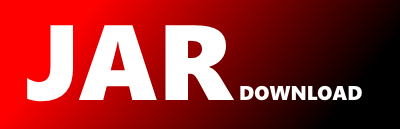
com.liferay.segments.service.persistence.SegmentsEntryRelPersistence Maven / Gradle / Ivy
/**
* SPDX-FileCopyrightText: (c) 2000 Liferay, Inc. https://liferay.com
* SPDX-License-Identifier: LGPL-2.1-or-later OR LicenseRef-Liferay-DXP-EULA-2.0.0-2023-06
*/
package com.liferay.segments.service.persistence;
import com.liferay.portal.kernel.service.persistence.BasePersistence;
import com.liferay.portal.kernel.service.persistence.change.tracking.CTPersistence;
import com.liferay.segments.exception.NoSuchEntryRelException;
import com.liferay.segments.model.SegmentsEntryRel;
import org.osgi.annotation.versioning.ProviderType;
/**
* The persistence interface for the segments entry rel service.
*
*
* Caching information and settings can be found in portal.properties
*
*
* @author Eduardo Garcia
* @see SegmentsEntryRelUtil
* @generated
*/
@ProviderType
public interface SegmentsEntryRelPersistence
extends BasePersistence, CTPersistence {
/*
* NOTE FOR DEVELOPERS:
*
* Never modify or reference this interface directly. Always use {@link SegmentsEntryRelUtil} to access the segments entry rel persistence. Modify service.xml
and rerun ServiceBuilder to regenerate this interface.
*/
/**
* Returns all the segments entry rels where segmentsEntryId = ?.
*
* @param segmentsEntryId the segments entry ID
* @return the matching segments entry rels
*/
public java.util.List findBySegmentsEntryId(
long segmentsEntryId);
/**
* Returns a range of all the segments entry rels where segmentsEntryId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryRelModelImpl
.
*
*
* @param segmentsEntryId the segments entry ID
* @param start the lower bound of the range of segments entry rels
* @param end the upper bound of the range of segments entry rels (not inclusive)
* @return the range of matching segments entry rels
*/
public java.util.List findBySegmentsEntryId(
long segmentsEntryId, int start, int end);
/**
* Returns an ordered range of all the segments entry rels where segmentsEntryId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryRelModelImpl
.
*
*
* @param segmentsEntryId the segments entry ID
* @param start the lower bound of the range of segments entry rels
* @param end the upper bound of the range of segments entry rels (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching segments entry rels
*/
public java.util.List findBySegmentsEntryId(
long segmentsEntryId, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns an ordered range of all the segments entry rels where segmentsEntryId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryRelModelImpl
.
*
*
* @param segmentsEntryId the segments entry ID
* @param start the lower bound of the range of segments entry rels
* @param end the upper bound of the range of segments entry rels (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching segments entry rels
*/
public java.util.List findBySegmentsEntryId(
long segmentsEntryId, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator,
boolean useFinderCache);
/**
* Returns the first segments entry rel in the ordered set where segmentsEntryId = ?.
*
* @param segmentsEntryId the segments entry ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching segments entry rel
* @throws NoSuchEntryRelException if a matching segments entry rel could not be found
*/
public SegmentsEntryRel findBySegmentsEntryId_First(
long segmentsEntryId,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryRelException;
/**
* Returns the first segments entry rel in the ordered set where segmentsEntryId = ?.
*
* @param segmentsEntryId the segments entry ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching segments entry rel, or null
if a matching segments entry rel could not be found
*/
public SegmentsEntryRel fetchBySegmentsEntryId_First(
long segmentsEntryId,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the last segments entry rel in the ordered set where segmentsEntryId = ?.
*
* @param segmentsEntryId the segments entry ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching segments entry rel
* @throws NoSuchEntryRelException if a matching segments entry rel could not be found
*/
public SegmentsEntryRel findBySegmentsEntryId_Last(
long segmentsEntryId,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryRelException;
/**
* Returns the last segments entry rel in the ordered set where segmentsEntryId = ?.
*
* @param segmentsEntryId the segments entry ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching segments entry rel, or null
if a matching segments entry rel could not be found
*/
public SegmentsEntryRel fetchBySegmentsEntryId_Last(
long segmentsEntryId,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the segments entry rels before and after the current segments entry rel in the ordered set where segmentsEntryId = ?.
*
* @param segmentsEntryRelId the primary key of the current segments entry rel
* @param segmentsEntryId the segments entry ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next segments entry rel
* @throws NoSuchEntryRelException if a segments entry rel with the primary key could not be found
*/
public SegmentsEntryRel[] findBySegmentsEntryId_PrevAndNext(
long segmentsEntryRelId, long segmentsEntryId,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryRelException;
/**
* Removes all the segments entry rels where segmentsEntryId = ? from the database.
*
* @param segmentsEntryId the segments entry ID
*/
public void removeBySegmentsEntryId(long segmentsEntryId);
/**
* Returns the number of segments entry rels where segmentsEntryId = ?.
*
* @param segmentsEntryId the segments entry ID
* @return the number of matching segments entry rels
*/
public int countBySegmentsEntryId(long segmentsEntryId);
/**
* Returns all the segments entry rels where classNameId = ? and classPK = ?.
*
* @param classNameId the class name ID
* @param classPK the class pk
* @return the matching segments entry rels
*/
public java.util.List findByCN_CPK(
long classNameId, long classPK);
/**
* Returns a range of all the segments entry rels where classNameId = ? and classPK = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryRelModelImpl
.
*
*
* @param classNameId the class name ID
* @param classPK the class pk
* @param start the lower bound of the range of segments entry rels
* @param end the upper bound of the range of segments entry rels (not inclusive)
* @return the range of matching segments entry rels
*/
public java.util.List findByCN_CPK(
long classNameId, long classPK, int start, int end);
/**
* Returns an ordered range of all the segments entry rels where classNameId = ? and classPK = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryRelModelImpl
.
*
*
* @param classNameId the class name ID
* @param classPK the class pk
* @param start the lower bound of the range of segments entry rels
* @param end the upper bound of the range of segments entry rels (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching segments entry rels
*/
public java.util.List findByCN_CPK(
long classNameId, long classPK, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns an ordered range of all the segments entry rels where classNameId = ? and classPK = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryRelModelImpl
.
*
*
* @param classNameId the class name ID
* @param classPK the class pk
* @param start the lower bound of the range of segments entry rels
* @param end the upper bound of the range of segments entry rels (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching segments entry rels
*/
public java.util.List findByCN_CPK(
long classNameId, long classPK, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator,
boolean useFinderCache);
/**
* Returns the first segments entry rel in the ordered set where classNameId = ? and classPK = ?.
*
* @param classNameId the class name ID
* @param classPK the class pk
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching segments entry rel
* @throws NoSuchEntryRelException if a matching segments entry rel could not be found
*/
public SegmentsEntryRel findByCN_CPK_First(
long classNameId, long classPK,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryRelException;
/**
* Returns the first segments entry rel in the ordered set where classNameId = ? and classPK = ?.
*
* @param classNameId the class name ID
* @param classPK the class pk
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching segments entry rel, or null
if a matching segments entry rel could not be found
*/
public SegmentsEntryRel fetchByCN_CPK_First(
long classNameId, long classPK,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the last segments entry rel in the ordered set where classNameId = ? and classPK = ?.
*
* @param classNameId the class name ID
* @param classPK the class pk
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching segments entry rel
* @throws NoSuchEntryRelException if a matching segments entry rel could not be found
*/
public SegmentsEntryRel findByCN_CPK_Last(
long classNameId, long classPK,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryRelException;
/**
* Returns the last segments entry rel in the ordered set where classNameId = ? and classPK = ?.
*
* @param classNameId the class name ID
* @param classPK the class pk
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching segments entry rel, or null
if a matching segments entry rel could not be found
*/
public SegmentsEntryRel fetchByCN_CPK_Last(
long classNameId, long classPK,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the segments entry rels before and after the current segments entry rel in the ordered set where classNameId = ? and classPK = ?.
*
* @param segmentsEntryRelId the primary key of the current segments entry rel
* @param classNameId the class name ID
* @param classPK the class pk
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next segments entry rel
* @throws NoSuchEntryRelException if a segments entry rel with the primary key could not be found
*/
public SegmentsEntryRel[] findByCN_CPK_PrevAndNext(
long segmentsEntryRelId, long classNameId, long classPK,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryRelException;
/**
* Removes all the segments entry rels where classNameId = ? and classPK = ? from the database.
*
* @param classNameId the class name ID
* @param classPK the class pk
*/
public void removeByCN_CPK(long classNameId, long classPK);
/**
* Returns the number of segments entry rels where classNameId = ? and classPK = ?.
*
* @param classNameId the class name ID
* @param classPK the class pk
* @return the number of matching segments entry rels
*/
public int countByCN_CPK(long classNameId, long classPK);
/**
* Returns all the segments entry rels where groupId = ? and classNameId = ? and classPK = ?.
*
* @param groupId the group ID
* @param classNameId the class name ID
* @param classPK the class pk
* @return the matching segments entry rels
*/
public java.util.List findByG_CN_CPK(
long groupId, long classNameId, long classPK);
/**
* Returns a range of all the segments entry rels where groupId = ? and classNameId = ? and classPK = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryRelModelImpl
.
*
*
* @param groupId the group ID
* @param classNameId the class name ID
* @param classPK the class pk
* @param start the lower bound of the range of segments entry rels
* @param end the upper bound of the range of segments entry rels (not inclusive)
* @return the range of matching segments entry rels
*/
public java.util.List findByG_CN_CPK(
long groupId, long classNameId, long classPK, int start, int end);
/**
* Returns an ordered range of all the segments entry rels where groupId = ? and classNameId = ? and classPK = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryRelModelImpl
.
*
*
* @param groupId the group ID
* @param classNameId the class name ID
* @param classPK the class pk
* @param start the lower bound of the range of segments entry rels
* @param end the upper bound of the range of segments entry rels (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching segments entry rels
*/
public java.util.List findByG_CN_CPK(
long groupId, long classNameId, long classPK, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns an ordered range of all the segments entry rels where groupId = ? and classNameId = ? and classPK = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryRelModelImpl
.
*
*
* @param groupId the group ID
* @param classNameId the class name ID
* @param classPK the class pk
* @param start the lower bound of the range of segments entry rels
* @param end the upper bound of the range of segments entry rels (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching segments entry rels
*/
public java.util.List findByG_CN_CPK(
long groupId, long classNameId, long classPK, int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator,
boolean useFinderCache);
/**
* Returns the first segments entry rel in the ordered set where groupId = ? and classNameId = ? and classPK = ?.
*
* @param groupId the group ID
* @param classNameId the class name ID
* @param classPK the class pk
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching segments entry rel
* @throws NoSuchEntryRelException if a matching segments entry rel could not be found
*/
public SegmentsEntryRel findByG_CN_CPK_First(
long groupId, long classNameId, long classPK,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryRelException;
/**
* Returns the first segments entry rel in the ordered set where groupId = ? and classNameId = ? and classPK = ?.
*
* @param groupId the group ID
* @param classNameId the class name ID
* @param classPK the class pk
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching segments entry rel, or null
if a matching segments entry rel could not be found
*/
public SegmentsEntryRel fetchByG_CN_CPK_First(
long groupId, long classNameId, long classPK,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the last segments entry rel in the ordered set where groupId = ? and classNameId = ? and classPK = ?.
*
* @param groupId the group ID
* @param classNameId the class name ID
* @param classPK the class pk
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching segments entry rel
* @throws NoSuchEntryRelException if a matching segments entry rel could not be found
*/
public SegmentsEntryRel findByG_CN_CPK_Last(
long groupId, long classNameId, long classPK,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryRelException;
/**
* Returns the last segments entry rel in the ordered set where groupId = ? and classNameId = ? and classPK = ?.
*
* @param groupId the group ID
* @param classNameId the class name ID
* @param classPK the class pk
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching segments entry rel, or null
if a matching segments entry rel could not be found
*/
public SegmentsEntryRel fetchByG_CN_CPK_Last(
long groupId, long classNameId, long classPK,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns the segments entry rels before and after the current segments entry rel in the ordered set where groupId = ? and classNameId = ? and classPK = ?.
*
* @param segmentsEntryRelId the primary key of the current segments entry rel
* @param groupId the group ID
* @param classNameId the class name ID
* @param classPK the class pk
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next segments entry rel
* @throws NoSuchEntryRelException if a segments entry rel with the primary key could not be found
*/
public SegmentsEntryRel[] findByG_CN_CPK_PrevAndNext(
long segmentsEntryRelId, long groupId, long classNameId,
long classPK,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator)
throws NoSuchEntryRelException;
/**
* Removes all the segments entry rels where groupId = ? and classNameId = ? and classPK = ? from the database.
*
* @param groupId the group ID
* @param classNameId the class name ID
* @param classPK the class pk
*/
public void removeByG_CN_CPK(long groupId, long classNameId, long classPK);
/**
* Returns the number of segments entry rels where groupId = ? and classNameId = ? and classPK = ?.
*
* @param groupId the group ID
* @param classNameId the class name ID
* @param classPK the class pk
* @return the number of matching segments entry rels
*/
public int countByG_CN_CPK(long groupId, long classNameId, long classPK);
/**
* Returns the segments entry rel where segmentsEntryId = ? and classNameId = ? and classPK = ? or throws a NoSuchEntryRelException
if it could not be found.
*
* @param segmentsEntryId the segments entry ID
* @param classNameId the class name ID
* @param classPK the class pk
* @return the matching segments entry rel
* @throws NoSuchEntryRelException if a matching segments entry rel could not be found
*/
public SegmentsEntryRel findByS_CN_CPK(
long segmentsEntryId, long classNameId, long classPK)
throws NoSuchEntryRelException;
/**
* Returns the segments entry rel where segmentsEntryId = ? and classNameId = ? and classPK = ? or returns null
if it could not be found. Uses the finder cache.
*
* @param segmentsEntryId the segments entry ID
* @param classNameId the class name ID
* @param classPK the class pk
* @return the matching segments entry rel, or null
if a matching segments entry rel could not be found
*/
public SegmentsEntryRel fetchByS_CN_CPK(
long segmentsEntryId, long classNameId, long classPK);
/**
* Returns the segments entry rel where segmentsEntryId = ? and classNameId = ? and classPK = ? or returns null
if it could not be found, optionally using the finder cache.
*
* @param segmentsEntryId the segments entry ID
* @param classNameId the class name ID
* @param classPK the class pk
* @param useFinderCache whether to use the finder cache
* @return the matching segments entry rel, or null
if a matching segments entry rel could not be found
*/
public SegmentsEntryRel fetchByS_CN_CPK(
long segmentsEntryId, long classNameId, long classPK,
boolean useFinderCache);
/**
* Removes the segments entry rel where segmentsEntryId = ? and classNameId = ? and classPK = ? from the database.
*
* @param segmentsEntryId the segments entry ID
* @param classNameId the class name ID
* @param classPK the class pk
* @return the segments entry rel that was removed
*/
public SegmentsEntryRel removeByS_CN_CPK(
long segmentsEntryId, long classNameId, long classPK)
throws NoSuchEntryRelException;
/**
* Returns the number of segments entry rels where segmentsEntryId = ? and classNameId = ? and classPK = ?.
*
* @param segmentsEntryId the segments entry ID
* @param classNameId the class name ID
* @param classPK the class pk
* @return the number of matching segments entry rels
*/
public int countByS_CN_CPK(
long segmentsEntryId, long classNameId, long classPK);
/**
* Caches the segments entry rel in the entity cache if it is enabled.
*
* @param segmentsEntryRel the segments entry rel
*/
public void cacheResult(SegmentsEntryRel segmentsEntryRel);
/**
* Caches the segments entry rels in the entity cache if it is enabled.
*
* @param segmentsEntryRels the segments entry rels
*/
public void cacheResult(java.util.List segmentsEntryRels);
/**
* Creates a new segments entry rel with the primary key. Does not add the segments entry rel to the database.
*
* @param segmentsEntryRelId the primary key for the new segments entry rel
* @return the new segments entry rel
*/
public SegmentsEntryRel create(long segmentsEntryRelId);
/**
* Removes the segments entry rel with the primary key from the database. Also notifies the appropriate model listeners.
*
* @param segmentsEntryRelId the primary key of the segments entry rel
* @return the segments entry rel that was removed
* @throws NoSuchEntryRelException if a segments entry rel with the primary key could not be found
*/
public SegmentsEntryRel remove(long segmentsEntryRelId)
throws NoSuchEntryRelException;
public SegmentsEntryRel updateImpl(SegmentsEntryRel segmentsEntryRel);
/**
* Returns the segments entry rel with the primary key or throws a NoSuchEntryRelException
if it could not be found.
*
* @param segmentsEntryRelId the primary key of the segments entry rel
* @return the segments entry rel
* @throws NoSuchEntryRelException if a segments entry rel with the primary key could not be found
*/
public SegmentsEntryRel findByPrimaryKey(long segmentsEntryRelId)
throws NoSuchEntryRelException;
/**
* Returns the segments entry rel with the primary key or returns null
if it could not be found.
*
* @param segmentsEntryRelId the primary key of the segments entry rel
* @return the segments entry rel, or null
if a segments entry rel with the primary key could not be found
*/
public SegmentsEntryRel fetchByPrimaryKey(long segmentsEntryRelId);
/**
* Returns all the segments entry rels.
*
* @return the segments entry rels
*/
public java.util.List findAll();
/**
* Returns a range of all the segments entry rels.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryRelModelImpl
.
*
*
* @param start the lower bound of the range of segments entry rels
* @param end the upper bound of the range of segments entry rels (not inclusive)
* @return the range of segments entry rels
*/
public java.util.List findAll(int start, int end);
/**
* Returns an ordered range of all the segments entry rels.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryRelModelImpl
.
*
*
* @param start the lower bound of the range of segments entry rels
* @param end the upper bound of the range of segments entry rels (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of segments entry rels
*/
public java.util.List findAll(
int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator);
/**
* Returns an ordered range of all the segments entry rels.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryRelModelImpl
.
*
*
* @param start the lower bound of the range of segments entry rels
* @param end the upper bound of the range of segments entry rels (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of segments entry rels
*/
public java.util.List findAll(
int start, int end,
com.liferay.portal.kernel.util.OrderByComparator
orderByComparator,
boolean useFinderCache);
/**
* Removes all the segments entry rels from the database.
*/
public void removeAll();
/**
* Returns the number of segments entry rels.
*
* @return the number of segments entry rels
*/
public int countAll();
}