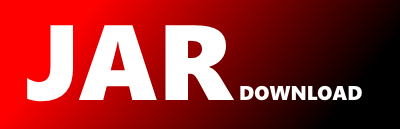
com.liferay.segments.service.persistence.SegmentsExperimentUtil Maven / Gradle / Ivy
/**
* SPDX-FileCopyrightText: (c) 2000 Liferay, Inc. https://liferay.com
* SPDX-License-Identifier: LGPL-2.1-or-later OR LicenseRef-Liferay-DXP-EULA-2.0.0-2023-06
*/
package com.liferay.segments.service.persistence;
import com.liferay.portal.kernel.dao.orm.DynamicQuery;
import com.liferay.portal.kernel.service.ServiceContext;
import com.liferay.portal.kernel.util.OrderByComparator;
import com.liferay.segments.model.SegmentsExperiment;
import java.io.Serializable;
import java.util.List;
import java.util.Map;
import java.util.Set;
/**
* The persistence utility for the segments experiment service. This utility wraps com.liferay.segments.service.persistence.impl.SegmentsExperimentPersistenceImpl
and provides direct access to the database for CRUD operations. This utility should only be used by the service layer, as it must operate within a transaction. Never access this utility in a JSP, controller, model, or other front-end class.
*
*
* Caching information and settings can be found in portal.properties
*
*
* @author Eduardo Garcia
* @see SegmentsExperimentPersistence
* @generated
*/
public class SegmentsExperimentUtil {
/*
* NOTE FOR DEVELOPERS:
*
* Never modify this class directly. Modify service.xml
and rerun ServiceBuilder to regenerate this class.
*/
/**
* @see com.liferay.portal.kernel.service.persistence.BasePersistence#clearCache()
*/
public static void clearCache() {
getPersistence().clearCache();
}
/**
* @see com.liferay.portal.kernel.service.persistence.BasePersistence#clearCache(com.liferay.portal.kernel.model.BaseModel)
*/
public static void clearCache(SegmentsExperiment segmentsExperiment) {
getPersistence().clearCache(segmentsExperiment);
}
/**
* @see com.liferay.portal.kernel.service.persistence.BasePersistence#countWithDynamicQuery(DynamicQuery)
*/
public static long countWithDynamicQuery(DynamicQuery dynamicQuery) {
return getPersistence().countWithDynamicQuery(dynamicQuery);
}
/**
* @see com.liferay.portal.kernel.service.persistence.BasePersistence#fetchByPrimaryKeys(Set)
*/
public static Map fetchByPrimaryKeys(
Set primaryKeys) {
return getPersistence().fetchByPrimaryKeys(primaryKeys);
}
/**
* @see com.liferay.portal.kernel.service.persistence.BasePersistence#findWithDynamicQuery(DynamicQuery)
*/
public static List findWithDynamicQuery(
DynamicQuery dynamicQuery) {
return getPersistence().findWithDynamicQuery(dynamicQuery);
}
/**
* @see com.liferay.portal.kernel.service.persistence.BasePersistence#findWithDynamicQuery(DynamicQuery, int, int)
*/
public static List findWithDynamicQuery(
DynamicQuery dynamicQuery, int start, int end) {
return getPersistence().findWithDynamicQuery(dynamicQuery, start, end);
}
/**
* @see com.liferay.portal.kernel.service.persistence.BasePersistence#findWithDynamicQuery(DynamicQuery, int, int, OrderByComparator)
*/
public static List findWithDynamicQuery(
DynamicQuery dynamicQuery, int start, int end,
OrderByComparator orderByComparator) {
return getPersistence().findWithDynamicQuery(
dynamicQuery, start, end, orderByComparator);
}
/**
* @see com.liferay.portal.kernel.service.persistence.BasePersistence#update(com.liferay.portal.kernel.model.BaseModel)
*/
public static SegmentsExperiment update(
SegmentsExperiment segmentsExperiment) {
return getPersistence().update(segmentsExperiment);
}
/**
* @see com.liferay.portal.kernel.service.persistence.BasePersistence#update(com.liferay.portal.kernel.model.BaseModel, ServiceContext)
*/
public static SegmentsExperiment update(
SegmentsExperiment segmentsExperiment, ServiceContext serviceContext) {
return getPersistence().update(segmentsExperiment, serviceContext);
}
/**
* Returns all the segments experiments where uuid = ?.
*
* @param uuid the uuid
* @return the matching segments experiments
*/
public static List findByUuid(String uuid) {
return getPersistence().findByUuid(uuid);
}
/**
* Returns a range of all the segments experiments where uuid = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsExperimentModelImpl
.
