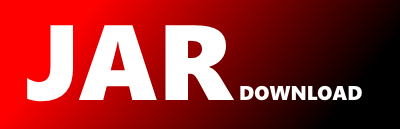
com.liferay.object.service.http.ObjectEntryServiceHttp Maven / Gradle / Ivy
/**
* SPDX-FileCopyrightText: (c) 2000 Liferay, Inc. https://liferay.com
* SPDX-License-Identifier: LGPL-2.1-or-later OR LicenseRef-Liferay-DXP-EULA-2.0.0-2023-06
*/
package com.liferay.object.service.http;
import com.liferay.object.service.ObjectEntryServiceUtil;
import com.liferay.portal.kernel.log.Log;
import com.liferay.portal.kernel.log.LogFactoryUtil;
import com.liferay.portal.kernel.security.auth.HttpPrincipal;
import com.liferay.portal.kernel.service.http.TunnelUtil;
import com.liferay.portal.kernel.util.MethodHandler;
import com.liferay.portal.kernel.util.MethodKey;
/**
* Provides the HTTP utility for the
* ObjectEntryServiceUtil
service
* utility. The
* static methods of this class calls the same methods of the service utility.
* However, the signatures are different because it requires an additional
* HttpPrincipal
parameter.
*
*
* The benefits of using the HTTP utility is that it is fast and allows for
* tunneling without the cost of serializing to text. The drawback is that it
* only works with Java.
*
*
*
* Set the property tunnel.servlet.hosts.allowed in portal.properties to
* configure security.
*
*
*
* The HTTP utility is only generated for remote services.
*
*
* @author Marco Leo
* @generated
*/
public class ObjectEntryServiceHttp {
public static com.liferay.object.model.ObjectEntry addObjectEntry(
HttpPrincipal httpPrincipal, long groupId, long objectDefinitionId,
java.util.Map values,
com.liferay.portal.kernel.service.ServiceContext serviceContext)
throws com.liferay.portal.kernel.exception.PortalException {
try {
MethodKey methodKey = new MethodKey(
ObjectEntryServiceUtil.class, "addObjectEntry",
_addObjectEntryParameterTypes0);
MethodHandler methodHandler = new MethodHandler(
methodKey, groupId, objectDefinitionId, values, serviceContext);
Object returnObj = null;
try {
returnObj = TunnelUtil.invoke(httpPrincipal, methodHandler);
}
catch (Exception exception) {
if (exception instanceof
com.liferay.portal.kernel.exception.PortalException) {
throw (com.liferay.portal.kernel.exception.PortalException)
exception;
}
throw new com.liferay.portal.kernel.exception.SystemException(
exception);
}
return (com.liferay.object.model.ObjectEntry)returnObj;
}
catch (com.liferay.portal.kernel.exception.SystemException
systemException) {
_log.error(systemException, systemException);
throw systemException;
}
}
public static com.liferay.object.model.ObjectEntry addOrUpdateObjectEntry(
HttpPrincipal httpPrincipal, String externalReferenceCode,
long groupId, long objectDefinitionId,
java.util.Map values,
com.liferay.portal.kernel.service.ServiceContext serviceContext)
throws com.liferay.portal.kernel.exception.PortalException {
try {
MethodKey methodKey = new MethodKey(
ObjectEntryServiceUtil.class, "addOrUpdateObjectEntry",
_addOrUpdateObjectEntryParameterTypes1);
MethodHandler methodHandler = new MethodHandler(
methodKey, externalReferenceCode, groupId, objectDefinitionId,
values, serviceContext);
Object returnObj = null;
try {
returnObj = TunnelUtil.invoke(httpPrincipal, methodHandler);
}
catch (Exception exception) {
if (exception instanceof
com.liferay.portal.kernel.exception.PortalException) {
throw (com.liferay.portal.kernel.exception.PortalException)
exception;
}
throw new com.liferay.portal.kernel.exception.SystemException(
exception);
}
return (com.liferay.object.model.ObjectEntry)returnObj;
}
catch (com.liferay.portal.kernel.exception.SystemException
systemException) {
_log.error(systemException, systemException);
throw systemException;
}
}
public static void checkModelResourcePermission(
HttpPrincipal httpPrincipal, long objectDefinitionId,
long objectEntryId, String actionId)
throws com.liferay.portal.kernel.exception.PortalException {
try {
MethodKey methodKey = new MethodKey(
