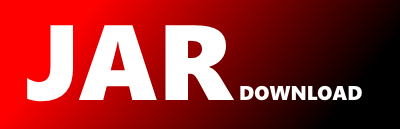
com.liferay.saml.internal.upgrade.v1_0_0.SamlProviderConfigurationPreferencesUpgradeProcess Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.liferay.saml.impl
Show all versions of com.liferay.saml.impl
Liferay SAML Implementation
The newest version!
/**
* SPDX-FileCopyrightText: (c) 2000 Liferay, Inc. https://liferay.com
* SPDX-License-Identifier: LGPL-2.1-or-later OR LicenseRef-Liferay-DXP-EULA-2.0.0-2023-06
*/
package com.liferay.saml.internal.upgrade.v1_0_0;
import com.liferay.portal.kernel.configuration.Filter;
import com.liferay.portal.kernel.model.CompanyConstants;
import com.liferay.portal.kernel.security.auth.CompanyThreadLocal;
import com.liferay.portal.kernel.service.CompanyLocalService;
import com.liferay.portal.kernel.util.ArrayUtil;
import com.liferay.portal.kernel.util.LoggingTimer;
import com.liferay.portal.kernel.util.PrefsProps;
import com.liferay.portal.kernel.util.Props;
import com.liferay.portal.kernel.util.UnicodeProperties;
import com.liferay.portal.kernel.util.Validator;
import com.liferay.saml.internal.constants.LegacySamlPropsKeys;
import com.liferay.saml.runtime.configuration.SamlProviderConfigurationHelper;
import java.util.HashSet;
import java.util.Objects;
import java.util.Set;
import javax.portlet.PortletPreferences;
/**
* @author Stian Sigvartsen
* @author Tomas Polesovsky
*/
public class SamlProviderConfigurationPreferencesUpgradeProcess
extends BaseUpgradeSaml {
public SamlProviderConfigurationPreferencesUpgradeProcess(
CompanyLocalService companyLocalService, PrefsProps prefsProps,
Props props,
SamlProviderConfigurationHelper samlProviderConfigurationHelper) {
_companyLocalService = companyLocalService;
_prefsProps = prefsProps;
_props = props;
_samlProviderConfigurationHelper = samlProviderConfigurationHelper;
}
public Set migrateSAMLProviderConfigurationPreferences(
long companyId)
throws Exception {
String prefsPropsFilterString = null;
Filter propsFilter = null;
PortletPreferences portletPreferences = _prefsProps.getPreferences(
companyId);
String entityId = portletPreferences.getValue(
LegacySamlPropsKeys.SAML_ENTITY_ID, null);
if (entityId == null) {
entityId = _props.get(LegacySamlPropsKeys.SAML_ENTITY_ID);
}
if (Validator.isNotNull(entityId)) {
prefsPropsFilterString = "[" + entityId + "]";
propsFilter = new Filter(entityId);
}
Set migratedPrefsPropsKeys = new HashSet<>();
UnicodeProperties unicodeProperties = new UnicodeProperties();
for (String key : LegacySamlPropsKeys.SAML_KEYS_PREFS_PROPS) {
if (ArrayUtil.contains(
LegacySamlPropsKeys.SAML_KEYS_DEPRECATED, key)) {
continue;
}
String value = null;
if ((prefsPropsFilterString != null) &&
ArrayUtil.contains(
LegacySamlPropsKeys.SAML_KEYS_FILTERED, key)) {
String prefsPropsKey = key + prefsPropsFilterString;
value = portletPreferences.getValue(prefsPropsKey, null);
if (value != null) {
migratedPrefsPropsKeys.add(prefsPropsKey);
}
}
if (value == null) {
value = portletPreferences.getValue(key, null);
if (value != null) {
migratedPrefsPropsKeys.add(key);
}
}
if (value == null) {
value = getPropsValue(_props, key, propsFilter);
}
if (value == null) {
continue;
}
if (!Objects.equals(value, getDefaultValue(key))) {
unicodeProperties.put(key, value);
}
}
if (!migratedPrefsPropsKeys.isEmpty()) {
long companyThreadLocalCompanyId =
CompanyThreadLocal.getCompanyId();
try {
CompanyThreadLocal.setCompanyId(companyId);
_samlProviderConfigurationHelper.updateProperties(
unicodeProperties);
}
finally {
CompanyThreadLocal.setCompanyId(companyThreadLocalCompanyId);
}
}
return migratedPrefsPropsKeys;
}
public void migrateSAMLProviderConfigurationSystemPreferences()
throws Exception {
Filter filter = null;
String entityId = _props.get(LegacySamlPropsKeys.SAML_ENTITY_ID);
if (Validator.isNotNull(entityId)) {
filter = new Filter(entityId);
}
UnicodeProperties unicodeProperties = new UnicodeProperties();
for (String key : LegacySamlPropsKeys.SAML_KEYS_PREFS_PROPS) {
if (ArrayUtil.contains(
LegacySamlPropsKeys.SAML_KEYS_DEPRECATED, key)) {
continue;
}
String value = getPropsValue(_props, key, filter);
if (value == null) {
continue;
}
if (!Objects.equals(value, getDefaultValue(key))) {
unicodeProperties.put(key, value);
}
}
if (!unicodeProperties.isEmpty()) {
long companyThreadLocalCompanyId =
CompanyThreadLocal.getCompanyId();
try {
CompanyThreadLocal.setCompanyId(CompanyConstants.SYSTEM);
_samlProviderConfigurationHelper.updateProperties(
unicodeProperties);
}
finally {
CompanyThreadLocal.setCompanyId(companyThreadLocalCompanyId);
}
}
}
@Override
protected void doUpgrade() throws Exception {
try (LoggingTimer loggingTimer = new LoggingTimer()) {
_companyLocalService.forEachCompanyId(
companyId -> {
Set migratedPrefsPropsKeys =
migrateSAMLProviderConfigurationPreferences(companyId);
if (migratedPrefsPropsKeys.isEmpty()) {
return;
}
_companyLocalService.removePreferences(
companyId,
migratedPrefsPropsKeys.toArray(new String[0]));
});
migrateSAMLProviderConfigurationSystemPreferences();
}
}
private final CompanyLocalService _companyLocalService;
private final PrefsProps _prefsProps;
private final Props _props;
private final SamlProviderConfigurationHelper
_samlProviderConfigurationHelper;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy