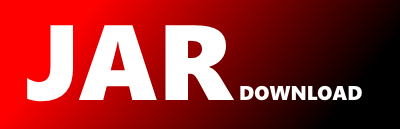
com.liferay.segments.service.persistence.SegmentsEntryRoleUtil Maven / Gradle / Ivy
/**
* SPDX-FileCopyrightText: (c) 2000 Liferay, Inc. https://liferay.com
* SPDX-License-Identifier: LGPL-2.1-or-later OR LicenseRef-Liferay-DXP-EULA-2.0.0-2023-06
*/
package com.liferay.segments.service.persistence;
import com.liferay.portal.kernel.dao.orm.DynamicQuery;
import com.liferay.portal.kernel.service.ServiceContext;
import com.liferay.portal.kernel.util.OrderByComparator;
import com.liferay.segments.model.SegmentsEntryRole;
import java.io.Serializable;
import java.util.List;
import java.util.Map;
import java.util.Set;
/**
* The persistence utility for the segments entry role service. This utility wraps com.liferay.segments.service.persistence.impl.SegmentsEntryRolePersistenceImpl
and provides direct access to the database for CRUD operations. This utility should only be used by the service layer, as it must operate within a transaction. Never access this utility in a JSP, controller, model, or other front-end class.
*
*
* Caching information and settings can be found in portal.properties
*
*
* @author Eduardo Garcia
* @see SegmentsEntryRolePersistence
* @generated
*/
public class SegmentsEntryRoleUtil {
/*
* NOTE FOR DEVELOPERS:
*
* Never modify this class directly. Modify service.xml
and rerun ServiceBuilder to regenerate this class.
*/
/**
* @see com.liferay.portal.kernel.service.persistence.BasePersistence#clearCache()
*/
public static void clearCache() {
getPersistence().clearCache();
}
/**
* @see com.liferay.portal.kernel.service.persistence.BasePersistence#clearCache(com.liferay.portal.kernel.model.BaseModel)
*/
public static void clearCache(SegmentsEntryRole segmentsEntryRole) {
getPersistence().clearCache(segmentsEntryRole);
}
/**
* @see com.liferay.portal.kernel.service.persistence.BasePersistence#countWithDynamicQuery(DynamicQuery)
*/
public static long countWithDynamicQuery(DynamicQuery dynamicQuery) {
return getPersistence().countWithDynamicQuery(dynamicQuery);
}
/**
* @see com.liferay.portal.kernel.service.persistence.BasePersistence#fetchByPrimaryKeys(Set)
*/
public static Map fetchByPrimaryKeys(
Set primaryKeys) {
return getPersistence().fetchByPrimaryKeys(primaryKeys);
}
/**
* @see com.liferay.portal.kernel.service.persistence.BasePersistence#findWithDynamicQuery(DynamicQuery)
*/
public static List findWithDynamicQuery(
DynamicQuery dynamicQuery) {
return getPersistence().findWithDynamicQuery(dynamicQuery);
}
/**
* @see com.liferay.portal.kernel.service.persistence.BasePersistence#findWithDynamicQuery(DynamicQuery, int, int)
*/
public static List findWithDynamicQuery(
DynamicQuery dynamicQuery, int start, int end) {
return getPersistence().findWithDynamicQuery(dynamicQuery, start, end);
}
/**
* @see com.liferay.portal.kernel.service.persistence.BasePersistence#findWithDynamicQuery(DynamicQuery, int, int, OrderByComparator)
*/
public static List findWithDynamicQuery(
DynamicQuery dynamicQuery, int start, int end,
OrderByComparator orderByComparator) {
return getPersistence().findWithDynamicQuery(
dynamicQuery, start, end, orderByComparator);
}
/**
* @see com.liferay.portal.kernel.service.persistence.BasePersistence#update(com.liferay.portal.kernel.model.BaseModel)
*/
public static SegmentsEntryRole update(
SegmentsEntryRole segmentsEntryRole) {
return getPersistence().update(segmentsEntryRole);
}
/**
* @see com.liferay.portal.kernel.service.persistence.BasePersistence#update(com.liferay.portal.kernel.model.BaseModel, ServiceContext)
*/
public static SegmentsEntryRole update(
SegmentsEntryRole segmentsEntryRole, ServiceContext serviceContext) {
return getPersistence().update(segmentsEntryRole, serviceContext);
}
/**
* Returns all the segments entry roles where segmentsEntryId = ?.
*
* @param segmentsEntryId the segments entry ID
* @return the matching segments entry roles
*/
public static List findBySegmentsEntryId(
long segmentsEntryId) {
return getPersistence().findBySegmentsEntryId(segmentsEntryId);
}
/**
* Returns a range of all the segments entry roles where segmentsEntryId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryRoleModelImpl
.
*
*
* @param segmentsEntryId the segments entry ID
* @param start the lower bound of the range of segments entry roles
* @param end the upper bound of the range of segments entry roles (not inclusive)
* @return the range of matching segments entry roles
*/
public static List findBySegmentsEntryId(
long segmentsEntryId, int start, int end) {
return getPersistence().findBySegmentsEntryId(
segmentsEntryId, start, end);
}
/**
* Returns an ordered range of all the segments entry roles where segmentsEntryId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryRoleModelImpl
.
*
*
* @param segmentsEntryId the segments entry ID
* @param start the lower bound of the range of segments entry roles
* @param end the upper bound of the range of segments entry roles (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching segments entry roles
*/
public static List findBySegmentsEntryId(
long segmentsEntryId, int start, int end,
OrderByComparator orderByComparator) {
return getPersistence().findBySegmentsEntryId(
segmentsEntryId, start, end, orderByComparator);
}
/**
* Returns an ordered range of all the segments entry roles where segmentsEntryId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryRoleModelImpl
.
*
*
* @param segmentsEntryId the segments entry ID
* @param start the lower bound of the range of segments entry roles
* @param end the upper bound of the range of segments entry roles (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching segments entry roles
*/
public static List findBySegmentsEntryId(
long segmentsEntryId, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
return getPersistence().findBySegmentsEntryId(
segmentsEntryId, start, end, orderByComparator, useFinderCache);
}
/**
* Returns the first segments entry role in the ordered set where segmentsEntryId = ?.
*
* @param segmentsEntryId the segments entry ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching segments entry role
* @throws NoSuchEntryRoleException if a matching segments entry role could not be found
*/
public static SegmentsEntryRole findBySegmentsEntryId_First(
long segmentsEntryId,
OrderByComparator orderByComparator)
throws com.liferay.segments.exception.NoSuchEntryRoleException {
return getPersistence().findBySegmentsEntryId_First(
segmentsEntryId, orderByComparator);
}
/**
* Returns the first segments entry role in the ordered set where segmentsEntryId = ?.
*
* @param segmentsEntryId the segments entry ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching segments entry role, or null
if a matching segments entry role could not be found
*/
public static SegmentsEntryRole fetchBySegmentsEntryId_First(
long segmentsEntryId,
OrderByComparator orderByComparator) {
return getPersistence().fetchBySegmentsEntryId_First(
segmentsEntryId, orderByComparator);
}
/**
* Returns the last segments entry role in the ordered set where segmentsEntryId = ?.
*
* @param segmentsEntryId the segments entry ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching segments entry role
* @throws NoSuchEntryRoleException if a matching segments entry role could not be found
*/
public static SegmentsEntryRole findBySegmentsEntryId_Last(
long segmentsEntryId,
OrderByComparator orderByComparator)
throws com.liferay.segments.exception.NoSuchEntryRoleException {
return getPersistence().findBySegmentsEntryId_Last(
segmentsEntryId, orderByComparator);
}
/**
* Returns the last segments entry role in the ordered set where segmentsEntryId = ?.
*
* @param segmentsEntryId the segments entry ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching segments entry role, or null
if a matching segments entry role could not be found
*/
public static SegmentsEntryRole fetchBySegmentsEntryId_Last(
long segmentsEntryId,
OrderByComparator orderByComparator) {
return getPersistence().fetchBySegmentsEntryId_Last(
segmentsEntryId, orderByComparator);
}
/**
* Returns the segments entry roles before and after the current segments entry role in the ordered set where segmentsEntryId = ?.
*
* @param segmentsEntryRoleId the primary key of the current segments entry role
* @param segmentsEntryId the segments entry ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next segments entry role
* @throws NoSuchEntryRoleException if a segments entry role with the primary key could not be found
*/
public static SegmentsEntryRole[] findBySegmentsEntryId_PrevAndNext(
long segmentsEntryRoleId, long segmentsEntryId,
OrderByComparator orderByComparator)
throws com.liferay.segments.exception.NoSuchEntryRoleException {
return getPersistence().findBySegmentsEntryId_PrevAndNext(
segmentsEntryRoleId, segmentsEntryId, orderByComparator);
}
/**
* Removes all the segments entry roles where segmentsEntryId = ? from the database.
*
* @param segmentsEntryId the segments entry ID
*/
public static void removeBySegmentsEntryId(long segmentsEntryId) {
getPersistence().removeBySegmentsEntryId(segmentsEntryId);
}
/**
* Returns the number of segments entry roles where segmentsEntryId = ?.
*
* @param segmentsEntryId the segments entry ID
* @return the number of matching segments entry roles
*/
public static int countBySegmentsEntryId(long segmentsEntryId) {
return getPersistence().countBySegmentsEntryId(segmentsEntryId);
}
/**
* Returns all the segments entry roles where roleId = ?.
*
* @param roleId the role ID
* @return the matching segments entry roles
*/
public static List findByRoleId(long roleId) {
return getPersistence().findByRoleId(roleId);
}
/**
* Returns a range of all the segments entry roles where roleId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryRoleModelImpl
.
*
*
* @param roleId the role ID
* @param start the lower bound of the range of segments entry roles
* @param end the upper bound of the range of segments entry roles (not inclusive)
* @return the range of matching segments entry roles
*/
public static List findByRoleId(
long roleId, int start, int end) {
return getPersistence().findByRoleId(roleId, start, end);
}
/**
* Returns an ordered range of all the segments entry roles where roleId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryRoleModelImpl
.
*
*
* @param roleId the role ID
* @param start the lower bound of the range of segments entry roles
* @param end the upper bound of the range of segments entry roles (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching segments entry roles
*/
public static List findByRoleId(
long roleId, int start, int end,
OrderByComparator orderByComparator) {
return getPersistence().findByRoleId(
roleId, start, end, orderByComparator);
}
/**
* Returns an ordered range of all the segments entry roles where roleId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryRoleModelImpl
.
*
*
* @param roleId the role ID
* @param start the lower bound of the range of segments entry roles
* @param end the upper bound of the range of segments entry roles (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching segments entry roles
*/
public static List findByRoleId(
long roleId, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
return getPersistence().findByRoleId(
roleId, start, end, orderByComparator, useFinderCache);
}
/**
* Returns the first segments entry role in the ordered set where roleId = ?.
*
* @param roleId the role ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching segments entry role
* @throws NoSuchEntryRoleException if a matching segments entry role could not be found
*/
public static SegmentsEntryRole findByRoleId_First(
long roleId, OrderByComparator orderByComparator)
throws com.liferay.segments.exception.NoSuchEntryRoleException {
return getPersistence().findByRoleId_First(roleId, orderByComparator);
}
/**
* Returns the first segments entry role in the ordered set where roleId = ?.
*
* @param roleId the role ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching segments entry role, or null
if a matching segments entry role could not be found
*/
public static SegmentsEntryRole fetchByRoleId_First(
long roleId, OrderByComparator orderByComparator) {
return getPersistence().fetchByRoleId_First(roleId, orderByComparator);
}
/**
* Returns the last segments entry role in the ordered set where roleId = ?.
*
* @param roleId the role ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching segments entry role
* @throws NoSuchEntryRoleException if a matching segments entry role could not be found
*/
public static SegmentsEntryRole findByRoleId_Last(
long roleId, OrderByComparator orderByComparator)
throws com.liferay.segments.exception.NoSuchEntryRoleException {
return getPersistence().findByRoleId_Last(roleId, orderByComparator);
}
/**
* Returns the last segments entry role in the ordered set where roleId = ?.
*
* @param roleId the role ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching segments entry role, or null
if a matching segments entry role could not be found
*/
public static SegmentsEntryRole fetchByRoleId_Last(
long roleId, OrderByComparator orderByComparator) {
return getPersistence().fetchByRoleId_Last(roleId, orderByComparator);
}
/**
* Returns the segments entry roles before and after the current segments entry role in the ordered set where roleId = ?.
*
* @param segmentsEntryRoleId the primary key of the current segments entry role
* @param roleId the role ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next segments entry role
* @throws NoSuchEntryRoleException if a segments entry role with the primary key could not be found
*/
public static SegmentsEntryRole[] findByRoleId_PrevAndNext(
long segmentsEntryRoleId, long roleId,
OrderByComparator orderByComparator)
throws com.liferay.segments.exception.NoSuchEntryRoleException {
return getPersistence().findByRoleId_PrevAndNext(
segmentsEntryRoleId, roleId, orderByComparator);
}
/**
* Removes all the segments entry roles where roleId = ? from the database.
*
* @param roleId the role ID
*/
public static void removeByRoleId(long roleId) {
getPersistence().removeByRoleId(roleId);
}
/**
* Returns the number of segments entry roles where roleId = ?.
*
* @param roleId the role ID
* @return the number of matching segments entry roles
*/
public static int countByRoleId(long roleId) {
return getPersistence().countByRoleId(roleId);
}
/**
* Returns the segments entry role where segmentsEntryId = ? and roleId = ? or throws a NoSuchEntryRoleException
if it could not be found.
*
* @param segmentsEntryId the segments entry ID
* @param roleId the role ID
* @return the matching segments entry role
* @throws NoSuchEntryRoleException if a matching segments entry role could not be found
*/
public static SegmentsEntryRole findByS_R(long segmentsEntryId, long roleId)
throws com.liferay.segments.exception.NoSuchEntryRoleException {
return getPersistence().findByS_R(segmentsEntryId, roleId);
}
/**
* Returns the segments entry role where segmentsEntryId = ? and roleId = ? or returns null
if it could not be found. Uses the finder cache.
*
* @param segmentsEntryId the segments entry ID
* @param roleId the role ID
* @return the matching segments entry role, or null
if a matching segments entry role could not be found
*/
public static SegmentsEntryRole fetchByS_R(
long segmentsEntryId, long roleId) {
return getPersistence().fetchByS_R(segmentsEntryId, roleId);
}
/**
* Returns the segments entry role where segmentsEntryId = ? and roleId = ? or returns null
if it could not be found, optionally using the finder cache.
*
* @param segmentsEntryId the segments entry ID
* @param roleId the role ID
* @param useFinderCache whether to use the finder cache
* @return the matching segments entry role, or null
if a matching segments entry role could not be found
*/
public static SegmentsEntryRole fetchByS_R(
long segmentsEntryId, long roleId, boolean useFinderCache) {
return getPersistence().fetchByS_R(
segmentsEntryId, roleId, useFinderCache);
}
/**
* Removes the segments entry role where segmentsEntryId = ? and roleId = ? from the database.
*
* @param segmentsEntryId the segments entry ID
* @param roleId the role ID
* @return the segments entry role that was removed
*/
public static SegmentsEntryRole removeByS_R(
long segmentsEntryId, long roleId)
throws com.liferay.segments.exception.NoSuchEntryRoleException {
return getPersistence().removeByS_R(segmentsEntryId, roleId);
}
/**
* Returns the number of segments entry roles where segmentsEntryId = ? and roleId = ?.
*
* @param segmentsEntryId the segments entry ID
* @param roleId the role ID
* @return the number of matching segments entry roles
*/
public static int countByS_R(long segmentsEntryId, long roleId) {
return getPersistence().countByS_R(segmentsEntryId, roleId);
}
/**
* Caches the segments entry role in the entity cache if it is enabled.
