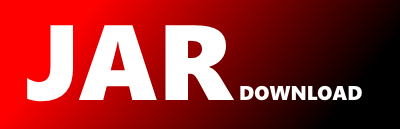
com.liferay.oauth.client.persistence.service.base.OAuthClientEntryLocalServiceBaseImpl Maven / Gradle / Ivy
Show all versions of com.liferay.oauth.client.persistence.service
/**
* SPDX-FileCopyrightText: (c) 2000 Liferay, Inc. https://liferay.com
* SPDX-License-Identifier: LGPL-2.1-or-later OR LicenseRef-Liferay-DXP-EULA-2.0.0-2023-06
*/
package com.liferay.oauth.client.persistence.service.base;
import com.liferay.oauth.client.persistence.model.OAuthClientEntry;
import com.liferay.oauth.client.persistence.service.OAuthClientEntryLocalService;
import com.liferay.oauth.client.persistence.service.persistence.OAuthClientEntryPersistence;
import com.liferay.petra.sql.dsl.query.DSLQuery;
import com.liferay.portal.aop.AopService;
import com.liferay.portal.kernel.dao.db.DB;
import com.liferay.portal.kernel.dao.db.DBManagerUtil;
import com.liferay.portal.kernel.dao.jdbc.SqlUpdate;
import com.liferay.portal.kernel.dao.jdbc.SqlUpdateFactoryUtil;
import com.liferay.portal.kernel.dao.orm.ActionableDynamicQuery;
import com.liferay.portal.kernel.dao.orm.DefaultActionableDynamicQuery;
import com.liferay.portal.kernel.dao.orm.DynamicQuery;
import com.liferay.portal.kernel.dao.orm.DynamicQueryFactoryUtil;
import com.liferay.portal.kernel.dao.orm.IndexableActionableDynamicQuery;
import com.liferay.portal.kernel.dao.orm.Projection;
import com.liferay.portal.kernel.exception.PortalException;
import com.liferay.portal.kernel.exception.SystemException;
import com.liferay.portal.kernel.log.Log;
import com.liferay.portal.kernel.log.LogFactoryUtil;
import com.liferay.portal.kernel.model.PersistedModel;
import com.liferay.portal.kernel.module.framework.service.IdentifiableOSGiService;
import com.liferay.portal.kernel.search.Indexable;
import com.liferay.portal.kernel.search.IndexableType;
import com.liferay.portal.kernel.service.BaseLocalServiceImpl;
import com.liferay.portal.kernel.service.PersistedModelLocalService;
import com.liferay.portal.kernel.service.persistence.BasePersistence;
import com.liferay.portal.kernel.transaction.Transactional;
import com.liferay.portal.kernel.util.OrderByComparator;
import com.liferay.portal.kernel.util.PortalUtil;
import java.io.Serializable;
import java.util.List;
import javax.sql.DataSource;
import org.osgi.service.component.annotations.Deactivate;
import org.osgi.service.component.annotations.Reference;
/**
* Provides the base implementation for the o auth client entry local service.
*
*
* This implementation exists only as a container for the default service methods generated by ServiceBuilder. All custom service methods should be put in {@link com.liferay.oauth.client.persistence.service.impl.OAuthClientEntryLocalServiceImpl}.
*
*
* @author Brian Wing Shun Chan
* @see com.liferay.oauth.client.persistence.service.impl.OAuthClientEntryLocalServiceImpl
* @generated
*/
public abstract class OAuthClientEntryLocalServiceBaseImpl
extends BaseLocalServiceImpl
implements AopService, IdentifiableOSGiService,
OAuthClientEntryLocalService {
/*
* NOTE FOR DEVELOPERS:
*
* Never modify or reference this class directly. Use OAuthClientEntryLocalService
via injection or a org.osgi.util.tracker.ServiceTracker
or use com.liferay.oauth.client.persistence.service.OAuthClientEntryLocalServiceUtil
.
*/
/**
* Adds the o auth client entry to the database. Also notifies the appropriate model listeners.
*
*
* Important: Inspect OAuthClientEntryLocalServiceImpl for overloaded versions of the method. If provided, use these entry points to the API, as the implementation logic may require the additional parameters defined there.
*
*
* @param oAuthClientEntry the o auth client entry
* @return the o auth client entry that was added
*/
@Indexable(type = IndexableType.REINDEX)
@Override
public OAuthClientEntry addOAuthClientEntry(
OAuthClientEntry oAuthClientEntry) {
oAuthClientEntry.setNew(true);
return oAuthClientEntryPersistence.update(oAuthClientEntry);
}
/**
* Creates a new o auth client entry with the primary key. Does not add the o auth client entry to the database.
*
* @param oAuthClientEntryId the primary key for the new o auth client entry
* @return the new o auth client entry
*/
@Override
@Transactional(enabled = false)
public OAuthClientEntry createOAuthClientEntry(long oAuthClientEntryId) {
return oAuthClientEntryPersistence.create(oAuthClientEntryId);
}
/**
* Deletes the o auth client entry with the primary key from the database. Also notifies the appropriate model listeners.
*
*
* Important: Inspect OAuthClientEntryLocalServiceImpl for overloaded versions of the method. If provided, use these entry points to the API, as the implementation logic may require the additional parameters defined there.
*
*
* @param oAuthClientEntryId the primary key of the o auth client entry
* @return the o auth client entry that was removed
* @throws PortalException if a o auth client entry with the primary key could not be found
*/
@Indexable(type = IndexableType.DELETE)
@Override
public OAuthClientEntry deleteOAuthClientEntry(long oAuthClientEntryId)
throws PortalException {
return oAuthClientEntryPersistence.remove(oAuthClientEntryId);
}
/**
* Deletes the o auth client entry from the database. Also notifies the appropriate model listeners.
*
*
* Important: Inspect OAuthClientEntryLocalServiceImpl for overloaded versions of the method. If provided, use these entry points to the API, as the implementation logic may require the additional parameters defined there.
*
*
* @param oAuthClientEntry the o auth client entry
* @return the o auth client entry that was removed
* @throws PortalException
*/
@Indexable(type = IndexableType.DELETE)
@Override
public OAuthClientEntry deleteOAuthClientEntry(
OAuthClientEntry oAuthClientEntry)
throws PortalException {
return oAuthClientEntryPersistence.remove(oAuthClientEntry);
}
@Override
public T dslQuery(DSLQuery dslQuery) {
return oAuthClientEntryPersistence.dslQuery(dslQuery);
}
@Override
public int dslQueryCount(DSLQuery dslQuery) {
Long count = dslQuery(dslQuery);
return count.intValue();
}
@Override
public DynamicQuery dynamicQuery() {
Class> clazz = getClass();
return DynamicQueryFactoryUtil.forClass(
OAuthClientEntry.class, clazz.getClassLoader());
}
/**
* Performs a dynamic query on the database and returns the matching rows.
*
* @param dynamicQuery the dynamic query
* @return the matching rows
*/
@Override
public List dynamicQuery(DynamicQuery dynamicQuery) {
return oAuthClientEntryPersistence.findWithDynamicQuery(dynamicQuery);
}
/**
* Performs a dynamic query on the database and returns a range of the matching rows.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to com.liferay.portal.kernel.dao.orm.QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from com.liferay.oauth.client.persistence.model.impl.OAuthClientEntryModelImpl
.
*
*
* @param dynamicQuery the dynamic query
* @param start the lower bound of the range of model instances
* @param end the upper bound of the range of model instances (not inclusive)
* @return the range of matching rows
*/
@Override
public List dynamicQuery(
DynamicQuery dynamicQuery, int start, int end) {
return oAuthClientEntryPersistence.findWithDynamicQuery(
dynamicQuery, start, end);
}
/**
* Performs a dynamic query on the database and returns an ordered range of the matching rows.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to com.liferay.portal.kernel.dao.orm.QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from com.liferay.oauth.client.persistence.model.impl.OAuthClientEntryModelImpl
.
