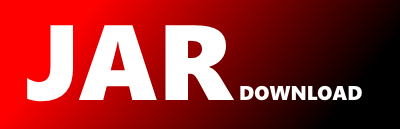
com.liferay.osgi.service.tracker.collections.map.ServiceTrackerCustomizerFactory Maven / Gradle / Ivy
/**
* Copyright (c) 2000-present Liferay, Inc. All rights reserved.
*
* This library is free software; you can redistribute it and/or modify it under
* the terms of the GNU Lesser General Public License as published by the Free
* Software Foundation; either version 2.1 of the License, or (at your option)
* any later version.
*
* This library is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License for more
* details.
*/
package com.liferay.osgi.service.tracker.collections.map;
import com.liferay.osgi.service.tracker.collections.ServiceReferenceServiceTuple;
import java.util.HashMap;
import java.util.Map;
import java.util.function.BiFunction;
import java.util.function.Function;
import org.osgi.framework.BundleContext;
import org.osgi.framework.ServiceReference;
import org.osgi.util.tracker.ServiceTrackerCustomizer;
/**
* @author Carlos Sierra Andrés
*/
public class ServiceTrackerCustomizerFactory {
public static Function>
createFromFunction(BiFunction, S, T> function) {
return b -> new ServiceTrackerCustomizer() {
@Override
public T addingService(ServiceReference serviceReference) {
S service = b.getService(serviceReference);
try {
return function.apply(serviceReference, service);
}
catch (Exception exception) {
b.ungetService(serviceReference);
throw exception;
}
}
@Override
public void modifiedService(
ServiceReference serviceReference, T t) {
removedService(serviceReference, t);
addingService(serviceReference);
}
@Override
public void removedService(
ServiceReference serviceReference, T t) {
b.ungetService(serviceReference);
}
};
}
public static
ServiceTrackerCustomizer>
serviceReferenceServiceTuple(final BundleContext bundleContext) {
return new ServiceTrackerCustomizer
>() {
@Override
public ServiceReferenceServiceTuple addingService(
ServiceReference serviceReference) {
S service = bundleContext.getService(serviceReference);
if (service == null) {
return null;
}
return new ServiceReferenceServiceTuple<>(
serviceReference, service);
}
@Override
public void modifiedService(
ServiceReference serviceReference,
ServiceReferenceServiceTuple
serviceReferenceServiceTuple) {
}
@Override
public void removedService(
ServiceReference serviceReference,
ServiceReferenceServiceTuple
serviceReferenceServiceTuple) {
bundleContext.ungetService(serviceReference);
}
};
}
public static ServiceTrackerCustomizer>
serviceWrapper(BundleContext bundleContext) {
return new ServiceTrackerCustomizer>() {
@Override
public ServiceWrapper addingService(
ServiceReference serviceReference) {
S service = bundleContext.getService(serviceReference);
if (service == null) {
return null;
}
try {
return new ServiceWrapperImpl<>(serviceReference, service);
}
catch (Throwable throwable) {
bundleContext.ungetService(serviceReference);
throw throwable;
}
}
@Override
public void modifiedService(
ServiceReference serviceReference,
ServiceWrapper serviceWrapper) {
ServiceWrapperImpl serviceWrapperImpl =
(ServiceWrapperImpl)serviceWrapper;
serviceWrapperImpl._resetProperties();
}
@Override
public void removedService(
ServiceReference serviceReference,
ServiceWrapper serviceWrapper) {
bundleContext.ungetService(serviceReference);
}
};
}
public interface ServiceWrapper {
public Map getProperties();
public S getService();
}
private static class ServiceWrapperImpl implements ServiceWrapper {
@Override
public Map getProperties() {
Map properties = _properties;
if (properties == null) {
properties = new HashMap<>();
String[] propertyKeys = _serviceReference.getPropertyKeys();
for (String propertyKey : propertyKeys) {
properties.put(
propertyKey,
_serviceReference.getProperty(propertyKey));
}
_properties = properties;
}
return properties;
}
@Override
public S getService() {
return _service;
}
private ServiceWrapperImpl(
ServiceReference serviceReference, S service) {
_serviceReference = serviceReference;
_service = service;
}
private void _resetProperties() {
_properties = null;
}
private volatile Map _properties;
private final S _service;
private final ServiceReference _serviceReference;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy