINDER_PATH_COUNT_BY_G_U = new FinderPath(JournalArticleModelImpl.ENTITY_CACHE_ENABLED,
JournalArticleModelImpl.FINDER_CACHE_ENABLED, Long.class,
FINDER_CLASS_NAME_LIST_WITHOUT_PAGINATION, "countByG_U",
new String[] { Long.class.getName(), Long.class.getName() });
/**
* Returns all the journal articles where groupId = ? and userId = ?.
*
* @param groupId the group ID
* @param userId the user ID
* @return the matching journal articles
*/
@Override
public List findByG_U(long groupId, long userId) {
return findByG_U(groupId, userId, QueryUtil.ALL_POS, QueryUtil.ALL_POS,
null);
}
/**
* Returns a range of all the journal articles where groupId = ? and userId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to {@link QueryUtil#ALL_POS} will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent and pagination is required (start
and end
are not {@link QueryUtil#ALL_POS}), then the query will include the default ORDER BY logic from {@link JournalArticleModelImpl}. If both orderByComparator
and pagination are absent, for performance reasons, the query will not have an ORDER BY clause and the returned result set will be sorted on by the primary key in an ascending order.
*
*
* @param groupId the group ID
* @param userId the user ID
* @param start the lower bound of the range of journal articles
* @param end the upper bound of the range of journal articles (not inclusive)
* @return the range of matching journal articles
*/
@Override
public List findByG_U(long groupId, long userId, int start,
int end) {
return findByG_U(groupId, userId, start, end, null);
}
/**
* Returns an ordered range of all the journal articles where groupId = ? and userId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to {@link QueryUtil#ALL_POS} will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent and pagination is required (start
and end
are not {@link QueryUtil#ALL_POS}), then the query will include the default ORDER BY logic from {@link JournalArticleModelImpl}. If both orderByComparator
and pagination are absent, for performance reasons, the query will not have an ORDER BY clause and the returned result set will be sorted on by the primary key in an ascending order.
*
*
* @param groupId the group ID
* @param userId the user ID
* @param start the lower bound of the range of journal articles
* @param end the upper bound of the range of journal articles (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching journal articles
*/
@Override
public List findByG_U(long groupId, long userId, int start,
int end, OrderByComparator orderByComparator) {
return findByG_U(groupId, userId, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the journal articles where groupId = ? and userId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to {@link QueryUtil#ALL_POS} will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent and pagination is required (start
and end
are not {@link QueryUtil#ALL_POS}), then the query will include the default ORDER BY logic from {@link JournalArticleModelImpl}. If both orderByComparator
and pagination are absent, for performance reasons, the query will not have an ORDER BY clause and the returned result set will be sorted on by the primary key in an ascending order.
*
*
* @param groupId the group ID
* @param userId the user ID
* @param start the lower bound of the range of journal articles
* @param end the upper bound of the range of journal articles (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param retrieveFromCache whether to retrieve from the finder cache
* @return the ordered range of matching journal articles
*/
@Override
public List findByG_U(long groupId, long userId, int start,
int end, OrderByComparator orderByComparator,
boolean retrieveFromCache) {
boolean pagination = true;
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
pagination = false;
finderPath = FINDER_PATH_WITHOUT_PAGINATION_FIND_BY_G_U;
finderArgs = new Object[] { groupId, userId };
}
else {
finderPath = FINDER_PATH_WITH_PAGINATION_FIND_BY_G_U;
finderArgs = new Object[] {
groupId, userId,
start, end, orderByComparator
};
}
List list = null;
if (retrieveFromCache) {
list = (List)finderCache.getResult(finderPath,
finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (JournalArticle journalArticle : list) {
if ((groupId != journalArticle.getGroupId()) ||
(userId != journalArticle.getUserId())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler query = null;
if (orderByComparator != null) {
query = new StringBundler(4 +
(orderByComparator.getOrderByFields().length * 2));
}
else {
query = new StringBundler(4);
}
query.append(_SQL_SELECT_JOURNALARTICLE_WHERE);
query.append(_FINDER_COLUMN_G_U_GROUPID_2);
query.append(_FINDER_COLUMN_G_U_USERID_2);
if (orderByComparator != null) {
appendOrderByComparator(query, _ORDER_BY_ENTITY_ALIAS,
orderByComparator);
}
else
if (pagination) {
query.append(JournalArticleModelImpl.ORDER_BY_JPQL);
}
String sql = query.toString();
Session session = null;
try {
session = openSession();
Query q = session.createQuery(sql);
QueryPos qPos = QueryPos.getInstance(q);
qPos.add(groupId);
qPos.add(userId);
if (!pagination) {
list = (List)QueryUtil.list(q,
getDialect(), start, end, false);
Collections.sort(list);
list = Collections.unmodifiableList(list);
}
else {
list = (List)QueryUtil.list(q,
getDialect(), start, end);
}
cacheResult(list);
finderCache.putResult(finderPath, finderArgs, list);
}
catch (Exception e) {
finderCache.removeResult(finderPath, finderArgs);
throw processException(e);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first journal article in the ordered set where groupId = ? and userId = ?.
*
* @param groupId the group ID
* @param userId the user ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching journal article
* @throws NoSuchArticleException if a matching journal article could not be found
*/
@Override
public JournalArticle findByG_U_First(long groupId, long userId,
OrderByComparator orderByComparator)
throws NoSuchArticleException {
JournalArticle journalArticle = fetchByG_U_First(groupId, userId,
orderByComparator);
if (journalArticle != null) {
return journalArticle;
}
StringBundler msg = new StringBundler(6);
msg.append(_NO_SUCH_ENTITY_WITH_KEY);
msg.append("groupId=");
msg.append(groupId);
msg.append(", userId=");
msg.append(userId);
msg.append(StringPool.CLOSE_CURLY_BRACE);
throw new NoSuchArticleException(msg.toString());
}
/**
* Returns the first journal article in the ordered set where groupId = ? and userId = ?.
*
* @param groupId the group ID
* @param userId the user ID
* @param orderByComparator the comparator to order the set by (optionally