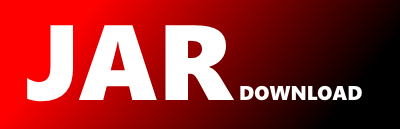
com.liferay.object.service.base.ObjectDefinitionLocalServiceBaseImpl Maven / Gradle / Ivy
/**
* SPDX-FileCopyrightText: (c) 2000 Liferay, Inc. https://liferay.com
* SPDX-License-Identifier: LGPL-2.1-or-later OR LicenseRef-Liferay-DXP-EULA-2.0.0-2023-06
*/
package com.liferay.object.service.base;
import com.liferay.exportimport.kernel.lar.ExportImportHelperUtil;
import com.liferay.exportimport.kernel.lar.ManifestSummary;
import com.liferay.exportimport.kernel.lar.PortletDataContext;
import com.liferay.exportimport.kernel.lar.StagedModelDataHandlerUtil;
import com.liferay.exportimport.kernel.lar.StagedModelType;
import com.liferay.object.model.ObjectDefinition;
import com.liferay.object.service.ObjectDefinitionLocalService;
import com.liferay.object.service.persistence.ObjectDefinitionPersistence;
import com.liferay.petra.sql.dsl.query.DSLQuery;
import com.liferay.portal.aop.AopService;
import com.liferay.portal.kernel.dao.db.DB;
import com.liferay.portal.kernel.dao.db.DBManagerUtil;
import com.liferay.portal.kernel.dao.jdbc.SqlUpdate;
import com.liferay.portal.kernel.dao.jdbc.SqlUpdateFactoryUtil;
import com.liferay.portal.kernel.dao.orm.ActionableDynamicQuery;
import com.liferay.portal.kernel.dao.orm.DefaultActionableDynamicQuery;
import com.liferay.portal.kernel.dao.orm.DynamicQuery;
import com.liferay.portal.kernel.dao.orm.DynamicQueryFactoryUtil;
import com.liferay.portal.kernel.dao.orm.ExportActionableDynamicQuery;
import com.liferay.portal.kernel.dao.orm.IndexableActionableDynamicQuery;
import com.liferay.portal.kernel.dao.orm.Projection;
import com.liferay.portal.kernel.exception.PortalException;
import com.liferay.portal.kernel.exception.SystemException;
import com.liferay.portal.kernel.log.Log;
import com.liferay.portal.kernel.log.LogFactoryUtil;
import com.liferay.portal.kernel.model.PersistedModel;
import com.liferay.portal.kernel.module.framework.service.IdentifiableOSGiService;
import com.liferay.portal.kernel.search.Indexable;
import com.liferay.portal.kernel.search.IndexableType;
import com.liferay.portal.kernel.service.BaseLocalServiceImpl;
import com.liferay.portal.kernel.service.PersistedModelLocalService;
import com.liferay.portal.kernel.service.persistence.BasePersistence;
import com.liferay.portal.kernel.transaction.Transactional;
import com.liferay.portal.kernel.util.OrderByComparator;
import com.liferay.portal.kernel.util.PortalUtil;
import java.io.Serializable;
import java.util.List;
import javax.sql.DataSource;
import org.osgi.service.component.annotations.Deactivate;
import org.osgi.service.component.annotations.Reference;
/**
* Provides the base implementation for the object definition local service.
*
*
* This implementation exists only as a container for the default service methods generated by ServiceBuilder. All custom service methods should be put in {@link com.liferay.object.service.impl.ObjectDefinitionLocalServiceImpl}.
*
*
* @author Marco Leo
* @see com.liferay.object.service.impl.ObjectDefinitionLocalServiceImpl
* @generated
*/
public abstract class ObjectDefinitionLocalServiceBaseImpl
extends BaseLocalServiceImpl
implements AopService, IdentifiableOSGiService,
ObjectDefinitionLocalService {
/*
* NOTE FOR DEVELOPERS:
*
* Never modify or reference this class directly. Use ObjectDefinitionLocalService
via injection or a org.osgi.util.tracker.ServiceTracker
or use com.liferay.object.service.ObjectDefinitionLocalServiceUtil
.
*/
/**
* Adds the object definition to the database. Also notifies the appropriate model listeners.
*
*
* Important: Inspect ObjectDefinitionLocalServiceImpl for overloaded versions of the method. If provided, use these entry points to the API, as the implementation logic may require the additional parameters defined there.