ObjectEntryServiceUtil.class, "checkModelResourcePermission",
_checkModelResourcePermissionParameterTypes2);
MethodHandler methodHandler = new MethodHandler(
methodKey, objectDefinitionId, objectEntryId, actionId);
try {
TunnelUtil.invoke(httpPrincipal, methodHandler);
}
catch (Exception exception) {
if (exception instanceof
com.liferay.portal.kernel.exception.PortalException) {
throw (com.liferay.portal.kernel.exception.PortalException)
exception;
}
throw new com.liferay.portal.kernel.exception.SystemException(
exception);
}
}
catch (com.liferay.portal.kernel.exception.SystemException
systemException) {
_log.error(systemException, systemException);
throw systemException;
}
}
public static com.liferay.object.model.ObjectEntry deleteObjectEntry(
HttpPrincipal httpPrincipal, long objectEntryId)
throws com.liferay.portal.kernel.exception.PortalException {
try {
MethodKey methodKey = new MethodKey(
ObjectEntryServiceUtil.class, "deleteObjectEntry",
_deleteObjectEntryParameterTypes3);
MethodHandler methodHandler = new MethodHandler(
methodKey, objectEntryId);
Object returnObj = null;
try {
returnObj = TunnelUtil.invoke(httpPrincipal, methodHandler);
}
catch (Exception exception) {
if (exception instanceof
com.liferay.portal.kernel.exception.PortalException) {
throw (com.liferay.portal.kernel.exception.PortalException)
exception;
}
throw new com.liferay.portal.kernel.exception.SystemException(
exception);
}
return (com.liferay.object.model.ObjectEntry)returnObj;
}
catch (com.liferay.portal.kernel.exception.SystemException
systemException) {
_log.error(systemException, systemException);
throw systemException;
}
}
public static com.liferay.object.model.ObjectEntry deleteObjectEntry(
HttpPrincipal httpPrincipal, String externalReferenceCode,
long companyId, long groupId)
throws com.liferay.portal.kernel.exception.PortalException {
try {
MethodKey methodKey = new MethodKey(
ObjectEntryServiceUtil.class, "deleteObjectEntry",
_deleteObjectEntryParameterTypes4);
MethodHandler methodHandler = new MethodHandler(
methodKey, externalReferenceCode, companyId, groupId);
Object returnObj = null;
try {
returnObj = TunnelUtil.invoke(httpPrincipal, methodHandler);
}
catch (Exception exception) {
if (exception instanceof
com.liferay.portal.kernel.exception.PortalException) {
throw (com.liferay.portal.kernel.exception.PortalException)
exception;
}
throw new com.liferay.portal.kernel.exception.SystemException(
exception);
}
return (com.liferay.object.model.ObjectEntry)returnObj;
}
catch (com.liferay.portal.kernel.exception.SystemException
systemException) {
_log.error(systemException, systemException);
throw systemException;
}
}
public static com.liferay.object.model.ObjectEntry
fetchManyToOneObjectEntry(
HttpPrincipal httpPrincipal, long groupId,
long objectRelationshipId, long primaryKey)
throws com.liferay.portal.kernel.exception.PortalException {
try {
MethodKey methodKey = new MethodKey(
ObjectEntryServiceUtil.class, "fetchManyToOneObjectEntry",
_fetchManyToOneObjectEntryParameterTypes5);
MethodHandler methodHandler = new MethodHandler(
methodKey, groupId, objectRelationshipId, primaryKey);
Object returnObj = null;
try {
returnObj = TunnelUtil.invoke(httpPrincipal, methodHandler);
}
catch (Exception exception) {
if (exception instanceof
com.liferay.portal.kernel.exception.PortalException) {
throw (com.liferay.portal.kernel.exception.PortalException)
exception;
}
throw new com.liferay.portal.kernel.exception.SystemException(
exception);
}
return (com.liferay.object.model.ObjectEntry)returnObj;
}
catch (com.liferay.portal.kernel.exception.SystemException
systemException) {
_log.error(systemException, systemException);
throw systemException;
}
}
public static com.liferay.object.model.ObjectEntry fetchObjectEntry(
HttpPrincipal httpPrincipal, long objectEntryId)
throws com.liferay.portal.kernel.exception.PortalException {
try {
MethodKey methodKey = new MethodKey(
ObjectEntryServiceUtil.class, "fetchObjectEntry",
_fetchObjectEntryParameterTypes6);
MethodHandler methodHandler = new MethodHandler(
methodKey, objectEntryId);
Object returnObj = null;
try {
returnObj = TunnelUtil.invoke(httpPrincipal, methodHandler);
}
catch (Exception exception) {
if (exception instanceof
com.liferay.portal.kernel.exception.PortalException) {
throw (com.liferay.portal.kernel.exception.PortalException)
exception;
}
throw new com.liferay.portal.kernel.exception.SystemException(
exception);
}
return (com.liferay.object.model.ObjectEntry)returnObj;
}
catch (com.liferay.portal.kernel.exception.SystemException
systemException) {
_log.error(systemException, systemException);
throw systemException;
}
}
public static java.util.List
getManyToManyObjectEntries(
HttpPrincipal httpPrincipal, long groupId,
long objectRelationshipId, long primaryKey, boolean related,
boolean reverse, String search, int start, int end)
throws com.liferay.portal.kernel.exception.PortalException {
try {
MethodKey methodKey = new MethodKey(
ObjectEntryServiceUtil.class, "getManyToManyObjectEntries",
_getManyToManyObjectEntriesParameterTypes7);
MethodHandler methodHandler = new MethodHandler(
methodKey, groupId, objectRelationshipId, primaryKey, related,
reverse, search, start, end);
Object returnObj = null;
try {
returnObj = TunnelUtil.invoke(httpPrincipal, methodHandler);
}
catch (Exception exception) {
if (exception instanceof
com.liferay.portal.kernel.exception.PortalException) {
throw (com.liferay.portal.kernel.exception.PortalException)
exception;
}
throw new com.liferay.portal.kernel.exception.SystemException(
exception);
}
return (java.util.List)
returnObj;
}
catch (com.liferay.portal.kernel.exception.SystemException
systemException) {
_log.error(systemException, systemException);
throw systemException;
}
}
public static int getManyToManyObjectEntriesCount(
HttpPrincipal httpPrincipal, long groupId,
long objectRelationshipId, long primaryKey, boolean related,
boolean reverse, String search)
throws com.liferay.portal.kernel.exception.PortalException {
try {
MethodKey methodKey = new MethodKey(
ObjectEntryServiceUtil.class, "getManyToManyObjectEntriesCount",
_getManyToManyObjectEntriesCountParameterTypes8);
MethodHandler methodHandler = new MethodHandler(
methodKey, groupId, objectRelationshipId, primaryKey, related,
reverse, search);
Object returnObj = null;
try {
returnObj = TunnelUtil.invoke(httpPrincipal, methodHandler);
}
catch (Exception exception) {
if (exception instanceof
com.liferay.portal.kernel.exception.PortalException) {
throw (com.liferay.portal.kernel.exception.PortalException)
exception;
}
throw new com.liferay.portal.kernel.exception.SystemException(
exception);
}
return ((Integer)returnObj).intValue();
}
catch (com.liferay.portal.kernel.exception.SystemException
systemException) {
_log.error(systemException, systemException);
throw systemException;
}
}
public static com.liferay.portal.kernel.security.permission.resource.
ModelResourcePermission
getModelResourcePermission(
HttpPrincipal httpPrincipal, long objectDefinitionId)
throws com.liferay.portal.kernel.exception.PortalException {
try {
MethodKey methodKey = new MethodKey(
ObjectEntryServiceUtil.class, "getModelResourcePermission",
_getModelResourcePermissionParameterTypes9);
MethodHandler methodHandler = new MethodHandler(
methodKey, objectDefinitionId);
Object returnObj = null;
try {
returnObj = TunnelUtil.invoke(httpPrincipal, methodHandler);
}
catch (Exception exception) {
if (exception instanceof
com.liferay.portal.kernel.exception.PortalException) {
throw (com.liferay.portal.kernel.exception.PortalException)
exception;
}
throw new com.liferay.portal.kernel.exception.SystemException(
exception);
}
return (com.liferay.portal.kernel.security.permission.resource.
ModelResourcePermission)
returnObj;
}
catch (com.liferay.portal.kernel.exception.SystemException
systemException) {
_log.error(systemException, systemException);
throw systemException;
}
}
public static com.liferay.object.model.ObjectEntry getObjectEntry(
HttpPrincipal httpPrincipal, long objectEntryId)
throws com.liferay.portal.kernel.exception.PortalException {
try {
MethodKey methodKey = new MethodKey(
ObjectEntryServiceUtil.class, "getObjectEntry",
_getObjectEntryParameterTypes10);
MethodHandler methodHandler = new MethodHandler(
methodKey, objectEntryId);
Object returnObj = null;
try {
returnObj = TunnelUtil.invoke(httpPrincipal, methodHandler);
}
catch (Exception exception) {
if (exception instanceof
com.liferay.portal.kernel.exception.PortalException) {
throw (com.liferay.portal.kernel.exception.PortalException)
exception;
}
throw new com.liferay.portal.kernel.exception.SystemException(
exception);
}
return (com.liferay.object.model.ObjectEntry)returnObj;
}
catch (com.liferay.portal.kernel.exception.SystemException
systemException) {
_log.error(systemException, systemException);
throw systemException;
}
}
public static com.liferay.object.model.ObjectEntry getObjectEntry(
HttpPrincipal httpPrincipal, String externalReferenceCode,
long companyId, long groupId)
throws com.liferay.portal.kernel.exception.PortalException {
try {
MethodKey methodKey = new MethodKey(
ObjectEntryServiceUtil.class, "getObjectEntry",
_getObjectEntryParameterTypes11);
MethodHandler methodHandler = new MethodHandler(
methodKey, externalReferenceCode, companyId, groupId);
Object returnObj = null;
try {
returnObj = TunnelUtil.invoke(httpPrincipal, methodHandler);
}
catch (Exception exception) {
if (exception instanceof
com.liferay.portal.kernel.exception.PortalException) {
throw (com.liferay.portal.kernel.exception.PortalException)
exception;
}
throw new com.liferay.portal.kernel.exception.SystemException(
exception);
}
return (com.liferay.object.model.ObjectEntry)returnObj;
}
catch (com.liferay.portal.kernel.exception.SystemException
systemException) {
_log.error(systemException, systemException);
throw systemException;
}
}
public static java.util.List
getOneToManyObjectEntries(
HttpPrincipal httpPrincipal, long groupId,
long objectRelationshipId, long primaryKey, boolean related,
String search, int start, int end)
throws com.liferay.portal.kernel.exception.PortalException {
try {
MethodKey methodKey = new MethodKey(
ObjectEntryServiceUtil.class, "getOneToManyObjectEntries",
_getOneToManyObjectEntriesParameterTypes12);
MethodHandler methodHandler = new MethodHandler(
methodKey, groupId, objectRelationshipId, primaryKey, related,
search, start, end);
Object returnObj = null;
try {
returnObj = TunnelUtil.invoke(httpPrincipal, methodHandler);
}
catch (Exception exception) {
if (exception instanceof
com.liferay.portal.kernel.exception.PortalException) {
throw (com.liferay.portal.kernel.exception.PortalException)
exception;
}
throw new com.liferay.portal.kernel.exception.SystemException(
exception);
}
return (java.util.List)
returnObj;
}
catch (com.liferay.portal.kernel.exception.SystemException
systemException) {
_log.error(systemException, systemException);
throw systemException;
}
}
public static int getOneToManyObjectEntriesCount(
HttpPrincipal httpPrincipal, long groupId,
long objectRelationshipId, long primaryKey, boolean related,
String search)
throws com.liferay.portal.kernel.exception.PortalException {
try {
MethodKey methodKey = new MethodKey(
ObjectEntryServiceUtil.class, "getOneToManyObjectEntriesCount",
_getOneToManyObjectEntriesCountParameterTypes13);
MethodHandler methodHandler = new MethodHandler(
methodKey, groupId, objectRelationshipId, primaryKey, related,
search);
Object returnObj = null;
try {
returnObj = TunnelUtil.invoke(httpPrincipal, methodHandler);
}
catch (Exception exception) {
if (exception instanceof
com.liferay.portal.kernel.exception.PortalException) {
throw (com.liferay.portal.kernel.exception.PortalException)
exception;
}
throw new com.liferay.portal.kernel.exception.SystemException(
exception);
}
return ((Integer)returnObj).intValue();
}
catch (com.liferay.portal.kernel.exception.SystemException
systemException) {
_log.error(systemException, systemException);
throw systemException;
}
}
public static boolean hasModelResourcePermission(
HttpPrincipal httpPrincipal, long objectDefinitionId,
long objectEntryId, String actionId)
throws com.liferay.portal.kernel.exception.PortalException {
try {
MethodKey methodKey = new MethodKey(
ObjectEntryServiceUtil.class, "hasModelResourcePermission",
_hasModelResourcePermissionParameterTypes14);
MethodHandler methodHandler = new MethodHandler(
methodKey, objectDefinitionId, objectEntryId, actionId);
Object returnObj = null;
try {
returnObj = TunnelUtil.invoke(httpPrincipal, methodHandler);
}
catch (Exception exception) {
if (exception instanceof
com.liferay.portal.kernel.exception.PortalException) {
throw (com.liferay.portal.kernel.exception.PortalException)
exception;
}
throw new com.liferay.portal.kernel.exception.SystemException(
exception);
}
return ((Boolean)returnObj).booleanValue();
}
catch (com.liferay.portal.kernel.exception.SystemException
systemException) {
_log.error(systemException, systemException);
throw systemException;
}
}
public static boolean hasModelResourcePermission(
HttpPrincipal httpPrincipal,
com.liferay.object.model.ObjectEntry objectEntry, String actionId)
throws com.liferay.portal.kernel.exception.PortalException {
try {
MethodKey methodKey = new MethodKey(
ObjectEntryServiceUtil.class, "hasModelResourcePermission",
_hasModelResourcePermissionParameterTypes15);
MethodHandler methodHandler = new MethodHandler(
methodKey, objectEntry, actionId);
Object returnObj = null;
try {
returnObj = TunnelUtil.invoke(httpPrincipal, methodHandler);
}
catch (Exception exception) {
if (exception instanceof
com.liferay.portal.kernel.exception.PortalException) {
throw (com.liferay.portal.kernel.exception.PortalException)
exception;
}
throw new com.liferay.portal.kernel.exception.SystemException(
exception);
}
return ((Boolean)returnObj).booleanValue();
}
catch (com.liferay.portal.kernel.exception.SystemException
systemException) {
_log.error(systemException, systemException);
throw systemException;
}
}
public static boolean hasModelResourcePermission(
HttpPrincipal httpPrincipal,
com.liferay.portal.kernel.model.User user, long objectEntryId,
String actionId)
throws com.liferay.portal.kernel.exception.PortalException {
try {
MethodKey methodKey = new MethodKey(
ObjectEntryServiceUtil.class, "hasModelResourcePermission",
_hasModelResourcePermissionParameterTypes16);
MethodHandler methodHandler = new MethodHandler(
methodKey, user, objectEntryId, actionId);
Object returnObj = null;
try {
returnObj = TunnelUtil.invoke(httpPrincipal, methodHandler);
}
catch (Exception exception) {
if (exception instanceof
com.liferay.portal.kernel.exception.PortalException) {
throw (com.liferay.portal.kernel.exception.PortalException)
exception;
}
throw new com.liferay.portal.kernel.exception.SystemException(
exception);
}
return ((Boolean)returnObj).booleanValue();
}
catch (com.liferay.portal.kernel.exception.SystemException
systemException) {
_log.error(systemException, systemException);
throw systemException;
}
}
public static boolean hasPortletResourcePermission(
HttpPrincipal httpPrincipal, long groupId, long objectDefinitionId,
String actionId)
throws com.liferay.portal.kernel.exception.PortalException {
try {
MethodKey methodKey = new MethodKey(
ObjectEntryServiceUtil.class, "hasPortletResourcePermission",
_hasPortletResourcePermissionParameterTypes17);
MethodHandler methodHandler = new MethodHandler(
methodKey, groupId, objectDefinitionId, actionId);
Object returnObj = null;
try {
returnObj = TunnelUtil.invoke(httpPrincipal, methodHandler);
}
catch (Exception exception) {
if (exception instanceof
com.liferay.portal.kernel.exception.PortalException) {
throw (com.liferay.portal.kernel.exception.PortalException)
exception;
}
throw new com.liferay.portal.kernel.exception.SystemException(
exception);
}
return ((Boolean)returnObj).booleanValue();
