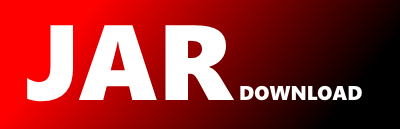
com.liferay.message.boards.service.persistence.impl.MBMessagePersistenceImpl Maven / Gradle / Ivy
Show all versions of com.liferay.message.boards.service
/**
* Copyright (c) 2000-present Liferay, Inc. All rights reserved.
*
* This library is free software; you can redistribute it and/or modify it under
* the terms of the GNU Lesser General Public License as published by the Free
* Software Foundation; either version 2.1 of the License, or (at your option)
* any later version.
*
* This library is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License for more
* details.
*/
package com.liferay.message.boards.service.persistence.impl;
import com.liferay.message.boards.exception.DuplicateMBMessageExternalReferenceCodeException;
import com.liferay.message.boards.exception.NoSuchMessageException;
import com.liferay.message.boards.model.MBMessage;
import com.liferay.message.boards.model.MBMessageTable;
import com.liferay.message.boards.model.impl.MBMessageImpl;
import com.liferay.message.boards.model.impl.MBMessageModelImpl;
import com.liferay.message.boards.service.persistence.MBMessagePersistence;
import com.liferay.message.boards.service.persistence.MBMessageUtil;
import com.liferay.message.boards.service.persistence.impl.constants.MBPersistenceConstants;
import com.liferay.petra.string.StringBundler;
import com.liferay.portal.kernel.change.tracking.CTColumnResolutionType;
import com.liferay.portal.kernel.configuration.Configuration;
import com.liferay.portal.kernel.dao.orm.EntityCache;
import com.liferay.portal.kernel.dao.orm.FinderCache;
import com.liferay.portal.kernel.dao.orm.FinderPath;
import com.liferay.portal.kernel.dao.orm.Query;
import com.liferay.portal.kernel.dao.orm.QueryPos;
import com.liferay.portal.kernel.dao.orm.QueryUtil;
import com.liferay.portal.kernel.dao.orm.SQLQuery;
import com.liferay.portal.kernel.dao.orm.Session;
import com.liferay.portal.kernel.dao.orm.SessionFactory;
import com.liferay.portal.kernel.exception.SystemException;
import com.liferay.portal.kernel.log.Log;
import com.liferay.portal.kernel.log.LogFactoryUtil;
import com.liferay.portal.kernel.sanitizer.Sanitizer;
import com.liferay.portal.kernel.sanitizer.SanitizerException;
import com.liferay.portal.kernel.sanitizer.SanitizerUtil;
import com.liferay.portal.kernel.security.auth.CompanyThreadLocal;
import com.liferay.portal.kernel.security.auth.PrincipalThreadLocal;
import com.liferay.portal.kernel.security.permission.InlineSQLHelperUtil;
import com.liferay.portal.kernel.service.ServiceContext;
import com.liferay.portal.kernel.service.ServiceContextThreadLocal;
import com.liferay.portal.kernel.service.persistence.change.tracking.helper.CTPersistenceHelper;
import com.liferay.portal.kernel.service.persistence.impl.BasePersistenceImpl;
import com.liferay.portal.kernel.util.ArrayUtil;
import com.liferay.portal.kernel.util.ContentTypes;
import com.liferay.portal.kernel.util.GetterUtil;
import com.liferay.portal.kernel.util.OrderByComparator;
import com.liferay.portal.kernel.util.PropsKeys;
import com.liferay.portal.kernel.util.PropsUtil;
import com.liferay.portal.kernel.util.ProxyUtil;
import com.liferay.portal.kernel.util.SetUtil;
import com.liferay.portal.kernel.util.StringUtil;
import com.liferay.portal.kernel.util.Validator;
import com.liferay.portal.kernel.uuid.PortalUUID;
import java.io.Serializable;
import java.lang.reflect.InvocationHandler;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Date;
import java.util.EnumMap;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Set;
import javax.sql.DataSource;
import org.osgi.service.component.annotations.Activate;
import org.osgi.service.component.annotations.Component;
import org.osgi.service.component.annotations.Deactivate;
import org.osgi.service.component.annotations.Reference;
/**
* The persistence implementation for the message-boards message service.
*
*
* Caching information and settings can be found in portal.properties
*
*
* @author Brian Wing Shun Chan
* @generated
*/
@Component(service = MBMessagePersistence.class)
public class MBMessagePersistenceImpl
extends BasePersistenceImpl implements MBMessagePersistence {
/*
* NOTE FOR DEVELOPERS:
*
* Never modify or reference this class directly. Always use MBMessageUtil
to access the message-boards message persistence. Modify service.xml
and rerun ServiceBuilder to regenerate this class.
*/
public static final String FINDER_CLASS_NAME_ENTITY =
MBMessageImpl.class.getName();
public static final String FINDER_CLASS_NAME_LIST_WITH_PAGINATION =
FINDER_CLASS_NAME_ENTITY + ".List1";
public static final String FINDER_CLASS_NAME_LIST_WITHOUT_PAGINATION =
FINDER_CLASS_NAME_ENTITY + ".List2";
private FinderPath _finderPathWithPaginationFindAll;
private FinderPath _finderPathWithoutPaginationFindAll;
private FinderPath _finderPathCountAll;
private FinderPath _finderPathWithPaginationFindByUuid;
private FinderPath _finderPathWithoutPaginationFindByUuid;
private FinderPath _finderPathCountByUuid;
/**
* Returns all the message-boards messages where uuid = ?.
*
* @param uuid the uuid
* @return the matching message-boards messages
*/
@Override
public List findByUuid(String uuid) {
return findByUuid(uuid, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the message-boards messages where uuid = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from MBMessageModelImpl
.
*
*
* @param uuid the uuid
* @param start the lower bound of the range of message-boards messages
* @param end the upper bound of the range of message-boards messages (not inclusive)
* @return the range of matching message-boards messages
*/
@Override
public List findByUuid(String uuid, int start, int end) {
return findByUuid(uuid, start, end, null);
}
/**
* Returns an ordered range of all the message-boards messages where uuid = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from MBMessageModelImpl
.
*
*
* @param uuid the uuid
* @param start the lower bound of the range of message-boards messages
* @param end the upper bound of the range of message-boards messages (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching message-boards messages
*/
@Override
public List findByUuid(
String uuid, int start, int end,
OrderByComparator orderByComparator) {
return findByUuid(uuid, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the message-boards messages where uuid = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from MBMessageModelImpl
.
*
*
* @param uuid the uuid
* @param start the lower bound of the range of message-boards messages
* @param end the upper bound of the range of message-boards messages (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching message-boards messages
*/
@Override
public List findByUuid(
String uuid, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
uuid = Objects.toString(uuid, "");
boolean productionMode = ctPersistenceHelper.isProductionMode(
MBMessage.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache && productionMode) {
finderPath = _finderPathWithoutPaginationFindByUuid;
finderArgs = new Object[] {uuid};
}
}
else if (useFinderCache && productionMode) {
finderPath = _finderPathWithPaginationFindByUuid;
finderArgs = new Object[] {uuid, start, end, orderByComparator};
}
List list = null;
if (useFinderCache && productionMode) {
list = (List)finderCache.getResult(
finderPath, finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (MBMessage mbMessage : list) {
if (!uuid.equals(mbMessage.getUuid())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
3 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(3);
}
sb.append(_SQL_SELECT_MBMESSAGE_WHERE);
boolean bindUuid = false;
if (uuid.isEmpty()) {
sb.append(_FINDER_COLUMN_UUID_UUID_3);
}
else {
bindUuid = true;
sb.append(_FINDER_COLUMN_UUID_UUID_2);
}
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(MBMessageModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
if (bindUuid) {
queryPos.add(uuid);
}
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache && productionMode) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first message-boards message in the ordered set where uuid = ?.
*
* @param uuid the uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching message-boards message
* @throws NoSuchMessageException if a matching message-boards message could not be found
*/
@Override
public MBMessage findByUuid_First(
String uuid, OrderByComparator orderByComparator)
throws NoSuchMessageException {
MBMessage mbMessage = fetchByUuid_First(uuid, orderByComparator);
if (mbMessage != null) {
return mbMessage;
}
StringBundler sb = new StringBundler(4);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("uuid=");
sb.append(uuid);
sb.append("}");
throw new NoSuchMessageException(sb.toString());
}
/**
* Returns the first message-boards message in the ordered set where uuid = ?.
*
* @param uuid the uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching message-boards message, or null
if a matching message-boards message could not be found
*/
@Override
public MBMessage fetchByUuid_First(
String uuid, OrderByComparator orderByComparator) {
List list = findByUuid(uuid, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last message-boards message in the ordered set where uuid = ?.
*
* @param uuid the uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching message-boards message
* @throws NoSuchMessageException if a matching message-boards message could not be found
*/
@Override
public MBMessage findByUuid_Last(
String uuid, OrderByComparator orderByComparator)
throws NoSuchMessageException {
MBMessage mbMessage = fetchByUuid_Last(uuid, orderByComparator);
if (mbMessage != null) {
return mbMessage;
}
StringBundler sb = new StringBundler(4);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("uuid=");
sb.append(uuid);
sb.append("}");
throw new NoSuchMessageException(sb.toString());
}
/**
* Returns the last message-boards message in the ordered set where uuid = ?.
*
* @param uuid the uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching message-boards message, or null
if a matching message-boards message could not be found
*/
@Override
public MBMessage fetchByUuid_Last(
String uuid, OrderByComparator orderByComparator) {
int count = countByUuid(uuid);
if (count == 0) {
return null;
}
List list = findByUuid(
uuid, count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the message-boards messages before and after the current message-boards message in the ordered set where uuid = ?.
*
* @param messageId the primary key of the current message-boards message
* @param uuid the uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next message-boards message
* @throws NoSuchMessageException if a message-boards message with the primary key could not be found
*/
@Override
public MBMessage[] findByUuid_PrevAndNext(
long messageId, String uuid,
OrderByComparator orderByComparator)
throws NoSuchMessageException {
uuid = Objects.toString(uuid, "");
MBMessage mbMessage = findByPrimaryKey(messageId);
Session session = null;
try {
session = openSession();
MBMessage[] array = new MBMessageImpl[3];
array[0] = getByUuid_PrevAndNext(
session, mbMessage, uuid, orderByComparator, true);
array[1] = mbMessage;
array[2] = getByUuid_PrevAndNext(
session, mbMessage, uuid, orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected MBMessage getByUuid_PrevAndNext(
Session session, MBMessage mbMessage, String uuid,
OrderByComparator orderByComparator, boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(3);
}
sb.append(_SQL_SELECT_MBMESSAGE_WHERE);
boolean bindUuid = false;
if (uuid.isEmpty()) {
sb.append(_FINDER_COLUMN_UUID_UUID_3);
}
else {
bindUuid = true;
sb.append(_FINDER_COLUMN_UUID_UUID_2);
}
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(MBMessageModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
if (bindUuid) {
queryPos.add(uuid);
}
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(mbMessage)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the message-boards messages where uuid = ? from the database.
*
* @param uuid the uuid
*/
@Override
public void removeByUuid(String uuid) {
for (MBMessage mbMessage :
findByUuid(uuid, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null)) {
remove(mbMessage);
}
}
/**
* Returns the number of message-boards messages where uuid = ?.
*
* @param uuid the uuid
* @return the number of matching message-boards messages
*/
@Override
public int countByUuid(String uuid) {
uuid = Objects.toString(uuid, "");
boolean productionMode = ctPersistenceHelper.isProductionMode(
MBMessage.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
Long count = null;
if (productionMode) {
finderPath = _finderPathCountByUuid;
finderArgs = new Object[] {uuid};
count = (Long)finderCache.getResult(finderPath, finderArgs, this);
}
if (count == null) {
StringBundler sb = new StringBundler(2);
sb.append(_SQL_COUNT_MBMESSAGE_WHERE);
boolean bindUuid = false;
if (uuid.isEmpty()) {
sb.append(_FINDER_COLUMN_UUID_UUID_3);
}
else {
bindUuid = true;
sb.append(_FINDER_COLUMN_UUID_UUID_2);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
if (bindUuid) {
queryPos.add(uuid);
}
count = (Long)query.uniqueResult();
if (productionMode) {
finderCache.putResult(finderPath, finderArgs, count);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String _FINDER_COLUMN_UUID_UUID_2 =
"mbMessage.uuid = ?";
private static final String _FINDER_COLUMN_UUID_UUID_3 =
"(mbMessage.uuid IS NULL OR mbMessage.uuid = '')";
private FinderPath _finderPathFetchByUUID_G;
private FinderPath _finderPathCountByUUID_G;
/**
* Returns the message-boards message where uuid = ? and groupId = ? or throws a NoSuchMessageException
if it could not be found.
*
* @param uuid the uuid
* @param groupId the group ID
* @return the matching message-boards message
* @throws NoSuchMessageException if a matching message-boards message could not be found
*/
@Override
public MBMessage findByUUID_G(String uuid, long groupId)
throws NoSuchMessageException {
MBMessage mbMessage = fetchByUUID_G(uuid, groupId);
if (mbMessage == null) {
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("uuid=");
sb.append(uuid);
sb.append(", groupId=");
sb.append(groupId);
sb.append("}");
if (_log.isDebugEnabled()) {
_log.debug(sb.toString());
}
throw new NoSuchMessageException(sb.toString());
}
return mbMessage;
}
/**
* Returns the message-boards message where uuid = ? and groupId = ? or returns null
if it could not be found. Uses the finder cache.
*
* @param uuid the uuid
* @param groupId the group ID
* @return the matching message-boards message, or null
if a matching message-boards message could not be found
*/
@Override
public MBMessage fetchByUUID_G(String uuid, long groupId) {
return fetchByUUID_G(uuid, groupId, true);
}
/**
* Returns the message-boards message where uuid = ? and groupId = ? or returns null
if it could not be found, optionally using the finder cache.
*
* @param uuid the uuid
* @param groupId the group ID
* @param useFinderCache whether to use the finder cache
* @return the matching message-boards message, or null
if a matching message-boards message could not be found
*/
@Override
public MBMessage fetchByUUID_G(
String uuid, long groupId, boolean useFinderCache) {
uuid = Objects.toString(uuid, "");
boolean productionMode = ctPersistenceHelper.isProductionMode(
MBMessage.class);
Object[] finderArgs = null;
if (useFinderCache && productionMode) {
finderArgs = new Object[] {uuid, groupId};
}
Object result = null;
if (useFinderCache && productionMode) {
result = finderCache.getResult(
_finderPathFetchByUUID_G, finderArgs, this);
}
if (result instanceof MBMessage) {
MBMessage mbMessage = (MBMessage)result;
if (!Objects.equals(uuid, mbMessage.getUuid()) ||
(groupId != mbMessage.getGroupId())) {
result = null;
}
}
if (result == null) {
StringBundler sb = new StringBundler(4);
sb.append(_SQL_SELECT_MBMESSAGE_WHERE);
boolean bindUuid = false;
if (uuid.isEmpty()) {
sb.append(_FINDER_COLUMN_UUID_G_UUID_3);
}
else {
bindUuid = true;
sb.append(_FINDER_COLUMN_UUID_G_UUID_2);
}
sb.append(_FINDER_COLUMN_UUID_G_GROUPID_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
if (bindUuid) {
queryPos.add(uuid);
}
queryPos.add(groupId);
List list = query.list();
if (list.isEmpty()) {
if (useFinderCache && productionMode) {
finderCache.putResult(
_finderPathFetchByUUID_G, finderArgs, list);
}
}
else {
MBMessage mbMessage = list.get(0);
result = mbMessage;
cacheResult(mbMessage);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
if (result instanceof List>) {
return null;
}
else {
return (MBMessage)result;
}
}
/**
* Removes the message-boards message where uuid = ? and groupId = ? from the database.
*
* @param uuid the uuid
* @param groupId the group ID
* @return the message-boards message that was removed
*/
@Override
public MBMessage removeByUUID_G(String uuid, long groupId)
throws NoSuchMessageException {
MBMessage mbMessage = findByUUID_G(uuid, groupId);
return remove(mbMessage);
}
/**
* Returns the number of message-boards messages where uuid = ? and groupId = ?.
*
* @param uuid the uuid
* @param groupId the group ID
* @return the number of matching message-boards messages
*/
@Override
public int countByUUID_G(String uuid, long groupId) {
uuid = Objects.toString(uuid, "");
boolean productionMode = ctPersistenceHelper.isProductionMode(
MBMessage.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
Long count = null;
if (productionMode) {
finderPath = _finderPathCountByUUID_G;
finderArgs = new Object[] {uuid, groupId};
count = (Long)finderCache.getResult(finderPath, finderArgs, this);
}
if (count == null) {
StringBundler sb = new StringBundler(3);
sb.append(_SQL_COUNT_MBMESSAGE_WHERE);
boolean bindUuid = false;
if (uuid.isEmpty()) {
sb.append(_FINDER_COLUMN_UUID_G_UUID_3);
}
else {
bindUuid = true;
sb.append(_FINDER_COLUMN_UUID_G_UUID_2);
}
sb.append(_FINDER_COLUMN_UUID_G_GROUPID_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
if (bindUuid) {
queryPos.add(uuid);
}
queryPos.add(groupId);
count = (Long)query.uniqueResult();
if (productionMode) {
finderCache.putResult(finderPath, finderArgs, count);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String _FINDER_COLUMN_UUID_G_UUID_2 =
"mbMessage.uuid = ? AND ";
private static final String _FINDER_COLUMN_UUID_G_UUID_3 =
"(mbMessage.uuid IS NULL OR mbMessage.uuid = '') AND ";
private static final String _FINDER_COLUMN_UUID_G_GROUPID_2 =
"mbMessage.groupId = ?";
private FinderPath _finderPathWithPaginationFindByUuid_C;
private FinderPath _finderPathWithoutPaginationFindByUuid_C;
private FinderPath _finderPathCountByUuid_C;
/**
* Returns all the message-boards messages where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @return the matching message-boards messages
*/
@Override
public List findByUuid_C(String uuid, long companyId) {
return findByUuid_C(
uuid, companyId, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the message-boards messages where uuid = ? and companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from MBMessageModelImpl
.
*
*
* @param uuid the uuid
* @param companyId the company ID
* @param start the lower bound of the range of message-boards messages
* @param end the upper bound of the range of message-boards messages (not inclusive)
* @return the range of matching message-boards messages
*/
@Override
public List findByUuid_C(
String uuid, long companyId, int start, int end) {
return findByUuid_C(uuid, companyId, start, end, null);
}
/**
* Returns an ordered range of all the message-boards messages where uuid = ? and companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from MBMessageModelImpl
.
*
*
* @param uuid the uuid
* @param companyId the company ID
* @param start the lower bound of the range of message-boards messages
* @param end the upper bound of the range of message-boards messages (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching message-boards messages
*/
@Override
public List findByUuid_C(
String uuid, long companyId, int start, int end,
OrderByComparator orderByComparator) {
return findByUuid_C(
uuid, companyId, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the message-boards messages where uuid = ? and companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from MBMessageModelImpl
.
*
*
* @param uuid the uuid
* @param companyId the company ID
* @param start the lower bound of the range of message-boards messages
* @param end the upper bound of the range of message-boards messages (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching message-boards messages
*/
@Override
public List findByUuid_C(
String uuid, long companyId, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
uuid = Objects.toString(uuid, "");
boolean productionMode = ctPersistenceHelper.isProductionMode(
MBMessage.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache && productionMode) {
finderPath = _finderPathWithoutPaginationFindByUuid_C;
finderArgs = new Object[] {uuid, companyId};
}
}
else if (useFinderCache && productionMode) {
finderPath = _finderPathWithPaginationFindByUuid_C;
finderArgs = new Object[] {
uuid, companyId, start, end, orderByComparator
};
}
List list = null;
if (useFinderCache && productionMode) {
list = (List)finderCache.getResult(
finderPath, finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (MBMessage mbMessage : list) {
if (!uuid.equals(mbMessage.getUuid()) ||
(companyId != mbMessage.getCompanyId())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_MBMESSAGE_WHERE);
boolean bindUuid = false;
if (uuid.isEmpty()) {
sb.append(_FINDER_COLUMN_UUID_C_UUID_3);
}
else {
bindUuid = true;
sb.append(_FINDER_COLUMN_UUID_C_UUID_2);
}
sb.append(_FINDER_COLUMN_UUID_C_COMPANYID_2);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(MBMessageModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
if (bindUuid) {
queryPos.add(uuid);
}
queryPos.add(companyId);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache && productionMode) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first message-boards message in the ordered set where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching message-boards message
* @throws NoSuchMessageException if a matching message-boards message could not be found
*/
@Override
public MBMessage findByUuid_C_First(
String uuid, long companyId,
OrderByComparator orderByComparator)
throws NoSuchMessageException {
MBMessage mbMessage = fetchByUuid_C_First(
uuid, companyId, orderByComparator);
if (mbMessage != null) {
return mbMessage;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("uuid=");
sb.append(uuid);
sb.append(", companyId=");
sb.append(companyId);
sb.append("}");
throw new NoSuchMessageException(sb.toString());
}
/**
* Returns the first message-boards message in the ordered set where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching message-boards message, or null
if a matching message-boards message could not be found
*/
@Override
public MBMessage fetchByUuid_C_First(
String uuid, long companyId,
OrderByComparator orderByComparator) {
List list = findByUuid_C(
uuid, companyId, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last message-boards message in the ordered set where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching message-boards message
* @throws NoSuchMessageException if a matching message-boards message could not be found
*/
@Override
public MBMessage findByUuid_C_Last(
String uuid, long companyId,
OrderByComparator orderByComparator)
throws NoSuchMessageException {
MBMessage mbMessage = fetchByUuid_C_Last(
uuid, companyId, orderByComparator);
if (mbMessage != null) {
return mbMessage;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("uuid=");
sb.append(uuid);
sb.append(", companyId=");
sb.append(companyId);
sb.append("}");
throw new NoSuchMessageException(sb.toString());
}
/**
* Returns the last message-boards message in the ordered set where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching message-boards message, or null
if a matching message-boards message could not be found
*/
@Override
public MBMessage fetchByUuid_C_Last(
String uuid, long companyId,
OrderByComparator orderByComparator) {
int count = countByUuid_C(uuid, companyId);
if (count == 0) {
return null;
}
List list = findByUuid_C(
uuid, companyId, count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the message-boards messages before and after the current message-boards message in the ordered set where uuid = ? and companyId = ?.
*
* @param messageId the primary key of the current message-boards message
* @param uuid the uuid
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next message-boards message
* @throws NoSuchMessageException if a message-boards message with the primary key could not be found
*/
@Override
public MBMessage[] findByUuid_C_PrevAndNext(
long messageId, String uuid, long companyId,
OrderByComparator orderByComparator)
throws NoSuchMessageException {
uuid = Objects.toString(uuid, "");
MBMessage mbMessage = findByPrimaryKey(messageId);
Session session = null;
try {
session = openSession();
MBMessage[] array = new MBMessageImpl[3];
array[0] = getByUuid_C_PrevAndNext(
session, mbMessage, uuid, companyId, orderByComparator, true);
array[1] = mbMessage;
array[2] = getByUuid_C_PrevAndNext(
session, mbMessage, uuid, companyId, orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected MBMessage getByUuid_C_PrevAndNext(
Session session, MBMessage mbMessage, String uuid, long companyId,
OrderByComparator orderByComparator, boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
5 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_MBMESSAGE_WHERE);
boolean bindUuid = false;
if (uuid.isEmpty()) {
sb.append(_FINDER_COLUMN_UUID_C_UUID_3);
}
else {
bindUuid = true;
sb.append(_FINDER_COLUMN_UUID_C_UUID_2);
}
sb.append(_FINDER_COLUMN_UUID_C_COMPANYID_2);
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(MBMessageModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
if (bindUuid) {
queryPos.add(uuid);
}
queryPos.add(companyId);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(mbMessage)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the message-boards messages where uuid = ? and companyId = ? from the database.
*
* @param uuid the uuid
* @param companyId the company ID
*/
@Override
public void removeByUuid_C(String uuid, long companyId) {
for (MBMessage mbMessage :
findByUuid_C(
uuid, companyId, QueryUtil.ALL_POS, QueryUtil.ALL_POS,
null)) {
remove(mbMessage);
}
}
/**
* Returns the number of message-boards messages where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @return the number of matching message-boards messages
*/
@Override
public int countByUuid_C(String uuid, long companyId) {
uuid = Objects.toString(uuid, "");
boolean productionMode = ctPersistenceHelper.isProductionMode(
MBMessage.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
Long count = null;
if (productionMode) {
finderPath = _finderPathCountByUuid_C;
finderArgs = new Object[] {uuid, companyId};
count = (Long)finderCache.getResult(finderPath, finderArgs, this);
}
if (count == null) {
StringBundler sb = new StringBundler(3);
sb.append(_SQL_COUNT_MBMESSAGE_WHERE);
boolean bindUuid = false;
if (uuid.isEmpty()) {
sb.append(_FINDER_COLUMN_UUID_C_UUID_3);
}
else {
bindUuid = true;
sb.append(_FINDER_COLUMN_UUID_C_UUID_2);
}
sb.append(_FINDER_COLUMN_UUID_C_COMPANYID_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
if (bindUuid) {
queryPos.add(uuid);
}
queryPos.add(companyId);
count = (Long)query.uniqueResult();
if (productionMode) {
finderCache.putResult(finderPath, finderArgs, count);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String _FINDER_COLUMN_UUID_C_UUID_2 =
"mbMessage.uuid = ? AND ";
private static final String _FINDER_COLUMN_UUID_C_UUID_3 =
"(mbMessage.uuid IS NULL OR mbMessage.uuid = '') AND ";
private static final String _FINDER_COLUMN_UUID_C_COMPANYID_2 =
"mbMessage.companyId = ?";
private FinderPath _finderPathWithPaginationFindByGroupId;
private FinderPath _finderPathWithoutPaginationFindByGroupId;
private FinderPath _finderPathCountByGroupId;
/**
* Returns all the message-boards messages where groupId = ?.
*
* @param groupId the group ID
* @return the matching message-boards messages
*/
@Override
public List findByGroupId(long groupId) {
return findByGroupId(
groupId, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the message-boards messages where groupId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from MBMessageModelImpl
.
*
*
* @param groupId the group ID
* @param start the lower bound of the range of message-boards messages
* @param end the upper bound of the range of message-boards messages (not inclusive)
* @return the range of matching message-boards messages
*/
@Override
public List findByGroupId(long groupId, int start, int end) {
return findByGroupId(groupId, start, end, null);
}
/**
* Returns an ordered range of all the message-boards messages where groupId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from MBMessageModelImpl
.
*
*
* @param groupId the group ID
* @param start the lower bound of the range of message-boards messages
* @param end the upper bound of the range of message-boards messages (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching message-boards messages
*/
@Override
public List findByGroupId(
long groupId, int start, int end,
OrderByComparator orderByComparator) {
return findByGroupId(groupId, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the message-boards messages where groupId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from MBMessageModelImpl
.
*
*
* @param groupId the group ID
* @param start the lower bound of the range of message-boards messages
* @param end the upper bound of the range of message-boards messages (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching message-boards messages
*/
@Override
public List findByGroupId(
long groupId, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
boolean productionMode = ctPersistenceHelper.isProductionMode(
MBMessage.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache && productionMode) {
finderPath = _finderPathWithoutPaginationFindByGroupId;
finderArgs = new Object[] {groupId};
}
}
else if (useFinderCache && productionMode) {
finderPath = _finderPathWithPaginationFindByGroupId;
finderArgs = new Object[] {groupId, start, end, orderByComparator};
}
List list = null;
if (useFinderCache && productionMode) {
list = (List)finderCache.getResult(
finderPath, finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (MBMessage mbMessage : list) {
if (groupId != mbMessage.getGroupId()) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
3 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(3);
}
sb.append(_SQL_SELECT_MBMESSAGE_WHERE);
sb.append(_FINDER_COLUMN_GROUPID_GROUPID_2);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(MBMessageModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache && productionMode) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first message-boards message in the ordered set where groupId = ?.
*
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching message-boards message
* @throws NoSuchMessageException if a matching message-boards message could not be found
*/
@Override
public MBMessage findByGroupId_First(
long groupId, OrderByComparator orderByComparator)
throws NoSuchMessageException {
MBMessage mbMessage = fetchByGroupId_First(groupId, orderByComparator);
if (mbMessage != null) {
return mbMessage;
}
StringBundler sb = new StringBundler(4);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("groupId=");
sb.append(groupId);
sb.append("}");
throw new NoSuchMessageException(sb.toString());
}
/**
* Returns the first message-boards message in the ordered set where groupId = ?.
*
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching message-boards message, or null
if a matching message-boards message could not be found
*/
@Override
public MBMessage fetchByGroupId_First(
long groupId, OrderByComparator orderByComparator) {
List list = findByGroupId(groupId, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last message-boards message in the ordered set where groupId = ?.
*
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching message-boards message
* @throws NoSuchMessageException if a matching message-boards message could not be found
*/
@Override
public MBMessage findByGroupId_Last(
long groupId, OrderByComparator orderByComparator)
throws NoSuchMessageException {
MBMessage mbMessage = fetchByGroupId_Last(groupId, orderByComparator);
if (mbMessage != null) {
return mbMessage;
}
StringBundler sb = new StringBundler(4);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("groupId=");
sb.append(groupId);
sb.append("}");
throw new NoSuchMessageException(sb.toString());
}
/**
* Returns the last message-boards message in the ordered set where groupId = ?.
*
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching message-boards message, or null
if a matching message-boards message could not be found
*/
@Override
public MBMessage fetchByGroupId_Last(
long groupId, OrderByComparator orderByComparator) {
int count = countByGroupId(groupId);
if (count == 0) {
return null;
}
List list = findByGroupId(
groupId, count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the message-boards messages before and after the current message-boards message in the ordered set where groupId = ?.
*
* @param messageId the primary key of the current message-boards message
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next message-boards message
* @throws NoSuchMessageException if a message-boards message with the primary key could not be found
*/
@Override
public MBMessage[] findByGroupId_PrevAndNext(
long messageId, long groupId,
OrderByComparator orderByComparator)
throws NoSuchMessageException {
MBMessage mbMessage = findByPrimaryKey(messageId);
Session session = null;
try {
session = openSession();
MBMessage[] array = new MBMessageImpl[3];
array[0] = getByGroupId_PrevAndNext(
session, mbMessage, groupId, orderByComparator, true);
array[1] = mbMessage;
array[2] = getByGroupId_PrevAndNext(
session, mbMessage, groupId, orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected MBMessage getByGroupId_PrevAndNext(
Session session, MBMessage mbMessage, long groupId,
OrderByComparator orderByComparator, boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(3);
}
sb.append(_SQL_SELECT_MBMESSAGE_WHERE);
sb.append(_FINDER_COLUMN_GROUPID_GROUPID_2);
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(MBMessageModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(mbMessage)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Returns all the message-boards messages that the user has permission to view where groupId = ?.
*
* @param groupId the group ID
* @return the matching message-boards messages that the user has permission to view
*/
@Override
public List filterFindByGroupId(long groupId) {
return filterFindByGroupId(
groupId, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the message-boards messages that the user has permission to view where groupId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from MBMessageModelImpl
.
*
*
* @param groupId the group ID
* @param start the lower bound of the range of message-boards messages
* @param end the upper bound of the range of message-boards messages (not inclusive)
* @return the range of matching message-boards messages that the user has permission to view
*/
@Override
public List filterFindByGroupId(
long groupId, int start, int end) {
return filterFindByGroupId(groupId, start, end, null);
}
/**
* Returns an ordered range of all the message-boards messages that the user has permissions to view where groupId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from MBMessageModelImpl
.
*
*
* @param groupId the group ID
* @param start the lower bound of the range of message-boards messages
* @param end the upper bound of the range of message-boards messages (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching message-boards messages that the user has permission to view
*/
@Override
public List filterFindByGroupId(
long groupId, int start, int end,
OrderByComparator orderByComparator) {
if (!InlineSQLHelperUtil.isEnabled(groupId)) {
return findByGroupId(groupId, start, end, orderByComparator);
}
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
3 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(4);
}
if (getDB().isSupportsInlineDistinct()) {
sb.append(_FILTER_SQL_SELECT_MBMESSAGE_WHERE);
}
else {
sb.append(_FILTER_SQL_SELECT_MBMESSAGE_NO_INLINE_DISTINCT_WHERE_1);
}
sb.append(_FINDER_COLUMN_GROUPID_GROUPID_2);
if (!getDB().isSupportsInlineDistinct()) {
sb.append(_FILTER_SQL_SELECT_MBMESSAGE_NO_INLINE_DISTINCT_WHERE_2);
}
if (orderByComparator != null) {
if (getDB().isSupportsInlineDistinct()) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator, true);
}
else {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_TABLE, orderByComparator, true);
}
}
else {
if (getDB().isSupportsInlineDistinct()) {
sb.append(MBMessageModelImpl.ORDER_BY_JPQL);
}
else {
sb.append(MBMessageModelImpl.ORDER_BY_SQL);
}
}
String sql = InlineSQLHelperUtil.replacePermissionCheck(
sb.toString(), MBMessage.class.getName(),
_FILTER_ENTITY_TABLE_FILTER_PK_COLUMN, groupId);
Session session = null;
try {
session = openSession();
SQLQuery sqlQuery = session.createSynchronizedSQLQuery(sql);
if (getDB().isSupportsInlineDistinct()) {
sqlQuery.addEntity(_FILTER_ENTITY_ALIAS, MBMessageImpl.class);
}
else {
sqlQuery.addEntity(_FILTER_ENTITY_TABLE, MBMessageImpl.class);
}
QueryPos queryPos = QueryPos.getInstance(sqlQuery);
queryPos.add(groupId);
return (List)QueryUtil.list(
sqlQuery, getDialect(), start, end);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
/**
* Returns the message-boards messages before and after the current message-boards message in the ordered set of message-boards messages that the user has permission to view where groupId = ?.
*
* @param messageId the primary key of the current message-boards message
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next message-boards message
* @throws NoSuchMessageException if a message-boards message with the primary key could not be found
*/
@Override
public MBMessage[] filterFindByGroupId_PrevAndNext(
long messageId, long groupId,
OrderByComparator orderByComparator)
throws NoSuchMessageException {
if (!InlineSQLHelperUtil.isEnabled(groupId)) {
return findByGroupId_PrevAndNext(
messageId, groupId, orderByComparator);
}
MBMessage mbMessage = findByPrimaryKey(messageId);
Session session = null;
try {
session = openSession();
MBMessage[] array = new MBMessageImpl[3];
array[0] = filterGetByGroupId_PrevAndNext(
session, mbMessage, groupId, orderByComparator, true);
array[1] = mbMessage;
array[2] = filterGetByGroupId_PrevAndNext(
session, mbMessage, groupId, orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected MBMessage filterGetByGroupId_PrevAndNext(
Session session, MBMessage mbMessage, long groupId,
OrderByComparator orderByComparator, boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
5 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(4);
}
if (getDB().isSupportsInlineDistinct()) {
sb.append(_FILTER_SQL_SELECT_MBMESSAGE_WHERE);
}
else {
sb.append(_FILTER_SQL_SELECT_MBMESSAGE_NO_INLINE_DISTINCT_WHERE_1);
}
sb.append(_FINDER_COLUMN_GROUPID_GROUPID_2);
if (!getDB().isSupportsInlineDistinct()) {
sb.append(_FILTER_SQL_SELECT_MBMESSAGE_NO_INLINE_DISTINCT_WHERE_2);
}
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
if (getDB().isSupportsInlineDistinct()) {
sb.append(
getColumnName(
_ORDER_BY_ENTITY_ALIAS, orderByConditionFields[i],
true));
}
else {
sb.append(
getColumnName(
_ORDER_BY_ENTITY_TABLE, orderByConditionFields[i],
true));
}
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
if (getDB().isSupportsInlineDistinct()) {
sb.append(
getColumnName(
_ORDER_BY_ENTITY_ALIAS, orderByFields[i], true));
}
else {
sb.append(
getColumnName(
_ORDER_BY_ENTITY_TABLE, orderByFields[i], true));
}
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
if (getDB().isSupportsInlineDistinct()) {
sb.append(MBMessageModelImpl.ORDER_BY_JPQL);
}
else {
sb.append(MBMessageModelImpl.ORDER_BY_SQL);
}
}
String sql = InlineSQLHelperUtil.replacePermissionCheck(
sb.toString(), MBMessage.class.getName(),
_FILTER_ENTITY_TABLE_FILTER_PK_COLUMN, groupId);
SQLQuery sqlQuery = session.createSynchronizedSQLQuery(sql);
sqlQuery.setFirstResult(0);
sqlQuery.setMaxResults(2);
if (getDB().isSupportsInlineDistinct()) {
sqlQuery.addEntity(_FILTER_ENTITY_ALIAS, MBMessageImpl.class);
}
else {
sqlQuery.addEntity(_FILTER_ENTITY_TABLE, MBMessageImpl.class);
}
QueryPos queryPos = QueryPos.getInstance(sqlQuery);
queryPos.add(groupId);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(mbMessage)) {
queryPos.add(orderByConditionValue);
}
}
List list = sqlQuery.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the message-boards messages where groupId = ? from the database.
*
* @param groupId the group ID
*/
@Override
public void removeByGroupId(long groupId) {
for (MBMessage mbMessage :
findByGroupId(
groupId, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null)) {
remove(mbMessage);
}
}
/**
* Returns the number of message-boards messages where groupId = ?.
*
* @param groupId the group ID
* @return the number of matching message-boards messages
*/
@Override
public int countByGroupId(long groupId) {
boolean productionMode = ctPersistenceHelper.isProductionMode(
MBMessage.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
Long count = null;
if (productionMode) {
finderPath = _finderPathCountByGroupId;
finderArgs = new Object[] {groupId};
count = (Long)finderCache.getResult(finderPath, finderArgs, this);
}
if (count == null) {
StringBundler sb = new StringBundler(2);
sb.append(_SQL_COUNT_MBMESSAGE_WHERE);
sb.append(_FINDER_COLUMN_GROUPID_GROUPID_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
count = (Long)query.uniqueResult();
if (productionMode) {
finderCache.putResult(finderPath, finderArgs, count);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
/**
* Returns the number of message-boards messages that the user has permission to view where groupId = ?.
*
* @param groupId the group ID
* @return the number of matching message-boards messages that the user has permission to view
*/
@Override
public int filterCountByGroupId(long groupId) {
if (!InlineSQLHelperUtil.isEnabled(groupId)) {
return countByGroupId(groupId);
}
StringBundler sb = new StringBundler(2);
sb.append(_FILTER_SQL_COUNT_MBMESSAGE_WHERE);
sb.append(_FINDER_COLUMN_GROUPID_GROUPID_2);
String sql = InlineSQLHelperUtil.replacePermissionCheck(
sb.toString(), MBMessage.class.getName(),
_FILTER_ENTITY_TABLE_FILTER_PK_COLUMN, groupId);
Session session = null;
try {
session = openSession();
SQLQuery sqlQuery = session.createSynchronizedSQLQuery(sql);
sqlQuery.addScalar(
COUNT_COLUMN_NAME, com.liferay.portal.kernel.dao.orm.Type.LONG);
QueryPos queryPos = QueryPos.getInstance(sqlQuery);
queryPos.add(groupId);
Long count = (Long)sqlQuery.uniqueResult();
return count.intValue();
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
private static final String _FINDER_COLUMN_GROUPID_GROUPID_2 =
"mbMessage.groupId = ? AND mbMessage.categoryId != -1";
private FinderPath _finderPathWithPaginationFindByCompanyId;
private FinderPath _finderPathWithoutPaginationFindByCompanyId;
private FinderPath _finderPathCountByCompanyId;
/**
* Returns all the message-boards messages where companyId = ?.
*
* @param companyId the company ID
* @return the matching message-boards messages
*/
@Override
public List findByCompanyId(long companyId) {
return findByCompanyId(
companyId, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the message-boards messages where companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from MBMessageModelImpl
.
*
*
* @param companyId the company ID
* @param start the lower bound of the range of message-boards messages
* @param end the upper bound of the range of message-boards messages (not inclusive)
* @return the range of matching message-boards messages
*/
@Override
public List findByCompanyId(long companyId, int start, int end) {
return findByCompanyId(companyId, start, end, null);
}
/**
* Returns an ordered range of all the message-boards messages where companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from MBMessageModelImpl
.
*
*
* @param companyId the company ID
* @param start the lower bound of the range of message-boards messages
* @param end the upper bound of the range of message-boards messages (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching message-boards messages
*/
@Override
public List findByCompanyId(
long companyId, int start, int end,
OrderByComparator orderByComparator) {
return findByCompanyId(companyId, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the message-boards messages where companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from MBMessageModelImpl
.
*
*
* @param companyId the company ID
* @param start the lower bound of the range of message-boards messages
* @param end the upper bound of the range of message-boards messages (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching message-boards messages
*/
@Override
public List findByCompanyId(
long companyId, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
boolean productionMode = ctPersistenceHelper.isProductionMode(
MBMessage.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache && productionMode) {
finderPath = _finderPathWithoutPaginationFindByCompanyId;
finderArgs = new Object[] {companyId};
}
}
else if (useFinderCache && productionMode) {
finderPath = _finderPathWithPaginationFindByCompanyId;
finderArgs = new Object[] {
companyId, start, end, orderByComparator
};
}
List list = null;
if (useFinderCache && productionMode) {
list = (List)finderCache.getResult(
finderPath, finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (MBMessage mbMessage : list) {
if (companyId != mbMessage.getCompanyId()) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
3 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(3);
}
sb.append(_SQL_SELECT_MBMESSAGE_WHERE);
sb.append(_FINDER_COLUMN_COMPANYID_COMPANYID_2);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(MBMessageModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(companyId);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache && productionMode) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first message-boards message in the ordered set where companyId = ?.
*
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching message-boards message
* @throws NoSuchMessageException if a matching message-boards message could not be found
*/
@Override
public MBMessage findByCompanyId_First(
long companyId, OrderByComparator orderByComparator)
throws NoSuchMessageException {
MBMessage mbMessage = fetchByCompanyId_First(
companyId, orderByComparator);
if (mbMessage != null) {
return mbMessage;
}
StringBundler sb = new StringBundler(4);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("companyId=");
sb.append(companyId);
sb.append("}");
throw new NoSuchMessageException(sb.toString());
}
/**
* Returns the first message-boards message in the ordered set where companyId = ?.
*
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching message-boards message, or null
if a matching message-boards message could not be found
*/
@Override
public MBMessage fetchByCompanyId_First(
long companyId, OrderByComparator orderByComparator) {
List list = findByCompanyId(
companyId, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last message-boards message in the ordered set where companyId = ?.
*
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching message-boards message
* @throws NoSuchMessageException if a matching message-boards message could not be found
*/
@Override
public MBMessage findByCompanyId_Last(
long companyId, OrderByComparator orderByComparator)
throws NoSuchMessageException {
MBMessage mbMessage = fetchByCompanyId_Last(
companyId, orderByComparator);
if (mbMessage != null) {
return mbMessage;
}
StringBundler sb = new StringBundler(4);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("companyId=");
sb.append(companyId);
sb.append("}");
throw new NoSuchMessageException(sb.toString());
}
/**
* Returns the last message-boards message in the ordered set where companyId = ?.
*
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching message-boards message, or null
if a matching message-boards message could not be found
*/
@Override
public MBMessage fetchByCompanyId_Last(
long companyId, OrderByComparator orderByComparator) {
int count = countByCompanyId(companyId);
if (count == 0) {
return null;
}
List list = findByCompanyId(
companyId, count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the message-boards messages before and after the current message-boards message in the ordered set where companyId = ?.
*
* @param messageId the primary key of the current message-boards message
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next message-boards message
* @throws NoSuchMessageException if a message-boards message with the primary key could not be found
*/
@Override
public MBMessage[] findByCompanyId_PrevAndNext(
long messageId, long companyId,
OrderByComparator orderByComparator)
throws NoSuchMessageException {
MBMessage mbMessage = findByPrimaryKey(messageId);
Session session = null;
try {
session = openSession();
MBMessage[] array = new MBMessageImpl[3];
array[0] = getByCompanyId_PrevAndNext(
session, mbMessage, companyId, orderByComparator, true);
array[1] = mbMessage;
array[2] = getByCompanyId_PrevAndNext(
session, mbMessage, companyId, orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected MBMessage getByCompanyId_PrevAndNext(
Session session, MBMessage mbMessage, long companyId,
OrderByComparator orderByComparator, boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(3);
}
sb.append(_SQL_SELECT_MBMESSAGE_WHERE);
sb.append(_FINDER_COLUMN_COMPANYID_COMPANYID_2);
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(MBMessageModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(companyId);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(mbMessage)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the message-boards messages where companyId = ? from the database.
*
* @param companyId the company ID
*/
@Override
public void removeByCompanyId(long companyId) {
for (MBMessage mbMessage :
findByCompanyId(
companyId, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null)) {
remove(mbMessage);
}
}
/**
* Returns the number of message-boards messages where companyId = ?.
*
* @param companyId the company ID
* @return the number of matching message-boards messages
*/
@Override
public int countByCompanyId(long companyId) {
boolean productionMode = ctPersistenceHelper.isProductionMode(
MBMessage.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
Long count = null;
if (productionMode) {
finderPath = _finderPathCountByCompanyId;
finderArgs = new Object[] {companyId};
count = (Long)finderCache.getResult(finderPath, finderArgs, this);
}
if (count == null) {
StringBundler sb = new StringBundler(2);
sb.append(_SQL_COUNT_MBMESSAGE_WHERE);
sb.append(_FINDER_COLUMN_COMPANYID_COMPANYID_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(companyId);
count = (Long)query.uniqueResult();
if (productionMode) {
finderCache.putResult(finderPath, finderArgs, count);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String _FINDER_COLUMN_COMPANYID_COMPANYID_2 =
"mbMessage.companyId = ? AND mbMessage.categoryId != -1";
private FinderPath _finderPathWithPaginationFindByUserId;
private FinderPath _finderPathWithoutPaginationFindByUserId;
private FinderPath _finderPathCountByUserId;
/**
* Returns all the message-boards messages where userId = ?.
*
* @param userId the user ID
* @return the matching message-boards messages
*/
@Override
public List findByUserId(long userId) {
return findByUserId(userId, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the message-boards messages where userId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from MBMessageModelImpl
.
*
*
* @param userId the user ID
* @param start the lower bound of the range of message-boards messages
* @param end the upper bound of the range of message-boards messages (not inclusive)
* @return the range of matching message-boards messages
*/
@Override
public List findByUserId(long userId, int start, int end) {
return findByUserId(userId, start, end, null);
}
/**
* Returns an ordered range of all the message-boards messages where userId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from MBMessageModelImpl
.
*
*
* @param userId the user ID
* @param start the lower bound of the range of message-boards messages
* @param end the upper bound of the range of message-boards messages (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching message-boards messages
*/
@Override
public List findByUserId(
long userId, int start, int end,
OrderByComparator orderByComparator) {
return findByUserId(userId, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the message-boards messages where userId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from MBMessageModelImpl
.
*
*
* @param userId the user ID
* @param start the lower bound of the range of message-boards messages
* @param end the upper bound of the range of message-boards messages (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching message-boards messages
*/
@Override
public List findByUserId(
long userId, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
boolean productionMode = ctPersistenceHelper.isProductionMode(
MBMessage.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache && productionMode) {
finderPath = _finderPathWithoutPaginationFindByUserId;
finderArgs = new Object[] {userId};
}
}
else if (useFinderCache && productionMode) {
finderPath = _finderPathWithPaginationFindByUserId;
finderArgs = new Object[] {userId, start, end, orderByComparator};
}
List list = null;
if (useFinderCache && productionMode) {
list = (List)finderCache.getResult(
finderPath, finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (MBMessage mbMessage : list) {
if (userId != mbMessage.getUserId()) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
3 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(3);
}
sb.append(_SQL_SELECT_MBMESSAGE_WHERE);
sb.append(_FINDER_COLUMN_USERID_USERID_2);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(MBMessageModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(userId);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache && productionMode) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first message-boards message in the ordered set where userId = ?.
*
* @param userId the user ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching message-boards message
* @throws NoSuchMessageException if a matching message-boards message could not be found
*/
@Override
public MBMessage findByUserId_First(
long userId, OrderByComparator orderByComparator)
throws NoSuchMessageException {
MBMessage mbMessage = fetchByUserId_First(userId, orderByComparator);
if (mbMessage != null) {
return mbMessage;
}
StringBundler sb = new StringBundler(4);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("userId=");
sb.append(userId);
sb.append("}");
throw new NoSuchMessageException(sb.toString());
}
/**
* Returns the first message-boards message in the ordered set where userId = ?.
*
* @param userId the user ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching message-boards message, or null
if a matching message-boards message could not be found
*/
@Override
public MBMessage fetchByUserId_First(
long userId, OrderByComparator orderByComparator) {
List list = findByUserId(userId, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last message-boards message in the ordered set where userId = ?.
*
* @param userId the user ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching message-boards message
* @throws NoSuchMessageException if a matching message-boards message could not be found
*/
@Override
public MBMessage findByUserId_Last(
long userId, OrderByComparator orderByComparator)
throws NoSuchMessageException {
MBMessage mbMessage = fetchByUserId_Last(userId, orderByComparator);
if (mbMessage != null) {
return mbMessage;
}
StringBundler sb = new StringBundler(4);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("userId=");
sb.append(userId);
sb.append("}");
throw new NoSuchMessageException(sb.toString());
}
/**
* Returns the last message-boards message in the ordered set where userId = ?.
*
* @param userId the user ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching message-boards message, or null
if a matching message-boards message could not be found
*/
@Override
public MBMessage fetchByUserId_Last(
long userId, OrderByComparator orderByComparator) {
int count = countByUserId(userId);
if (count == 0) {
return null;
}
List list = findByUserId(
userId, count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the message-boards messages before and after the current message-boards message in the ordered set where userId = ?.
*
* @param messageId the primary key of the current message-boards message
* @param userId the user ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next message-boards message
* @throws NoSuchMessageException if a message-boards message with the primary key could not be found
*/
@Override
public MBMessage[] findByUserId_PrevAndNext(
long messageId, long userId,
OrderByComparator orderByComparator)
throws NoSuchMessageException {
MBMessage mbMessage = findByPrimaryKey(messageId);
Session session = null;
try {
session = openSession();
MBMessage[] array = new MBMessageImpl[3];
array[0] = getByUserId_PrevAndNext(
session, mbMessage, userId, orderByComparator, true);
array[1] = mbMessage;
array[2] = getByUserId_PrevAndNext(
session, mbMessage, userId, orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected MBMessage getByUserId_PrevAndNext(
Session session, MBMessage mbMessage, long userId,
OrderByComparator orderByComparator, boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(3);
}
sb.append(_SQL_SELECT_MBMESSAGE_WHERE);
sb.append(_FINDER_COLUMN_USERID_USERID_2);
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(MBMessageModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(userId);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(mbMessage)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the message-boards messages where userId = ? from the database.
*
* @param userId the user ID
*/
@Override
public void removeByUserId(long userId) {
for (MBMessage mbMessage :
findByUserId(
userId, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null)) {
remove(mbMessage);
}
}
/**
* Returns the number of message-boards messages where userId = ?.
*
* @param userId the user ID
* @return the number of matching message-boards messages
*/
@Override
public int countByUserId(long userId) {
boolean productionMode = ctPersistenceHelper.isProductionMode(
MBMessage.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
Long count = null;
if (productionMode) {
finderPath = _finderPathCountByUserId;
finderArgs = new Object[] {userId};
count = (Long)finderCache.getResult(finderPath, finderArgs, this);
}
if (count == null) {
StringBundler sb = new StringBundler(2);
sb.append(_SQL_COUNT_MBMESSAGE_WHERE);
sb.append(_FINDER_COLUMN_USERID_USERID_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(userId);
count = (Long)query.uniqueResult();
if (productionMode) {
finderCache.putResult(finderPath, finderArgs, count);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String _FINDER_COLUMN_USERID_USERID_2 =
"mbMessage.userId = ? AND mbMessage.categoryId != -1";
private FinderPath _finderPathWithPaginationFindByThreadId;
private FinderPath _finderPathWithoutPaginationFindByThreadId;
private FinderPath _finderPathCountByThreadId;
/**
* Returns all the message-boards messages where threadId = ?.
*
* @param threadId the thread ID
* @return the matching message-boards messages
*/
@Override
public List findByThreadId(long threadId) {
return findByThreadId(
threadId, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the message-boards messages where threadId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from MBMessageModelImpl
.
*
*
* @param threadId the thread ID
* @param start the lower bound of the range of message-boards messages
* @param end the upper bound of the range of message-boards messages (not inclusive)
* @return the range of matching message-boards messages
*/
@Override
public List findByThreadId(long threadId, int start, int end) {
return findByThreadId(threadId, start, end, null);
}
/**
* Returns an ordered range of all the message-boards messages where threadId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from MBMessageModelImpl
.
*
*
* @param threadId the thread ID
* @param start the lower bound of the range of message-boards messages
* @param end the upper bound of the range of message-boards messages (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching message-boards messages
*/
@Override
public List findByThreadId(
long threadId, int start, int end,
OrderByComparator orderByComparator) {
return findByThreadId(threadId, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the message-boards messages where threadId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from MBMessageModelImpl
.
*
*
* @param threadId the thread ID
* @param start the lower bound of the range of message-boards messages
* @param end the upper bound of the range of message-boards messages (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching message-boards messages
*/
@Override
public List findByThreadId(
long threadId, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
boolean productionMode = ctPersistenceHelper.isProductionMode(
MBMessage.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache && productionMode) {
finderPath = _finderPathWithoutPaginationFindByThreadId;
finderArgs = new Object[] {threadId};
}
}
else if (useFinderCache && productionMode) {
finderPath = _finderPathWithPaginationFindByThreadId;
finderArgs = new Object[] {threadId, start, end, orderByComparator};
}
List list = null;
if (useFinderCache && productionMode) {
list = (List)finderCache.getResult(
finderPath, finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (MBMessage mbMessage : list) {
if (threadId != mbMessage.getThreadId()) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
3 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(3);
}
sb.append(_SQL_SELECT_MBMESSAGE_WHERE);
sb.append(_FINDER_COLUMN_THREADID_THREADID_2);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(MBMessageModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(threadId);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache && productionMode) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first message-boards message in the ordered set where threadId = ?.
*
* @param threadId the thread ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching message-boards message
* @throws NoSuchMessageException if a matching message-boards message could not be found
*/
@Override
public MBMessage findByThreadId_First(
long threadId, OrderByComparator orderByComparator)
throws NoSuchMessageException {
MBMessage mbMessage = fetchByThreadId_First(
threadId, orderByComparator);
if (mbMessage != null) {
return mbMessage;
}
StringBundler sb = new StringBundler(4);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("threadId=");
sb.append(threadId);
sb.append("}");
throw new NoSuchMessageException(sb.toString());
}
/**
* Returns the first message-boards message in the ordered set where threadId = ?.
*
* @param threadId the thread ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching message-boards message, or null
if a matching message-boards message could not be found
*/
@Override
public MBMessage fetchByThreadId_First(
long threadId, OrderByComparator orderByComparator) {
List list = findByThreadId(
threadId, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last message-boards message in the ordered set where threadId = ?.
*
* @param threadId the thread ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching message-boards message
* @throws NoSuchMessageException if a matching message-boards message could not be found
*/
@Override
public MBMessage findByThreadId_Last(
long threadId, OrderByComparator orderByComparator)
throws NoSuchMessageException {
MBMessage mbMessage = fetchByThreadId_Last(threadId, orderByComparator);
if (mbMessage != null) {
return mbMessage;
}
StringBundler sb = new StringBundler(4);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("threadId=");
sb.append(threadId);
sb.append("}");
throw new NoSuchMessageException(sb.toString());
}
/**
* Returns the last message-boards message in the ordered set where threadId = ?.
*
* @param threadId the thread ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching message-boards message, or null
if a matching message-boards message could not be found
*/
@Override
public MBMessage fetchByThreadId_Last(
long threadId, OrderByComparator orderByComparator) {
int count = countByThreadId(threadId);
if (count == 0) {
return null;
}
List list = findByThreadId(
threadId, count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the message-boards messages before and after the current message-boards message in the ordered set where threadId = ?.
*
* @param messageId the primary key of the current message-boards message
* @param threadId the thread ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next message-boards message
* @throws NoSuchMessageException if a message-boards message with the primary key could not be found
*/
@Override
public MBMessage[] findByThreadId_PrevAndNext(
long messageId, long threadId,
OrderByComparator orderByComparator)
throws NoSuchMessageException {
MBMessage mbMessage = findByPrimaryKey(messageId);
Session session = null;
try {
session = openSession();
MBMessage[] array = new MBMessageImpl[3];
array[0] = getByThreadId_PrevAndNext(
session, mbMessage, threadId, orderByComparator, true);
array[1] = mbMessage;
array[2] = getByThreadId_PrevAndNext(
session, mbMessage, threadId, orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected MBMessage getByThreadId_PrevAndNext(
Session session, MBMessage mbMessage, long threadId,
OrderByComparator orderByComparator, boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(3);
}
sb.append(_SQL_SELECT_MBMESSAGE_WHERE);
sb.append(_FINDER_COLUMN_THREADID_THREADID_2);
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(MBMessageModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(threadId);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(mbMessage)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the message-boards messages where threadId = ? from the database.
*
* @param threadId the thread ID
*/
@Override
public void removeByThreadId(long threadId) {
for (MBMessage mbMessage :
findByThreadId(
threadId, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null)) {
remove(mbMessage);
}
}
/**
* Returns the number of message-boards messages where threadId = ?.
*
* @param threadId the thread ID
* @return the number of matching message-boards messages
*/
@Override
public int countByThreadId(long threadId) {
boolean productionMode = ctPersistenceHelper.isProductionMode(
MBMessage.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
Long count = null;
if (productionMode) {
finderPath = _finderPathCountByThreadId;
finderArgs = new Object[] {threadId};
count = (Long)finderCache.getResult(finderPath, finderArgs, this);
}
if (count == null) {
StringBundler sb = new StringBundler(2);
sb.append(_SQL_COUNT_MBMESSAGE_WHERE);
sb.append(_FINDER_COLUMN_THREADID_THREADID_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(threadId);
count = (Long)query.uniqueResult();
if (productionMode) {
finderCache.putResult(finderPath, finderArgs, count);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String _FINDER_COLUMN_THREADID_THREADID_2 =
"mbMessage.threadId = ?";
private FinderPath _finderPathWithPaginationFindByThreadIdReplies;
private FinderPath _finderPathWithoutPaginationFindByThreadIdReplies;
private FinderPath _finderPathCountByThreadIdReplies;
/**
* Returns all the message-boards messages where threadId = ?.
*
* @param threadId the thread ID
* @return the matching message-boards messages
*/
@Override
public List findByThreadIdReplies(long threadId) {
return findByThreadIdReplies(
threadId, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the message-boards messages where threadId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from MBMessageModelImpl
.
*
*
* @param threadId the thread ID
* @param start the lower bound of the range of message-boards messages
* @param end the upper bound of the range of message-boards messages (not inclusive)
* @return the range of matching message-boards messages
*/
@Override
public List findByThreadIdReplies(
long threadId, int start, int end) {
return findByThreadIdReplies(threadId, start, end, null);
}
/**
* Returns an ordered range of all the message-boards messages where threadId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from MBMessageModelImpl
.
*
*
* @param threadId the thread ID
* @param start the lower bound of the range of message-boards messages
* @param end the upper bound of the range of message-boards messages (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching message-boards messages
*/
@Override
public List findByThreadIdReplies(
long threadId, int start, int end,
OrderByComparator orderByComparator) {
return findByThreadIdReplies(
threadId, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the message-boards messages where threadId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from MBMessageModelImpl
.
*
*
* @param threadId the thread ID
* @param start the lower bound of the range of message-boards messages
* @param end the upper bound of the range of message-boards messages (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching message-boards messages
*/
@Override
public List findByThreadIdReplies(
long threadId, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
boolean productionMode = ctPersistenceHelper.isProductionMode(
MBMessage.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache && productionMode) {
finderPath = _finderPathWithoutPaginationFindByThreadIdReplies;
finderArgs = new Object[] {threadId};
}
}
else if (useFinderCache && productionMode) {
finderPath = _finderPathWithPaginationFindByThreadIdReplies;
finderArgs = new Object[] {threadId, start, end, orderByComparator};
}
List list = null;
if (useFinderCache && productionMode) {
list = (List)finderCache.getResult(
finderPath, finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (MBMessage mbMessage : list) {
if (threadId != mbMessage.getThreadId()) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
3 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(3);
}
sb.append(_SQL_SELECT_MBMESSAGE_WHERE);
sb.append(_FINDER_COLUMN_THREADIDREPLIES_THREADID_2);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(MBMessageModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(threadId);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache && productionMode) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first message-boards message in the ordered set where threadId = ?.
*
* @param threadId the thread ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching message-boards message
* @throws NoSuchMessageException if a matching message-boards message could not be found
*/
@Override
public MBMessage findByThreadIdReplies_First(
long threadId, OrderByComparator orderByComparator)
throws NoSuchMessageException {
MBMessage mbMessage = fetchByThreadIdReplies_First(
threadId, orderByComparator);
if (mbMessage != null) {
return mbMessage;
}
StringBundler sb = new StringBundler(4);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("threadId=");
sb.append(threadId);
sb.append("}");
throw new NoSuchMessageException(sb.toString());
}
/**
* Returns the first message-boards message in the ordered set where threadId = ?.
*
* @param threadId the thread ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching message-boards message, or null
if a matching message-boards message could not be found
*/
@Override
public MBMessage fetchByThreadIdReplies_First(
long threadId, OrderByComparator orderByComparator) {
List list = findByThreadIdReplies(
threadId, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last message-boards message in the ordered set where threadId = ?.
*
* @param threadId the thread ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching message-boards message
* @throws NoSuchMessageException if a matching message-boards message could not be found
*/
@Override
public MBMessage findByThreadIdReplies_Last(
long threadId, OrderByComparator orderByComparator)
throws NoSuchMessageException {
MBMessage mbMessage = fetchByThreadIdReplies_Last(
threadId, orderByComparator);
if (mbMessage != null) {
return mbMessage;
}
StringBundler sb = new StringBundler(4);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("threadId=");
sb.append(threadId);
sb.append("}");
throw new NoSuchMessageException(sb.toString());
}
/**
* Returns the last message-boards message in the ordered set where threadId = ?.
*
* @param threadId the thread ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching message-boards message, or null
if a matching message-boards message could not be found
*/
@Override
public MBMessage fetchByThreadIdReplies_Last(
long threadId, OrderByComparator orderByComparator) {
int count = countByThreadIdReplies(threadId);
if (count == 0) {
return null;
}
List list = findByThreadIdReplies(
threadId, count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the message-boards messages before and after the current message-boards message in the ordered set where threadId = ?.
*
* @param messageId the primary key of the current message-boards message
* @param threadId the thread ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next message-boards message
* @throws NoSuchMessageException if a message-boards message with the primary key could not be found
*/
@Override
public MBMessage[] findByThreadIdReplies_PrevAndNext(
long messageId, long threadId,
OrderByComparator orderByComparator)
throws NoSuchMessageException {
MBMessage mbMessage = findByPrimaryKey(messageId);
Session session = null;
try {
session = openSession();
MBMessage[] array = new MBMessageImpl[3];
array[0] = getByThreadIdReplies_PrevAndNext(
session, mbMessage, threadId, orderByComparator, true);
array[1] = mbMessage;
array[2] = getByThreadIdReplies_PrevAndNext(
session, mbMessage, threadId, orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected MBMessage getByThreadIdReplies_PrevAndNext(
Session session, MBMessage mbMessage, long threadId,
OrderByComparator orderByComparator, boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(3);
}
sb.append(_SQL_SELECT_MBMESSAGE_WHERE);
sb.append(_FINDER_COLUMN_THREADIDREPLIES_THREADID_2);
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(MBMessageModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(threadId);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(mbMessage)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the message-boards messages where threadId = ? from the database.
*
* @param threadId the thread ID
*/
@Override
public void removeByThreadIdReplies(long threadId) {
for (MBMessage mbMessage :
findByThreadIdReplies(
threadId, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null)) {
remove(mbMessage);
}
}
/**
* Returns the number of message-boards messages where threadId = ?.
*
* @param threadId the thread ID
* @return the number of matching message-boards messages
*/
@Override
public int countByThreadIdReplies(long threadId) {
boolean productionMode = ctPersistenceHelper.isProductionMode(
MBMessage.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
Long count = null;
if (productionMode) {
finderPath = _finderPathCountByThreadIdReplies;
finderArgs = new Object[] {threadId};
count = (Long)finderCache.getResult(finderPath, finderArgs, this);
}
if (count == null) {
StringBundler sb = new StringBundler(2);
sb.append(_SQL_COUNT_MBMESSAGE_WHERE);
sb.append(_FINDER_COLUMN_THREADIDREPLIES_THREADID_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(threadId);
count = (Long)query.uniqueResult();
if (productionMode) {
finderCache.putResult(finderPath, finderArgs, count);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String _FINDER_COLUMN_THREADIDREPLIES_THREADID_2 =
"mbMessage.threadId = ? AND mbMessage.parentMessageId != 0";
private FinderPath _finderPathWithPaginationFindByParentMessageId;
private FinderPath _finderPathWithoutPaginationFindByParentMessageId;
private FinderPath _finderPathCountByParentMessageId;
/**
* Returns all the message-boards messages where parentMessageId = ?.
*
* @param parentMessageId the parent message ID
* @return the matching message-boards messages
*/
@Override
public List findByParentMessageId(long parentMessageId) {
return findByParentMessageId(
parentMessageId, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the message-boards messages where parentMessageId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from MBMessageModelImpl
.
*
*
* @param parentMessageId the parent message ID
* @param start the lower bound of the range of message-boards messages
* @param end the upper bound of the range of message-boards messages (not inclusive)
* @return the range of matching message-boards messages
*/
@Override
public List findByParentMessageId(
long parentMessageId, int start, int end) {
return findByParentMessageId(parentMessageId, start, end, null);
}
/**
* Returns an ordered range of all the message-boards messages where parentMessageId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from MBMessageModelImpl
.
*
*
* @param parentMessageId the parent message ID
* @param start the lower bound of the range of message-boards messages
* @param end the upper bound of the range of message-boards messages (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching message-boards messages
*/
@Override
public List findByParentMessageId(
long parentMessageId, int start, int end,
OrderByComparator orderByComparator) {
return findByParentMessageId(
parentMessageId, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the message-boards messages where parentMessageId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from MBMessageModelImpl
.
*
*
* @param parentMessageId the parent message ID
* @param start the lower bound of the range of message-boards messages
* @param end the upper bound of the range of message-boards messages (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching message-boards messages
*/
@Override
public List findByParentMessageId(
long parentMessageId, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
boolean productionMode = ctPersistenceHelper.isProductionMode(
MBMessage.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache && productionMode) {
finderPath = _finderPathWithoutPaginationFindByParentMessageId;
finderArgs = new Object[] {parentMessageId};
}
}
else if (useFinderCache && productionMode) {
finderPath = _finderPathWithPaginationFindByParentMessageId;
finderArgs = new Object[] {
parentMessageId, start, end, orderByComparator
};
}
List list = null;
if (useFinderCache && productionMode) {
list = (List)finderCache.getResult(
finderPath, finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (MBMessage mbMessage : list) {
if (parentMessageId != mbMessage.getParentMessageId()) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
3 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(3);
}
sb.append(_SQL_SELECT_MBMESSAGE_WHERE);
sb.append(_FINDER_COLUMN_PARENTMESSAGEID_PARENTMESSAGEID_2);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(MBMessageModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(parentMessageId);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache && productionMode) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first message-boards message in the ordered set where parentMessageId = ?.
*
* @param parentMessageId the parent message ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching message-boards message
* @throws NoSuchMessageException if a matching message-boards message could not be found
*/
@Override
public MBMessage findByParentMessageId_First(
long parentMessageId,
OrderByComparator orderByComparator)
throws NoSuchMessageException {
MBMessage mbMessage = fetchByParentMessageId_First(
parentMessageId, orderByComparator);
if (mbMessage != null) {
return mbMessage;
}
StringBundler sb = new StringBundler(4);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("parentMessageId=");
sb.append(parentMessageId);
sb.append("}");
throw new NoSuchMessageException(sb.toString());
}
/**
* Returns the first message-boards message in the ordered set where parentMessageId = ?.
*
* @param parentMessageId the parent message ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching message-boards message, or null
if a matching message-boards message could not be found
*/
@Override
public MBMessage fetchByParentMessageId_First(
long parentMessageId, OrderByComparator orderByComparator) {
List list = findByParentMessageId(
parentMessageId, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last message-boards message in the ordered set where parentMessageId = ?.
*
* @param parentMessageId the parent message ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching message-boards message
* @throws NoSuchMessageException if a matching message-boards message could not be found
*/
@Override
public MBMessage findByParentMessageId_Last(
long parentMessageId,
OrderByComparator orderByComparator)
throws NoSuchMessageException {
MBMessage mbMessage = fetchByParentMessageId_Last(
parentMessageId, orderByComparator);
if (mbMessage != null) {
return mbMessage;
}
StringBundler sb = new StringBundler(4);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("parentMessageId=");
sb.append(parentMessageId);
sb.append("}");
throw new NoSuchMessageException(sb.toString());
}
/**
* Returns the last message-boards message in the ordered set where parentMessageId = ?.
*
* @param parentMessageId the parent message ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching message-boards message, or null
if a matching message-boards message could not be found
*/
@Override
public MBMessage fetchByParentMessageId_Last(
long parentMessageId, OrderByComparator orderByComparator) {
int count = countByParentMessageId(parentMessageId);
if (count == 0) {
return null;
}
List list = findByParentMessageId(
parentMessageId, count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the message-boards messages before and after the current message-boards message in the ordered set where parentMessageId = ?.
*
* @param messageId the primary key of the current message-boards message
* @param parentMessageId the parent message ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next message-boards message
* @throws NoSuchMessageException if a message-boards message with the primary key could not be found
*/
@Override
public MBMessage[] findByParentMessageId_PrevAndNext(
long messageId, long parentMessageId,
OrderByComparator orderByComparator)
throws NoSuchMessageException {
MBMessage mbMessage = findByPrimaryKey(messageId);
Session session = null;
try {
session = openSession();
MBMessage[] array = new MBMessageImpl[3];
array[0] = getByParentMessageId_PrevAndNext(
session, mbMessage, parentMessageId, orderByComparator, true);
array[1] = mbMessage;
array[2] = getByParentMessageId_PrevAndNext(
session, mbMessage, parentMessageId, orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected MBMessage getByParentMessageId_PrevAndNext(
Session session, MBMessage mbMessage, long parentMessageId,
OrderByComparator orderByComparator, boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(3);
}
sb.append(_SQL_SELECT_MBMESSAGE_WHERE);
sb.append(_FINDER_COLUMN_PARENTMESSAGEID_PARENTMESSAGEID_2);
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(MBMessageModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(parentMessageId);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(mbMessage)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the message-boards messages where parentMessageId = ? from the database.
*
* @param parentMessageId the parent message ID
*/
@Override
public void removeByParentMessageId(long parentMessageId) {
for (MBMessage mbMessage :
findByParentMessageId(
parentMessageId, QueryUtil.ALL_POS, QueryUtil.ALL_POS,
null)) {
remove(mbMessage);
}
}
/**
* Returns the number of message-boards messages where parentMessageId = ?.
*
* @param parentMessageId the parent message ID
* @return the number of matching message-boards messages
*/
@Override
public int countByParentMessageId(long parentMessageId) {
boolean productionMode = ctPersistenceHelper.isProductionMode(
MBMessage.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
Long count = null;
if (productionMode) {
finderPath = _finderPathCountByParentMessageId;
finderArgs = new Object[] {parentMessageId};
count = (Long)finderCache.getResult(finderPath, finderArgs, this);
}
if (count == null) {
StringBundler sb = new StringBundler(2);
sb.append(_SQL_COUNT_MBMESSAGE_WHERE);
sb.append(_FINDER_COLUMN_PARENTMESSAGEID_PARENTMESSAGEID_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(parentMessageId);
count = (Long)query.uniqueResult();
if (productionMode) {
finderCache.putResult(finderPath, finderArgs, count);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String
_FINDER_COLUMN_PARENTMESSAGEID_PARENTMESSAGEID_2 =
"mbMessage.parentMessageId = ?";
private FinderPath _finderPathWithPaginationFindByG_U;
private FinderPath _finderPathWithoutPaginationFindByG_U;
private FinderPath _finderPathCountByG_U;
/**
* Returns all the message-boards messages where groupId = ? and userId = ?.
*
* @param groupId the group ID
* @param userId the user ID
* @return the matching message-boards messages
*/
@Override
public List findByG_U(long groupId, long userId) {
return findByG_U(
groupId, userId, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the message-boards messages where groupId = ? and userId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from MBMessageModelImpl
.
*
*
* @param groupId the group ID
* @param userId the user ID
* @param start the lower bound of the range of message-boards messages
* @param end the upper bound of the range of message-boards messages (not inclusive)
* @return the range of matching message-boards messages
*/
@Override
public List findByG_U(
long groupId, long userId, int start, int end) {
return findByG_U(groupId, userId, start, end, null);
}
/**
* Returns an ordered range of all the message-boards messages where groupId = ? and userId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from MBMessageModelImpl
.
*
*
* @param groupId the group ID
* @param userId the user ID
* @param start the lower bound of the range of message-boards messages
* @param end the upper bound of the range of message-boards messages (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching message-boards messages
*/
@Override
public List findByG_U(
long groupId, long userId, int start, int end,
OrderByComparator orderByComparator) {
return findByG_U(groupId, userId, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the message-boards messages where groupId = ? and userId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from MBMessageModelImpl
.
*
*
* @param groupId the group ID
* @param userId the user ID
* @param start the lower bound of the range of message-boards messages
* @param end the upper bound of the range of message-boards messages (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching message-boards messages
*/
@Override
public List findByG_U(
long groupId, long userId, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
boolean productionMode = ctPersistenceHelper.isProductionMode(
MBMessage.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache && productionMode) {
finderPath = _finderPathWithoutPaginationFindByG_U;
finderArgs = new Object[] {groupId, userId};
}
}
else if (useFinderCache && productionMode) {
finderPath = _finderPathWithPaginationFindByG_U;
finderArgs = new Object[] {
groupId, userId, start, end, orderByComparator
};
}
List list = null;
if (useFinderCache && productionMode) {
list = (List)finderCache.getResult(
finderPath, finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (MBMessage mbMessage : list) {
if ((groupId != mbMessage.getGroupId()) ||
(userId != mbMessage.getUserId())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_MBMESSAGE_WHERE);
sb.append(_FINDER_COLUMN_G_U_GROUPID_2);
sb.append(_FINDER_COLUMN_G_U_USERID_2);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(MBMessageModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
queryPos.add(userId);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache && productionMode) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first message-boards message in the ordered set where groupId = ? and userId = ?.
*
* @param groupId the group ID
* @param userId the user ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching message-boards message
* @throws NoSuchMessageException if a matching message-boards message could not be found
*/
@Override
public MBMessage findByG_U_First(
long groupId, long userId,
OrderByComparator orderByComparator)
throws NoSuchMessageException {
MBMessage mbMessage = fetchByG_U_First(
groupId, userId, orderByComparator);
if (mbMessage != null) {
return mbMessage;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("groupId=");
sb.append(groupId);
sb.append(", userId=");
sb.append(userId);
sb.append("}");
throw new NoSuchMessageException(sb.toString());
}
/**
* Returns the first message-boards message in the ordered set where groupId = ? and userId = ?.
*
* @param groupId the group ID
* @param userId the user ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching message-boards message, or null
if a matching message-boards message could not be found
*/
@Override
public MBMessage fetchByG_U_First(
long groupId, long userId,
OrderByComparator orderByComparator) {
List list = findByG_U(
groupId, userId, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last message-boards message in the ordered set where groupId = ? and userId = ?.
*
* @param groupId the group ID
* @param userId the user ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching message-boards message
* @throws NoSuchMessageException if a matching message-boards message could not be found
*/
@Override
public MBMessage findByG_U_Last(
long groupId, long userId,
OrderByComparator orderByComparator)
throws NoSuchMessageException {
MBMessage mbMessage = fetchByG_U_Last(
groupId, userId, orderByComparator);
if (mbMessage != null) {
return mbMessage;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("groupId=");
sb.append(groupId);
sb.append(", userId=");
sb.append(userId);
sb.append("}");
throw new NoSuchMessageException(sb.toString());
}
/**
* Returns the last message-boards message in the ordered set where groupId = ? and userId = ?.
*
* @param groupId the group ID
* @param userId the user ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching message-boards message, or null
if a matching message-boards message could not be found
*/
@Override
public MBMessage fetchByG_U_Last(
long groupId, long userId,
OrderByComparator orderByComparator) {
int count = countByG_U(groupId, userId);
if (count == 0) {
return null;
}
List list = findByG_U(
groupId, userId, count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the message-boards messages before and after the current message-boards message in the ordered set where groupId = ? and userId = ?.
*
* @param messageId the primary key of the current message-boards message
* @param groupId the group ID
* @param userId the user ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next message-boards message
* @throws NoSuchMessageException if a message-boards message with the primary key could not be found
*/
@Override
public MBMessage[] findByG_U_PrevAndNext(
long messageId, long groupId, long userId,
OrderByComparator orderByComparator)
throws NoSuchMessageException {
MBMessage mbMessage = findByPrimaryKey(messageId);
Session session = null;
try {
session = openSession();
MBMessage[] array = new MBMessageImpl[3];
array[0] = getByG_U_PrevAndNext(
session, mbMessage, groupId, userId, orderByComparator, true);
array[1] = mbMessage;
array[2] = getByG_U_PrevAndNext(
session, mbMessage, groupId, userId, orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected MBMessage getByG_U_PrevAndNext(
Session session, MBMessage mbMessage, long groupId, long userId,
OrderByComparator orderByComparator, boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
5 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_MBMESSAGE_WHERE);
sb.append(_FINDER_COLUMN_G_U_GROUPID_2);
sb.append(_FINDER_COLUMN_G_U_USERID_2);
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(MBMessageModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
queryPos.add(userId);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(mbMessage)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Returns all the message-boards messages that the user has permission to view where groupId = ? and userId = ?.
*
* @param groupId the group ID
* @param userId the user ID
* @return the matching message-boards messages that the user has permission to view
*/
@Override
public List filterFindByG_U(long groupId, long userId) {
return filterFindByG_U(
groupId, userId, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the message-boards messages that the user has permission to view where groupId = ? and userId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from MBMessageModelImpl
.
*
*
* @param groupId the group ID
* @param userId the user ID
* @param start the lower bound of the range of message-boards messages
* @param end the upper bound of the range of message-boards messages (not inclusive)
* @return the range of matching message-boards messages that the user has permission to view
*/
@Override
public List filterFindByG_U(
long groupId, long userId, int start, int end) {
return filterFindByG_U(groupId, userId, start, end, null);
}
/**
* Returns an ordered range of all the message-boards messages that the user has permissions to view where groupId = ? and userId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from MBMessageModelImpl
.
*
*
* @param groupId the group ID
* @param userId the user ID
* @param start the lower bound of the range of message-boards messages
* @param end the upper bound of the range of message-boards messages (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching message-boards messages that the user has permission to view
*/
@Override
public List filterFindByG_U(
long groupId, long userId, int start, int end,
OrderByComparator orderByComparator) {
if (!InlineSQLHelperUtil.isEnabled(groupId)) {
return findByG_U(groupId, userId, start, end, orderByComparator);
}
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(5);
}
if (getDB().isSupportsInlineDistinct()) {
sb.append(_FILTER_SQL_SELECT_MBMESSAGE_WHERE);
}
else {
sb.append(_FILTER_SQL_SELECT_MBMESSAGE_NO_INLINE_DISTINCT_WHERE_1);
}
sb.append(_FINDER_COLUMN_G_U_GROUPID_2);
sb.append(_FINDER_COLUMN_G_U_USERID_2);
if (!getDB().isSupportsInlineDistinct()) {
sb.append(_FILTER_SQL_SELECT_MBMESSAGE_NO_INLINE_DISTINCT_WHERE_2);
}
if (orderByComparator != null) {
if (getDB().isSupportsInlineDistinct()) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator, true);
}
else {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_TABLE, orderByComparator, true);
}
}
else {
if (getDB().isSupportsInlineDistinct()) {
sb.append(MBMessageModelImpl.ORDER_BY_JPQL);
}
else {
sb.append(MBMessageModelImpl.ORDER_BY_SQL);
}
}
String sql = InlineSQLHelperUtil.replacePermissionCheck(
sb.toString(), MBMessage.class.getName(),
_FILTER_ENTITY_TABLE_FILTER_PK_COLUMN, groupId);
Session session = null;
try {
session = openSession();
SQLQuery sqlQuery = session.createSynchronizedSQLQuery(sql);
if (getDB().isSupportsInlineDistinct()) {
sqlQuery.addEntity(_FILTER_ENTITY_ALIAS, MBMessageImpl.class);
}
else {
sqlQuery.addEntity(_FILTER_ENTITY_TABLE, MBMessageImpl.class);
}
QueryPos queryPos = QueryPos.getInstance(sqlQuery);
queryPos.add(groupId);
queryPos.add(userId);
return (List)QueryUtil.list(
sqlQuery, getDialect(), start, end);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
/**
* Returns the message-boards messages before and after the current message-boards message in the ordered set of message-boards messages that the user has permission to view where groupId = ? and userId = ?.
*
* @param messageId the primary key of the current message-boards message
* @param groupId the group ID
* @param userId the user ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next message-boards message
* @throws NoSuchMessageException if a message-boards message with the primary key could not be found
*/
@Override
public MBMessage[] filterFindByG_U_PrevAndNext(
long messageId, long groupId, long userId,
OrderByComparator orderByComparator)
throws NoSuchMessageException {
if (!InlineSQLHelperUtil.isEnabled(groupId)) {
return findByG_U_PrevAndNext(
messageId, groupId, userId, orderByComparator);
}
MBMessage mbMessage = findByPrimaryKey(messageId);
Session session = null;
try {
session = openSession();
MBMessage[] array = new MBMessageImpl[3];
array[0] = filterGetByG_U_PrevAndNext(
session, mbMessage, groupId, userId, orderByComparator, true);
array[1] = mbMessage;
array[2] = filterGetByG_U_PrevAndNext(
session, mbMessage, groupId, userId, orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected MBMessage filterGetByG_U_PrevAndNext(
Session session, MBMessage mbMessage, long groupId, long userId,
OrderByComparator orderByComparator, boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
6 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(5);
}
if (getDB().isSupportsInlineDistinct()) {
sb.append(_FILTER_SQL_SELECT_MBMESSAGE_WHERE);
}
else {
sb.append(_FILTER_SQL_SELECT_MBMESSAGE_NO_INLINE_DISTINCT_WHERE_1);
}
sb.append(_FINDER_COLUMN_G_U_GROUPID_2);
sb.append(_FINDER_COLUMN_G_U_USERID_2);
if (!getDB().isSupportsInlineDistinct()) {
sb.append(_FILTER_SQL_SELECT_MBMESSAGE_NO_INLINE_DISTINCT_WHERE_2);
}
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
if (getDB().isSupportsInlineDistinct()) {
sb.append(
getColumnName(
_ORDER_BY_ENTITY_ALIAS, orderByConditionFields[i],
true));
}
else {
sb.append(
getColumnName(
_ORDER_BY_ENTITY_TABLE, orderByConditionFields[i],
true));
}
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
if (getDB().isSupportsInlineDistinct()) {
sb.append(
getColumnName(
_ORDER_BY_ENTITY_ALIAS, orderByFields[i], true));
}
else {
sb.append(
getColumnName(
_ORDER_BY_ENTITY_TABLE, orderByFields[i], true));
}
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
if (getDB().isSupportsInlineDistinct()) {
sb.append(MBMessageModelImpl.ORDER_BY_JPQL);
}
else {
sb.append(MBMessageModelImpl.ORDER_BY_SQL);
}
}
String sql = InlineSQLHelperUtil.replacePermissionCheck(
sb.toString(), MBMessage.class.getName(),
_FILTER_ENTITY_TABLE_FILTER_PK_COLUMN, groupId);
SQLQuery sqlQuery = session.createSynchronizedSQLQuery(sql);
sqlQuery.setFirstResult(0);
sqlQuery.setMaxResults(2);
if (getDB().isSupportsInlineDistinct()) {
sqlQuery.addEntity(_FILTER_ENTITY_ALIAS, MBMessageImpl.class);
}
else {
sqlQuery.addEntity(_FILTER_ENTITY_TABLE, MBMessageImpl.class);
}
QueryPos queryPos = QueryPos.getInstance(sqlQuery);
queryPos.add(groupId);
queryPos.add(userId);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(mbMessage)) {
queryPos.add(orderByConditionValue);
}
}
List list = sqlQuery.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the message-boards messages where groupId = ? and userId = ? from the database.
*
* @param groupId the group ID
* @param userId the user ID
*/
@Override
public void removeByG_U(long groupId, long userId) {
for (MBMessage mbMessage :
findByG_U(
groupId, userId, QueryUtil.ALL_POS, QueryUtil.ALL_POS,
null)) {
remove(mbMessage);
}
}
/**
* Returns the number of message-boards messages where groupId = ? and userId = ?.
*
* @param groupId the group ID
* @param userId the user ID
* @return the number of matching message-boards messages
*/
@Override
public int countByG_U(long groupId, long userId) {
boolean productionMode = ctPersistenceHelper.isProductionMode(
MBMessage.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
Long count = null;
if (productionMode) {
finderPath = _finderPathCountByG_U;
finderArgs = new Object[] {groupId, userId};
count = (Long)finderCache.getResult(finderPath, finderArgs, this);
}
if (count == null) {
StringBundler sb = new StringBundler(3);
sb.append(_SQL_COUNT_MBMESSAGE_WHERE);
sb.append(_FINDER_COLUMN_G_U_GROUPID_2);
sb.append(_FINDER_COLUMN_G_U_USERID_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
queryPos.add(userId);
count = (Long)query.uniqueResult();
if (productionMode) {
finderCache.putResult(finderPath, finderArgs, count);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
/**
* Returns the number of message-boards messages that the user has permission to view where groupId = ? and userId = ?.
*
* @param groupId the group ID
* @param userId the user ID
* @return the number of matching message-boards messages that the user has permission to view
*/
@Override
public int filterCountByG_U(long groupId, long userId) {
if (!InlineSQLHelperUtil.isEnabled(groupId)) {
return countByG_U(groupId, userId);
}
StringBundler sb = new StringBundler(3);
sb.append(_FILTER_SQL_COUNT_MBMESSAGE_WHERE);
sb.append(_FINDER_COLUMN_G_U_GROUPID_2);
sb.append(_FINDER_COLUMN_G_U_USERID_2);
String sql = InlineSQLHelperUtil.replacePermissionCheck(
sb.toString(), MBMessage.class.getName(),
_FILTER_ENTITY_TABLE_FILTER_PK_COLUMN, groupId);
Session session = null;
try {
session = openSession();
SQLQuery sqlQuery = session.createSynchronizedSQLQuery(sql);
sqlQuery.addScalar(
COUNT_COLUMN_NAME, com.liferay.portal.kernel.dao.orm.Type.LONG);
QueryPos queryPos = QueryPos.getInstance(sqlQuery);
queryPos.add(groupId);
queryPos.add(userId);
Long count = (Long)sqlQuery.uniqueResult();
return count.intValue();
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
private static final String _FINDER_COLUMN_G_U_GROUPID_2 =
"mbMessage.groupId = ? AND ";
private static final String _FINDER_COLUMN_G_U_USERID_2 =
"mbMessage.userId = ? AND (mbMessage.categoryId != -1) AND (mbMessage.anonymous = [$FALSE$])";
private FinderPath _finderPathWithPaginationFindByG_C;
private FinderPath _finderPathWithoutPaginationFindByG_C;
private FinderPath _finderPathCountByG_C;
/**
* Returns all the message-boards messages where groupId = ? and categoryId = ?.
*
* @param groupId the group ID
* @param categoryId the category ID
* @return the matching message-boards messages
*/
@Override
public List findByG_C(long groupId, long categoryId) {
return findByG_C(
groupId, categoryId, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the message-boards messages where groupId = ? and categoryId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from MBMessageModelImpl
.
*
*
* @param groupId the group ID
* @param categoryId the category ID
* @param start the lower bound of the range of message-boards messages
* @param end the upper bound of the range of message-boards messages (not inclusive)
* @return the range of matching message-boards messages
*/
@Override
public List findByG_C(
long groupId, long categoryId, int start, int end) {
return findByG_C(groupId, categoryId, start, end, null);
}
/**
* Returns an ordered range of all the message-boards messages where groupId = ? and categoryId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from MBMessageModelImpl
.
*
*
* @param groupId the group ID
* @param categoryId the category ID
* @param start the lower bound of the range of message-boards messages
* @param end the upper bound of the range of message-boards messages (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching message-boards messages
*/
@Override
public List findByG_C(
long groupId, long categoryId, int start, int end,
OrderByComparator orderByComparator) {
return findByG_C(
groupId, categoryId, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the message-boards messages where groupId = ? and categoryId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from MBMessageModelImpl
.
*
*
* @param groupId the group ID
* @param categoryId the category ID
* @param start the lower bound of the range of message-boards messages
* @param end the upper bound of the range of message-boards messages (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching message-boards messages
*/
@Override
public List findByG_C(
long groupId, long categoryId, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
boolean productionMode = ctPersistenceHelper.isProductionMode(
MBMessage.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache && productionMode) {
finderPath = _finderPathWithoutPaginationFindByG_C;
finderArgs = new Object[] {groupId, categoryId};
}
}
else if (useFinderCache && productionMode) {
finderPath = _finderPathWithPaginationFindByG_C;
finderArgs = new Object[] {
groupId, categoryId, start, end, orderByComparator
};
}
List list = null;
if (useFinderCache && productionMode) {
list = (List)finderCache.getResult(
finderPath, finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (MBMessage mbMessage : list) {
if ((groupId != mbMessage.getGroupId()) ||
(categoryId != mbMessage.getCategoryId())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_MBMESSAGE_WHERE);
sb.append(_FINDER_COLUMN_G_C_GROUPID_2);
sb.append(_FINDER_COLUMN_G_C_CATEGORYID_2);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(MBMessageModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
queryPos.add(categoryId);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache && productionMode) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first message-boards message in the ordered set where groupId = ? and categoryId = ?.
*
* @param groupId the group ID
* @param categoryId the category ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching message-boards message
* @throws NoSuchMessageException if a matching message-boards message could not be found
*/
@Override
public MBMessage findByG_C_First(
long groupId, long categoryId,
OrderByComparator orderByComparator)
throws NoSuchMessageException {
MBMessage mbMessage = fetchByG_C_First(
groupId, categoryId, orderByComparator);
if (mbMessage != null) {
return mbMessage;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("groupId=");
sb.append(groupId);
sb.append(", categoryId=");
sb.append(categoryId);
sb.append("}");
throw new NoSuchMessageException(sb.toString());
}
/**
* Returns the first message-boards message in the ordered set where groupId = ? and categoryId = ?.
*
* @param groupId the group ID
* @param categoryId the category ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching message-boards message, or null
if a matching message-boards message could not be found
*/
@Override
public MBMessage fetchByG_C_First(
long groupId, long categoryId,
OrderByComparator orderByComparator) {
List list = findByG_C(
groupId, categoryId, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last message-boards message in the ordered set where groupId = ? and categoryId = ?.
*
* @param groupId the group ID
* @param categoryId the category ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching message-boards message
* @throws NoSuchMessageException if a matching message-boards message could not be found
*/
@Override
public MBMessage findByG_C_Last(
long groupId, long categoryId,
OrderByComparator orderByComparator)
throws NoSuchMessageException {
MBMessage mbMessage = fetchByG_C_Last(
groupId, categoryId, orderByComparator);
if (mbMessage != null) {
return mbMessage;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("groupId=");
sb.append(groupId);
sb.append(", categoryId=");
sb.append(categoryId);
sb.append("}");
throw new NoSuchMessageException(sb.toString());
}
/**
* Returns the last message-boards message in the ordered set where groupId = ? and categoryId = ?.
*
* @param groupId the group ID
* @param categoryId the category ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching message-boards message, or null
if a matching message-boards message could not be found
*/
@Override
public MBMessage fetchByG_C_Last(
long groupId, long categoryId,
OrderByComparator orderByComparator) {
int count = countByG_C(groupId, categoryId);
if (count == 0) {
return null;
}
List list = findByG_C(
groupId, categoryId, count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the message-boards messages before and after the current message-boards message in the ordered set where groupId = ? and categoryId = ?.
*
* @param messageId the primary key of the current message-boards message
* @param groupId the group ID
* @param categoryId the category ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next message-boards message
* @throws NoSuchMessageException if a message-boards message with the primary key could not be found
*/
@Override
public MBMessage[] findByG_C_PrevAndNext(
long messageId, long groupId, long categoryId,
OrderByComparator orderByComparator)
throws NoSuchMessageException {
MBMessage mbMessage = findByPrimaryKey(messageId);
Session session = null;
try {
session = openSession();
MBMessage[] array = new MBMessageImpl[3];
array[0] = getByG_C_PrevAndNext(
session, mbMessage, groupId, categoryId, orderByComparator,
true);
array[1] = mbMessage;
array[2] = getByG_C_PrevAndNext(
session, mbMessage, groupId, categoryId, orderByComparator,
false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected MBMessage getByG_C_PrevAndNext(
Session session, MBMessage mbMessage, long groupId, long categoryId,
OrderByComparator orderByComparator, boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
5 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_MBMESSAGE_WHERE);
sb.append(_FINDER_COLUMN_G_C_GROUPID_2);
sb.append(_FINDER_COLUMN_G_C_CATEGORYID_2);
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(MBMessageModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
queryPos.add(categoryId);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(mbMessage)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Returns all the message-boards messages that the user has permission to view where groupId = ? and categoryId = ?.
*
* @param groupId the group ID
* @param categoryId the category ID
* @return the matching message-boards messages that the user has permission to view
*/
@Override
public List filterFindByG_C(long groupId, long categoryId) {
return filterFindByG_C(
groupId, categoryId, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the message-boards messages that the user has permission to view where groupId = ? and categoryId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from MBMessageModelImpl
.
*
*
* @param groupId the group ID
* @param categoryId the category ID
* @param start the lower bound of the range of message-boards messages
* @param end the upper bound of the range of message-boards messages (not inclusive)
* @return the range of matching message-boards messages that the user has permission to view
*/
@Override
public List filterFindByG_C(
long groupId, long categoryId, int start, int end) {
return filterFindByG_C(groupId, categoryId, start, end, null);
}
/**
* Returns an ordered range of all the message-boards messages that the user has permissions to view where groupId = ? and categoryId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from MBMessageModelImpl
.
*
*
* @param groupId the group ID
* @param categoryId the category ID
* @param start the lower bound of the range of message-boards messages
* @param end the upper bound of the range of message-boards messages (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching message-boards messages that the user has permission to view
*/
@Override
public List filterFindByG_C(
long groupId, long categoryId, int start, int end,
OrderByComparator orderByComparator) {
if (!InlineSQLHelperUtil.isEnabled(groupId)) {
return findByG_C(
groupId, categoryId, start, end, orderByComparator);
}
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(5);
}
if (getDB().isSupportsInlineDistinct()) {
sb.append(_FILTER_SQL_SELECT_MBMESSAGE_WHERE);
}
else {
sb.append(_FILTER_SQL_SELECT_MBMESSAGE_NO_INLINE_DISTINCT_WHERE_1);
}
sb.append(_FINDER_COLUMN_G_C_GROUPID_2);
sb.append(_FINDER_COLUMN_G_C_CATEGORYID_2);
if (!getDB().isSupportsInlineDistinct()) {
sb.append(_FILTER_SQL_SELECT_MBMESSAGE_NO_INLINE_DISTINCT_WHERE_2);
}
if (orderByComparator != null) {
if (getDB().isSupportsInlineDistinct()) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator, true);
}
else {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_TABLE, orderByComparator, true);
}
}
else {
if (getDB().isSupportsInlineDistinct()) {
sb.append(MBMessageModelImpl.ORDER_BY_JPQL);
}
else {
sb.append(MBMessageModelImpl.ORDER_BY_SQL);
}
}
String sql = InlineSQLHelperUtil.replacePermissionCheck(
sb.toString(), MBMessage.class.getName(),
_FILTER_ENTITY_TABLE_FILTER_PK_COLUMN, groupId);
Session session = null;
try {
session = openSession();
SQLQuery sqlQuery = session.createSynchronizedSQLQuery(sql);
if (getDB().isSupportsInlineDistinct()) {
sqlQuery.addEntity(_FILTER_ENTITY_ALIAS, MBMessageImpl.class);
}
else {
sqlQuery.addEntity(_FILTER_ENTITY_TABLE, MBMessageImpl.class);
}
QueryPos queryPos = QueryPos.getInstance(sqlQuery);
queryPos.add(groupId);
queryPos.add(categoryId);
return (List)QueryUtil.list(
sqlQuery, getDialect(), start, end);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
/**
* Returns the message-boards messages before and after the current message-boards message in the ordered set of message-boards messages that the user has permission to view where groupId = ? and categoryId = ?.
*
* @param messageId the primary key of the current message-boards message
* @param groupId the group ID
* @param categoryId the category ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next message-boards message
* @throws NoSuchMessageException if a message-boards message with the primary key could not be found
*/
@Override
public MBMessage[] filterFindByG_C_PrevAndNext(
long messageId, long groupId, long categoryId,
OrderByComparator orderByComparator)
throws NoSuchMessageException {
if (!InlineSQLHelperUtil.isEnabled(groupId)) {
return findByG_C_PrevAndNext(
messageId, groupId, categoryId, orderByComparator);
}
MBMessage mbMessage = findByPrimaryKey(messageId);
Session session = null;
try {
session = openSession();
MBMessage[] array = new MBMessageImpl[3];
array[0] = filterGetByG_C_PrevAndNext(
session, mbMessage, groupId, categoryId, orderByComparator,
true);
array[1] = mbMessage;
array[2] = filterGetByG_C_PrevAndNext(
session, mbMessage, groupId, categoryId, orderByComparator,
false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected MBMessage filterGetByG_C_PrevAndNext(
Session session, MBMessage mbMessage, long groupId, long categoryId,
OrderByComparator orderByComparator, boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
6 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(5);
}
if (getDB().isSupportsInlineDistinct()) {
sb.append(_FILTER_SQL_SELECT_MBMESSAGE_WHERE);
}
else {
sb.append(_FILTER_SQL_SELECT_MBMESSAGE_NO_INLINE_DISTINCT_WHERE_1);
}
sb.append(_FINDER_COLUMN_G_C_GROUPID_2);
sb.append(_FINDER_COLUMN_G_C_CATEGORYID_2);
if (!getDB().isSupportsInlineDistinct()) {
sb.append(_FILTER_SQL_SELECT_MBMESSAGE_NO_INLINE_DISTINCT_WHERE_2);
}
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
if (getDB().isSupportsInlineDistinct()) {
sb.append(
getColumnName(
_ORDER_BY_ENTITY_ALIAS, orderByConditionFields[i],
true));
}
else {
sb.append(
getColumnName(
_ORDER_BY_ENTITY_TABLE, orderByConditionFields[i],
true));
}
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
if (getDB().isSupportsInlineDistinct()) {
sb.append(
getColumnName(
_ORDER_BY_ENTITY_ALIAS, orderByFields[i], true));
}
else {
sb.append(
getColumnName(
_ORDER_BY_ENTITY_TABLE, orderByFields[i], true));
}
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
if (getDB().isSupportsInlineDistinct()) {
sb.append(MBMessageModelImpl.ORDER_BY_JPQL);
}
else {
sb.append(MBMessageModelImpl.ORDER_BY_SQL);
}
}
String sql = InlineSQLHelperUtil.replacePermissionCheck(
sb.toString(), MBMessage.class.getName(),
_FILTER_ENTITY_TABLE_FILTER_PK_COLUMN, groupId);
SQLQuery sqlQuery = session.createSynchronizedSQLQuery(sql);
sqlQuery.setFirstResult(0);
sqlQuery.setMaxResults(2);
if (getDB().isSupportsInlineDistinct()) {
sqlQuery.addEntity(_FILTER_ENTITY_ALIAS, MBMessageImpl.class);
}
else {
sqlQuery.addEntity(_FILTER_ENTITY_TABLE, MBMessageImpl.class);
}
QueryPos queryPos = QueryPos.getInstance(sqlQuery);
queryPos.add(groupId);
queryPos.add(categoryId);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(mbMessage)) {
queryPos.add(orderByConditionValue);
}
}
List list = sqlQuery.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the message-boards messages where groupId = ? and categoryId = ? from the database.
*
* @param groupId the group ID
* @param categoryId the category ID
*/
@Override
public void removeByG_C(long groupId, long categoryId) {
for (MBMessage mbMessage :
findByG_C(
groupId, categoryId, QueryUtil.ALL_POS, QueryUtil.ALL_POS,
null)) {
remove(mbMessage);
}
}
/**
* Returns the number of message-boards messages where groupId = ? and categoryId = ?.
*
* @param groupId the group ID
* @param categoryId the category ID
* @return the number of matching message-boards messages
*/
@Override
public int countByG_C(long groupId, long categoryId) {
boolean productionMode = ctPersistenceHelper.isProductionMode(
MBMessage.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
Long count = null;
if (productionMode) {
finderPath = _finderPathCountByG_C;
finderArgs = new Object[] {groupId, categoryId};
count = (Long)finderCache.getResult(finderPath, finderArgs, this);
}
if (count == null) {
StringBundler sb = new StringBundler(3);
sb.append(_SQL_COUNT_MBMESSAGE_WHERE);
sb.append(_FINDER_COLUMN_G_C_GROUPID_2);
sb.append(_FINDER_COLUMN_G_C_CATEGORYID_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
queryPos.add(categoryId);
count = (Long)query.uniqueResult();
if (productionMode) {
finderCache.putResult(finderPath, finderArgs, count);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
/**
* Returns the number of message-boards messages that the user has permission to view where groupId = ? and categoryId = ?.
*
* @param groupId the group ID
* @param categoryId the category ID
* @return the number of matching message-boards messages that the user has permission to view
*/
@Override
public int filterCountByG_C(long groupId, long categoryId) {
if (!InlineSQLHelperUtil.isEnabled(groupId)) {
return countByG_C(groupId, categoryId);
}
StringBundler sb = new StringBundler(3);
sb.append(_FILTER_SQL_COUNT_MBMESSAGE_WHERE);
sb.append(_FINDER_COLUMN_G_C_GROUPID_2);
sb.append(_FINDER_COLUMN_G_C_CATEGORYID_2);
String sql = InlineSQLHelperUtil.replacePermissionCheck(
sb.toString(), MBMessage.class.getName(),
_FILTER_ENTITY_TABLE_FILTER_PK_COLUMN, groupId);
Session session = null;
try {
session = openSession();
SQLQuery sqlQuery = session.createSynchronizedSQLQuery(sql);
sqlQuery.addScalar(
COUNT_COLUMN_NAME, com.liferay.portal.kernel.dao.orm.Type.LONG);
QueryPos queryPos = QueryPos.getInstance(sqlQuery);
queryPos.add(groupId);
queryPos.add(categoryId);
Long count = (Long)sqlQuery.uniqueResult();
return count.intValue();
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
private static final String _FINDER_COLUMN_G_C_GROUPID_2 =
"mbMessage.groupId = ? AND ";
private static final String _FINDER_COLUMN_G_C_CATEGORYID_2 =
"mbMessage.categoryId = ?";
private FinderPath _finderPathFetchByG_US;
private FinderPath _finderPathCountByG_US;
/**
* Returns the message-boards message where groupId = ? and urlSubject = ? or throws a NoSuchMessageException
if it could not be found.
*
* @param groupId the group ID
* @param urlSubject the url subject
* @return the matching message-boards message
* @throws NoSuchMessageException if a matching message-boards message could not be found
*/
@Override
public MBMessage findByG_US(long groupId, String urlSubject)
throws NoSuchMessageException {
MBMessage mbMessage = fetchByG_US(groupId, urlSubject);
if (mbMessage == null) {
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("groupId=");
sb.append(groupId);
sb.append(", urlSubject=");
sb.append(urlSubject);
sb.append("}");
if (_log.isDebugEnabled()) {
_log.debug(sb.toString());
}
throw new NoSuchMessageException(sb.toString());
}
return mbMessage;
}
/**
* Returns the message-boards message where groupId = ? and urlSubject = ? or returns null
if it could not be found. Uses the finder cache.
*
* @param groupId the group ID
* @param urlSubject the url subject
* @return the matching message-boards message, or null
if a matching message-boards message could not be found
*/
@Override
public MBMessage fetchByG_US(long groupId, String urlSubject) {
return fetchByG_US(groupId, urlSubject, true);
}
/**
* Returns the message-boards message where groupId = ? and urlSubject = ? or returns null
if it could not be found, optionally using the finder cache.
*
* @param groupId the group ID
* @param urlSubject the url subject
* @param useFinderCache whether to use the finder cache
* @return the matching message-boards message, or null
if a matching message-boards message could not be found
*/
@Override
public MBMessage fetchByG_US(
long groupId, String urlSubject, boolean useFinderCache) {
urlSubject = Objects.toString(urlSubject, "");
boolean productionMode = ctPersistenceHelper.isProductionMode(
MBMessage.class);
Object[] finderArgs = null;
if (useFinderCache && productionMode) {
finderArgs = new Object[] {groupId, urlSubject};
}
Object result = null;
if (useFinderCache && productionMode) {
result = finderCache.getResult(
_finderPathFetchByG_US, finderArgs, this);
}
if (result instanceof MBMessage) {
MBMessage mbMessage = (MBMessage)result;
if ((groupId != mbMessage.getGroupId()) ||
!Objects.equals(urlSubject, mbMessage.getUrlSubject())) {
result = null;
}
}
if (result == null) {
StringBundler sb = new StringBundler(4);
sb.append(_SQL_SELECT_MBMESSAGE_WHERE);
sb.append(_FINDER_COLUMN_G_US_GROUPID_2);
boolean bindUrlSubject = false;
if (urlSubject.isEmpty()) {
sb.append(_FINDER_COLUMN_G_US_URLSUBJECT_3);
}
else {
bindUrlSubject = true;
sb.append(_FINDER_COLUMN_G_US_URLSUBJECT_2);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
if (bindUrlSubject) {
queryPos.add(urlSubject);
}
List list = query.list();
if (list.isEmpty()) {
if (useFinderCache && productionMode) {
finderCache.putResult(
_finderPathFetchByG_US, finderArgs, list);
}
}
else {
MBMessage mbMessage = list.get(0);
result = mbMessage;
cacheResult(mbMessage);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
if (result instanceof List>) {
return null;
}
else {
return (MBMessage)result;
}
}
/**
* Removes the message-boards message where groupId = ? and urlSubject = ? from the database.
*
* @param groupId the group ID
* @param urlSubject the url subject
* @return the message-boards message that was removed
*/
@Override
public MBMessage removeByG_US(long groupId, String urlSubject)
throws NoSuchMessageException {
MBMessage mbMessage = findByG_US(groupId, urlSubject);
return remove(mbMessage);
}
/**
* Returns the number of message-boards messages where groupId = ? and urlSubject = ?.
*
* @param groupId the group ID
* @param urlSubject the url subject
* @return the number of matching message-boards messages
*/
@Override
public int countByG_US(long groupId, String urlSubject) {
urlSubject = Objects.toString(urlSubject, "");
boolean productionMode = ctPersistenceHelper.isProductionMode(
MBMessage.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
Long count = null;
if (productionMode) {
finderPath = _finderPathCountByG_US;
finderArgs = new Object[] {groupId, urlSubject};
count = (Long)finderCache.getResult(finderPath, finderArgs, this);
}
if (count == null) {
StringBundler sb = new StringBundler(3);
sb.append(_SQL_COUNT_MBMESSAGE_WHERE);
sb.append(_FINDER_COLUMN_G_US_GROUPID_2);
boolean bindUrlSubject = false;
if (urlSubject.isEmpty()) {
sb.append(_FINDER_COLUMN_G_US_URLSUBJECT_3);
}
else {
bindUrlSubject = true;
sb.append(_FINDER_COLUMN_G_US_URLSUBJECT_2);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
if (bindUrlSubject) {
queryPos.add(urlSubject);
}
count = (Long)query.uniqueResult();
if (productionMode) {
finderCache.putResult(finderPath, finderArgs, count);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String _FINDER_COLUMN_G_US_GROUPID_2 =
"mbMessage.groupId = ? AND ";
private static final String _FINDER_COLUMN_G_US_URLSUBJECT_2 =
"mbMessage.urlSubject = ?";
private static final String _FINDER_COLUMN_G_US_URLSUBJECT_3 =
"(mbMessage.urlSubject IS NULL OR mbMessage.urlSubject = '')";
private FinderPath _finderPathWithPaginationFindByG_S;
private FinderPath _finderPathWithoutPaginationFindByG_S;
private FinderPath _finderPathCountByG_S;
/**
* Returns all the message-boards messages where groupId = ? and status = ?.
*
* @param groupId the group ID
* @param status the status
* @return the matching message-boards messages
*/
@Override
public List findByG_S(long groupId, int status) {
return findByG_S(
groupId, status, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the message-boards messages where groupId = ? and status = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from MBMessageModelImpl
.
*
*
* @param groupId the group ID
* @param status the status
* @param start the lower bound of the range of message-boards messages
* @param end the upper bound of the range of message-boards messages (not inclusive)
* @return the range of matching message-boards messages
*/
@Override
public List findByG_S(
long groupId, int status, int start, int end) {
return findByG_S(groupId, status, start, end, null);
}
/**
* Returns an ordered range of all the message-boards messages where groupId = ? and status = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from MBMessageModelImpl
.
*
*
* @param groupId the group ID
* @param status the status
* @param start the lower bound of the range of message-boards messages
* @param end the upper bound of the range of message-boards messages (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching message-boards messages
*/
@Override
public List findByG_S(
long groupId, int status, int start, int end,
OrderByComparator orderByComparator) {
return findByG_S(groupId, status, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the message-boards messages where groupId = ? and status = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from MBMessageModelImpl
.
*
*
* @param groupId the group ID
* @param status the status
* @param start the lower bound of the range of message-boards messages
* @param end the upper bound of the range of message-boards messages (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching message-boards messages
*/
@Override
public List findByG_S(
long groupId, int status, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
boolean productionMode = ctPersistenceHelper.isProductionMode(
MBMessage.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache && productionMode) {
finderPath = _finderPathWithoutPaginationFindByG_S;
finderArgs = new Object[] {groupId, status};
}
}
else if (useFinderCache && productionMode) {
finderPath = _finderPathWithPaginationFindByG_S;
finderArgs = new Object[] {
groupId, status, start, end, orderByComparator
};
}
List list = null;
if (useFinderCache && productionMode) {
list = (List)finderCache.getResult(
finderPath, finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (MBMessage mbMessage : list) {
if ((groupId != mbMessage.getGroupId()) ||
(status != mbMessage.getStatus())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_MBMESSAGE_WHERE);
sb.append(_FINDER_COLUMN_G_S_GROUPID_2);
sb.append(_FINDER_COLUMN_G_S_STATUS_2);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(MBMessageModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
queryPos.add(status);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache && productionMode) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first message-boards message in the ordered set where groupId = ? and status = ?.
*
* @param groupId the group ID
* @param status the status
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching message-boards message
* @throws NoSuchMessageException if a matching message-boards message could not be found
*/
@Override
public MBMessage findByG_S_First(
long groupId, int status,
OrderByComparator orderByComparator)
throws NoSuchMessageException {
MBMessage mbMessage = fetchByG_S_First(
groupId, status, orderByComparator);
if (mbMessage != null) {
return mbMessage;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("groupId=");
sb.append(groupId);
sb.append(", status=");
sb.append(status);
sb.append("}");
throw new NoSuchMessageException(sb.toString());
}
/**
* Returns the first message-boards message in the ordered set where groupId = ? and status = ?.
*
* @param groupId the group ID
* @param status the status
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching message-boards message, or null
if a matching message-boards message could not be found
*/
@Override
public MBMessage fetchByG_S_First(
long groupId, int status,
OrderByComparator orderByComparator) {
List list = findByG_S(
groupId, status, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last message-boards message in the ordered set where groupId = ? and status = ?.
*
* @param groupId the group ID
* @param status the status
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching message-boards message
* @throws NoSuchMessageException if a matching message-boards message could not be found
*/
@Override
public MBMessage findByG_S_Last(
long groupId, int status,
OrderByComparator orderByComparator)
throws NoSuchMessageException {
MBMessage mbMessage = fetchByG_S_Last(
groupId, status, orderByComparator);
if (mbMessage != null) {
return mbMessage;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("groupId=");
sb.append(groupId);
sb.append(", status=");
sb.append(status);
sb.append("}");
throw new NoSuchMessageException(sb.toString());
}
/**
* Returns the last message-boards message in the ordered set where groupId = ? and status = ?.
*
* @param groupId the group ID
* @param status the status
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching message-boards message, or null
if a matching message-boards message could not be found
*/
@Override
public MBMessage fetchByG_S_Last(
long groupId, int status,
OrderByComparator orderByComparator) {
int count = countByG_S(groupId, status);
if (count == 0) {
return null;
}
List list = findByG_S(
groupId, status, count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the message-boards messages before and after the current message-boards message in the ordered set where groupId = ? and status = ?.
*
* @param messageId the primary key of the current message-boards message
* @param groupId the group ID
* @param status the status
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next message-boards message
* @throws NoSuchMessageException if a message-boards message with the primary key could not be found
*/
@Override
public MBMessage[] findByG_S_PrevAndNext(
long messageId, long groupId, int status,
OrderByComparator orderByComparator)
throws NoSuchMessageException {
MBMessage mbMessage = findByPrimaryKey(messageId);
Session session = null;
try {
session = openSession();
MBMessage[] array = new MBMessageImpl[3];
array[0] = getByG_S_PrevAndNext(
session, mbMessage, groupId, status, orderByComparator, true);
array[1] = mbMessage;
array[2] = getByG_S_PrevAndNext(
session, mbMessage, groupId, status, orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected MBMessage getByG_S_PrevAndNext(
Session session, MBMessage mbMessage, long groupId, int status,
OrderByComparator orderByComparator, boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
5 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_MBMESSAGE_WHERE);
sb.append(_FINDER_COLUMN_G_S_GROUPID_2);
sb.append(_FINDER_COLUMN_G_S_STATUS_2);
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(MBMessageModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
queryPos.add(status);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(mbMessage)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Returns all the message-boards messages that the user has permission to view where groupId = ? and status = ?.
*
* @param groupId the group ID
* @param status the status
* @return the matching message-boards messages that the user has permission to view
*/
@Override
public List filterFindByG_S(long groupId, int status) {
return filterFindByG_S(
groupId, status, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the message-boards messages that the user has permission to view where groupId = ? and status = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from MBMessageModelImpl
.
*
*
* @param groupId the group ID
* @param status the status
* @param start the lower bound of the range of message-boards messages
* @param end the upper bound of the range of message-boards messages (not inclusive)
* @return the range of matching message-boards messages that the user has permission to view
*/
@Override
public List filterFindByG_S(
long groupId, int status, int start, int end) {
return filterFindByG_S(groupId, status, start, end, null);
}
/**
* Returns an ordered range of all the message-boards messages that the user has permissions to view where groupId = ? and status = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from MBMessageModelImpl
.
*
*
* @param groupId the group ID
* @param status the status
* @param start the lower bound of the range of message-boards messages
* @param end the upper bound of the range of message-boards messages (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching message-boards messages that the user has permission to view
*/
@Override
public List filterFindByG_S(
long groupId, int status, int start, int end,
OrderByComparator orderByComparator) {
if (!InlineSQLHelperUtil.isEnabled(groupId)) {
return findByG_S(groupId, status, start, end, orderByComparator);
}
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(5);
}
if (getDB().isSupportsInlineDistinct()) {
sb.append(_FILTER_SQL_SELECT_MBMESSAGE_WHERE);
}
else {
sb.append(_FILTER_SQL_SELECT_MBMESSAGE_NO_INLINE_DISTINCT_WHERE_1);
}
sb.append(_FINDER_COLUMN_G_S_GROUPID_2);
sb.append(_FINDER_COLUMN_G_S_STATUS_2);
if (!getDB().isSupportsInlineDistinct()) {
sb.append(_FILTER_SQL_SELECT_MBMESSAGE_NO_INLINE_DISTINCT_WHERE_2);
}
if (orderByComparator != null) {
if (getDB().isSupportsInlineDistinct()) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator, true);
}
else {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_TABLE, orderByComparator, true);
}
}
else {
if (getDB().isSupportsInlineDistinct()) {
sb.append(MBMessageModelImpl.ORDER_BY_JPQL);
}
else {
sb.append(MBMessageModelImpl.ORDER_BY_SQL);
}
}
String sql = InlineSQLHelperUtil.replacePermissionCheck(
sb.toString(), MBMessage.class.getName(),
_FILTER_ENTITY_TABLE_FILTER_PK_COLUMN, groupId);
Session session = null;
try {
session = openSession();
SQLQuery sqlQuery = session.createSynchronizedSQLQuery(sql);
if (getDB().isSupportsInlineDistinct()) {
sqlQuery.addEntity(_FILTER_ENTITY_ALIAS, MBMessageImpl.class);
}
else {
sqlQuery.addEntity(_FILTER_ENTITY_TABLE, MBMessageImpl.class);
}
QueryPos queryPos = QueryPos.getInstance(sqlQuery);
queryPos.add(groupId);
queryPos.add(status);
return (List)QueryUtil.list(
sqlQuery, getDialect(), start, end);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
/**
* Returns the message-boards messages before and after the current message-boards message in the ordered set of message-boards messages that the user has permission to view where groupId = ? and status = ?.
*
* @param messageId the primary key of the current message-boards message
* @param groupId the group ID
* @param status the status
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next message-boards message
* @throws NoSuchMessageException if a message-boards message with the primary key could not be found
*/
@Override
public MBMessage[] filterFindByG_S_PrevAndNext(
long messageId, long groupId, int status,
OrderByComparator orderByComparator)
throws NoSuchMessageException {
if (!InlineSQLHelperUtil.isEnabled(groupId)) {
return findByG_S_PrevAndNext(
messageId, groupId, status, orderByComparator);
}
MBMessage mbMessage = findByPrimaryKey(messageId);
Session session = null;
try {
session = openSession();
MBMessage[] array = new MBMessageImpl[3];
array[0] = filterGetByG_S_PrevAndNext(
session, mbMessage, groupId, status, orderByComparator, true);
array[1] = mbMessage;
array[2] = filterGetByG_S_PrevAndNext(
session, mbMessage, groupId, status, orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected MBMessage filterGetByG_S_PrevAndNext(
Session session, MBMessage mbMessage, long groupId, int status,
OrderByComparator orderByComparator, boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
6 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(5);
}
if (getDB().isSupportsInlineDistinct()) {
sb.append(_FILTER_SQL_SELECT_MBMESSAGE_WHERE);
}
else {
sb.append(_FILTER_SQL_SELECT_MBMESSAGE_NO_INLINE_DISTINCT_WHERE_1);
}
sb.append(_FINDER_COLUMN_G_S_GROUPID_2);
sb.append(_FINDER_COLUMN_G_S_STATUS_2);
if (!getDB().isSupportsInlineDistinct()) {
sb.append(_FILTER_SQL_SELECT_MBMESSAGE_NO_INLINE_DISTINCT_WHERE_2);
}
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
if (getDB().isSupportsInlineDistinct()) {
sb.append(
getColumnName(
_ORDER_BY_ENTITY_ALIAS, orderByConditionFields[i],
true));
}
else {
sb.append(
getColumnName(
_ORDER_BY_ENTITY_TABLE, orderByConditionFields[i],
true));
}
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
if (getDB().isSupportsInlineDistinct()) {
sb.append(
getColumnName(
_ORDER_BY_ENTITY_ALIAS, orderByFields[i], true));
}
else {
sb.append(
getColumnName(
_ORDER_BY_ENTITY_TABLE, orderByFields[i], true));
}
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
if (getDB().isSupportsInlineDistinct()) {
sb.append(MBMessageModelImpl.ORDER_BY_JPQL);
}
else {
sb.append(MBMessageModelImpl.ORDER_BY_SQL);
}
}
String sql = InlineSQLHelperUtil.replacePermissionCheck(
sb.toString(), MBMessage.class.getName(),
_FILTER_ENTITY_TABLE_FILTER_PK_COLUMN, groupId);
SQLQuery sqlQuery = session.createSynchronizedSQLQuery(sql);
sqlQuery.setFirstResult(0);
sqlQuery.setMaxResults(2);
if (getDB().isSupportsInlineDistinct()) {
sqlQuery.addEntity(_FILTER_ENTITY_ALIAS, MBMessageImpl.class);
}
else {
sqlQuery.addEntity(_FILTER_ENTITY_TABLE, MBMessageImpl.class);
}
QueryPos queryPos = QueryPos.getInstance(sqlQuery);
queryPos.add(groupId);
queryPos.add(status);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(mbMessage)) {
queryPos.add(orderByConditionValue);
}
}
List list = sqlQuery.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the message-boards messages where groupId = ? and status = ? from the database.
*
* @param groupId the group ID
* @param status the status
*/
@Override
public void removeByG_S(long groupId, int status) {
for (MBMessage mbMessage :
findByG_S(
groupId, status, QueryUtil.ALL_POS, QueryUtil.ALL_POS,
null)) {
remove(mbMessage);
}
}
/**
* Returns the number of message-boards messages where groupId = ? and status = ?.
*
* @param groupId the group ID
* @param status the status
* @return the number of matching message-boards messages
*/
@Override
public int countByG_S(long groupId, int status) {
boolean productionMode = ctPersistenceHelper.isProductionMode(
MBMessage.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
Long count = null;
if (productionMode) {
finderPath = _finderPathCountByG_S;
finderArgs = new Object[] {groupId, status};
count = (Long)finderCache.getResult(finderPath, finderArgs, this);
}
if (count == null) {
StringBundler sb = new StringBundler(3);
sb.append(_SQL_COUNT_MBMESSAGE_WHERE);
sb.append(_FINDER_COLUMN_G_S_GROUPID_2);
sb.append(_FINDER_COLUMN_G_S_STATUS_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(groupId);
queryPos.add(status);
count = (Long)query.uniqueResult();
if (productionMode) {
finderCache.putResult(finderPath, finderArgs, count);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
/**
* Returns the number of message-boards messages that the user has permission to view where groupId = ? and status = ?.
*
* @param groupId the group ID
* @param status the status
* @return the number of matching message-boards messages that the user has permission to view
*/
@Override
public int filterCountByG_S(long groupId, int status) {
if (!InlineSQLHelperUtil.isEnabled(groupId)) {
return countByG_S(groupId, status);
}
StringBundler sb = new StringBundler(3);
sb.append(_FILTER_SQL_COUNT_MBMESSAGE_WHERE);
sb.append(_FINDER_COLUMN_G_S_GROUPID_2);
sb.append(_FINDER_COLUMN_G_S_STATUS_2);
String sql = InlineSQLHelperUtil.replacePermissionCheck(
sb.toString(), MBMessage.class.getName(),
_FILTER_ENTITY_TABLE_FILTER_PK_COLUMN, groupId);
Session session = null;
try {
session = openSession();
SQLQuery sqlQuery = session.createSynchronizedSQLQuery(sql);
sqlQuery.addScalar(
COUNT_COLUMN_NAME, com.liferay.portal.kernel.dao.orm.Type.LONG);
QueryPos queryPos = QueryPos.getInstance(sqlQuery);
queryPos.add(groupId);
queryPos.add(status);
Long count = (Long)sqlQuery.uniqueResult();
return count.intValue();
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
private static final String _FINDER_COLUMN_G_S_GROUPID_2 =
"mbMessage.groupId = ? AND ";
private static final String _FINDER_COLUMN_G_S_STATUS_2 =
"mbMessage.status = ? AND mbMessage.categoryId != -1";
private FinderPath _finderPathWithPaginationFindByC_S;
private FinderPath _finderPathWithoutPaginationFindByC_S;
private FinderPath _finderPathCountByC_S;
/**
* Returns all the message-boards messages where companyId = ? and status = ?.
*
* @param companyId the company ID
* @param status the status
* @return the matching message-boards messages
*/
@Override
public List findByC_S(long companyId, int status) {
return findByC_S(
companyId, status, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the message-boards messages where companyId = ? and status = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from MBMessageModelImpl
.
*
*
* @param companyId the company ID
* @param status the status
* @param start the lower bound of the range of message-boards messages
* @param end the upper bound of the range of message-boards messages (not inclusive)
* @return the range of matching message-boards messages
*/
@Override
public List findByC_S(
long companyId, int status, int start, int end) {
return findByC_S(companyId, status, start, end, null);
}
/**
* Returns an ordered range of all the message-boards messages where companyId = ? and status = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from MBMessageModelImpl
.
*
*
* @param companyId the company ID
* @param status the status
* @param start the lower bound of the range of message-boards messages
* @param end the upper bound of the range of message-boards messages (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching message-boards messages
*/
@Override
public List findByC_S(
long companyId, int status, int start, int end,
OrderByComparator orderByComparator) {
return findByC_S(
companyId, status, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the message-boards messages where companyId = ? and status = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from MBMessageModelImpl
.
*
*
* @param companyId the company ID
* @param status the status
* @param start the lower bound of the range of message-boards messages
* @param end the upper bound of the range of message-boards messages (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching message-boards messages
*/
@Override
public List findByC_S(
long companyId, int status, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
boolean productionMode = ctPersistenceHelper.isProductionMode(
MBMessage.class);
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache && productionMode) {
finderPath = _finderPathWithoutPaginationFindByC_S;
finderArgs = new Object[] {companyId, status};
}
}
else if (useFinderCache && productionMode) {
finderPath = _finderPathWithPaginationFindByC_S;
finderArgs = new Object[] {
companyId, status, start, end, orderByComparator
};
}
List list = null;
if (useFinderCache && productionMode) {
list = (List)finderCache.getResult(
finderPath, finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (MBMessage mbMessage : list) {
if ((companyId != mbMessage.getCompanyId()) ||
(status != mbMessage.getStatus())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_MBMESSAGE_WHERE);
sb.append(_FINDER_COLUMN_C_S_COMPANYID_2);
sb.append(_FINDER_COLUMN_C_S_STATUS_2);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(MBMessageModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(companyId);
queryPos.add(status);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache && productionMode) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first message-boards message in the ordered set where companyId = ? and status = ?.
*
* @param companyId the company ID
* @param status the status
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching message-boards message
* @throws NoSuchMessageException if a matching message-boards message could not be found
*/
@Override
public MBMessage findByC_S_First(
long companyId, int status,
OrderByComparator orderByComparator)
throws NoSuchMessageException {
MBMessage mbMessage = fetchByC_S_First(
companyId, status, orderByComparator);
if (mbMessage != null) {
return mbMessage;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("companyId=");
sb.append(companyId);
sb.append(", status=");
sb.append(status);
sb.append("}");
throw new NoSuchMessageException(sb.toString());
}
/**
* Returns the first message-boards message in the ordered set where companyId = ? and status = ?.
*
* @param companyId the company ID
* @param status the status
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching message-boards message, or null
if a matching message-boards message could not be found
*/
@Override
public MBMessage fetchByC_S_First(
long companyId, int status,
OrderByComparator orderByComparator) {
List list = findByC_S(
companyId, status, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last message-boards message in the ordered set where companyId = ? and status = ?.
*
* @param companyId the company ID
* @param status the status
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching message-boards message
* @throws NoSuchMessageException if a matching message-boards message could not be found
*/
@Override
public MBMessage findByC_S_Last(
long companyId, int status,
OrderByComparator orderByComparator)
throws NoSuchMessageException {
MBMessage mbMessage = fetchByC_S_Last(
companyId, status, orderByComparator);
if (mbMessage != null) {
return mbMessage;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("companyId=");
sb.append(companyId);
sb.append(", status=");
sb.append(status);
sb.append("}");
throw new NoSuchMessageException(sb.toString());
}
/**
* Returns the last message-boards message in the ordered set where companyId = ? and status = ?.
*
* @param companyId the company ID
* @param status the status
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching message-boards message, or null
if a matching message-boards message could not be found
*/
@Override
public MBMessage fetchByC_S_Last(
long companyId, int status,
OrderByComparator orderByComparator) {
int count = countByC_S(companyId, status);
if (count == 0) {
return null;
}
List list = findByC_S(
companyId, status, count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the message-boards messages before and after the current message-boards message in the ordered set where companyId = ? and status = ?.
*
* @param messageId the primary key of the current message-boards message
* @param companyId the company ID
* @param status the status
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next message-boards message
* @throws NoSuchMessageException if a message-boards message with the primary key could not be found
*/
@Override
public MBMessage[] findByC_S_PrevAndNext(
long messageId, long companyId, int status,
OrderByComparator orderByComparator)
throws NoSuchMessageException {
MBMessage mbMessage = findByPrimaryKey(messageId);
Session session = null;
try {
session = openSession();
MBMessage[] array = new MBMessageImpl[3];
array[0] = getByC_S_PrevAndNext(
session, mbMessage, companyId, status, orderByComparator, true);
array[1] = mbMessage;
array[2] = getByC_S_PrevAndNext(
session, mbMessage, companyId, status, orderByComparator,
false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected MBMessage getByC_S_PrevAndNext(
Session session, MBMessage mbMessage, long companyId, int status,
OrderByComparator orderByComparator, boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
5 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_MBMESSAGE_WHERE);
sb.append(_FINDER_COLUMN_C_S_COMPANYID_2);
sb.append(_FINDER_COLUMN_C_S_STATUS_2);
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(MBMessageModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(companyId);
queryPos.add(status);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(mbMessage)) {
queryPos.add(orderByConditionValue);
}
}
List