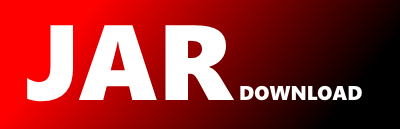
com.liferay.search.experiences.rest.dto.v1_0.Clause Maven / Gradle / Ivy
/**
* SPDX-FileCopyrightText: (c) 2000 Liferay, Inc. https://liferay.com
* SPDX-License-Identifier: LGPL-2.1-or-later OR LicenseRef-Liferay-DXP-EULA-2.0.0-2023-06
*/
package com.liferay.search.experiences.rest.dto.v1_0;
import com.fasterxml.jackson.annotation.JsonFilter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.liferay.petra.function.UnsafeSupplier;
import com.liferay.petra.string.StringBundler;
import com.liferay.portal.kernel.json.JSONFactoryUtil;
import com.liferay.portal.kernel.util.StringUtil;
import com.liferay.portal.vulcan.graphql.annotation.GraphQLField;
import com.liferay.portal.vulcan.graphql.annotation.GraphQLName;
import com.liferay.portal.vulcan.util.ObjectMapperUtil;
import io.swagger.v3.oas.annotations.media.Schema;
import java.io.Serializable;
import java.util.Iterator;
import java.util.Map;
import java.util.Objects;
import java.util.Set;
import java.util.function.Supplier;
import javax.annotation.Generated;
import javax.validation.Valid;
import javax.xml.bind.annotation.XmlRootElement;
/**
* @author Brian Wing Shun Chan
* @generated
*/
@Generated("")
@GraphQLName("Clause")
@JsonFilter("Liferay.Vulcan")
@XmlRootElement(name = "Clause")
public class Clause implements Serializable {
public static Clause toDTO(String json) {
return ObjectMapperUtil.readValue(Clause.class, json);
}
public static Clause unsafeToDTO(String json) {
return ObjectMapperUtil.unsafeReadValue(Clause.class, json);
}
@Schema
public Boolean getAdditive() {
if (_additiveSupplier != null) {
additive = _additiveSupplier.get();
_additiveSupplier = null;
}
return additive;
}
public void setAdditive(Boolean additive) {
this.additive = additive;
_additiveSupplier = null;
}
@JsonIgnore
public void setAdditive(
UnsafeSupplier additiveUnsafeSupplier) {
_additiveSupplier = () -> {
try {
return additiveUnsafeSupplier.get();
}
catch (RuntimeException runtimeException) {
throw runtimeException;
}
catch (Exception exception) {
throw new RuntimeException(exception);
}
};
}
@GraphQLField
@JsonProperty(access = JsonProperty.Access.READ_WRITE)
protected Boolean additive;
@JsonIgnore
private Supplier _additiveSupplier;
@Schema
@Valid
public Float getBoost() {
if (_boostSupplier != null) {
boost = _boostSupplier.get();
_boostSupplier = null;
}
return boost;
}
public void setBoost(Float boost) {
this.boost = boost;
_boostSupplier = null;
}
@JsonIgnore
public void setBoost(UnsafeSupplier boostUnsafeSupplier) {
_boostSupplier = () -> {
try {
return boostUnsafeSupplier.get();
}
catch (RuntimeException runtimeException) {
throw runtimeException;
}
catch (Exception exception) {
throw new RuntimeException(exception);
}
};
}
@GraphQLField
@JsonProperty(access = JsonProperty.Access.READ_WRITE)
protected Float boost;
@JsonIgnore
private Supplier _boostSupplier;
@Schema
public String getContext() {
if (_contextSupplier != null) {
context = _contextSupplier.get();
_contextSupplier = null;
}
return context;
}
public void setContext(String context) {
this.context = context;
_contextSupplier = null;
}
@JsonIgnore
public void setContext(
UnsafeSupplier contextUnsafeSupplier) {
_contextSupplier = () -> {
try {
return contextUnsafeSupplier.get();
}
catch (RuntimeException runtimeException) {
throw runtimeException;
}
catch (Exception exception) {
throw new RuntimeException(exception);
}
};
}
@GraphQLField
@JsonProperty(access = JsonProperty.Access.READ_WRITE)
protected String context;
@JsonIgnore
private Supplier _contextSupplier;
@Schema