*
*
* @param objectDefinition the object definition
* @return the object definition that was added
*/
@Indexable(type = IndexableType.REINDEX)
@Override
public ObjectDefinition addObjectDefinition(
ObjectDefinition objectDefinition) {
objectDefinition.setNew(true);
return objectDefinitionPersistence.update(objectDefinition);
}
/**
* Creates a new object definition with the primary key. Does not add the object definition to the database.
*
* @param objectDefinitionId the primary key for the new object definition
* @return the new object definition
*/
@Override
@Transactional(enabled = false)
public ObjectDefinition createObjectDefinition(long objectDefinitionId) {
return objectDefinitionPersistence.create(objectDefinitionId);
}
/**
* Deletes the object definition with the primary key from the database. Also notifies the appropriate model listeners.
*
*
* Important: Inspect ObjectDefinitionLocalServiceImpl for overloaded versions of the method. If provided, use these entry points to the API, as the implementation logic may require the additional parameters defined there.
*
*
* @param objectDefinitionId the primary key of the object definition
* @return the object definition that was removed
* @throws PortalException if a object definition with the primary key could not be found
*/
@Indexable(type = IndexableType.DELETE)
@Override
public ObjectDefinition deleteObjectDefinition(long objectDefinitionId)
throws PortalException {
return objectDefinitionPersistence.remove(objectDefinitionId);
}
/**
* Deletes the object definition from the database. Also notifies the appropriate model listeners.
*
*
* Important: Inspect ObjectDefinitionLocalServiceImpl for overloaded versions of the method. If provided, use these entry points to the API, as the implementation logic may require the additional parameters defined there.
*
*
* @param objectDefinition the object definition
* @return the object definition that was removed
* @throws PortalException
*/
@Indexable(type = IndexableType.DELETE)
@Override
public ObjectDefinition deleteObjectDefinition(
ObjectDefinition objectDefinition)
throws PortalException {
return objectDefinitionPersistence.remove(objectDefinition);
}
@Override
public T dslQuery(DSLQuery dslQuery) {
return objectDefinitionPersistence.dslQuery(dslQuery);
}
@Override
public int dslQueryCount(DSLQuery dslQuery) {
Long count = dslQuery(dslQuery);
return count.intValue();
}
@Override
public DynamicQuery dynamicQuery() {
Class> clazz = getClass();
return DynamicQueryFactoryUtil.forClass(
ObjectDefinition.class, clazz.getClassLoader());
}
/**
* Performs a dynamic query on the database and returns the matching rows.
*
* @param dynamicQuery the dynamic query
* @return the matching rows
*/
@Override
public List dynamicQuery(DynamicQuery dynamicQuery) {
return objectDefinitionPersistence.findWithDynamicQuery(dynamicQuery);
}
/**
* Performs a dynamic query on the database and returns a range of the matching rows.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to com.liferay.portal.kernel.dao.orm.QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from com.liferay.object.model.impl.ObjectDefinitionModelImpl
.
*
*
* @param dynamicQuery the dynamic query
* @param start the lower bound of the range of model instances
* @param end the upper bound of the range of model instances (not inclusive)
* @return the range of matching rows
*/
@Override
public List dynamicQuery(
DynamicQuery dynamicQuery, int start, int end) {
return objectDefinitionPersistence.findWithDynamicQuery(
dynamicQuery, start, end);
}
/**
* Performs a dynamic query on the database and returns an ordered range of the matching rows.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to com.liferay.portal.kernel.dao.orm.QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from com.liferay.object.model.impl.ObjectDefinitionModelImpl
.
*
*
* @param dynamicQuery the dynamic query
* @param start the lower bound of the range of model instances
* @param end the upper bound of the range of model instances (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching rows
*/
@Override
public List dynamicQuery(
DynamicQuery dynamicQuery, int start, int end,
OrderByComparator orderByComparator) {
return objectDefinitionPersistence.findWithDynamicQuery(
dynamicQuery, start, end, orderByComparator);
}
/**
* Returns the number of rows matching the dynamic query.
*
* @param dynamicQuery the dynamic query
* @return the number of rows matching the dynamic query
*/
@Override
public long dynamicQueryCount(DynamicQuery dynamicQuery) {
return objectDefinitionPersistence.countWithDynamicQuery(dynamicQuery);
}
/**
* Returns the number of rows matching the dynamic query.
*
* @param dynamicQuery the dynamic query
* @param projection the projection to apply to the query
* @return the number of rows matching the dynamic query
*/
@Override
public long dynamicQueryCount(
DynamicQuery dynamicQuery, Projection projection) {
return objectDefinitionPersistence.countWithDynamicQuery(
dynamicQuery, projection);
}
@Override
public ObjectDefinition fetchObjectDefinition(long objectDefinitionId) {
return objectDefinitionPersistence.fetchByPrimaryKey(
objectDefinitionId);
}
/**
* Returns the object definition with the matching UUID and company.
