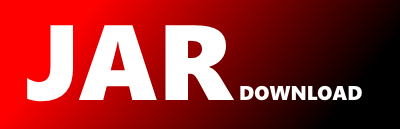
org.apache.neethi.builders.PrimitiveAssertion Maven / Gradle / Ivy
/**
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.apache.neethi.builders;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
import javax.xml.XMLConstants;
import javax.xml.namespace.QName;
import javax.xml.stream.XMLStreamException;
import javax.xml.stream.XMLStreamWriter;
import org.apache.neethi.All;
import org.apache.neethi.Assertion;
import org.apache.neethi.Constants;
import org.apache.neethi.ExactlyOne;
import org.apache.neethi.Policy;
import org.apache.neethi.PolicyComponent;
/**
*
*/
public class PrimitiveAssertion implements Assertion {
protected QName name;
protected boolean optional;
protected boolean ignorable;
protected String textValue;
protected Map attributes;
public PrimitiveAssertion() {
this((QName)null);
}
public PrimitiveAssertion(QName n) {
this(n, false);
}
public PrimitiveAssertion(QName n, boolean o) {
this(n, o, false);
}
public PrimitiveAssertion(QName n, boolean o, boolean i) {
this(n, o, i, null, null);
}
public PrimitiveAssertion(QName n, boolean o, boolean i,
Map atts) {
this(n, o, i, atts, null);
}
public PrimitiveAssertion(QName n, boolean o, boolean i,
Map atts,
String value) {
name = n;
optional = o;
ignorable = i;
if (atts != null) {
attributes = new HashMap(atts);
}
textValue = value;
}
public String getAttribute(QName n) {
if (attributes != null) {
return attributes.get(n);
}
return null;
}
public Map getAttributes() {
if (attributes != null) {
return new HashMap(attributes);
} else {
return Collections.emptyMap();
}
}
public synchronized void addAttribute(QName n, String value) {
if (attributes == null) {
attributes = new HashMap();
}
attributes.put(n, value);
}
public synchronized void addAttributes(Map atts) {
if (attributes == null) {
attributes = new HashMap(atts);
} else {
attributes.putAll(atts);
}
}
public String getTextValue() {
return textValue;
}
public void setTextValue(String s) {
textValue = s;
}
public String toString() {
return name.toString();
}
public boolean equal(PolicyComponent policyComponent) {
if (this == policyComponent) {
return true;
}
if (policyComponent.getType() != Constants.TYPE_ASSERTION) {
return false;
}
return getName().equals(((Assertion)policyComponent).getName());
}
@Override
public boolean equals(Object obj) {
if (!(obj instanceof PrimitiveAssertion)) {
return false;
}
PrimitiveAssertion pa = (PrimitiveAssertion) obj;
if ((pa.getName() != null) && (!pa.getName().equals(this.getName()))) {
return false;
}
if ((this.getName() != null) && (!this.getName().equals(pa.getName()))) {
return false;
}
if ((pa.isOptional() ^ this.isOptional()) || (pa.isIgnorable() ^ this.isIgnorable())) {
return false;
}
if ( (pa.getTextValue() != null) && (!pa.getTextValue().equals(this.getTextValue()))) {
return false;
}
if ( (this.getTextValue() != null) && (!this.getTextValue().equals(pa.getTextValue()))) {
return false;
}
Map attrs1 = getAttributes();
Map attrs2 = pa.getAttributes();
for (QName key : attrs1.keySet()) {
if ((!attrs2.containsKey(key)) || (!attrs1.get(key).equals(attrs2.get(key)))) {
return false;
} else {
attrs2.remove(key);
}
}
if (!attrs2.isEmpty()) {
return false;
}
return true;
}
@Override
public int hashCode() {
int hash = 17;
hash = 31 * hash + (name != null ? name.hashCode() : 0);
hash = 31 * hash + ((optional) ? 1 : 0);
hash = 31 * hash + ((ignorable) ? 1 : 0);
hash = 31 * hash + (textValue != null ? textValue.hashCode() : 0);
return hash;
}
public short getType() {
return Constants.TYPE_ASSERTION;
}
public QName getName() {
return name;
}
public void setName(QName n) {
name = n;
}
public boolean isOptional() {
return optional;
}
public void setOptional(boolean o) {
optional = o;
}
public boolean isIgnorable() {
return ignorable;
}
public void setIgnorable(boolean i) {
ignorable = i;
}
public PolicyComponent normalize() {
if (isOptional()) {
Policy policy = new Policy();
ExactlyOne exactlyOne = new ExactlyOne();
All all = new All();
all.addPolicyComponent(clone(false));
exactlyOne.addPolicyComponent(all);
exactlyOne.addPolicyComponent(new All());
policy.addPolicyComponent(exactlyOne);
return policy;
}
return clone(false);
}
public void serialize(XMLStreamWriter writer) throws XMLStreamException {
String namespace = Constants.findPolicyNamespace(writer);
String pfx = writer.getPrefix(name.getNamespaceURI());
boolean writeNS = false;
if (pfx == null && name.getPrefix() != null && !"".equals(name.getPrefix())) {
pfx = name.getPrefix();
writeNS = true;
}
if ("".equals(pfx) || pfx == null) {
pfx = "";
writeNS = true;
}
if (!hasContents() && attributes == null && !writeNS) {
writer.writeEmptyElement(pfx, name.getLocalPart(), name.getNamespaceURI());
return;
}
writer.writeStartElement(pfx, name.getLocalPart(), name.getNamespaceURI());
if (writeNS) {
if ("".equals(pfx) || pfx == null) {
writer.writeDefaultNamespace(name.getNamespaceURI());
} else if (attributes == null
|| !attributes.containsKey(new QName(XMLConstants.XMLNS_ATTRIBUTE_NS_URI, pfx))) {
writer.writeNamespace(pfx, name.getNamespaceURI());
}
}
if (optional) {
writer.writeAttribute(namespace, Constants.ATTR_OPTIONAL, "true");
}
if (ignorable) {
writer.writeAttribute(namespace, Constants.ATTR_IGNORABLE, "true");
}
writeAttributes(writer);
writeContents(writer);
writer.writeEndElement();
}
protected void writeContents(XMLStreamWriter writer) throws XMLStreamException {
if (textValue != null) {
writer.writeCharacters(textValue);
}
}
protected boolean hasContents() {
return textValue != null && !"".equals(textValue);
}
protected void writeAttributes(XMLStreamWriter writer) throws XMLStreamException {
if (attributes != null) {
for (Map.Entry att : attributes.entrySet()) {
if (Constants.isIgnorableAttribute(att.getKey())) {
//already handled
continue;
}
if (Constants.isOptionalAttribute(att.getKey())) {
//already handled
continue;
}
if (XMLConstants.XMLNS_ATTRIBUTE_NS_URI.equals(att.getKey().getNamespaceURI())) {
writer.writeNamespace(att.getKey().getLocalPart(), att.getValue());
continue;
}
String ns = att.getKey().getNamespaceURI();
if (ns != null && !"".equals(ns)) {
String prefix = getOrCreatePrefix(att.getKey().getNamespaceURI(), writer);
writer.writeAttribute(prefix, att.getKey().getNamespaceURI(),
att.getKey().getLocalPart(),
att.getValue());
} else {
writer.writeAttribute(att.getKey().getLocalPart(), att.getValue());
}
}
}
}
protected String getOrCreatePrefix(String ns, XMLStreamWriter writer) throws XMLStreamException {
if (XMLConstants.XMLNS_ATTRIBUTE_NS_URI.equals(ns)) {
return null;
}
String prefix = writer.getPrefix(ns);
int count = 1;
while (prefix == null || "".equals(prefix)) {
prefix = "ns" + count++;
String ns2 = writer.getNamespaceContext().getNamespaceURI(prefix);
if (ns2 == null || "".equals(ns2)) {
//found one that will work
writer.writeNamespace(prefix, ns);
} else {
prefix = null;
}
}
return prefix;
}
protected Assertion clone(boolean isoptional) {
return new PrimitiveAssertion(name, isoptional, ignorable, attributes, textValue);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy