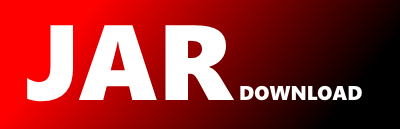
com.liferay.journal.web.internal.display.context.JournalArticleItemSelectorViewDisplayContext Maven / Gradle / Ivy
/**
* SPDX-FileCopyrightText: (c) 2000 Liferay, Inc. https://liferay.com
* SPDX-License-Identifier: LGPL-2.1-or-later OR LicenseRef-Liferay-DXP-EULA-2.0.0-2023-06
*/
package com.liferay.journal.web.internal.display.context;
import com.liferay.asset.kernel.AssetRendererFactoryRegistryUtil;
import com.liferay.asset.kernel.model.AssetEntry;
import com.liferay.asset.kernel.model.AssetRendererFactory;
import com.liferay.asset.kernel.model.ClassType;
import com.liferay.asset.kernel.model.ClassTypeReader;
import com.liferay.asset.kernel.service.AssetEntryLocalServiceUtil;
import com.liferay.depot.util.SiteConnectedGroupGroupProviderUtil;
import com.liferay.dynamic.data.mapping.model.DDMStructure;
import com.liferay.dynamic.data.mapping.service.DDMStructureLocalServiceUtil;
import com.liferay.item.selector.criteria.InfoItemItemSelectorReturnType;
import com.liferay.item.selector.criteria.info.item.criterion.InfoItemItemSelectorCriterion;
import com.liferay.journal.constants.JournalArticleConstants;
import com.liferay.journal.constants.JournalFolderConstants;
import com.liferay.journal.constants.JournalPortletKeys;
import com.liferay.journal.model.JournalArticle;
import com.liferay.journal.model.JournalFolder;
import com.liferay.journal.service.JournalArticleLocalServiceUtil;
import com.liferay.journal.service.JournalFolderLocalServiceUtil;
import com.liferay.journal.service.JournalFolderServiceUtil;
import com.liferay.journal.util.comparator.FolderArticleArticleIdComparator;
import com.liferay.journal.util.comparator.FolderArticleModifiedDateComparator;
import com.liferay.journal.util.comparator.FolderArticleTitleComparator;
import com.liferay.journal.web.internal.asset.model.JournalArticleAssetRenderer;
import com.liferay.journal.web.internal.configuration.JournalWebConfiguration;
import com.liferay.journal.web.internal.dao.search.JournalRowChecker;
import com.liferay.journal.web.internal.item.selector.JournalArticleItemSelectorView;
import com.liferay.journal.web.internal.util.JournalSearcherUtil;
import com.liferay.petra.function.transform.TransformUtil;
import com.liferay.petra.string.StringBundler;
import com.liferay.petra.string.StringPool;
import com.liferay.portal.kernel.bean.BeanParamUtil;
import com.liferay.portal.kernel.dao.search.SearchContainer;
import com.liferay.portal.kernel.exception.PortalException;
import com.liferay.portal.kernel.json.JSONUtil;
import com.liferay.portal.kernel.language.LanguageUtil;
import com.liferay.portal.kernel.model.Group;
import com.liferay.portal.kernel.model.ResourceConstants;
import com.liferay.portal.kernel.model.Role;
import com.liferay.portal.kernel.model.role.RoleConstants;
import com.liferay.portal.kernel.portlet.PortletURLUtil;
import com.liferay.portal.kernel.portlet.SearchDisplayStyleUtil;
import com.liferay.portal.kernel.portlet.SearchOrderByUtil;
import com.liferay.portal.kernel.portlet.url.builder.PortletURLBuilder;
import com.liferay.portal.kernel.search.Field;
import com.liferay.portal.kernel.search.QueryConfig;
import com.liferay.portal.kernel.search.SearchContext;
import com.liferay.portal.kernel.search.Sort;
import com.liferay.portal.kernel.security.permission.ActionKeys;
import com.liferay.portal.kernel.security.permission.ResourceActionsUtil;
import com.liferay.portal.kernel.service.GroupLocalServiceUtil;
import com.liferay.portal.kernel.service.GroupServiceUtil;
import com.liferay.portal.kernel.service.ResourcePermissionLocalService;
import com.liferay.portal.kernel.service.RoleLocalService;
import com.liferay.portal.kernel.servlet.taglib.ui.BreadcrumbEntry;
import com.liferay.portal.kernel.theme.ThemeDisplay;
import com.liferay.portal.kernel.util.GetterUtil;
import com.liferay.portal.kernel.util.JavaConstants;
import com.liferay.portal.kernel.util.LinkedHashMapBuilder;
import com.liferay.portal.kernel.util.OrderByComparator;
