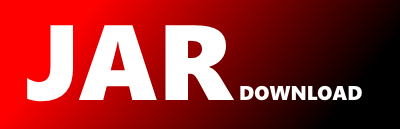
com.liferay.message.boards.service.impl.MBStatsUserLocalServiceImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.liferay.message.boards.service
Show all versions of com.liferay.message.boards.service
Liferay Message Boards Service
/**
* Copyright (c) 2000-present Liferay, Inc. All rights reserved.
*
* This library is free software; you can redistribute it and/or modify it under
* the terms of the GNU Lesser General Public License as published by the Free
* Software Foundation; either version 2.1 of the License, or (at your option)
* any later version.
*
* This library is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License for more
* details.
*/
package com.liferay.message.boards.service.impl;
import com.liferay.message.boards.constants.MBCategoryConstants;
import com.liferay.message.boards.model.MBMessageTable;
import com.liferay.message.boards.model.MBStatsUser;
import com.liferay.message.boards.model.MBThreadTable;
import com.liferay.message.boards.model.impl.MBStatsUserImpl;
import com.liferay.message.boards.service.base.MBStatsUserLocalServiceBaseImpl;
import com.liferay.message.boards.service.persistence.MBMessagePersistence;
import com.liferay.message.boards.service.persistence.MBThreadPersistence;
import com.liferay.message.boards.settings.MBGroupServiceSettings;
import com.liferay.petra.sql.dsl.DSLFunctionFactoryUtil;
import com.liferay.petra.sql.dsl.DSLQueryFactoryUtil;
import com.liferay.petra.sql.dsl.expression.Expression;
import com.liferay.petra.string.CharPool;
import com.liferay.petra.string.StringPool;
import com.liferay.portal.aop.AopService;
import com.liferay.portal.kernel.exception.PortalException;
import com.liferay.portal.kernel.log.Log;
import com.liferay.portal.kernel.log.LogFactoryUtil;
import com.liferay.portal.kernel.model.Group;
import com.liferay.portal.kernel.model.Organization;
import com.liferay.portal.kernel.model.Role;
import com.liferay.portal.kernel.model.User;
import com.liferay.portal.kernel.model.UserGroup;
import com.liferay.portal.kernel.service.GroupLocalService;
import com.liferay.portal.kernel.service.OrganizationLocalService;
import com.liferay.portal.kernel.service.RoleLocalService;
import com.liferay.portal.kernel.service.UserGroupLocalService;
import com.liferay.portal.kernel.service.UserGroupRoleLocalService;
import com.liferay.portal.kernel.service.UserLocalService;
import com.liferay.portal.kernel.util.GetterUtil;
import com.liferay.portal.kernel.util.StringUtil;
import com.liferay.portal.kernel.workflow.WorkflowConstants;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import org.osgi.service.component.annotations.Component;
import org.osgi.service.component.annotations.Reference;
/**
* @author Brian Wing Shun Chan
*/
@Component(
property = "model.class.name=com.liferay.message.boards.model.MBStatsUser",
service = AopService.class
)
public class MBStatsUserLocalServiceImpl
extends MBStatsUserLocalServiceBaseImpl {
@Override
public Date getLastPostDateByUserId(long groupId, long userId) {
List results = _mbThreadPersistence.dslQuery(
DSLQueryFactoryUtil.select(
DSLFunctionFactoryUtil.max(
MBThreadTable.INSTANCE.lastPostDate
).as(
"maxLastPostDate"
)
).from(
MBThreadTable.INSTANCE
).where(
MBThreadTable.INSTANCE.userId.eq(
userId
).and(
MBThreadTable.INSTANCE.status.neq(
WorkflowConstants.STATUS_IN_TRASH)
)
));
return results.get(0);
}
@Override
public int getMessageCount(long groupId, long userId) {
return _mbMessagePersistence.countByG_U_S(
groupId, userId, WorkflowConstants.STATUS_APPROVED);
}
@Override
public long getMessageCountByGroupId(long groupId) throws PortalException {
Group group = _groupLocalService.getGroup(groupId);
return _mbMessagePersistence.dslQuery(
DSLQueryFactoryUtil.count(
).from(
MBMessageTable.INSTANCE
).where(
MBMessageTable.INSTANCE.groupId.eq(
groupId
).and(
MBMessageTable.INSTANCE.userId.neq(
_userLocalService.getDefaultUserId(
group.getCompanyId()))
).and(
MBMessageTable.INSTANCE.categoryId.neq(
MBCategoryConstants.DISCUSSION_CATEGORY_ID)
).and(
MBMessageTable.INSTANCE.status.eq(
WorkflowConstants.STATUS_APPROVED)
)
));
}
@Override
public long getMessageCountByUserId(long userId) {
return _mbMessagePersistence.dslQuery(
DSLQueryFactoryUtil.count(
).from(
MBMessageTable.INSTANCE
).where(
MBMessageTable.INSTANCE.userId.eq(
userId
).and(
MBMessageTable.INSTANCE.categoryId.neq(
MBCategoryConstants.DISCUSSION_CATEGORY_ID)
).and(
MBMessageTable.INSTANCE.status.eq(
WorkflowConstants.STATUS_APPROVED)
)
));
}
@Override
public List getStatsUsersByGroupId(
long groupId, int start, int end)
throws PortalException {
Group group = _groupLocalService.getGroup(groupId);
long defaultUserId = _userLocalService.getDefaultUserId(
group.getCompanyId());
Expression countExpression = DSLFunctionFactoryUtil.count(
MBMessageTable.INSTANCE.messageId
).as(
"messageCount"
);
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy