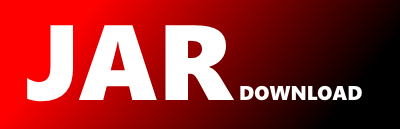
com.liferay.object.service.persistence.impl.ObjectDefinitionPersistenceImpl Maven / Gradle / Ivy
/**
* SPDX-FileCopyrightText: (c) 2000 Liferay, Inc. https://liferay.com
* SPDX-License-Identifier: LGPL-2.1-or-later OR LicenseRef-Liferay-DXP-EULA-2.0.0-2023-06
*/
package com.liferay.object.service.persistence.impl;
import com.liferay.object.exception.DuplicateObjectDefinitionExternalReferenceCodeException;
import com.liferay.object.exception.NoSuchObjectDefinitionException;
import com.liferay.object.model.ObjectDefinition;
import com.liferay.object.model.ObjectDefinitionTable;
import com.liferay.object.model.impl.ObjectDefinitionImpl;
import com.liferay.object.model.impl.ObjectDefinitionModelImpl;
import com.liferay.object.service.persistence.ObjectDefinitionPersistence;
import com.liferay.object.service.persistence.ObjectDefinitionUtil;
import com.liferay.object.service.persistence.impl.constants.ObjectPersistenceConstants;
import com.liferay.petra.string.StringBundler;
import com.liferay.portal.kernel.configuration.Configuration;
import com.liferay.portal.kernel.dao.orm.EntityCache;
import com.liferay.portal.kernel.dao.orm.FinderCache;
import com.liferay.portal.kernel.dao.orm.FinderPath;
import com.liferay.portal.kernel.dao.orm.Query;
import com.liferay.portal.kernel.dao.orm.QueryPos;
import com.liferay.portal.kernel.dao.orm.QueryUtil;
import com.liferay.portal.kernel.dao.orm.SQLQuery;
import com.liferay.portal.kernel.dao.orm.Session;
import com.liferay.portal.kernel.dao.orm.SessionFactory;
import com.liferay.portal.kernel.exception.SystemException;
import com.liferay.portal.kernel.log.Log;
import com.liferay.portal.kernel.log.LogFactoryUtil;
import com.liferay.portal.kernel.sanitizer.Sanitizer;
import com.liferay.portal.kernel.sanitizer.SanitizerException;
import com.liferay.portal.kernel.sanitizer.SanitizerUtil;
import com.liferay.portal.kernel.security.auth.CompanyThreadLocal;
import com.liferay.portal.kernel.security.auth.PrincipalThreadLocal;
import com.liferay.portal.kernel.security.permission.InlineSQLHelperUtil;
import com.liferay.portal.kernel.service.ServiceContext;
import com.liferay.portal.kernel.service.ServiceContextThreadLocal;
import com.liferay.portal.kernel.service.persistence.impl.BasePersistenceImpl;
import com.liferay.portal.kernel.util.ContentTypes;
import com.liferay.portal.kernel.util.GetterUtil;
import com.liferay.portal.kernel.util.OrderByComparator;
import com.liferay.portal.kernel.util.PropsKeys;
import com.liferay.portal.kernel.util.PropsUtil;
import com.liferay.portal.kernel.util.ProxyUtil;
import com.liferay.portal.kernel.util.SetUtil;
import com.liferay.portal.kernel.util.StringUtil;
import com.liferay.portal.kernel.util.Validator;
import com.liferay.portal.kernel.uuid.PortalUUIDUtil;
import java.io.Serializable;
import java.lang.reflect.InvocationHandler;
import java.util.Collections;
import java.util.Date;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Set;
import javax.sql.DataSource;
import org.osgi.service.component.annotations.Activate;
import org.osgi.service.component.annotations.Component;
import org.osgi.service.component.annotations.Deactivate;
import org.osgi.service.component.annotations.Reference;
/**
* The persistence implementation for the object definition service.
*
*
* Caching information and settings can be found in portal.properties
*
*
* @author Marco Leo
* @generated
*/
@Component(service = ObjectDefinitionPersistence.class)
public class ObjectDefinitionPersistenceImpl
extends BasePersistenceImpl
implements ObjectDefinitionPersistence {
/*
* NOTE FOR DEVELOPERS:
*
* Never modify or reference this class directly. Always use ObjectDefinitionUtil
to access the object definition persistence. Modify service.xml
and rerun ServiceBuilder to regenerate this class.
*/
public static final String FINDER_CLASS_NAME_ENTITY =
ObjectDefinitionImpl.class.getName();
public static final String FINDER_CLASS_NAME_LIST_WITH_PAGINATION =
FINDER_CLASS_NAME_ENTITY + ".List1";
public static final String FINDER_CLASS_NAME_LIST_WITHOUT_PAGINATION =
FINDER_CLASS_NAME_ENTITY + ".List2";
private FinderPath _finderPathWithPaginationFindAll;
private FinderPath _finderPathWithoutPaginationFindAll;
private FinderPath _finderPathCountAll;
private FinderPath _finderPathWithPaginationFindByUuid;
private FinderPath _finderPathWithoutPaginationFindByUuid;
private FinderPath _finderPathCountByUuid;
/**
* Returns all the object definitions where uuid = ?.
*
* @param uuid the uuid
* @return the matching object definitions
*/
@Override
public List findByUuid(String uuid) {
return findByUuid(uuid, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the object definitions where uuid = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectDefinitionModelImpl
.
*
*
* @param uuid the uuid
* @param start the lower bound of the range of object definitions
* @param end the upper bound of the range of object definitions (not inclusive)
* @return the range of matching object definitions
*/
@Override
public List findByUuid(String uuid, int start, int end) {
return findByUuid(uuid, start, end, null);
}
/**
* Returns an ordered range of all the object definitions where uuid = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectDefinitionModelImpl
.
*
*
* @param uuid the uuid
* @param start the lower bound of the range of object definitions
* @param end the upper bound of the range of object definitions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching object definitions
*/
@Override
public List findByUuid(
String uuid, int start, int end,
OrderByComparator orderByComparator) {
return findByUuid(uuid, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the object definitions where uuid = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectDefinitionModelImpl
.
*
*
* @param uuid the uuid
* @param start the lower bound of the range of object definitions
* @param end the upper bound of the range of object definitions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching object definitions
*/
@Override
public List findByUuid(
String uuid, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
uuid = Objects.toString(uuid, "");
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache) {
finderPath = _finderPathWithoutPaginationFindByUuid;
finderArgs = new Object[] {uuid};
}
}
else if (useFinderCache) {
finderPath = _finderPathWithPaginationFindByUuid;
finderArgs = new Object[] {uuid, start, end, orderByComparator};
}
List list = null;
if (useFinderCache) {
list = (List)finderCache.getResult(
finderPath, finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (ObjectDefinition objectDefinition : list) {
if (!uuid.equals(objectDefinition.getUuid())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
3 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(3);
}
sb.append(_SQL_SELECT_OBJECTDEFINITION_WHERE);
boolean bindUuid = false;
if (uuid.isEmpty()) {
sb.append(_FINDER_COLUMN_UUID_UUID_3);
}
else {
bindUuid = true;
sb.append(_FINDER_COLUMN_UUID_UUID_2);
}
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(ObjectDefinitionModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
if (bindUuid) {
queryPos.add(uuid);
}
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first object definition in the ordered set where uuid = ?.
*
* @param uuid the uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching object definition
* @throws NoSuchObjectDefinitionException if a matching object definition could not be found
*/
@Override
public ObjectDefinition findByUuid_First(
String uuid, OrderByComparator orderByComparator)
throws NoSuchObjectDefinitionException {
ObjectDefinition objectDefinition = fetchByUuid_First(
uuid, orderByComparator);
if (objectDefinition != null) {
return objectDefinition;
}
StringBundler sb = new StringBundler(4);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("uuid=");
sb.append(uuid);
sb.append("}");
throw new NoSuchObjectDefinitionException(sb.toString());
}
/**
* Returns the first object definition in the ordered set where uuid = ?.
*
* @param uuid the uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching object definition, or null
if a matching object definition could not be found
*/
@Override
public ObjectDefinition fetchByUuid_First(
String uuid, OrderByComparator orderByComparator) {
List list = findByUuid(uuid, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last object definition in the ordered set where uuid = ?.
*
* @param uuid the uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching object definition
* @throws NoSuchObjectDefinitionException if a matching object definition could not be found
*/
@Override
public ObjectDefinition findByUuid_Last(
String uuid, OrderByComparator orderByComparator)
throws NoSuchObjectDefinitionException {
ObjectDefinition objectDefinition = fetchByUuid_Last(
uuid, orderByComparator);
if (objectDefinition != null) {
return objectDefinition;
}
StringBundler sb = new StringBundler(4);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("uuid=");
sb.append(uuid);
sb.append("}");
throw new NoSuchObjectDefinitionException(sb.toString());
}
/**
* Returns the last object definition in the ordered set where uuid = ?.
*
* @param uuid the uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching object definition, or null
if a matching object definition could not be found
*/
@Override
public ObjectDefinition fetchByUuid_Last(
String uuid, OrderByComparator orderByComparator) {
int count = countByUuid(uuid);
if (count == 0) {
return null;
}
List list = findByUuid(
uuid, count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the object definitions before and after the current object definition in the ordered set where uuid = ?.
*
* @param objectDefinitionId the primary key of the current object definition
* @param uuid the uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next object definition
* @throws NoSuchObjectDefinitionException if a object definition with the primary key could not be found
*/
@Override
public ObjectDefinition[] findByUuid_PrevAndNext(
long objectDefinitionId, String uuid,
OrderByComparator orderByComparator)
throws NoSuchObjectDefinitionException {
uuid = Objects.toString(uuid, "");
ObjectDefinition objectDefinition = findByPrimaryKey(
objectDefinitionId);
Session session = null;
try {
session = openSession();
ObjectDefinition[] array = new ObjectDefinitionImpl[3];
array[0] = getByUuid_PrevAndNext(
session, objectDefinition, uuid, orderByComparator, true);
array[1] = objectDefinition;
array[2] = getByUuid_PrevAndNext(
session, objectDefinition, uuid, orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected ObjectDefinition getByUuid_PrevAndNext(
Session session, ObjectDefinition objectDefinition, String uuid,
OrderByComparator orderByComparator,
boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(3);
}
sb.append(_SQL_SELECT_OBJECTDEFINITION_WHERE);
boolean bindUuid = false;
if (uuid.isEmpty()) {
sb.append(_FINDER_COLUMN_UUID_UUID_3);
}
else {
bindUuid = true;
sb.append(_FINDER_COLUMN_UUID_UUID_2);
}
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(ObjectDefinitionModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
if (bindUuid) {
queryPos.add(uuid);
}
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
objectDefinition)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Returns all the object definitions that the user has permission to view where uuid = ?.
*
* @param uuid the uuid
* @return the matching object definitions that the user has permission to view
*/
@Override
public List filterFindByUuid(String uuid) {
return filterFindByUuid(
uuid, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the object definitions that the user has permission to view where uuid = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectDefinitionModelImpl
.
*
*
* @param uuid the uuid
* @param start the lower bound of the range of object definitions
* @param end the upper bound of the range of object definitions (not inclusive)
* @return the range of matching object definitions that the user has permission to view
*/
@Override
public List filterFindByUuid(
String uuid, int start, int end) {
return filterFindByUuid(uuid, start, end, null);
}
/**
* Returns an ordered range of all the object definitions that the user has permissions to view where uuid = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectDefinitionModelImpl
.
*
*
* @param uuid the uuid
* @param start the lower bound of the range of object definitions
* @param end the upper bound of the range of object definitions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching object definitions that the user has permission to view
*/
@Override
public List filterFindByUuid(
String uuid, int start, int end,
OrderByComparator orderByComparator) {
if (!InlineSQLHelperUtil.isEnabled()) {
return findByUuid(uuid, start, end, orderByComparator);
}
uuid = Objects.toString(uuid, "");
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
3 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(4);
}
if (getDB().isSupportsInlineDistinct()) {
sb.append(_FILTER_SQL_SELECT_OBJECTDEFINITION_WHERE);
}
else {
sb.append(
_FILTER_SQL_SELECT_OBJECTDEFINITION_NO_INLINE_DISTINCT_WHERE_1);
}
boolean bindUuid = false;
if (uuid.isEmpty()) {
sb.append(_FINDER_COLUMN_UUID_UUID_3_SQL);
}
else {
bindUuid = true;
sb.append(_FINDER_COLUMN_UUID_UUID_2_SQL);
}
if (!getDB().isSupportsInlineDistinct()) {
sb.append(
_FILTER_SQL_SELECT_OBJECTDEFINITION_NO_INLINE_DISTINCT_WHERE_2);
}
if (orderByComparator != null) {
if (getDB().isSupportsInlineDistinct()) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator, true);
}
else {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_TABLE, orderByComparator, true);
}
}
else {
if (getDB().isSupportsInlineDistinct()) {
sb.append(
ObjectDefinitionModelImpl.ORDER_BY_SQL_INLINE_DISTINCT);
}
else {
sb.append(ObjectDefinitionModelImpl.ORDER_BY_SQL);
}
}
String sql = InlineSQLHelperUtil.replacePermissionCheck(
sb.toString(), ObjectDefinition.class.getName(),
_FILTER_ENTITY_TABLE_FILTER_PK_COLUMN);
Session session = null;
try {
session = openSession();
SQLQuery sqlQuery = session.createSynchronizedSQLQuery(sql);
if (getDB().isSupportsInlineDistinct()) {
sqlQuery.addEntity(
_FILTER_ENTITY_ALIAS, ObjectDefinitionImpl.class);
}
else {
sqlQuery.addEntity(
_FILTER_ENTITY_TABLE, ObjectDefinitionImpl.class);
}
QueryPos queryPos = QueryPos.getInstance(sqlQuery);
if (bindUuid) {
queryPos.add(uuid);
}
return (List)QueryUtil.list(
sqlQuery, getDialect(), start, end);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
/**
* Returns the object definitions before and after the current object definition in the ordered set of object definitions that the user has permission to view where uuid = ?.
*
* @param objectDefinitionId the primary key of the current object definition
* @param uuid the uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next object definition
* @throws NoSuchObjectDefinitionException if a object definition with the primary key could not be found
*/
@Override
public ObjectDefinition[] filterFindByUuid_PrevAndNext(
long objectDefinitionId, String uuid,
OrderByComparator orderByComparator)
throws NoSuchObjectDefinitionException {
if (!InlineSQLHelperUtil.isEnabled()) {
return findByUuid_PrevAndNext(
objectDefinitionId, uuid, orderByComparator);
}
uuid = Objects.toString(uuid, "");
ObjectDefinition objectDefinition = findByPrimaryKey(
objectDefinitionId);
Session session = null;
try {
session = openSession();
ObjectDefinition[] array = new ObjectDefinitionImpl[3];
array[0] = filterGetByUuid_PrevAndNext(
session, objectDefinition, uuid, orderByComparator, true);
array[1] = objectDefinition;
array[2] = filterGetByUuid_PrevAndNext(
session, objectDefinition, uuid, orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected ObjectDefinition filterGetByUuid_PrevAndNext(
Session session, ObjectDefinition objectDefinition, String uuid,
OrderByComparator orderByComparator,
boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
5 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(4);
}
if (getDB().isSupportsInlineDistinct()) {
sb.append(_FILTER_SQL_SELECT_OBJECTDEFINITION_WHERE);
}
else {
sb.append(
_FILTER_SQL_SELECT_OBJECTDEFINITION_NO_INLINE_DISTINCT_WHERE_1);
}
boolean bindUuid = false;
if (uuid.isEmpty()) {
sb.append(_FINDER_COLUMN_UUID_UUID_3_SQL);
}
else {
bindUuid = true;
sb.append(_FINDER_COLUMN_UUID_UUID_2_SQL);
}
if (!getDB().isSupportsInlineDistinct()) {
sb.append(
_FILTER_SQL_SELECT_OBJECTDEFINITION_NO_INLINE_DISTINCT_WHERE_2);
}
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
if (getDB().isSupportsInlineDistinct()) {
sb.append(
getColumnName(
_ORDER_BY_ENTITY_ALIAS, orderByConditionFields[i],
true));
}
else {
sb.append(
getColumnName(
_ORDER_BY_ENTITY_TABLE, orderByConditionFields[i],
true));
}
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
if (getDB().isSupportsInlineDistinct()) {
sb.append(
getColumnName(
_ORDER_BY_ENTITY_ALIAS, orderByFields[i], true));
}
else {
sb.append(
getColumnName(
_ORDER_BY_ENTITY_TABLE, orderByFields[i], true));
}
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
if (getDB().isSupportsInlineDistinct()) {
sb.append(
ObjectDefinitionModelImpl.ORDER_BY_SQL_INLINE_DISTINCT);
}
else {
sb.append(ObjectDefinitionModelImpl.ORDER_BY_SQL);
}
}
String sql = InlineSQLHelperUtil.replacePermissionCheck(
sb.toString(), ObjectDefinition.class.getName(),
_FILTER_ENTITY_TABLE_FILTER_PK_COLUMN);
SQLQuery sqlQuery = session.createSynchronizedSQLQuery(sql);
sqlQuery.setFirstResult(0);
sqlQuery.setMaxResults(2);
if (getDB().isSupportsInlineDistinct()) {
sqlQuery.addEntity(
_FILTER_ENTITY_ALIAS, ObjectDefinitionImpl.class);
}
else {
sqlQuery.addEntity(
_FILTER_ENTITY_TABLE, ObjectDefinitionImpl.class);
}
QueryPos queryPos = QueryPos.getInstance(sqlQuery);
if (bindUuid) {
queryPos.add(uuid);
}
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
objectDefinition)) {
queryPos.add(orderByConditionValue);
}
}
List list = sqlQuery.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the object definitions where uuid = ? from the database.
*
* @param uuid the uuid
*/
@Override
public void removeByUuid(String uuid) {
for (ObjectDefinition objectDefinition :
findByUuid(uuid, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null)) {
remove(objectDefinition);
}
}
/**
* Returns the number of object definitions where uuid = ?.
*
* @param uuid the uuid
* @return the number of matching object definitions
*/
@Override
public int countByUuid(String uuid) {
uuid = Objects.toString(uuid, "");
FinderPath finderPath = _finderPathCountByUuid;
Object[] finderArgs = new Object[] {uuid};
Long count = (Long)finderCache.getResult(finderPath, finderArgs, this);
if (count == null) {
StringBundler sb = new StringBundler(2);
sb.append(_SQL_COUNT_OBJECTDEFINITION_WHERE);
boolean bindUuid = false;
if (uuid.isEmpty()) {
sb.append(_FINDER_COLUMN_UUID_UUID_3);
}
else {
bindUuid = true;
sb.append(_FINDER_COLUMN_UUID_UUID_2);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
if (bindUuid) {
queryPos.add(uuid);
}
count = (Long)query.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
/**
* Returns the number of object definitions that the user has permission to view where uuid = ?.
*
* @param uuid the uuid
* @return the number of matching object definitions that the user has permission to view
*/
@Override
public int filterCountByUuid(String uuid) {
if (!InlineSQLHelperUtil.isEnabled()) {
return countByUuid(uuid);
}
uuid = Objects.toString(uuid, "");
StringBundler sb = new StringBundler(2);
sb.append(_FILTER_SQL_COUNT_OBJECTDEFINITION_WHERE);
boolean bindUuid = false;
if (uuid.isEmpty()) {
sb.append(_FINDER_COLUMN_UUID_UUID_3_SQL);
}
else {
bindUuid = true;
sb.append(_FINDER_COLUMN_UUID_UUID_2_SQL);
}
String sql = InlineSQLHelperUtil.replacePermissionCheck(
sb.toString(), ObjectDefinition.class.getName(),
_FILTER_ENTITY_TABLE_FILTER_PK_COLUMN);
Session session = null;
try {
session = openSession();
SQLQuery sqlQuery = session.createSynchronizedSQLQuery(sql);
sqlQuery.addScalar(
COUNT_COLUMN_NAME, com.liferay.portal.kernel.dao.orm.Type.LONG);
QueryPos queryPos = QueryPos.getInstance(sqlQuery);
if (bindUuid) {
queryPos.add(uuid);
}
Long count = (Long)sqlQuery.uniqueResult();
return count.intValue();
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
private static final String _FINDER_COLUMN_UUID_UUID_2 =
"objectDefinition.uuid = ?";
private static final String _FINDER_COLUMN_UUID_UUID_3 =
"(objectDefinition.uuid IS NULL OR objectDefinition.uuid = '')";
private static final String _FINDER_COLUMN_UUID_UUID_2_SQL =
"objectDefinition.uuid_ = ?";
private static final String _FINDER_COLUMN_UUID_UUID_3_SQL =
"(objectDefinition.uuid_ IS NULL OR objectDefinition.uuid_ = '')";
private FinderPath _finderPathWithPaginationFindByUuid_C;
private FinderPath _finderPathWithoutPaginationFindByUuid_C;
private FinderPath _finderPathCountByUuid_C;
/**
* Returns all the object definitions where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @return the matching object definitions
*/
@Override
public List findByUuid_C(String uuid, long companyId) {
return findByUuid_C(
uuid, companyId, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the object definitions where uuid = ? and companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectDefinitionModelImpl
.
*
*
* @param uuid the uuid
* @param companyId the company ID
* @param start the lower bound of the range of object definitions
* @param end the upper bound of the range of object definitions (not inclusive)
* @return the range of matching object definitions
*/
@Override
public List findByUuid_C(
String uuid, long companyId, int start, int end) {
return findByUuid_C(uuid, companyId, start, end, null);
}
/**
* Returns an ordered range of all the object definitions where uuid = ? and companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectDefinitionModelImpl
.
*
*
* @param uuid the uuid
* @param companyId the company ID
* @param start the lower bound of the range of object definitions
* @param end the upper bound of the range of object definitions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching object definitions
*/
@Override
public List findByUuid_C(
String uuid, long companyId, int start, int end,
OrderByComparator orderByComparator) {
return findByUuid_C(
uuid, companyId, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the object definitions where uuid = ? and companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectDefinitionModelImpl
.
*
*
* @param uuid the uuid
* @param companyId the company ID
* @param start the lower bound of the range of object definitions
* @param end the upper bound of the range of object definitions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching object definitions
*/
@Override
public List findByUuid_C(
String uuid, long companyId, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
uuid = Objects.toString(uuid, "");
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache) {
finderPath = _finderPathWithoutPaginationFindByUuid_C;
finderArgs = new Object[] {uuid, companyId};
}
}
else if (useFinderCache) {
finderPath = _finderPathWithPaginationFindByUuid_C;
finderArgs = new Object[] {
uuid, companyId, start, end, orderByComparator
};
}
List list = null;
if (useFinderCache) {
list = (List)finderCache.getResult(
finderPath, finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (ObjectDefinition objectDefinition : list) {
if (!uuid.equals(objectDefinition.getUuid()) ||
(companyId != objectDefinition.getCompanyId())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_OBJECTDEFINITION_WHERE);
boolean bindUuid = false;
if (uuid.isEmpty()) {
sb.append(_FINDER_COLUMN_UUID_C_UUID_3);
}
else {
bindUuid = true;
sb.append(_FINDER_COLUMN_UUID_C_UUID_2);
}
sb.append(_FINDER_COLUMN_UUID_C_COMPANYID_2);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(ObjectDefinitionModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
if (bindUuid) {
queryPos.add(uuid);
}
queryPos.add(companyId);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first object definition in the ordered set where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching object definition
* @throws NoSuchObjectDefinitionException if a matching object definition could not be found
*/
@Override
public ObjectDefinition findByUuid_C_First(
String uuid, long companyId,
OrderByComparator orderByComparator)
throws NoSuchObjectDefinitionException {
ObjectDefinition objectDefinition = fetchByUuid_C_First(
uuid, companyId, orderByComparator);
if (objectDefinition != null) {
return objectDefinition;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("uuid=");
sb.append(uuid);
sb.append(", companyId=");
sb.append(companyId);
sb.append("}");
throw new NoSuchObjectDefinitionException(sb.toString());
}
/**
* Returns the first object definition in the ordered set where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching object definition, or null
if a matching object definition could not be found
*/
@Override
public ObjectDefinition fetchByUuid_C_First(
String uuid, long companyId,
OrderByComparator orderByComparator) {
List list = findByUuid_C(
uuid, companyId, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last object definition in the ordered set where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching object definition
* @throws NoSuchObjectDefinitionException if a matching object definition could not be found
*/
@Override
public ObjectDefinition findByUuid_C_Last(
String uuid, long companyId,
OrderByComparator orderByComparator)
throws NoSuchObjectDefinitionException {
ObjectDefinition objectDefinition = fetchByUuid_C_Last(
uuid, companyId, orderByComparator);
if (objectDefinition != null) {
return objectDefinition;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("uuid=");
sb.append(uuid);
sb.append(", companyId=");
sb.append(companyId);
sb.append("}");
throw new NoSuchObjectDefinitionException(sb.toString());
}
/**
* Returns the last object definition in the ordered set where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching object definition, or null
if a matching object definition could not be found
*/
@Override
public ObjectDefinition fetchByUuid_C_Last(
String uuid, long companyId,
OrderByComparator orderByComparator) {
int count = countByUuid_C(uuid, companyId);
if (count == 0) {
return null;
}
List list = findByUuid_C(
uuid, companyId, count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the object definitions before and after the current object definition in the ordered set where uuid = ? and companyId = ?.
*
* @param objectDefinitionId the primary key of the current object definition
* @param uuid the uuid
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next object definition
* @throws NoSuchObjectDefinitionException if a object definition with the primary key could not be found
*/
@Override
public ObjectDefinition[] findByUuid_C_PrevAndNext(
long objectDefinitionId, String uuid, long companyId,
OrderByComparator orderByComparator)
throws NoSuchObjectDefinitionException {
uuid = Objects.toString(uuid, "");
ObjectDefinition objectDefinition = findByPrimaryKey(
objectDefinitionId);
Session session = null;
try {
session = openSession();
ObjectDefinition[] array = new ObjectDefinitionImpl[3];
array[0] = getByUuid_C_PrevAndNext(
session, objectDefinition, uuid, companyId, orderByComparator,
true);
array[1] = objectDefinition;
array[2] = getByUuid_C_PrevAndNext(
session, objectDefinition, uuid, companyId, orderByComparator,
false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected ObjectDefinition getByUuid_C_PrevAndNext(
Session session, ObjectDefinition objectDefinition, String uuid,
long companyId, OrderByComparator orderByComparator,
boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
5 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_OBJECTDEFINITION_WHERE);
boolean bindUuid = false;
if (uuid.isEmpty()) {
sb.append(_FINDER_COLUMN_UUID_C_UUID_3);
}
else {
bindUuid = true;
sb.append(_FINDER_COLUMN_UUID_C_UUID_2);
}
sb.append(_FINDER_COLUMN_UUID_C_COMPANYID_2);
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(ObjectDefinitionModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
if (bindUuid) {
queryPos.add(uuid);
}
queryPos.add(companyId);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
objectDefinition)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Returns all the object definitions that the user has permission to view where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @return the matching object definitions that the user has permission to view
*/
@Override
public List filterFindByUuid_C(
String uuid, long companyId) {
return filterFindByUuid_C(
uuid, companyId, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the object definitions that the user has permission to view where uuid = ? and companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectDefinitionModelImpl
.
*
*
* @param uuid the uuid
* @param companyId the company ID
* @param start the lower bound of the range of object definitions
* @param end the upper bound of the range of object definitions (not inclusive)
* @return the range of matching object definitions that the user has permission to view
*/
@Override
public List filterFindByUuid_C(
String uuid, long companyId, int start, int end) {
return filterFindByUuid_C(uuid, companyId, start, end, null);
}
/**
* Returns an ordered range of all the object definitions that the user has permissions to view where uuid = ? and companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectDefinitionModelImpl
.
*
*
* @param uuid the uuid
* @param companyId the company ID
* @param start the lower bound of the range of object definitions
* @param end the upper bound of the range of object definitions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching object definitions that the user has permission to view
*/
@Override
public List filterFindByUuid_C(
String uuid, long companyId, int start, int end,
OrderByComparator orderByComparator) {
if (!InlineSQLHelperUtil.isEnabled(companyId, 0)) {
return findByUuid_C(uuid, companyId, start, end, orderByComparator);
}
uuid = Objects.toString(uuid, "");
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(5);
}
if (getDB().isSupportsInlineDistinct()) {
sb.append(_FILTER_SQL_SELECT_OBJECTDEFINITION_WHERE);
}
else {
sb.append(
_FILTER_SQL_SELECT_OBJECTDEFINITION_NO_INLINE_DISTINCT_WHERE_1);
}
boolean bindUuid = false;
if (uuid.isEmpty()) {
sb.append(_FINDER_COLUMN_UUID_C_UUID_3_SQL);
}
else {
bindUuid = true;
sb.append(_FINDER_COLUMN_UUID_C_UUID_2_SQL);
}
sb.append(_FINDER_COLUMN_UUID_C_COMPANYID_2);
if (!getDB().isSupportsInlineDistinct()) {
sb.append(
_FILTER_SQL_SELECT_OBJECTDEFINITION_NO_INLINE_DISTINCT_WHERE_2);
}
if (orderByComparator != null) {
if (getDB().isSupportsInlineDistinct()) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator, true);
}
else {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_TABLE, orderByComparator, true);
}
}
else {
if (getDB().isSupportsInlineDistinct()) {
sb.append(
ObjectDefinitionModelImpl.ORDER_BY_SQL_INLINE_DISTINCT);
}
else {
sb.append(ObjectDefinitionModelImpl.ORDER_BY_SQL);
}
}
String sql = InlineSQLHelperUtil.replacePermissionCheck(
sb.toString(), ObjectDefinition.class.getName(),
_FILTER_ENTITY_TABLE_FILTER_PK_COLUMN);
Session session = null;
try {
session = openSession();
SQLQuery sqlQuery = session.createSynchronizedSQLQuery(sql);
if (getDB().isSupportsInlineDistinct()) {
sqlQuery.addEntity(
_FILTER_ENTITY_ALIAS, ObjectDefinitionImpl.class);
}
else {
sqlQuery.addEntity(
_FILTER_ENTITY_TABLE, ObjectDefinitionImpl.class);
}
QueryPos queryPos = QueryPos.getInstance(sqlQuery);
if (bindUuid) {
queryPos.add(uuid);
}
queryPos.add(companyId);
return (List)QueryUtil.list(
sqlQuery, getDialect(), start, end);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
/**
* Returns the object definitions before and after the current object definition in the ordered set of object definitions that the user has permission to view where uuid = ? and companyId = ?.
*
* @param objectDefinitionId the primary key of the current object definition
* @param uuid the uuid
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next object definition
* @throws NoSuchObjectDefinitionException if a object definition with the primary key could not be found
*/
@Override
public ObjectDefinition[] filterFindByUuid_C_PrevAndNext(
long objectDefinitionId, String uuid, long companyId,
OrderByComparator orderByComparator)
throws NoSuchObjectDefinitionException {
if (!InlineSQLHelperUtil.isEnabled(companyId, 0)) {
return findByUuid_C_PrevAndNext(
objectDefinitionId, uuid, companyId, orderByComparator);
}
uuid = Objects.toString(uuid, "");
ObjectDefinition objectDefinition = findByPrimaryKey(
objectDefinitionId);
Session session = null;
try {
session = openSession();
ObjectDefinition[] array = new ObjectDefinitionImpl[3];
array[0] = filterGetByUuid_C_PrevAndNext(
session, objectDefinition, uuid, companyId, orderByComparator,
true);
array[1] = objectDefinition;
array[2] = filterGetByUuid_C_PrevAndNext(
session, objectDefinition, uuid, companyId, orderByComparator,
false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected ObjectDefinition filterGetByUuid_C_PrevAndNext(
Session session, ObjectDefinition objectDefinition, String uuid,
long companyId, OrderByComparator orderByComparator,
boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
6 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(5);
}
if (getDB().isSupportsInlineDistinct()) {
sb.append(_FILTER_SQL_SELECT_OBJECTDEFINITION_WHERE);
}
else {
sb.append(
_FILTER_SQL_SELECT_OBJECTDEFINITION_NO_INLINE_DISTINCT_WHERE_1);
}
boolean bindUuid = false;
if (uuid.isEmpty()) {
sb.append(_FINDER_COLUMN_UUID_C_UUID_3_SQL);
}
else {
bindUuid = true;
sb.append(_FINDER_COLUMN_UUID_C_UUID_2_SQL);
}
sb.append(_FINDER_COLUMN_UUID_C_COMPANYID_2);
if (!getDB().isSupportsInlineDistinct()) {
sb.append(
_FILTER_SQL_SELECT_OBJECTDEFINITION_NO_INLINE_DISTINCT_WHERE_2);
}
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
if (getDB().isSupportsInlineDistinct()) {
sb.append(
getColumnName(
_ORDER_BY_ENTITY_ALIAS, orderByConditionFields[i],
true));
}
else {
sb.append(
getColumnName(
_ORDER_BY_ENTITY_TABLE, orderByConditionFields[i],
true));
}
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
if (getDB().isSupportsInlineDistinct()) {
sb.append(
getColumnName(
_ORDER_BY_ENTITY_ALIAS, orderByFields[i], true));
}
else {
sb.append(
getColumnName(
_ORDER_BY_ENTITY_TABLE, orderByFields[i], true));
}
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
if (getDB().isSupportsInlineDistinct()) {
sb.append(
ObjectDefinitionModelImpl.ORDER_BY_SQL_INLINE_DISTINCT);
}
else {
sb.append(ObjectDefinitionModelImpl.ORDER_BY_SQL);
}
}
String sql = InlineSQLHelperUtil.replacePermissionCheck(
sb.toString(), ObjectDefinition.class.getName(),
_FILTER_ENTITY_TABLE_FILTER_PK_COLUMN);
SQLQuery sqlQuery = session.createSynchronizedSQLQuery(sql);
sqlQuery.setFirstResult(0);
sqlQuery.setMaxResults(2);
if (getDB().isSupportsInlineDistinct()) {
sqlQuery.addEntity(
_FILTER_ENTITY_ALIAS, ObjectDefinitionImpl.class);
}
else {
sqlQuery.addEntity(
_FILTER_ENTITY_TABLE, ObjectDefinitionImpl.class);
}
QueryPos queryPos = QueryPos.getInstance(sqlQuery);
if (bindUuid) {
queryPos.add(uuid);
}
queryPos.add(companyId);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
objectDefinition)) {
queryPos.add(orderByConditionValue);
}
}
List list = sqlQuery.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the object definitions where uuid = ? and companyId = ? from the database.
*
* @param uuid the uuid
* @param companyId the company ID
*/
@Override
public void removeByUuid_C(String uuid, long companyId) {
for (ObjectDefinition objectDefinition :
findByUuid_C(
uuid, companyId, QueryUtil.ALL_POS, QueryUtil.ALL_POS,
null)) {
remove(objectDefinition);
}
}
/**
* Returns the number of object definitions where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @return the number of matching object definitions
*/
@Override
public int countByUuid_C(String uuid, long companyId) {
uuid = Objects.toString(uuid, "");
FinderPath finderPath = _finderPathCountByUuid_C;
Object[] finderArgs = new Object[] {uuid, companyId};
Long count = (Long)finderCache.getResult(finderPath, finderArgs, this);
if (count == null) {
StringBundler sb = new StringBundler(3);
sb.append(_SQL_COUNT_OBJECTDEFINITION_WHERE);
boolean bindUuid = false;
if (uuid.isEmpty()) {
sb.append(_FINDER_COLUMN_UUID_C_UUID_3);
}
else {
bindUuid = true;
sb.append(_FINDER_COLUMN_UUID_C_UUID_2);
}
sb.append(_FINDER_COLUMN_UUID_C_COMPANYID_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
if (bindUuid) {
queryPos.add(uuid);
}
queryPos.add(companyId);
count = (Long)query.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
/**
* Returns the number of object definitions that the user has permission to view where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @return the number of matching object definitions that the user has permission to view
*/
@Override
public int filterCountByUuid_C(String uuid, long companyId) {
if (!InlineSQLHelperUtil.isEnabled(companyId, 0)) {
return countByUuid_C(uuid, companyId);
}
uuid = Objects.toString(uuid, "");
StringBundler sb = new StringBundler(3);
sb.append(_FILTER_SQL_COUNT_OBJECTDEFINITION_WHERE);
boolean bindUuid = false;
if (uuid.isEmpty()) {
sb.append(_FINDER_COLUMN_UUID_C_UUID_3_SQL);
}
else {
bindUuid = true;
sb.append(_FINDER_COLUMN_UUID_C_UUID_2_SQL);
}
sb.append(_FINDER_COLUMN_UUID_C_COMPANYID_2);
String sql = InlineSQLHelperUtil.replacePermissionCheck(
sb.toString(), ObjectDefinition.class.getName(),
_FILTER_ENTITY_TABLE_FILTER_PK_COLUMN);
Session session = null;
try {
session = openSession();
SQLQuery sqlQuery = session.createSynchronizedSQLQuery(sql);
sqlQuery.addScalar(
COUNT_COLUMN_NAME, com.liferay.portal.kernel.dao.orm.Type.LONG);
QueryPos queryPos = QueryPos.getInstance(sqlQuery);
if (bindUuid) {
queryPos.add(uuid);
}
queryPos.add(companyId);
Long count = (Long)sqlQuery.uniqueResult();
return count.intValue();
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
private static final String _FINDER_COLUMN_UUID_C_UUID_2 =
"objectDefinition.uuid = ? AND ";
private static final String _FINDER_COLUMN_UUID_C_UUID_3 =
"(objectDefinition.uuid IS NULL OR objectDefinition.uuid = '') AND ";
private static final String _FINDER_COLUMN_UUID_C_UUID_2_SQL =
"objectDefinition.uuid_ = ? AND ";
private static final String _FINDER_COLUMN_UUID_C_UUID_3_SQL =
"(objectDefinition.uuid_ IS NULL OR objectDefinition.uuid_ = '') AND ";
private static final String _FINDER_COLUMN_UUID_C_COMPANYID_2 =
"objectDefinition.companyId = ?";
private FinderPath _finderPathWithPaginationFindByCompanyId;
private FinderPath _finderPathWithoutPaginationFindByCompanyId;
private FinderPath _finderPathCountByCompanyId;
/**
* Returns all the object definitions where companyId = ?.
*
* @param companyId the company ID
* @return the matching object definitions
*/
@Override
public List findByCompanyId(long companyId) {
return findByCompanyId(
companyId, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the object definitions where companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectDefinitionModelImpl
.
*
*
* @param companyId the company ID
* @param start the lower bound of the range of object definitions
* @param end the upper bound of the range of object definitions (not inclusive)
* @return the range of matching object definitions
*/
@Override
public List findByCompanyId(
long companyId, int start, int end) {
return findByCompanyId(companyId, start, end, null);
}
/**
* Returns an ordered range of all the object definitions where companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectDefinitionModelImpl
.
*
*
* @param companyId the company ID
* @param start the lower bound of the range of object definitions
* @param end the upper bound of the range of object definitions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching object definitions
*/
@Override
public List findByCompanyId(
long companyId, int start, int end,
OrderByComparator orderByComparator) {
return findByCompanyId(companyId, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the object definitions where companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectDefinitionModelImpl
.
*
*
* @param companyId the company ID
* @param start the lower bound of the range of object definitions
* @param end the upper bound of the range of object definitions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching object definitions
*/
@Override
public List findByCompanyId(
long companyId, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache) {
finderPath = _finderPathWithoutPaginationFindByCompanyId;
finderArgs = new Object[] {companyId};
}
}
else if (useFinderCache) {
finderPath = _finderPathWithPaginationFindByCompanyId;
finderArgs = new Object[] {
companyId, start, end, orderByComparator
};
}
List list = null;
if (useFinderCache) {
list = (List)finderCache.getResult(
finderPath, finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (ObjectDefinition objectDefinition : list) {
if (companyId != objectDefinition.getCompanyId()) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
3 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(3);
}
sb.append(_SQL_SELECT_OBJECTDEFINITION_WHERE);
sb.append(_FINDER_COLUMN_COMPANYID_COMPANYID_2);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(ObjectDefinitionModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(companyId);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first object definition in the ordered set where companyId = ?.
*
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching object definition
* @throws NoSuchObjectDefinitionException if a matching object definition could not be found
*/
@Override
public ObjectDefinition findByCompanyId_First(
long companyId,
OrderByComparator orderByComparator)
throws NoSuchObjectDefinitionException {
ObjectDefinition objectDefinition = fetchByCompanyId_First(
companyId, orderByComparator);
if (objectDefinition != null) {
return objectDefinition;
}
StringBundler sb = new StringBundler(4);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("companyId=");
sb.append(companyId);
sb.append("}");
throw new NoSuchObjectDefinitionException(sb.toString());
}
/**
* Returns the first object definition in the ordered set where companyId = ?.
*
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching object definition, or null
if a matching object definition could not be found
*/
@Override
public ObjectDefinition fetchByCompanyId_First(
long companyId, OrderByComparator orderByComparator) {
List list = findByCompanyId(
companyId, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last object definition in the ordered set where companyId = ?.
*
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching object definition
* @throws NoSuchObjectDefinitionException if a matching object definition could not be found
*/
@Override
public ObjectDefinition findByCompanyId_Last(
long companyId,
OrderByComparator orderByComparator)
throws NoSuchObjectDefinitionException {
ObjectDefinition objectDefinition = fetchByCompanyId_Last(
companyId, orderByComparator);
if (objectDefinition != null) {
return objectDefinition;
}
StringBundler sb = new StringBundler(4);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("companyId=");
sb.append(companyId);
sb.append("}");
throw new NoSuchObjectDefinitionException(sb.toString());
}
/**
* Returns the last object definition in the ordered set where companyId = ?.
*
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching object definition, or null
if a matching object definition could not be found
*/
@Override
public ObjectDefinition fetchByCompanyId_Last(
long companyId, OrderByComparator orderByComparator) {
int count = countByCompanyId(companyId);
if (count == 0) {
return null;
}
List list = findByCompanyId(
companyId, count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the object definitions before and after the current object definition in the ordered set where companyId = ?.
*
* @param objectDefinitionId the primary key of the current object definition
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next object definition
* @throws NoSuchObjectDefinitionException if a object definition with the primary key could not be found
*/
@Override
public ObjectDefinition[] findByCompanyId_PrevAndNext(
long objectDefinitionId, long companyId,
OrderByComparator orderByComparator)
throws NoSuchObjectDefinitionException {
ObjectDefinition objectDefinition = findByPrimaryKey(
objectDefinitionId);
Session session = null;
try {
session = openSession();
ObjectDefinition[] array = new ObjectDefinitionImpl[3];
array[0] = getByCompanyId_PrevAndNext(
session, objectDefinition, companyId, orderByComparator, true);
array[1] = objectDefinition;
array[2] = getByCompanyId_PrevAndNext(
session, objectDefinition, companyId, orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected ObjectDefinition getByCompanyId_PrevAndNext(
Session session, ObjectDefinition objectDefinition, long companyId,
OrderByComparator orderByComparator,
boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(3);
}
sb.append(_SQL_SELECT_OBJECTDEFINITION_WHERE);
sb.append(_FINDER_COLUMN_COMPANYID_COMPANYID_2);
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(ObjectDefinitionModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(companyId);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
objectDefinition)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Returns all the object definitions that the user has permission to view where companyId = ?.
*
* @param companyId the company ID
* @return the matching object definitions that the user has permission to view
*/
@Override
public List filterFindByCompanyId(long companyId) {
return filterFindByCompanyId(
companyId, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the object definitions that the user has permission to view where companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectDefinitionModelImpl
.
*
*
* @param companyId the company ID
* @param start the lower bound of the range of object definitions
* @param end the upper bound of the range of object definitions (not inclusive)
* @return the range of matching object definitions that the user has permission to view
*/
@Override
public List filterFindByCompanyId(
long companyId, int start, int end) {
return filterFindByCompanyId(companyId, start, end, null);
}
/**
* Returns an ordered range of all the object definitions that the user has permissions to view where companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectDefinitionModelImpl
.
*
*
* @param companyId the company ID
* @param start the lower bound of the range of object definitions
* @param end the upper bound of the range of object definitions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching object definitions that the user has permission to view
*/
@Override
public List filterFindByCompanyId(
long companyId, int start, int end,
OrderByComparator orderByComparator) {
if (!InlineSQLHelperUtil.isEnabled(companyId, 0)) {
return findByCompanyId(companyId, start, end, orderByComparator);
}
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
3 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(4);
}
if (getDB().isSupportsInlineDistinct()) {
sb.append(_FILTER_SQL_SELECT_OBJECTDEFINITION_WHERE);
}
else {
sb.append(
_FILTER_SQL_SELECT_OBJECTDEFINITION_NO_INLINE_DISTINCT_WHERE_1);
}
sb.append(_FINDER_COLUMN_COMPANYID_COMPANYID_2);
if (!getDB().isSupportsInlineDistinct()) {
sb.append(
_FILTER_SQL_SELECT_OBJECTDEFINITION_NO_INLINE_DISTINCT_WHERE_2);
}
if (orderByComparator != null) {
if (getDB().isSupportsInlineDistinct()) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator, true);
}
else {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_TABLE, orderByComparator, true);
}
}
else {
if (getDB().isSupportsInlineDistinct()) {
sb.append(
ObjectDefinitionModelImpl.ORDER_BY_SQL_INLINE_DISTINCT);
}
else {
sb.append(ObjectDefinitionModelImpl.ORDER_BY_SQL);
}
}
String sql = InlineSQLHelperUtil.replacePermissionCheck(
sb.toString(), ObjectDefinition.class.getName(),
_FILTER_ENTITY_TABLE_FILTER_PK_COLUMN);
Session session = null;
try {
session = openSession();
SQLQuery sqlQuery = session.createSynchronizedSQLQuery(sql);
if (getDB().isSupportsInlineDistinct()) {
sqlQuery.addEntity(
_FILTER_ENTITY_ALIAS, ObjectDefinitionImpl.class);
}
else {
sqlQuery.addEntity(
_FILTER_ENTITY_TABLE, ObjectDefinitionImpl.class);
}
QueryPos queryPos = QueryPos.getInstance(sqlQuery);
queryPos.add(companyId);
return (List)QueryUtil.list(
sqlQuery, getDialect(), start, end);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
/**
* Returns the object definitions before and after the current object definition in the ordered set of object definitions that the user has permission to view where companyId = ?.
*
* @param objectDefinitionId the primary key of the current object definition
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next object definition
* @throws NoSuchObjectDefinitionException if a object definition with the primary key could not be found
*/
@Override
public ObjectDefinition[] filterFindByCompanyId_PrevAndNext(
long objectDefinitionId, long companyId,
OrderByComparator orderByComparator)
throws NoSuchObjectDefinitionException {
if (!InlineSQLHelperUtil.isEnabled(companyId, 0)) {
return findByCompanyId_PrevAndNext(
objectDefinitionId, companyId, orderByComparator);
}
ObjectDefinition objectDefinition = findByPrimaryKey(
objectDefinitionId);
Session session = null;
try {
session = openSession();
ObjectDefinition[] array = new ObjectDefinitionImpl[3];
array[0] = filterGetByCompanyId_PrevAndNext(
session, objectDefinition, companyId, orderByComparator, true);
array[1] = objectDefinition;
array[2] = filterGetByCompanyId_PrevAndNext(
session, objectDefinition, companyId, orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected ObjectDefinition filterGetByCompanyId_PrevAndNext(
Session session, ObjectDefinition objectDefinition, long companyId,
OrderByComparator orderByComparator,
boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
5 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(4);
}
if (getDB().isSupportsInlineDistinct()) {
sb.append(_FILTER_SQL_SELECT_OBJECTDEFINITION_WHERE);
}
else {
sb.append(
_FILTER_SQL_SELECT_OBJECTDEFINITION_NO_INLINE_DISTINCT_WHERE_1);
}
sb.append(_FINDER_COLUMN_COMPANYID_COMPANYID_2);
if (!getDB().isSupportsInlineDistinct()) {
sb.append(
_FILTER_SQL_SELECT_OBJECTDEFINITION_NO_INLINE_DISTINCT_WHERE_2);
}
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
if (getDB().isSupportsInlineDistinct()) {
sb.append(
getColumnName(
_ORDER_BY_ENTITY_ALIAS, orderByConditionFields[i],
true));
}
else {
sb.append(
getColumnName(
_ORDER_BY_ENTITY_TABLE, orderByConditionFields[i],
true));
}
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
if (getDB().isSupportsInlineDistinct()) {
sb.append(
getColumnName(
_ORDER_BY_ENTITY_ALIAS, orderByFields[i], true));
}
else {
sb.append(
getColumnName(
_ORDER_BY_ENTITY_TABLE, orderByFields[i], true));
}
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
if (getDB().isSupportsInlineDistinct()) {
sb.append(
ObjectDefinitionModelImpl.ORDER_BY_SQL_INLINE_DISTINCT);
}
else {
sb.append(ObjectDefinitionModelImpl.ORDER_BY_SQL);
}
}
String sql = InlineSQLHelperUtil.replacePermissionCheck(
sb.toString(), ObjectDefinition.class.getName(),
_FILTER_ENTITY_TABLE_FILTER_PK_COLUMN);
SQLQuery sqlQuery = session.createSynchronizedSQLQuery(sql);
sqlQuery.setFirstResult(0);
sqlQuery.setMaxResults(2);
if (getDB().isSupportsInlineDistinct()) {
sqlQuery.addEntity(
_FILTER_ENTITY_ALIAS, ObjectDefinitionImpl.class);
}
else {
sqlQuery.addEntity(
_FILTER_ENTITY_TABLE, ObjectDefinitionImpl.class);
}
QueryPos queryPos = QueryPos.getInstance(sqlQuery);
queryPos.add(companyId);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
objectDefinition)) {
queryPos.add(orderByConditionValue);
}
}
List list = sqlQuery.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the object definitions where companyId = ? from the database.
*
* @param companyId the company ID
*/
@Override
public void removeByCompanyId(long companyId) {
for (ObjectDefinition objectDefinition :
findByCompanyId(
companyId, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null)) {
remove(objectDefinition);
}
}
/**
* Returns the number of object definitions where companyId = ?.
*
* @param companyId the company ID
* @return the number of matching object definitions
*/
@Override
public int countByCompanyId(long companyId) {
FinderPath finderPath = _finderPathCountByCompanyId;
Object[] finderArgs = new Object[] {companyId};
Long count = (Long)finderCache.getResult(finderPath, finderArgs, this);
if (count == null) {
StringBundler sb = new StringBundler(2);
sb.append(_SQL_COUNT_OBJECTDEFINITION_WHERE);
sb.append(_FINDER_COLUMN_COMPANYID_COMPANYID_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(companyId);
count = (Long)query.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
/**
* Returns the number of object definitions that the user has permission to view where companyId = ?.
*
* @param companyId the company ID
* @return the number of matching object definitions that the user has permission to view
*/
@Override
public int filterCountByCompanyId(long companyId) {
if (!InlineSQLHelperUtil.isEnabled(companyId, 0)) {
return countByCompanyId(companyId);
}
StringBundler sb = new StringBundler(2);
sb.append(_FILTER_SQL_COUNT_OBJECTDEFINITION_WHERE);
sb.append(_FINDER_COLUMN_COMPANYID_COMPANYID_2);
String sql = InlineSQLHelperUtil.replacePermissionCheck(
sb.toString(), ObjectDefinition.class.getName(),
_FILTER_ENTITY_TABLE_FILTER_PK_COLUMN);
Session session = null;
try {
session = openSession();
SQLQuery sqlQuery = session.createSynchronizedSQLQuery(sql);
sqlQuery.addScalar(
COUNT_COLUMN_NAME, com.liferay.portal.kernel.dao.orm.Type.LONG);
QueryPos queryPos = QueryPos.getInstance(sqlQuery);
queryPos.add(companyId);
Long count = (Long)sqlQuery.uniqueResult();
return count.intValue();
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
private static final String _FINDER_COLUMN_COMPANYID_COMPANYID_2 =
"objectDefinition.companyId = ?";
private FinderPath _finderPathWithPaginationFindByObjectFolderId;
private FinderPath _finderPathWithoutPaginationFindByObjectFolderId;
private FinderPath _finderPathCountByObjectFolderId;
/**
* Returns all the object definitions where objectFolderId = ?.
*
* @param objectFolderId the object folder ID
* @return the matching object definitions
*/
@Override
public List findByObjectFolderId(long objectFolderId) {
return findByObjectFolderId(
objectFolderId, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the object definitions where objectFolderId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectDefinitionModelImpl
.
*
*
* @param objectFolderId the object folder ID
* @param start the lower bound of the range of object definitions
* @param end the upper bound of the range of object definitions (not inclusive)
* @return the range of matching object definitions
*/
@Override
public List findByObjectFolderId(
long objectFolderId, int start, int end) {
return findByObjectFolderId(objectFolderId, start, end, null);
}
/**
* Returns an ordered range of all the object definitions where objectFolderId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectDefinitionModelImpl
.
*
*
* @param objectFolderId the object folder ID
* @param start the lower bound of the range of object definitions
* @param end the upper bound of the range of object definitions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching object definitions
*/
@Override
public List findByObjectFolderId(
long objectFolderId, int start, int end,
OrderByComparator orderByComparator) {
return findByObjectFolderId(
objectFolderId, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the object definitions where objectFolderId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectDefinitionModelImpl
.
*
*
* @param objectFolderId the object folder ID
* @param start the lower bound of the range of object definitions
* @param end the upper bound of the range of object definitions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching object definitions
*/
@Override
public List findByObjectFolderId(
long objectFolderId, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache) {
finderPath = _finderPathWithoutPaginationFindByObjectFolderId;
finderArgs = new Object[] {objectFolderId};
}
}
else if (useFinderCache) {
finderPath = _finderPathWithPaginationFindByObjectFolderId;
finderArgs = new Object[] {
objectFolderId, start, end, orderByComparator
};
}
List list = null;
if (useFinderCache) {
list = (List)finderCache.getResult(
finderPath, finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (ObjectDefinition objectDefinition : list) {
if (objectFolderId !=
objectDefinition.getObjectFolderId()) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
3 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(3);
}
sb.append(_SQL_SELECT_OBJECTDEFINITION_WHERE);
sb.append(_FINDER_COLUMN_OBJECTFOLDERID_OBJECTFOLDERID_2);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(ObjectDefinitionModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(objectFolderId);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first object definition in the ordered set where objectFolderId = ?.
*
* @param objectFolderId the object folder ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching object definition
* @throws NoSuchObjectDefinitionException if a matching object definition could not be found
*/
@Override
public ObjectDefinition findByObjectFolderId_First(
long objectFolderId,
OrderByComparator orderByComparator)
throws NoSuchObjectDefinitionException {
ObjectDefinition objectDefinition = fetchByObjectFolderId_First(
objectFolderId, orderByComparator);
if (objectDefinition != null) {
return objectDefinition;
}
StringBundler sb = new StringBundler(4);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("objectFolderId=");
sb.append(objectFolderId);
sb.append("}");
throw new NoSuchObjectDefinitionException(sb.toString());
}
/**
* Returns the first object definition in the ordered set where objectFolderId = ?.
*
* @param objectFolderId the object folder ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching object definition, or null
if a matching object definition could not be found
*/
@Override
public ObjectDefinition fetchByObjectFolderId_First(
long objectFolderId,
OrderByComparator orderByComparator) {
List list = findByObjectFolderId(
objectFolderId, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last object definition in the ordered set where objectFolderId = ?.
*
* @param objectFolderId the object folder ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching object definition
* @throws NoSuchObjectDefinitionException if a matching object definition could not be found
*/
@Override
public ObjectDefinition findByObjectFolderId_Last(
long objectFolderId,
OrderByComparator orderByComparator)
throws NoSuchObjectDefinitionException {
ObjectDefinition objectDefinition = fetchByObjectFolderId_Last(
objectFolderId, orderByComparator);
if (objectDefinition != null) {
return objectDefinition;
}
StringBundler sb = new StringBundler(4);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("objectFolderId=");
sb.append(objectFolderId);
sb.append("}");
throw new NoSuchObjectDefinitionException(sb.toString());
}
/**
* Returns the last object definition in the ordered set where objectFolderId = ?.
*
* @param objectFolderId the object folder ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching object definition, or null
if a matching object definition could not be found
*/
@Override
public ObjectDefinition fetchByObjectFolderId_Last(
long objectFolderId,
OrderByComparator orderByComparator) {
int count = countByObjectFolderId(objectFolderId);
if (count == 0) {
return null;
}
List list = findByObjectFolderId(
objectFolderId, count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the object definitions before and after the current object definition in the ordered set where objectFolderId = ?.
*
* @param objectDefinitionId the primary key of the current object definition
* @param objectFolderId the object folder ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next object definition
* @throws NoSuchObjectDefinitionException if a object definition with the primary key could not be found
*/
@Override
public ObjectDefinition[] findByObjectFolderId_PrevAndNext(
long objectDefinitionId, long objectFolderId,
OrderByComparator orderByComparator)
throws NoSuchObjectDefinitionException {
ObjectDefinition objectDefinition = findByPrimaryKey(
objectDefinitionId);
Session session = null;
try {
session = openSession();
ObjectDefinition[] array = new ObjectDefinitionImpl[3];
array[0] = getByObjectFolderId_PrevAndNext(
session, objectDefinition, objectFolderId, orderByComparator,
true);
array[1] = objectDefinition;
array[2] = getByObjectFolderId_PrevAndNext(
session, objectDefinition, objectFolderId, orderByComparator,
false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected ObjectDefinition getByObjectFolderId_PrevAndNext(
Session session, ObjectDefinition objectDefinition, long objectFolderId,
OrderByComparator orderByComparator,
boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(3);
}
sb.append(_SQL_SELECT_OBJECTDEFINITION_WHERE);
sb.append(_FINDER_COLUMN_OBJECTFOLDERID_OBJECTFOLDERID_2);
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(ObjectDefinitionModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(objectFolderId);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
objectDefinition)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Returns all the object definitions that the user has permission to view where objectFolderId = ?.
*
* @param objectFolderId the object folder ID
* @return the matching object definitions that the user has permission to view
*/
@Override
public List filterFindByObjectFolderId(
long objectFolderId) {
return filterFindByObjectFolderId(
objectFolderId, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the object definitions that the user has permission to view where objectFolderId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectDefinitionModelImpl
.
*
*
* @param objectFolderId the object folder ID
* @param start the lower bound of the range of object definitions
* @param end the upper bound of the range of object definitions (not inclusive)
* @return the range of matching object definitions that the user has permission to view
*/
@Override
public List filterFindByObjectFolderId(
long objectFolderId, int start, int end) {
return filterFindByObjectFolderId(objectFolderId, start, end, null);
}
/**
* Returns an ordered range of all the object definitions that the user has permissions to view where objectFolderId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectDefinitionModelImpl
.
*
*
* @param objectFolderId the object folder ID
* @param start the lower bound of the range of object definitions
* @param end the upper bound of the range of object definitions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching object definitions that the user has permission to view
*/
@Override
public List filterFindByObjectFolderId(
long objectFolderId, int start, int end,
OrderByComparator orderByComparator) {
if (!InlineSQLHelperUtil.isEnabled()) {
return findByObjectFolderId(
objectFolderId, start, end, orderByComparator);
}
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
3 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(4);
}
if (getDB().isSupportsInlineDistinct()) {
sb.append(_FILTER_SQL_SELECT_OBJECTDEFINITION_WHERE);
}
else {
sb.append(
_FILTER_SQL_SELECT_OBJECTDEFINITION_NO_INLINE_DISTINCT_WHERE_1);
}
sb.append(_FINDER_COLUMN_OBJECTFOLDERID_OBJECTFOLDERID_2);
if (!getDB().isSupportsInlineDistinct()) {
sb.append(
_FILTER_SQL_SELECT_OBJECTDEFINITION_NO_INLINE_DISTINCT_WHERE_2);
}
if (orderByComparator != null) {
if (getDB().isSupportsInlineDistinct()) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator, true);
}
else {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_TABLE, orderByComparator, true);
}
}
else {
if (getDB().isSupportsInlineDistinct()) {
sb.append(
ObjectDefinitionModelImpl.ORDER_BY_SQL_INLINE_DISTINCT);
}
else {
sb.append(ObjectDefinitionModelImpl.ORDER_BY_SQL);
}
}
String sql = InlineSQLHelperUtil.replacePermissionCheck(
sb.toString(), ObjectDefinition.class.getName(),
_FILTER_ENTITY_TABLE_FILTER_PK_COLUMN);
Session session = null;
try {
session = openSession();
SQLQuery sqlQuery = session.createSynchronizedSQLQuery(sql);
if (getDB().isSupportsInlineDistinct()) {
sqlQuery.addEntity(
_FILTER_ENTITY_ALIAS, ObjectDefinitionImpl.class);
}
else {
sqlQuery.addEntity(
_FILTER_ENTITY_TABLE, ObjectDefinitionImpl.class);
}
QueryPos queryPos = QueryPos.getInstance(sqlQuery);
queryPos.add(objectFolderId);
return (List)QueryUtil.list(
sqlQuery, getDialect(), start, end);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
/**
* Returns the object definitions before and after the current object definition in the ordered set of object definitions that the user has permission to view where objectFolderId = ?.
*
* @param objectDefinitionId the primary key of the current object definition
* @param objectFolderId the object folder ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next object definition
* @throws NoSuchObjectDefinitionException if a object definition with the primary key could not be found
*/
@Override
public ObjectDefinition[] filterFindByObjectFolderId_PrevAndNext(
long objectDefinitionId, long objectFolderId,
OrderByComparator orderByComparator)
throws NoSuchObjectDefinitionException {
if (!InlineSQLHelperUtil.isEnabled()) {
return findByObjectFolderId_PrevAndNext(
objectDefinitionId, objectFolderId, orderByComparator);
}
ObjectDefinition objectDefinition = findByPrimaryKey(
objectDefinitionId);
Session session = null;
try {
session = openSession();
ObjectDefinition[] array = new ObjectDefinitionImpl[3];
array[0] = filterGetByObjectFolderId_PrevAndNext(
session, objectDefinition, objectFolderId, orderByComparator,
true);
array[1] = objectDefinition;
array[2] = filterGetByObjectFolderId_PrevAndNext(
session, objectDefinition, objectFolderId, orderByComparator,
false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected ObjectDefinition filterGetByObjectFolderId_PrevAndNext(
Session session, ObjectDefinition objectDefinition, long objectFolderId,
OrderByComparator orderByComparator,
boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
5 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(4);
}
if (getDB().isSupportsInlineDistinct()) {
sb.append(_FILTER_SQL_SELECT_OBJECTDEFINITION_WHERE);
}
else {
sb.append(
_FILTER_SQL_SELECT_OBJECTDEFINITION_NO_INLINE_DISTINCT_WHERE_1);
}
sb.append(_FINDER_COLUMN_OBJECTFOLDERID_OBJECTFOLDERID_2);
if (!getDB().isSupportsInlineDistinct()) {
sb.append(
_FILTER_SQL_SELECT_OBJECTDEFINITION_NO_INLINE_DISTINCT_WHERE_2);
}
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
if (getDB().isSupportsInlineDistinct()) {
sb.append(
getColumnName(
_ORDER_BY_ENTITY_ALIAS, orderByConditionFields[i],
true));
}
else {
sb.append(
getColumnName(
_ORDER_BY_ENTITY_TABLE, orderByConditionFields[i],
true));
}
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
if (getDB().isSupportsInlineDistinct()) {
sb.append(
getColumnName(
_ORDER_BY_ENTITY_ALIAS, orderByFields[i], true));
}
else {
sb.append(
getColumnName(
_ORDER_BY_ENTITY_TABLE, orderByFields[i], true));
}
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
if (getDB().isSupportsInlineDistinct()) {
sb.append(
ObjectDefinitionModelImpl.ORDER_BY_SQL_INLINE_DISTINCT);
}
else {
sb.append(ObjectDefinitionModelImpl.ORDER_BY_SQL);
}
}
String sql = InlineSQLHelperUtil.replacePermissionCheck(
sb.toString(), ObjectDefinition.class.getName(),
_FILTER_ENTITY_TABLE_FILTER_PK_COLUMN);
SQLQuery sqlQuery = session.createSynchronizedSQLQuery(sql);
sqlQuery.setFirstResult(0);
sqlQuery.setMaxResults(2);
if (getDB().isSupportsInlineDistinct()) {
sqlQuery.addEntity(
_FILTER_ENTITY_ALIAS, ObjectDefinitionImpl.class);
}
else {
sqlQuery.addEntity(
_FILTER_ENTITY_TABLE, ObjectDefinitionImpl.class);
}
QueryPos queryPos = QueryPos.getInstance(sqlQuery);
queryPos.add(objectFolderId);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
objectDefinition)) {
queryPos.add(orderByConditionValue);
}
}
List list = sqlQuery.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the object definitions where objectFolderId = ? from the database.
*
* @param objectFolderId the object folder ID
*/
@Override
public void removeByObjectFolderId(long objectFolderId) {
for (ObjectDefinition objectDefinition :
findByObjectFolderId(
objectFolderId, QueryUtil.ALL_POS, QueryUtil.ALL_POS,
null)) {
remove(objectDefinition);
}
}
/**
* Returns the number of object definitions where objectFolderId = ?.
*
* @param objectFolderId the object folder ID
* @return the number of matching object definitions
*/
@Override
public int countByObjectFolderId(long objectFolderId) {
FinderPath finderPath = _finderPathCountByObjectFolderId;
Object[] finderArgs = new Object[] {objectFolderId};
Long count = (Long)finderCache.getResult(finderPath, finderArgs, this);
if (count == null) {
StringBundler sb = new StringBundler(2);
sb.append(_SQL_COUNT_OBJECTDEFINITION_WHERE);
sb.append(_FINDER_COLUMN_OBJECTFOLDERID_OBJECTFOLDERID_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(objectFolderId);
count = (Long)query.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
/**
* Returns the number of object definitions that the user has permission to view where objectFolderId = ?.
*
* @param objectFolderId the object folder ID
* @return the number of matching object definitions that the user has permission to view
*/
@Override
public int filterCountByObjectFolderId(long objectFolderId) {
if (!InlineSQLHelperUtil.isEnabled()) {
return countByObjectFolderId(objectFolderId);
}
StringBundler sb = new StringBundler(2);
sb.append(_FILTER_SQL_COUNT_OBJECTDEFINITION_WHERE);
sb.append(_FINDER_COLUMN_OBJECTFOLDERID_OBJECTFOLDERID_2);
String sql = InlineSQLHelperUtil.replacePermissionCheck(
sb.toString(), ObjectDefinition.class.getName(),
_FILTER_ENTITY_TABLE_FILTER_PK_COLUMN);
Session session = null;
try {
session = openSession();
SQLQuery sqlQuery = session.createSynchronizedSQLQuery(sql);
sqlQuery.addScalar(
COUNT_COLUMN_NAME, com.liferay.portal.kernel.dao.orm.Type.LONG);
QueryPos queryPos = QueryPos.getInstance(sqlQuery);
queryPos.add(objectFolderId);
Long count = (Long)sqlQuery.uniqueResult();
return count.intValue();
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
private static final String _FINDER_COLUMN_OBJECTFOLDERID_OBJECTFOLDERID_2 =
"objectDefinition.objectFolderId = ?";
private FinderPath _finderPathWithPaginationFindByAccountEntryRestricted;
private FinderPath _finderPathWithoutPaginationFindByAccountEntryRestricted;
private FinderPath _finderPathCountByAccountEntryRestricted;
/**
* Returns all the object definitions where accountEntryRestricted = ?.
*
* @param accountEntryRestricted the account entry restricted
* @return the matching object definitions
*/
@Override
public List findByAccountEntryRestricted(
boolean accountEntryRestricted) {
return findByAccountEntryRestricted(
accountEntryRestricted, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the object definitions where accountEntryRestricted = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectDefinitionModelImpl
.
*
*
* @param accountEntryRestricted the account entry restricted
* @param start the lower bound of the range of object definitions
* @param end the upper bound of the range of object definitions (not inclusive)
* @return the range of matching object definitions
*/
@Override
public List findByAccountEntryRestricted(
boolean accountEntryRestricted, int start, int end) {
return findByAccountEntryRestricted(
accountEntryRestricted, start, end, null);
}
/**
* Returns an ordered range of all the object definitions where accountEntryRestricted = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectDefinitionModelImpl
.
*
*
* @param accountEntryRestricted the account entry restricted
* @param start the lower bound of the range of object definitions
* @param end the upper bound of the range of object definitions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching object definitions
*/
@Override
public List findByAccountEntryRestricted(
boolean accountEntryRestricted, int start, int end,
OrderByComparator orderByComparator) {
return findByAccountEntryRestricted(
accountEntryRestricted, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the object definitions where accountEntryRestricted = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectDefinitionModelImpl
.
*
*
* @param accountEntryRestricted the account entry restricted
* @param start the lower bound of the range of object definitions
* @param end the upper bound of the range of object definitions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching object definitions
*/
@Override
public List findByAccountEntryRestricted(
boolean accountEntryRestricted, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache) {
finderPath =
_finderPathWithoutPaginationFindByAccountEntryRestricted;
finderArgs = new Object[] {accountEntryRestricted};
}
}
else if (useFinderCache) {
finderPath = _finderPathWithPaginationFindByAccountEntryRestricted;
finderArgs = new Object[] {
accountEntryRestricted, start, end, orderByComparator
};
}
List list = null;
if (useFinderCache) {
list = (List)finderCache.getResult(
finderPath, finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (ObjectDefinition objectDefinition : list) {
if (accountEntryRestricted !=
objectDefinition.isAccountEntryRestricted()) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
3 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(3);
}
sb.append(_SQL_SELECT_OBJECTDEFINITION_WHERE);
sb.append(
_FINDER_COLUMN_ACCOUNTENTRYRESTRICTED_ACCOUNTENTRYRESTRICTED_2);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(ObjectDefinitionModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(accountEntryRestricted);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first object definition in the ordered set where accountEntryRestricted = ?.
*
* @param accountEntryRestricted the account entry restricted
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching object definition
* @throws NoSuchObjectDefinitionException if a matching object definition could not be found
*/
@Override
public ObjectDefinition findByAccountEntryRestricted_First(
boolean accountEntryRestricted,
OrderByComparator orderByComparator)
throws NoSuchObjectDefinitionException {
ObjectDefinition objectDefinition = fetchByAccountEntryRestricted_First(
accountEntryRestricted, orderByComparator);
if (objectDefinition != null) {
return objectDefinition;
}
StringBundler sb = new StringBundler(4);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("accountEntryRestricted=");
sb.append(accountEntryRestricted);
sb.append("}");
throw new NoSuchObjectDefinitionException(sb.toString());
}
/**
* Returns the first object definition in the ordered set where accountEntryRestricted = ?.
*
* @param accountEntryRestricted the account entry restricted
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching object definition, or null
if a matching object definition could not be found
*/
@Override
public ObjectDefinition fetchByAccountEntryRestricted_First(
boolean accountEntryRestricted,
OrderByComparator orderByComparator) {
List list = findByAccountEntryRestricted(
accountEntryRestricted, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last object definition in the ordered set where accountEntryRestricted = ?.
*
* @param accountEntryRestricted the account entry restricted
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching object definition
* @throws NoSuchObjectDefinitionException if a matching object definition could not be found
*/
@Override
public ObjectDefinition findByAccountEntryRestricted_Last(
boolean accountEntryRestricted,
OrderByComparator orderByComparator)
throws NoSuchObjectDefinitionException {
ObjectDefinition objectDefinition = fetchByAccountEntryRestricted_Last(
accountEntryRestricted, orderByComparator);
if (objectDefinition != null) {
return objectDefinition;
}
StringBundler sb = new StringBundler(4);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("accountEntryRestricted=");
sb.append(accountEntryRestricted);
sb.append("}");
throw new NoSuchObjectDefinitionException(sb.toString());
}
/**
* Returns the last object definition in the ordered set where accountEntryRestricted = ?.
*
* @param accountEntryRestricted the account entry restricted
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching object definition, or null
if a matching object definition could not be found
*/
@Override
public ObjectDefinition fetchByAccountEntryRestricted_Last(
boolean accountEntryRestricted,
OrderByComparator orderByComparator) {
int count = countByAccountEntryRestricted(accountEntryRestricted);
if (count == 0) {
return null;
}
List list = findByAccountEntryRestricted(
accountEntryRestricted, count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the object definitions before and after the current object definition in the ordered set where accountEntryRestricted = ?.
*
* @param objectDefinitionId the primary key of the current object definition
* @param accountEntryRestricted the account entry restricted
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next object definition
* @throws NoSuchObjectDefinitionException if a object definition with the primary key could not be found
*/
@Override
public ObjectDefinition[] findByAccountEntryRestricted_PrevAndNext(
long objectDefinitionId, boolean accountEntryRestricted,
OrderByComparator orderByComparator)
throws NoSuchObjectDefinitionException {
ObjectDefinition objectDefinition = findByPrimaryKey(
objectDefinitionId);
Session session = null;
try {
session = openSession();
ObjectDefinition[] array = new ObjectDefinitionImpl[3];
array[0] = getByAccountEntryRestricted_PrevAndNext(
session, objectDefinition, accountEntryRestricted,
orderByComparator, true);
array[1] = objectDefinition;
array[2] = getByAccountEntryRestricted_PrevAndNext(
session, objectDefinition, accountEntryRestricted,
orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected ObjectDefinition getByAccountEntryRestricted_PrevAndNext(
Session session, ObjectDefinition objectDefinition,
boolean accountEntryRestricted,
OrderByComparator orderByComparator,
boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(3);
}
sb.append(_SQL_SELECT_OBJECTDEFINITION_WHERE);
sb.append(
_FINDER_COLUMN_ACCOUNTENTRYRESTRICTED_ACCOUNTENTRYRESTRICTED_2);
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(ObjectDefinitionModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(accountEntryRestricted);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
objectDefinition)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Returns all the object definitions that the user has permission to view where accountEntryRestricted = ?.
*
* @param accountEntryRestricted the account entry restricted
* @return the matching object definitions that the user has permission to view
*/
@Override
public List filterFindByAccountEntryRestricted(
boolean accountEntryRestricted) {
return filterFindByAccountEntryRestricted(
accountEntryRestricted, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the object definitions that the user has permission to view where accountEntryRestricted = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectDefinitionModelImpl
.
*
*
* @param accountEntryRestricted the account entry restricted
* @param start the lower bound of the range of object definitions
* @param end the upper bound of the range of object definitions (not inclusive)
* @return the range of matching object definitions that the user has permission to view
*/
@Override
public List filterFindByAccountEntryRestricted(
boolean accountEntryRestricted, int start, int end) {
return filterFindByAccountEntryRestricted(
accountEntryRestricted, start, end, null);
}
/**
* Returns an ordered range of all the object definitions that the user has permissions to view where accountEntryRestricted = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectDefinitionModelImpl
.
*
*
* @param accountEntryRestricted the account entry restricted
* @param start the lower bound of the range of object definitions
* @param end the upper bound of the range of object definitions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching object definitions that the user has permission to view
*/
@Override
public List filterFindByAccountEntryRestricted(
boolean accountEntryRestricted, int start, int end,
OrderByComparator orderByComparator) {
if (!InlineSQLHelperUtil.isEnabled()) {
return findByAccountEntryRestricted(
accountEntryRestricted, start, end, orderByComparator);
}
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
3 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(4);
}
if (getDB().isSupportsInlineDistinct()) {
sb.append(_FILTER_SQL_SELECT_OBJECTDEFINITION_WHERE);
}
else {
sb.append(
_FILTER_SQL_SELECT_OBJECTDEFINITION_NO_INLINE_DISTINCT_WHERE_1);
}
sb.append(
_FINDER_COLUMN_ACCOUNTENTRYRESTRICTED_ACCOUNTENTRYRESTRICTED_2);
if (!getDB().isSupportsInlineDistinct()) {
sb.append(
_FILTER_SQL_SELECT_OBJECTDEFINITION_NO_INLINE_DISTINCT_WHERE_2);
}
if (orderByComparator != null) {
if (getDB().isSupportsInlineDistinct()) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator, true);
}
else {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_TABLE, orderByComparator, true);
}
}
else {
if (getDB().isSupportsInlineDistinct()) {
sb.append(
ObjectDefinitionModelImpl.ORDER_BY_SQL_INLINE_DISTINCT);
}
else {
sb.append(ObjectDefinitionModelImpl.ORDER_BY_SQL);
}
}
String sql = InlineSQLHelperUtil.replacePermissionCheck(
sb.toString(), ObjectDefinition.class.getName(),
_FILTER_ENTITY_TABLE_FILTER_PK_COLUMN);
Session session = null;
try {
session = openSession();
SQLQuery sqlQuery = session.createSynchronizedSQLQuery(sql);
if (getDB().isSupportsInlineDistinct()) {
sqlQuery.addEntity(
_FILTER_ENTITY_ALIAS, ObjectDefinitionImpl.class);
}
else {
sqlQuery.addEntity(
_FILTER_ENTITY_TABLE, ObjectDefinitionImpl.class);
}
QueryPos queryPos = QueryPos.getInstance(sqlQuery);
queryPos.add(accountEntryRestricted);
return (List)QueryUtil.list(
sqlQuery, getDialect(), start, end);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
/**
* Returns the object definitions before and after the current object definition in the ordered set of object definitions that the user has permission to view where accountEntryRestricted = ?.
*
* @param objectDefinitionId the primary key of the current object definition
* @param accountEntryRestricted the account entry restricted
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next object definition
* @throws NoSuchObjectDefinitionException if a object definition with the primary key could not be found
*/
@Override
public ObjectDefinition[] filterFindByAccountEntryRestricted_PrevAndNext(
long objectDefinitionId, boolean accountEntryRestricted,
OrderByComparator orderByComparator)
throws NoSuchObjectDefinitionException {
if (!InlineSQLHelperUtil.isEnabled()) {
return findByAccountEntryRestricted_PrevAndNext(
objectDefinitionId, accountEntryRestricted, orderByComparator);
}
ObjectDefinition objectDefinition = findByPrimaryKey(
objectDefinitionId);
Session session = null;
try {
session = openSession();
ObjectDefinition[] array = new ObjectDefinitionImpl[3];
array[0] = filterGetByAccountEntryRestricted_PrevAndNext(
session, objectDefinition, accountEntryRestricted,
orderByComparator, true);
array[1] = objectDefinition;
array[2] = filterGetByAccountEntryRestricted_PrevAndNext(
session, objectDefinition, accountEntryRestricted,
orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected ObjectDefinition filterGetByAccountEntryRestricted_PrevAndNext(
Session session, ObjectDefinition objectDefinition,
boolean accountEntryRestricted,
OrderByComparator orderByComparator,
boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
5 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(4);
}
if (getDB().isSupportsInlineDistinct()) {
sb.append(_FILTER_SQL_SELECT_OBJECTDEFINITION_WHERE);
}
else {
sb.append(
_FILTER_SQL_SELECT_OBJECTDEFINITION_NO_INLINE_DISTINCT_WHERE_1);
}
sb.append(
_FINDER_COLUMN_ACCOUNTENTRYRESTRICTED_ACCOUNTENTRYRESTRICTED_2);
if (!getDB().isSupportsInlineDistinct()) {
sb.append(
_FILTER_SQL_SELECT_OBJECTDEFINITION_NO_INLINE_DISTINCT_WHERE_2);
}
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
if (getDB().isSupportsInlineDistinct()) {
sb.append(
getColumnName(
_ORDER_BY_ENTITY_ALIAS, orderByConditionFields[i],
true));
}
else {
sb.append(
getColumnName(
_ORDER_BY_ENTITY_TABLE, orderByConditionFields[i],
true));
}
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
if (getDB().isSupportsInlineDistinct()) {
sb.append(
getColumnName(
_ORDER_BY_ENTITY_ALIAS, orderByFields[i], true));
}
else {
sb.append(
getColumnName(
_ORDER_BY_ENTITY_TABLE, orderByFields[i], true));
}
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
if (getDB().isSupportsInlineDistinct()) {
sb.append(
ObjectDefinitionModelImpl.ORDER_BY_SQL_INLINE_DISTINCT);
}
else {
sb.append(ObjectDefinitionModelImpl.ORDER_BY_SQL);
}
}
String sql = InlineSQLHelperUtil.replacePermissionCheck(
sb.toString(), ObjectDefinition.class.getName(),
_FILTER_ENTITY_TABLE_FILTER_PK_COLUMN);
SQLQuery sqlQuery = session.createSynchronizedSQLQuery(sql);
sqlQuery.setFirstResult(0);
sqlQuery.setMaxResults(2);
if (getDB().isSupportsInlineDistinct()) {
sqlQuery.addEntity(
_FILTER_ENTITY_ALIAS, ObjectDefinitionImpl.class);
}
else {
sqlQuery.addEntity(
_FILTER_ENTITY_TABLE, ObjectDefinitionImpl.class);
}
QueryPos queryPos = QueryPos.getInstance(sqlQuery);
queryPos.add(accountEntryRestricted);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
objectDefinition)) {
queryPos.add(orderByConditionValue);
}
}
List list = sqlQuery.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the object definitions where accountEntryRestricted = ? from the database.
*
* @param accountEntryRestricted the account entry restricted
*/
@Override
public void removeByAccountEntryRestricted(boolean accountEntryRestricted) {
for (ObjectDefinition objectDefinition :
findByAccountEntryRestricted(
accountEntryRestricted, QueryUtil.ALL_POS,
QueryUtil.ALL_POS, null)) {
remove(objectDefinition);
}
}
/**
* Returns the number of object definitions where accountEntryRestricted = ?.
*
* @param accountEntryRestricted the account entry restricted
* @return the number of matching object definitions
*/
@Override
public int countByAccountEntryRestricted(boolean accountEntryRestricted) {
FinderPath finderPath = _finderPathCountByAccountEntryRestricted;
Object[] finderArgs = new Object[] {accountEntryRestricted};
Long count = (Long)finderCache.getResult(finderPath, finderArgs, this);
if (count == null) {
StringBundler sb = new StringBundler(2);
sb.append(_SQL_COUNT_OBJECTDEFINITION_WHERE);
sb.append(
_FINDER_COLUMN_ACCOUNTENTRYRESTRICTED_ACCOUNTENTRYRESTRICTED_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(accountEntryRestricted);
count = (Long)query.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
/**
* Returns the number of object definitions that the user has permission to view where accountEntryRestricted = ?.
*
* @param accountEntryRestricted the account entry restricted
* @return the number of matching object definitions that the user has permission to view
*/
@Override
public int filterCountByAccountEntryRestricted(
boolean accountEntryRestricted) {
if (!InlineSQLHelperUtil.isEnabled()) {
return countByAccountEntryRestricted(accountEntryRestricted);
}
StringBundler sb = new StringBundler(2);
sb.append(_FILTER_SQL_COUNT_OBJECTDEFINITION_WHERE);
sb.append(
_FINDER_COLUMN_ACCOUNTENTRYRESTRICTED_ACCOUNTENTRYRESTRICTED_2);
String sql = InlineSQLHelperUtil.replacePermissionCheck(
sb.toString(), ObjectDefinition.class.getName(),
_FILTER_ENTITY_TABLE_FILTER_PK_COLUMN);
Session session = null;
try {
session = openSession();
SQLQuery sqlQuery = session.createSynchronizedSQLQuery(sql);
sqlQuery.addScalar(
COUNT_COLUMN_NAME, com.liferay.portal.kernel.dao.orm.Type.LONG);
QueryPos queryPos = QueryPos.getInstance(sqlQuery);
queryPos.add(accountEntryRestricted);
Long count = (Long)sqlQuery.uniqueResult();
return count.intValue();
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
private static final String
_FINDER_COLUMN_ACCOUNTENTRYRESTRICTED_ACCOUNTENTRYRESTRICTED_2 =
"objectDefinition.accountEntryRestricted = ?";
private FinderPath _finderPathWithPaginationFindBySystem;
private FinderPath _finderPathWithoutPaginationFindBySystem;
private FinderPath _finderPathCountBySystem;
/**
* Returns all the object definitions where system = ?.
*
* @param system the system
* @return the matching object definitions
*/
@Override
public List findBySystem(boolean system) {
return findBySystem(system, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the object definitions where system = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectDefinitionModelImpl
.
*
*
* @param system the system
* @param start the lower bound of the range of object definitions
* @param end the upper bound of the range of object definitions (not inclusive)
* @return the range of matching object definitions
*/
@Override
public List findBySystem(
boolean system, int start, int end) {
return findBySystem(system, start, end, null);
}
/**
* Returns an ordered range of all the object definitions where system = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectDefinitionModelImpl
.
*
*
* @param system the system
* @param start the lower bound of the range of object definitions
* @param end the upper bound of the range of object definitions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching object definitions
*/
@Override
public List findBySystem(
boolean system, int start, int end,
OrderByComparator orderByComparator) {
return findBySystem(system, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the object definitions where system = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectDefinitionModelImpl
.
*
*
* @param system the system
* @param start the lower bound of the range of object definitions
* @param end the upper bound of the range of object definitions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching object definitions
*/
@Override
public List findBySystem(
boolean system, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache) {
finderPath = _finderPathWithoutPaginationFindBySystem;
finderArgs = new Object[] {system};
}
}
else if (useFinderCache) {
finderPath = _finderPathWithPaginationFindBySystem;
finderArgs = new Object[] {system, start, end, orderByComparator};
}
List list = null;
if (useFinderCache) {
list = (List)finderCache.getResult(
finderPath, finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (ObjectDefinition objectDefinition : list) {
if (system != objectDefinition.isSystem()) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
3 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(3);
}
sb.append(_SQL_SELECT_OBJECTDEFINITION_WHERE);
sb.append(_FINDER_COLUMN_SYSTEM_SYSTEM_2);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(ObjectDefinitionModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(system);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first object definition in the ordered set where system = ?.
*
* @param system the system
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching object definition
* @throws NoSuchObjectDefinitionException if a matching object definition could not be found
*/
@Override
public ObjectDefinition findBySystem_First(
boolean system,
OrderByComparator orderByComparator)
throws NoSuchObjectDefinitionException {
ObjectDefinition objectDefinition = fetchBySystem_First(
system, orderByComparator);
if (objectDefinition != null) {
return objectDefinition;
}
StringBundler sb = new StringBundler(4);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("system=");
sb.append(system);
sb.append("}");
throw new NoSuchObjectDefinitionException(sb.toString());
}
/**
* Returns the first object definition in the ordered set where system = ?.
*
* @param system the system
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching object definition, or null
if a matching object definition could not be found
*/
@Override
public ObjectDefinition fetchBySystem_First(
boolean system, OrderByComparator orderByComparator) {
List list = findBySystem(
system, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last object definition in the ordered set where system = ?.
*
* @param system the system
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching object definition
* @throws NoSuchObjectDefinitionException if a matching object definition could not be found
*/
@Override
public ObjectDefinition findBySystem_Last(
boolean system,
OrderByComparator orderByComparator)
throws NoSuchObjectDefinitionException {
ObjectDefinition objectDefinition = fetchBySystem_Last(
system, orderByComparator);
if (objectDefinition != null) {
return objectDefinition;
}
StringBundler sb = new StringBundler(4);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("system=");
sb.append(system);
sb.append("}");
throw new NoSuchObjectDefinitionException(sb.toString());
}
/**
* Returns the last object definition in the ordered set where system = ?.
*
* @param system the system
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching object definition, or null
if a matching object definition could not be found
*/
@Override
public ObjectDefinition fetchBySystem_Last(
boolean system, OrderByComparator orderByComparator) {
int count = countBySystem(system);
if (count == 0) {
return null;
}
List list = findBySystem(
system, count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the object definitions before and after the current object definition in the ordered set where system = ?.
*
* @param objectDefinitionId the primary key of the current object definition
* @param system the system
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next object definition
* @throws NoSuchObjectDefinitionException if a object definition with the primary key could not be found
*/
@Override
public ObjectDefinition[] findBySystem_PrevAndNext(
long objectDefinitionId, boolean system,
OrderByComparator orderByComparator)
throws NoSuchObjectDefinitionException {
ObjectDefinition objectDefinition = findByPrimaryKey(
objectDefinitionId);
Session session = null;
try {
session = openSession();
ObjectDefinition[] array = new ObjectDefinitionImpl[3];
array[0] = getBySystem_PrevAndNext(
session, objectDefinition, system, orderByComparator, true);
array[1] = objectDefinition;
array[2] = getBySystem_PrevAndNext(
session, objectDefinition, system, orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected ObjectDefinition getBySystem_PrevAndNext(
Session session, ObjectDefinition objectDefinition, boolean system,
OrderByComparator orderByComparator,
boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(3);
}
sb.append(_SQL_SELECT_OBJECTDEFINITION_WHERE);
sb.append(_FINDER_COLUMN_SYSTEM_SYSTEM_2);
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(ObjectDefinitionModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(system);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
objectDefinition)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Returns all the object definitions that the user has permission to view where system = ?.
*
* @param system the system
* @return the matching object definitions that the user has permission to view
*/
@Override
public List filterFindBySystem(boolean system) {
return filterFindBySystem(
system, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the object definitions that the user has permission to view where system = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectDefinitionModelImpl
.
*
*
* @param system the system
* @param start the lower bound of the range of object definitions
* @param end the upper bound of the range of object definitions (not inclusive)
* @return the range of matching object definitions that the user has permission to view
*/
@Override
public List filterFindBySystem(
boolean system, int start, int end) {
return filterFindBySystem(system, start, end, null);
}
/**
* Returns an ordered range of all the object definitions that the user has permissions to view where system = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectDefinitionModelImpl
.
*
*
* @param system the system
* @param start the lower bound of the range of object definitions
* @param end the upper bound of the range of object definitions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching object definitions that the user has permission to view
*/
@Override
public List filterFindBySystem(
boolean system, int start, int end,
OrderByComparator orderByComparator) {
if (!InlineSQLHelperUtil.isEnabled()) {
return findBySystem(system, start, end, orderByComparator);
}
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
3 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(4);
}
if (getDB().isSupportsInlineDistinct()) {
sb.append(_FILTER_SQL_SELECT_OBJECTDEFINITION_WHERE);
}
else {
sb.append(
_FILTER_SQL_SELECT_OBJECTDEFINITION_NO_INLINE_DISTINCT_WHERE_1);
}
sb.append(_FINDER_COLUMN_SYSTEM_SYSTEM_2_SQL);
if (!getDB().isSupportsInlineDistinct()) {
sb.append(
_FILTER_SQL_SELECT_OBJECTDEFINITION_NO_INLINE_DISTINCT_WHERE_2);
}
if (orderByComparator != null) {
if (getDB().isSupportsInlineDistinct()) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator, true);
}
else {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_TABLE, orderByComparator, true);
}
}
else {
if (getDB().isSupportsInlineDistinct()) {
sb.append(
ObjectDefinitionModelImpl.ORDER_BY_SQL_INLINE_DISTINCT);
}
else {
sb.append(ObjectDefinitionModelImpl.ORDER_BY_SQL);
}
}
String sql = InlineSQLHelperUtil.replacePermissionCheck(
sb.toString(), ObjectDefinition.class.getName(),
_FILTER_ENTITY_TABLE_FILTER_PK_COLUMN);
Session session = null;
try {
session = openSession();
SQLQuery sqlQuery = session.createSynchronizedSQLQuery(sql);
if (getDB().isSupportsInlineDistinct()) {
sqlQuery.addEntity(
_FILTER_ENTITY_ALIAS, ObjectDefinitionImpl.class);
}
else {
sqlQuery.addEntity(
_FILTER_ENTITY_TABLE, ObjectDefinitionImpl.class);
}
QueryPos queryPos = QueryPos.getInstance(sqlQuery);
queryPos.add(system);
return (List)QueryUtil.list(
sqlQuery, getDialect(), start, end);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
/**
* Returns the object definitions before and after the current object definition in the ordered set of object definitions that the user has permission to view where system = ?.
*
* @param objectDefinitionId the primary key of the current object definition
* @param system the system
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next object definition
* @throws NoSuchObjectDefinitionException if a object definition with the primary key could not be found
*/
@Override
public ObjectDefinition[] filterFindBySystem_PrevAndNext(
long objectDefinitionId, boolean system,
OrderByComparator orderByComparator)
throws NoSuchObjectDefinitionException {
if (!InlineSQLHelperUtil.isEnabled()) {
return findBySystem_PrevAndNext(
objectDefinitionId, system, orderByComparator);
}
ObjectDefinition objectDefinition = findByPrimaryKey(
objectDefinitionId);
Session session = null;
try {
session = openSession();
ObjectDefinition[] array = new ObjectDefinitionImpl[3];
array[0] = filterGetBySystem_PrevAndNext(
session, objectDefinition, system, orderByComparator, true);
array[1] = objectDefinition;
array[2] = filterGetBySystem_PrevAndNext(
session, objectDefinition, system, orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected ObjectDefinition filterGetBySystem_PrevAndNext(
Session session, ObjectDefinition objectDefinition, boolean system,
OrderByComparator orderByComparator,
boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
5 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(4);
}
if (getDB().isSupportsInlineDistinct()) {
sb.append(_FILTER_SQL_SELECT_OBJECTDEFINITION_WHERE);
}
else {
sb.append(
_FILTER_SQL_SELECT_OBJECTDEFINITION_NO_INLINE_DISTINCT_WHERE_1);
}
sb.append(_FINDER_COLUMN_SYSTEM_SYSTEM_2_SQL);
if (!getDB().isSupportsInlineDistinct()) {
sb.append(
_FILTER_SQL_SELECT_OBJECTDEFINITION_NO_INLINE_DISTINCT_WHERE_2);
}
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
if (getDB().isSupportsInlineDistinct()) {
sb.append(
getColumnName(
_ORDER_BY_ENTITY_ALIAS, orderByConditionFields[i],
true));
}
else {
sb.append(
getColumnName(
_ORDER_BY_ENTITY_TABLE, orderByConditionFields[i],
true));
}
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
if (getDB().isSupportsInlineDistinct()) {
sb.append(
getColumnName(
_ORDER_BY_ENTITY_ALIAS, orderByFields[i], true));
}
else {
sb.append(
getColumnName(
_ORDER_BY_ENTITY_TABLE, orderByFields[i], true));
}
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
if (getDB().isSupportsInlineDistinct()) {
sb.append(
ObjectDefinitionModelImpl.ORDER_BY_SQL_INLINE_DISTINCT);
}
else {
sb.append(ObjectDefinitionModelImpl.ORDER_BY_SQL);
}
}
String sql = InlineSQLHelperUtil.replacePermissionCheck(
sb.toString(), ObjectDefinition.class.getName(),
_FILTER_ENTITY_TABLE_FILTER_PK_COLUMN);
SQLQuery sqlQuery = session.createSynchronizedSQLQuery(sql);
sqlQuery.setFirstResult(0);
sqlQuery.setMaxResults(2);
if (getDB().isSupportsInlineDistinct()) {
sqlQuery.addEntity(
_FILTER_ENTITY_ALIAS, ObjectDefinitionImpl.class);
}
else {
sqlQuery.addEntity(
_FILTER_ENTITY_TABLE, ObjectDefinitionImpl.class);
}
QueryPos queryPos = QueryPos.getInstance(sqlQuery);
queryPos.add(system);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
objectDefinition)) {
queryPos.add(orderByConditionValue);
}
}
List list = sqlQuery.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the object definitions where system = ? from the database.
*
* @param system the system
*/
@Override
public void removeBySystem(boolean system) {
for (ObjectDefinition objectDefinition :
findBySystem(
system, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null)) {
remove(objectDefinition);
}
}
/**
* Returns the number of object definitions where system = ?.
*
* @param system the system
* @return the number of matching object definitions
*/
@Override
public int countBySystem(boolean system) {
FinderPath finderPath = _finderPathCountBySystem;
Object[] finderArgs = new Object[] {system};
Long count = (Long)finderCache.getResult(finderPath, finderArgs, this);
if (count == null) {
StringBundler sb = new StringBundler(2);
sb.append(_SQL_COUNT_OBJECTDEFINITION_WHERE);
sb.append(_FINDER_COLUMN_SYSTEM_SYSTEM_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(system);
count = (Long)query.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
/**
* Returns the number of object definitions that the user has permission to view where system = ?.
*
* @param system the system
* @return the number of matching object definitions that the user has permission to view
*/
@Override
public int filterCountBySystem(boolean system) {
if (!InlineSQLHelperUtil.isEnabled()) {
return countBySystem(system);
}
StringBundler sb = new StringBundler(2);
sb.append(_FILTER_SQL_COUNT_OBJECTDEFINITION_WHERE);
sb.append(_FINDER_COLUMN_SYSTEM_SYSTEM_2_SQL);
String sql = InlineSQLHelperUtil.replacePermissionCheck(
sb.toString(), ObjectDefinition.class.getName(),
_FILTER_ENTITY_TABLE_FILTER_PK_COLUMN);
Session session = null;
try {
session = openSession();
SQLQuery sqlQuery = session.createSynchronizedSQLQuery(sql);
sqlQuery.addScalar(
COUNT_COLUMN_NAME, com.liferay.portal.kernel.dao.orm.Type.LONG);
QueryPos queryPos = QueryPos.getInstance(sqlQuery);
queryPos.add(system);
Long count = (Long)sqlQuery.uniqueResult();
return count.intValue();
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
private static final String _FINDER_COLUMN_SYSTEM_SYSTEM_2 =
"objectDefinition.system = ?";
private static final String _FINDER_COLUMN_SYSTEM_SYSTEM_2_SQL =
"objectDefinition.system_ = ?";
private FinderPath _finderPathWithPaginationFindByC_U;
private FinderPath _finderPathWithoutPaginationFindByC_U;
private FinderPath _finderPathCountByC_U;
/**
* Returns all the object definitions where companyId = ? and userId = ?.
*
* @param companyId the company ID
* @param userId the user ID
* @return the matching object definitions
*/
@Override
public List findByC_U(long companyId, long userId) {
return findByC_U(
companyId, userId, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the object definitions where companyId = ? and userId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectDefinitionModelImpl
.
*
*
* @param companyId the company ID
* @param userId the user ID
* @param start the lower bound of the range of object definitions
* @param end the upper bound of the range of object definitions (not inclusive)
* @return the range of matching object definitions
*/
@Override
public List findByC_U(
long companyId, long userId, int start, int end) {
return findByC_U(companyId, userId, start, end, null);
}
/**
* Returns an ordered range of all the object definitions where companyId = ? and userId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectDefinitionModelImpl
.
*
*
* @param companyId the company ID
* @param userId the user ID
* @param start the lower bound of the range of object definitions
* @param end the upper bound of the range of object definitions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching object definitions
*/
@Override
public List findByC_U(
long companyId, long userId, int start, int end,
OrderByComparator orderByComparator) {
return findByC_U(
companyId, userId, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the object definitions where companyId = ? and userId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectDefinitionModelImpl
.
*
*
* @param companyId the company ID
* @param userId the user ID
* @param start the lower bound of the range of object definitions
* @param end the upper bound of the range of object definitions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching object definitions
*/
@Override
public List findByC_U(
long companyId, long userId, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache) {
finderPath = _finderPathWithoutPaginationFindByC_U;
finderArgs = new Object[] {companyId, userId};
}
}
else if (useFinderCache) {
finderPath = _finderPathWithPaginationFindByC_U;
finderArgs = new Object[] {
companyId, userId, start, end, orderByComparator
};
}
List list = null;
if (useFinderCache) {
list = (List)finderCache.getResult(
finderPath, finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (ObjectDefinition objectDefinition : list) {
if ((companyId != objectDefinition.getCompanyId()) ||
(userId != objectDefinition.getUserId())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_OBJECTDEFINITION_WHERE);
sb.append(_FINDER_COLUMN_C_U_COMPANYID_2);
sb.append(_FINDER_COLUMN_C_U_USERID_2);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(ObjectDefinitionModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(companyId);
queryPos.add(userId);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first object definition in the ordered set where companyId = ? and userId = ?.
*
* @param companyId the company ID
* @param userId the user ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching object definition
* @throws NoSuchObjectDefinitionException if a matching object definition could not be found
*/
@Override
public ObjectDefinition findByC_U_First(
long companyId, long userId,
OrderByComparator orderByComparator)
throws NoSuchObjectDefinitionException {
ObjectDefinition objectDefinition = fetchByC_U_First(
companyId, userId, orderByComparator);
if (objectDefinition != null) {
return objectDefinition;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("companyId=");
sb.append(companyId);
sb.append(", userId=");
sb.append(userId);
sb.append("}");
throw new NoSuchObjectDefinitionException(sb.toString());
}
/**
* Returns the first object definition in the ordered set where companyId = ? and userId = ?.
*
* @param companyId the company ID
* @param userId the user ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching object definition, or null
if a matching object definition could not be found
*/
@Override
public ObjectDefinition fetchByC_U_First(
long companyId, long userId,
OrderByComparator orderByComparator) {
List list = findByC_U(
companyId, userId, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last object definition in the ordered set where companyId = ? and userId = ?.
*
* @param companyId the company ID
* @param userId the user ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching object definition
* @throws NoSuchObjectDefinitionException if a matching object definition could not be found
*/
@Override
public ObjectDefinition findByC_U_Last(
long companyId, long userId,
OrderByComparator orderByComparator)
throws NoSuchObjectDefinitionException {
ObjectDefinition objectDefinition = fetchByC_U_Last(
companyId, userId, orderByComparator);
if (objectDefinition != null) {
return objectDefinition;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("companyId=");
sb.append(companyId);
sb.append(", userId=");
sb.append(userId);
sb.append("}");
throw new NoSuchObjectDefinitionException(sb.toString());
}
/**
* Returns the last object definition in the ordered set where companyId = ? and userId = ?.
*
* @param companyId the company ID
* @param userId the user ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching object definition, or null
if a matching object definition could not be found
*/
@Override
public ObjectDefinition fetchByC_U_Last(
long companyId, long userId,
OrderByComparator orderByComparator) {
int count = countByC_U(companyId, userId);
if (count == 0) {
return null;
}
List list = findByC_U(
companyId, userId, count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the object definitions before and after the current object definition in the ordered set where companyId = ? and userId = ?.
*
* @param objectDefinitionId the primary key of the current object definition
* @param companyId the company ID
* @param userId the user ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next object definition
* @throws NoSuchObjectDefinitionException if a object definition with the primary key could not be found
*/
@Override
public ObjectDefinition[] findByC_U_PrevAndNext(
long objectDefinitionId, long companyId, long userId,
OrderByComparator orderByComparator)
throws NoSuchObjectDefinitionException {
ObjectDefinition objectDefinition = findByPrimaryKey(
objectDefinitionId);
Session session = null;
try {
session = openSession();
ObjectDefinition[] array = new ObjectDefinitionImpl[3];
array[0] = getByC_U_PrevAndNext(
session, objectDefinition, companyId, userId, orderByComparator,
true);
array[1] = objectDefinition;
array[2] = getByC_U_PrevAndNext(
session, objectDefinition, companyId, userId, orderByComparator,
false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected ObjectDefinition getByC_U_PrevAndNext(
Session session, ObjectDefinition objectDefinition, long companyId,
long userId, OrderByComparator orderByComparator,
boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
5 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_OBJECTDEFINITION_WHERE);
sb.append(_FINDER_COLUMN_C_U_COMPANYID_2);
sb.append(_FINDER_COLUMN_C_U_USERID_2);
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(ObjectDefinitionModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(companyId);
queryPos.add(userId);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
objectDefinition)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Returns all the object definitions that the user has permission to view where companyId = ? and userId = ?.
*
* @param companyId the company ID
* @param userId the user ID
* @return the matching object definitions that the user has permission to view
*/
@Override
public List filterFindByC_U(long companyId, long userId) {
return filterFindByC_U(
companyId, userId, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the object definitions that the user has permission to view where companyId = ? and userId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectDefinitionModelImpl
.
*
*
* @param companyId the company ID
* @param userId the user ID
* @param start the lower bound of the range of object definitions
* @param end the upper bound of the range of object definitions (not inclusive)
* @return the range of matching object definitions that the user has permission to view
*/
@Override
public List filterFindByC_U(
long companyId, long userId, int start, int end) {
return filterFindByC_U(companyId, userId, start, end, null);
}
/**
* Returns an ordered range of all the object definitions that the user has permissions to view where companyId = ? and userId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectDefinitionModelImpl
.
*
*
* @param companyId the company ID
* @param userId the user ID
* @param start the lower bound of the range of object definitions
* @param end the upper bound of the range of object definitions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching object definitions that the user has permission to view
*/
@Override
public List filterFindByC_U(
long companyId, long userId, int start, int end,
OrderByComparator orderByComparator) {
if (!InlineSQLHelperUtil.isEnabled(companyId, 0)) {
return findByC_U(companyId, userId, start, end, orderByComparator);
}
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(5);
}
if (getDB().isSupportsInlineDistinct()) {
sb.append(_FILTER_SQL_SELECT_OBJECTDEFINITION_WHERE);
}
else {
sb.append(
_FILTER_SQL_SELECT_OBJECTDEFINITION_NO_INLINE_DISTINCT_WHERE_1);
}
sb.append(_FINDER_COLUMN_C_U_COMPANYID_2);
sb.append(_FINDER_COLUMN_C_U_USERID_2);
if (!getDB().isSupportsInlineDistinct()) {
sb.append(
_FILTER_SQL_SELECT_OBJECTDEFINITION_NO_INLINE_DISTINCT_WHERE_2);
}
if (orderByComparator != null) {
if (getDB().isSupportsInlineDistinct()) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator, true);
}
else {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_TABLE, orderByComparator, true);
}
}
else {
if (getDB().isSupportsInlineDistinct()) {
sb.append(
ObjectDefinitionModelImpl.ORDER_BY_SQL_INLINE_DISTINCT);
}
else {
sb.append(ObjectDefinitionModelImpl.ORDER_BY_SQL);
}
}
String sql = InlineSQLHelperUtil.replacePermissionCheck(
sb.toString(), ObjectDefinition.class.getName(),
_FILTER_ENTITY_TABLE_FILTER_PK_COLUMN);
Session session = null;
try {
session = openSession();
SQLQuery sqlQuery = session.createSynchronizedSQLQuery(sql);
if (getDB().isSupportsInlineDistinct()) {
sqlQuery.addEntity(
_FILTER_ENTITY_ALIAS, ObjectDefinitionImpl.class);
}
else {
sqlQuery.addEntity(
_FILTER_ENTITY_TABLE, ObjectDefinitionImpl.class);
}
QueryPos queryPos = QueryPos.getInstance(sqlQuery);
queryPos.add(companyId);
queryPos.add(userId);
return (List)QueryUtil.list(
sqlQuery, getDialect(), start, end);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
/**
* Returns the object definitions before and after the current object definition in the ordered set of object definitions that the user has permission to view where companyId = ? and userId = ?.
*
* @param objectDefinitionId the primary key of the current object definition
* @param companyId the company ID
* @param userId the user ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next object definition
* @throws NoSuchObjectDefinitionException if a object definition with the primary key could not be found
*/
@Override
public ObjectDefinition[] filterFindByC_U_PrevAndNext(
long objectDefinitionId, long companyId, long userId,
OrderByComparator orderByComparator)
throws NoSuchObjectDefinitionException {
if (!InlineSQLHelperUtil.isEnabled(companyId, 0)) {
return findByC_U_PrevAndNext(
objectDefinitionId, companyId, userId, orderByComparator);
}
ObjectDefinition objectDefinition = findByPrimaryKey(
objectDefinitionId);
Session session = null;
try {
session = openSession();
ObjectDefinition[] array = new ObjectDefinitionImpl[3];
array[0] = filterGetByC_U_PrevAndNext(
session, objectDefinition, companyId, userId, orderByComparator,
true);
array[1] = objectDefinition;
array[2] = filterGetByC_U_PrevAndNext(
session, objectDefinition, companyId, userId, orderByComparator,
false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected ObjectDefinition filterGetByC_U_PrevAndNext(
Session session, ObjectDefinition objectDefinition, long companyId,
long userId, OrderByComparator orderByComparator,
boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
6 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(5);
}
if (getDB().isSupportsInlineDistinct()) {
sb.append(_FILTER_SQL_SELECT_OBJECTDEFINITION_WHERE);
}
else {
sb.append(
_FILTER_SQL_SELECT_OBJECTDEFINITION_NO_INLINE_DISTINCT_WHERE_1);
}
sb.append(_FINDER_COLUMN_C_U_COMPANYID_2);
sb.append(_FINDER_COLUMN_C_U_USERID_2);
if (!getDB().isSupportsInlineDistinct()) {
sb.append(
_FILTER_SQL_SELECT_OBJECTDEFINITION_NO_INLINE_DISTINCT_WHERE_2);
}
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
if (getDB().isSupportsInlineDistinct()) {
sb.append(
getColumnName(
_ORDER_BY_ENTITY_ALIAS, orderByConditionFields[i],
true));
}
else {
sb.append(
getColumnName(
_ORDER_BY_ENTITY_TABLE, orderByConditionFields[i],
true));
}
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
if (getDB().isSupportsInlineDistinct()) {
sb.append(
getColumnName(
_ORDER_BY_ENTITY_ALIAS, orderByFields[i], true));
}
else {
sb.append(
getColumnName(
_ORDER_BY_ENTITY_TABLE, orderByFields[i], true));
}
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
if (getDB().isSupportsInlineDistinct()) {
sb.append(
ObjectDefinitionModelImpl.ORDER_BY_SQL_INLINE_DISTINCT);
}
else {
sb.append(ObjectDefinitionModelImpl.ORDER_BY_SQL);
}
}
String sql = InlineSQLHelperUtil.replacePermissionCheck(
sb.toString(), ObjectDefinition.class.getName(),
_FILTER_ENTITY_TABLE_FILTER_PK_COLUMN);
SQLQuery sqlQuery = session.createSynchronizedSQLQuery(sql);
sqlQuery.setFirstResult(0);
sqlQuery.setMaxResults(2);
if (getDB().isSupportsInlineDistinct()) {
sqlQuery.addEntity(
_FILTER_ENTITY_ALIAS, ObjectDefinitionImpl.class);
}
else {
sqlQuery.addEntity(
_FILTER_ENTITY_TABLE, ObjectDefinitionImpl.class);
}
QueryPos queryPos = QueryPos.getInstance(sqlQuery);
queryPos.add(companyId);
queryPos.add(userId);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
objectDefinition)) {
queryPos.add(orderByConditionValue);
}
}
List list = sqlQuery.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the object definitions where companyId = ? and userId = ? from the database.
*
* @param companyId the company ID
* @param userId the user ID
*/
@Override
public void removeByC_U(long companyId, long userId) {
for (ObjectDefinition objectDefinition :
findByC_U(
companyId, userId, QueryUtil.ALL_POS, QueryUtil.ALL_POS,
null)) {
remove(objectDefinition);
}
}
/**
* Returns the number of object definitions where companyId = ? and userId = ?.
*
* @param companyId the company ID
* @param userId the user ID
* @return the number of matching object definitions
*/
@Override
public int countByC_U(long companyId, long userId) {
FinderPath finderPath = _finderPathCountByC_U;
Object[] finderArgs = new Object[] {companyId, userId};
Long count = (Long)finderCache.getResult(finderPath, finderArgs, this);
if (count == null) {
StringBundler sb = new StringBundler(3);
sb.append(_SQL_COUNT_OBJECTDEFINITION_WHERE);
sb.append(_FINDER_COLUMN_C_U_COMPANYID_2);
sb.append(_FINDER_COLUMN_C_U_USERID_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(companyId);
queryPos.add(userId);
count = (Long)query.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
/**
* Returns the number of object definitions that the user has permission to view where companyId = ? and userId = ?.
*
* @param companyId the company ID
* @param userId the user ID
* @return the number of matching object definitions that the user has permission to view
*/
@Override
public int filterCountByC_U(long companyId, long userId) {
if (!InlineSQLHelperUtil.isEnabled(companyId, 0)) {
return countByC_U(companyId, userId);
}
StringBundler sb = new StringBundler(3);
sb.append(_FILTER_SQL_COUNT_OBJECTDEFINITION_WHERE);
sb.append(_FINDER_COLUMN_C_U_COMPANYID_2);
sb.append(_FINDER_COLUMN_C_U_USERID_2);
String sql = InlineSQLHelperUtil.replacePermissionCheck(
sb.toString(), ObjectDefinition.class.getName(),
_FILTER_ENTITY_TABLE_FILTER_PK_COLUMN);
Session session = null;
try {
session = openSession();
SQLQuery sqlQuery = session.createSynchronizedSQLQuery(sql);
sqlQuery.addScalar(
COUNT_COLUMN_NAME, com.liferay.portal.kernel.dao.orm.Type.LONG);
QueryPos queryPos = QueryPos.getInstance(sqlQuery);
queryPos.add(companyId);
queryPos.add(userId);
Long count = (Long)sqlQuery.uniqueResult();
return count.intValue();
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
private static final String _FINDER_COLUMN_C_U_COMPANYID_2 =
"objectDefinition.companyId = ? AND ";
private static final String _FINDER_COLUMN_C_U_USERID_2 =
"objectDefinition.userId = ?";
private FinderPath _finderPathWithPaginationFindByC_RODI;
private FinderPath _finderPathWithoutPaginationFindByC_RODI;
private FinderPath _finderPathCountByC_RODI;
/**
* Returns all the object definitions where companyId = ? and rootObjectDefinitionId = ?.
*
* @param companyId the company ID
* @param rootObjectDefinitionId the root object definition ID
* @return the matching object definitions
*/
@Override
public List findByC_RODI(
long companyId, long rootObjectDefinitionId) {
return findByC_RODI(
companyId, rootObjectDefinitionId, QueryUtil.ALL_POS,
QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the object definitions where companyId = ? and rootObjectDefinitionId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectDefinitionModelImpl
.
*
*
* @param companyId the company ID
* @param rootObjectDefinitionId the root object definition ID
* @param start the lower bound of the range of object definitions
* @param end the upper bound of the range of object definitions (not inclusive)
* @return the range of matching object definitions
*/
@Override
public List findByC_RODI(
long companyId, long rootObjectDefinitionId, int start, int end) {
return findByC_RODI(
companyId, rootObjectDefinitionId, start, end, null);
}
/**
* Returns an ordered range of all the object definitions where companyId = ? and rootObjectDefinitionId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectDefinitionModelImpl
.
*
*
* @param companyId the company ID
* @param rootObjectDefinitionId the root object definition ID
* @param start the lower bound of the range of object definitions
* @param end the upper bound of the range of object definitions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching object definitions
*/
@Override
public List findByC_RODI(
long companyId, long rootObjectDefinitionId, int start, int end,
OrderByComparator orderByComparator) {
return findByC_RODI(
companyId, rootObjectDefinitionId, start, end, orderByComparator,
true);
}
/**
* Returns an ordered range of all the object definitions where companyId = ? and rootObjectDefinitionId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectDefinitionModelImpl
.
*
*
* @param companyId the company ID
* @param rootObjectDefinitionId the root object definition ID
* @param start the lower bound of the range of object definitions
* @param end the upper bound of the range of object definitions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching object definitions
*/
@Override
public List findByC_RODI(
long companyId, long rootObjectDefinitionId, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache) {
finderPath = _finderPathWithoutPaginationFindByC_RODI;
finderArgs = new Object[] {companyId, rootObjectDefinitionId};
}
}
else if (useFinderCache) {
finderPath = _finderPathWithPaginationFindByC_RODI;
finderArgs = new Object[] {
companyId, rootObjectDefinitionId, start, end, orderByComparator
};
}
List list = null;
if (useFinderCache) {
list = (List)finderCache.getResult(
finderPath, finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (ObjectDefinition objectDefinition : list) {
if ((companyId != objectDefinition.getCompanyId()) ||
(rootObjectDefinitionId !=
objectDefinition.getRootObjectDefinitionId())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_OBJECTDEFINITION_WHERE);
sb.append(_FINDER_COLUMN_C_RODI_COMPANYID_2);
sb.append(_FINDER_COLUMN_C_RODI_ROOTOBJECTDEFINITIONID_2);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(ObjectDefinitionModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(companyId);
queryPos.add(rootObjectDefinitionId);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first object definition in the ordered set where companyId = ? and rootObjectDefinitionId = ?.
*
* @param companyId the company ID
* @param rootObjectDefinitionId the root object definition ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching object definition
* @throws NoSuchObjectDefinitionException if a matching object definition could not be found
*/
@Override
public ObjectDefinition findByC_RODI_First(
long companyId, long rootObjectDefinitionId,
OrderByComparator orderByComparator)
throws NoSuchObjectDefinitionException {
ObjectDefinition objectDefinition = fetchByC_RODI_First(
companyId, rootObjectDefinitionId, orderByComparator);
if (objectDefinition != null) {
return objectDefinition;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("companyId=");
sb.append(companyId);
sb.append(", rootObjectDefinitionId=");
sb.append(rootObjectDefinitionId);
sb.append("}");
throw new NoSuchObjectDefinitionException(sb.toString());
}
/**
* Returns the first object definition in the ordered set where companyId = ? and rootObjectDefinitionId = ?.
*
* @param companyId the company ID
* @param rootObjectDefinitionId the root object definition ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching object definition, or null
if a matching object definition could not be found
*/
@Override
public ObjectDefinition fetchByC_RODI_First(
long companyId, long rootObjectDefinitionId,
OrderByComparator orderByComparator) {
List list = findByC_RODI(
companyId, rootObjectDefinitionId, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last object definition in the ordered set where companyId = ? and rootObjectDefinitionId = ?.
*
* @param companyId the company ID
* @param rootObjectDefinitionId the root object definition ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching object definition
* @throws NoSuchObjectDefinitionException if a matching object definition could not be found
*/
@Override
public ObjectDefinition findByC_RODI_Last(
long companyId, long rootObjectDefinitionId,
OrderByComparator orderByComparator)
throws NoSuchObjectDefinitionException {
ObjectDefinition objectDefinition = fetchByC_RODI_Last(
companyId, rootObjectDefinitionId, orderByComparator);
if (objectDefinition != null) {
return objectDefinition;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("companyId=");
sb.append(companyId);
sb.append(", rootObjectDefinitionId=");
sb.append(rootObjectDefinitionId);
sb.append("}");
throw new NoSuchObjectDefinitionException(sb.toString());
}
/**
* Returns the last object definition in the ordered set where companyId = ? and rootObjectDefinitionId = ?.
*
* @param companyId the company ID
* @param rootObjectDefinitionId the root object definition ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching object definition, or null
if a matching object definition could not be found
*/
@Override
public ObjectDefinition fetchByC_RODI_Last(
long companyId, long rootObjectDefinitionId,
OrderByComparator orderByComparator) {
int count = countByC_RODI(companyId, rootObjectDefinitionId);
if (count == 0) {
return null;
}
List list = findByC_RODI(
companyId, rootObjectDefinitionId, count - 1, count,
orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the object definitions before and after the current object definition in the ordered set where companyId = ? and rootObjectDefinitionId = ?.
*
* @param objectDefinitionId the primary key of the current object definition
* @param companyId the company ID
* @param rootObjectDefinitionId the root object definition ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next object definition
* @throws NoSuchObjectDefinitionException if a object definition with the primary key could not be found
*/
@Override
public ObjectDefinition[] findByC_RODI_PrevAndNext(
long objectDefinitionId, long companyId,
long rootObjectDefinitionId,
OrderByComparator orderByComparator)
throws NoSuchObjectDefinitionException {
ObjectDefinition objectDefinition = findByPrimaryKey(
objectDefinitionId);
Session session = null;
try {
session = openSession();
ObjectDefinition[] array = new ObjectDefinitionImpl[3];
array[0] = getByC_RODI_PrevAndNext(
session, objectDefinition, companyId, rootObjectDefinitionId,
orderByComparator, true);
array[1] = objectDefinition;
array[2] = getByC_RODI_PrevAndNext(
session, objectDefinition, companyId, rootObjectDefinitionId,
orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected ObjectDefinition getByC_RODI_PrevAndNext(
Session session, ObjectDefinition objectDefinition, long companyId,
long rootObjectDefinitionId,
OrderByComparator orderByComparator,
boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
5 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_OBJECTDEFINITION_WHERE);
sb.append(_FINDER_COLUMN_C_RODI_COMPANYID_2);
sb.append(_FINDER_COLUMN_C_RODI_ROOTOBJECTDEFINITIONID_2);
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(ObjectDefinitionModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(companyId);
queryPos.add(rootObjectDefinitionId);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
objectDefinition)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Returns all the object definitions that the user has permission to view where companyId = ? and rootObjectDefinitionId = ?.