import com.liferay.portal.kernel.util.ParamUtil;
import com.liferay.portal.kernel.util.Portal;
import com.liferay.portal.kernel.util.Validator;
import com.liferay.portal.kernel.util.WebKeys;
import com.liferay.portal.kernel.workflow.WorkflowConstants;
import com.liferay.portal.search.constants.SearchContextAttributes;
import com.liferay.portal.search.searcher.SearchResponse;
import com.liferay.site.navigation.taglib.servlet.taglib.util.BreadcrumbEntryBuilder;
import com.liferay.site.navigation.taglib.servlet.taglib.util.BreadcrumbEntryListBuilder;
import com.liferay.staging.StagingGroupHelper;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Locale;
import java.util.Objects;
import javax.portlet.PortletException;
import javax.portlet.PortletRequest;
import javax.portlet.PortletResponse;
import javax.portlet.PortletURL;
import javax.portlet.RenderResponse;
import javax.servlet.http.HttpServletRequest;
/**
* @author Eudaldo Alonso
*/
public class JournalArticleItemSelectorViewDisplayContext {
public JournalArticleItemSelectorViewDisplayContext(
HttpServletRequest httpServletRequest,
InfoItemItemSelectorCriterion infoItemItemSelectorCriterion,
String itemSelectedEventName,
JournalArticleItemSelectorView journalArticleItemSelectorView,
JournalWebConfiguration journalWebConfiguration, Portal portal,
PortletURL portletURL,
ResourcePermissionLocalService resourcePermissionLocalService,
RoleLocalService roleLocalService, boolean search,
StagingGroupHelper stagingGroupHelper) {
_httpServletRequest = httpServletRequest;
_infoItemItemSelectorCriterion = infoItemItemSelectorCriterion;
_itemSelectedEventName = itemSelectedEventName;
_journalArticleItemSelectorView = journalArticleItemSelectorView;
_journalWebConfiguration = journalWebConfiguration;
_portal = portal;
_portletURL = portletURL;
_resourcePermissionLocalService = resourcePermissionLocalService;
_roleLocalService = roleLocalService;
_search = search;
_stagingGroupHelper = stagingGroupHelper;
_portletRequest = (PortletRequest)httpServletRequest.getAttribute(
JavaConstants.JAVAX_PORTLET_REQUEST);
_portletResponse = (RenderResponse)httpServletRequest.getAttribute(
JavaConstants.JAVAX_PORTLET_RESPONSE);
_themeDisplay = (ThemeDisplay)httpServletRequest.getAttribute(
WebKeys.THEME_DISPLAY);
}
public String getDisplayStyle() {
if (Validator.isNotNull(_displayStyle)) {
return _displayStyle;
}
_displayStyle = SearchDisplayStyleUtil.getDisplayStyle(
_httpServletRequest, JournalPortletKeys.JOURNAL,
"item-selector-display-style", "descriptive");
return _displayStyle;
}
public String getGroupCssIcon(long groupId) throws PortalException {
Group group = GroupServiceUtil.getGroup(groupId);
return group.getIconCssClass();
}
public String getGroupLabel(long groupId, Locale locale)
throws PortalException {
Group group = GroupServiceUtil.getGroup(groupId);
return group.getDescriptiveName(locale);
}
public String getItemSelectedEventName() {
return _itemSelectedEventName;
}
public String getKeywords() {
if (_keywords != null) {
return _keywords;
}
_keywords = ParamUtil.getString(_httpServletRequest, "keywords");
return _keywords;
}
public JournalArticle getLatestArticle(JournalArticle journalArticle) {
JournalArticle latestArticle =
JournalArticleLocalServiceUtil.fetchLatestArticle(
journalArticle.getGroupId(), journalArticle.getArticleId(),
WorkflowConstants.STATUS_ANY);
if (latestArticle != null) {
return latestArticle;
}
return journalArticle;
}
public String getPayload(JournalArticle journalArticle)
throws PortalException {
AssetEntry assetEntry = AssetEntryLocalServiceUtil.fetchEntry(
JournalArticle.class.getName(),
JournalArticleAssetRenderer.getClassPK(journalArticle));
DDMStructure ddmStructure = DDMStructureLocalServiceUtil.fetchStructure(
journalArticle.getDDMStructureId());
return JSONUtil.put(
"assetEntryId", String.valueOf(assetEntry.getEntryId())
).put(
"assetType",
() -> {
AssetRendererFactory> assetRendererFactory =
AssetRendererFactoryRegistryUtil.