public Boolean getDisabled() {
if (_disabledSupplier != null) {
disabled = _disabledSupplier.get();
_disabledSupplier = null;
}
return disabled;
}
public void setDisabled(Boolean disabled) {
this.disabled = disabled;
_disabledSupplier = null;
}
@JsonIgnore
public void setDisabled(
UnsafeSupplier disabledUnsafeSupplier) {
_disabledSupplier = () -> {
try {
return disabledUnsafeSupplier.get();
}
catch (RuntimeException runtimeException) {
throw runtimeException;
}
catch (Exception exception) {
throw new RuntimeException(exception);
}
};
}
@GraphQLField
@JsonProperty(access = JsonProperty.Access.READ_WRITE)
protected Boolean disabled;
@JsonIgnore
private Supplier _disabledSupplier;
@Schema
public String getField() {
if (_fieldSupplier != null) {
field = _fieldSupplier.get();
_fieldSupplier = null;
}
return field;
}
public void setField(String field) {
this.field = field;
_fieldSupplier = null;
}
@JsonIgnore
public void setField(
UnsafeSupplier fieldUnsafeSupplier) {
_fieldSupplier = () -> {
try {
return fieldUnsafeSupplier.get();
}
catch (RuntimeException runtimeException) {
throw runtimeException;
}
catch (Exception exception) {
throw new RuntimeException(exception);
}
};
}
@GraphQLField
@JsonProperty(access = JsonProperty.Access.READ_WRITE)
protected String field;
@JsonIgnore
private Supplier _fieldSupplier;
@Schema
public String getName() {
if (_nameSupplier != null) {
name = _nameSupplier.get();
_nameSupplier = null;
}
return name;
}
public void setName(String name) {
this.name = name;
_nameSupplier = null;
}
@JsonIgnore
public void setName(UnsafeSupplier nameUnsafeSupplier) {
_nameSupplier = () -> {
try {
return nameUnsafeSupplier.get();
}
catch (RuntimeException runtimeException) {
throw runtimeException;
}
catch (Exception exception) {
throw new RuntimeException(exception);
}
};
}
@GraphQLField
@JsonProperty(access = JsonProperty.Access.READ_WRITE)
protected String name;
@JsonIgnore
private Supplier _nameSupplier;
@Schema
public String getOccur() {
if (_occurSupplier != null) {
occur = _occurSupplier.get();
_occurSupplier = null;
}
return occur;
}
public void setOccur(String occur) {
this.occur = occur;
_occurSupplier = null;
}
@JsonIgnore
public void setOccur(
UnsafeSupplier occurUnsafeSupplier) {
_occurSupplier = () -> {
try {
return occurUnsafeSupplier.get();
}
catch (RuntimeException runtimeException) {
throw runtimeException;
}
catch (Exception exception) {
throw new RuntimeException(exception);
}
};
}
@GraphQLField
@JsonProperty(access = JsonProperty.Access.READ_WRITE)
protected String occur;
@JsonIgnore
private Supplier _occurSupplier;
@Schema
public String getParent() {
if (_parentSupplier != null) {
parent = _parentSupplier.get();
_parentSupplier = null;
}
return parent;
}
public void setParent(String parent) {
this.parent = parent;
_parentSupplier = null;
}
@JsonIgnore
public void setParent(
UnsafeSupplier parentUnsafeSupplier) {
_parentSupplier = () -> {
try {
return parentUnsafeSupplier.get();
}
catch (RuntimeException runtimeException) {
throw runtimeException;
}
catch (Exception exception) {
throw new RuntimeException(exception);
}
};
}
@GraphQLField
@JsonProperty(access = JsonProperty.Access.READ_WRITE)
protected String parent;
@JsonIgnore
private Supplier _parentSupplier;
@Schema
@Valid
public Object getQuery() {
if (_querySupplier != null) {
query = _querySupplier.get();
_querySupplier = null;
}
return query;
}
public void setQuery(Object query) {
this.query = query;
_querySupplier = null;
}
@JsonIgnore
public void setQuery(
UnsafeSupplier
© 2015 - 2025 Weber Informatics LLC | Privacy Policy