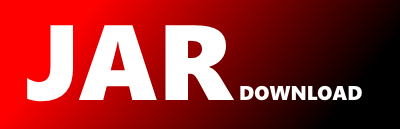
com.liferay.layout.util.structure.collection.EmptyCollectionOptions Maven / Gradle / Ivy
/**
* SPDX-FileCopyrightText: (c) 2000 Liferay, Inc. https://liferay.com
* SPDX-License-Identifier: LGPL-2.1-or-later OR LicenseRef-Liferay-DXP-EULA-2.0.0-2023-06
*/
package com.liferay.layout.util.structure.collection;
import com.liferay.petra.function.UnsafeSupplier;
import com.liferay.portal.kernel.json.JSONFactoryUtil;
import com.liferay.portal.kernel.json.JSONObject;
import com.liferay.portal.kernel.json.JSONUtil;
import java.util.HashMap;
import java.util.Map;
import java.util.Objects;
/**
* @author Rubén Pulido
*/
public class EmptyCollectionOptions {
public static EmptyCollectionOptions of(JSONObject jsonObject) {
if ((jsonObject == null) || (jsonObject.length() == 0)) {
return null;
}
return new EmptyCollectionOptions() {
{
setDisplayMessage(
() -> {
if (jsonObject.has("displayMessage")) {
return jsonObject.getBoolean("displayMessage");
}
return null;
});
setMessage(
() -> {
if (jsonObject.has("message")) {
return JSONUtil.toStringMap(
jsonObject.getJSONObject("message"));
}
return null;
});
}
};
}
@Override
public boolean equals(Object object) {
if (this == object) {
return true;
}
if (!(object instanceof EmptyCollectionOptions)) {
return false;
}
EmptyCollectionOptions emptyCollectionOptions =
(EmptyCollectionOptions)object;
if (!Objects.equals(
_displayMessage, emptyCollectionOptions._displayMessage) ||
!Objects.equals(_message, emptyCollectionOptions._message)) {
return false;
}
return super.equals(object);
}
public Map getMessage() {
return _message;
}
@Override
public int hashCode() {
String string = toString();
return string.hashCode();
}
public Boolean isDisplayMessage() {
return _displayMessage;
}
public void setDisplayMessage(Boolean displayMessage) {
_displayMessage = displayMessage;
}
public void setDisplayMessage(
UnsafeSupplier displayMessageUnsafeSupplier) {
try {
_displayMessage = displayMessageUnsafeSupplier.get();
}
catch (RuntimeException runtimeException) {
throw runtimeException;
}
catch (Exception exception) {
throw new RuntimeException(exception);
}
}
public void setMessage(Map message) {
_message = message;
}
public void setMessage(
UnsafeSupplier
© 2015 - 2024 Weber Informatics LLC | Privacy Policy