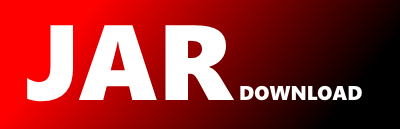
com.liferay.segments.service.persistence.SegmentsEntryPersistence Maven / Gradle / Ivy
/**
* SPDX-FileCopyrightText: (c) 2000 Liferay, Inc. https://liferay.com
* SPDX-License-Identifier: LGPL-2.1-or-later OR LicenseRef-Liferay-DXP-EULA-2.0.0-2023-06
*/
package com.liferay.segments.service.persistence;
import com.liferay.portal.kernel.service.persistence.BasePersistence;
import com.liferay.portal.kernel.service.persistence.change.tracking.CTPersistence;
import com.liferay.segments.exception.NoSuchEntryException;
import com.liferay.segments.model.SegmentsEntry;
import org.osgi.annotation.versioning.ProviderType;
/**
* The persistence interface for the segments entry service.
*
*
* Caching information and settings can be found in portal.properties
*
*
* @author Eduardo Garcia
* @see SegmentsEntryUtil
* @generated
*/
@ProviderType
public interface SegmentsEntryPersistence
extends BasePersistence, CTPersistence {
/*
* NOTE FOR DEVELOPERS:
*
* Never modify or reference this interface directly. Always use {@link SegmentsEntryUtil} to access the segments entry persistence. Modify service.xml
and rerun ServiceBuilder to regenerate this interface.
*/
/**
* Returns all the segments entries where uuid = ?.
*
* @param uuid the uuid
* @return the matching segments entries
*/
public java.util.List findByUuid(String uuid);
/**
* Returns a range of all the segments entries where uuid = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param uuid the uuid
* @param start the lower bound of the range of segments entries
* @param end the upper bound of the range of segments entries (not inclusive)
* @return the range of matching segments entries
*/
public java.util.List findByUuid(
String uuid, int start, int end);
/**
* Returns an ordered range of all the segments entries where uuid = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param uuid the uuid
* @param start the lower bound of the range of segments entries
* @param end the upper bound of the range of segments entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching segments entries
*/
public java.util.List findByUuid(
String uuid, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns an ordered range of all the segments entries where uuid = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param uuid the uuid
* @param start the lower bound of the range of segments entries
* @param end the upper bound of the range of segments entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching segments entries
*/
public java.util.List findByUuid(
String uuid, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator,
boolean useFinderCache);
/**
* Returns the first segments entry in the ordered set where uuid = ?.
*
* @param uuid the uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching segments entry
* @throws NoSuchEntryException if a matching segments entry could not be found
*/
public SegmentsEntry findByUuid_First(
String uuid,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryException;
/**
* Returns the first segments entry in the ordered set where uuid = ?.
*
* @param uuid the uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching segments entry, or null
if a matching segments entry could not be found
*/
public SegmentsEntry fetchByUuid_First(
String uuid,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the last segments entry in the ordered set where uuid = ?.
*
* @param uuid the uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching segments entry
* @throws NoSuchEntryException if a matching segments entry could not be found
*/
public SegmentsEntry findByUuid_Last(
String uuid,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryException;
/**
* Returns the last segments entry in the ordered set where uuid = ?.
*
* @param uuid the uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching segments entry, or null
if a matching segments entry could not be found
*/
public SegmentsEntry fetchByUuid_Last(
String uuid,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the segments entries before and after the current segments entry in the ordered set where uuid = ?.
*
* @param segmentsEntryId the primary key of the current segments entry
* @param uuid the uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next segments entry
* @throws NoSuchEntryException if a segments entry with the primary key could not be found
*/
public SegmentsEntry[] findByUuid_PrevAndNext(
long segmentsEntryId, String uuid,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryException;
/**
* Removes all the segments entries where uuid = ? from the database.
*
* @param uuid the uuid
*/
public void removeByUuid(String uuid);
/**
* Returns the number of segments entries where uuid = ?.
*
* @param uuid the uuid
* @return the number of matching segments entries
*/
public int countByUuid(String uuid);
/**
* Returns the segments entry where uuid = ? and groupId = ? or throws a NoSuchEntryException
if it could not be found.
*
* @param uuid the uuid
* @param groupId the group ID
* @return the matching segments entry
* @throws NoSuchEntryException if a matching segments entry could not be found
*/
public SegmentsEntry findByUUID_G(String uuid, long groupId)
throws NoSuchEntryException;
/**
* Returns the segments entry where uuid = ? and groupId = ? or returns null
if it could not be found. Uses the finder cache.
*
* @param uuid the uuid
* @param groupId the group ID
* @return the matching segments entry, or null
if a matching segments entry could not be found
*/
public SegmentsEntry fetchByUUID_G(String uuid, long groupId);
/**
* Returns the segments entry where uuid = ? and groupId = ? or returns null
if it could not be found, optionally using the finder cache.
*
* @param uuid the uuid
* @param groupId the group ID
* @param useFinderCache whether to use the finder cache
* @return the matching segments entry, or null
if a matching segments entry could not be found
*/
public SegmentsEntry fetchByUUID_G(
String uuid, long groupId, boolean useFinderCache);
/**
* Removes the segments entry where uuid = ? and groupId = ? from the database.
*
* @param uuid the uuid
* @param groupId the group ID
* @return the segments entry that was removed
*/
public SegmentsEntry removeByUUID_G(String uuid, long groupId)
throws NoSuchEntryException;
/**
* Returns the number of segments entries where uuid = ? and groupId = ?.
*
* @param uuid the uuid
* @param groupId the group ID
* @return the number of matching segments entries
*/
public int countByUUID_G(String uuid, long groupId);
/**
* Returns all the segments entries where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @return the matching segments entries
*/
public java.util.List findByUuid_C(
String uuid, long companyId);
/**
* Returns a range of all the segments entries where uuid = ? and companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param uuid the uuid
* @param companyId the company ID
* @param start the lower bound of the range of segments entries
* @param end the upper bound of the range of segments entries (not inclusive)
* @return the range of matching segments entries
*/
public java.util.List findByUuid_C(
String uuid, long companyId, int start, int end);
/**
* Returns an ordered range of all the segments entries where uuid = ? and companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param uuid the uuid
* @param companyId the company ID
* @param start the lower bound of the range of segments entries
* @param end the upper bound of the range of segments entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching segments entries
*/
public java.util.List findByUuid_C(
String uuid, long companyId, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns an ordered range of all the segments entries where uuid = ? and companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param uuid the uuid
* @param companyId the company ID
* @param start the lower bound of the range of segments entries
* @param end the upper bound of the range of segments entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching segments entries
*/
public java.util.List findByUuid_C(
String uuid, long companyId, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator,
boolean useFinderCache);
/**
* Returns the first segments entry in the ordered set where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching segments entry
* @throws NoSuchEntryException if a matching segments entry could not be found
*/
public SegmentsEntry findByUuid_C_First(
String uuid, long companyId,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryException;
/**
* Returns the first segments entry in the ordered set where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching segments entry, or null
if a matching segments entry could not be found
*/
public SegmentsEntry fetchByUuid_C_First(
String uuid, long companyId,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the last segments entry in the ordered set where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching segments entry
* @throws NoSuchEntryException if a matching segments entry could not be found
*/
public SegmentsEntry findByUuid_C_Last(
String uuid, long companyId,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryException;
/**
* Returns the last segments entry in the ordered set where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching segments entry, or null
if a matching segments entry could not be found
*/
public SegmentsEntry fetchByUuid_C_Last(
String uuid, long companyId,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the segments entries before and after the current segments entry in the ordered set where uuid = ? and companyId = ?.
*
* @param segmentsEntryId the primary key of the current segments entry
* @param uuid the uuid
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next segments entry
* @throws NoSuchEntryException if a segments entry with the primary key could not be found
*/
public SegmentsEntry[] findByUuid_C_PrevAndNext(
long segmentsEntryId, String uuid, long companyId,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryException;
/**
* Removes all the segments entries where uuid = ? and companyId = ? from the database.
*
* @param uuid the uuid
* @param companyId the company ID
*/
public void removeByUuid_C(String uuid, long companyId);
/**
* Returns the number of segments entries where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @return the number of matching segments entries
*/
public int countByUuid_C(String uuid, long companyId);
/**
* Returns all the segments entries where groupId = ?.
*
* @param groupId the group ID
* @return the matching segments entries
*/
public java.util.List findByGroupId(long groupId);
/**
* Returns a range of all the segments entries where groupId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param groupId the group ID
* @param start the lower bound of the range of segments entries
* @param end the upper bound of the range of segments entries (not inclusive)
* @return the range of matching segments entries
*/
public java.util.List findByGroupId(
long groupId, int start, int end);
/**
* Returns an ordered range of all the segments entries where groupId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param groupId the group ID
* @param start the lower bound of the range of segments entries
* @param end the upper bound of the range of segments entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching segments entries
*/
public java.util.List findByGroupId(
long groupId, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns an ordered range of all the segments entries where groupId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param groupId the group ID
* @param start the lower bound of the range of segments entries
* @param end the upper bound of the range of segments entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching segments entries
*/
public java.util.List findByGroupId(
long groupId, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator,
boolean useFinderCache);
/**
* Returns the first segments entry in the ordered set where groupId = ?.
*
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching segments entry
* @throws NoSuchEntryException if a matching segments entry could not be found
*/
public SegmentsEntry findByGroupId_First(
long groupId,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryException;
/**
* Returns the first segments entry in the ordered set where groupId = ?.
*
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching segments entry, or null
if a matching segments entry could not be found
*/
public SegmentsEntry fetchByGroupId_First(
long groupId,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the last segments entry in the ordered set where groupId = ?.
*
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching segments entry
* @throws NoSuchEntryException if a matching segments entry could not be found
*/
public SegmentsEntry findByGroupId_Last(
long groupId,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryException;
/**
* Returns the last segments entry in the ordered set where groupId = ?.
*
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching segments entry, or null
if a matching segments entry could not be found
*/
public SegmentsEntry fetchByGroupId_Last(
long groupId,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the segments entries before and after the current segments entry in the ordered set where groupId = ?.
*
* @param segmentsEntryId the primary key of the current segments entry
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next segments entry
* @throws NoSuchEntryException if a segments entry with the primary key could not be found
*/
public SegmentsEntry[] findByGroupId_PrevAndNext(
long segmentsEntryId, long groupId,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryException;
/**
* Returns all the segments entries that the user has permission to view where groupId = ?.