}
catch (com.liferay.portal.kernel.exception.SystemException
systemException) {
_log.error(systemException, systemException);
throw systemException;
}
}
public static com.liferay.object.model.ObjectEntry updateObjectEntry(
HttpPrincipal httpPrincipal, long objectEntryId,
java.util.Map values,
com.liferay.portal.kernel.service.ServiceContext serviceContext)
throws com.liferay.portal.kernel.exception.PortalException {
try {
MethodKey methodKey = new MethodKey(
ObjectEntryServiceUtil.class, "updateObjectEntry",
_updateObjectEntryParameterTypes18);
MethodHandler methodHandler = new MethodHandler(
methodKey, objectEntryId, values, serviceContext);
Object returnObj = null;
try {
returnObj = TunnelUtil.invoke(httpPrincipal, methodHandler);
}
catch (Exception exception) {
if (exception instanceof
com.liferay.portal.kernel.exception.PortalException) {
throw (com.liferay.portal.kernel.exception.PortalException)
exception;
}
throw new com.liferay.portal.kernel.exception.SystemException(
exception);
}
return (com.liferay.object.model.ObjectEntry)returnObj;
}
catch (com.liferay.portal.kernel.exception.SystemException
systemException) {
_log.error(systemException, systemException);
throw systemException;
}
}
private static Log _log = LogFactoryUtil.getLog(
ObjectEntryServiceHttp.class);
private static final Class>[] _addObjectEntryParameterTypes0 =
new Class[] {
long.class, long.class, java.util.Map.class,
com.liferay.portal.kernel.service.ServiceContext.class
};
private static final Class>[] _addOrUpdateObjectEntryParameterTypes1 =
new Class[] {
String.class, long.class, long.class, java.util.Map.class,
com.liferay.portal.kernel.service.ServiceContext.class
};
private static final Class>[]
_checkModelResourcePermissionParameterTypes2 = new Class[] {
long.class, long.class, String.class
};
private static final Class>[] _deleteObjectEntryParameterTypes3 =
new Class[] {long.class};
private static final Class>[] _deleteObjectEntryParameterTypes4 =
new Class[] {String.class, long.class, long.class};
private static final Class>[] _fetchManyToOneObjectEntryParameterTypes5 =
new Class[] {long.class, long.class, long.class};
private static final Class>[] _fetchObjectEntryParameterTypes6 =
new Class[] {long.class};
private static final Class>[] _getManyToManyObjectEntriesParameterTypes7 =
new Class[] {
long.class, long.class, long.class, boolean.class, boolean.class,
String.class, int.class, int.class
};
private static final Class>[]
_getManyToManyObjectEntriesCountParameterTypes8 = new Class[] {
long.class, long.class, long.class, boolean.class, boolean.class,
String.class
};
private static final Class>[] _getModelResourcePermissionParameterTypes9 =
new Class[] {long.class};
private static final Class>[] _getObjectEntryParameterTypes10 =
new Class[] {long.class};
private static final Class>[] _getObjectEntryParameterTypes11 =
new Class[] {String.class, long.class, long.class};
private static final Class>[] _getOneToManyObjectEntriesParameterTypes12 =
new Class[] {
long.class, long.class, long.class, boolean.class, String.class,
int.class, int.class
};
private static final Class>[]
_getOneToManyObjectEntriesCountParameterTypes13 = new Class[] {
long.class, long.class, long.class, boolean.class, String.class
};
private static final Class>[]
_hasModelResourcePermissionParameterTypes14 = new Class[] {
long.class, long.class, String.class
};
private static final Class>[]
_hasModelResourcePermissionParameterTypes15 = new Class[] {
com.liferay.object.model.ObjectEntry.class, String.class
};
private static final Class>[]
_hasModelResourcePermissionParameterTypes16 = new Class[] {
com.liferay.portal.kernel.model.User.class, long.class, String.class
};
private static final Class>[]
_hasPortletResourcePermissionParameterTypes17 = new Class[] {
long.class, long.class, String.class
};
private static final Class>[] _updateObjectEntryParameterTypes18 =
new Class[] {
long.class, java.util.Map.class,
com.liferay.portal.kernel.service.ServiceContext.class
};
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy