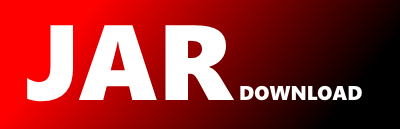
org.apache.woden.wsdl20.xml.BindingOperationElement Maven / Gradle / Ivy
/**
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.woden.wsdl20.xml;
import javax.xml.namespace.QName;
/**
* Represents the WSDL 2.0 <operation> element, declared as a child
* of the <binding> element.
*
* @author John Kaputin ([email protected])
*/
public interface BindingOperationElement extends DocumentableElement,
NestedElement
{
/*
* Attributes
*/
/**
* Specify the name of the InterfaceOperationElement referred to by this BindingOperationElement.
* The specified QName corresponds to the ref
attribute of the binding
* <operation> element.
*
* @param operName the QName of the interface operation.
*/
public void setRef(QName operName);
/**
* Return the name of the InterfaceOperationElement referred to by this BindingOperationElement.
* This corresponds to the ref
attribute of the binding <operation> element.
*
* @return the QName of the interface operation
*/
public QName getRef();
/**
* Return the InterfaceOperationElement referred to by this BindingOperationElement.
* This equates to the interface <operation> element referred to by the
* ref
attribute of the binding <operation> element.
* If this reference cannot be resolved to an InterfaceOperationElement, this method will
* return null.
*
* @return the InterfaceOperationElement
*/
public InterfaceOperationElement getInterfaceOperationElement();
/*
* Elements
*/
/**
* Create a BindingMessageReferenceElement with this BindingOperationElement as its parent
* and return a reference to it.
* This equates to adding an <input> or <output> element
* to the binding <operation> element.
*
* @return the BindingMessageReferenceElement
*/
public BindingMessageReferenceElement addBindingMessageReferenceElement();
/**
* Remove the specified BindingMessageReferenceElement from the set of
* BindingMessageReferenceElements within this BindingOperationElement.
* This equates to removing an <input> or <output> element
* from the binding <operation> element.
* If the specified BindingMessageReferenceElement does not exist or if a
* null value is specified, no action is performed.
*
* @param msgRef the BindingMessageReferenceElement to be removed
*/
public void removeBindingMessageReferenceElement(BindingMessageReferenceElement msgRef);
/**
* Return the set of BindingMessageReferenceElements within this BindingOperationElement.
* This equates to the set of <input> and <output> elements
* within the binding <operation> element.
* If no BindingMessageReferenceElements exist, an empty array is returned.
*
* @return an array of BindingMessageReferenceElement
*/
public BindingMessageReferenceElement[] getBindingMessageReferenceElements();
/**
* Create a BindingFaultReferenceElement with this BindingOperationElement as its parent
* and return a reference to it.
* This equates to adding an <infault> or <outfault> element
* to the binding <operation> element.
*
* @return the BindingFaultReferenceElement
*/
public BindingFaultReferenceElement addBindingFaultReferenceElement();
/**
* Remove the specified BindingFaultReferenceElement from the set of
* BindingFaultReferenceElements within this BindingOperationElement.
* This equates to removing an <infault> or <outfault> element
* from the binding <operation> element.
* If the specified BindingFaultReferenceElement does not exist or if a
* null value is specified, no action is performed.
*
* @param faultRef the BindingFaultReferenceElement to be removed
*/
public void removeBindingFaultReferenceElement(BindingFaultReferenceElement faultRef);
/**
* Return the set of BindingFaultReferenceElements within this BindingOperationElement.
* This equates to the set of <infault> and <outfault> elements
* within the binding <operation> element.
* If no BindingFaultReferenceElements exist, an empty array is returned.
*
* @return an array of BindingFaultReferenceElement
*/
public BindingFaultReferenceElement[] getBindingFaultReferenceElements();
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy