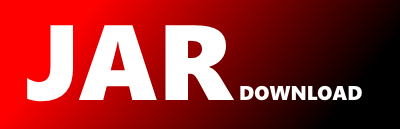
com.liferay.object.service.persistence.impl.ObjectRelationshipPersistenceImpl Maven / Gradle / Ivy
/**
* SPDX-FileCopyrightText: (c) 2000 Liferay, Inc. https://liferay.com
* SPDX-License-Identifier: LGPL-2.1-or-later OR LicenseRef-Liferay-DXP-EULA-2.0.0-2023-06
*/
package com.liferay.object.service.persistence.impl;
import com.liferay.object.exception.NoSuchObjectRelationshipException;
import com.liferay.object.model.ObjectRelationship;
import com.liferay.object.model.ObjectRelationshipTable;
import com.liferay.object.model.impl.ObjectRelationshipImpl;
import com.liferay.object.model.impl.ObjectRelationshipModelImpl;
import com.liferay.object.service.persistence.ObjectRelationshipPersistence;
import com.liferay.object.service.persistence.ObjectRelationshipUtil;
import com.liferay.object.service.persistence.impl.constants.ObjectPersistenceConstants;
import com.liferay.petra.string.StringBundler;
import com.liferay.portal.kernel.configuration.Configuration;
import com.liferay.portal.kernel.dao.orm.EntityCache;
import com.liferay.portal.kernel.dao.orm.FinderCache;
import com.liferay.portal.kernel.dao.orm.FinderPath;
import com.liferay.portal.kernel.dao.orm.Query;
import com.liferay.portal.kernel.dao.orm.QueryPos;
import com.liferay.portal.kernel.dao.orm.QueryUtil;
import com.liferay.portal.kernel.dao.orm.Session;
import com.liferay.portal.kernel.dao.orm.SessionFactory;
import com.liferay.portal.kernel.exception.SystemException;
import com.liferay.portal.kernel.log.Log;
import com.liferay.portal.kernel.log.LogFactoryUtil;
import com.liferay.portal.kernel.sanitizer.Sanitizer;
import com.liferay.portal.kernel.sanitizer.SanitizerException;
import com.liferay.portal.kernel.sanitizer.SanitizerUtil;
import com.liferay.portal.kernel.security.auth.CompanyThreadLocal;
import com.liferay.portal.kernel.security.auth.PrincipalThreadLocal;
import com.liferay.portal.kernel.service.ServiceContext;
import com.liferay.portal.kernel.service.ServiceContextThreadLocal;
import com.liferay.portal.kernel.service.persistence.impl.BasePersistenceImpl;
import com.liferay.portal.kernel.util.ContentTypes;
import com.liferay.portal.kernel.util.GetterUtil;
import com.liferay.portal.kernel.util.OrderByComparator;
import com.liferay.portal.kernel.util.PropsKeys;
import com.liferay.portal.kernel.util.PropsUtil;
import com.liferay.portal.kernel.util.ProxyUtil;
import com.liferay.portal.kernel.util.SetUtil;
import com.liferay.portal.kernel.util.StringUtil;
import com.liferay.portal.kernel.util.Validator;
import com.liferay.portal.kernel.uuid.PortalUUIDUtil;
import java.io.Serializable;
import java.lang.reflect.InvocationHandler;
import java.util.Collections;
import java.util.Date;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Set;
import javax.sql.DataSource;
import org.osgi.service.component.annotations.Activate;
import org.osgi.service.component.annotations.Component;
import org.osgi.service.component.annotations.Deactivate;
import org.osgi.service.component.annotations.Reference;
/**
* The persistence implementation for the object relationship service.
*
*
* Caching information and settings can be found in portal.properties
*
*
* @author Marco Leo
* @generated
*/
@Component(service = ObjectRelationshipPersistence.class)
public class ObjectRelationshipPersistenceImpl
extends BasePersistenceImpl
implements ObjectRelationshipPersistence {
/*
* NOTE FOR DEVELOPERS:
*
* Never modify or reference this class directly. Always use ObjectRelationshipUtil
to access the object relationship persistence. Modify service.xml
and rerun ServiceBuilder to regenerate this class.
*/
public static final String FINDER_CLASS_NAME_ENTITY =
ObjectRelationshipImpl.class.getName();
public static final String FINDER_CLASS_NAME_LIST_WITH_PAGINATION =
FINDER_CLASS_NAME_ENTITY + ".List1";
public static final String FINDER_CLASS_NAME_LIST_WITHOUT_PAGINATION =
FINDER_CLASS_NAME_ENTITY + ".List2";
private FinderPath _finderPathWithPaginationFindAll;
private FinderPath _finderPathWithoutPaginationFindAll;
private FinderPath _finderPathCountAll;
private FinderPath _finderPathWithPaginationFindByUuid;
private FinderPath _finderPathWithoutPaginationFindByUuid;
private FinderPath _finderPathCountByUuid;
/**
* Returns all the object relationships where uuid = ?.
*
* @param uuid the uuid
* @return the matching object relationships
*/
@Override
public List findByUuid(String uuid) {
return findByUuid(uuid, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the object relationships where uuid = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectRelationshipModelImpl
.
*
*
* @param uuid the uuid
* @param start the lower bound of the range of object relationships
* @param end the upper bound of the range of object relationships (not inclusive)
* @return the range of matching object relationships
*/
@Override
public List findByUuid(
String uuid, int start, int end) {
return findByUuid(uuid, start, end, null);
}
/**
* Returns an ordered range of all the object relationships where uuid = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectRelationshipModelImpl
.
*
*
* @param uuid the uuid
* @param start the lower bound of the range of object relationships
* @param end the upper bound of the range of object relationships (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching object relationships
*/
@Override
public List findByUuid(
String uuid, int start, int end,
OrderByComparator orderByComparator) {
return findByUuid(uuid, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the object relationships where uuid = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectRelationshipModelImpl
.
*
*
* @param uuid the uuid
* @param start the lower bound of the range of object relationships
* @param end the upper bound of the range of object relationships (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching object relationships
*/
@Override
public List findByUuid(
String uuid, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
uuid = Objects.toString(uuid, "");
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache) {
finderPath = _finderPathWithoutPaginationFindByUuid;
finderArgs = new Object[] {uuid};
}
}
else if (useFinderCache) {
finderPath = _finderPathWithPaginationFindByUuid;
finderArgs = new Object[] {uuid, start, end, orderByComparator};
}
List list = null;
if (useFinderCache) {
list = (List)finderCache.getResult(
finderPath, finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (ObjectRelationship objectRelationship : list) {
if (!uuid.equals(objectRelationship.getUuid())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
3 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(3);
}
sb.append(_SQL_SELECT_OBJECTRELATIONSHIP_WHERE);
boolean bindUuid = false;
if (uuid.isEmpty()) {
sb.append(_FINDER_COLUMN_UUID_UUID_3);
}
else {
bindUuid = true;
sb.append(_FINDER_COLUMN_UUID_UUID_2);
}
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(ObjectRelationshipModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
if (bindUuid) {
queryPos.add(uuid);
}
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first object relationship in the ordered set where uuid = ?.
*
* @param uuid the uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching object relationship
* @throws NoSuchObjectRelationshipException if a matching object relationship could not be found
*/
@Override
public ObjectRelationship findByUuid_First(
String uuid,
OrderByComparator orderByComparator)
throws NoSuchObjectRelationshipException {
ObjectRelationship objectRelationship = fetchByUuid_First(
uuid, orderByComparator);
if (objectRelationship != null) {
return objectRelationship;
}
StringBundler sb = new StringBundler(4);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("uuid=");
sb.append(uuid);
sb.append("}");
throw new NoSuchObjectRelationshipException(sb.toString());
}
/**
* Returns the first object relationship in the ordered set where uuid = ?.
*
* @param uuid the uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching object relationship, or null
if a matching object relationship could not be found
*/
@Override
public ObjectRelationship fetchByUuid_First(
String uuid, OrderByComparator orderByComparator) {
List list = findByUuid(
uuid, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last object relationship in the ordered set where uuid = ?.
*
* @param uuid the uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching object relationship
* @throws NoSuchObjectRelationshipException if a matching object relationship could not be found
*/
@Override
public ObjectRelationship findByUuid_Last(
String uuid,
OrderByComparator orderByComparator)
throws NoSuchObjectRelationshipException {
ObjectRelationship objectRelationship = fetchByUuid_Last(
uuid, orderByComparator);
if (objectRelationship != null) {
return objectRelationship;
}
StringBundler sb = new StringBundler(4);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("uuid=");
sb.append(uuid);
sb.append("}");
throw new NoSuchObjectRelationshipException(sb.toString());
}
/**
* Returns the last object relationship in the ordered set where uuid = ?.
*
* @param uuid the uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching object relationship, or null
if a matching object relationship could not be found
*/
@Override
public ObjectRelationship fetchByUuid_Last(
String uuid, OrderByComparator orderByComparator) {
int count = countByUuid(uuid);
if (count == 0) {
return null;
}
List list = findByUuid(
uuid, count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the object relationships before and after the current object relationship in the ordered set where uuid = ?.
*
* @param objectRelationshipId the primary key of the current object relationship
* @param uuid the uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next object relationship
* @throws NoSuchObjectRelationshipException if a object relationship with the primary key could not be found
*/
@Override
public ObjectRelationship[] findByUuid_PrevAndNext(
long objectRelationshipId, String uuid,
OrderByComparator orderByComparator)
throws NoSuchObjectRelationshipException {
uuid = Objects.toString(uuid, "");
ObjectRelationship objectRelationship = findByPrimaryKey(
objectRelationshipId);
Session session = null;
try {
session = openSession();
ObjectRelationship[] array = new ObjectRelationshipImpl[3];
array[0] = getByUuid_PrevAndNext(
session, objectRelationship, uuid, orderByComparator, true);
array[1] = objectRelationship;
array[2] = getByUuid_PrevAndNext(
session, objectRelationship, uuid, orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected ObjectRelationship getByUuid_PrevAndNext(
Session session, ObjectRelationship objectRelationship, String uuid,
OrderByComparator orderByComparator,
boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(3);
}
sb.append(_SQL_SELECT_OBJECTRELATIONSHIP_WHERE);
boolean bindUuid = false;
if (uuid.isEmpty()) {
sb.append(_FINDER_COLUMN_UUID_UUID_3);
}
else {
bindUuid = true;
sb.append(_FINDER_COLUMN_UUID_UUID_2);
}
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(ObjectRelationshipModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
if (bindUuid) {
queryPos.add(uuid);
}
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
objectRelationship)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the object relationships where uuid = ? from the database.
*
* @param uuid the uuid
*/
@Override
public void removeByUuid(String uuid) {
for (ObjectRelationship objectRelationship :
findByUuid(uuid, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null)) {
remove(objectRelationship);
}
}
/**
* Returns the number of object relationships where uuid = ?.
*
* @param uuid the uuid
* @return the number of matching object relationships
*/
@Override
public int countByUuid(String uuid) {
uuid = Objects.toString(uuid, "");
FinderPath finderPath = _finderPathCountByUuid;
Object[] finderArgs = new Object[] {uuid};
Long count = (Long)finderCache.getResult(finderPath, finderArgs, this);
if (count == null) {
StringBundler sb = new StringBundler(2);
sb.append(_SQL_COUNT_OBJECTRELATIONSHIP_WHERE);
boolean bindUuid = false;
if (uuid.isEmpty()) {
sb.append(_FINDER_COLUMN_UUID_UUID_3);
}
else {
bindUuid = true;
sb.append(_FINDER_COLUMN_UUID_UUID_2);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
if (bindUuid) {
queryPos.add(uuid);
}
count = (Long)query.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String _FINDER_COLUMN_UUID_UUID_2 =
"objectRelationship.uuid = ?";
private static final String _FINDER_COLUMN_UUID_UUID_3 =
"(objectRelationship.uuid IS NULL OR objectRelationship.uuid = '')";
private FinderPath _finderPathWithPaginationFindByUuid_C;
private FinderPath _finderPathWithoutPaginationFindByUuid_C;
private FinderPath _finderPathCountByUuid_C;
/**
* Returns all the object relationships where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @return the matching object relationships
*/
@Override
public List findByUuid_C(String uuid, long companyId) {
return findByUuid_C(
uuid, companyId, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the object relationships where uuid = ? and companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectRelationshipModelImpl
.
*
*
* @param uuid the uuid
* @param companyId the company ID
* @param start the lower bound of the range of object relationships
* @param end the upper bound of the range of object relationships (not inclusive)
* @return the range of matching object relationships
*/
@Override
public List findByUuid_C(
String uuid, long companyId, int start, int end) {
return findByUuid_C(uuid, companyId, start, end, null);
}
/**
* Returns an ordered range of all the object relationships where uuid = ? and companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectRelationshipModelImpl
.
*
*
* @param uuid the uuid
* @param companyId the company ID
* @param start the lower bound of the range of object relationships
* @param end the upper bound of the range of object relationships (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching object relationships
*/
@Override
public List findByUuid_C(
String uuid, long companyId, int start, int end,
OrderByComparator orderByComparator) {
return findByUuid_C(
uuid, companyId, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the object relationships where uuid = ? and companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectRelationshipModelImpl
.
*
*
* @param uuid the uuid
* @param companyId the company ID
* @param start the lower bound of the range of object relationships
* @param end the upper bound of the range of object relationships (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching object relationships
*/
@Override
public List findByUuid_C(
String uuid, long companyId, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
uuid = Objects.toString(uuid, "");
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache) {
finderPath = _finderPathWithoutPaginationFindByUuid_C;
finderArgs = new Object[] {uuid, companyId};
}
}
else if (useFinderCache) {
finderPath = _finderPathWithPaginationFindByUuid_C;
finderArgs = new Object[] {
uuid, companyId, start, end, orderByComparator
};
}
List list = null;
if (useFinderCache) {
list = (List)finderCache.getResult(
finderPath, finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (ObjectRelationship objectRelationship : list) {
if (!uuid.equals(objectRelationship.getUuid()) ||
(companyId != objectRelationship.getCompanyId())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_OBJECTRELATIONSHIP_WHERE);
boolean bindUuid = false;
if (uuid.isEmpty()) {
sb.append(_FINDER_COLUMN_UUID_C_UUID_3);
}
else {
bindUuid = true;
sb.append(_FINDER_COLUMN_UUID_C_UUID_2);
}
sb.append(_FINDER_COLUMN_UUID_C_COMPANYID_2);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(ObjectRelationshipModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
if (bindUuid) {
queryPos.add(uuid);
}
queryPos.add(companyId);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first object relationship in the ordered set where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching object relationship
* @throws NoSuchObjectRelationshipException if a matching object relationship could not be found
*/
@Override
public ObjectRelationship findByUuid_C_First(
String uuid, long companyId,
OrderByComparator orderByComparator)
throws NoSuchObjectRelationshipException {
ObjectRelationship objectRelationship = fetchByUuid_C_First(
uuid, companyId, orderByComparator);
if (objectRelationship != null) {
return objectRelationship;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("uuid=");
sb.append(uuid);
sb.append(", companyId=");
sb.append(companyId);
sb.append("}");
throw new NoSuchObjectRelationshipException(sb.toString());
}
/**
* Returns the first object relationship in the ordered set where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching object relationship, or null
if a matching object relationship could not be found
*/
@Override
public ObjectRelationship fetchByUuid_C_First(
String uuid, long companyId,
OrderByComparator orderByComparator) {
List list = findByUuid_C(
uuid, companyId, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last object relationship in the ordered set where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching object relationship
* @throws NoSuchObjectRelationshipException if a matching object relationship could not be found
*/
@Override
public ObjectRelationship findByUuid_C_Last(
String uuid, long companyId,
OrderByComparator orderByComparator)
throws NoSuchObjectRelationshipException {
ObjectRelationship objectRelationship = fetchByUuid_C_Last(
uuid, companyId, orderByComparator);
if (objectRelationship != null) {
return objectRelationship;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("uuid=");
sb.append(uuid);
sb.append(", companyId=");
sb.append(companyId);
sb.append("}");
throw new NoSuchObjectRelationshipException(sb.toString());
}
/**
* Returns the last object relationship in the ordered set where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching object relationship, or null
if a matching object relationship could not be found
*/
@Override
public ObjectRelationship fetchByUuid_C_Last(
String uuid, long companyId,
OrderByComparator orderByComparator) {
int count = countByUuid_C(uuid, companyId);
if (count == 0) {
return null;
}
List list = findByUuid_C(
uuid, companyId, count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the object relationships before and after the current object relationship in the ordered set where uuid = ? and companyId = ?.
*
* @param objectRelationshipId the primary key of the current object relationship
* @param uuid the uuid
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next object relationship
* @throws NoSuchObjectRelationshipException if a object relationship with the primary key could not be found
*/
@Override
public ObjectRelationship[] findByUuid_C_PrevAndNext(
long objectRelationshipId, String uuid, long companyId,
OrderByComparator orderByComparator)
throws NoSuchObjectRelationshipException {
uuid = Objects.toString(uuid, "");
ObjectRelationship objectRelationship = findByPrimaryKey(
objectRelationshipId);
Session session = null;
try {
session = openSession();
ObjectRelationship[] array = new ObjectRelationshipImpl[3];
array[0] = getByUuid_C_PrevAndNext(
session, objectRelationship, uuid, companyId, orderByComparator,
true);
array[1] = objectRelationship;
array[2] = getByUuid_C_PrevAndNext(
session, objectRelationship, uuid, companyId, orderByComparator,
false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected ObjectRelationship getByUuid_C_PrevAndNext(
Session session, ObjectRelationship objectRelationship, String uuid,
long companyId, OrderByComparator orderByComparator,
boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
5 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_OBJECTRELATIONSHIP_WHERE);
boolean bindUuid = false;
if (uuid.isEmpty()) {
sb.append(_FINDER_COLUMN_UUID_C_UUID_3);
}
else {
bindUuid = true;
sb.append(_FINDER_COLUMN_UUID_C_UUID_2);
}
sb.append(_FINDER_COLUMN_UUID_C_COMPANYID_2);
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(ObjectRelationshipModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
if (bindUuid) {
queryPos.add(uuid);
}
queryPos.add(companyId);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
objectRelationship)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the object relationships where uuid = ? and companyId = ? from the database.
*
* @param uuid the uuid
* @param companyId the company ID
*/
@Override
public void removeByUuid_C(String uuid, long companyId) {
for (ObjectRelationship objectRelationship :
findByUuid_C(
uuid, companyId, QueryUtil.ALL_POS, QueryUtil.ALL_POS,
null)) {
remove(objectRelationship);
}
}
/**
* Returns the number of object relationships where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @return the number of matching object relationships
*/
@Override
public int countByUuid_C(String uuid, long companyId) {
uuid = Objects.toString(uuid, "");
FinderPath finderPath = _finderPathCountByUuid_C;
Object[] finderArgs = new Object[] {uuid, companyId};
Long count = (Long)finderCache.getResult(finderPath, finderArgs, this);
if (count == null) {
StringBundler sb = new StringBundler(3);
sb.append(_SQL_COUNT_OBJECTRELATIONSHIP_WHERE);
boolean bindUuid = false;
if (uuid.isEmpty()) {
sb.append(_FINDER_COLUMN_UUID_C_UUID_3);
}
else {
bindUuid = true;
sb.append(_FINDER_COLUMN_UUID_C_UUID_2);
}
sb.append(_FINDER_COLUMN_UUID_C_COMPANYID_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
if (bindUuid) {
queryPos.add(uuid);
}
queryPos.add(companyId);
count = (Long)query.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String _FINDER_COLUMN_UUID_C_UUID_2 =
"objectRelationship.uuid = ? AND ";
private static final String _FINDER_COLUMN_UUID_C_UUID_3 =
"(objectRelationship.uuid IS NULL OR objectRelationship.uuid = '') AND ";
private static final String _FINDER_COLUMN_UUID_C_COMPANYID_2 =
"objectRelationship.companyId = ?";
private FinderPath _finderPathWithPaginationFindByObjectDefinitionId1;
private FinderPath _finderPathWithoutPaginationFindByObjectDefinitionId1;
private FinderPath _finderPathCountByObjectDefinitionId1;
/**
* Returns all the object relationships where objectDefinitionId1 = ?.
*
* @param objectDefinitionId1 the object definition id1
* @return the matching object relationships
*/
@Override
public List findByObjectDefinitionId1(
long objectDefinitionId1) {
return findByObjectDefinitionId1(
objectDefinitionId1, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the object relationships where objectDefinitionId1 = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectRelationshipModelImpl
.
*
*
* @param objectDefinitionId1 the object definition id1
* @param start the lower bound of the range of object relationships
* @param end the upper bound of the range of object relationships (not inclusive)
* @return the range of matching object relationships
*/
@Override
public List findByObjectDefinitionId1(
long objectDefinitionId1, int start, int end) {
return findByObjectDefinitionId1(objectDefinitionId1, start, end, null);
}
/**
* Returns an ordered range of all the object relationships where objectDefinitionId1 = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectRelationshipModelImpl
.
*
*
* @param objectDefinitionId1 the object definition id1
* @param start the lower bound of the range of object relationships
* @param end the upper bound of the range of object relationships (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching object relationships
*/
@Override
public List findByObjectDefinitionId1(
long objectDefinitionId1, int start, int end,
OrderByComparator orderByComparator) {
return findByObjectDefinitionId1(
objectDefinitionId1, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the object relationships where objectDefinitionId1 = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectRelationshipModelImpl
.
*
*
* @param objectDefinitionId1 the object definition id1
* @param start the lower bound of the range of object relationships
* @param end the upper bound of the range of object relationships (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching object relationships
*/
@Override
public List findByObjectDefinitionId1(
long objectDefinitionId1, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache) {
finderPath =
_finderPathWithoutPaginationFindByObjectDefinitionId1;
finderArgs = new Object[] {objectDefinitionId1};
}
}
else if (useFinderCache) {
finderPath = _finderPathWithPaginationFindByObjectDefinitionId1;
finderArgs = new Object[] {
objectDefinitionId1, start, end, orderByComparator
};
}
List list = null;
if (useFinderCache) {
list = (List)finderCache.getResult(
finderPath, finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (ObjectRelationship objectRelationship : list) {
if (objectDefinitionId1 !=
objectRelationship.getObjectDefinitionId1()) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
3 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(3);
}
sb.append(_SQL_SELECT_OBJECTRELATIONSHIP_WHERE);
sb.append(_FINDER_COLUMN_OBJECTDEFINITIONID1_OBJECTDEFINITIONID1_2);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(ObjectRelationshipModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(objectDefinitionId1);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first object relationship in the ordered set where objectDefinitionId1 = ?.
*
* @param objectDefinitionId1 the object definition id1
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching object relationship
* @throws NoSuchObjectRelationshipException if a matching object relationship could not be found
*/
@Override
public ObjectRelationship findByObjectDefinitionId1_First(
long objectDefinitionId1,
OrderByComparator orderByComparator)
throws NoSuchObjectRelationshipException {
ObjectRelationship objectRelationship =
fetchByObjectDefinitionId1_First(
objectDefinitionId1, orderByComparator);
if (objectRelationship != null) {
return objectRelationship;
}
StringBundler sb = new StringBundler(4);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("objectDefinitionId1=");
sb.append(objectDefinitionId1);
sb.append("}");
throw new NoSuchObjectRelationshipException(sb.toString());
}
/**
* Returns the first object relationship in the ordered set where objectDefinitionId1 = ?.
*
* @param objectDefinitionId1 the object definition id1
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching object relationship, or null
if a matching object relationship could not be found
*/
@Override
public ObjectRelationship fetchByObjectDefinitionId1_First(
long objectDefinitionId1,
OrderByComparator orderByComparator) {
List list = findByObjectDefinitionId1(
objectDefinitionId1, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last object relationship in the ordered set where objectDefinitionId1 = ?.
*
* @param objectDefinitionId1 the object definition id1
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching object relationship
* @throws NoSuchObjectRelationshipException if a matching object relationship could not be found
*/
@Override
public ObjectRelationship findByObjectDefinitionId1_Last(
long objectDefinitionId1,
OrderByComparator orderByComparator)
throws NoSuchObjectRelationshipException {
ObjectRelationship objectRelationship = fetchByObjectDefinitionId1_Last(
objectDefinitionId1, orderByComparator);
if (objectRelationship != null) {
return objectRelationship;
}
StringBundler sb = new StringBundler(4);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("objectDefinitionId1=");
sb.append(objectDefinitionId1);
sb.append("}");
throw new NoSuchObjectRelationshipException(sb.toString());
}
/**
* Returns the last object relationship in the ordered set where objectDefinitionId1 = ?.
*
* @param objectDefinitionId1 the object definition id1
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching object relationship, or null
if a matching object relationship could not be found
*/
@Override
public ObjectRelationship fetchByObjectDefinitionId1_Last(
long objectDefinitionId1,
OrderByComparator orderByComparator) {
int count = countByObjectDefinitionId1(objectDefinitionId1);
if (count == 0) {
return null;
}
List list = findByObjectDefinitionId1(
objectDefinitionId1, count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the object relationships before and after the current object relationship in the ordered set where objectDefinitionId1 = ?.
*
* @param objectRelationshipId the primary key of the current object relationship
* @param objectDefinitionId1 the object definition id1
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next object relationship
* @throws NoSuchObjectRelationshipException if a object relationship with the primary key could not be found
*/
@Override
public ObjectRelationship[] findByObjectDefinitionId1_PrevAndNext(
long objectRelationshipId, long objectDefinitionId1,
OrderByComparator orderByComparator)
throws NoSuchObjectRelationshipException {
ObjectRelationship objectRelationship = findByPrimaryKey(
objectRelationshipId);
Session session = null;
try {
session = openSession();
ObjectRelationship[] array = new ObjectRelationshipImpl[3];
array[0] = getByObjectDefinitionId1_PrevAndNext(
session, objectRelationship, objectDefinitionId1,
orderByComparator, true);
array[1] = objectRelationship;
array[2] = getByObjectDefinitionId1_PrevAndNext(
session, objectRelationship, objectDefinitionId1,
orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected ObjectRelationship getByObjectDefinitionId1_PrevAndNext(
Session session, ObjectRelationship objectRelationship,
long objectDefinitionId1,
OrderByComparator orderByComparator,
boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(3);
}
sb.append(_SQL_SELECT_OBJECTRELATIONSHIP_WHERE);
sb.append(_FINDER_COLUMN_OBJECTDEFINITIONID1_OBJECTDEFINITIONID1_2);
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(ObjectRelationshipModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(objectDefinitionId1);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
objectRelationship)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the object relationships where objectDefinitionId1 = ? from the database.
*
* @param objectDefinitionId1 the object definition id1
*/
@Override
public void removeByObjectDefinitionId1(long objectDefinitionId1) {
for (ObjectRelationship objectRelationship :
findByObjectDefinitionId1(
objectDefinitionId1, QueryUtil.ALL_POS, QueryUtil.ALL_POS,
null)) {
remove(objectRelationship);
}
}
/**
* Returns the number of object relationships where objectDefinitionId1 = ?.
*
* @param objectDefinitionId1 the object definition id1
* @return the number of matching object relationships
*/
@Override
public int countByObjectDefinitionId1(long objectDefinitionId1) {
FinderPath finderPath = _finderPathCountByObjectDefinitionId1;
Object[] finderArgs = new Object[] {objectDefinitionId1};
Long count = (Long)finderCache.getResult(finderPath, finderArgs, this);
if (count == null) {
StringBundler sb = new StringBundler(2);
sb.append(_SQL_COUNT_OBJECTRELATIONSHIP_WHERE);
sb.append(_FINDER_COLUMN_OBJECTDEFINITIONID1_OBJECTDEFINITIONID1_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(objectDefinitionId1);
count = (Long)query.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String
_FINDER_COLUMN_OBJECTDEFINITIONID1_OBJECTDEFINITIONID1_2 =
"objectRelationship.objectDefinitionId1 = ?";
private FinderPath _finderPathWithPaginationFindByObjectDefinitionId2;
private FinderPath _finderPathWithoutPaginationFindByObjectDefinitionId2;
private FinderPath _finderPathCountByObjectDefinitionId2;
/**
* Returns all the object relationships where objectDefinitionId2 = ?.
*
* @param objectDefinitionId2 the object definition id2
* @return the matching object relationships
*/
@Override
public List findByObjectDefinitionId2(
long objectDefinitionId2) {
return findByObjectDefinitionId2(
objectDefinitionId2, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the object relationships where objectDefinitionId2 = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectRelationshipModelImpl
.
*
*
* @param objectDefinitionId2 the object definition id2
* @param start the lower bound of the range of object relationships
* @param end the upper bound of the range of object relationships (not inclusive)
* @return the range of matching object relationships
*/
@Override
public List findByObjectDefinitionId2(
long objectDefinitionId2, int start, int end) {
return findByObjectDefinitionId2(objectDefinitionId2, start, end, null);
}
/**
* Returns an ordered range of all the object relationships where objectDefinitionId2 = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectRelationshipModelImpl
.
*
*
* @param objectDefinitionId2 the object definition id2
* @param start the lower bound of the range of object relationships
* @param end the upper bound of the range of object relationships (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching object relationships
*/
@Override
public List findByObjectDefinitionId2(
long objectDefinitionId2, int start, int end,
OrderByComparator orderByComparator) {
return findByObjectDefinitionId2(
objectDefinitionId2, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the object relationships where objectDefinitionId2 = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectRelationshipModelImpl
.
*
*
* @param objectDefinitionId2 the object definition id2
* @param start the lower bound of the range of object relationships
* @param end the upper bound of the range of object relationships (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching object relationships
*/
@Override
public List findByObjectDefinitionId2(
long objectDefinitionId2, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache) {
finderPath =
_finderPathWithoutPaginationFindByObjectDefinitionId2;
finderArgs = new Object[] {objectDefinitionId2};
}
}
else if (useFinderCache) {
finderPath = _finderPathWithPaginationFindByObjectDefinitionId2;
finderArgs = new Object[] {
objectDefinitionId2, start, end, orderByComparator
};
}
List list = null;
if (useFinderCache) {
list = (List)finderCache.getResult(
finderPath, finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (ObjectRelationship objectRelationship : list) {
if (objectDefinitionId2 !=
objectRelationship.getObjectDefinitionId2()) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
3 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(3);
}
sb.append(_SQL_SELECT_OBJECTRELATIONSHIP_WHERE);
sb.append(_FINDER_COLUMN_OBJECTDEFINITIONID2_OBJECTDEFINITIONID2_2);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(ObjectRelationshipModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(objectDefinitionId2);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first object relationship in the ordered set where objectDefinitionId2 = ?.
*
* @param objectDefinitionId2 the object definition id2
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching object relationship
* @throws NoSuchObjectRelationshipException if a matching object relationship could not be found
*/
@Override
public ObjectRelationship findByObjectDefinitionId2_First(
long objectDefinitionId2,
OrderByComparator orderByComparator)
throws NoSuchObjectRelationshipException {
ObjectRelationship objectRelationship =
fetchByObjectDefinitionId2_First(
objectDefinitionId2, orderByComparator);
if (objectRelationship != null) {
return objectRelationship;
}
StringBundler sb = new StringBundler(4);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("objectDefinitionId2=");
sb.append(objectDefinitionId2);
sb.append("}");
throw new NoSuchObjectRelationshipException(sb.toString());
}
/**
* Returns the first object relationship in the ordered set where objectDefinitionId2 = ?.
*
* @param objectDefinitionId2 the object definition id2
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching object relationship, or null
if a matching object relationship could not be found
*/
@Override
public ObjectRelationship fetchByObjectDefinitionId2_First(
long objectDefinitionId2,
OrderByComparator orderByComparator) {
List list = findByObjectDefinitionId2(
objectDefinitionId2, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last object relationship in the ordered set where objectDefinitionId2 = ?.
*
* @param objectDefinitionId2 the object definition id2
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching object relationship
* @throws NoSuchObjectRelationshipException if a matching object relationship could not be found
*/
@Override
public ObjectRelationship findByObjectDefinitionId2_Last(
long objectDefinitionId2,
OrderByComparator orderByComparator)
throws NoSuchObjectRelationshipException {
ObjectRelationship objectRelationship = fetchByObjectDefinitionId2_Last(
objectDefinitionId2, orderByComparator);
if (objectRelationship != null) {
return objectRelationship;
}
StringBundler sb = new StringBundler(4);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("objectDefinitionId2=");
sb.append(objectDefinitionId2);
sb.append("}");
throw new NoSuchObjectRelationshipException(sb.toString());
}
/**
* Returns the last object relationship in the ordered set where objectDefinitionId2 = ?.
*
* @param objectDefinitionId2 the object definition id2
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching object relationship, or null
if a matching object relationship could not be found
*/
@Override
public ObjectRelationship fetchByObjectDefinitionId2_Last(
long objectDefinitionId2,
OrderByComparator orderByComparator) {
int count = countByObjectDefinitionId2(objectDefinitionId2);
if (count == 0) {
return null;
}
List list = findByObjectDefinitionId2(
objectDefinitionId2, count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the object relationships before and after the current object relationship in the ordered set where objectDefinitionId2 = ?.
*
* @param objectRelationshipId the primary key of the current object relationship
* @param objectDefinitionId2 the object definition id2
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next object relationship
* @throws NoSuchObjectRelationshipException if a object relationship with the primary key could not be found
*/
@Override
public ObjectRelationship[] findByObjectDefinitionId2_PrevAndNext(
long objectRelationshipId, long objectDefinitionId2,
OrderByComparator orderByComparator)
throws NoSuchObjectRelationshipException {
ObjectRelationship objectRelationship = findByPrimaryKey(
objectRelationshipId);
Session session = null;
try {
session = openSession();
ObjectRelationship[] array = new ObjectRelationshipImpl[3];
array[0] = getByObjectDefinitionId2_PrevAndNext(
session, objectRelationship, objectDefinitionId2,
orderByComparator, true);
array[1] = objectRelationship;
array[2] = getByObjectDefinitionId2_PrevAndNext(
session, objectRelationship, objectDefinitionId2,
orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected ObjectRelationship getByObjectDefinitionId2_PrevAndNext(
Session session, ObjectRelationship objectRelationship,
long objectDefinitionId2,
OrderByComparator orderByComparator,
boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(3);
}
sb.append(_SQL_SELECT_OBJECTRELATIONSHIP_WHERE);
sb.append(_FINDER_COLUMN_OBJECTDEFINITIONID2_OBJECTDEFINITIONID2_2);
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(ObjectRelationshipModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(objectDefinitionId2);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
objectRelationship)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the object relationships where objectDefinitionId2 = ? from the database.
*
* @param objectDefinitionId2 the object definition id2
*/
@Override
public void removeByObjectDefinitionId2(long objectDefinitionId2) {
for (ObjectRelationship objectRelationship :
findByObjectDefinitionId2(
objectDefinitionId2, QueryUtil.ALL_POS, QueryUtil.ALL_POS,
null)) {
remove(objectRelationship);
}
}
/**
* Returns the number of object relationships where objectDefinitionId2 = ?.
*
* @param objectDefinitionId2 the object definition id2
* @return the number of matching object relationships
*/
@Override
public int countByObjectDefinitionId2(long objectDefinitionId2) {
FinderPath finderPath = _finderPathCountByObjectDefinitionId2;
Object[] finderArgs = new Object[] {objectDefinitionId2};
Long count = (Long)finderCache.getResult(finderPath, finderArgs, this);
if (count == null) {
StringBundler sb = new StringBundler(2);
sb.append(_SQL_COUNT_OBJECTRELATIONSHIP_WHERE);
sb.append(_FINDER_COLUMN_OBJECTDEFINITIONID2_OBJECTDEFINITIONID2_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(objectDefinitionId2);
count = (Long)query.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String
_FINDER_COLUMN_OBJECTDEFINITIONID2_OBJECTDEFINITIONID2_2 =
"objectRelationship.objectDefinitionId2 = ?";
private FinderPath _finderPathFetchByObjectFieldId2;
/**
* Returns the object relationship where objectFieldId2 = ? or throws a NoSuchObjectRelationshipException
if it could not be found.
*
* @param objectFieldId2 the object field id2
* @return the matching object relationship
* @throws NoSuchObjectRelationshipException if a matching object relationship could not be found
*/
@Override
public ObjectRelationship findByObjectFieldId2(long objectFieldId2)
throws NoSuchObjectRelationshipException {
ObjectRelationship objectRelationship = fetchByObjectFieldId2(
objectFieldId2);
if (objectRelationship == null) {
StringBundler sb = new StringBundler(4);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("objectFieldId2=");
sb.append(objectFieldId2);
sb.append("}");
if (_log.isDebugEnabled()) {
_log.debug(sb.toString());
}
throw new NoSuchObjectRelationshipException(sb.toString());
}
return objectRelationship;
}
/**
* Returns the object relationship where objectFieldId2 = ? or returns null
if it could not be found. Uses the finder cache.
*
* @param objectFieldId2 the object field id2
* @return the matching object relationship, or null
if a matching object relationship could not be found
*/
@Override
public ObjectRelationship fetchByObjectFieldId2(long objectFieldId2) {
return fetchByObjectFieldId2(objectFieldId2, true);
}
/**
* Returns the object relationship where objectFieldId2 = ? or returns null
if it could not be found, optionally using the finder cache.
*
* @param objectFieldId2 the object field id2
* @param useFinderCache whether to use the finder cache
* @return the matching object relationship, or null
if a matching object relationship could not be found
*/
@Override
public ObjectRelationship fetchByObjectFieldId2(
long objectFieldId2, boolean useFinderCache) {
Object[] finderArgs = null;
if (useFinderCache) {
finderArgs = new Object[] {objectFieldId2};
}
Object result = null;
if (useFinderCache) {
result = finderCache.getResult(
_finderPathFetchByObjectFieldId2, finderArgs, this);
}
if (result instanceof ObjectRelationship) {
ObjectRelationship objectRelationship = (ObjectRelationship)result;
if (objectFieldId2 != objectRelationship.getObjectFieldId2()) {
result = null;
}
}
if (result == null) {
StringBundler sb = new StringBundler(3);
sb.append(_SQL_SELECT_OBJECTRELATIONSHIP_WHERE);
sb.append(_FINDER_COLUMN_OBJECTFIELDID2_OBJECTFIELDID2_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(objectFieldId2);
List list = query.list();
if (list.isEmpty()) {
if (useFinderCache) {
finderCache.putResult(
_finderPathFetchByObjectFieldId2, finderArgs, list);
}
}
else {
if (list.size() > 1) {
Collections.sort(list, Collections.reverseOrder());
if (_log.isWarnEnabled()) {
if (!useFinderCache) {
finderArgs = new Object[] {objectFieldId2};
}
_log.warn(
"ObjectRelationshipPersistenceImpl.fetchByObjectFieldId2(long, boolean) with parameters (" +
StringUtil.merge(finderArgs) +
") yields a result set with more than 1 result. This violates the logical unique restriction. There is no order guarantee on which result is returned by this finder.");
}
}
ObjectRelationship objectRelationship = list.get(0);
result = objectRelationship;
cacheResult(objectRelationship);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
if (result instanceof List>) {
return null;
}
else {
return (ObjectRelationship)result;
}
}
/**
* Removes the object relationship where objectFieldId2 = ? from the database.
*
* @param objectFieldId2 the object field id2
* @return the object relationship that was removed
*/
@Override
public ObjectRelationship removeByObjectFieldId2(long objectFieldId2)
throws NoSuchObjectRelationshipException {
ObjectRelationship objectRelationship = findByObjectFieldId2(
objectFieldId2);
return remove(objectRelationship);
}
/**
* Returns the number of object relationships where objectFieldId2 = ?.
*
* @param objectFieldId2 the object field id2
* @return the number of matching object relationships
*/
@Override
public int countByObjectFieldId2(long objectFieldId2) {
ObjectRelationship objectRelationship = fetchByObjectFieldId2(
objectFieldId2);
if (objectRelationship == null) {
return 0;
}
return 1;
}
private static final String _FINDER_COLUMN_OBJECTFIELDID2_OBJECTFIELDID2_2 =
"objectRelationship.objectFieldId2 = ?";
private FinderPath _finderPathWithPaginationFindByParameterObjectFieldId;
private FinderPath _finderPathWithoutPaginationFindByParameterObjectFieldId;
private FinderPath _finderPathCountByParameterObjectFieldId;
/**
* Returns all the object relationships where parameterObjectFieldId = ?.
*
* @param parameterObjectFieldId the parameter object field ID
* @return the matching object relationships
*/
@Override
public List findByParameterObjectFieldId(
long parameterObjectFieldId) {
return findByParameterObjectFieldId(
parameterObjectFieldId, QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the object relationships where parameterObjectFieldId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectRelationshipModelImpl
.
*
*
* @param parameterObjectFieldId the parameter object field ID
* @param start the lower bound of the range of object relationships
* @param end the upper bound of the range of object relationships (not inclusive)
* @return the range of matching object relationships
*/
@Override
public List findByParameterObjectFieldId(
long parameterObjectFieldId, int start, int end) {
return findByParameterObjectFieldId(
parameterObjectFieldId, start, end, null);
}
/**
* Returns an ordered range of all the object relationships where parameterObjectFieldId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectRelationshipModelImpl
.
*
*
* @param parameterObjectFieldId the parameter object field ID
* @param start the lower bound of the range of object relationships
* @param end the upper bound of the range of object relationships (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching object relationships
*/
@Override
public List findByParameterObjectFieldId(
long parameterObjectFieldId, int start, int end,
OrderByComparator orderByComparator) {
return findByParameterObjectFieldId(
parameterObjectFieldId, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the object relationships where parameterObjectFieldId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectRelationshipModelImpl
.
*
*
* @param parameterObjectFieldId the parameter object field ID
* @param start the lower bound of the range of object relationships
* @param end the upper bound of the range of object relationships (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching object relationships
*/
@Override
public List findByParameterObjectFieldId(
long parameterObjectFieldId, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache) {
finderPath =
_finderPathWithoutPaginationFindByParameterObjectFieldId;
finderArgs = new Object[] {parameterObjectFieldId};
}
}
else if (useFinderCache) {
finderPath = _finderPathWithPaginationFindByParameterObjectFieldId;
finderArgs = new Object[] {
parameterObjectFieldId, start, end, orderByComparator
};
}
List list = null;
if (useFinderCache) {
list = (List)finderCache.getResult(
finderPath, finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (ObjectRelationship objectRelationship : list) {
if (parameterObjectFieldId !=
objectRelationship.getParameterObjectFieldId()) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
3 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(3);
}
sb.append(_SQL_SELECT_OBJECTRELATIONSHIP_WHERE);
sb.append(
_FINDER_COLUMN_PARAMETEROBJECTFIELDID_PARAMETEROBJECTFIELDID_2);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(ObjectRelationshipModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(parameterObjectFieldId);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first object relationship in the ordered set where parameterObjectFieldId = ?.
*
* @param parameterObjectFieldId the parameter object field ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching object relationship
* @throws NoSuchObjectRelationshipException if a matching object relationship could not be found
*/
@Override
public ObjectRelationship findByParameterObjectFieldId_First(
long parameterObjectFieldId,
OrderByComparator orderByComparator)
throws NoSuchObjectRelationshipException {
ObjectRelationship objectRelationship =
fetchByParameterObjectFieldId_First(
parameterObjectFieldId, orderByComparator);
if (objectRelationship != null) {
return objectRelationship;
}
StringBundler sb = new StringBundler(4);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("parameterObjectFieldId=");
sb.append(parameterObjectFieldId);
sb.append("}");
throw new NoSuchObjectRelationshipException(sb.toString());
}
/**
* Returns the first object relationship in the ordered set where parameterObjectFieldId = ?.
*
* @param parameterObjectFieldId the parameter object field ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching object relationship, or null
if a matching object relationship could not be found
*/
@Override
public ObjectRelationship fetchByParameterObjectFieldId_First(
long parameterObjectFieldId,
OrderByComparator orderByComparator) {
List list = findByParameterObjectFieldId(
parameterObjectFieldId, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last object relationship in the ordered set where parameterObjectFieldId = ?.
*
* @param parameterObjectFieldId the parameter object field ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching object relationship
* @throws NoSuchObjectRelationshipException if a matching object relationship could not be found
*/
@Override
public ObjectRelationship findByParameterObjectFieldId_Last(
long parameterObjectFieldId,
OrderByComparator orderByComparator)
throws NoSuchObjectRelationshipException {
ObjectRelationship objectRelationship =
fetchByParameterObjectFieldId_Last(
parameterObjectFieldId, orderByComparator);
if (objectRelationship != null) {
return objectRelationship;
}
StringBundler sb = new StringBundler(4);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("parameterObjectFieldId=");
sb.append(parameterObjectFieldId);
sb.append("}");
throw new NoSuchObjectRelationshipException(sb.toString());
}
/**
* Returns the last object relationship in the ordered set where parameterObjectFieldId = ?.
*
* @param parameterObjectFieldId the parameter object field ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching object relationship, or null
if a matching object relationship could not be found
*/
@Override
public ObjectRelationship fetchByParameterObjectFieldId_Last(
long parameterObjectFieldId,
OrderByComparator orderByComparator) {
int count = countByParameterObjectFieldId(parameterObjectFieldId);
if (count == 0) {
return null;
}
List list = findByParameterObjectFieldId(
parameterObjectFieldId, count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the object relationships before and after the current object relationship in the ordered set where parameterObjectFieldId = ?.
*
* @param objectRelationshipId the primary key of the current object relationship
* @param parameterObjectFieldId the parameter object field ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next object relationship
* @throws NoSuchObjectRelationshipException if a object relationship with the primary key could not be found
*/
@Override
public ObjectRelationship[] findByParameterObjectFieldId_PrevAndNext(
long objectRelationshipId, long parameterObjectFieldId,
OrderByComparator orderByComparator)
throws NoSuchObjectRelationshipException {
ObjectRelationship objectRelationship = findByPrimaryKey(
objectRelationshipId);
Session session = null;
try {
session = openSession();
ObjectRelationship[] array = new ObjectRelationshipImpl[3];
array[0] = getByParameterObjectFieldId_PrevAndNext(
session, objectRelationship, parameterObjectFieldId,
orderByComparator, true);
array[1] = objectRelationship;
array[2] = getByParameterObjectFieldId_PrevAndNext(
session, objectRelationship, parameterObjectFieldId,
orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected ObjectRelationship getByParameterObjectFieldId_PrevAndNext(
Session session, ObjectRelationship objectRelationship,
long parameterObjectFieldId,
OrderByComparator orderByComparator,
boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(3);
}
sb.append(_SQL_SELECT_OBJECTRELATIONSHIP_WHERE);
sb.append(
_FINDER_COLUMN_PARAMETEROBJECTFIELDID_PARAMETEROBJECTFIELDID_2);
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(ObjectRelationshipModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(parameterObjectFieldId);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
objectRelationship)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the object relationships where parameterObjectFieldId = ? from the database.
*
* @param parameterObjectFieldId the parameter object field ID
*/
@Override
public void removeByParameterObjectFieldId(long parameterObjectFieldId) {
for (ObjectRelationship objectRelationship :
findByParameterObjectFieldId(
parameterObjectFieldId, QueryUtil.ALL_POS,
QueryUtil.ALL_POS, null)) {
remove(objectRelationship);
}
}
/**
* Returns the number of object relationships where parameterObjectFieldId = ?.
*
* @param parameterObjectFieldId the parameter object field ID
* @return the number of matching object relationships
*/
@Override
public int countByParameterObjectFieldId(long parameterObjectFieldId) {
FinderPath finderPath = _finderPathCountByParameterObjectFieldId;
Object[] finderArgs = new Object[] {parameterObjectFieldId};
Long count = (Long)finderCache.getResult(finderPath, finderArgs, this);
if (count == null) {
StringBundler sb = new StringBundler(2);
sb.append(_SQL_COUNT_OBJECTRELATIONSHIP_WHERE);
sb.append(
_FINDER_COLUMN_PARAMETEROBJECTFIELDID_PARAMETEROBJECTFIELDID_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(parameterObjectFieldId);
count = (Long)query.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String
_FINDER_COLUMN_PARAMETEROBJECTFIELDID_PARAMETEROBJECTFIELDID_2 =
"objectRelationship.parameterObjectFieldId = ?";
private FinderPath _finderPathWithPaginationFindByODI1_E;
private FinderPath _finderPathWithoutPaginationFindByODI1_E;
private FinderPath _finderPathCountByODI1_E;
/**
* Returns all the object relationships where objectDefinitionId1 = ? and edge = ?.
*
* @param objectDefinitionId1 the object definition id1
* @param edge the edge
* @return the matching object relationships
*/
@Override
public List findByODI1_E(
long objectDefinitionId1, boolean edge) {
return findByODI1_E(
objectDefinitionId1, edge, QueryUtil.ALL_POS, QueryUtil.ALL_POS,
null);
}
/**
* Returns a range of all the object relationships where objectDefinitionId1 = ? and edge = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectRelationshipModelImpl
.
*
*
* @param objectDefinitionId1 the object definition id1
* @param edge the edge
* @param start the lower bound of the range of object relationships
* @param end the upper bound of the range of object relationships (not inclusive)
* @return the range of matching object relationships
*/
@Override
public List findByODI1_E(
long objectDefinitionId1, boolean edge, int start, int end) {
return findByODI1_E(objectDefinitionId1, edge, start, end, null);
}
/**
* Returns an ordered range of all the object relationships where objectDefinitionId1 = ? and edge = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectRelationshipModelImpl
.
*
*
* @param objectDefinitionId1 the object definition id1
* @param edge the edge
* @param start the lower bound of the range of object relationships
* @param end the upper bound of the range of object relationships (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching object relationships
*/
@Override
public List findByODI1_E(
long objectDefinitionId1, boolean edge, int start, int end,
OrderByComparator orderByComparator) {
return findByODI1_E(
objectDefinitionId1, edge, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the object relationships where objectDefinitionId1 = ? and edge = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectRelationshipModelImpl
.
*
*
* @param objectDefinitionId1 the object definition id1
* @param edge the edge
* @param start the lower bound of the range of object relationships
* @param end the upper bound of the range of object relationships (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching object relationships
*/
@Override
public List findByODI1_E(
long objectDefinitionId1, boolean edge, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache) {
finderPath = _finderPathWithoutPaginationFindByODI1_E;
finderArgs = new Object[] {objectDefinitionId1, edge};
}
}
else if (useFinderCache) {
finderPath = _finderPathWithPaginationFindByODI1_E;
finderArgs = new Object[] {
objectDefinitionId1, edge, start, end, orderByComparator
};
}
List list = null;
if (useFinderCache) {
list = (List)finderCache.getResult(
finderPath, finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (ObjectRelationship objectRelationship : list) {
if ((objectDefinitionId1 !=
objectRelationship.getObjectDefinitionId1()) ||
(edge != objectRelationship.isEdge())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_OBJECTRELATIONSHIP_WHERE);
sb.append(_FINDER_COLUMN_ODI1_E_OBJECTDEFINITIONID1_2);
sb.append(_FINDER_COLUMN_ODI1_E_EDGE_2);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(ObjectRelationshipModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(objectDefinitionId1);
queryPos.add(edge);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first object relationship in the ordered set where objectDefinitionId1 = ? and edge = ?.
*
* @param objectDefinitionId1 the object definition id1
* @param edge the edge
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching object relationship
* @throws NoSuchObjectRelationshipException if a matching object relationship could not be found
*/
@Override
public ObjectRelationship findByODI1_E_First(
long objectDefinitionId1, boolean edge,
OrderByComparator orderByComparator)
throws NoSuchObjectRelationshipException {
ObjectRelationship objectRelationship = fetchByODI1_E_First(
objectDefinitionId1, edge, orderByComparator);
if (objectRelationship != null) {
return objectRelationship;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("objectDefinitionId1=");
sb.append(objectDefinitionId1);
sb.append(", edge=");
sb.append(edge);
sb.append("}");
throw new NoSuchObjectRelationshipException(sb.toString());
}
/**
* Returns the first object relationship in the ordered set where objectDefinitionId1 = ? and edge = ?.
*
* @param objectDefinitionId1 the object definition id1
* @param edge the edge
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching object relationship, or null
if a matching object relationship could not be found
*/
@Override
public ObjectRelationship fetchByODI1_E_First(
long objectDefinitionId1, boolean edge,
OrderByComparator orderByComparator) {
List list = findByODI1_E(
objectDefinitionId1, edge, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last object relationship in the ordered set where objectDefinitionId1 = ? and edge = ?.
*
* @param objectDefinitionId1 the object definition id1
* @param edge the edge
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching object relationship
* @throws NoSuchObjectRelationshipException if a matching object relationship could not be found
*/
@Override
public ObjectRelationship findByODI1_E_Last(
long objectDefinitionId1, boolean edge,
OrderByComparator orderByComparator)
throws NoSuchObjectRelationshipException {
ObjectRelationship objectRelationship = fetchByODI1_E_Last(
objectDefinitionId1, edge, orderByComparator);
if (objectRelationship != null) {
return objectRelationship;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("objectDefinitionId1=");
sb.append(objectDefinitionId1);
sb.append(", edge=");
sb.append(edge);
sb.append("}");
throw new NoSuchObjectRelationshipException(sb.toString());
}
/**
* Returns the last object relationship in the ordered set where objectDefinitionId1 = ? and edge = ?.
*
* @param objectDefinitionId1 the object definition id1
* @param edge the edge
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching object relationship, or null
if a matching object relationship could not be found
*/
@Override
public ObjectRelationship fetchByODI1_E_Last(
long objectDefinitionId1, boolean edge,
OrderByComparator orderByComparator) {
int count = countByODI1_E(objectDefinitionId1, edge);
if (count == 0) {
return null;
}
List list = findByODI1_E(
objectDefinitionId1, edge, count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the object relationships before and after the current object relationship in the ordered set where objectDefinitionId1 = ? and edge = ?.
*
* @param objectRelationshipId the primary key of the current object relationship
* @param objectDefinitionId1 the object definition id1
* @param edge the edge
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next object relationship
* @throws NoSuchObjectRelationshipException if a object relationship with the primary key could not be found
*/
@Override
public ObjectRelationship[] findByODI1_E_PrevAndNext(
long objectRelationshipId, long objectDefinitionId1, boolean edge,
OrderByComparator orderByComparator)
throws NoSuchObjectRelationshipException {
ObjectRelationship objectRelationship = findByPrimaryKey(
objectRelationshipId);
Session session = null;
try {
session = openSession();
ObjectRelationship[] array = new ObjectRelationshipImpl[3];
array[0] = getByODI1_E_PrevAndNext(
session, objectRelationship, objectDefinitionId1, edge,
orderByComparator, true);
array[1] = objectRelationship;
array[2] = getByODI1_E_PrevAndNext(
session, objectRelationship, objectDefinitionId1, edge,
orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected ObjectRelationship getByODI1_E_PrevAndNext(
Session session, ObjectRelationship objectRelationship,
long objectDefinitionId1, boolean edge,
OrderByComparator orderByComparator,
boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
5 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_OBJECTRELATIONSHIP_WHERE);
sb.append(_FINDER_COLUMN_ODI1_E_OBJECTDEFINITIONID1_2);
sb.append(_FINDER_COLUMN_ODI1_E_EDGE_2);
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(ObjectRelationshipModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(objectDefinitionId1);
queryPos.add(edge);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
objectRelationship)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the object relationships where objectDefinitionId1 = ? and edge = ? from the database.
*
* @param objectDefinitionId1 the object definition id1
* @param edge the edge
*/
@Override
public void removeByODI1_E(long objectDefinitionId1, boolean edge) {
for (ObjectRelationship objectRelationship :
findByODI1_E(
objectDefinitionId1, edge, QueryUtil.ALL_POS,
QueryUtil.ALL_POS, null)) {
remove(objectRelationship);
}
}
/**
* Returns the number of object relationships where objectDefinitionId1 = ? and edge = ?.
*
* @param objectDefinitionId1 the object definition id1
* @param edge the edge
* @return the number of matching object relationships
*/
@Override
public int countByODI1_E(long objectDefinitionId1, boolean edge) {
FinderPath finderPath = _finderPathCountByODI1_E;
Object[] finderArgs = new Object[] {objectDefinitionId1, edge};
Long count = (Long)finderCache.getResult(finderPath, finderArgs, this);
if (count == null) {
StringBundler sb = new StringBundler(3);
sb.append(_SQL_COUNT_OBJECTRELATIONSHIP_WHERE);
sb.append(_FINDER_COLUMN_ODI1_E_OBJECTDEFINITIONID1_2);
sb.append(_FINDER_COLUMN_ODI1_E_EDGE_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(objectDefinitionId1);
queryPos.add(edge);
count = (Long)query.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String _FINDER_COLUMN_ODI1_E_OBJECTDEFINITIONID1_2 =
"objectRelationship.objectDefinitionId1 = ? AND ";
private static final String _FINDER_COLUMN_ODI1_E_EDGE_2 =
"objectRelationship.edge = ?";
private FinderPath _finderPathWithPaginationFindByODI1_N;
private FinderPath _finderPathWithoutPaginationFindByODI1_N;
private FinderPath _finderPathCountByODI1_N;
/**
* Returns all the object relationships where objectDefinitionId1 = ? and name = ?.
*
* @param objectDefinitionId1 the object definition id1
* @param name the name
* @return the matching object relationships
*/
@Override
public List findByODI1_N(
long objectDefinitionId1, String name) {
return findByODI1_N(
objectDefinitionId1, name, QueryUtil.ALL_POS, QueryUtil.ALL_POS,
null);
}
/**
* Returns a range of all the object relationships where objectDefinitionId1 = ? and name = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectRelationshipModelImpl
.
*
*
* @param objectDefinitionId1 the object definition id1
* @param name the name
* @param start the lower bound of the range of object relationships
* @param end the upper bound of the range of object relationships (not inclusive)
* @return the range of matching object relationships
*/
@Override
public List findByODI1_N(
long objectDefinitionId1, String name, int start, int end) {
return findByODI1_N(objectDefinitionId1, name, start, end, null);
}
/**
* Returns an ordered range of all the object relationships where objectDefinitionId1 = ? and name = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectRelationshipModelImpl
.
*
*
* @param objectDefinitionId1 the object definition id1
* @param name the name
* @param start the lower bound of the range of object relationships
* @param end the upper bound of the range of object relationships (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching object relationships
*/
@Override
public List findByODI1_N(
long objectDefinitionId1, String name, int start, int end,
OrderByComparator orderByComparator) {
return findByODI1_N(
objectDefinitionId1, name, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the object relationships where objectDefinitionId1 = ? and name = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectRelationshipModelImpl
.
*
*
* @param objectDefinitionId1 the object definition id1
* @param name the name
* @param start the lower bound of the range of object relationships
* @param end the upper bound of the range of object relationships (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching object relationships
*/
@Override
public List findByODI1_N(
long objectDefinitionId1, String name, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
name = Objects.toString(name, "");
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache) {
finderPath = _finderPathWithoutPaginationFindByODI1_N;
finderArgs = new Object[] {objectDefinitionId1, name};
}
}
else if (useFinderCache) {
finderPath = _finderPathWithPaginationFindByODI1_N;
finderArgs = new Object[] {
objectDefinitionId1, name, start, end, orderByComparator
};
}
List list = null;
if (useFinderCache) {
list = (List)finderCache.getResult(
finderPath, finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (ObjectRelationship objectRelationship : list) {
if ((objectDefinitionId1 !=
objectRelationship.getObjectDefinitionId1()) ||
!name.equals(objectRelationship.getName())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_OBJECTRELATIONSHIP_WHERE);
sb.append(_FINDER_COLUMN_ODI1_N_OBJECTDEFINITIONID1_2);
boolean bindName = false;
if (name.isEmpty()) {
sb.append(_FINDER_COLUMN_ODI1_N_NAME_3);
}
else {
bindName = true;
sb.append(_FINDER_COLUMN_ODI1_N_NAME_2);
}
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(ObjectRelationshipModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(objectDefinitionId1);
if (bindName) {
queryPos.add(name);
}
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first object relationship in the ordered set where objectDefinitionId1 = ? and name = ?.
*
* @param objectDefinitionId1 the object definition id1
* @param name the name
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching object relationship
* @throws NoSuchObjectRelationshipException if a matching object relationship could not be found
*/
@Override
public ObjectRelationship findByODI1_N_First(
long objectDefinitionId1, String name,
OrderByComparator orderByComparator)
throws NoSuchObjectRelationshipException {
ObjectRelationship objectRelationship = fetchByODI1_N_First(
objectDefinitionId1, name, orderByComparator);
if (objectRelationship != null) {
return objectRelationship;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("objectDefinitionId1=");
sb.append(objectDefinitionId1);
sb.append(", name=");
sb.append(name);
sb.append("}");
throw new NoSuchObjectRelationshipException(sb.toString());
}
/**
* Returns the first object relationship in the ordered set where objectDefinitionId1 = ? and name = ?.
*
* @param objectDefinitionId1 the object definition id1
* @param name the name
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching object relationship, or null
if a matching object relationship could not be found
*/
@Override
public ObjectRelationship fetchByODI1_N_First(
long objectDefinitionId1, String name,
OrderByComparator orderByComparator) {
List list = findByODI1_N(
objectDefinitionId1, name, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last object relationship in the ordered set where objectDefinitionId1 = ? and name = ?.
*
* @param objectDefinitionId1 the object definition id1
* @param name the name
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching object relationship
* @throws NoSuchObjectRelationshipException if a matching object relationship could not be found
*/
@Override
public ObjectRelationship findByODI1_N_Last(
long objectDefinitionId1, String name,
OrderByComparator orderByComparator)
throws NoSuchObjectRelationshipException {
ObjectRelationship objectRelationship = fetchByODI1_N_Last(
objectDefinitionId1, name, orderByComparator);
if (objectRelationship != null) {
return objectRelationship;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("objectDefinitionId1=");
sb.append(objectDefinitionId1);
sb.append(", name=");
sb.append(name);
sb.append("}");
throw new NoSuchObjectRelationshipException(sb.toString());
}
/**
* Returns the last object relationship in the ordered set where objectDefinitionId1 = ? and name = ?.
*
* @param objectDefinitionId1 the object definition id1
* @param name the name
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching object relationship, or null
if a matching object relationship could not be found
*/
@Override
public ObjectRelationship fetchByODI1_N_Last(
long objectDefinitionId1, String name,
OrderByComparator orderByComparator) {
int count = countByODI1_N(objectDefinitionId1, name);
if (count == 0) {
return null;
}
List list = findByODI1_N(
objectDefinitionId1, name, count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the object relationships before and after the current object relationship in the ordered set where objectDefinitionId1 = ? and name = ?.
*
* @param objectRelationshipId the primary key of the current object relationship
* @param objectDefinitionId1 the object definition id1
* @param name the name
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next object relationship
* @throws NoSuchObjectRelationshipException if a object relationship with the primary key could not be found
*/
@Override
public ObjectRelationship[] findByODI1_N_PrevAndNext(
long objectRelationshipId, long objectDefinitionId1, String name,
OrderByComparator orderByComparator)
throws NoSuchObjectRelationshipException {
name = Objects.toString(name, "");
ObjectRelationship objectRelationship = findByPrimaryKey(
objectRelationshipId);
Session session = null;
try {
session = openSession();
ObjectRelationship[] array = new ObjectRelationshipImpl[3];
array[0] = getByODI1_N_PrevAndNext(
session, objectRelationship, objectDefinitionId1, name,
orderByComparator, true);
array[1] = objectRelationship;
array[2] = getByODI1_N_PrevAndNext(
session, objectRelationship, objectDefinitionId1, name,
orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected ObjectRelationship getByODI1_N_PrevAndNext(
Session session, ObjectRelationship objectRelationship,
long objectDefinitionId1, String name,
OrderByComparator orderByComparator,
boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
5 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_OBJECTRELATIONSHIP_WHERE);
sb.append(_FINDER_COLUMN_ODI1_N_OBJECTDEFINITIONID1_2);
boolean bindName = false;
if (name.isEmpty()) {
sb.append(_FINDER_COLUMN_ODI1_N_NAME_3);
}
else {
bindName = true;
sb.append(_FINDER_COLUMN_ODI1_N_NAME_2);
}
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(ObjectRelationshipModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(objectDefinitionId1);
if (bindName) {
queryPos.add(name);
}
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
objectRelationship)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the object relationships where objectDefinitionId1 = ? and name = ? from the database.
*
* @param objectDefinitionId1 the object definition id1
* @param name the name
*/
@Override
public void removeByODI1_N(long objectDefinitionId1, String name) {
for (ObjectRelationship objectRelationship :
findByODI1_N(
objectDefinitionId1, name, QueryUtil.ALL_POS,
QueryUtil.ALL_POS, null)) {
remove(objectRelationship);
}
}
/**
* Returns the number of object relationships where objectDefinitionId1 = ? and name = ?.
*
* @param objectDefinitionId1 the object definition id1
* @param name the name
* @return the number of matching object relationships
*/
@Override
public int countByODI1_N(long objectDefinitionId1, String name) {
name = Objects.toString(name, "");
FinderPath finderPath = _finderPathCountByODI1_N;
Object[] finderArgs = new Object[] {objectDefinitionId1, name};
Long count = (Long)finderCache.getResult(finderPath, finderArgs, this);
if (count == null) {
StringBundler sb = new StringBundler(3);
sb.append(_SQL_COUNT_OBJECTRELATIONSHIP_WHERE);
sb.append(_FINDER_COLUMN_ODI1_N_OBJECTDEFINITIONID1_2);
boolean bindName = false;
if (name.isEmpty()) {
sb.append(_FINDER_COLUMN_ODI1_N_NAME_3);
}
else {
bindName = true;
sb.append(_FINDER_COLUMN_ODI1_N_NAME_2);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(objectDefinitionId1);
if (bindName) {
queryPos.add(name);
}
count = (Long)query.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String _FINDER_COLUMN_ODI1_N_OBJECTDEFINITIONID1_2 =
"objectRelationship.objectDefinitionId1 = ? AND ";
private static final String _FINDER_COLUMN_ODI1_N_NAME_2 =
"objectRelationship.name = ?";
private static final String _FINDER_COLUMN_ODI1_N_NAME_3 =
"(objectRelationship.name IS NULL OR objectRelationship.name = '')";
private FinderPath _finderPathWithPaginationFindByODI1_R;
private FinderPath _finderPathWithoutPaginationFindByODI1_R;
private FinderPath _finderPathCountByODI1_R;
/**
* Returns all the object relationships where objectDefinitionId1 = ? and reverse = ?.
*
* @param objectDefinitionId1 the object definition id1
* @param reverse the reverse
* @return the matching object relationships
*/
@Override
public List findByODI1_R(
long objectDefinitionId1, boolean reverse) {
return findByODI1_R(
objectDefinitionId1, reverse, QueryUtil.ALL_POS, QueryUtil.ALL_POS,
null);
}
/**
* Returns a range of all the object relationships where objectDefinitionId1 = ? and reverse = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectRelationshipModelImpl
.
*
*
* @param objectDefinitionId1 the object definition id1
* @param reverse the reverse
* @param start the lower bound of the range of object relationships
* @param end the upper bound of the range of object relationships (not inclusive)
* @return the range of matching object relationships
*/
@Override
public List findByODI1_R(
long objectDefinitionId1, boolean reverse, int start, int end) {
return findByODI1_R(objectDefinitionId1, reverse, start, end, null);
}
/**
* Returns an ordered range of all the object relationships where objectDefinitionId1 = ? and reverse = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectRelationshipModelImpl
.
*
*
* @param objectDefinitionId1 the object definition id1
* @param reverse the reverse
* @param start the lower bound of the range of object relationships
* @param end the upper bound of the range of object relationships (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching object relationships
*/
@Override
public List findByODI1_R(
long objectDefinitionId1, boolean reverse, int start, int end,
OrderByComparator orderByComparator) {
return findByODI1_R(
objectDefinitionId1, reverse, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the object relationships where objectDefinitionId1 = ? and reverse = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectRelationshipModelImpl
.
*
*
* @param objectDefinitionId1 the object definition id1
* @param reverse the reverse
* @param start the lower bound of the range of object relationships
* @param end the upper bound of the range of object relationships (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching object relationships
*/
@Override
public List findByODI1_R(
long objectDefinitionId1, boolean reverse, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache) {
finderPath = _finderPathWithoutPaginationFindByODI1_R;
finderArgs = new Object[] {objectDefinitionId1, reverse};
}
}
else if (useFinderCache) {
finderPath = _finderPathWithPaginationFindByODI1_R;
finderArgs = new Object[] {
objectDefinitionId1, reverse, start, end, orderByComparator
};
}
List list = null;
if (useFinderCache) {
list = (List)finderCache.getResult(
finderPath, finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (ObjectRelationship objectRelationship : list) {
if ((objectDefinitionId1 !=
objectRelationship.getObjectDefinitionId1()) ||
(reverse != objectRelationship.isReverse())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_OBJECTRELATIONSHIP_WHERE);
sb.append(_FINDER_COLUMN_ODI1_R_OBJECTDEFINITIONID1_2);
sb.append(_FINDER_COLUMN_ODI1_R_REVERSE_2);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(ObjectRelationshipModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(objectDefinitionId1);
queryPos.add(reverse);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first object relationship in the ordered set where objectDefinitionId1 = ? and reverse = ?.
*
* @param objectDefinitionId1 the object definition id1
* @param reverse the reverse
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching object relationship
* @throws NoSuchObjectRelationshipException if a matching object relationship could not be found
*/
@Override
public ObjectRelationship findByODI1_R_First(
long objectDefinitionId1, boolean reverse,
OrderByComparator orderByComparator)
throws NoSuchObjectRelationshipException {
ObjectRelationship objectRelationship = fetchByODI1_R_First(
objectDefinitionId1, reverse, orderByComparator);
if (objectRelationship != null) {
return objectRelationship;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("objectDefinitionId1=");
sb.append(objectDefinitionId1);
sb.append(", reverse=");
sb.append(reverse);
sb.append("}");
throw new NoSuchObjectRelationshipException(sb.toString());
}
/**
* Returns the first object relationship in the ordered set where objectDefinitionId1 = ? and reverse = ?.
*
* @param objectDefinitionId1 the object definition id1
* @param reverse the reverse
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching object relationship, or null
if a matching object relationship could not be found
*/
@Override
public ObjectRelationship fetchByODI1_R_First(
long objectDefinitionId1, boolean reverse,
OrderByComparator orderByComparator) {
List list = findByODI1_R(
objectDefinitionId1, reverse, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last object relationship in the ordered set where objectDefinitionId1 = ? and reverse = ?.
*
* @param objectDefinitionId1 the object definition id1
* @param reverse the reverse
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching object relationship
* @throws NoSuchObjectRelationshipException if a matching object relationship could not be found
*/
@Override
public ObjectRelationship findByODI1_R_Last(
long objectDefinitionId1, boolean reverse,
OrderByComparator orderByComparator)
throws NoSuchObjectRelationshipException {
ObjectRelationship objectRelationship = fetchByODI1_R_Last(
objectDefinitionId1, reverse, orderByComparator);
if (objectRelationship != null) {
return objectRelationship;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("objectDefinitionId1=");
sb.append(objectDefinitionId1);
sb.append(", reverse=");
sb.append(reverse);
sb.append("}");
throw new NoSuchObjectRelationshipException(sb.toString());
}
/**
* Returns the last object relationship in the ordered set where objectDefinitionId1 = ? and reverse = ?.
*
* @param objectDefinitionId1 the object definition id1
* @param reverse the reverse
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching object relationship, or null
if a matching object relationship could not be found
*/
@Override
public ObjectRelationship fetchByODI1_R_Last(
long objectDefinitionId1, boolean reverse,
OrderByComparator orderByComparator) {
int count = countByODI1_R(objectDefinitionId1, reverse);
if (count == 0) {
return null;
}
List list = findByODI1_R(
objectDefinitionId1, reverse, count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the object relationships before and after the current object relationship in the ordered set where objectDefinitionId1 = ? and reverse = ?.
*
* @param objectRelationshipId the primary key of the current object relationship
* @param objectDefinitionId1 the object definition id1
* @param reverse the reverse
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next object relationship
* @throws NoSuchObjectRelationshipException if a object relationship with the primary key could not be found
*/
@Override
public ObjectRelationship[] findByODI1_R_PrevAndNext(
long objectRelationshipId, long objectDefinitionId1,
boolean reverse,
OrderByComparator orderByComparator)
throws NoSuchObjectRelationshipException {
ObjectRelationship objectRelationship = findByPrimaryKey(
objectRelationshipId);
Session session = null;
try {
session = openSession();
ObjectRelationship[] array = new ObjectRelationshipImpl[3];
array[0] = getByODI1_R_PrevAndNext(
session, objectRelationship, objectDefinitionId1, reverse,
orderByComparator, true);
array[1] = objectRelationship;
array[2] = getByODI1_R_PrevAndNext(
session, objectRelationship, objectDefinitionId1, reverse,
orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected ObjectRelationship getByODI1_R_PrevAndNext(
Session session, ObjectRelationship objectRelationship,
long objectDefinitionId1, boolean reverse,
OrderByComparator orderByComparator,
boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
5 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_OBJECTRELATIONSHIP_WHERE);
sb.append(_FINDER_COLUMN_ODI1_R_OBJECTDEFINITIONID1_2);
sb.append(_FINDER_COLUMN_ODI1_R_REVERSE_2);
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(ObjectRelationshipModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(objectDefinitionId1);
queryPos.add(reverse);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
objectRelationship)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the object relationships where objectDefinitionId1 = ? and reverse = ? from the database.
*
* @param objectDefinitionId1 the object definition id1
* @param reverse the reverse
*/
@Override
public void removeByODI1_R(long objectDefinitionId1, boolean reverse) {
for (ObjectRelationship objectRelationship :
findByODI1_R(
objectDefinitionId1, reverse, QueryUtil.ALL_POS,
QueryUtil.ALL_POS, null)) {
remove(objectRelationship);
}
}
/**
* Returns the number of object relationships where objectDefinitionId1 = ? and reverse = ?.
*
* @param objectDefinitionId1 the object definition id1
* @param reverse the reverse
* @return the number of matching object relationships
*/
@Override
public int countByODI1_R(long objectDefinitionId1, boolean reverse) {
FinderPath finderPath = _finderPathCountByODI1_R;
Object[] finderArgs = new Object[] {objectDefinitionId1, reverse};
Long count = (Long)finderCache.getResult(finderPath, finderArgs, this);
if (count == null) {
StringBundler sb = new StringBundler(3);
sb.append(_SQL_COUNT_OBJECTRELATIONSHIP_WHERE);
sb.append(_FINDER_COLUMN_ODI1_R_OBJECTDEFINITIONID1_2);
sb.append(_FINDER_COLUMN_ODI1_R_REVERSE_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(objectDefinitionId1);
queryPos.add(reverse);
count = (Long)query.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String _FINDER_COLUMN_ODI1_R_OBJECTDEFINITIONID1_2 =
"objectRelationship.objectDefinitionId1 = ? AND ";
private static final String _FINDER_COLUMN_ODI1_R_REVERSE_2 =
"objectRelationship.reverse = ?";
private FinderPath _finderPathFetchByODI2_E;
/**
* Returns the object relationship where objectDefinitionId2 = ? and edge = ? or throws a NoSuchObjectRelationshipException
if it could not be found.
*
* @param objectDefinitionId2 the object definition id2
* @param edge the edge
* @return the matching object relationship
* @throws NoSuchObjectRelationshipException if a matching object relationship could not be found
*/
@Override
public ObjectRelationship findByODI2_E(
long objectDefinitionId2, boolean edge)
throws NoSuchObjectRelationshipException {
ObjectRelationship objectRelationship = fetchByODI2_E(
objectDefinitionId2, edge);
if (objectRelationship == null) {
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("objectDefinitionId2=");
sb.append(objectDefinitionId2);
sb.append(", edge=");
sb.append(edge);
sb.append("}");
if (_log.isDebugEnabled()) {
_log.debug(sb.toString());
}
throw new NoSuchObjectRelationshipException(sb.toString());
}
return objectRelationship;
}
/**
* Returns the object relationship where objectDefinitionId2 = ? and edge = ? or returns null
if it could not be found. Uses the finder cache.
*
* @param objectDefinitionId2 the object definition id2
* @param edge the edge
* @return the matching object relationship, or null
if a matching object relationship could not be found
*/
@Override
public ObjectRelationship fetchByODI2_E(
long objectDefinitionId2, boolean edge) {
return fetchByODI2_E(objectDefinitionId2, edge, true);
}
/**
* Returns the object relationship where objectDefinitionId2 = ? and edge = ? or returns null
if it could not be found, optionally using the finder cache.
*
* @param objectDefinitionId2 the object definition id2
* @param edge the edge
* @param useFinderCache whether to use the finder cache
* @return the matching object relationship, or null
if a matching object relationship could not be found
*/
@Override
public ObjectRelationship fetchByODI2_E(
long objectDefinitionId2, boolean edge, boolean useFinderCache) {
Object[] finderArgs = null;
if (useFinderCache) {
finderArgs = new Object[] {objectDefinitionId2, edge};
}
Object result = null;
if (useFinderCache) {
result = finderCache.getResult(
_finderPathFetchByODI2_E, finderArgs, this);
}
if (result instanceof ObjectRelationship) {
ObjectRelationship objectRelationship = (ObjectRelationship)result;
if ((objectDefinitionId2 !=
objectRelationship.getObjectDefinitionId2()) ||
(edge != objectRelationship.isEdge())) {
result = null;
}
}
if (result == null) {
StringBundler sb = new StringBundler(4);
sb.append(_SQL_SELECT_OBJECTRELATIONSHIP_WHERE);
sb.append(_FINDER_COLUMN_ODI2_E_OBJECTDEFINITIONID2_2);
sb.append(_FINDER_COLUMN_ODI2_E_EDGE_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(objectDefinitionId2);
queryPos.add(edge);
List list = query.list();
if (list.isEmpty()) {
if (useFinderCache) {
finderCache.putResult(
_finderPathFetchByODI2_E, finderArgs, list);
}
}
else {
if (list.size() > 1) {
Collections.sort(list, Collections.reverseOrder());
if (_log.isWarnEnabled()) {
if (!useFinderCache) {
finderArgs = new Object[] {
objectDefinitionId2, edge
};
}
_log.warn(
"ObjectRelationshipPersistenceImpl.fetchByODI2_E(long, boolean, boolean) with parameters (" +
StringUtil.merge(finderArgs) +
") yields a result set with more than 1 result. This violates the logical unique restriction. There is no order guarantee on which result is returned by this finder.");
}
}
ObjectRelationship objectRelationship = list.get(0);
result = objectRelationship;
cacheResult(objectRelationship);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
if (result instanceof List>) {
return null;
}
else {
return (ObjectRelationship)result;
}
}
/**
* Removes the object relationship where objectDefinitionId2 = ? and edge = ? from the database.
*
* @param objectDefinitionId2 the object definition id2
* @param edge the edge
* @return the object relationship that was removed
*/
@Override
public ObjectRelationship removeByODI2_E(
long objectDefinitionId2, boolean edge)
throws NoSuchObjectRelationshipException {
ObjectRelationship objectRelationship = findByODI2_E(
objectDefinitionId2, edge);
return remove(objectRelationship);
}
/**
* Returns the number of object relationships where objectDefinitionId2 = ? and edge = ?.
*
* @param objectDefinitionId2 the object definition id2
* @param edge the edge
* @return the number of matching object relationships
*/
@Override
public int countByODI2_E(long objectDefinitionId2, boolean edge) {
ObjectRelationship objectRelationship = fetchByODI2_E(
objectDefinitionId2, edge);
if (objectRelationship == null) {
return 0;
}
return 1;
}
private static final String _FINDER_COLUMN_ODI2_E_OBJECTDEFINITIONID2_2 =
"objectRelationship.objectDefinitionId2 = ? AND ";
private static final String _FINDER_COLUMN_ODI2_E_EDGE_2 =
"objectRelationship.edge = ?";
private FinderPath _finderPathWithPaginationFindByODI2_R;
private FinderPath _finderPathWithoutPaginationFindByODI2_R;
private FinderPath _finderPathCountByODI2_R;
/**
* Returns all the object relationships where objectDefinitionId2 = ? and reverse = ?.
*
* @param objectDefinitionId2 the object definition id2
* @param reverse the reverse
* @return the matching object relationships
*/
@Override
public List findByODI2_R(
long objectDefinitionId2, boolean reverse) {
return findByODI2_R(
objectDefinitionId2, reverse, QueryUtil.ALL_POS, QueryUtil.ALL_POS,
null);
}
/**
* Returns a range of all the object relationships where objectDefinitionId2 = ? and reverse = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectRelationshipModelImpl
.
*
*
* @param objectDefinitionId2 the object definition id2
* @param reverse the reverse
* @param start the lower bound of the range of object relationships
* @param end the upper bound of the range of object relationships (not inclusive)
* @return the range of matching object relationships
*/
@Override
public List findByODI2_R(
long objectDefinitionId2, boolean reverse, int start, int end) {
return findByODI2_R(objectDefinitionId2, reverse, start, end, null);
}
/**
* Returns an ordered range of all the object relationships where objectDefinitionId2 = ? and reverse = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectRelationshipModelImpl
.
*
*
* @param objectDefinitionId2 the object definition id2
* @param reverse the reverse
* @param start the lower bound of the range of object relationships
* @param end the upper bound of the range of object relationships (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching object relationships
*/
@Override
public List findByODI2_R(
long objectDefinitionId2, boolean reverse, int start, int end,
OrderByComparator orderByComparator) {
return findByODI2_R(
objectDefinitionId2, reverse, start, end, orderByComparator, true);
}
/**
* Returns an ordered range of all the object relationships where objectDefinitionId2 = ? and reverse = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectRelationshipModelImpl
.
*
*
* @param objectDefinitionId2 the object definition id2
* @param reverse the reverse
* @param start the lower bound of the range of object relationships
* @param end the upper bound of the range of object relationships (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching object relationships
*/
@Override
public List findByODI2_R(
long objectDefinitionId2, boolean reverse, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache) {
finderPath = _finderPathWithoutPaginationFindByODI2_R;
finderArgs = new Object[] {objectDefinitionId2, reverse};
}
}
else if (useFinderCache) {
finderPath = _finderPathWithPaginationFindByODI2_R;
finderArgs = new Object[] {
objectDefinitionId2, reverse, start, end, orderByComparator
};
}
List list = null;
if (useFinderCache) {
list = (List)finderCache.getResult(
finderPath, finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (ObjectRelationship objectRelationship : list) {
if ((objectDefinitionId2 !=
objectRelationship.getObjectDefinitionId2()) ||
(reverse != objectRelationship.isReverse())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
4 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_OBJECTRELATIONSHIP_WHERE);
sb.append(_FINDER_COLUMN_ODI2_R_OBJECTDEFINITIONID2_2);
sb.append(_FINDER_COLUMN_ODI2_R_REVERSE_2);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(ObjectRelationshipModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(objectDefinitionId2);
queryPos.add(reverse);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first object relationship in the ordered set where objectDefinitionId2 = ? and reverse = ?.
*
* @param objectDefinitionId2 the object definition id2
* @param reverse the reverse
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching object relationship
* @throws NoSuchObjectRelationshipException if a matching object relationship could not be found
*/
@Override
public ObjectRelationship findByODI2_R_First(
long objectDefinitionId2, boolean reverse,
OrderByComparator orderByComparator)
throws NoSuchObjectRelationshipException {
ObjectRelationship objectRelationship = fetchByODI2_R_First(
objectDefinitionId2, reverse, orderByComparator);
if (objectRelationship != null) {
return objectRelationship;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("objectDefinitionId2=");
sb.append(objectDefinitionId2);
sb.append(", reverse=");
sb.append(reverse);
sb.append("}");
throw new NoSuchObjectRelationshipException(sb.toString());
}
/**
* Returns the first object relationship in the ordered set where objectDefinitionId2 = ? and reverse = ?.
*
* @param objectDefinitionId2 the object definition id2
* @param reverse the reverse
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching object relationship, or null
if a matching object relationship could not be found
*/
@Override
public ObjectRelationship fetchByODI2_R_First(
long objectDefinitionId2, boolean reverse,
OrderByComparator orderByComparator) {
List list = findByODI2_R(
objectDefinitionId2, reverse, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last object relationship in the ordered set where objectDefinitionId2 = ? and reverse = ?.
*
* @param objectDefinitionId2 the object definition id2
* @param reverse the reverse
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching object relationship
* @throws NoSuchObjectRelationshipException if a matching object relationship could not be found
*/
@Override
public ObjectRelationship findByODI2_R_Last(
long objectDefinitionId2, boolean reverse,
OrderByComparator orderByComparator)
throws NoSuchObjectRelationshipException {
ObjectRelationship objectRelationship = fetchByODI2_R_Last(
objectDefinitionId2, reverse, orderByComparator);
if (objectRelationship != null) {
return objectRelationship;
}
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("objectDefinitionId2=");
sb.append(objectDefinitionId2);
sb.append(", reverse=");
sb.append(reverse);
sb.append("}");
throw new NoSuchObjectRelationshipException(sb.toString());
}
/**
* Returns the last object relationship in the ordered set where objectDefinitionId2 = ? and reverse = ?.
*
* @param objectDefinitionId2 the object definition id2
* @param reverse the reverse
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching object relationship, or null
if a matching object relationship could not be found
*/
@Override
public ObjectRelationship fetchByODI2_R_Last(
long objectDefinitionId2, boolean reverse,
OrderByComparator orderByComparator) {
int count = countByODI2_R(objectDefinitionId2, reverse);
if (count == 0) {
return null;
}
List list = findByODI2_R(
objectDefinitionId2, reverse, count - 1, count, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the object relationships before and after the current object relationship in the ordered set where objectDefinitionId2 = ? and reverse = ?.
*
* @param objectRelationshipId the primary key of the current object relationship
* @param objectDefinitionId2 the object definition id2
* @param reverse the reverse
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next object relationship
* @throws NoSuchObjectRelationshipException if a object relationship with the primary key could not be found
*/
@Override
public ObjectRelationship[] findByODI2_R_PrevAndNext(
long objectRelationshipId, long objectDefinitionId2,
boolean reverse,
OrderByComparator orderByComparator)
throws NoSuchObjectRelationshipException {
ObjectRelationship objectRelationship = findByPrimaryKey(
objectRelationshipId);
Session session = null;
try {
session = openSession();
ObjectRelationship[] array = new ObjectRelationshipImpl[3];
array[0] = getByODI2_R_PrevAndNext(
session, objectRelationship, objectDefinitionId2, reverse,
orderByComparator, true);
array[1] = objectRelationship;
array[2] = getByODI2_R_PrevAndNext(
session, objectRelationship, objectDefinitionId2, reverse,
orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected ObjectRelationship getByODI2_R_PrevAndNext(
Session session, ObjectRelationship objectRelationship,
long objectDefinitionId2, boolean reverse,
OrderByComparator orderByComparator,
boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
5 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(4);
}
sb.append(_SQL_SELECT_OBJECTRELATIONSHIP_WHERE);
sb.append(_FINDER_COLUMN_ODI2_R_OBJECTDEFINITIONID2_2);
sb.append(_FINDER_COLUMN_ODI2_R_REVERSE_2);
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(ObjectRelationshipModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(objectDefinitionId2);
queryPos.add(reverse);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
objectRelationship)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the object relationships where objectDefinitionId2 = ? and reverse = ? from the database.
*
* @param objectDefinitionId2 the object definition id2
* @param reverse the reverse
*/
@Override
public void removeByODI2_R(long objectDefinitionId2, boolean reverse) {
for (ObjectRelationship objectRelationship :
findByODI2_R(
objectDefinitionId2, reverse, QueryUtil.ALL_POS,
QueryUtil.ALL_POS, null)) {
remove(objectRelationship);
}
}
/**
* Returns the number of object relationships where objectDefinitionId2 = ? and reverse = ?.
*
* @param objectDefinitionId2 the object definition id2
* @param reverse the reverse
* @return the number of matching object relationships
*/
@Override
public int countByODI2_R(long objectDefinitionId2, boolean reverse) {
FinderPath finderPath = _finderPathCountByODI2_R;
Object[] finderArgs = new Object[] {objectDefinitionId2, reverse};
Long count = (Long)finderCache.getResult(finderPath, finderArgs, this);
if (count == null) {
StringBundler sb = new StringBundler(3);
sb.append(_SQL_COUNT_OBJECTRELATIONSHIP_WHERE);
sb.append(_FINDER_COLUMN_ODI2_R_OBJECTDEFINITIONID2_2);
sb.append(_FINDER_COLUMN_ODI2_R_REVERSE_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(objectDefinitionId2);
queryPos.add(reverse);
count = (Long)query.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String _FINDER_COLUMN_ODI2_R_OBJECTDEFINITIONID2_2 =
"objectRelationship.objectDefinitionId2 = ? AND ";
private static final String _FINDER_COLUMN_ODI2_R_REVERSE_2 =
"objectRelationship.reverse = ?";
private FinderPath _finderPathFetchByDTN_R;
/**
* Returns the object relationship where dbTableName = ? and reverse = ? or throws a NoSuchObjectRelationshipException
if it could not be found.
*
* @param dbTableName the db table name
* @param reverse the reverse
* @return the matching object relationship
* @throws NoSuchObjectRelationshipException if a matching object relationship could not be found
*/
@Override
public ObjectRelationship findByDTN_R(String dbTableName, boolean reverse)
throws NoSuchObjectRelationshipException {
ObjectRelationship objectRelationship = fetchByDTN_R(
dbTableName, reverse);
if (objectRelationship == null) {
StringBundler sb = new StringBundler(6);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("dbTableName=");
sb.append(dbTableName);
sb.append(", reverse=");
sb.append(reverse);
sb.append("}");
if (_log.isDebugEnabled()) {
_log.debug(sb.toString());
}
throw new NoSuchObjectRelationshipException(sb.toString());
}
return objectRelationship;
}
/**
* Returns the object relationship where dbTableName = ? and reverse = ? or returns null
if it could not be found. Uses the finder cache.
*
* @param dbTableName the db table name
* @param reverse the reverse
* @return the matching object relationship, or null
if a matching object relationship could not be found
*/
@Override
public ObjectRelationship fetchByDTN_R(
String dbTableName, boolean reverse) {
return fetchByDTN_R(dbTableName, reverse, true);
}
/**
* Returns the object relationship where dbTableName = ? and reverse = ? or returns null
if it could not be found, optionally using the finder cache.
*
* @param dbTableName the db table name
* @param reverse the reverse
* @param useFinderCache whether to use the finder cache
* @return the matching object relationship, or null
if a matching object relationship could not be found
*/
@Override
public ObjectRelationship fetchByDTN_R(
String dbTableName, boolean reverse, boolean useFinderCache) {
dbTableName = Objects.toString(dbTableName, "");
Object[] finderArgs = null;
if (useFinderCache) {
finderArgs = new Object[] {dbTableName, reverse};
}
Object result = null;
if (useFinderCache) {
result = finderCache.getResult(
_finderPathFetchByDTN_R, finderArgs, this);
}
if (result instanceof ObjectRelationship) {
ObjectRelationship objectRelationship = (ObjectRelationship)result;
if (!Objects.equals(
dbTableName, objectRelationship.getDBTableName()) ||
(reverse != objectRelationship.isReverse())) {
result = null;
}
}
if (result == null) {
StringBundler sb = new StringBundler(4);
sb.append(_SQL_SELECT_OBJECTRELATIONSHIP_WHERE);
boolean bindDBTableName = false;
if (dbTableName.isEmpty()) {
sb.append(_FINDER_COLUMN_DTN_R_DBTABLENAME_3);
}
else {
bindDBTableName = true;
sb.append(_FINDER_COLUMN_DTN_R_DBTABLENAME_2);
}
sb.append(_FINDER_COLUMN_DTN_R_REVERSE_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
if (bindDBTableName) {
queryPos.add(dbTableName);
}
queryPos.add(reverse);
List list = query.list();
if (list.isEmpty()) {
if (useFinderCache) {
finderCache.putResult(
_finderPathFetchByDTN_R, finderArgs, list);
}
}
else {
if (list.size() > 1) {
Collections.sort(list, Collections.reverseOrder());
if (_log.isWarnEnabled()) {
if (!useFinderCache) {
finderArgs = new Object[] {
dbTableName, reverse
};
}
_log.warn(
"ObjectRelationshipPersistenceImpl.fetchByDTN_R(String, boolean, boolean) with parameters (" +
StringUtil.merge(finderArgs) +
") yields a result set with more than 1 result. This violates the logical unique restriction. There is no order guarantee on which result is returned by this finder.");
}
}
ObjectRelationship objectRelationship = list.get(0);
result = objectRelationship;
cacheResult(objectRelationship);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
if (result instanceof List>) {
return null;
}
else {
return (ObjectRelationship)result;
}
}
/**
* Removes the object relationship where dbTableName = ? and reverse = ? from the database.
*
* @param dbTableName the db table name
* @param reverse the reverse
* @return the object relationship that was removed
*/
@Override
public ObjectRelationship removeByDTN_R(String dbTableName, boolean reverse)
throws NoSuchObjectRelationshipException {
ObjectRelationship objectRelationship = findByDTN_R(
dbTableName, reverse);
return remove(objectRelationship);
}
/**
* Returns the number of object relationships where dbTableName = ? and reverse = ?.
*
* @param dbTableName the db table name
* @param reverse the reverse
* @return the number of matching object relationships
*/
@Override
public int countByDTN_R(String dbTableName, boolean reverse) {
ObjectRelationship objectRelationship = fetchByDTN_R(
dbTableName, reverse);
if (objectRelationship == null) {
return 0;
}
return 1;
}
private static final String _FINDER_COLUMN_DTN_R_DBTABLENAME_2 =
"objectRelationship.dbTableName = ? AND ";
private static final String _FINDER_COLUMN_DTN_R_DBTABLENAME_3 =
"(objectRelationship.dbTableName IS NULL OR objectRelationship.dbTableName = '') AND ";
private static final String _FINDER_COLUMN_DTN_R_REVERSE_2 =
"objectRelationship.reverse = ?";
private FinderPath _finderPathFetchByERC_C_ODI1;
/**
* Returns the object relationship where externalReferenceCode = ? and companyId = ? and objectDefinitionId1 = ? or throws a NoSuchObjectRelationshipException
if it could not be found.
*
* @param externalReferenceCode the external reference code
* @param companyId the company ID
* @param objectDefinitionId1 the object definition id1
* @return the matching object relationship
* @throws NoSuchObjectRelationshipException if a matching object relationship could not be found
*/
@Override
public ObjectRelationship findByERC_C_ODI1(
String externalReferenceCode, long companyId,
long objectDefinitionId1)
throws NoSuchObjectRelationshipException {
ObjectRelationship objectRelationship = fetchByERC_C_ODI1(
externalReferenceCode, companyId, objectDefinitionId1);
if (objectRelationship == null) {
StringBundler sb = new StringBundler(8);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("externalReferenceCode=");
sb.append(externalReferenceCode);
sb.append(", companyId=");
sb.append(companyId);
sb.append(", objectDefinitionId1=");
sb.append(objectDefinitionId1);
sb.append("}");
if (_log.isDebugEnabled()) {
_log.debug(sb.toString());
}
throw new NoSuchObjectRelationshipException(sb.toString());
}
return objectRelationship;
}
/**
* Returns the object relationship where externalReferenceCode = ? and companyId = ? and objectDefinitionId1 = ? or returns null
if it could not be found. Uses the finder cache.
*
* @param externalReferenceCode the external reference code
* @param companyId the company ID
* @param objectDefinitionId1 the object definition id1
* @return the matching object relationship, or null
if a matching object relationship could not be found
*/
@Override
public ObjectRelationship fetchByERC_C_ODI1(
String externalReferenceCode, long companyId,
long objectDefinitionId1) {
return fetchByERC_C_ODI1(
externalReferenceCode, companyId, objectDefinitionId1, true);
}
/**
* Returns the object relationship where externalReferenceCode = ? and companyId = ? and objectDefinitionId1 = ? or returns null
if it could not be found, optionally using the finder cache.
*
* @param externalReferenceCode the external reference code
* @param companyId the company ID
* @param objectDefinitionId1 the object definition id1
* @param useFinderCache whether to use the finder cache
* @return the matching object relationship, or null
if a matching object relationship could not be found
*/
@Override
public ObjectRelationship fetchByERC_C_ODI1(
String externalReferenceCode, long companyId, long objectDefinitionId1,
boolean useFinderCache) {
externalReferenceCode = Objects.toString(externalReferenceCode, "");
Object[] finderArgs = null;
if (useFinderCache) {
finderArgs = new Object[] {
externalReferenceCode, companyId, objectDefinitionId1
};
}
Object result = null;
if (useFinderCache) {
result = finderCache.getResult(
_finderPathFetchByERC_C_ODI1, finderArgs, this);
}
if (result instanceof ObjectRelationship) {
ObjectRelationship objectRelationship = (ObjectRelationship)result;
if (!Objects.equals(
externalReferenceCode,
objectRelationship.getExternalReferenceCode()) ||
(companyId != objectRelationship.getCompanyId()) ||
(objectDefinitionId1 !=
objectRelationship.getObjectDefinitionId1())) {
result = null;
}
}
if (result == null) {
StringBundler sb = new StringBundler(5);
sb.append(_SQL_SELECT_OBJECTRELATIONSHIP_WHERE);
boolean bindExternalReferenceCode = false;
if (externalReferenceCode.isEmpty()) {
sb.append(_FINDER_COLUMN_ERC_C_ODI1_EXTERNALREFERENCECODE_3);
}
else {
bindExternalReferenceCode = true;
sb.append(_FINDER_COLUMN_ERC_C_ODI1_EXTERNALREFERENCECODE_2);
}
sb.append(_FINDER_COLUMN_ERC_C_ODI1_COMPANYID_2);
sb.append(_FINDER_COLUMN_ERC_C_ODI1_OBJECTDEFINITIONID1_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
if (bindExternalReferenceCode) {
queryPos.add(externalReferenceCode);
}
queryPos.add(companyId);
queryPos.add(objectDefinitionId1);
List list = query.list();
if (list.isEmpty()) {
if (useFinderCache) {
finderCache.putResult(
_finderPathFetchByERC_C_ODI1, finderArgs, list);
}
}
else {
if (list.size() > 1) {
Collections.sort(list, Collections.reverseOrder());
if (_log.isWarnEnabled()) {
if (!useFinderCache) {
finderArgs = new Object[] {
externalReferenceCode, companyId,
objectDefinitionId1
};
}
_log.warn(
"ObjectRelationshipPersistenceImpl.fetchByERC_C_ODI1(String, long, long, boolean) with parameters (" +
StringUtil.merge(finderArgs) +
") yields a result set with more than 1 result. This violates the logical unique restriction. There is no order guarantee on which result is returned by this finder.");
}
}
ObjectRelationship objectRelationship = list.get(0);
result = objectRelationship;
cacheResult(objectRelationship);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
if (result instanceof List>) {
return null;
}
else {
return (ObjectRelationship)result;
}
}
/**
* Removes the object relationship where externalReferenceCode = ? and companyId = ? and objectDefinitionId1 = ? from the database.
*
* @param externalReferenceCode the external reference code
* @param companyId the company ID
* @param objectDefinitionId1 the object definition id1
* @return the object relationship that was removed
*/
@Override
public ObjectRelationship removeByERC_C_ODI1(
String externalReferenceCode, long companyId,
long objectDefinitionId1)
throws NoSuchObjectRelationshipException {
ObjectRelationship objectRelationship = findByERC_C_ODI1(
externalReferenceCode, companyId, objectDefinitionId1);
return remove(objectRelationship);
}
/**
* Returns the number of object relationships where externalReferenceCode = ? and companyId = ? and objectDefinitionId1 = ?.
*
* @param externalReferenceCode the external reference code
* @param companyId the company ID
* @param objectDefinitionId1 the object definition id1
* @return the number of matching object relationships
*/
@Override
public int countByERC_C_ODI1(
String externalReferenceCode, long companyId,
long objectDefinitionId1) {
ObjectRelationship objectRelationship = fetchByERC_C_ODI1(
externalReferenceCode, companyId, objectDefinitionId1);
if (objectRelationship == null) {
return 0;
}
return 1;
}
private static final String
_FINDER_COLUMN_ERC_C_ODI1_EXTERNALREFERENCECODE_2 =
"objectRelationship.externalReferenceCode = ? AND ";
private static final String
_FINDER_COLUMN_ERC_C_ODI1_EXTERNALREFERENCECODE_3 =
"(objectRelationship.externalReferenceCode IS NULL OR objectRelationship.externalReferenceCode = '') AND ";
private static final String _FINDER_COLUMN_ERC_C_ODI1_COMPANYID_2 =
"objectRelationship.companyId = ? AND ";
private static final String
_FINDER_COLUMN_ERC_C_ODI1_OBJECTDEFINITIONID1_2 =
"objectRelationship.objectDefinitionId1 = ?";
private FinderPath _finderPathWithPaginationFindByODI1_ODI2_T;
private FinderPath _finderPathWithoutPaginationFindByODI1_ODI2_T;
private FinderPath _finderPathCountByODI1_ODI2_T;
/**
* Returns all the object relationships where objectDefinitionId1 = ? and objectDefinitionId2 = ? and type = ?.
*
* @param objectDefinitionId1 the object definition id1
* @param objectDefinitionId2 the object definition id2
* @param type the type
* @return the matching object relationships
*/
@Override
public List findByODI1_ODI2_T(
long objectDefinitionId1, long objectDefinitionId2, String type) {
return findByODI1_ODI2_T(
objectDefinitionId1, objectDefinitionId2, type, QueryUtil.ALL_POS,
QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the object relationships where objectDefinitionId1 = ? and objectDefinitionId2 = ? and type = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectRelationshipModelImpl
.
*
*
* @param objectDefinitionId1 the object definition id1
* @param objectDefinitionId2 the object definition id2
* @param type the type
* @param start the lower bound of the range of object relationships
* @param end the upper bound of the range of object relationships (not inclusive)
* @return the range of matching object relationships
*/
@Override
public List findByODI1_ODI2_T(
long objectDefinitionId1, long objectDefinitionId2, String type,
int start, int end) {
return findByODI1_ODI2_T(
objectDefinitionId1, objectDefinitionId2, type, start, end, null);
}
/**
* Returns an ordered range of all the object relationships where objectDefinitionId1 = ? and objectDefinitionId2 = ? and type = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectRelationshipModelImpl
.
*
*
* @param objectDefinitionId1 the object definition id1
* @param objectDefinitionId2 the object definition id2
* @param type the type
* @param start the lower bound of the range of object relationships
* @param end the upper bound of the range of object relationships (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching object relationships
*/
@Override
public List findByODI1_ODI2_T(
long objectDefinitionId1, long objectDefinitionId2, String type,
int start, int end,
OrderByComparator orderByComparator) {
return findByODI1_ODI2_T(
objectDefinitionId1, objectDefinitionId2, type, start, end,
orderByComparator, true);
}
/**
* Returns an ordered range of all the object relationships where objectDefinitionId1 = ? and objectDefinitionId2 = ? and type = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectRelationshipModelImpl
.
*
*
* @param objectDefinitionId1 the object definition id1
* @param objectDefinitionId2 the object definition id2
* @param type the type
* @param start the lower bound of the range of object relationships
* @param end the upper bound of the range of object relationships (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching object relationships
*/
@Override
public List findByODI1_ODI2_T(
long objectDefinitionId1, long objectDefinitionId2, String type,
int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
type = Objects.toString(type, "");
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache) {
finderPath = _finderPathWithoutPaginationFindByODI1_ODI2_T;
finderArgs = new Object[] {
objectDefinitionId1, objectDefinitionId2, type
};
}
}
else if (useFinderCache) {
finderPath = _finderPathWithPaginationFindByODI1_ODI2_T;
finderArgs = new Object[] {
objectDefinitionId1, objectDefinitionId2, type, start, end,
orderByComparator
};
}
List list = null;
if (useFinderCache) {
list = (List)finderCache.getResult(
finderPath, finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (ObjectRelationship objectRelationship : list) {
if ((objectDefinitionId1 !=
objectRelationship.getObjectDefinitionId1()) ||
(objectDefinitionId2 !=
objectRelationship.getObjectDefinitionId2()) ||
!type.equals(objectRelationship.getType())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
5 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(5);
}
sb.append(_SQL_SELECT_OBJECTRELATIONSHIP_WHERE);
sb.append(_FINDER_COLUMN_ODI1_ODI2_T_OBJECTDEFINITIONID1_2);
sb.append(_FINDER_COLUMN_ODI1_ODI2_T_OBJECTDEFINITIONID2_2);
boolean bindType = false;
if (type.isEmpty()) {
sb.append(_FINDER_COLUMN_ODI1_ODI2_T_TYPE_3);
}
else {
bindType = true;
sb.append(_FINDER_COLUMN_ODI1_ODI2_T_TYPE_2);
}
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(ObjectRelationshipModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(objectDefinitionId1);
queryPos.add(objectDefinitionId2);
if (bindType) {
queryPos.add(type);
}
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first object relationship in the ordered set where objectDefinitionId1 = ? and objectDefinitionId2 = ? and type = ?.
*
* @param objectDefinitionId1 the object definition id1
* @param objectDefinitionId2 the object definition id2
* @param type the type
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching object relationship
* @throws NoSuchObjectRelationshipException if a matching object relationship could not be found
*/
@Override
public ObjectRelationship findByODI1_ODI2_T_First(
long objectDefinitionId1, long objectDefinitionId2, String type,
OrderByComparator orderByComparator)
throws NoSuchObjectRelationshipException {
ObjectRelationship objectRelationship = fetchByODI1_ODI2_T_First(
objectDefinitionId1, objectDefinitionId2, type, orderByComparator);
if (objectRelationship != null) {
return objectRelationship;
}
StringBundler sb = new StringBundler(8);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("objectDefinitionId1=");
sb.append(objectDefinitionId1);
sb.append(", objectDefinitionId2=");
sb.append(objectDefinitionId2);
sb.append(", type=");
sb.append(type);
sb.append("}");
throw new NoSuchObjectRelationshipException(sb.toString());
}
/**
* Returns the first object relationship in the ordered set where objectDefinitionId1 = ? and objectDefinitionId2 = ? and type = ?.
*
* @param objectDefinitionId1 the object definition id1
* @param objectDefinitionId2 the object definition id2
* @param type the type
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching object relationship, or null
if a matching object relationship could not be found
*/
@Override
public ObjectRelationship fetchByODI1_ODI2_T_First(
long objectDefinitionId1, long objectDefinitionId2, String type,
OrderByComparator orderByComparator) {
List list = findByODI1_ODI2_T(
objectDefinitionId1, objectDefinitionId2, type, 0, 1,
orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last object relationship in the ordered set where objectDefinitionId1 = ? and objectDefinitionId2 = ? and type = ?.
*
* @param objectDefinitionId1 the object definition id1
* @param objectDefinitionId2 the object definition id2
* @param type the type
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching object relationship
* @throws NoSuchObjectRelationshipException if a matching object relationship could not be found
*/
@Override
public ObjectRelationship findByODI1_ODI2_T_Last(
long objectDefinitionId1, long objectDefinitionId2, String type,
OrderByComparator orderByComparator)
throws NoSuchObjectRelationshipException {
ObjectRelationship objectRelationship = fetchByODI1_ODI2_T_Last(
objectDefinitionId1, objectDefinitionId2, type, orderByComparator);
if (objectRelationship != null) {
return objectRelationship;
}
StringBundler sb = new StringBundler(8);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("objectDefinitionId1=");
sb.append(objectDefinitionId1);
sb.append(", objectDefinitionId2=");
sb.append(objectDefinitionId2);
sb.append(", type=");
sb.append(type);
sb.append("}");
throw new NoSuchObjectRelationshipException(sb.toString());
}
/**
* Returns the last object relationship in the ordered set where objectDefinitionId1 = ? and objectDefinitionId2 = ? and type = ?.
*
* @param objectDefinitionId1 the object definition id1
* @param objectDefinitionId2 the object definition id2
* @param type the type
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching object relationship, or null
if a matching object relationship could not be found
*/
@Override
public ObjectRelationship fetchByODI1_ODI2_T_Last(
long objectDefinitionId1, long objectDefinitionId2, String type,
OrderByComparator orderByComparator) {
int count = countByODI1_ODI2_T(
objectDefinitionId1, objectDefinitionId2, type);
if (count == 0) {
return null;
}
List list = findByODI1_ODI2_T(
objectDefinitionId1, objectDefinitionId2, type, count - 1, count,
orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the object relationships before and after the current object relationship in the ordered set where objectDefinitionId1 = ? and objectDefinitionId2 = ? and type = ?.
*
* @param objectRelationshipId the primary key of the current object relationship
* @param objectDefinitionId1 the object definition id1
* @param objectDefinitionId2 the object definition id2
* @param type the type
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next object relationship
* @throws NoSuchObjectRelationshipException if a object relationship with the primary key could not be found
*/
@Override
public ObjectRelationship[] findByODI1_ODI2_T_PrevAndNext(
long objectRelationshipId, long objectDefinitionId1,
long objectDefinitionId2, String type,
OrderByComparator orderByComparator)
throws NoSuchObjectRelationshipException {
type = Objects.toString(type, "");
ObjectRelationship objectRelationship = findByPrimaryKey(
objectRelationshipId);
Session session = null;
try {
session = openSession();
ObjectRelationship[] array = new ObjectRelationshipImpl[3];
array[0] = getByODI1_ODI2_T_PrevAndNext(
session, objectRelationship, objectDefinitionId1,
objectDefinitionId2, type, orderByComparator, true);
array[1] = objectRelationship;
array[2] = getByODI1_ODI2_T_PrevAndNext(
session, objectRelationship, objectDefinitionId1,
objectDefinitionId2, type, orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected ObjectRelationship getByODI1_ODI2_T_PrevAndNext(
Session session, ObjectRelationship objectRelationship,
long objectDefinitionId1, long objectDefinitionId2, String type,
OrderByComparator orderByComparator,
boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
6 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(5);
}
sb.append(_SQL_SELECT_OBJECTRELATIONSHIP_WHERE);
sb.append(_FINDER_COLUMN_ODI1_ODI2_T_OBJECTDEFINITIONID1_2);
sb.append(_FINDER_COLUMN_ODI1_ODI2_T_OBJECTDEFINITIONID2_2);
boolean bindType = false;
if (type.isEmpty()) {
sb.append(_FINDER_COLUMN_ODI1_ODI2_T_TYPE_3);
}
else {
bindType = true;
sb.append(_FINDER_COLUMN_ODI1_ODI2_T_TYPE_2);
}
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(ObjectRelationshipModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(objectDefinitionId1);
queryPos.add(objectDefinitionId2);
if (bindType) {
queryPos.add(type);
}
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
objectRelationship)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the object relationships where objectDefinitionId1 = ? and objectDefinitionId2 = ? and type = ? from the database.
*
* @param objectDefinitionId1 the object definition id1
* @param objectDefinitionId2 the object definition id2
* @param type the type
*/
@Override
public void removeByODI1_ODI2_T(
long objectDefinitionId1, long objectDefinitionId2, String type) {
for (ObjectRelationship objectRelationship :
findByODI1_ODI2_T(
objectDefinitionId1, objectDefinitionId2, type,
QueryUtil.ALL_POS, QueryUtil.ALL_POS, null)) {
remove(objectRelationship);
}
}
/**
* Returns the number of object relationships where objectDefinitionId1 = ? and objectDefinitionId2 = ? and type = ?.
*
* @param objectDefinitionId1 the object definition id1
* @param objectDefinitionId2 the object definition id2
* @param type the type
* @return the number of matching object relationships
*/
@Override
public int countByODI1_ODI2_T(
long objectDefinitionId1, long objectDefinitionId2, String type) {
type = Objects.toString(type, "");
FinderPath finderPath = _finderPathCountByODI1_ODI2_T;
Object[] finderArgs = new Object[] {
objectDefinitionId1, objectDefinitionId2, type
};
Long count = (Long)finderCache.getResult(finderPath, finderArgs, this);
if (count == null) {
StringBundler sb = new StringBundler(4);
sb.append(_SQL_COUNT_OBJECTRELATIONSHIP_WHERE);
sb.append(_FINDER_COLUMN_ODI1_ODI2_T_OBJECTDEFINITIONID1_2);
sb.append(_FINDER_COLUMN_ODI1_ODI2_T_OBJECTDEFINITIONID2_2);
boolean bindType = false;
if (type.isEmpty()) {
sb.append(_FINDER_COLUMN_ODI1_ODI2_T_TYPE_3);
}
else {
bindType = true;
sb.append(_FINDER_COLUMN_ODI1_ODI2_T_TYPE_2);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(objectDefinitionId1);
queryPos.add(objectDefinitionId2);
if (bindType) {
queryPos.add(type);
}
count = (Long)query.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String
_FINDER_COLUMN_ODI1_ODI2_T_OBJECTDEFINITIONID1_2 =
"objectRelationship.objectDefinitionId1 = ? AND ";
private static final String
_FINDER_COLUMN_ODI1_ODI2_T_OBJECTDEFINITIONID2_2 =
"objectRelationship.objectDefinitionId2 = ? AND ";
private static final String _FINDER_COLUMN_ODI1_ODI2_T_TYPE_2 =
"objectRelationship.type = ?";
private static final String _FINDER_COLUMN_ODI1_ODI2_T_TYPE_3 =
"(objectRelationship.type IS NULL OR objectRelationship.type = '')";
private FinderPath _finderPathWithPaginationFindByODI1_DT_R;
private FinderPath _finderPathWithoutPaginationFindByODI1_DT_R;
private FinderPath _finderPathCountByODI1_DT_R;
/**
* Returns all the object relationships where objectDefinitionId1 = ? and deletionType = ? and reverse = ?.
*
* @param objectDefinitionId1 the object definition id1
* @param deletionType the deletion type
* @param reverse the reverse
* @return the matching object relationships
*/
@Override
public List findByODI1_DT_R(
long objectDefinitionId1, String deletionType, boolean reverse) {
return findByODI1_DT_R(
objectDefinitionId1, deletionType, reverse, QueryUtil.ALL_POS,
QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the object relationships where objectDefinitionId1 = ? and deletionType = ? and reverse = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectRelationshipModelImpl
.
*
*
* @param objectDefinitionId1 the object definition id1
* @param deletionType the deletion type
* @param reverse the reverse
* @param start the lower bound of the range of object relationships
* @param end the upper bound of the range of object relationships (not inclusive)
* @return the range of matching object relationships
*/
@Override
public List findByODI1_DT_R(
long objectDefinitionId1, String deletionType, boolean reverse,
int start, int end) {
return findByODI1_DT_R(
objectDefinitionId1, deletionType, reverse, start, end, null);
}
/**
* Returns an ordered range of all the object relationships where objectDefinitionId1 = ? and deletionType = ? and reverse = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectRelationshipModelImpl
.
*
*
* @param objectDefinitionId1 the object definition id1
* @param deletionType the deletion type
* @param reverse the reverse
* @param start the lower bound of the range of object relationships
* @param end the upper bound of the range of object relationships (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching object relationships
*/
@Override
public List findByODI1_DT_R(
long objectDefinitionId1, String deletionType, boolean reverse,
int start, int end,
OrderByComparator orderByComparator) {
return findByODI1_DT_R(
objectDefinitionId1, deletionType, reverse, start, end,
orderByComparator, true);
}
/**
* Returns an ordered range of all the object relationships where objectDefinitionId1 = ? and deletionType = ? and reverse = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectRelationshipModelImpl
.
*
*
* @param objectDefinitionId1 the object definition id1
* @param deletionType the deletion type
* @param reverse the reverse
* @param start the lower bound of the range of object relationships
* @param end the upper bound of the range of object relationships (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching object relationships
*/
@Override
public List findByODI1_DT_R(
long objectDefinitionId1, String deletionType, boolean reverse,
int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
deletionType = Objects.toString(deletionType, "");
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache) {
finderPath = _finderPathWithoutPaginationFindByODI1_DT_R;
finderArgs = new Object[] {
objectDefinitionId1, deletionType, reverse
};
}
}
else if (useFinderCache) {
finderPath = _finderPathWithPaginationFindByODI1_DT_R;
finderArgs = new Object[] {
objectDefinitionId1, deletionType, reverse, start, end,
orderByComparator
};
}
List list = null;
if (useFinderCache) {
list = (List)finderCache.getResult(
finderPath, finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (ObjectRelationship objectRelationship : list) {
if ((objectDefinitionId1 !=
objectRelationship.getObjectDefinitionId1()) ||
!deletionType.equals(
objectRelationship.getDeletionType()) ||
(reverse != objectRelationship.isReverse())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
5 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(5);
}
sb.append(_SQL_SELECT_OBJECTRELATIONSHIP_WHERE);
sb.append(_FINDER_COLUMN_ODI1_DT_R_OBJECTDEFINITIONID1_2);
boolean bindDeletionType = false;
if (deletionType.isEmpty()) {
sb.append(_FINDER_COLUMN_ODI1_DT_R_DELETIONTYPE_3);
}
else {
bindDeletionType = true;
sb.append(_FINDER_COLUMN_ODI1_DT_R_DELETIONTYPE_2);
}
sb.append(_FINDER_COLUMN_ODI1_DT_R_REVERSE_2);
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(ObjectRelationshipModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(objectDefinitionId1);
if (bindDeletionType) {
queryPos.add(deletionType);
}
queryPos.add(reverse);
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first object relationship in the ordered set where objectDefinitionId1 = ? and deletionType = ? and reverse = ?.
*
* @param objectDefinitionId1 the object definition id1
* @param deletionType the deletion type
* @param reverse the reverse
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching object relationship
* @throws NoSuchObjectRelationshipException if a matching object relationship could not be found
*/
@Override
public ObjectRelationship findByODI1_DT_R_First(
long objectDefinitionId1, String deletionType, boolean reverse,
OrderByComparator orderByComparator)
throws NoSuchObjectRelationshipException {
ObjectRelationship objectRelationship = fetchByODI1_DT_R_First(
objectDefinitionId1, deletionType, reverse, orderByComparator);
if (objectRelationship != null) {
return objectRelationship;
}
StringBundler sb = new StringBundler(8);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("objectDefinitionId1=");
sb.append(objectDefinitionId1);
sb.append(", deletionType=");
sb.append(deletionType);
sb.append(", reverse=");
sb.append(reverse);
sb.append("}");
throw new NoSuchObjectRelationshipException(sb.toString());
}
/**
* Returns the first object relationship in the ordered set where objectDefinitionId1 = ? and deletionType = ? and reverse = ?.