*
* @param uuid the object definition's UUID
* @param companyId the primary key of the company
* @return the matching object definition, or null
if a matching object definition could not be found
*/
@Override
public ObjectDefinition fetchObjectDefinitionByUuidAndCompanyId(
String uuid, long companyId) {
return objectDefinitionPersistence.fetchByUuid_C_First(
uuid, companyId, null);
}
@Override
public ObjectDefinition fetchObjectDefinitionByExternalReferenceCode(
String externalReferenceCode, long companyId) {
return objectDefinitionPersistence.fetchByERC_C(
externalReferenceCode, companyId);
}
@Override
public ObjectDefinition getObjectDefinitionByExternalReferenceCode(
String externalReferenceCode, long companyId)
throws PortalException {
return objectDefinitionPersistence.findByERC_C(
externalReferenceCode, companyId);
}
/**
* Returns the object definition with the primary key.
*
* @param objectDefinitionId the primary key of the object definition
* @return the object definition
* @throws PortalException if a object definition with the primary key could not be found
*/
@Override
public ObjectDefinition getObjectDefinition(long objectDefinitionId)
throws PortalException {
return objectDefinitionPersistence.findByPrimaryKey(objectDefinitionId);
}
@Override
public ActionableDynamicQuery getActionableDynamicQuery() {
ActionableDynamicQuery actionableDynamicQuery =
new DefaultActionableDynamicQuery();
actionableDynamicQuery.setBaseLocalService(
objectDefinitionLocalService);
actionableDynamicQuery.setClassLoader(getClassLoader());
actionableDynamicQuery.setModelClass(ObjectDefinition.class);
actionableDynamicQuery.setPrimaryKeyPropertyName("objectDefinitionId");
return actionableDynamicQuery;
}
@Override
public IndexableActionableDynamicQuery
getIndexableActionableDynamicQuery() {
IndexableActionableDynamicQuery indexableActionableDynamicQuery =
new IndexableActionableDynamicQuery();
indexableActionableDynamicQuery.setBaseLocalService(
objectDefinitionLocalService);
indexableActionableDynamicQuery.setClassLoader(getClassLoader());
indexableActionableDynamicQuery.setModelClass(ObjectDefinition.class);
indexableActionableDynamicQuery.setPrimaryKeyPropertyName(
"objectDefinitionId");
return indexableActionableDynamicQuery;
}
protected void initActionableDynamicQuery(
ActionableDynamicQuery actionableDynamicQuery) {
actionableDynamicQuery.setBaseLocalService(
objectDefinitionLocalService);
actionableDynamicQuery.setClassLoader(getClassLoader());
actionableDynamicQuery.setModelClass(ObjectDefinition.class);
actionableDynamicQuery.setPrimaryKeyPropertyName("objectDefinitionId");
}
@Override
public ExportActionableDynamicQuery getExportActionableDynamicQuery(
final PortletDataContext portletDataContext) {
final ExportActionableDynamicQuery exportActionableDynamicQuery =
new ExportActionableDynamicQuery() {
@Override
public long performCount() throws PortalException {
ManifestSummary manifestSummary =
portletDataContext.getManifestSummary();
StagedModelType stagedModelType = getStagedModelType();
long modelAdditionCount = super.performCount();
manifestSummary.addModelAdditionCount(
stagedModelType, modelAdditionCount);
long modelDeletionCount =
ExportImportHelperUtil.getModelDeletionCount(
portletDataContext, stagedModelType);
manifestSummary.addModelDeletionCount(
stagedModelType, modelDeletionCount);
return modelAdditionCount;
}
};
initActionableDynamicQuery(exportActionableDynamicQuery);
exportActionableDynamicQuery.setAddCriteriaMethod(
new ActionableDynamicQuery.AddCriteriaMethod() {
@Override
public void addCriteria(DynamicQuery dynamicQuery) {
portletDataContext.addDateRangeCriteria(
dynamicQuery, "modifiedDate");
}
});
exportActionableDynamicQuery.setCompanyId(
portletDataContext.getCompanyId());
exportActionableDynamicQuery.setPerformActionMethod(
new ActionableDynamicQuery.PerformActionMethod() {
@Override
public void performAction(ObjectDefinition objectDefinition)
throws PortalException {
StagedModelDataHandlerUtil.exportStagedModel(
portletDataContext, objectDefinition);
}
});
exportActionableDynamicQuery.setStagedModelType(
new StagedModelType(
PortalUtil.getClassNameId(ObjectDefinition.class.getName())));
return exportActionableDynamicQuery;
}
/**
* @throws PortalException
*/
@Override
public PersistedModel createPersistedModel(Serializable primaryKeyObj)
throws PortalException {
return objectDefinitionPersistence.create(
((Long)primaryKeyObj).longValue());
}
/**
* @throws PortalException
*/
@Override
public PersistedModel deletePersistedModel(PersistedModel persistedModel)
throws PortalException {
if (_log.isWarnEnabled()) {
_log.warn(
"Implement ObjectDefinitionLocalServiceImpl#deleteObjectDefinition(ObjectDefinition) to avoid orphaned data");
}
return objectDefinitionLocalService.deleteObjectDefinition(
(ObjectDefinition)persistedModel);
}
@Override
public BasePersistence getBasePersistence() {
return objectDefinitionPersistence;
}
/**
* @throws PortalException
*/
@Override
public PersistedModel getPersistedModel(Serializable primaryKeyObj)
throws PortalException {
return objectDefinitionPersistence.findByPrimaryKey(primaryKeyObj);
}
/**
* Returns the object definition with the matching UUID and company.
*
* @param uuid the object definition's UUID
* @param companyId the primary key of the company
* @return the matching object definition
* @throws PortalException if a matching object definition could not be found
*/
@Override
public ObjectDefinition getObjectDefinitionByUuidAndCompanyId(
String uuid, long companyId)
throws PortalException {
return objectDefinitionPersistence.findByUuid_C_First(
uuid, companyId, null);
}
/**
* Returns a range of all the object definitions.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to com.liferay.portal.kernel.dao.orm.QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from com.liferay.object.model.impl.ObjectDefinitionModelImpl
.
*
*
* @param start the lower bound of the range of object definitions
* @param end the upper bound of the range of object definitions (not inclusive)
* @return the range of object definitions
*/
@Override
public List getObjectDefinitions(int start, int end) {
return objectDefinitionPersistence.findAll(start, end);
}
/**
* Returns the number of object definitions.
*
* @return the number of object definitions
*/
@Override
public int getObjectDefinitionsCount() {
return objectDefinitionPersistence.countAll();
}
/**
* Updates the object definition in the database or adds it if it does not yet exist. Also notifies the appropriate model listeners.
*
*
* Important: Inspect ObjectDefinitionLocalServiceImpl for overloaded versions of the method. If provided, use these entry points to the API, as the implementation logic may require the additional parameters defined there.
*
*
* @param objectDefinition the object definition
* @return the object definition that was updated
*/
@Indexable(type = IndexableType.REINDEX)
@Override
public ObjectDefinition updateObjectDefinition(
ObjectDefinition objectDefinition) {
return objectDefinitionPersistence.update(objectDefinition);
}
@Deactivate
protected void deactivate() {
}
@Override
public Class>[] getAopInterfaces() {
return new Class>[] {
ObjectDefinitionLocalService.class, IdentifiableOSGiService.class,
PersistedModelLocalService.class
};
}
@Override
public void setAopProxy(Object aopProxy) {
objectDefinitionLocalService = (ObjectDefinitionLocalService)aopProxy;
}
/**
* Returns the OSGi service identifier.
*
* @return the OSGi service identifier
*/
@Override
public String getOSGiServiceIdentifier() {
return ObjectDefinitionLocalService.class.getName();
}
protected Class> getModelClass() {
return ObjectDefinition.class;
}
protected String getModelClassName() {
return ObjectDefinition.class.getName();
}
/**
* Performs a SQL query.
*
* @param sql the sql query
*/
protected void runSQL(String sql) {
try {
DataSource dataSource = objectDefinitionPersistence.getDataSource();
DB db = DBManagerUtil.getDB();
sql = db.buildSQL(sql);
sql = PortalUtil.transformSQL(sql);
SqlUpdate sqlUpdate = SqlUpdateFactoryUtil.getSqlUpdate(
dataSource, sql);
sqlUpdate.update();
}
catch (Exception exception) {
throw new SystemException(exception);
}
}
protected ObjectDefinitionLocalService objectDefinitionLocalService;
@Reference
protected ObjectDefinitionPersistence objectDefinitionPersistence;
@Reference
protected com.liferay.counter.kernel.service.CounterLocalService
counterLocalService;
private static final Log _log = LogFactoryUtil.getLog(
ObjectDefinitionLocalServiceBaseImpl.class);
}