*
* @param segmentsEntryRole the segments entry role
*/
public static void cacheResult(SegmentsEntryRole segmentsEntryRole) {
getPersistence().cacheResult(segmentsEntryRole);
}
/**
* Caches the segments entry roles in the entity cache if it is enabled.
*
* @param segmentsEntryRoles the segments entry roles
*/
public static void cacheResult(List segmentsEntryRoles) {
getPersistence().cacheResult(segmentsEntryRoles);
}
/**
* Creates a new segments entry role with the primary key. Does not add the segments entry role to the database.
*
* @param segmentsEntryRoleId the primary key for the new segments entry role
* @return the new segments entry role
*/
public static SegmentsEntryRole create(long segmentsEntryRoleId) {
return getPersistence().create(segmentsEntryRoleId);
}
/**
* Removes the segments entry role with the primary key from the database. Also notifies the appropriate model listeners.
*
* @param segmentsEntryRoleId the primary key of the segments entry role
* @return the segments entry role that was removed
* @throws NoSuchEntryRoleException if a segments entry role with the primary key could not be found
*/
public static SegmentsEntryRole remove(long segmentsEntryRoleId)
throws com.liferay.segments.exception.NoSuchEntryRoleException {
return getPersistence().remove(segmentsEntryRoleId);
}
public static SegmentsEntryRole updateImpl(
SegmentsEntryRole segmentsEntryRole) {
return getPersistence().updateImpl(segmentsEntryRole);
}
/**
* Returns the segments entry role with the primary key or throws a NoSuchEntryRoleException
if it could not be found.
*
* @param segmentsEntryRoleId the primary key of the segments entry role
* @return the segments entry role
* @throws NoSuchEntryRoleException if a segments entry role with the primary key could not be found
*/
public static SegmentsEntryRole findByPrimaryKey(long segmentsEntryRoleId)
throws com.liferay.segments.exception.NoSuchEntryRoleException {
return getPersistence().findByPrimaryKey(segmentsEntryRoleId);
}
/**
* Returns the segments entry role with the primary key or returns null
if it could not be found.
*
* @param segmentsEntryRoleId the primary key of the segments entry role
* @return the segments entry role, or null
if a segments entry role with the primary key could not be found
*/
public static SegmentsEntryRole fetchByPrimaryKey(
long segmentsEntryRoleId) {
return getPersistence().fetchByPrimaryKey(segmentsEntryRoleId);
}
/**
* Returns all the segments entry roles.
*
* @return the segments entry roles
*/
public static List findAll() {
return getPersistence().findAll();
}
/**
* Returns a range of all the segments entry roles.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryRoleModelImpl
.
*
*
* @param start the lower bound of the range of segments entry roles
* @param end the upper bound of the range of segments entry roles (not inclusive)
* @return the range of segments entry roles
*/
public static List findAll(int start, int end) {
return getPersistence().findAll(start, end);
}
/**
* Returns an ordered range of all the segments entry roles.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryRoleModelImpl
.
*
*
* @param start the lower bound of the range of segments entry roles
* @param end the upper bound of the range of segments entry roles (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of segments entry roles
*/
public static List findAll(
int start, int end,
OrderByComparator orderByComparator) {
return getPersistence().findAll(start, end, orderByComparator);
}
/**
* Returns an ordered range of all the segments entry roles.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsEntryRoleModelImpl
.
*
*
* @param start the lower bound of the range of segments entry roles
* @param end the upper bound of the range of segments entry roles (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of segments entry roles
*/
public static List findAll(
int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
return getPersistence().findAll(
start, end, orderByComparator, useFinderCache);
}
/**
* Removes all the segments entry roles from the database.
*/
public static void removeAll() {
getPersistence().removeAll();
}
/**
* Returns the number of segments entry roles.
*
* @return the number of segments entry roles
*/
public static int countAll() {
return getPersistence().countAll();
}
public static SegmentsEntryRolePersistence getPersistence() {
return _persistence;
}
public static void setPersistence(
SegmentsEntryRolePersistence persistence) {
_persistence = persistence;
}
private static volatile SegmentsEntryRolePersistence _persistence;
}