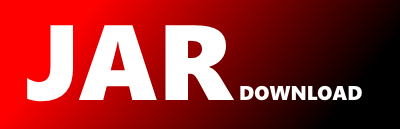
com.liferay.saml.persistence.service.persistence.SamlIdpSsoSessionPersistence Maven / Gradle / Ivy
/**
* SPDX-FileCopyrightText: (c) 2000 Liferay, Inc. https://liferay.com
* SPDX-License-Identifier: LGPL-2.1-or-later OR LicenseRef-Liferay-DXP-EULA-2.0.0-2023-06
*/
package com.liferay.saml.persistence.service.persistence;
import com.liferay.portal.kernel.service.persistence.BasePersistence;
import com.liferay.saml.persistence.exception.NoSuchIdpSsoSessionException;
import com.liferay.saml.persistence.model.SamlIdpSsoSession;
import java.util.Date;
import org.osgi.annotation.versioning.ProviderType;
/**
* The persistence interface for the saml idp sso session service.
*
*
* Caching information and settings can be found in portal.properties
*
*
* @author Mika Koivisto
* @see SamlIdpSsoSessionUtil
* @generated
*/
@ProviderType
public interface SamlIdpSsoSessionPersistence
extends BasePersistence {
/*
* NOTE FOR DEVELOPERS:
*
* Never modify or reference this interface directly. Always use {@link SamlIdpSsoSessionUtil} to access the saml idp sso session persistence. Modify service.xml
and rerun ServiceBuilder to regenerate this interface.
*/
/**
* Returns all the saml idp sso sessions where createDate < ?.
*
* @param createDate the create date
* @return the matching saml idp sso sessions
*/
public java.util.List findByLtCreateDate(
Date createDate);
/**
* Returns a range of all the saml idp sso sessions where createDate < ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SamlIdpSsoSessionModelImpl
.
*
*
* @param createDate the create date
* @param start the lower bound of the range of saml idp sso sessions
* @param end the upper bound of the range of saml idp sso sessions (not inclusive)
* @return the range of matching saml idp sso sessions
*/
public java.util.List findByLtCreateDate(
Date createDate, int start, int end);
/**
* Returns an ordered range of all the saml idp sso sessions where createDate < ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SamlIdpSsoSessionModelImpl
.
*
*
* @param createDate the create date
* @param start the lower bound of the range of saml idp sso sessions
* @param end the upper bound of the range of saml idp sso sessions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching saml idp sso sessions
*/
public java.util.List findByLtCreateDate(
Date createDate, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns an ordered range of all the saml idp sso sessions where createDate < ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SamlIdpSsoSessionModelImpl
.
*
*
* @param createDate the create date
* @param start the lower bound of the range of saml idp sso sessions
* @param end the upper bound of the range of saml idp sso sessions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching saml idp sso sessions
*/
public java.util.List findByLtCreateDate(
Date createDate, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator,
boolean useFinderCache);
/**
* Returns the first saml idp sso session in the ordered set where createDate < ?.
*
* @param createDate the create date
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching saml idp sso session
* @throws NoSuchIdpSsoSessionException if a matching saml idp sso session could not be found
*/
public SamlIdpSsoSession findByLtCreateDate_First(
Date createDate,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchIdpSsoSessionException;
/**
* Returns the first saml idp sso session in the ordered set where createDate < ?.
*
* @param createDate the create date
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching saml idp sso session, or null
if a matching saml idp sso session could not be found
*/
public SamlIdpSsoSession fetchByLtCreateDate_First(
Date createDate,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the last saml idp sso session in the ordered set where createDate < ?.
*
* @param createDate the create date
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching saml idp sso session
* @throws NoSuchIdpSsoSessionException if a matching saml idp sso session could not be found
*/
public SamlIdpSsoSession findByLtCreateDate_Last(
Date createDate,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchIdpSsoSessionException;
/**
* Returns the last saml idp sso session in the ordered set where createDate < ?.
*
* @param createDate the create date
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching saml idp sso session, or null
if a matching saml idp sso session could not be found
*/
public SamlIdpSsoSession fetchByLtCreateDate_Last(
Date createDate,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the saml idp sso sessions before and after the current saml idp sso session in the ordered set where createDate < ?.
*
* @param samlIdpSsoSessionId the primary key of the current saml idp sso session
* @param createDate the create date
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next saml idp sso session
* @throws NoSuchIdpSsoSessionException if a saml idp sso session with the primary key could not be found
*/
public SamlIdpSsoSession[] findByLtCreateDate_PrevAndNext(
long samlIdpSsoSessionId, Date createDate,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchIdpSsoSessionException;
/**
* Removes all the saml idp sso sessions where createDate < ? from the database.
*
* @param createDate the create date
*/
public void removeByLtCreateDate(Date createDate);
/**
* Returns the number of saml idp sso sessions where createDate < ?.
*
* @param createDate the create date
* @return the number of matching saml idp sso sessions
*/
public int countByLtCreateDate(Date createDate);
/**
* Returns the saml idp sso session where samlIdpSsoSessionKey = ? or throws a NoSuchIdpSsoSessionException
if it could not be found.
*
* @param samlIdpSsoSessionKey the saml idp sso session key
* @return the matching saml idp sso session
* @throws NoSuchIdpSsoSessionException if a matching saml idp sso session could not be found
*/
public SamlIdpSsoSession findBySamlIdpSsoSessionKey(
String samlIdpSsoSessionKey)
throws NoSuchIdpSsoSessionException;
/**
* Returns the saml idp sso session where samlIdpSsoSessionKey = ? or returns null
if it could not be found. Uses the finder cache.
*
* @param samlIdpSsoSessionKey the saml idp sso session key
* @return the matching saml idp sso session, or null
if a matching saml idp sso session could not be found
*/
public SamlIdpSsoSession fetchBySamlIdpSsoSessionKey(
String samlIdpSsoSessionKey);
/**
* Returns the saml idp sso session where samlIdpSsoSessionKey = ? or returns null
if it could not be found, optionally using the finder cache.
*
* @param samlIdpSsoSessionKey the saml idp sso session key
* @param useFinderCache whether to use the finder cache
* @return the matching saml idp sso session, or null
if a matching saml idp sso session could not be found
*/
public SamlIdpSsoSession fetchBySamlIdpSsoSessionKey(
String samlIdpSsoSessionKey, boolean useFinderCache);
/**
* Removes the saml idp sso session where samlIdpSsoSessionKey = ? from the database.
*
* @param samlIdpSsoSessionKey the saml idp sso session key
* @return the saml idp sso session that was removed
*/
public SamlIdpSsoSession removeBySamlIdpSsoSessionKey(
String samlIdpSsoSessionKey)
throws NoSuchIdpSsoSessionException;
/**
* Returns the number of saml idp sso sessions where samlIdpSsoSessionKey = ?.
*
* @param samlIdpSsoSessionKey the saml idp sso session key
* @return the number of matching saml idp sso sessions
*/
public int countBySamlIdpSsoSessionKey(String samlIdpSsoSessionKey);
/**
* Caches the saml idp sso session in the entity cache if it is enabled.
*
* @param samlIdpSsoSession the saml idp sso session
*/
public void cacheResult(SamlIdpSsoSession samlIdpSsoSession);
/**
* Caches the saml idp sso sessions in the entity cache if it is enabled.
*
* @param samlIdpSsoSessions the saml idp sso sessions
*/
public void cacheResult(
java.util.List samlIdpSsoSessions);
/**
* Creates a new saml idp sso session with the primary key. Does not add the saml idp sso session to the database.
*
* @param samlIdpSsoSessionId the primary key for the new saml idp sso session
* @return the new saml idp sso session
*/
public SamlIdpSsoSession create(long samlIdpSsoSessionId);
/**
* Removes the saml idp sso session with the primary key from the database. Also notifies the appropriate model listeners.
*
* @param samlIdpSsoSessionId the primary key of the saml idp sso session
* @return the saml idp sso session that was removed
* @throws NoSuchIdpSsoSessionException if a saml idp sso session with the primary key could not be found
*/
public SamlIdpSsoSession remove(long samlIdpSsoSessionId)
throws NoSuchIdpSsoSessionException;
public SamlIdpSsoSession updateImpl(SamlIdpSsoSession samlIdpSsoSession);
/**
* Returns the saml idp sso session with the primary key or throws a NoSuchIdpSsoSessionException
if it could not be found.
*
* @param samlIdpSsoSessionId the primary key of the saml idp sso session
* @return the saml idp sso session
* @throws NoSuchIdpSsoSessionException if a saml idp sso session with the primary key could not be found
*/
public SamlIdpSsoSession findByPrimaryKey(long samlIdpSsoSessionId)
throws NoSuchIdpSsoSessionException;
/**
* Returns the saml idp sso session with the primary key or returns null
if it could not be found.
*
* @param samlIdpSsoSessionId the primary key of the saml idp sso session
* @return the saml idp sso session, or null
if a saml idp sso session with the primary key could not be found
*/
public SamlIdpSsoSession fetchByPrimaryKey(long samlIdpSsoSessionId);
/**
* Returns all the saml idp sso sessions.
*
* @return the saml idp sso sessions
*/
public java.util.List findAll();
/**
* Returns a range of all the saml idp sso sessions.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SamlIdpSsoSessionModelImpl
.
*
*
* @param start the lower bound of the range of saml idp sso sessions
* @param end the upper bound of the range of saml idp sso sessions (not inclusive)
* @return the range of saml idp sso sessions
*/
public java.util.List findAll(int start, int end);
/**
* Returns an ordered range of all the saml idp sso sessions.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SamlIdpSsoSessionModelImpl
.
*
*
* @param start the lower bound of the range of saml idp sso sessions
* @param end the upper bound of the range of saml idp sso sessions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of saml idp sso sessions
*/
public java.util.List findAll(
int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns an ordered range of all the saml idp sso sessions.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SamlIdpSsoSessionModelImpl
.
*
*
* @param start the lower bound of the range of saml idp sso sessions
* @param end the upper bound of the range of saml idp sso sessions (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of saml idp sso sessions
*/
public java.util.List findAll(
int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator,
boolean useFinderCache);
/**
* Removes all the saml idp sso sessions from the database.
*/
public void removeAll();
/**
* Returns the number of saml idp sso sessions.
*
* @return the number of saml idp sso sessions
*/
public int countAll();
}