*
* @param objectDefinitionId1 the object definition id1
* @param deletionType the deletion type
* @param reverse the reverse
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching object relationship, or null
if a matching object relationship could not be found
*/
@Override
public ObjectRelationship fetchByODI1_DT_R_First(
long objectDefinitionId1, String deletionType, boolean reverse,
OrderByComparator orderByComparator) {
List list = findByODI1_DT_R(
objectDefinitionId1, deletionType, reverse, 0, 1,
orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last object relationship in the ordered set where objectDefinitionId1 = ? and deletionType = ? and reverse = ?.
*
* @param objectDefinitionId1 the object definition id1
* @param deletionType the deletion type
* @param reverse the reverse
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching object relationship
* @throws NoSuchObjectRelationshipException if a matching object relationship could not be found
*/
@Override
public ObjectRelationship findByODI1_DT_R_Last(
long objectDefinitionId1, String deletionType, boolean reverse,
OrderByComparator orderByComparator)
throws NoSuchObjectRelationshipException {
ObjectRelationship objectRelationship = fetchByODI1_DT_R_Last(
objectDefinitionId1, deletionType, reverse, orderByComparator);
if (objectRelationship != null) {
return objectRelationship;
}
StringBundler sb = new StringBundler(8);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("objectDefinitionId1=");
sb.append(objectDefinitionId1);
sb.append(", deletionType=");
sb.append(deletionType);
sb.append(", reverse=");
sb.append(reverse);
sb.append("}");
throw new NoSuchObjectRelationshipException(sb.toString());
}
/**
* Returns the last object relationship in the ordered set where objectDefinitionId1 = ? and deletionType = ? and reverse = ?.
*
* @param objectDefinitionId1 the object definition id1
* @param deletionType the deletion type
* @param reverse the reverse
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching object relationship, or null
if a matching object relationship could not be found
*/
@Override
public ObjectRelationship fetchByODI1_DT_R_Last(
long objectDefinitionId1, String deletionType, boolean reverse,
OrderByComparator orderByComparator) {
int count = countByODI1_DT_R(
objectDefinitionId1, deletionType, reverse);
if (count == 0) {
return null;
}
List list = findByODI1_DT_R(
objectDefinitionId1, deletionType, reverse, count - 1, count,
orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the object relationships before and after the current object relationship in the ordered set where objectDefinitionId1 = ? and deletionType = ? and reverse = ?.
*
* @param objectRelationshipId the primary key of the current object relationship
* @param objectDefinitionId1 the object definition id1
* @param deletionType the deletion type
* @param reverse the reverse
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next object relationship
* @throws NoSuchObjectRelationshipException if a object relationship with the primary key could not be found
*/
@Override
public ObjectRelationship[] findByODI1_DT_R_PrevAndNext(
long objectRelationshipId, long objectDefinitionId1,
String deletionType, boolean reverse,
OrderByComparator orderByComparator)
throws NoSuchObjectRelationshipException {
deletionType = Objects.toString(deletionType, "");
ObjectRelationship objectRelationship = findByPrimaryKey(
objectRelationshipId);
Session session = null;
try {
session = openSession();
ObjectRelationship[] array = new ObjectRelationshipImpl[3];
array[0] = getByODI1_DT_R_PrevAndNext(
session, objectRelationship, objectDefinitionId1, deletionType,
reverse, orderByComparator, true);
array[1] = objectRelationship;
array[2] = getByODI1_DT_R_PrevAndNext(
session, objectRelationship, objectDefinitionId1, deletionType,
reverse, orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected ObjectRelationship getByODI1_DT_R_PrevAndNext(
Session session, ObjectRelationship objectRelationship,
long objectDefinitionId1, String deletionType, boolean reverse,
OrderByComparator orderByComparator,
boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
6 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(5);
}
sb.append(_SQL_SELECT_OBJECTRELATIONSHIP_WHERE);
sb.append(_FINDER_COLUMN_ODI1_DT_R_OBJECTDEFINITIONID1_2);
boolean bindDeletionType = false;
if (deletionType.isEmpty()) {
sb.append(_FINDER_COLUMN_ODI1_DT_R_DELETIONTYPE_3);
}
else {
bindDeletionType = true;
sb.append(_FINDER_COLUMN_ODI1_DT_R_DELETIONTYPE_2);
}
sb.append(_FINDER_COLUMN_ODI1_DT_R_REVERSE_2);
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(ObjectRelationshipModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(objectDefinitionId1);
if (bindDeletionType) {
queryPos.add(deletionType);
}
queryPos.add(reverse);
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
objectRelationship)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the object relationships where objectDefinitionId1 = ? and deletionType = ? and reverse = ? from the database.
*
* @param objectDefinitionId1 the object definition id1
* @param deletionType the deletion type
* @param reverse the reverse
*/
@Override
public void removeByODI1_DT_R(
long objectDefinitionId1, String deletionType, boolean reverse) {
for (ObjectRelationship objectRelationship :
findByODI1_DT_R(
objectDefinitionId1, deletionType, reverse,
QueryUtil.ALL_POS, QueryUtil.ALL_POS, null)) {
remove(objectRelationship);
}
}
/**
* Returns the number of object relationships where objectDefinitionId1 = ? and deletionType = ? and reverse = ?.
*
* @param objectDefinitionId1 the object definition id1
* @param deletionType the deletion type
* @param reverse the reverse
* @return the number of matching object relationships
*/
@Override
public int countByODI1_DT_R(
long objectDefinitionId1, String deletionType, boolean reverse) {
deletionType = Objects.toString(deletionType, "");
FinderPath finderPath = _finderPathCountByODI1_DT_R;
Object[] finderArgs = new Object[] {
objectDefinitionId1, deletionType, reverse
};
Long count = (Long)finderCache.getResult(finderPath, finderArgs, this);
if (count == null) {
StringBundler sb = new StringBundler(4);
sb.append(_SQL_COUNT_OBJECTRELATIONSHIP_WHERE);
sb.append(_FINDER_COLUMN_ODI1_DT_R_OBJECTDEFINITIONID1_2);
boolean bindDeletionType = false;
if (deletionType.isEmpty()) {
sb.append(_FINDER_COLUMN_ODI1_DT_R_DELETIONTYPE_3);
}
else {
bindDeletionType = true;
sb.append(_FINDER_COLUMN_ODI1_DT_R_DELETIONTYPE_2);
}
sb.append(_FINDER_COLUMN_ODI1_DT_R_REVERSE_2);
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(objectDefinitionId1);
if (bindDeletionType) {
queryPos.add(deletionType);
}
queryPos.add(reverse);
count = (Long)query.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String _FINDER_COLUMN_ODI1_DT_R_OBJECTDEFINITIONID1_2 =
"objectRelationship.objectDefinitionId1 = ? AND ";
private static final String _FINDER_COLUMN_ODI1_DT_R_DELETIONTYPE_2 =
"objectRelationship.deletionType = ? AND ";
private static final String _FINDER_COLUMN_ODI1_DT_R_DELETIONTYPE_3 =
"(objectRelationship.deletionType IS NULL OR objectRelationship.deletionType = '') AND ";
private static final String _FINDER_COLUMN_ODI1_DT_R_REVERSE_2 =
"objectRelationship.reverse = ?";
private FinderPath _finderPathWithPaginationFindByODI1_R_T;
private FinderPath _finderPathWithoutPaginationFindByODI1_R_T;
private FinderPath _finderPathCountByODI1_R_T;
/**
* Returns all the object relationships where objectDefinitionId1 = ? and reverse = ? and type = ?.
*
* @param objectDefinitionId1 the object definition id1
* @param reverse the reverse
* @param type the type
* @return the matching object relationships
*/
@Override
public List findByODI1_R_T(
long objectDefinitionId1, boolean reverse, String type) {
return findByODI1_R_T(
objectDefinitionId1, reverse, type, QueryUtil.ALL_POS,
QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the object relationships where objectDefinitionId1 = ? and reverse = ? and type = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectRelationshipModelImpl
.
*
*
* @param objectDefinitionId1 the object definition id1
* @param reverse the reverse
* @param type the type
* @param start the lower bound of the range of object relationships
* @param end the upper bound of the range of object relationships (not inclusive)
* @return the range of matching object relationships
*/
@Override
public List findByODI1_R_T(
long objectDefinitionId1, boolean reverse, String type, int start,
int end) {
return findByODI1_R_T(
objectDefinitionId1, reverse, type, start, end, null);
}
/**
* Returns an ordered range of all the object relationships where objectDefinitionId1 = ? and reverse = ? and type = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectRelationshipModelImpl
.
*
*
* @param objectDefinitionId1 the object definition id1
* @param reverse the reverse
* @param type the type
* @param start the lower bound of the range of object relationships
* @param end the upper bound of the range of object relationships (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching object relationships
*/
@Override
public List findByODI1_R_T(
long objectDefinitionId1, boolean reverse, String type, int start,
int end, OrderByComparator orderByComparator) {
return findByODI1_R_T(
objectDefinitionId1, reverse, type, start, end, orderByComparator,
true);
}
/**
* Returns an ordered range of all the object relationships where objectDefinitionId1 = ? and reverse = ? and type = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectRelationshipModelImpl
.
*
*
* @param objectDefinitionId1 the object definition id1
* @param reverse the reverse
* @param type the type
* @param start the lower bound of the range of object relationships
* @param end the upper bound of the range of object relationships (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching object relationships
*/
@Override
public List findByODI1_R_T(
long objectDefinitionId1, boolean reverse, String type, int start,
int end, OrderByComparator orderByComparator,
boolean useFinderCache) {
type = Objects.toString(type, "");
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache) {
finderPath = _finderPathWithoutPaginationFindByODI1_R_T;
finderArgs = new Object[] {objectDefinitionId1, reverse, type};
}
}
else if (useFinderCache) {
finderPath = _finderPathWithPaginationFindByODI1_R_T;
finderArgs = new Object[] {
objectDefinitionId1, reverse, type, start, end,
orderByComparator
};
}
List list = null;
if (useFinderCache) {
list = (List)finderCache.getResult(
finderPath, finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (ObjectRelationship objectRelationship : list) {
if ((objectDefinitionId1 !=
objectRelationship.getObjectDefinitionId1()) ||
(reverse != objectRelationship.isReverse()) ||
!type.equals(objectRelationship.getType())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
5 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(5);
}
sb.append(_SQL_SELECT_OBJECTRELATIONSHIP_WHERE);
sb.append(_FINDER_COLUMN_ODI1_R_T_OBJECTDEFINITIONID1_2);
sb.append(_FINDER_COLUMN_ODI1_R_T_REVERSE_2);
boolean bindType = false;
if (type.isEmpty()) {
sb.append(_FINDER_COLUMN_ODI1_R_T_TYPE_3);
}
else {
bindType = true;
sb.append(_FINDER_COLUMN_ODI1_R_T_TYPE_2);
}
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(ObjectRelationshipModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(objectDefinitionId1);
queryPos.add(reverse);
if (bindType) {
queryPos.add(type);
}
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first object relationship in the ordered set where objectDefinitionId1 = ? and reverse = ? and type = ?.
*
* @param objectDefinitionId1 the object definition id1
* @param reverse the reverse
* @param type the type
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching object relationship
* @throws NoSuchObjectRelationshipException if a matching object relationship could not be found
*/
@Override
public ObjectRelationship findByODI1_R_T_First(
long objectDefinitionId1, boolean reverse, String type,
OrderByComparator orderByComparator)
throws NoSuchObjectRelationshipException {
ObjectRelationship objectRelationship = fetchByODI1_R_T_First(
objectDefinitionId1, reverse, type, orderByComparator);
if (objectRelationship != null) {
return objectRelationship;
}
StringBundler sb = new StringBundler(8);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("objectDefinitionId1=");
sb.append(objectDefinitionId1);
sb.append(", reverse=");
sb.append(reverse);
sb.append(", type=");
sb.append(type);
sb.append("}");
throw new NoSuchObjectRelationshipException(sb.toString());
}
/**
* Returns the first object relationship in the ordered set where objectDefinitionId1 = ? and reverse = ? and type = ?.
*
* @param objectDefinitionId1 the object definition id1
* @param reverse the reverse
* @param type the type
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching object relationship, or null
if a matching object relationship could not be found
*/
@Override
public ObjectRelationship fetchByODI1_R_T_First(
long objectDefinitionId1, boolean reverse, String type,
OrderByComparator orderByComparator) {
List list = findByODI1_R_T(
objectDefinitionId1, reverse, type, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last object relationship in the ordered set where objectDefinitionId1 = ? and reverse = ? and type = ?.
*
* @param objectDefinitionId1 the object definition id1
* @param reverse the reverse
* @param type the type
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching object relationship
* @throws NoSuchObjectRelationshipException if a matching object relationship could not be found
*/
@Override
public ObjectRelationship findByODI1_R_T_Last(
long objectDefinitionId1, boolean reverse, String type,
OrderByComparator orderByComparator)
throws NoSuchObjectRelationshipException {
ObjectRelationship objectRelationship = fetchByODI1_R_T_Last(
objectDefinitionId1, reverse, type, orderByComparator);
if (objectRelationship != null) {
return objectRelationship;
}
StringBundler sb = new StringBundler(8);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("objectDefinitionId1=");
sb.append(objectDefinitionId1);
sb.append(", reverse=");
sb.append(reverse);
sb.append(", type=");
sb.append(type);
sb.append("}");
throw new NoSuchObjectRelationshipException(sb.toString());
}
/**
* Returns the last object relationship in the ordered set where objectDefinitionId1 = ? and reverse = ? and type = ?.
*
* @param objectDefinitionId1 the object definition id1
* @param reverse the reverse
* @param type the type
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching object relationship, or null
if a matching object relationship could not be found
*/
@Override
public ObjectRelationship fetchByODI1_R_T_Last(
long objectDefinitionId1, boolean reverse, String type,
OrderByComparator orderByComparator) {
int count = countByODI1_R_T(objectDefinitionId1, reverse, type);
if (count == 0) {
return null;
}
List list = findByODI1_R_T(
objectDefinitionId1, reverse, type, count - 1, count,
orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the object relationships before and after the current object relationship in the ordered set where objectDefinitionId1 = ? and reverse = ? and type = ?.
*
* @param objectRelationshipId the primary key of the current object relationship
* @param objectDefinitionId1 the object definition id1
* @param reverse the reverse
* @param type the type
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next object relationship
* @throws NoSuchObjectRelationshipException if a object relationship with the primary key could not be found
*/
@Override
public ObjectRelationship[] findByODI1_R_T_PrevAndNext(
long objectRelationshipId, long objectDefinitionId1,
boolean reverse, String type,
OrderByComparator orderByComparator)
throws NoSuchObjectRelationshipException {
type = Objects.toString(type, "");
ObjectRelationship objectRelationship = findByPrimaryKey(
objectRelationshipId);
Session session = null;
try {
session = openSession();
ObjectRelationship[] array = new ObjectRelationshipImpl[3];
array[0] = getByODI1_R_T_PrevAndNext(
session, objectRelationship, objectDefinitionId1, reverse, type,
orderByComparator, true);
array[1] = objectRelationship;
array[2] = getByODI1_R_T_PrevAndNext(
session, objectRelationship, objectDefinitionId1, reverse, type,
orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected ObjectRelationship getByODI1_R_T_PrevAndNext(
Session session, ObjectRelationship objectRelationship,
long objectDefinitionId1, boolean reverse, String type,
OrderByComparator orderByComparator,
boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
6 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(5);
}
sb.append(_SQL_SELECT_OBJECTRELATIONSHIP_WHERE);
sb.append(_FINDER_COLUMN_ODI1_R_T_OBJECTDEFINITIONID1_2);
sb.append(_FINDER_COLUMN_ODI1_R_T_REVERSE_2);
boolean bindType = false;
if (type.isEmpty()) {
sb.append(_FINDER_COLUMN_ODI1_R_T_TYPE_3);
}
else {
bindType = true;
sb.append(_FINDER_COLUMN_ODI1_R_T_TYPE_2);
}
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(ObjectRelationshipModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(objectDefinitionId1);
queryPos.add(reverse);
if (bindType) {
queryPos.add(type);
}
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
objectRelationship)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the object relationships where objectDefinitionId1 = ? and reverse = ? and type = ? from the database.
*
* @param objectDefinitionId1 the object definition id1
* @param reverse the reverse
* @param type the type
*/
@Override
public void removeByODI1_R_T(
long objectDefinitionId1, boolean reverse, String type) {
for (ObjectRelationship objectRelationship :
findByODI1_R_T(
objectDefinitionId1, reverse, type, QueryUtil.ALL_POS,
QueryUtil.ALL_POS, null)) {
remove(objectRelationship);
}
}
/**
* Returns the number of object relationships where objectDefinitionId1 = ? and reverse = ? and type = ?.
*
* @param objectDefinitionId1 the object definition id1
* @param reverse the reverse
* @param type the type
* @return the number of matching object relationships
*/
@Override
public int countByODI1_R_T(
long objectDefinitionId1, boolean reverse, String type) {
type = Objects.toString(type, "");
FinderPath finderPath = _finderPathCountByODI1_R_T;
Object[] finderArgs = new Object[] {objectDefinitionId1, reverse, type};
Long count = (Long)finderCache.getResult(finderPath, finderArgs, this);
if (count == null) {
StringBundler sb = new StringBundler(4);
sb.append(_SQL_COUNT_OBJECTRELATIONSHIP_WHERE);
sb.append(_FINDER_COLUMN_ODI1_R_T_OBJECTDEFINITIONID1_2);
sb.append(_FINDER_COLUMN_ODI1_R_T_REVERSE_2);
boolean bindType = false;
if (type.isEmpty()) {
sb.append(_FINDER_COLUMN_ODI1_R_T_TYPE_3);
}
else {
bindType = true;
sb.append(_FINDER_COLUMN_ODI1_R_T_TYPE_2);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(objectDefinitionId1);
queryPos.add(reverse);
if (bindType) {
queryPos.add(type);
}
count = (Long)query.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String _FINDER_COLUMN_ODI1_R_T_OBJECTDEFINITIONID1_2 =
"objectRelationship.objectDefinitionId1 = ? AND ";
private static final String _FINDER_COLUMN_ODI1_R_T_REVERSE_2 =
"objectRelationship.reverse = ? AND ";
private static final String _FINDER_COLUMN_ODI1_R_T_TYPE_2 =
"objectRelationship.type = ?";
private static final String _FINDER_COLUMN_ODI1_R_T_TYPE_3 =
"(objectRelationship.type IS NULL OR objectRelationship.type = '')";
private FinderPath _finderPathWithPaginationFindByODI2_R_T;
private FinderPath _finderPathWithoutPaginationFindByODI2_R_T;
private FinderPath _finderPathCountByODI2_R_T;
/**
* Returns all the object relationships where objectDefinitionId2 = ? and reverse = ? and type = ?.
*
* @param objectDefinitionId2 the object definition id2
* @param reverse the reverse
* @param type the type
* @return the matching object relationships
*/
@Override
public List findByODI2_R_T(
long objectDefinitionId2, boolean reverse, String type) {
return findByODI2_R_T(
objectDefinitionId2, reverse, type, QueryUtil.ALL_POS,
QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the object relationships where objectDefinitionId2 = ? and reverse = ? and type = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectRelationshipModelImpl
.
*
*
* @param objectDefinitionId2 the object definition id2
* @param reverse the reverse
* @param type the type
* @param start the lower bound of the range of object relationships
* @param end the upper bound of the range of object relationships (not inclusive)
* @return the range of matching object relationships
*/
@Override
public List findByODI2_R_T(
long objectDefinitionId2, boolean reverse, String type, int start,
int end) {
return findByODI2_R_T(
objectDefinitionId2, reverse, type, start, end, null);
}
/**
* Returns an ordered range of all the object relationships where objectDefinitionId2 = ? and reverse = ? and type = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectRelationshipModelImpl
.
*
*
* @param objectDefinitionId2 the object definition id2
* @param reverse the reverse
* @param type the type
* @param start the lower bound of the range of object relationships
* @param end the upper bound of the range of object relationships (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching object relationships
*/
@Override
public List findByODI2_R_T(
long objectDefinitionId2, boolean reverse, String type, int start,
int end, OrderByComparator orderByComparator) {
return findByODI2_R_T(
objectDefinitionId2, reverse, type, start, end, orderByComparator,
true);
}
/**
* Returns an ordered range of all the object relationships where objectDefinitionId2 = ? and reverse = ? and type = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectRelationshipModelImpl
.
*
*
* @param objectDefinitionId2 the object definition id2
* @param reverse the reverse
* @param type the type
* @param start the lower bound of the range of object relationships
* @param end the upper bound of the range of object relationships (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching object relationships
*/
@Override
public List findByODI2_R_T(
long objectDefinitionId2, boolean reverse, String type, int start,
int end, OrderByComparator orderByComparator,
boolean useFinderCache) {
type = Objects.toString(type, "");
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache) {
finderPath = _finderPathWithoutPaginationFindByODI2_R_T;
finderArgs = new Object[] {objectDefinitionId2, reverse, type};
}
}
else if (useFinderCache) {
finderPath = _finderPathWithPaginationFindByODI2_R_T;
finderArgs = new Object[] {
objectDefinitionId2, reverse, type, start, end,
orderByComparator
};
}
List list = null;
if (useFinderCache) {
list = (List)finderCache.getResult(
finderPath, finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (ObjectRelationship objectRelationship : list) {
if ((objectDefinitionId2 !=
objectRelationship.getObjectDefinitionId2()) ||
(reverse != objectRelationship.isReverse()) ||
!type.equals(objectRelationship.getType())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
5 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(5);
}
sb.append(_SQL_SELECT_OBJECTRELATIONSHIP_WHERE);
sb.append(_FINDER_COLUMN_ODI2_R_T_OBJECTDEFINITIONID2_2);
sb.append(_FINDER_COLUMN_ODI2_R_T_REVERSE_2);
boolean bindType = false;
if (type.isEmpty()) {
sb.append(_FINDER_COLUMN_ODI2_R_T_TYPE_3);
}
else {
bindType = true;
sb.append(_FINDER_COLUMN_ODI2_R_T_TYPE_2);
}
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(ObjectRelationshipModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(objectDefinitionId2);
queryPos.add(reverse);
if (bindType) {
queryPos.add(type);
}
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first object relationship in the ordered set where objectDefinitionId2 = ? and reverse = ? and type = ?.
*
* @param objectDefinitionId2 the object definition id2
* @param reverse the reverse
* @param type the type
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching object relationship
* @throws NoSuchObjectRelationshipException if a matching object relationship could not be found
*/
@Override
public ObjectRelationship findByODI2_R_T_First(
long objectDefinitionId2, boolean reverse, String type,
OrderByComparator orderByComparator)
throws NoSuchObjectRelationshipException {
ObjectRelationship objectRelationship = fetchByODI2_R_T_First(
objectDefinitionId2, reverse, type, orderByComparator);
if (objectRelationship != null) {
return objectRelationship;
}
StringBundler sb = new StringBundler(8);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("objectDefinitionId2=");
sb.append(objectDefinitionId2);
sb.append(", reverse=");
sb.append(reverse);
sb.append(", type=");
sb.append(type);
sb.append("}");
throw new NoSuchObjectRelationshipException(sb.toString());
}
/**
* Returns the first object relationship in the ordered set where objectDefinitionId2 = ? and reverse = ? and type = ?.
*
* @param objectDefinitionId2 the object definition id2
* @param reverse the reverse
* @param type the type
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching object relationship, or null
if a matching object relationship could not be found
*/
@Override
public ObjectRelationship fetchByODI2_R_T_First(
long objectDefinitionId2, boolean reverse, String type,
OrderByComparator orderByComparator) {
List list = findByODI2_R_T(
objectDefinitionId2, reverse, type, 0, 1, orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the last object relationship in the ordered set where objectDefinitionId2 = ? and reverse = ? and type = ?.
*
* @param objectDefinitionId2 the object definition id2
* @param reverse the reverse
* @param type the type
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching object relationship
* @throws NoSuchObjectRelationshipException if a matching object relationship could not be found
*/
@Override
public ObjectRelationship findByODI2_R_T_Last(
long objectDefinitionId2, boolean reverse, String type,
OrderByComparator orderByComparator)
throws NoSuchObjectRelationshipException {
ObjectRelationship objectRelationship = fetchByODI2_R_T_Last(
objectDefinitionId2, reverse, type, orderByComparator);
if (objectRelationship != null) {
return objectRelationship;
}
StringBundler sb = new StringBundler(8);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("objectDefinitionId2=");
sb.append(objectDefinitionId2);
sb.append(", reverse=");
sb.append(reverse);
sb.append(", type=");
sb.append(type);
sb.append("}");
throw new NoSuchObjectRelationshipException(sb.toString());
}
/**
* Returns the last object relationship in the ordered set where objectDefinitionId2 = ? and reverse = ? and type = ?.
*
* @param objectDefinitionId2 the object definition id2
* @param reverse the reverse
* @param type the type
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching object relationship, or null
if a matching object relationship could not be found
*/
@Override
public ObjectRelationship fetchByODI2_R_T_Last(
long objectDefinitionId2, boolean reverse, String type,
OrderByComparator orderByComparator) {
int count = countByODI2_R_T(objectDefinitionId2, reverse, type);
if (count == 0) {
return null;
}
List list = findByODI2_R_T(
objectDefinitionId2, reverse, type, count - 1, count,
orderByComparator);
if (!list.isEmpty()) {
return list.get(0);
}
return null;
}
/**
* Returns the object relationships before and after the current object relationship in the ordered set where objectDefinitionId2 = ? and reverse = ? and type = ?.
*
* @param objectRelationshipId the primary key of the current object relationship
* @param objectDefinitionId2 the object definition id2
* @param reverse the reverse
* @param type the type
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next object relationship
* @throws NoSuchObjectRelationshipException if a object relationship with the primary key could not be found
*/
@Override
public ObjectRelationship[] findByODI2_R_T_PrevAndNext(
long objectRelationshipId, long objectDefinitionId2,
boolean reverse, String type,
OrderByComparator orderByComparator)
throws NoSuchObjectRelationshipException {
type = Objects.toString(type, "");
ObjectRelationship objectRelationship = findByPrimaryKey(
objectRelationshipId);
Session session = null;
try {
session = openSession();
ObjectRelationship[] array = new ObjectRelationshipImpl[3];
array[0] = getByODI2_R_T_PrevAndNext(
session, objectRelationship, objectDefinitionId2, reverse, type,
orderByComparator, true);
array[1] = objectRelationship;
array[2] = getByODI2_R_T_PrevAndNext(
session, objectRelationship, objectDefinitionId2, reverse, type,
orderByComparator, false);
return array;
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
protected ObjectRelationship getByODI2_R_T_PrevAndNext(
Session session, ObjectRelationship objectRelationship,
long objectDefinitionId2, boolean reverse, String type,
OrderByComparator orderByComparator,
boolean previous) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
6 + (orderByComparator.getOrderByConditionFields().length * 3) +
(orderByComparator.getOrderByFields().length * 3));
}
else {
sb = new StringBundler(5);
}
sb.append(_SQL_SELECT_OBJECTRELATIONSHIP_WHERE);
sb.append(_FINDER_COLUMN_ODI2_R_T_OBJECTDEFINITIONID2_2);
sb.append(_FINDER_COLUMN_ODI2_R_T_REVERSE_2);
boolean bindType = false;
if (type.isEmpty()) {
sb.append(_FINDER_COLUMN_ODI2_R_T_TYPE_3);
}
else {
bindType = true;
sb.append(_FINDER_COLUMN_ODI2_R_T_TYPE_2);
}
if (orderByComparator != null) {
String[] orderByConditionFields =
orderByComparator.getOrderByConditionFields();
if (orderByConditionFields.length > 0) {
sb.append(WHERE_AND);
}
for (int i = 0; i < orderByConditionFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByConditionFields[i]);
if ((i + 1) < orderByConditionFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN_HAS_NEXT);
}
else {
sb.append(WHERE_LESSER_THAN_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(WHERE_GREATER_THAN);
}
else {
sb.append(WHERE_LESSER_THAN);
}
}
}
sb.append(ORDER_BY_CLAUSE);
String[] orderByFields = orderByComparator.getOrderByFields();
for (int i = 0; i < orderByFields.length; i++) {
sb.append(_ORDER_BY_ENTITY_ALIAS);
sb.append(orderByFields[i]);
if ((i + 1) < orderByFields.length) {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC_HAS_NEXT);
}
else {
sb.append(ORDER_BY_DESC_HAS_NEXT);
}
}
else {
if (orderByComparator.isAscending() ^ previous) {
sb.append(ORDER_BY_ASC);
}
else {
sb.append(ORDER_BY_DESC);
}
}
}
}
else {
sb.append(ObjectRelationshipModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Query query = session.createQuery(sql);
query.setFirstResult(0);
query.setMaxResults(2);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(objectDefinitionId2);
queryPos.add(reverse);
if (bindType) {
queryPos.add(type);
}
if (orderByComparator != null) {
for (Object orderByConditionValue :
orderByComparator.getOrderByConditionValues(
objectRelationship)) {
queryPos.add(orderByConditionValue);
}
}
List list = query.list();
if (list.size() == 2) {
return list.get(1);
}
else {
return null;
}
}
/**
* Removes all the object relationships where objectDefinitionId2 = ? and reverse = ? and type = ? from the database.
*
* @param objectDefinitionId2 the object definition id2
* @param reverse the reverse
* @param type the type
*/
@Override
public void removeByODI2_R_T(
long objectDefinitionId2, boolean reverse, String type) {
for (ObjectRelationship objectRelationship :
findByODI2_R_T(
objectDefinitionId2, reverse, type, QueryUtil.ALL_POS,
QueryUtil.ALL_POS, null)) {
remove(objectRelationship);
}
}
/**
* Returns the number of object relationships where objectDefinitionId2 = ? and reverse = ? and type = ?.
*
* @param objectDefinitionId2 the object definition id2
* @param reverse the reverse
* @param type the type
* @return the number of matching object relationships
*/
@Override
public int countByODI2_R_T(
long objectDefinitionId2, boolean reverse, String type) {
type = Objects.toString(type, "");
FinderPath finderPath = _finderPathCountByODI2_R_T;
Object[] finderArgs = new Object[] {objectDefinitionId2, reverse, type};
Long count = (Long)finderCache.getResult(finderPath, finderArgs, this);
if (count == null) {
StringBundler sb = new StringBundler(4);
sb.append(_SQL_COUNT_OBJECTRELATIONSHIP_WHERE);
sb.append(_FINDER_COLUMN_ODI2_R_T_OBJECTDEFINITIONID2_2);
sb.append(_FINDER_COLUMN_ODI2_R_T_REVERSE_2);
boolean bindType = false;
if (type.isEmpty()) {
sb.append(_FINDER_COLUMN_ODI2_R_T_TYPE_3);
}
else {
bindType = true;
sb.append(_FINDER_COLUMN_ODI2_R_T_TYPE_2);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(objectDefinitionId2);
queryPos.add(reverse);
if (bindType) {
queryPos.add(type);
}
count = (Long)query.uniqueResult();
finderCache.putResult(finderPath, finderArgs, count);
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return count.intValue();
}
private static final String _FINDER_COLUMN_ODI2_R_T_OBJECTDEFINITIONID2_2 =
"objectRelationship.objectDefinitionId2 = ? AND ";
private static final String _FINDER_COLUMN_ODI2_R_T_REVERSE_2 =
"objectRelationship.reverse = ? AND ";
private static final String _FINDER_COLUMN_ODI2_R_T_TYPE_2 =
"objectRelationship.type = ?";
private static final String _FINDER_COLUMN_ODI2_R_T_TYPE_3 =
"(objectRelationship.type IS NULL OR objectRelationship.type = '')";
private FinderPath _finderPathWithPaginationFindByODI1_ODI2_N_T;
private FinderPath _finderPathWithoutPaginationFindByODI1_ODI2_N_T;
private FinderPath _finderPathCountByODI1_ODI2_N_T;
/**
* Returns all the object relationships where objectDefinitionId1 = ? and objectDefinitionId2 = ? and name = ? and type = ?.
*
* @param objectDefinitionId1 the object definition id1
* @param objectDefinitionId2 the object definition id2
* @param name the name
* @param type the type
* @return the matching object relationships
*/
@Override
public List findByODI1_ODI2_N_T(
long objectDefinitionId1, long objectDefinitionId2, String name,
String type) {
return findByODI1_ODI2_N_T(
objectDefinitionId1, objectDefinitionId2, name, type,
QueryUtil.ALL_POS, QueryUtil.ALL_POS, null);
}
/**
* Returns a range of all the object relationships where objectDefinitionId1 = ? and objectDefinitionId2 = ? and name = ? and type = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectRelationshipModelImpl
.
*
*
* @param objectDefinitionId1 the object definition id1
* @param objectDefinitionId2 the object definition id2
* @param name the name
* @param type the type
* @param start the lower bound of the range of object relationships
* @param end the upper bound of the range of object relationships (not inclusive)
* @return the range of matching object relationships
*/
@Override
public List findByODI1_ODI2_N_T(
long objectDefinitionId1, long objectDefinitionId2, String name,
String type, int start, int end) {
return findByODI1_ODI2_N_T(
objectDefinitionId1, objectDefinitionId2, name, type, start, end,
null);
}
/**
* Returns an ordered range of all the object relationships where objectDefinitionId1 = ? and objectDefinitionId2 = ? and name = ? and type = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectRelationshipModelImpl
.
*
*
* @param objectDefinitionId1 the object definition id1
* @param objectDefinitionId2 the object definition id2
* @param name the name
* @param type the type
* @param start the lower bound of the range of object relationships
* @param end the upper bound of the range of object relationships (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching object relationships
*/
@Override
public List findByODI1_ODI2_N_T(
long objectDefinitionId1, long objectDefinitionId2, String name,
String type, int start, int end,
OrderByComparator orderByComparator) {
return findByODI1_ODI2_N_T(
objectDefinitionId1, objectDefinitionId2, name, type, start, end,
orderByComparator, true);
}
/**
* Returns an ordered range of all the object relationships where objectDefinitionId1 = ? and objectDefinitionId2 = ? and name = ? and type = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from ObjectRelationshipModelImpl
.
*
*
* @param objectDefinitionId1 the object definition id1
* @param objectDefinitionId2 the object definition id2
* @param name the name
* @param type the type
* @param start the lower bound of the range of object relationships
* @param end the upper bound of the range of object relationships (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching object relationships
*/
@Override
public List findByODI1_ODI2_N_T(
long objectDefinitionId1, long objectDefinitionId2, String name,
String type, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
name = Objects.toString(name, "");
type = Objects.toString(type, "");
FinderPath finderPath = null;
Object[] finderArgs = null;
if ((start == QueryUtil.ALL_POS) && (end == QueryUtil.ALL_POS) &&
(orderByComparator == null)) {
if (useFinderCache) {
finderPath = _finderPathWithoutPaginationFindByODI1_ODI2_N_T;
finderArgs = new Object[] {
objectDefinitionId1, objectDefinitionId2, name, type
};
}
}
else if (useFinderCache) {
finderPath = _finderPathWithPaginationFindByODI1_ODI2_N_T;
finderArgs = new Object[] {
objectDefinitionId1, objectDefinitionId2, name, type, start,
end, orderByComparator
};
}
List list = null;
if (useFinderCache) {
list = (List)finderCache.getResult(
finderPath, finderArgs, this);
if ((list != null) && !list.isEmpty()) {
for (ObjectRelationship objectRelationship : list) {
if ((objectDefinitionId1 !=
objectRelationship.getObjectDefinitionId1()) ||
(objectDefinitionId2 !=
objectRelationship.getObjectDefinitionId2()) ||
!name.equals(objectRelationship.getName()) ||
!type.equals(objectRelationship.getType())) {
list = null;
break;
}
}
}
}
if (list == null) {
StringBundler sb = null;
if (orderByComparator != null) {
sb = new StringBundler(
6 + (orderByComparator.getOrderByFields().length * 2));
}
else {
sb = new StringBundler(6);
}
sb.append(_SQL_SELECT_OBJECTRELATIONSHIP_WHERE);
sb.append(_FINDER_COLUMN_ODI1_ODI2_N_T_OBJECTDEFINITIONID1_2);
sb.append(_FINDER_COLUMN_ODI1_ODI2_N_T_OBJECTDEFINITIONID2_2);
boolean bindName = false;
if (name.isEmpty()) {
sb.append(_FINDER_COLUMN_ODI1_ODI2_N_T_NAME_3);
}
else {
bindName = true;
sb.append(_FINDER_COLUMN_ODI1_ODI2_N_T_NAME_2);
}
boolean bindType = false;
if (type.isEmpty()) {
sb.append(_FINDER_COLUMN_ODI1_ODI2_N_T_TYPE_3);
}
else {
bindType = true;
sb.append(_FINDER_COLUMN_ODI1_ODI2_N_T_TYPE_2);
}
if (orderByComparator != null) {
appendOrderByComparator(
sb, _ORDER_BY_ENTITY_ALIAS, orderByComparator);
}
else {
sb.append(ObjectRelationshipModelImpl.ORDER_BY_JPQL);
}
String sql = sb.toString();
Session session = null;
try {
session = openSession();
Query query = session.createQuery(sql);
QueryPos queryPos = QueryPos.getInstance(query);
queryPos.add(objectDefinitionId1);
queryPos.add(objectDefinitionId2);
if (bindName) {
queryPos.add(name);
}
if (bindType) {
queryPos.add(type);
}
list = (List)QueryUtil.list(
query, getDialect(), start, end);
cacheResult(list);
if (useFinderCache) {
finderCache.putResult(finderPath, finderArgs, list);
}
}
catch (Exception exception) {
throw processException(exception);
}
finally {
closeSession(session);
}
}
return list;
}
/**
* Returns the first object relationship in the ordered set where objectDefinitionId1 = ? and objectDefinitionId2 = ? and name = ? and type = ?.
*
* @param objectDefinitionId1 the object definition id1
* @param objectDefinitionId2 the object definition id2
* @param name the name
* @param type the type
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching object relationship
* @throws NoSuchObjectRelationshipException if a matching object relationship could not be found
*/
@Override
public ObjectRelationship findByODI1_ODI2_N_T_First(
long objectDefinitionId1, long objectDefinitionId2, String name,
String type,
OrderByComparator orderByComparator)
throws NoSuchObjectRelationshipException {
ObjectRelationship objectRelationship = fetchByODI1_ODI2_N_T_First(
objectDefinitionId1, objectDefinitionId2, name, type,
orderByComparator);
if (objectRelationship != null) {
return objectRelationship;
}
StringBundler sb = new StringBundler(10);
sb.append(_NO_SUCH_ENTITY_WITH_KEY);
sb.append("objectDefinitionId1=");
sb.append(objectDefinitionId1);
sb.append(", objectDefinitionId2=");
sb.append(objectDefinitionId2);
sb.append(", name=");
sb.append(name);
sb.append(", type=");
sb.append(type);
sb.append("}");
throw new NoSuchObjectRelationshipException(sb.toString());
}
/**
* Returns the first object relationship in the ordered set where objectDefinitionId1 = ? and objectDefinitionId2 = ? and name = ? and type = ?.
*
* @param objectDefinitionId1 the object definition id1
* @param objectDefinitionId2 the object definition id2
* @param name the name
* @param type the type
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching object relationship, or null
if a matching object relationship could not be found
*/
@Override
public ObjectRelationship fetchByODI1_ODI2_N_T_First(
long objectDefinitionId1, long objectDefinitionId2, String name,
String type, OrderByComparator orderByComparator) {
List