getAssetRendererFactoryByClassName(
JournalArticle.class.getName());
if (!assetRendererFactory.isSupportsClassTypes()) {
return assetRendererFactory.getTypeName(
_themeDisplay.getLocale(), assetEntry.getClassTypeId());
}
ClassTypeReader classTypeReader =
assetRendererFactory.getClassTypeReader();
ClassType classType = classTypeReader.getClassType(
assetEntry.getClassTypeId(), _themeDisplay.getLocale());
return classType.getName();
}
).put(
"className", JournalArticle.class.getName()
).put(
"classNameId", _getJournalArticleClassNameId()
).put(
"classPK", journalArticle.getResourcePrimKey()
).put(
"classTypeId", _getClassTypeId(ddmStructure)
).put(
"groupDescriptiveName",
() -> {
Group group = GroupLocalServiceUtil.fetchGroup(
assetEntry.getGroupId());
return group.getDescriptiveName(_themeDisplay.getLocale());
}
).put(
"subtype", _getSubtype(ddmStructure)
).put(
"title", journalArticle.getTitle(_themeDisplay.getLocale(), true)
).put(
"titleMap", journalArticle.getTitleMap()
).put(
"type",
ResourceActionsUtil.getModelResource(
_themeDisplay.getLocale(), JournalArticle.class.getName())
).toString();
}
public List getPortletBreadcrumbEntries() {
JournalFolder folder = _getFolder();
return BreadcrumbEntryListBuilder.add(
breadcrumbEntry -> {
breadcrumbEntry.setTitle(
LanguageUtil.get(
_httpServletRequest, "sites-and-libraries"));
breadcrumbEntry.setURL(
PortletURLBuilder.create(
getPortletURL()
).setParameter(
"showGroupSelector", true
).buildString());
}
).add(
breadcrumbEntry -> {
Group group = GroupLocalServiceUtil.getGroup(_getGroupId());
breadcrumbEntry.setTitle(
group.getDescriptiveName(_themeDisplay.getLocale()));
breadcrumbEntry.setURL(
PortletURLBuilder.create(
getPortletURL()
).setParameter(
"folderId",
JournalFolderConstants.DEFAULT_PARENT_FOLDER_ID
).buildString());
}
).addAll(
() -> folder != null,
() -> {
List ancestorFolders = folder.getAncestors();
Collections.reverse(ancestorFolders);
return TransformUtil.transform(
ancestorFolders,
ancestorFolder -> BreadcrumbEntryBuilder.setTitle(
ancestorFolder.getName()
).setURL(
PortletURLBuilder.create(
getPortletURL()
).setParameter(
"folderId", ancestorFolder.getFolderId()
).buildString()
).build());
}
).add(
() -> folder != null,
breadcrumbEntry -> {
JournalFolder unescapedFolder = folder.toUnescapedModel();
breadcrumbEntry.setTitle(unescapedFolder.getName());
}
).build();
}
public PortletURL getPortletURL() throws PortletException {
return PortletURLBuilder.create(
PortletURLUtil.clone(
_portletURL,
_portal.getLiferayPortletResponse(_portletResponse))
).setParameter(
"displayStyle", getDisplayStyle()
).setParameter(
"groupType", "site"
).setParameter(
"scopeGroupType",
ParamUtil.getBoolean(_httpServletRequest, "scopeGroupType")
).setParameter(
"selectedTab", _getTitle(_httpServletRequest.getLocale())
).buildPortletURL();
}
public String getReturnType() {
return InfoItemItemSelectorReturnType.class.getName();
}
public SearchContainer> getSearchContainer() throws Exception {
if (_articleSearchContainer != null) {
return _articleSearchContainer;
}
PortletURL portletURL = PortletURLBuilder.create(
getPortletURL()
).setParameter(
"folderId", _getFolderId()
).setParameter(
"scope", _getScopeFilter()
).buildPortletURL();
SearchContainer
© 2015 - 2024 Weber Informatics LLC | Privacy Policy