*
*
* @param dynamicQuery the dynamic query
* @param start the lower bound of the range of model instances
* @param end the upper bound of the range of model instances (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching rows
*/
@Override
public List dynamicQuery(
DynamicQuery dynamicQuery, int start, int end,
OrderByComparator orderByComparator) {
return oAuthClientEntryPersistence.findWithDynamicQuery(
dynamicQuery, start, end, orderByComparator);
}
/**
* Returns the number of rows matching the dynamic query.
*
* @param dynamicQuery the dynamic query
* @return the number of rows matching the dynamic query
*/
@Override
public long dynamicQueryCount(DynamicQuery dynamicQuery) {
return oAuthClientEntryPersistence.countWithDynamicQuery(dynamicQuery);
}
/**
* Returns the number of rows matching the dynamic query.
*
* @param dynamicQuery the dynamic query
* @param projection the projection to apply to the query
* @return the number of rows matching the dynamic query
*/
@Override
public long dynamicQueryCount(
DynamicQuery dynamicQuery, Projection projection) {
return oAuthClientEntryPersistence.countWithDynamicQuery(
dynamicQuery, projection);
}
@Override
public OAuthClientEntry fetchOAuthClientEntry(long oAuthClientEntryId) {
return oAuthClientEntryPersistence.fetchByPrimaryKey(
oAuthClientEntryId);
}
/**
* Returns the o auth client entry with the primary key.
*
* @param oAuthClientEntryId the primary key of the o auth client entry
* @return the o auth client entry
* @throws PortalException if a o auth client entry with the primary key could not be found
*/
@Override
public OAuthClientEntry getOAuthClientEntry(long oAuthClientEntryId)
throws PortalException {
return oAuthClientEntryPersistence.findByPrimaryKey(oAuthClientEntryId);
}
@Override
public ActionableDynamicQuery getActionableDynamicQuery() {
ActionableDynamicQuery actionableDynamicQuery =
new DefaultActionableDynamicQuery();
actionableDynamicQuery.setBaseLocalService(
oAuthClientEntryLocalService);
actionableDynamicQuery.setClassLoader(getClassLoader());
actionableDynamicQuery.setModelClass(OAuthClientEntry.class);
actionableDynamicQuery.setPrimaryKeyPropertyName("oAuthClientEntryId");
return actionableDynamicQuery;
}
@Override
public IndexableActionableDynamicQuery
getIndexableActionableDynamicQuery() {
IndexableActionableDynamicQuery indexableActionableDynamicQuery =
new IndexableActionableDynamicQuery();
indexableActionableDynamicQuery.setBaseLocalService(
oAuthClientEntryLocalService);
indexableActionableDynamicQuery.setClassLoader(getClassLoader());
indexableActionableDynamicQuery.setModelClass(OAuthClientEntry.class);
indexableActionableDynamicQuery.setPrimaryKeyPropertyName(
"oAuthClientEntryId");
return indexableActionableDynamicQuery;
}
protected void initActionableDynamicQuery(
ActionableDynamicQuery actionableDynamicQuery) {
actionableDynamicQuery.setBaseLocalService(
oAuthClientEntryLocalService);
actionableDynamicQuery.setClassLoader(getClassLoader());
actionableDynamicQuery.setModelClass(OAuthClientEntry.class);
actionableDynamicQuery.setPrimaryKeyPropertyName("oAuthClientEntryId");
}
/**
* @throws PortalException
*/
@Override
public PersistedModel createPersistedModel(Serializable primaryKeyObj)
throws PortalException {
return oAuthClientEntryPersistence.create(
((Long)primaryKeyObj).longValue());
}
/**
* @throws PortalException
*/
@Override
public PersistedModel deletePersistedModel(PersistedModel persistedModel)
throws PortalException {
if (_log.isWarnEnabled()) {
_log.warn(
"Implement OAuthClientEntryLocalServiceImpl#deleteOAuthClientEntry(OAuthClientEntry) to avoid orphaned data");
}
return oAuthClientEntryLocalService.deleteOAuthClientEntry(
(OAuthClientEntry)persistedModel);
}
@Override
public BasePersistence getBasePersistence() {
return oAuthClientEntryPersistence;
}
/**
* @throws PortalException
*/
@Override
public PersistedModel getPersistedModel(Serializable primaryKeyObj)
throws PortalException {
return oAuthClientEntryPersistence.findByPrimaryKey(primaryKeyObj);
}
/**
* Returns a range of all the o auth client entries.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to com.liferay.portal.kernel.dao.orm.QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from com.liferay.oauth.client.persistence.model.impl.OAuthClientEntryModelImpl
.
*
*
* @param start the lower bound of the range of o auth client entries
* @param end the upper bound of the range of o auth client entries (not inclusive)
* @return the range of o auth client entries
*/
@Override
public List getOAuthClientEntries(int start, int end) {
return oAuthClientEntryPersistence.findAll(start, end);
}
/**
* Returns the number of o auth client entries.
*
* @return the number of o auth client entries
*/
@Override
public int getOAuthClientEntriesCount() {
return oAuthClientEntryPersistence.countAll();
}
/**
* Updates the o auth client entry in the database or adds it if it does not yet exist. Also notifies the appropriate model listeners.
*
*
* Important: Inspect OAuthClientEntryLocalServiceImpl for overloaded versions of the method. If provided, use these entry points to the API, as the implementation logic may require the additional parameters defined there.
*
*
* @param oAuthClientEntry the o auth client entry
* @return the o auth client entry that was updated
*/
@Indexable(type = IndexableType.REINDEX)
@Override
public OAuthClientEntry updateOAuthClientEntry(
OAuthClientEntry oAuthClientEntry) {
return oAuthClientEntryPersistence.update(oAuthClientEntry);
}
@Deactivate
protected void deactivate() {
}
@Override
public Class>[] getAopInterfaces() {
return new Class>[] {
OAuthClientEntryLocalService.class, IdentifiableOSGiService.class,
PersistedModelLocalService.class
};
}
@Override
public void setAopProxy(Object aopProxy) {
oAuthClientEntryLocalService = (OAuthClientEntryLocalService)aopProxy;
}
/**
* Returns the OSGi service identifier.
*
* @return the OSGi service identifier
*/
@Override
public String getOSGiServiceIdentifier() {
return OAuthClientEntryLocalService.class.getName();
}
protected Class> getModelClass() {
return OAuthClientEntry.class;
}
protected String getModelClassName() {
return OAuthClientEntry.class.getName();
}
/**
* Performs a SQL query.
*
* @param sql the sql query
*/
protected void runSQL(String sql) {
try {
DataSource dataSource = oAuthClientEntryPersistence.getDataSource();
DB db = DBManagerUtil.getDB();
sql = db.buildSQL(sql);
sql = PortalUtil.transformSQL(sql);
SqlUpdate sqlUpdate = SqlUpdateFactoryUtil.getSqlUpdate(
dataSource, sql);
sqlUpdate.update();
}
catch (Exception exception) {
throw new SystemException(exception);
}
}
protected OAuthClientEntryLocalService oAuthClientEntryLocalService;
@Reference
protected OAuthClientEntryPersistence oAuthClientEntryPersistence;
@Reference
protected com.liferay.counter.kernel.service.CounterLocalService
counterLocalService;
private static final Log _log = LogFactoryUtil.getLog(
OAuthClientEntryLocalServiceBaseImpl.class);
}