*
*
* @param uuid the uuid
* @param start the lower bound of the range of segments experiments
* @param end the upper bound of the range of segments experiments (not inclusive)
* @return the range of matching segments experiments
*/
public static List findByUuid(
String uuid, int start, int end) {
return getPersistence().findByUuid(uuid, start, end);
}
/**
* Returns an ordered range of all the segments experiments where uuid = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsExperimentModelImpl
.
*
*
* @param uuid the uuid
* @param start the lower bound of the range of segments experiments
* @param end the upper bound of the range of segments experiments (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching segments experiments
*/
public static List findByUuid(
String uuid, int start, int end,
OrderByComparator orderByComparator) {
return getPersistence().findByUuid(uuid, start, end, orderByComparator);
}
/**
* Returns an ordered range of all the segments experiments where uuid = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsExperimentModelImpl
.
*
*
* @param uuid the uuid
* @param start the lower bound of the range of segments experiments
* @param end the upper bound of the range of segments experiments (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching segments experiments
*/
public static List findByUuid(
String uuid, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
return getPersistence().findByUuid(
uuid, start, end, orderByComparator, useFinderCache);
}
/**
* Returns the first segments experiment in the ordered set where uuid = ?.
*
* @param uuid the uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching segments experiment
* @throws NoSuchExperimentException if a matching segments experiment could not be found
*/
public static SegmentsExperiment findByUuid_First(
String uuid,
OrderByComparator orderByComparator)
throws com.liferay.segments.exception.NoSuchExperimentException {
return getPersistence().findByUuid_First(uuid, orderByComparator);
}
/**
* Returns the first segments experiment in the ordered set where uuid = ?.
*
* @param uuid the uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching segments experiment, or null
if a matching segments experiment could not be found
*/
public static SegmentsExperiment fetchByUuid_First(
String uuid, OrderByComparator orderByComparator) {
return getPersistence().fetchByUuid_First(uuid, orderByComparator);
}
/**
* Returns the last segments experiment in the ordered set where uuid = ?.
*
* @param uuid the uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching segments experiment
* @throws NoSuchExperimentException if a matching segments experiment could not be found
*/
public static SegmentsExperiment findByUuid_Last(
String uuid,
OrderByComparator orderByComparator)
throws com.liferay.segments.exception.NoSuchExperimentException {
return getPersistence().findByUuid_Last(uuid, orderByComparator);
}
/**
* Returns the last segments experiment in the ordered set where uuid = ?.
*
* @param uuid the uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching segments experiment, or null
if a matching segments experiment could not be found
*/
public static SegmentsExperiment fetchByUuid_Last(
String uuid, OrderByComparator orderByComparator) {
return getPersistence().fetchByUuid_Last(uuid, orderByComparator);
}
/**
* Returns the segments experiments before and after the current segments experiment in the ordered set where uuid = ?.
*
* @param segmentsExperimentId the primary key of the current segments experiment
* @param uuid the uuid
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next segments experiment
* @throws NoSuchExperimentException if a segments experiment with the primary key could not be found
*/
public static SegmentsExperiment[] findByUuid_PrevAndNext(
long segmentsExperimentId, String uuid,
OrderByComparator orderByComparator)
throws com.liferay.segments.exception.NoSuchExperimentException {
return getPersistence().findByUuid_PrevAndNext(
segmentsExperimentId, uuid, orderByComparator);
}
/**
* Removes all the segments experiments where uuid = ? from the database.
*
* @param uuid the uuid
*/
public static void removeByUuid(String uuid) {
getPersistence().removeByUuid(uuid);
}
/**
* Returns the number of segments experiments where uuid = ?.
*
* @param uuid the uuid
* @return the number of matching segments experiments
*/
public static int countByUuid(String uuid) {
return getPersistence().countByUuid(uuid);
}
/**
* Returns the segments experiment where uuid = ? and groupId = ? or throws a NoSuchExperimentException
if it could not be found.
*
* @param uuid the uuid
* @param groupId the group ID
* @return the matching segments experiment
* @throws NoSuchExperimentException if a matching segments experiment could not be found
*/
public static SegmentsExperiment findByUUID_G(String uuid, long groupId)
throws com.liferay.segments.exception.NoSuchExperimentException {
return getPersistence().findByUUID_G(uuid, groupId);
}
/**
* Returns the segments experiment where uuid = ? and groupId = ? or returns null
if it could not be found. Uses the finder cache.