*
* @param groupId the group ID
* @return the matching segments entries that the user has permission to view
*/
public java.util.List filterFindByGroupId(long groupId);
/**
* Returns a range of all the segments entries that the user has permission to view where groupId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param groupId the group ID
* @param start the lower bound of the range of segments entries
* @param end the upper bound of the range of segments entries (not inclusive)
* @return the range of matching segments entries that the user has permission to view
*/
public java.util.List filterFindByGroupId(
long groupId, int start, int end);
/**
* Returns an ordered range of all the segments entries that the user has permissions to view where groupId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param groupId the group ID
* @param start the lower bound of the range of segments entries
* @param end the upper bound of the range of segments entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching segments entries that the user has permission to view
*/
public java.util.List filterFindByGroupId(
long groupId, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the segments entries before and after the current segments entry in the ordered set of segments entries that the user has permission to view where groupId = ?.
*
* @param segmentsEntryId the primary key of the current segments entry
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next segments entry
* @throws NoSuchEntryException if a segments entry with the primary key could not be found
*/
public SegmentsEntry[] filterFindByGroupId_PrevAndNext(
long segmentsEntryId, long groupId,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryException;
/**
* Returns all the segments entries that the user has permission to view where groupId = any ?.
*
* @param groupIds the group IDs
* @return the matching segments entries that the user has permission to view
*/
public java.util.List filterFindByGroupId(long[] groupIds);
/**
* Returns a range of all the segments entries that the user has permission to view where groupId = any ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param groupIds the group IDs
* @param start the lower bound of the range of segments entries
* @param end the upper bound of the range of segments entries (not inclusive)
* @return the range of matching segments entries that the user has permission to view
*/
public java.util.List filterFindByGroupId(
long[] groupIds, int start, int end);
/**
* Returns an ordered range of all the segments entries that the user has permission to view where groupId = any ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param groupIds the group IDs
* @param start the lower bound of the range of segments entries
* @param end the upper bound of the range of segments entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching segments entries that the user has permission to view
*/
public java.util.List filterFindByGroupId(
long[] groupIds, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns all the segments entries where groupId = any ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param groupIds the group IDs
* @return the matching segments entries
*/
public java.util.List findByGroupId(long[] groupIds);
/**
* Returns a range of all the segments entries where groupId = any ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param groupIds the group IDs
* @param start the lower bound of the range of segments entries
* @param end the upper bound of the range of segments entries (not inclusive)
* @return the range of matching segments entries
*/
public java.util.List findByGroupId(
long[] groupIds, int start, int end);
/**
* Returns an ordered range of all the segments entries where groupId = any ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param groupIds the group IDs
* @param start the lower bound of the range of segments entries
* @param end the upper bound of the range of segments entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching segments entries
*/
public java.util.List findByGroupId(
long[] groupIds, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns an ordered range of all the segments entries where groupId = ?, optionally using the finder cache.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param groupIds the group IDs
* @param start the lower bound of the range of segments entries
* @param end the upper bound of the range of segments entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching segments entries
*/
public java.util.List findByGroupId(
long[] groupIds, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator,
boolean useFinderCache);
/**
* Removes all the segments entries where groupId = ? from the database.
*
* @param groupId the group ID
*/
public void removeByGroupId(long groupId);
/**
* Returns the number of segments entries where groupId = ?.
*
* @param groupId the group ID
* @return the number of matching segments entries
*/
public int countByGroupId(long groupId);
/**
* Returns the number of segments entries where groupId = any ?.
*
* @param groupIds the group IDs
* @return the number of matching segments entries
*/
public int countByGroupId(long[] groupIds);
/**
* Returns the number of segments entries that the user has permission to view where groupId = ?.
*
* @param groupId the group ID
* @return the number of matching segments entries that the user has permission to view
*/
public int filterCountByGroupId(long groupId);
/**
* Returns the number of segments entries that the user has permission to view where groupId = any ?.
*
* @param groupIds the group IDs
* @return the number of matching segments entries that the user has permission to view
*/
public int filterCountByGroupId(long[] groupIds);
/**
* Returns all the segments entries where companyId = ?.
*
* @param companyId the company ID
* @return the matching segments entries
*/
public java.util.List findByCompanyId(long companyId);
/**
* Returns a range of all the segments entries where companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param companyId the company ID
* @param start the lower bound of the range of segments entries
* @param end the upper bound of the range of segments entries (not inclusive)
* @return the range of matching segments entries
*/
public java.util.List findByCompanyId(
long companyId, int start, int end);
/**
* Returns an ordered range of all the segments entries where companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param companyId the company ID
* @param start the lower bound of the range of segments entries
* @param end the upper bound of the range of segments entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching segments entries
*/
public java.util.List findByCompanyId(
long companyId, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns an ordered range of all the segments entries where companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param companyId the company ID
* @param start the lower bound of the range of segments entries
* @param end the upper bound of the range of segments entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching segments entries
*/
public java.util.List findByCompanyId(
long companyId, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator,
boolean useFinderCache);
/**
* Returns the first segments entry in the ordered set where companyId = ?.
*
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching segments entry
* @throws NoSuchEntryException if a matching segments entry could not be found
*/
public SegmentsEntry findByCompanyId_First(
long companyId,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryException;
/**
* Returns the first segments entry in the ordered set where companyId = ?.
*
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching segments entry, or null
if a matching segments entry could not be found
*/
public SegmentsEntry fetchByCompanyId_First(
long companyId,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the last segments entry in the ordered set where companyId = ?.
*
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching segments entry
* @throws NoSuchEntryException if a matching segments entry could not be found
*/
public SegmentsEntry findByCompanyId_Last(
long companyId,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryException;
/**
* Returns the last segments entry in the ordered set where companyId = ?.
*
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching segments entry, or null
if a matching segments entry could not be found
*/
public SegmentsEntry fetchByCompanyId_Last(
long companyId,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the segments entries before and after the current segments entry in the ordered set where companyId = ?.
*
* @param segmentsEntryId the primary key of the current segments entry
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next segments entry
* @throws NoSuchEntryException if a segments entry with the primary key could not be found
*/
public SegmentsEntry[] findByCompanyId_PrevAndNext(
long segmentsEntryId, long companyId,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryException;
/**
* Returns all the segments entries where companyId = any ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param companyIds the company IDs
* @return the matching segments entries
*/
public java.util.List findByCompanyId(long[] companyIds);
/**
* Returns a range of all the segments entries where companyId = any ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param companyIds the company IDs
* @param start the lower bound of the range of segments entries
* @param end the upper bound of the range of segments entries (not inclusive)
* @return the range of matching segments entries
*/
public java.util.List findByCompanyId(
long[] companyIds, int start, int end);
/**
* Returns an ordered range of all the segments entries where companyId = any ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param companyIds the company IDs
* @param start the lower bound of the range of segments entries
* @param end the upper bound of the range of segments entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching segments entries
*/
public java.util.List findByCompanyId(
long[] companyIds, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns an ordered range of all the segments entries where companyId = ?, optionally using the finder cache.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param companyIds the company IDs
* @param start the lower bound of the range of segments entries
* @param end the upper bound of the range of segments entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching segments entries
*/
public java.util.List findByCompanyId(
long[] companyIds, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator,
boolean useFinderCache);
/**
* Removes all the segments entries where companyId = ? from the database.
*
* @param companyId the company ID
*/
public void removeByCompanyId(long companyId);
/**
* Returns the number of segments entries where companyId = ?.
*
* @param companyId the company ID
* @return the number of matching segments entries
*/
public int countByCompanyId(long companyId);
/**
* Returns the number of segments entries where companyId = any ?.
*
* @param companyIds the company IDs
* @return the number of matching segments entries
*/
public int countByCompanyId(long[] companyIds);
/**
* Returns all the segments entries where active = ?.
*
* @param active the active
* @return the matching segments entries
*/
public java.util.List findByActive(boolean active);
/**
* Returns a range of all the segments entries where active = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param active the active
* @param start the lower bound of the range of segments entries
* @param end the upper bound of the range of segments entries (not inclusive)
* @return the range of matching segments entries
*/
public java.util.List findByActive(
boolean active, int start, int end);
/**
* Returns an ordered range of all the segments entries where active = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param active the active
* @param start the lower bound of the range of segments entries
* @param end the upper bound of the range of segments entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching segments entries
*/
public java.util.List findByActive(
boolean active, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns an ordered range of all the segments entries where active = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param active the active
* @param start the lower bound of the range of segments entries
* @param end the upper bound of the range of segments entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching segments entries
*/
public java.util.List findByActive(
boolean active, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator,
boolean useFinderCache);
/**
* Returns the first segments entry in the ordered set where active = ?.
*
* @param active the active
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching segments entry
* @throws NoSuchEntryException if a matching segments entry could not be found
*/
public SegmentsEntry findByActive_First(
boolean active,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryException;
/**
* Returns the first segments entry in the ordered set where active = ?.
*
* @param active the active
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching segments entry, or null
if a matching segments entry could not be found
*/
public SegmentsEntry fetchByActive_First(
boolean active,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the last segments entry in the ordered set where active = ?.
*
* @param active the active
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching segments entry
* @throws NoSuchEntryException if a matching segments entry could not be found
*/
public SegmentsEntry findByActive_Last(
boolean active,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryException;
/**
* Returns the last segments entry in the ordered set where active = ?.
*
* @param active the active
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching segments entry, or null
if a matching segments entry could not be found
*/
public SegmentsEntry fetchByActive_Last(
boolean active,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the segments entries before and after the current segments entry in the ordered set where active = ?.
*
* @param segmentsEntryId the primary key of the current segments entry
* @param active the active
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next segments entry
* @throws NoSuchEntryException if a segments entry with the primary key could not be found
*/
public SegmentsEntry[] findByActive_PrevAndNext(
long segmentsEntryId, boolean active,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryException;
/**
* Removes all the segments entries where active = ? from the database.
*
* @param active the active
*/
public void removeByActive(boolean active);
/**
* Returns the number of segments entries where active = ?.
*
* @param active the active
* @return the number of matching segments entries
*/
public int countByActive(boolean active);
/**
* Returns all the segments entries where source = ?.
*
* @param source the source
* @return the matching segments entries
*/
public java.util.List findBySource(String source);
/**
* Returns a range of all the segments entries where source = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param source the source
* @param start the lower bound of the range of segments entries
* @param end the upper bound of the range of segments entries (not inclusive)
* @return the range of matching segments entries
*/
public java.util.List findBySource(
String source, int start, int end);
/**
* Returns an ordered range of all the segments entries where source = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param source the source
* @param start the lower bound of the range of segments entries
* @param end the upper bound of the range of segments entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching segments entries
*/
public java.util.List findBySource(
String source, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns an ordered range of all the segments entries where source = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param source the source
* @param start the lower bound of the range of segments entries
* @param end the upper bound of the range of segments entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching segments entries
*/
public java.util.List findBySource(
String source, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator,
boolean useFinderCache);
/**
* Returns the first segments entry in the ordered set where source = ?.
*
* @param source the source
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching segments entry
* @throws NoSuchEntryException if a matching segments entry could not be found
*/
public SegmentsEntry findBySource_First(
String source,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryException;
/**
* Returns the first segments entry in the ordered set where source = ?.
*
* @param source the source
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching segments entry, or null
if a matching segments entry could not be found
*/
public SegmentsEntry fetchBySource_First(
String source,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the last segments entry in the ordered set where source = ?.
*
* @param source the source
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching segments entry
* @throws NoSuchEntryException if a matching segments entry could not be found
*/
public SegmentsEntry findBySource_Last(
String source,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryException;
/**
* Returns the last segments entry in the ordered set where source = ?.
*
* @param source the source
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching segments entry, or null
if a matching segments entry could not be found
*/
public SegmentsEntry fetchBySource_Last(
String source,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the segments entries before and after the current segments entry in the ordered set where source = ?.
*
* @param segmentsEntryId the primary key of the current segments entry
* @param source the source
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next segments entry
* @throws NoSuchEntryException if a segments entry with the primary key could not be found
*/
public SegmentsEntry[] findBySource_PrevAndNext(
long segmentsEntryId, String source,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryException;
/**
* Removes all the segments entries where source = ? from the database.
*
* @param source the source
*/
public void removeBySource(String source);
/**
* Returns the number of segments entries where source = ?.
*
* @param source the source
* @return the number of matching segments entries
*/
public int countBySource(String source);
/**
* Returns the segments entry where groupId = ? and segmentsEntryKey = ? or throws a NoSuchEntryException
if it could not be found.
*
* @param groupId the group ID
* @param segmentsEntryKey the segments entry key
* @return the matching segments entry
* @throws NoSuchEntryException if a matching segments entry could not be found
*/
public SegmentsEntry findByG_S(long groupId, String segmentsEntryKey)
throws NoSuchEntryException;
/**
* Returns the segments entry where groupId = ? and segmentsEntryKey = ? or returns null
if it could not be found. Uses the finder cache.
*
* @param groupId the group ID
* @param segmentsEntryKey the segments entry key
* @return the matching segments entry, or null
if a matching segments entry could not be found
*/
public SegmentsEntry fetchByG_S(long groupId, String segmentsEntryKey);
/**
* Returns the segments entry where groupId = ? and segmentsEntryKey = ? or returns null
if it could not be found, optionally using the finder cache.
*
* @param groupId the group ID
* @param segmentsEntryKey the segments entry key
* @param useFinderCache whether to use the finder cache
* @return the matching segments entry, or null
if a matching segments entry could not be found
*/
public SegmentsEntry fetchByG_S(
long groupId, String segmentsEntryKey, boolean useFinderCache);
/**
* Removes the segments entry where groupId = ? and segmentsEntryKey = ? from the database.
*
* @param groupId the group ID
* @param segmentsEntryKey the segments entry key
* @return the segments entry that was removed
*/
public SegmentsEntry removeByG_S(long groupId, String segmentsEntryKey)
throws NoSuchEntryException;
/**
* Returns the number of segments entries where groupId = ? and segmentsEntryKey = ?.
*
* @param groupId the group ID
* @param segmentsEntryKey the segments entry key
* @return the number of matching segments entries
*/
public int countByG_S(long groupId, String segmentsEntryKey);
/**
* Returns all the segments entries where groupId = ? and active = ?.
*
* @param groupId the group ID
* @param active the active
* @return the matching segments entries
*/
public java.util.List findByG_A(
long groupId, boolean active);
/**
* Returns a range of all the segments entries where groupId = ? and active = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param groupId the group ID
* @param active the active
* @param start the lower bound of the range of segments entries
* @param end the upper bound of the range of segments entries (not inclusive)
* @return the range of matching segments entries
*/
public java.util.List findByG_A(
long groupId, boolean active, int start, int end);
/**
* Returns an ordered range of all the segments entries where groupId = ? and active = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param groupId the group ID
* @param active the active
* @param start the lower bound of the range of segments entries
* @param end the upper bound of the range of segments entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching segments entries
*/
public java.util.List findByG_A(
long groupId, boolean active, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns an ordered range of all the segments entries where groupId = ? and active = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param groupId the group ID
* @param active the active
* @param start the lower bound of the range of segments entries
* @param end the upper bound of the range of segments entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching segments entries
*/
public java.util.List findByG_A(
long groupId, boolean active, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator,
boolean useFinderCache);
/**
* Returns the first segments entry in the ordered set where groupId = ? and active = ?.
*
* @param groupId the group ID
* @param active the active
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching segments entry
* @throws NoSuchEntryException if a matching segments entry could not be found
*/
public SegmentsEntry findByG_A_First(
long groupId, boolean active,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryException;
/**
* Returns the first segments entry in the ordered set where groupId = ? and active = ?.
*
* @param groupId the group ID
* @param active the active
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching segments entry, or null
if a matching segments entry could not be found
*/
public SegmentsEntry fetchByG_A_First(
long groupId, boolean active,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the last segments entry in the ordered set where groupId = ? and active = ?.
*
* @param groupId the group ID
* @param active the active
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching segments entry
* @throws NoSuchEntryException if a matching segments entry could not be found
*/
public SegmentsEntry findByG_A_Last(
long groupId, boolean active,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryException;
/**
* Returns the last segments entry in the ordered set where groupId = ? and active = ?.
*
* @param groupId the group ID
* @param active the active
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching segments entry, or null
if a matching segments entry could not be found
*/
public SegmentsEntry fetchByG_A_Last(
long groupId, boolean active,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the segments entries before and after the current segments entry in the ordered set where groupId = ? and active = ?.
*
* @param segmentsEntryId the primary key of the current segments entry
* @param groupId the group ID
* @param active the active
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next segments entry
* @throws NoSuchEntryException if a segments entry with the primary key could not be found
*/
public SegmentsEntry[] findByG_A_PrevAndNext(
long segmentsEntryId, long groupId, boolean active,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryException;
/**
* Returns all the segments entries that the user has permission to view where groupId = ? and active = ?.
*
* @param groupId the group ID
* @param active the active
* @return the matching segments entries that the user has permission to view
*/
public java.util.List filterFindByG_A(
long groupId, boolean active);
/**
* Returns a range of all the segments entries that the user has permission to view where groupId = ? and active = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param groupId the group ID
* @param active the active
* @param start the lower bound of the range of segments entries
* @param end the upper bound of the range of segments entries (not inclusive)
* @return the range of matching segments entries that the user has permission to view
*/
public java.util.List filterFindByG_A(
long groupId, boolean active, int start, int end);
/**
* Returns an ordered range of all the segments entries that the user has permissions to view where groupId = ? and active = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param groupId the group ID
* @param active the active
* @param start the lower bound of the range of segments entries
* @param end the upper bound of the range of segments entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching segments entries that the user has permission to view
*/
public java.util.List filterFindByG_A(
long groupId, boolean active, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the segments entries before and after the current segments entry in the ordered set of segments entries that the user has permission to view where groupId = ? and active = ?.
*
* @param segmentsEntryId the primary key of the current segments entry
* @param groupId the group ID
* @param active the active
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next segments entry
* @throws NoSuchEntryException if a segments entry with the primary key could not be found
*/
public SegmentsEntry[] filterFindByG_A_PrevAndNext(
long segmentsEntryId, long groupId, boolean active,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryException;
/**
* Returns all the segments entries that the user has permission to view where groupId = any ? and active = ?.
*
* @param groupIds the group IDs
* @param active the active
* @return the matching segments entries that the user has permission to view
*/
public java.util.List filterFindByG_A(
long[] groupIds, boolean active);
/**
* Returns a range of all the segments entries that the user has permission to view where groupId = any ? and active = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param groupIds the group IDs
* @param active the active
* @param start the lower bound of the range of segments entries
* @param end the upper bound of the range of segments entries (not inclusive)
* @return the range of matching segments entries that the user has permission to view
*/
public java.util.List filterFindByG_A(
long[] groupIds, boolean active, int start, int end);
/**
* Returns an ordered range of all the segments entries that the user has permission to view where groupId = any ? and active = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param groupIds the group IDs
* @param active the active
* @param start the lower bound of the range of segments entries
* @param end the upper bound of the range of segments entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching segments entries that the user has permission to view
*/
public java.util.List filterFindByG_A(
long[] groupIds, boolean active, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns all the segments entries where groupId = any ? and active = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param groupIds the group IDs
* @param active the active
* @return the matching segments entries
*/
public java.util.List findByG_A(
long[] groupIds, boolean active);
/**
* Returns a range of all the segments entries where groupId = any ? and active = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param groupIds the group IDs
* @param active the active
* @param start the lower bound of the range of segments entries
* @param end the upper bound of the range of segments entries (not inclusive)
* @return the range of matching segments entries
*/
public java.util.List findByG_A(
long[] groupIds, boolean active, int start, int end);
/**
* Returns an ordered range of all the segments entries where groupId = any ? and active = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param groupIds the group IDs
* @param active the active
* @param start the lower bound of the range of segments entries
* @param end the upper bound of the range of segments entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching segments entries
*/
public java.util.List findByG_A(
long[] groupIds, boolean active, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns an ordered range of all the segments entries where groupId = ? and active = ?, optionally using the finder cache.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param groupIds the group IDs
* @param active the active
* @param start the lower bound of the range of segments entries
* @param end the upper bound of the range of segments entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching segments entries
*/
public java.util.List findByG_A(
long[] groupIds, boolean active, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator,
boolean useFinderCache);
/**
* Removes all the segments entries where groupId = ? and active = ? from the database.
*
* @param groupId the group ID
* @param active the active
*/
public void removeByG_A(long groupId, boolean active);
/**
* Returns the number of segments entries where groupId = ? and active = ?.
*
* @param groupId the group ID
* @param active the active
* @return the number of matching segments entries
*/
public int countByG_A(long groupId, boolean active);
/**
* Returns the number of segments entries where groupId = any ? and active = ?.
*
* @param groupIds the group IDs
* @param active the active
* @return the number of matching segments entries
*/
public int countByG_A(long[] groupIds, boolean active);
/**
* Returns the number of segments entries that the user has permission to view where groupId = ? and active = ?.
*
* @param groupId the group ID
* @param active the active
* @return the number of matching segments entries that the user has permission to view
*/
public int filterCountByG_A(long groupId, boolean active);
/**
* Returns the number of segments entries that the user has permission to view where groupId = any ? and active = ?.
*
* @param groupIds the group IDs
* @param active the active
* @return the number of matching segments entries that the user has permission to view
*/
public int filterCountByG_A(long[] groupIds, boolean active);
/**
* Returns all the segments entries where groupId = ? and source = ?.
*
* @param groupId the group ID
* @param source the source
* @return the matching segments entries
*/
public java.util.List findByG_SRC(
long groupId, String source);
/**
* Returns a range of all the segments entries where groupId = ? and source = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param groupId the group ID
* @param source the source
* @param start the lower bound of the range of segments entries
* @param end the upper bound of the range of segments entries (not inclusive)
* @return the range of matching segments entries
*/
public java.util.List findByG_SRC(
long groupId, String source, int start, int end);
/**
* Returns an ordered range of all the segments entries where groupId = ? and source = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param groupId the group ID
* @param source the source
* @param start the lower bound of the range of segments entries
* @param end the upper bound of the range of segments entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching segments entries
*/
public java.util.List findByG_SRC(
long groupId, String source, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns an ordered range of all the segments entries where groupId = ? and source = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param groupId the group ID
* @param source the source
* @param start the lower bound of the range of segments entries
* @param end the upper bound of the range of segments entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching segments entries
*/
public java.util.List findByG_SRC(
long groupId, String source, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator,
boolean useFinderCache);
/**
* Returns the first segments entry in the ordered set where groupId = ? and source = ?.
*
* @param groupId the group ID
* @param source the source
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching segments entry
* @throws NoSuchEntryException if a matching segments entry could not be found
*/
public SegmentsEntry findByG_SRC_First(
long groupId, String source,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryException;
/**
* Returns the first segments entry in the ordered set where groupId = ? and source = ?.
*
* @param groupId the group ID
* @param source the source
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching segments entry, or null
if a matching segments entry could not be found
*/
public SegmentsEntry fetchByG_SRC_First(
long groupId, String source,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the last segments entry in the ordered set where groupId = ? and source = ?.
*
* @param groupId the group ID
* @param source the source
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching segments entry
* @throws NoSuchEntryException if a matching segments entry could not be found
*/
public SegmentsEntry findByG_SRC_Last(
long groupId, String source,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryException;
/**
* Returns the last segments entry in the ordered set where groupId = ? and source = ?.
*
* @param groupId the group ID
* @param source the source
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching segments entry, or null
if a matching segments entry could not be found
*/
public SegmentsEntry fetchByG_SRC_Last(
long groupId, String source,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the segments entries before and after the current segments entry in the ordered set where groupId = ? and source = ?.
*
* @param segmentsEntryId the primary key of the current segments entry
* @param groupId the group ID
* @param source the source
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next segments entry
* @throws NoSuchEntryException if a segments entry with the primary key could not be found
*/
public SegmentsEntry[] findByG_SRC_PrevAndNext(
long segmentsEntryId, long groupId, String source,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryException;
/**
* Returns all the segments entries that the user has permission to view where groupId = ? and source = ?.
*
* @param groupId the group ID
* @param source the source
* @return the matching segments entries that the user has permission to view
*/
public java.util.List filterFindByG_SRC(
long groupId, String source);
/**
* Returns a range of all the segments entries that the user has permission to view where groupId = ? and source = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param groupId the group ID
* @param source the source
* @param start the lower bound of the range of segments entries
* @param end the upper bound of the range of segments entries (not inclusive)
* @return the range of matching segments entries that the user has permission to view
*/
public java.util.List filterFindByG_SRC(
long groupId, String source, int start, int end);
/**
* Returns an ordered range of all the segments entries that the user has permissions to view where groupId = ? and source = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param groupId the group ID
* @param source the source
* @param start the lower bound of the range of segments entries
* @param end the upper bound of the range of segments entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching segments entries that the user has permission to view
*/
public java.util.List filterFindByG_SRC(
long groupId, String source, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the segments entries before and after the current segments entry in the ordered set of segments entries that the user has permission to view where groupId = ? and source = ?.
*
* @param segmentsEntryId the primary key of the current segments entry
* @param groupId the group ID
* @param source the source
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next segments entry
* @throws NoSuchEntryException if a segments entry with the primary key could not be found
*/
public SegmentsEntry[] filterFindByG_SRC_PrevAndNext(
long segmentsEntryId, long groupId, String source,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryException;
/**
* Returns all the segments entries that the user has permission to view where groupId = any ? and source = ?.
*
* @param groupIds the group IDs
* @param source the source
* @return the matching segments entries that the user has permission to view
*/
public java.util.List filterFindByG_SRC(
long[] groupIds, String source);
/**
* Returns a range of all the segments entries that the user has permission to view where groupId = any ? and source = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param groupIds the group IDs
* @param source the source
* @param start the lower bound of the range of segments entries
* @param end the upper bound of the range of segments entries (not inclusive)
* @return the range of matching segments entries that the user has permission to view
*/
public java.util.List filterFindByG_SRC(
long[] groupIds, String source, int start, int end);
/**
* Returns an ordered range of all the segments entries that the user has permission to view where groupId = any ? and source = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param groupIds the group IDs
* @param source the source
* @param start the lower bound of the range of segments entries
* @param end the upper bound of the range of segments entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching segments entries that the user has permission to view
*/
public java.util.List filterFindByG_SRC(
long[] groupIds, String source, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns all the segments entries where groupId = any ? and source = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param groupIds the group IDs
* @param source the source
* @return the matching segments entries
*/
public java.util.List findByG_SRC(
long[] groupIds, String source);
/**
* Returns a range of all the segments entries where groupId = any ? and source = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param groupIds the group IDs
* @param source the source
* @param start the lower bound of the range of segments entries
* @param end the upper bound of the range of segments entries (not inclusive)
* @return the range of matching segments entries
*/
public java.util.List findByG_SRC(
long[] groupIds, String source, int start, int end);
/**
* Returns an ordered range of all the segments entries where groupId = any ? and source = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param groupIds the group IDs
* @param source the source
* @param start the lower bound of the range of segments entries
* @param end the upper bound of the range of segments entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching segments entries
*/
public java.util.List findByG_SRC(
long[] groupIds, String source, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns an ordered range of all the segments entries where groupId = ? and source = ?, optionally using the finder cache.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param groupIds the group IDs
* @param source the source
* @param start the lower bound of the range of segments entries
* @param end the upper bound of the range of segments entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching segments entries
*/
public java.util.List findByG_SRC(
long[] groupIds, String source, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator,
boolean useFinderCache);
/**
* Removes all the segments entries where groupId = ? and source = ? from the database.
*
* @param groupId the group ID
* @param source the source
*/
public void removeByG_SRC(long groupId, String source);
/**
* Returns the number of segments entries where groupId = ? and source = ?.
*
* @param groupId the group ID
* @param source the source
* @return the number of matching segments entries
*/
public int countByG_SRC(long groupId, String source);
/**
* Returns the number of segments entries where groupId = any ? and source = ?.
*
* @param groupIds the group IDs
* @param source the source
* @return the number of matching segments entries
*/
public int countByG_SRC(long[] groupIds, String source);
/**
* Returns the number of segments entries that the user has permission to view where groupId = ? and source = ?.
*
* @param groupId the group ID
* @param source the source
* @return the number of matching segments entries that the user has permission to view
*/
public int filterCountByG_SRC(long groupId, String source);
/**
* Returns the number of segments entries that the user has permission to view where groupId = any ? and source = ?.
*
* @param groupIds the group IDs
* @param source the source
* @return the number of matching segments entries that the user has permission to view
*/
public int filterCountByG_SRC(long[] groupIds, String source);
/**
* Caches the segments entry in the entity cache if it is enabled.
*
* @param segmentsEntry the segments entry
*/
public void cacheResult(SegmentsEntry segmentsEntry);
/**
* Caches the segments entries in the entity cache if it is enabled.
*
* @param segmentsEntries the segments entries
*/
public void cacheResult(java.util.List segmentsEntries);
/**
* Creates a new segments entry with the primary key. Does not add the segments entry to the database.
*
* @param segmentsEntryId the primary key for the new segments entry
* @return the new segments entry
*/
public SegmentsEntry create(long segmentsEntryId);
/**
* Removes the segments entry with the primary key from the database. Also notifies the appropriate model listeners.
*
* @param segmentsEntryId the primary key of the segments entry
* @return the segments entry that was removed
* @throws NoSuchEntryException if a segments entry with the primary key could not be found
*/
public SegmentsEntry remove(long segmentsEntryId)
throws NoSuchEntryException;
public SegmentsEntry updateImpl(SegmentsEntry segmentsEntry);
/**
* Returns the segments entry with the primary key or throws a NoSuchEntryException
if it could not be found.
*
* @param segmentsEntryId the primary key of the segments entry
* @return the segments entry
* @throws NoSuchEntryException if a segments entry with the primary key could not be found
*/
public SegmentsEntry findByPrimaryKey(long segmentsEntryId)
throws NoSuchEntryException;
/**
* Returns the segments entry with the primary key or returns null
if it could not be found.
*
* @param segmentsEntryId the primary key of the segments entry
* @return the segments entry, or null
if a segments entry with the primary key could not be found
*/
public SegmentsEntry fetchByPrimaryKey(long segmentsEntryId);
/**
* Returns all the segments entries.
*
* @return the segments entries
*/
public java.util.List findAll();
/**
* Returns a range of all the segments entries.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param start the lower bound of the range of segments entries
* @param end the upper bound of the range of segments entries (not inclusive)
* @return the range of segments entries
*/
public java.util.List findAll(int start, int end);
/**
* Returns an ordered range of all the segments entries.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param start the lower bound of the range of segments entries
* @param end the upper bound of the range of segments entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of segments entries
*/
public java.util.List findAll(
int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns an ordered range of all the segments entries.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryModelImpl
.
*
*
* @param start the lower bound of the range of segments entries
* @param end the upper bound of the range of segments entries (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of segments entries
*/
public java.util.List findAll(
int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator,
boolean useFinderCache);
/**
* Removes all the segments entries from the database.
*/
public void removeAll();
/**
* Returns the number of segments entries.
*
* @return the number of segments entries
*/
public int countAll();
}