*
* @param companyId the company ID
* @param rootObjectDefinitionId the root object definition ID
* @return the matching object definitions that the user has permission to view
*/
@Override
public List filterFindByC_RODI(
long companyId, long rootObjectDefinitionId) {
return filterFindByC_RODI(
companyId, rootObjectDefinitionId, QueryUtil.ALL_POS,
QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the object definitions that the user has permission to view where companyId = ? and rootObjectDefinitionId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectDefinitionModelImpl
.
*
*
* @param companyId the company ID
* @param rootObjectDefinitionId the root object definition ID
* @param start the lower bound of the range of object definitions
* @param end the upper bound of the range of object definitions (not inclusive)
* @return the range of matching object definitions that the user has permission to view
*/
@Override
public List filterFindByC_RODI(
long companyId, long rootObjectDefinitionId, int start, int end) {
return filterFindByC_RODI(
companyId, rootObjectDefinitionId, start, end, null);
}
/**
* Returns an ordered range of all the object definitions that the user has permissions to view where companyId = ? and rootObjectDefinitionId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectDefinitionModelImpl
.
*
*
* @param companyId the company ID
* @param rootObjectDefinitionId the root object definition ID
* @param start the lower bound of the range of object definitions
* @param end the upper bound of the range of object definitions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching object definitions that the user has permission to view
*/
@Override
public List filterFindByC_RODI(
long companyId, long rootObjectDefinitionId, int start, int end,
OrderByComparator orderByComparator) {
if (!InlineSQLHelperUtil.isEnabled(companyId, 0)) {
return findByC_RODI(
companyId, rootObjectDefinitionId, start, end,
orderByComparator);
}
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(5);
}
if (getDB().isSupportsInlineDistinct()) {
sb.append(_FILTER_SQL_SELECT_OBJECTDEFINITION_WHERE);
}
else {
sb.append(
_FILTER_SQL_SELECT_OBJECTDEFINITION_NO_INLINE_DISTINCT_WHERE_1);
}
sb.append(_FINDER_COLUMN_C_RODI_COMPANYID_2);
sb.append(_FINDER_COLUMN_C_RODI_ROOTOBJECTDEFINITIONID_2);
if (!getDB().isSupportsInlineDistinct()) {
sb.append(
_FILTER_SQL_SELECT_OBJECTDEFINITION_NO_INLINE_DISTINCT_WHERE_2);
}
if (orderByComparator != null) {
if (getDB().isSupportsInlineDistinct()) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator, true);
}
else {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_TABLE, orderByComparator, true);
}
}
else {
if (getDB().isSupportsInlineDistinct()) {
sb.append(
ObjectDefinitionModelImpl.ORDER_BY_SQL_INLINE_DISTINCT);
}
else {
sb.append(ObjectDefinitionModelImpl.ORDER_BY_SQL);
}
}
String sql = InlineSQLHelperUtil.replacePermissionCheck(
sb.toString(), ObjectDefinition.class.getName(),
_FILTER_ENTITY_TABLE_FILTER_PK_COLUMN);
Session session = null;
try {
session = openSession();
SQLQuery sqlQuery = session.createSynchronizedSQLQuery(sql);
if (getDB().isSupportsInlineDistinct()) {
sqlQuery.addEntity(
_FILTER_ENTITY_ALIAS, ObjectDefinitionImpl.class);
}
else {
sqlQuery.addEntity(
_FILTER_ENTITY_TABLE, ObjectDefinitionImpl.class);
}
QueryPos queryPos = QueryPos.getInstance(sqlQuery);
queryPos.add(companyId);
queryPos.add(rootObjectDefinitionId);
return (List)QueryUtil.list(
sqlQuery, getDialect(), start, end);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
/**
* Returns the object definitions before and after the current object definition in the ordered set of object definitions that the user has permission to view where companyId = ? and rootObjectDefinitionId = ?.
*
* @param objectDefinitionId the primary key of the current object definition
* @param companyId the company ID
* @param rootObjectDefinitionId the root object definition ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next object definition
* @throws NoSuchObjectDefinitionException if a object definition with the primary key could not be found
*/
@Override
public ObjectDefinition[] filterFindByC_RODI_PrevAndNext(
long objectDefinitionId, long companyId,
long rootObjectDefinitionId,
OrderByComparator orderByComparator)
throws NoSuchObjectDefinitionException {
if (!InlineSQLHelperUtil.isEnabled(companyId, 0)) {
return findByC_RODI_PrevAndNext(
objectDefinitionId, companyId, rootObjectDefinitionId,
orderByComparator);
}
ObjectDefinition objectDefinition = findByPrimaryKey(
objectDefinitionId);
Session session = null;
try {
session = openSession();
ObjectDefinition[] array = new ObjectDefinitionImpl[3];
array[0] = filterGetByC_RODI_PrevAndNext(
session, objectDefinition, companyId, rootObjectDefinitionId,
orderByComparator, true);
array[1] = objectDefinition;
array[2] = filterGetByC_RODI_PrevAndNext(
session, objectDefinition, companyId, rootObjectDefinitionId,
orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected ObjectDefinition filterGetByC_RODI_PrevAndNext(
Session session, ObjectDefinition objectDefinition, long companyId,
long rootObjectDefinitionId,
OrderByComparator orderByComparator,
boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
6 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(5);
}
if (getDB().isSupportsInlineDistinct()) {
sb.append(_FILTER_SQL_SELECT_OBJECTDEFINITION_WHERE);
}
else {
sb.append(
_FILTER_SQL_SELECT_OBJECTDEFINITION_NO_INLINE_DISTINCT_WHERE_1);
}
sb.append(_FINDER_COLUMN_C_RODI_COMPANYID_2);
sb.append(_FINDER_COLUMN_C_RODI_ROOTOBJECTDEFINITIONID_2);
if (!getDB().isSupportsInlineDistinct()) {
sb.append(
_FILTER_SQL_SELECT_OBJECTDEFINITION_NO_INLINE_DISTINCT_WHERE_2);
}
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
if (getDB().isSupportsInlineDistinct()) {
sb.append(
getColumnName(
_ORDER_BY_ENTITY_ALIAS, orderByConditionFields[i],
true));
}
else {
sb.append(
getColumnName(
_ORDER_BY_ENTITY_TABLE, orderByConditionFields[i],
true));
}
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
if (getDB().isSupportsInlineDistinct()) {
sb.append(
getColumnName(
_ORDER_BY_ENTITY_ALIAS, orderByFields[i], true));
}
else {
sb.append(
getColumnName(
_ORDER_BY_ENTITY_TABLE, orderByFields[i], true));
}
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
if (getDB().isSupportsInlineDistinct()) {
sb.append(
ObjectDefinitionModelImpl.ORDER_BY_SQL_INLINE_DISTINCT);
}
else {
sb.append(ObjectDefinitionModelImpl.ORDER_BY_SQL);
}
}
String sql = InlineSQLHelperUtil.replacePermissionCheck(
sb.toString(), ObjectDefinition.class.getName(),
_FILTER_ENTITY_TABLE_FILTER_PK_COLUMN);
SQLQuery sqlQuery = session.createSynchronizedSQLQuery(sql);
sqlQuery.setFirstResult(0);
sqlQuery.setMaxResults(2);
if (getDB().isSupportsInlineDistinct()) {
sqlQuery.addEntity(
_FILTER_ENTITY_ALIAS, ObjectDefinitionImpl.class);
}
else {
sqlQuery.addEntity(
_FILTER_ENTITY_TABLE, ObjectDefinitionImpl.class);
}
QueryPos queryPos = QueryPos.getInstance(sqlQuery);
queryPos.add(companyId);
queryPos.add(rootObjectDefinitionId);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
objectDefinition)) {
queryPos.add(orderByConditionValue);
}
}
List list = sqlQuery.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the object definitions where companyId = ? and rootObjectDefinitionId = ? from the database.
*
* @param companyId the company ID
* @param rootObjectDefinitionId the root object definition ID
*/
@Override
public void removeByC_RODI(long companyId, long rootObjectDefinitionId) {
for (ObjectDefinition objectDefinition :
findByC_RODI(
companyId, rootObjectDefinitionId, QueryUtil.ALL_POS,
QueryUtil.ALL_POS, null)) {
remove(objectDefinition);
}
}
/**
* Returns the number of object definitions where companyId = ? and rootObjectDefinitionId = ?.
*
* @param companyId the company ID
* @param rootObjectDefinitionId the root object definition ID
* @return the number of matching object definitions
*/
@Override
public int countByC_RODI(long companyId, long rootObjectDefinitionId) {
FinderPath finderPath = _finderPathCountByC_RODI;
Object[] finderArgs = new Object[] {companyId, rootObjectDefinitionId};
Long count = (Long)finderCache.getResult(finderPath, finderArgs, this);
if (count == null) {
StringBundler sb = new StringBundler(3);
sb.append(_SQL_COUNT_OBJECTDEFINITION_WHERE);
sb.append(_FINDER_COLUMN_C_RODI_COMPANYID_2);
sb.append(_FINDER_COLUMN_C_RODI_ROOTOBJECTDEFINITIONID_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(companyId);
queryPos.add(rootObjectDefinitionId);
count = (Long)query.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
/**
* Returns the number of object definitions that the user has permission to view where companyId = ? and rootObjectDefinitionId = ?.
*
* @param companyId the company ID
* @param rootObjectDefinitionId the root object definition ID
* @return the number of matching object definitions that the user has permission to view
*/
@Override
public int filterCountByC_RODI(
long companyId, long rootObjectDefinitionId) {
if (!InlineSQLHelperUtil.isEnabled(companyId, 0)) {
return countByC_RODI(companyId, rootObjectDefinitionId);
}
StringBundler sb = new StringBundler(3);
sb.append(_FILTER_SQL_COUNT_OBJECTDEFINITION_WHERE);
sb.append(_FINDER_COLUMN_C_RODI_COMPANYID_2);
sb.append(_FINDER_COLUMN_C_RODI_ROOTOBJECTDEFINITIONID_2);
String sql = InlineSQLHelperUtil.replacePermissionCheck(
sb.toString(), ObjectDefinition.class.getName(),
_FILTER_ENTITY_TABLE_FILTER_PK_COLUMN);
Session session = null;
try {
session = openSession();
SQLQuery sqlQuery = session.createSynchronizedSQLQuery(sql);
sqlQuery.addScalar(
COUNT_COLUMN_NAME, com.liferay.portal.kernel.dao.orm.Type.LONG);
QueryPos queryPos = QueryPos.getInstance(sqlQuery);
queryPos.add(companyId);
queryPos.add(rootObjectDefinitionId);
Long count = (Long)sqlQuery.uniqueResult();
return count.intValue();
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
private static final String _FINDER_COLUMN_C_RODI_COMPANYID_2 =
"objectDefinition.companyId = ? AND ";
private static final String _FINDER_COLUMN_C_RODI_ROOTOBJECTDEFINITIONID_2 =
"objectDefinition.rootObjectDefinitionId = ?";
private FinderPath _finderPathFetchByC_C;
private FinderPath _finderPathCountByC_C;
/**
* Returns the object definition where companyId = ? and className = ? or throws a NoSuchObjectDefinitionException
if it could not be found.
*
* @param companyId the company ID
* @param className the class name
* @return the matching object definition
* @throws NoSuchObjectDefinitionException if a matching object definition could not be found
*/
@Override
public ObjectDefinition findByC_C(long companyId, String className)
throws NoSuchObjectDefinitionException {
ObjectDefinition objectDefinition = fetchByC_C(companyId, className);
if (objectDefinition == null) {
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("companyId=");
sb.append(companyId);
sb.append(", className=");
sb.append(className);
sb.append("}");
if (_log.isDebugEnabled()) {
_log.debug(sb.toString());
}
throw new NoSuchObjectDefinitionException(sb.toString());
}
return objectDefinition;
}
/**
* Returns the object definition where companyId = ? and className = ? or returns null
if it could not be found. Uses the finder cache.
*
* @param companyId the company ID
* @param className the class name
* @return the matching object definition, or null
if a matching object definition could not be found
*/
@Override
public ObjectDefinition fetchByC_C(long companyId, String className) {
return fetchByC_C(companyId, className, true);
}
/**
* Returns the object definition where companyId = ? and className = ? or returns null
if it could not be found, optionally using the finder cache.
*
* @param companyId the company ID
* @param className the class name
* @param useFinderCache whether to use the finder cache
* @return the matching object definition, or null
if a matching object definition could not be found
*/
@Override
public ObjectDefinition fetchByC_C(
long companyId, String className, boolean useFinderCache) {
className = Objects.toString(className, "");
Object[] finderArgs = null;
if (useFinderCache) {
finderArgs = new Object[] {companyId, className};
}
Object result = null;
if (useFinderCache) {
result = finderCache.getResult(
_finderPathFetchByC_C, finderArgs, this);
}
if (result instanceof ObjectDefinition) {
ObjectDefinition objectDefinition = (ObjectDefinition)result;
if ((companyId != objectDefinition.getCompanyId()) ||
!Objects.equals(className, objectDefinition.getClassName())) {
result = null;
}
}
if (result == null) {
StringBundler sb = new StringBundler(4);
sb.append(_SQL_SELECT_OBJECTDEFINITION_WHERE);
sb.append(_FINDER_COLUMN_C_C_COMPANYID_2);
boolean bindClassName = false;
if (className.isEmpty()) {
sb.append(_FINDER_COLUMN_C_C_CLASSNAME_3);
}
else {
bindClassName = true;
sb.append(_FINDER_COLUMN_C_C_CLASSNAME_2);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(companyId);
if (bindClassName) {
queryPos.add(className);
}
List list = query.list();
if (list.isEmpty()) {
if (useFinderCache) {
finderCache.putResult(
_finderPathFetchByC_C, finderArgs, list);
}
}
else {
if (list.size() > 1) {
Collections.sort(list, Collections.reverseOrder());
if (_log.isWarnEnabled()) {
if (!useFinderCache) {
finderArgs = new Object[] {
companyId, className
};
}
_log.warn(
"ObjectDefinitionPersistenceImpl.fetchByC_C(long, String, boolean) with parameters (" +
StringUtil.merge(finderArgs) +
") yields a result set with more than 1 result. This violates the logical unique restriction. There is no order guarantee on which result is returned by this finder.");
}
}
ObjectDefinition objectDefinition = list.get(0);
result = objectDefinition;
cacheResult(objectDefinition);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
if (result instanceof List>) {
return null;
}
else {
return (ObjectDefinition)result;
}
}
/**
* Removes the object definition where companyId = ? and className = ? from the database.
*
* @param companyId the company ID
* @param className the class name
* @return the object definition that was removed
*/
@Override
public ObjectDefinition removeByC_C(long companyId, String className)
throws NoSuchObjectDefinitionException {
ObjectDefinition objectDefinition = findByC_C(companyId, className);
return remove(objectDefinition);
}
/**
* Returns the number of object definitions where companyId = ? and className = ?.
*
* @param companyId the company ID
* @param className the class name
* @return the number of matching object definitions
*/
@Override
public int countByC_C(long companyId, String className) {
className = Objects.toString(className, "");
FinderPath finderPath = _finderPathCountByC_C;
Object[] finderArgs = new Object[] {companyId, className};
Long count = (Long)finderCache.getResult(finderPath, finderArgs, this);
if (count == null) {
StringBundler sb = new StringBundler(3);
sb.append(_SQL_COUNT_OBJECTDEFINITION_WHERE);
sb.append(_FINDER_COLUMN_C_C_COMPANYID_2);
boolean bindClassName = false;
if (className.isEmpty()) {
sb.append(_FINDER_COLUMN_C_C_CLASSNAME_3);
}
else {
bindClassName = true;
sb.append(_FINDER_COLUMN_C_C_CLASSNAME_2);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(companyId);
if (bindClassName) {
queryPos.add(className);
}
count = (Long)query.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String _FINDER_COLUMN_C_C_COMPANYID_2 =
"objectDefinition.companyId = ? AND ";
private static final String _FINDER_COLUMN_C_C_CLASSNAME_2 =
"objectDefinition.className = ?";
private static final String _FINDER_COLUMN_C_C_CLASSNAME_3 =
"(objectDefinition.className IS NULL OR objectDefinition.className = '')";
private FinderPath _finderPathFetchByC_N;
private FinderPath _finderPathCountByC_N;
/**
* Returns the object definition where companyId = ? and name = ? or throws a NoSuchObjectDefinitionException
if it could not be found.
*
* @param companyId the company ID
* @param name the name
* @return the matching object definition
* @throws NoSuchObjectDefinitionException if a matching object definition could not be found
*/
@Override
public ObjectDefinition findByC_N(long companyId, String name)
throws NoSuchObjectDefinitionException {
ObjectDefinition objectDefinition = fetchByC_N(companyId, name);
if (objectDefinition == null) {
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("companyId=");
sb.append(companyId);
sb.append(", name=");
sb.append(name);
sb.append("}");
if (_log.isDebugEnabled()) {
_log.debug(sb.toString());
}
throw new NoSuchObjectDefinitionException(sb.toString());
}
return objectDefinition;
}
/**
* Returns the object definition where companyId = ? and name = ? or returns null
if it could not be found. Uses the finder cache.
*
* @param companyId the company ID
* @param name the name
* @return the matching object definition, or null
if a matching object definition could not be found
*/
@Override
public ObjectDefinition fetchByC_N(long companyId, String name) {
return fetchByC_N(companyId, name, true);
}
/**
* Returns the object definition where companyId = ? and name = ? or returns null
if it could not be found, optionally using the finder cache.
*
* @param companyId the company ID
* @param name the name
* @param useFinderCache whether to use the finder cache
* @return the matching object definition, or null
if a matching object definition could not be found
*/
@Override
public ObjectDefinition fetchByC_N(
long companyId, String name, boolean useFinderCache) {
name = Objects.toString(name, "");
Object[] finderArgs = null;
if (useFinderCache) {
finderArgs = new Object[] {companyId, name};
}
Object result = null;
if (useFinderCache) {
result = finderCache.getResult(
_finderPathFetchByC_N, finderArgs, this);
}
if (result instanceof ObjectDefinition) {
ObjectDefinition objectDefinition = (ObjectDefinition)result;
if ((companyId != objectDefinition.getCompanyId()) ||
!Objects.equals(name, objectDefinition.getName())) {
result = null;
}
}
if (result == null) {
StringBundler sb = new StringBundler(4);
sb.append(_SQL_SELECT_OBJECTDEFINITION_WHERE);
sb.append(_FINDER_COLUMN_C_N_COMPANYID_2);
boolean bindName = false;
if (name.isEmpty()) {
sb.append(_FINDER_COLUMN_C_N_NAME_3);
}
else {
bindName = true;
sb.append(_FINDER_COLUMN_C_N_NAME_2);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(companyId);
if (bindName) {
queryPos.add(name);
}
List list = query.list();
if (list.isEmpty()) {
if (useFinderCache) {
finderCache.putResult(
_finderPathFetchByC_N, finderArgs, list);
}
}
else {
if (list.size() > 1) {
Collections.sort(list, Collections.reverseOrder());
if (_log.isWarnEnabled()) {
if (!useFinderCache) {
finderArgs = new Object[] {companyId, name};
}
_log.warn(
"ObjectDefinitionPersistenceImpl.fetchByC_N(long, String, boolean) with parameters (" +
StringUtil.merge(finderArgs) +
") yields a result set with more than 1 result. This violates the logical unique restriction. There is no order guarantee on which result is returned by this finder.");
}
}
ObjectDefinition objectDefinition = list.get(0);
result = objectDefinition;
cacheResult(objectDefinition);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
if (result instanceof List>) {
return null;
}
else {
return (ObjectDefinition)result;
}
}
/**
* Removes the object definition where companyId = ? and name = ? from the database.
*
* @param companyId the company ID
* @param name the name
* @return the object definition that was removed
*/
@Override
public ObjectDefinition removeByC_N(long companyId, String name)
throws NoSuchObjectDefinitionException {
ObjectDefinition objectDefinition = findByC_N(companyId, name);
return remove(objectDefinition);
}
/**
* Returns the number of object definitions where companyId = ? and name = ?.
*
* @param companyId the company ID
* @param name the name
* @return the number of matching object definitions
*/
@Override
public int countByC_N(long companyId, String name) {
name = Objects.toString(name, "");
FinderPath finderPath = _finderPathCountByC_N;
Object[] finderArgs = new Object[] {companyId, name};
Long count = (Long)finderCache.getResult(finderPath, finderArgs, this);
if (count == null) {
StringBundler sb = new StringBundler(3);
sb.append(_SQL_COUNT_OBJECTDEFINITION_WHERE);
sb.append(_FINDER_COLUMN_C_N_COMPANYID_2);
boolean bindName = false;
if (name.isEmpty()) {
sb.append(_FINDER_COLUMN_C_N_NAME_3);
}
else {
bindName = true;
sb.append(_FINDER_COLUMN_C_N_NAME_2);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(companyId);
if (bindName) {
queryPos.add(name);
}
count = (Long)query.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String _FINDER_COLUMN_C_N_COMPANYID_2 =
"objectDefinition.companyId = ? AND ";
private static final String _FINDER_COLUMN_C_N_NAME_2 =
"objectDefinition.name = ?";
private static final String _FINDER_COLUMN_C_N_NAME_3 =
"(objectDefinition.name IS NULL OR objectDefinition.name = '')";
private FinderPath _finderPathWithPaginationFindByC_S;
private FinderPath _finderPathWithoutPaginationFindByC_S;
private FinderPath _finderPathCountByC_S;
/**
* Returns all the object definitions where companyId = ? and status = ?.
*
* @param companyId the company ID
* @param status the status
* @return the matching object definitions
*/
@Override
public List findByC_S(long companyId, int status) {
return findByC_S(
companyId, status, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the object definitions where companyId = ? and status = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectDefinitionModelImpl
.
*
*
* @param companyId the company ID
* @param status the status
* @param start the lower bound of the range of object definitions
* @param end the upper bound of the range of object definitions (not inclusive)
* @return the range of matching object definitions
*/
@Override
public List findByC_S(
long companyId, int status, int start, int end) {
return findByC_S(companyId, status, start, end, null);
}
/**
* Returns an ordered range of all the object definitions where companyId = ? and status = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectDefinitionModelImpl
.
*
*
* @param companyId the company ID
* @param status the status
* @param start the lower bound of the range of object definitions
* @param end the upper bound of the range of object definitions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching object definitions
*/
@Override
public List findByC_S(
long companyId, int status, int start, int end,
OrderByComparator orderByComparator) {
return findByC_S(
companyId, status, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the object definitions where companyId = ? and status = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectDefinitionModelImpl
.
*
*
* @param companyId the company ID
* @param status the status
* @param start the lower bound of the range of object definitions
* @param end the upper bound of the range of object definitions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching object definitions
*/
@Override
public List findByC_S(
long companyId, int status, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache) {
finderPath = _finderPathWithoutPaginationFindByC_S;
finderArgs = new Object[] {companyId, status};
}
}
else if (useFinderCache) {
finderPath = _finderPathWithPaginationFindByC_S;
finderArgs = new Object[] {
companyId, status, start, end, orderByComparator
};
}
List list = null;
if (useFinderCache) {
list = (List)finderCache.getResult(
finderPath, finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (ObjectDefinition objectDefinition : list) {
if ((companyId != objectDefinition.getCompanyId()) ||
(status != objectDefinition.getStatus())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_OBJECTDEFINITION_WHERE);
sb.append(_FINDER_COLUMN_C_S_COMPANYID_2);
sb.append(_FINDER_COLUMN_C_S_STATUS_2);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(ObjectDefinitionModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(companyId);
queryPos.add(status);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first object definition in the ordered set where companyId = ? and status = ?.
*
* @param companyId the company ID
* @param status the status
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching object definition
* @throws NoSuchObjectDefinitionException if a matching object definition could not be found
*/
@Override
public ObjectDefinition findByC_S_First(
long companyId, int status,
OrderByComparator orderByComparator)
throws NoSuchObjectDefinitionException {
ObjectDefinition objectDefinition = fetchByC_S_First(
companyId, status, orderByComparator);
if (objectDefinition != null) {
return objectDefinition;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("companyId=");
sb.append(companyId);
sb.append(", status=");
sb.append(status);
sb.append("}");
throw new NoSuchObjectDefinitionException(sb.toString());
}
/**
* Returns the first object definition in the ordered set where companyId = ? and status = ?.
*
* @param companyId the company ID
* @param status the status
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching object definition, or null
if a matching object definition could not be found
*/
@Override
public ObjectDefinition fetchByC_S_First(
long companyId, int status,
OrderByComparator orderByComparator) {
List list = findByC_S(
companyId, status, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last object definition in the ordered set where companyId = ? and status = ?.
*
* @param companyId the company ID
* @param status the status
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching object definition
* @throws NoSuchObjectDefinitionException if a matching object definition could not be found
*/
@Override
public ObjectDefinition findByC_S_Last(
long companyId, int status,
OrderByComparator orderByComparator)
throws NoSuchObjectDefinitionException {
ObjectDefinition objectDefinition = fetchByC_S_Last(
companyId, status, orderByComparator);
if (objectDefinition != null) {
return objectDefinition;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("companyId=");
sb.append(companyId);
sb.append(", status=");
sb.append(status);
sb.append("}");
throw new NoSuchObjectDefinitionException(sb.toString());
}
/**
* Returns the last object definition in the ordered set where companyId = ? and status = ?.
*
* @param companyId the company ID
* @param status the status
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching object definition, or null
if a matching object definition could not be found
*/
@Override
public ObjectDefinition fetchByC_S_Last(
long companyId, int status,
OrderByComparator orderByComparator) {
int count = countByC_S(companyId, status);
if (count == 0) {
return null;
}
List list = findByC_S(
companyId, status, count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the object definitions before and after the current object definition in the ordered set where companyId = ? and status = ?.
*
* @param objectDefinitionId the primary key of the current object definition
* @param companyId the company ID
* @param status the status
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next object definition
* @throws NoSuchObjectDefinitionException if a object definition with the primary key could not be found
*/
@Override
public ObjectDefinition[] findByC_S_PrevAndNext(
long objectDefinitionId, long companyId, int status,
OrderByComparator orderByComparator)
throws NoSuchObjectDefinitionException {
ObjectDefinition objectDefinition = findByPrimaryKey(
objectDefinitionId);
Session session = null;
try {
session = openSession();
ObjectDefinition[] array = new ObjectDefinitionImpl[3];
array[0] = getByC_S_PrevAndNext(
session, objectDefinition, companyId, status, orderByComparator,
true);
array[1] = objectDefinition;
array[2] = getByC_S_PrevAndNext(
session, objectDefinition, companyId, status, orderByComparator,
false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected ObjectDefinition getByC_S_PrevAndNext(
Session session, ObjectDefinition objectDefinition, long companyId,
int status, OrderByComparator orderByComparator,
boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
5 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_OBJECTDEFINITION_WHERE);
sb.append(_FINDER_COLUMN_C_S_COMPANYID_2);
sb.append(_FINDER_COLUMN_C_S_STATUS_2);
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(ObjectDefinitionModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(companyId);
queryPos.add(status);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
objectDefinition)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Returns all the object definitions that the user has permission to view where companyId = ? and status = ?.
*
* @param companyId the company ID
* @param status the status
* @return the matching object definitions that the user has permission to view
*/
@Override
public List filterFindByC_S(long companyId, int status) {
return filterFindByC_S(
companyId, status, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the object definitions that the user has permission to view where companyId = ? and status = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectDefinitionModelImpl
.
*
*
* @param companyId the company ID
* @param status the status
* @param start the lower bound of the range of object definitions
* @param end the upper bound of the range of object definitions (not inclusive)
* @return the range of matching object definitions that the user has permission to view
*/
@Override
public List filterFindByC_S(
long companyId, int status, int start, int end) {
return filterFindByC_S(companyId, status, start, end, null);
}
/**
* Returns an ordered range of all the object definitions that the user has permissions to view where companyId = ? and status = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectDefinitionModelImpl
.
*
*
* @param companyId the company ID
* @param status the status
* @param start the lower bound of the range of object definitions
* @param end the upper bound of the range of object definitions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching object definitions that the user has permission to view
*/
@Override
public List filterFindByC_S(
long companyId, int status, int start, int end,
OrderByComparator orderByComparator) {
if (!InlineSQLHelperUtil.isEnabled(companyId, 0)) {
return findByC_S(companyId, status, start, end, orderByComparator);
}
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(5);
}
if (getDB().isSupportsInlineDistinct()) {
sb.append(_FILTER_SQL_SELECT_OBJECTDEFINITION_WHERE);
}
else {
sb.append(
_FILTER_SQL_SELECT_OBJECTDEFINITION_NO_INLINE_DISTINCT_WHERE_1);
}
sb.append(_FINDER_COLUMN_C_S_COMPANYID_2);
sb.append(_FINDER_COLUMN_C_S_STATUS_2);
if (!getDB().isSupportsInlineDistinct()) {
sb.append(
_FILTER_SQL_SELECT_OBJECTDEFINITION_NO_INLINE_DISTINCT_WHERE_2);
}
if (orderByComparator != null) {
if (getDB().isSupportsInlineDistinct()) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator, true);
}
else {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_TABLE, orderByComparator, true);
}
}
else {
if (getDB().isSupportsInlineDistinct()) {
sb.append(
ObjectDefinitionModelImpl.ORDER_BY_SQL_INLINE_DISTINCT);
}
else {
sb.append(ObjectDefinitionModelImpl.ORDER_BY_SQL);
}
}
String sql = InlineSQLHelperUtil.replacePermissionCheck(
sb.toString(), ObjectDefinition.class.getName(),
_FILTER_ENTITY_TABLE_FILTER_PK_COLUMN);
Session session = null;
try {
session = openSession();
SQLQuery sqlQuery = session.createSynchronizedSQLQuery(sql);
if (getDB().isSupportsInlineDistinct()) {
sqlQuery.addEntity(
_FILTER_ENTITY_ALIAS, ObjectDefinitionImpl.class);
}
else {
sqlQuery.addEntity(
_FILTER_ENTITY_TABLE, ObjectDefinitionImpl.class);
}
QueryPos queryPos = QueryPos.getInstance(sqlQuery);
queryPos.add(companyId);
queryPos.add(status);
return (List)QueryUtil.list(
sqlQuery, getDialect(), start, end);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
/**
* Returns the object definitions before and after the current object definition in the ordered set of object definitions that the user has permission to view where companyId = ? and status = ?.
*
* @param objectDefinitionId the primary key of the current object definition
* @param companyId the company ID
* @param status the status
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next object definition
* @throws NoSuchObjectDefinitionException if a object definition with the primary key could not be found
*/
@Override
public ObjectDefinition[] filterFindByC_S_PrevAndNext(
long objectDefinitionId, long companyId, int status,
OrderByComparator orderByComparator)
throws NoSuchObjectDefinitionException {
if (!InlineSQLHelperUtil.isEnabled(companyId, 0)) {
return findByC_S_PrevAndNext(
objectDefinitionId, companyId, status, orderByComparator);
}
ObjectDefinition objectDefinition = findByPrimaryKey(
objectDefinitionId);
Session session = null;
try {
session = openSession();
ObjectDefinition[] array = new ObjectDefinitionImpl[3];
array[0] = filterGetByC_S_PrevAndNext(
session, objectDefinition, companyId, status, orderByComparator,
true);
array[1] = objectDefinition;
array[2] = filterGetByC_S_PrevAndNext(
session, objectDefinition, companyId, status, orderByComparator,
false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected ObjectDefinition filterGetByC_S_PrevAndNext(
Session session, ObjectDefinition objectDefinition, long companyId,
int status, OrderByComparator orderByComparator,
boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
6 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(5);
}
if (getDB().isSupportsInlineDistinct()) {
sb.append(_FILTER_SQL_SELECT_OBJECTDEFINITION_WHERE);
}
else {
sb.append(
_FILTER_SQL_SELECT_OBJECTDEFINITION_NO_INLINE_DISTINCT_WHERE_1);
}
sb.append(_FINDER_COLUMN_C_S_COMPANYID_2);
sb.append(_FINDER_COLUMN_C_S_STATUS_2);
if (!getDB().isSupportsInlineDistinct()) {
sb.append(
_FILTER_SQL_SELECT_OBJECTDEFINITION_NO_INLINE_DISTINCT_WHERE_2);
}
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
if (getDB().isSupportsInlineDistinct()) {
sb.append(
getColumnName(
_ORDER_BY_ENTITY_ALIAS, orderByConditionFields[i],
true));
}
else {
sb.append(
getColumnName(
_ORDER_BY_ENTITY_TABLE, orderByConditionFields[i],
true));
}
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
if (getDB().isSupportsInlineDistinct()) {
sb.append(
getColumnName(
_ORDER_BY_ENTITY_ALIAS, orderByFields[i], true));
}
else {
sb.append(
getColumnName(
_ORDER_BY_ENTITY_TABLE, orderByFields[i], true));
}
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
if (getDB().isSupportsInlineDistinct()) {
sb.append(
ObjectDefinitionModelImpl.ORDER_BY_SQL_INLINE_DISTINCT);
}
else {
sb.append(ObjectDefinitionModelImpl.ORDER_BY_SQL);
}
}
String sql = InlineSQLHelperUtil.replacePermissionCheck(
sb.toString(), ObjectDefinition.class.getName(),
_FILTER_ENTITY_TABLE_FILTER_PK_COLUMN);
SQLQuery sqlQuery = session.createSynchronizedSQLQuery(sql);
sqlQuery.setFirstResult(0);
sqlQuery.setMaxResults(2);
if (getDB().isSupportsInlineDistinct()) {
sqlQuery.addEntity(
_FILTER_ENTITY_ALIAS, ObjectDefinitionImpl.class);
}
else {
sqlQuery.addEntity(
_FILTER_ENTITY_TABLE, ObjectDefinitionImpl.class);
}
QueryPos queryPos = QueryPos.getInstance(sqlQuery);
queryPos.add(companyId);
queryPos.add(status);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
objectDefinition)) {
queryPos.add(orderByConditionValue);
}
}
List list = sqlQuery.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the object definitions where companyId = ? and status = ? from the database.
*
* @param companyId the company ID
* @param status the status
*/
@Override
public void removeByC_S(long companyId, int status) {
for (ObjectDefinition objectDefinition :
findByC_S(
companyId, status, QueryUtil.ALL_POS, QueryUtil.ALL_POS,
null)) {
remove(objectDefinition);
}
}
/**
* Returns the number of object definitions where companyId = ? and status = ?.
*
* @param companyId the company ID
* @param status the status
* @return the number of matching object definitions
*/
@Override
public int countByC_S(long companyId, int status) {
FinderPath finderPath = _finderPathCountByC_S;
Object[] finderArgs = new Object[] {companyId, status};
Long count = (Long)finderCache.getResult(finderPath, finderArgs, this);
if (count == null) {
StringBundler sb = new StringBundler(3);
sb.append(_SQL_COUNT_OBJECTDEFINITION_WHERE);
sb.append(_FINDER_COLUMN_C_S_COMPANYID_2);
sb.append(_FINDER_COLUMN_C_S_STATUS_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(companyId);
queryPos.add(status);
count = (Long)query.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
/**
* Returns the number of object definitions that the user has permission to view where companyId = ? and status = ?.
*
* @param companyId the company ID
* @param status the status
* @return the number of matching object definitions that the user has permission to view
*/
@Override
public int filterCountByC_S(long companyId, int status) {
if (!InlineSQLHelperUtil.isEnabled(companyId, 0)) {
return countByC_S(companyId, status);
}
StringBundler sb = new StringBundler(3);
sb.append(_FILTER_SQL_COUNT_OBJECTDEFINITION_WHERE);
sb.append(_FINDER_COLUMN_C_S_COMPANYID_2);
sb.append(_FINDER_COLUMN_C_S_STATUS_2);
String sql = InlineSQLHelperUtil.replacePermissionCheck(
sb.toString(), ObjectDefinition.class.getName(),
_FILTER_ENTITY_TABLE_FILTER_PK_COLUMN);
Session session = null;
try {
session = openSession();
SQLQuery sqlQuery = session.createSynchronizedSQLQuery(sql);
sqlQuery.addScalar(
COUNT_COLUMN_NAME, com.liferay.portal.kernel.dao.orm.Type.LONG);
QueryPos queryPos = QueryPos.getInstance(sqlQuery);
queryPos.add(companyId);
queryPos.add(status);
Long count = (Long)sqlQuery.uniqueResult();
return count.intValue();
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
private static final String _FINDER_COLUMN_C_S_COMPANYID_2 =
"objectDefinition.companyId = ? AND ";
private static final String _FINDER_COLUMN_C_S_STATUS_2 =
"objectDefinition.status = ?";
private FinderPath _finderPathWithPaginationFindByS_S;
private FinderPath _finderPathWithoutPaginationFindByS_S;
private FinderPath _finderPathCountByS_S;
/**
* Returns all the object definitions where system = ? and status = ?.
*
* @param system the system
* @param status the status
* @return the matching object definitions
*/
@Override
public List findByS_S(boolean system, int status) {
return findByS_S(
system, status, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the object definitions where system = ? and status = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectDefinitionModelImpl
.
*
*
* @param system the system
* @param status the status
* @param start the lower bound of the range of object definitions
* @param end the upper bound of the range of object definitions (not inclusive)
* @return the range of matching object definitions
*/
@Override
public List findByS_S(
boolean system, int status, int start, int end) {
return findByS_S(system, status, start, end, null);
}
/**
* Returns an ordered range of all the object definitions where system = ? and status = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectDefinitionModelImpl
.
*
*
* @param system the system
* @param status the status
* @param start the lower bound of the range of object definitions
* @param end the upper bound of the range of object definitions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching object definitions
*/
@Override
public List findByS_S(
boolean system, int status, int start, int end,
OrderByComparator orderByComparator) {
return findByS_S(system, status, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the object definitions where system = ? and status = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectDefinitionModelImpl
.
*
*
* @param system the system
* @param status the status
* @param start the lower bound of the range of object definitions
* @param end the upper bound of the range of object definitions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching object definitions
*/
@Override
public List findByS_S(
boolean system, int status, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache) {
finderPath = _finderPathWithoutPaginationFindByS_S;
finderArgs = new Object[] {system, status};
}
}
else if (useFinderCache) {
finderPath = _finderPathWithPaginationFindByS_S;
finderArgs = new Object[] {
system, status, start, end, orderByComparator
};
}
List list = null;
if (useFinderCache) {
list = (List)finderCache.getResult(
finderPath, finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (ObjectDefinition objectDefinition : list) {
if ((system != objectDefinition.isSystem()) ||
(status != objectDefinition.getStatus())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_OBJECTDEFINITION_WHERE);
sb.append(_FINDER_COLUMN_S_S_SYSTEM_2);
sb.append(_FINDER_COLUMN_S_S_STATUS_2);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(ObjectDefinitionModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(system);
queryPos.add(status);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first object definition in the ordered set where system = ? and status = ?.
*
* @param system the system
* @param status the status
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching object definition
* @throws NoSuchObjectDefinitionException if a matching object definition could not be found
*/
@Override
public ObjectDefinition findByS_S_First(
boolean system, int status,
OrderByComparator orderByComparator)
throws NoSuchObjectDefinitionException {
ObjectDefinition objectDefinition = fetchByS_S_First(
system, status, orderByComparator);
if (objectDefinition != null) {
return objectDefinition;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("system=");
sb.append(system);
sb.append(", status=");
sb.append(status);
sb.append("}");
throw new NoSuchObjectDefinitionException(sb.toString());
}
/**
* Returns the first object definition in the ordered set where system = ? and status = ?.
*
* @param system the system
* @param status the status
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching object definition, or null
if a matching object definition could not be found
*/
@Override
public ObjectDefinition fetchByS_S_First(
boolean system, int status,
OrderByComparator orderByComparator) {
List list = findByS_S(
system, status, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last object definition in the ordered set where system = ? and status = ?.
*
* @param system the system
* @param status the status
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching object definition
* @throws NoSuchObjectDefinitionException if a matching object definition could not be found
*/
@Override
public ObjectDefinition findByS_S_Last(
boolean system, int status,
OrderByComparator orderByComparator)
throws NoSuchObjectDefinitionException {
ObjectDefinition objectDefinition = fetchByS_S_Last(
system, status, orderByComparator);
if (objectDefinition != null) {
return objectDefinition;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("system=");
sb.append(system);
sb.append(", status=");
sb.append(status);
sb.append("}");
throw new NoSuchObjectDefinitionException(sb.toString());
}
/**
* Returns the last object definition in the ordered set where system = ? and status = ?.
*
* @param system the system
* @param status the status
* @param orderByComparator the comparator to order the set by (optionally