*
* @param uuid the uuid
* @param groupId the group ID
* @return the matching segments experiment, or null
if a matching segments experiment could not be found
*/
public static SegmentsExperiment fetchByUUID_G(String uuid, long groupId) {
return getPersistence().fetchByUUID_G(uuid, groupId);
}
/**
* Returns the segments experiment where uuid = ? and groupId = ? or returns null
if it could not be found, optionally using the finder cache.
*
* @param uuid the uuid
* @param groupId the group ID
* @param useFinderCache whether to use the finder cache
* @return the matching segments experiment, or null
if a matching segments experiment could not be found
*/
public static SegmentsExperiment fetchByUUID_G(
String uuid, long groupId, boolean useFinderCache) {
return getPersistence().fetchByUUID_G(uuid, groupId, useFinderCache);
}
/**
* Removes the segments experiment where uuid = ? and groupId = ? from the database.
*
* @param uuid the uuid
* @param groupId the group ID
* @return the segments experiment that was removed
*/
public static SegmentsExperiment removeByUUID_G(String uuid, long groupId)
throws com.liferay.segments.exception.NoSuchExperimentException {
return getPersistence().removeByUUID_G(uuid, groupId);
}
/**
* Returns the number of segments experiments where uuid = ? and groupId = ?.
*
* @param uuid the uuid
* @param groupId the group ID
* @return the number of matching segments experiments
*/
public static int countByUUID_G(String uuid, long groupId) {
return getPersistence().countByUUID_G(uuid, groupId);
}
/**
* Returns all the segments experiments where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @return the matching segments experiments
*/
public static List findByUuid_C(
String uuid, long companyId) {
return getPersistence().findByUuid_C(uuid, companyId);
}
/**
* Returns a range of all the segments experiments where uuid = ? and companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsExperimentModelImpl
.
*
*
* @param uuid the uuid
* @param companyId the company ID
* @param start the lower bound of the range of segments experiments
* @param end the upper bound of the range of segments experiments (not inclusive)
* @return the range of matching segments experiments
*/
public static List findByUuid_C(
String uuid, long companyId, int start, int end) {
return getPersistence().findByUuid_C(uuid, companyId, start, end);
}
/**
* Returns an ordered range of all the segments experiments where uuid = ? and companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsExperimentModelImpl
.
*
*
* @param uuid the uuid
* @param companyId the company ID
* @param start the lower bound of the range of segments experiments
* @param end the upper bound of the range of segments experiments (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching segments experiments
*/
public static List findByUuid_C(
String uuid, long companyId, int start, int end,
OrderByComparator orderByComparator) {
return getPersistence().findByUuid_C(
uuid, companyId, start, end, orderByComparator);
}
/**
* Returns an ordered range of all the segments experiments where uuid = ? and companyId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsExperimentModelImpl
.
*
*
* @param uuid the uuid
* @param companyId the company ID
* @param start the lower bound of the range of segments experiments
* @param end the upper bound of the range of segments experiments (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching segments experiments
*/
public static List findByUuid_C(
String uuid, long companyId, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
return getPersistence().findByUuid_C(
uuid, companyId, start, end, orderByComparator, useFinderCache);
}
/**
* Returns the first segments experiment in the ordered set where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching segments experiment
* @throws NoSuchExperimentException if a matching segments experiment could not be found
*/
public static SegmentsExperiment findByUuid_C_First(
String uuid, long companyId,
OrderByComparator orderByComparator)
throws com.liferay.segments.exception.NoSuchExperimentException {
return getPersistence().findByUuid_C_First(
uuid, companyId, orderByComparator);
}
/**
* Returns the first segments experiment in the ordered set where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching segments experiment, or null
if a matching segments experiment could not be found
*/
public static SegmentsExperiment fetchByUuid_C_First(
String uuid, long companyId,
OrderByComparator orderByComparator) {
return getPersistence().fetchByUuid_C_First(
uuid, companyId, orderByComparator);
}
/**
* Returns the last segments experiment in the ordered set where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching segments experiment
* @throws NoSuchExperimentException if a matching segments experiment could not be found
*/
public static SegmentsExperiment findByUuid_C_Last(
String uuid, long companyId,
OrderByComparator orderByComparator)
throws com.liferay.segments.exception.NoSuchExperimentException {
return getPersistence().findByUuid_C_Last(
uuid, companyId, orderByComparator);
}
/**
* Returns the last segments experiment in the ordered set where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching segments experiment, or null
if a matching segments experiment could not be found
*/
public static SegmentsExperiment fetchByUuid_C_Last(
String uuid, long companyId,
OrderByComparator orderByComparator) {
return getPersistence().fetchByUuid_C_Last(
uuid, companyId, orderByComparator);
}
/**
* Returns the segments experiments before and after the current segments experiment in the ordered set where uuid = ? and companyId = ?.
*
* @param segmentsExperimentId the primary key of the current segments experiment
* @param uuid the uuid
* @param companyId the company ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next segments experiment
* @throws NoSuchExperimentException if a segments experiment with the primary key could not be found
*/
public static SegmentsExperiment[] findByUuid_C_PrevAndNext(
long segmentsExperimentId, String uuid, long companyId,
OrderByComparator orderByComparator)
throws com.liferay.segments.exception.NoSuchExperimentException {
return getPersistence().findByUuid_C_PrevAndNext(
segmentsExperimentId, uuid, companyId, orderByComparator);
}
/**
* Removes all the segments experiments where uuid = ? and companyId = ? from the database.
*
* @param uuid the uuid
* @param companyId the company ID
*/
public static void removeByUuid_C(String uuid, long companyId) {
getPersistence().removeByUuid_C(uuid, companyId);
}
/**
* Returns the number of segments experiments where uuid = ? and companyId = ?.
*
* @param uuid the uuid
* @param companyId the company ID
* @return the number of matching segments experiments
*/
public static int countByUuid_C(String uuid, long companyId) {
return getPersistence().countByUuid_C(uuid, companyId);
}
/**
* Returns all the segments experiments where groupId = ?.
*
* @param groupId the group ID
* @return the matching segments experiments
*/
public static List findByGroupId(long groupId) {
return getPersistence().findByGroupId(groupId);
}
/**
* Returns a range of all the segments experiments where groupId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsExperimentModelImpl
.
*
*
* @param groupId the group ID
* @param start the lower bound of the range of segments experiments
* @param end the upper bound of the range of segments experiments (not inclusive)
* @return the range of matching segments experiments
*/
public static List findByGroupId(
long groupId, int start, int end) {
return getPersistence().findByGroupId(groupId, start, end);
}
/**
* Returns an ordered range of all the segments experiments where groupId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsExperimentModelImpl
.
*
*
* @param groupId the group ID
* @param start the lower bound of the range of segments experiments
* @param end the upper bound of the range of segments experiments (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching segments experiments
*/
public static List findByGroupId(
long groupId, int start, int end,
OrderByComparator orderByComparator) {
return getPersistence().findByGroupId(
groupId, start, end, orderByComparator);
}
/**
* Returns an ordered range of all the segments experiments where groupId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsExperimentModelImpl
.
*
*
* @param groupId the group ID
* @param start the lower bound of the range of segments experiments
* @param end the upper bound of the range of segments experiments (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching segments experiments
*/
public static List findByGroupId(
long groupId, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
return getPersistence().findByGroupId(
groupId, start, end, orderByComparator, useFinderCache);
}
/**
* Returns the first segments experiment in the ordered set where groupId = ?.
*
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching segments experiment
* @throws NoSuchExperimentException if a matching segments experiment could not be found
*/
public static SegmentsExperiment findByGroupId_First(
long groupId,
OrderByComparator orderByComparator)
throws com.liferay.segments.exception.NoSuchExperimentException {
return getPersistence().findByGroupId_First(groupId, orderByComparator);
}
/**
* Returns the first segments experiment in the ordered set where groupId = ?.
*
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching segments experiment, or null
if a matching segments experiment could not be found
*/
public static SegmentsExperiment fetchByGroupId_First(
long groupId, OrderByComparator orderByComparator) {
return getPersistence().fetchByGroupId_First(
groupId, orderByComparator);
}
/**
* Returns the last segments experiment in the ordered set where groupId = ?.
*
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching segments experiment
* @throws NoSuchExperimentException if a matching segments experiment could not be found
*/
public static SegmentsExperiment findByGroupId_Last(
long groupId,
OrderByComparator orderByComparator)
throws com.liferay.segments.exception.NoSuchExperimentException {
return getPersistence().findByGroupId_Last(groupId, orderByComparator);
}
/**
* Returns the last segments experiment in the ordered set where groupId = ?.
*
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching segments experiment, or null
if a matching segments experiment could not be found
*/
public static SegmentsExperiment fetchByGroupId_Last(
long groupId, OrderByComparator orderByComparator) {
return getPersistence().fetchByGroupId_Last(groupId, orderByComparator);
}
/**
* Returns the segments experiments before and after the current segments experiment in the ordered set where groupId = ?.
*
* @param segmentsExperimentId the primary key of the current segments experiment
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next segments experiment
* @throws NoSuchExperimentException if a segments experiment with the primary key could not be found
*/
public static SegmentsExperiment[] findByGroupId_PrevAndNext(
long segmentsExperimentId, long groupId,
OrderByComparator orderByComparator)
throws com.liferay.segments.exception.NoSuchExperimentException {
return getPersistence().findByGroupId_PrevAndNext(
segmentsExperimentId, groupId, orderByComparator);
}
/**
* Returns all the segments experiments that the user has permission to view where groupId = ?.
*
* @param groupId the group ID
* @return the matching segments experiments that the user has permission to view
*/
public static List filterFindByGroupId(long groupId) {
return getPersistence().filterFindByGroupId(groupId);
}
/**
* Returns a range of all the segments experiments that the user has permission to view where groupId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsExperimentModelImpl
.
*
*
* @param groupId the group ID
* @param start the lower bound of the range of segments experiments
* @param end the upper bound of the range of segments experiments (not inclusive)
* @return the range of matching segments experiments that the user has permission to view
*/
public static List filterFindByGroupId(
long groupId, int start, int end) {
return getPersistence().filterFindByGroupId(groupId, start, end);
}
/**
* Returns an ordered range of all the segments experiments that the user has permissions to view where groupId = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsExperimentModelImpl
.
*
*
* @param groupId the group ID
* @param start the lower bound of the range of segments experiments
* @param end the upper bound of the range of segments experiments (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching segments experiments that the user has permission to view
*/
public static List filterFindByGroupId(
long groupId, int start, int end,
OrderByComparator orderByComparator) {
return getPersistence().filterFindByGroupId(
groupId, start, end, orderByComparator);
}
/**
* Returns the segments experiments before and after the current segments experiment in the ordered set of segments experiments that the user has permission to view where groupId = ?.
*
* @param segmentsExperimentId the primary key of the current segments experiment
* @param groupId the group ID
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next segments experiment
* @throws NoSuchExperimentException if a segments experiment with the primary key could not be found
*/
public static SegmentsExperiment[] filterFindByGroupId_PrevAndNext(
long segmentsExperimentId, long groupId,
OrderByComparator orderByComparator)
throws com.liferay.segments.exception.NoSuchExperimentException {
return getPersistence().filterFindByGroupId_PrevAndNext(
segmentsExperimentId, groupId, orderByComparator);
}
/**
* Removes all the segments experiments where groupId = ? from the database.
*
* @param groupId the group ID
*/
public static void removeByGroupId(long groupId) {
getPersistence().removeByGroupId(groupId);
}
/**
* Returns the number of segments experiments where groupId = ?.
*
* @param groupId the group ID
* @return the number of matching segments experiments
*/
public static int countByGroupId(long groupId) {
return getPersistence().countByGroupId(groupId);
}
/**
* Returns the number of segments experiments that the user has permission to view where groupId = ?.
*
* @param groupId the group ID
* @return the number of matching segments experiments that the user has permission to view
*/
public static int filterCountByGroupId(long groupId) {
return getPersistence().filterCountByGroupId(groupId);
}
/**
* Returns all the segments experiments where segmentsExperimentKey = ?.
*
* @param segmentsExperimentKey the segments experiment key
* @return the matching segments experiments
*/
public static List findBySegmentsExperimentKey(
String segmentsExperimentKey) {
return getPersistence().findBySegmentsExperimentKey(
segmentsExperimentKey);
}
/**
* Returns a range of all the segments experiments where segmentsExperimentKey = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsExperimentModelImpl
.
*
*
* @param segmentsExperimentKey the segments experiment key
* @param start the lower bound of the range of segments experiments
* @param end the upper bound of the range of segments experiments (not inclusive)
* @return the range of matching segments experiments
*/
public static List findBySegmentsExperimentKey(
String segmentsExperimentKey, int start, int end) {
return getPersistence().findBySegmentsExperimentKey(
segmentsExperimentKey, start, end);
}
/**
* Returns an ordered range of all the segments experiments where segmentsExperimentKey = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsExperimentModelImpl
.
*
*
* @param segmentsExperimentKey the segments experiment key
* @param start the lower bound of the range of segments experiments
* @param end the upper bound of the range of segments experiments (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of matching segments experiments
*/
public static List findBySegmentsExperimentKey(
String segmentsExperimentKey, int start, int end,
OrderByComparator orderByComparator) {
return getPersistence().findBySegmentsExperimentKey(
segmentsExperimentKey, start, end, orderByComparator);
}
/**
* Returns an ordered range of all the segments experiments where segmentsExperimentKey = ?.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsExperimentModelImpl
.
*
*
* @param segmentsExperimentKey the segments experiment key
* @param start the lower bound of the range of segments experiments
* @param end the upper bound of the range of segments experiments (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of matching segments experiments
*/
public static List findBySegmentsExperimentKey(
String segmentsExperimentKey, int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
return getPersistence().findBySegmentsExperimentKey(
segmentsExperimentKey, start, end, orderByComparator,
useFinderCache);
}
/**
* Returns the first segments experiment in the ordered set where segmentsExperimentKey = ?.
*
* @param segmentsExperimentKey the segments experiment key
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching segments experiment
* @throws NoSuchExperimentException if a matching segments experiment could not be found
*/
public static SegmentsExperiment findBySegmentsExperimentKey_First(
String segmentsExperimentKey,
OrderByComparator orderByComparator)
throws com.liferay.segments.exception.NoSuchExperimentException {
return getPersistence().findBySegmentsExperimentKey_First(
segmentsExperimentKey, orderByComparator);
}
/**
* Returns the first segments experiment in the ordered set where segmentsExperimentKey = ?.
*
* @param segmentsExperimentKey the segments experiment key
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the first matching segments experiment, or null
if a matching segments experiment could not be found
*/
public static SegmentsExperiment fetchBySegmentsExperimentKey_First(
String segmentsExperimentKey,
OrderByComparator orderByComparator) {
return getPersistence().fetchBySegmentsExperimentKey_First(
segmentsExperimentKey, orderByComparator);
}
/**
* Returns the last segments experiment in the ordered set where segmentsExperimentKey = ?.
*
* @param segmentsExperimentKey the segments experiment key
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching segments experiment
* @throws NoSuchExperimentException if a matching segments experiment could not be found
*/
public static SegmentsExperiment findBySegmentsExperimentKey_Last(
String segmentsExperimentKey,
OrderByComparator orderByComparator)
throws com.liferay.segments.exception.NoSuchExperimentException {
return getPersistence().findBySegmentsExperimentKey_Last(
segmentsExperimentKey, orderByComparator);
}
/**
* Returns the last segments experiment in the ordered set where segmentsExperimentKey = ?.
*
* @param segmentsExperimentKey the segments experiment key
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the last matching segments experiment, or null
if a matching segments experiment could not be found
*/
public static SegmentsExperiment fetchBySegmentsExperimentKey_Last(
String segmentsExperimentKey,
OrderByComparator orderByComparator) {
return getPersistence().fetchBySegmentsExperimentKey_Last(
segmentsExperimentKey, orderByComparator);
}
/**
* Returns the segments experiments before and after the current segments experiment in the ordered set where segmentsExperimentKey = ?.
*
* @param segmentsExperimentId the primary key of the current segments experiment
* @param segmentsExperimentKey the segments experiment key
* @param orderByComparator the comparator to order the set by (optionally null
)
* @return the previous, current, and next segments experiment
* @throws NoSuchExperimentException if a segments experiment with the primary key could not be found
*/
public static SegmentsExperiment[] findBySegmentsExperimentKey_PrevAndNext(
long segmentsExperimentId, String segmentsExperimentKey,
OrderByComparator orderByComparator)
throws com.liferay.segments.exception.NoSuchExperimentException {
return getPersistence().findBySegmentsExperimentKey_PrevAndNext(
segmentsExperimentId, segmentsExperimentKey, orderByComparator);
}
/**
* Removes all the segments experiments where segmentsExperimentKey = ? from the database.
*
* @param segmentsExperimentKey the segments experiment key
*/
public static void removeBySegmentsExperimentKey(
String segmentsExperimentKey) {
getPersistence().removeBySegmentsExperimentKey(segmentsExperimentKey);
}
/**
* Returns the number of segments experiments where segmentsExperimentKey = ?.
*
* @param segmentsExperimentKey the segments experiment key
* @return the number of matching segments experiments
*/
public static int countBySegmentsExperimentKey(
String segmentsExperimentKey) {
return getPersistence().countBySegmentsExperimentKey(
segmentsExperimentKey);
}
/**
* Returns the segments experiment where groupId = ? and segmentsExperimentKey = ? or throws a NoSuchExperimentException
if it could not be found.
*
* @param groupId the group ID
* @param segmentsExperimentKey the segments experiment key
* @return the matching segments experiment
* @throws NoSuchExperimentException if a matching segments experiment could not be found
*/
public static SegmentsExperiment findByG_S(
long groupId, String segmentsExperimentKey)
throws com.liferay.segments.exception.NoSuchExperimentException {
return getPersistence().findByG_S(groupId, segmentsExperimentKey);
}
/**
* Returns the segments experiment where groupId = ? and segmentsExperimentKey = ? or returns null
if it could not be found. Uses the finder cache.
*
* @param groupId the group ID
* @param segmentsExperimentKey the segments experiment key
* @return the matching segments experiment, or null
if a matching segments experiment could not be found
*/
public static SegmentsExperiment fetchByG_S(
long groupId, String segmentsExperimentKey) {
return getPersistence().fetchByG_S(groupId, segmentsExperimentKey);
}
/**
* Returns the segments experiment where groupId = ? and segmentsExperimentKey = ? or returns null
if it could not be found, optionally using the finder cache.
*
* @param groupId the group ID
* @param segmentsExperimentKey the segments experiment key
* @param useFinderCache whether to use the finder cache
* @return the matching segments experiment, or null
if a matching segments experiment could not be found
*/
public static SegmentsExperiment fetchByG_S(
long groupId, String segmentsExperimentKey, boolean useFinderCache) {
return getPersistence().fetchByG_S(
groupId, segmentsExperimentKey, useFinderCache);
}
/**
* Removes the segments experiment where groupId = ? and segmentsExperimentKey = ? from the database.
*
* @param groupId the group ID
* @param segmentsExperimentKey the segments experiment key
* @return the segments experiment that was removed
*/
public static SegmentsExperiment removeByG_S(
long groupId, String segmentsExperimentKey)
throws com.liferay.segments.exception.NoSuchExperimentException {
return getPersistence().removeByG_S(groupId, segmentsExperimentKey);
}
/**
* Returns the number of segments experiments where groupId = ? and segmentsExperimentKey = ?.
*
* @param groupId the group ID
* @param segmentsExperimentKey the segments experiment key
* @return the number of matching segments experiments
*/
public static int countByG_S(long groupId, String segmentsExperimentKey) {
return getPersistence().countByG_S(groupId, segmentsExperimentKey);
}
/**
* Returns the segments experiment where groupId = ? and segmentsExperienceId = ? and plid = ? or throws a NoSuchExperimentException
if it could not be found.
*
* @param groupId the group ID
* @param segmentsExperienceId the segments experience ID
* @param plid the plid
* @return the matching segments experiment
* @throws NoSuchExperimentException if a matching segments experiment could not be found
*/
public static SegmentsExperiment findByG_S_P(
long groupId, long segmentsExperienceId, long plid)
throws com.liferay.segments.exception.NoSuchExperimentException {
return getPersistence().findByG_S_P(
groupId, segmentsExperienceId, plid);
}
/**
* Returns the segments experiment where groupId = ? and segmentsExperienceId = ? and plid = ? or returns null
if it could not be found. Uses the finder cache.
*
* @param groupId the group ID
* @param segmentsExperienceId the segments experience ID
* @param plid the plid
* @return the matching segments experiment, or null
if a matching segments experiment could not be found
*/
public static SegmentsExperiment fetchByG_S_P(
long groupId, long segmentsExperienceId, long plid) {
return getPersistence().fetchByG_S_P(
groupId, segmentsExperienceId, plid);
}
/**
* Returns the segments experiment where groupId = ? and segmentsExperienceId = ? and plid = ? or returns null
if it could not be found, optionally using the finder cache.
*
* @param groupId the group ID
* @param segmentsExperienceId the segments experience ID
* @param plid the plid
* @param useFinderCache whether to use the finder cache
* @return the matching segments experiment, or null
if a matching segments experiment could not be found
*/
public static SegmentsExperiment fetchByG_S_P(
long groupId, long segmentsExperienceId, long plid,
boolean useFinderCache) {
return getPersistence().fetchByG_S_P(
groupId, segmentsExperienceId, plid, useFinderCache);
}
/**
* Removes the segments experiment where groupId = ? and segmentsExperienceId = ? and plid = ? from the database.
*
* @param groupId the group ID
* @param segmentsExperienceId the segments experience ID
* @param plid the plid
* @return the segments experiment that was removed
*/
public static SegmentsExperiment removeByG_S_P(
long groupId, long segmentsExperienceId, long plid)
throws com.liferay.segments.exception.NoSuchExperimentException {
return getPersistence().removeByG_S_P(
groupId, segmentsExperienceId, plid);
}
/**
* Returns the number of segments experiments where groupId = ? and segmentsExperienceId = ? and plid = ?.
*
* @param groupId the group ID
* @param segmentsExperienceId the segments experience ID
* @param plid the plid
* @return the number of matching segments experiments
*/
public static int countByG_S_P(
long groupId, long segmentsExperienceId, long plid) {
return getPersistence().countByG_S_P(
groupId, segmentsExperienceId, plid);
}
/**
* Caches the segments experiment in the entity cache if it is enabled.
*
* @param segmentsExperiment the segments experiment
*/
public static void cacheResult(SegmentsExperiment segmentsExperiment) {
getPersistence().cacheResult(segmentsExperiment);
}
/**
* Caches the segments experiments in the entity cache if it is enabled.
*
* @param segmentsExperiments the segments experiments
*/
public static void cacheResult(
List segmentsExperiments) {
getPersistence().cacheResult(segmentsExperiments);
}
/**
* Creates a new segments experiment with the primary key. Does not add the segments experiment to the database.
*
* @param segmentsExperimentId the primary key for the new segments experiment
* @return the new segments experiment
*/
public static SegmentsExperiment create(long segmentsExperimentId) {
return getPersistence().create(segmentsExperimentId);
}
/**
* Removes the segments experiment with the primary key from the database. Also notifies the appropriate model listeners.
*
* @param segmentsExperimentId the primary key of the segments experiment
* @return the segments experiment that was removed
* @throws NoSuchExperimentException if a segments experiment with the primary key could not be found
*/
public static SegmentsExperiment remove(long segmentsExperimentId)
throws com.liferay.segments.exception.NoSuchExperimentException {
return getPersistence().remove(segmentsExperimentId);
}
public static SegmentsExperiment updateImpl(
SegmentsExperiment segmentsExperiment) {
return getPersistence().updateImpl(segmentsExperiment);
}
/**
* Returns the segments experiment with the primary key or throws a NoSuchExperimentException
if it could not be found.
*
* @param segmentsExperimentId the primary key of the segments experiment
* @return the segments experiment
* @throws NoSuchExperimentException if a segments experiment with the primary key could not be found
*/
public static SegmentsExperiment findByPrimaryKey(long segmentsExperimentId)
throws com.liferay.segments.exception.NoSuchExperimentException {
return getPersistence().findByPrimaryKey(segmentsExperimentId);
}
/**
* Returns the segments experiment with the primary key or returns null
if it could not be found.
*
* @param segmentsExperimentId the primary key of the segments experiment
* @return the segments experiment, or null
if a segments experiment with the primary key could not be found
*/
public static SegmentsExperiment fetchByPrimaryKey(
long segmentsExperimentId) {
return getPersistence().fetchByPrimaryKey(segmentsExperimentId);
}
/**
* Returns all the segments experiments.
*
* @return the segments experiments
*/
public static List findAll() {
return getPersistence().findAll();
}
/**
* Returns a range of all the segments experiments.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsExperimentModelImpl
.
*
*
* @param start the lower bound of the range of segments experiments
* @param end the upper bound of the range of segments experiments (not inclusive)
* @return the range of segments experiments
*/
public static List findAll(int start, int end) {
return getPersistence().findAll(start, end);
}
/**
* Returns an ordered range of all the segments experiments.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsExperimentModelImpl
.
*
*
* @param start the lower bound of the range of segments experiments
* @param end the upper bound of the range of segments experiments (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @return the ordered range of segments experiments
*/
public static List findAll(
int start, int end,
OrderByComparator orderByComparator) {
return getPersistence().findAll(start, end, orderByComparator);
}
/**
* Returns an ordered range of all the segments experiments.
*
*
* Useful when paginating results. Returns a maximum of end - start
instances. start
and end
are not primary keys, they are indexes in the result set. Thus, 0
refers to the first result in the set. Setting both start
and end
to QueryUtil#ALL_POS
will return the full result set. If orderByComparator
is specified, then the query will include the given ORDER BY logic. If orderByComparator
is absent, then the query will include the default ORDER BY logic from SegmentsExperimentModelImpl
.
*
*
* @param start the lower bound of the range of segments experiments
* @param end the upper bound of the range of segments experiments (not inclusive)
* @param orderByComparator the comparator to order the results by (optionally null
)
* @param useFinderCache whether to use the finder cache
* @return the ordered range of segments experiments
*/
public static List findAll(
int start, int end,
OrderByComparator orderByComparator,
boolean useFinderCache) {
return getPersistence().findAll(
start, end, orderByComparator, useFinderCache);
}
/**
* Removes all the segments experiments from the database.
*/
public static void removeAll() {
getPersistence().removeAll();
}
/**
* Returns the number of segments experiments.
*
* @return the number of segments experiments
*/
public static int countAll() {
return getPersistence().countAll();
}
public static SegmentsExperimentPersistence getPersistence() {
return _persistence;
}
public static void setPersistence(
SegmentsExperimentPersistence persistence) {
_persistence = persistence;
}
private static volatile SegmentsExperimentPersistence _persistence;
}